In this article, I would like to give an overview of common linter & analysis tools for various areas, some of which we also use to improve and validate code. After the relaunch of TutKit.com in November 2021, we were busy with bugfixing and refactoring for about 1.5 years and somehow it seems like it just won't stop. TutKit.com is a custom development based on the PHP framework Laravel and the JavaScript framework vue.js. Anyone who runs a professional portal or software will sooner or later have no choice but to use such linter and analysis tools. These code checkers and validators are even suitable for smaller websites or special requirements, as they offer a quick way to point out errors and problems.
Table of contents
What are linter messages?
Linter messages refer to error messages, warnings or hints that are generated by a linter tool. A linter is an analysis program or analysis software that analyzes source code to detect potential errors, style issues, incorrect syntax, potential security vulnerabilities or other problems in the code. It is often used in app, website and software development to ensure that code meets certain standards and is well written.
Linter messages can be output in various formats, e.g. as text, XML or JSON. They can also appear in different levels of severity, e.g. as errors, warnings or notes.
Here are some common types of linter messages:
Error messages: These messages indicate serious problems in the code that may cause the program to malfunction or crash. These errors are so serious that they can invalidate the code or lead to unexpected behavior. Errors must be corrected before the code can be compiled or executed. Examples of errors are syntax errors, undefined variables or invalid functions.
A Linter error message could look like this:
Error: Variable 'undefined' is not defined
This error message indicates that a variable is being used that has not yet been defined.
Warnings: Warnings indicate potential problems that may not directly lead to errors, but can still cause unwanted behavior or inefficient code. For example, warnings could indicate unused variables or unused imports. Warnings can be ignored, but should usually be fixed to improve the quality of the code.
A linter warning could look like this:
Warning: Use of deprecated function 'foo()'
This warning indicates that a function is being used that is deprecated and could be removed in future versions of the programming language.
Notes or recommendations: This type of message indicates improvements or best practices that can make the code more readable, maintainable or efficient. They are less critical than errors or warnings and often serve to raise the standard of code quality.
A linter note could look like this:
Note: Code could be more efficient
This note indicates that the code could be improved, e.g. by using more efficient algorithms.
Why should developers use linter tools?
Developers should use linter tools for a number of reasons, as they offer a range of benefits that can help improve code quality, increase productivity and reduce errors. Here are some of the main reasons why developers use linter tools & code checkers:
- Error detection: linter tools can identify potential errors in code before they lead to runtime errors. This allows developers to fix bugs early, saving time and effort in troubleshooting.
- Compliance with coding standards: Linter tools can ensure that code conforms to established coding standards and style guidelines. This makes code maintenance and team collaboration easier as the code becomes more consistent.
- Readability and maintainability: Linter tools can pay attention to the readability and maintainability of the code. This results in more understandable and maintainable code that is more accessible to both the developer and other team members.
- Best practices: Most linter tools can review best practices and design patterns and ensure that they are used in the code. This contributes to the development of high-quality code.
- Efficiency: By identifying inefficient sections of code, linter tools can help improve the performance of the code by uncovering inefficient algorithms or wasted resources.
- Automation: Linter tools automate the process of code review, saving developers time and effort. They can be integrated into development workflows and run continuously to check the code in the background.
- Prevention of security vulnerabilities: Some Linter tools can detect potential security vulnerabilities in the code, which helps to minimize security risks.
- Team consistency: Linter tools promote adherence to common conventions and standards within the team, which makes the code base more homogeneous and reduces discussions about coding styles.
- Documentation and training: Linter tools can provide developers with hints and advice on how to improve their code, which helps to increase knowledge and skills within the team.
Overall, linter tools can help to increase the quality and reliability of software and web projects and make development more efficient and less error-prone. They are an important part of the modern development process and are used in a variety of programming languages and development platforms.
Linter & analysis tools for HTML
There are several linter tools and code review tools for HTML to identify errors and style issues in HTML code and improve code quality. These linter tools can help developers check HTML code for various types of errors, such as:
- Missing or incorrectly nested HTML tags.
- Invalid attributes or values.
- HTML tags that are not closed.
- Missing mandatory HTML elements.
- Style guidelines and best practices for HTML.
Here are two of the most popular linter tools for HTML:
The Nu HTML Checker an online HTML validation service from W3C that checks HTML documents for conformance to HTML5 standards. It is free and available online. Here you simply enter your URL and receive the check result - also activate the options to see the anomalies directly in the code:
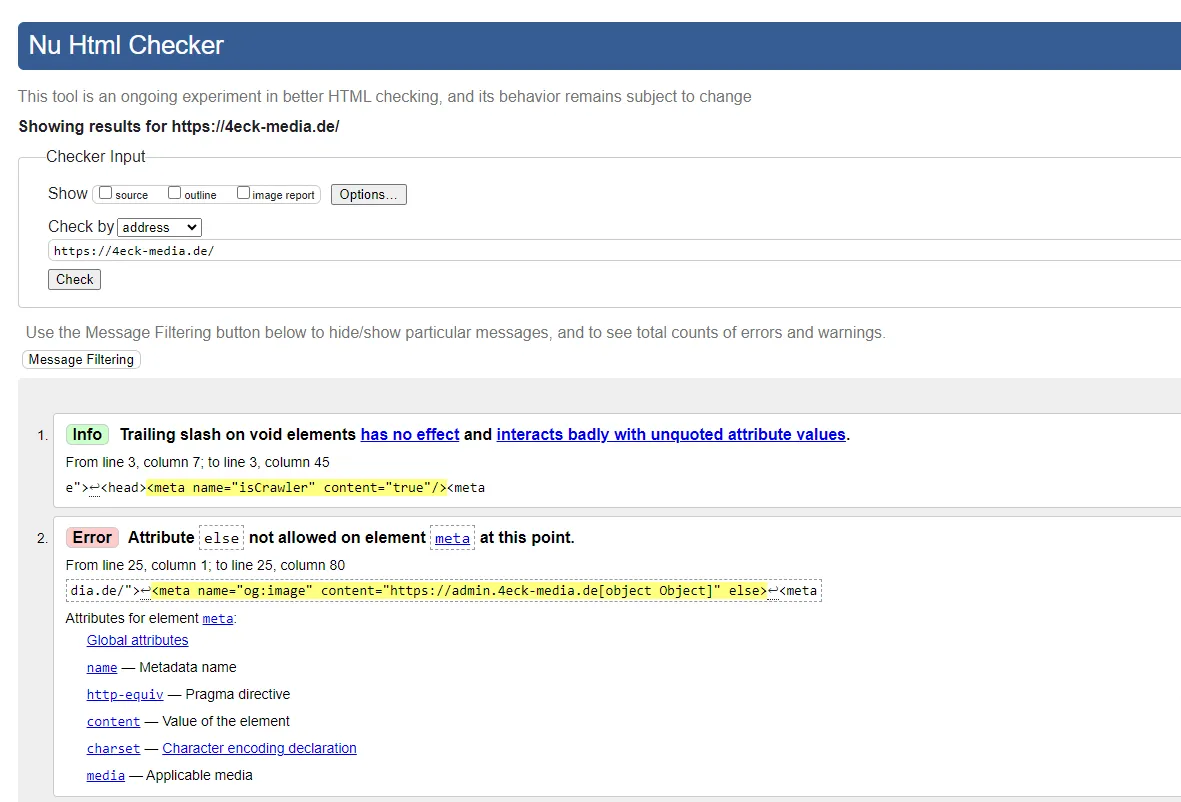
HTMLHint: HTMLHint is a widely used linter tool for HTML based on Node.js. It checks HTML files for errors and warns of best practices and coding standards. You can use it as an online tool, install it as a CLI (Command Line Interface) tool or activate it as an IDE extension for Visual Code Studio, Atom, Sublime Text or other editors. In the online tool, you enter your code and receive notes at the beginning of the line marked with a cross - on mouseover you can see what is not correct.
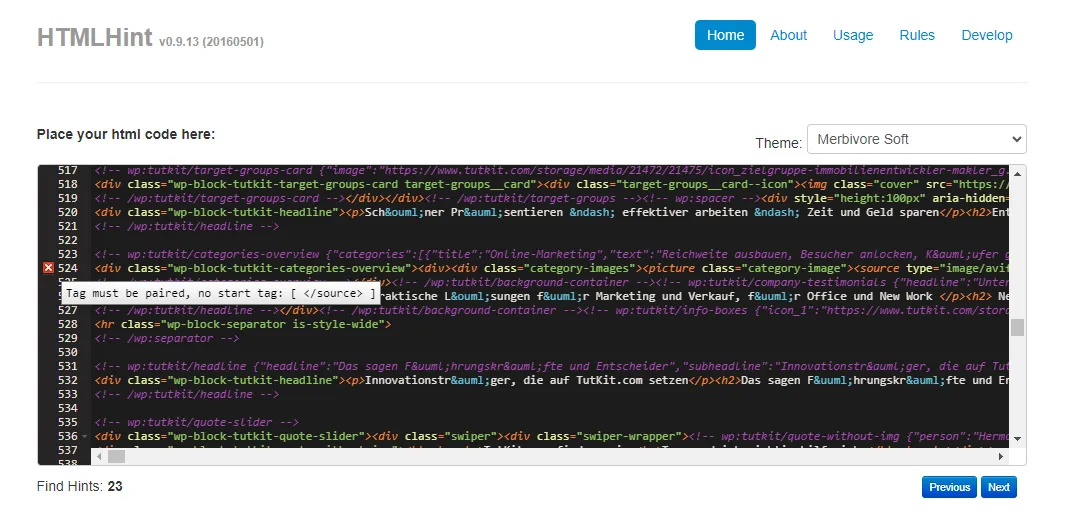
There are also HTML plugins for other linters that focus on JavaScript, for example. You can also find suitable extensions for most editors, see below. Using an HTML linter helps you to ensure that your HTML documents comply with common standards, are correctly validated and well written, which improves the readability and maintainability of your website.
Linter tools for CSS
Of course, what applies to HTML also applies to CSS (Cascading Style Sheets). The linter tools for CSS help developers to detect various types of errors and style problems, including
- Missing or incorrect CSS selectors.
- Invalid CSS properties or values.
- Unused CSS rules or selectors.
- Style guidelines and best practices for CSS and CSS preprocessors such as SCSS or LESS.
- Consistent formatting and indentation.
Here are two linter tools for CSS for you:
stylelint: stylelint is one of the most commonly used linter tools for CSS and SCSS. It checks CSS files for errors and style guidelines and allows for extensive customization of rules and configurations. stylelint ensures that CSS style guidelines and best practices are followed.
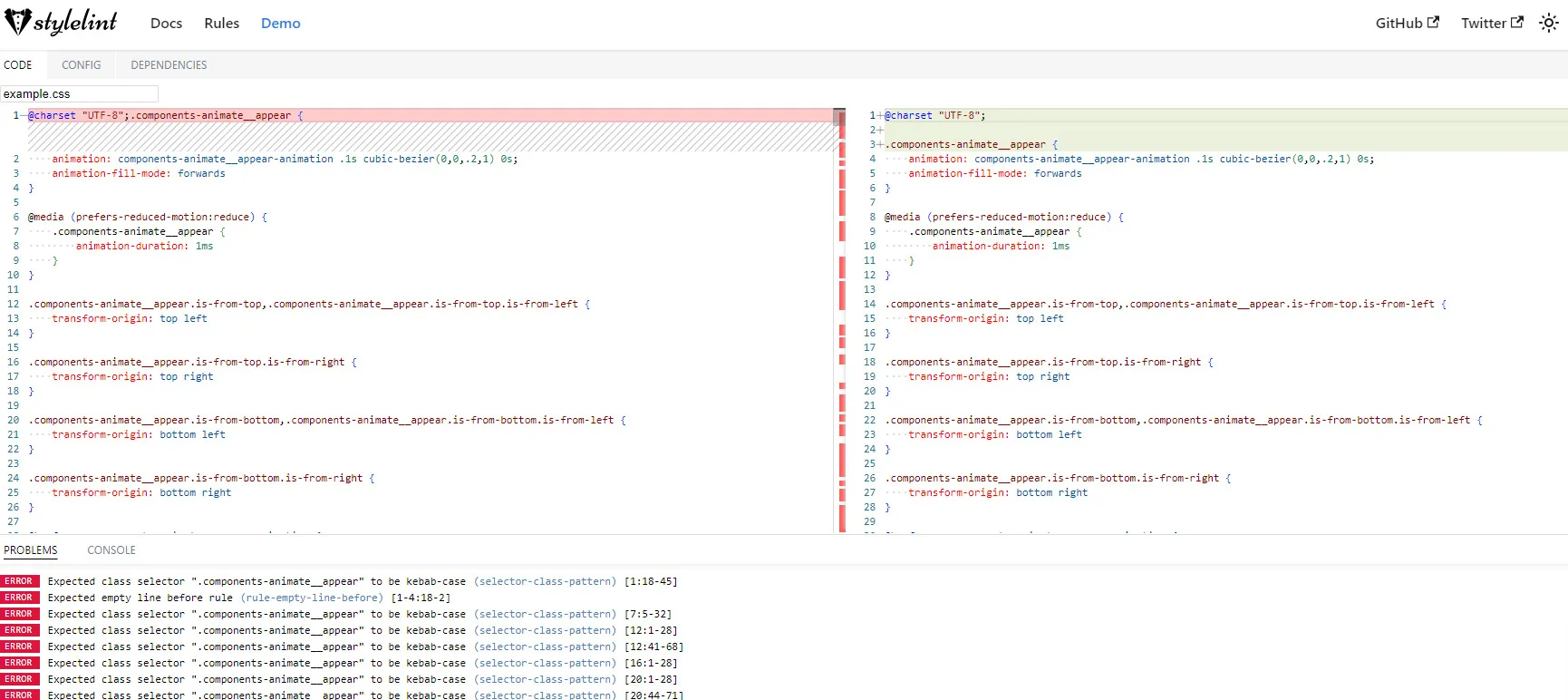
Prettier: Although Prettier is primarily a code formatter, it can also be used to check and format CSS code to ensure consistent formatting and indentation.
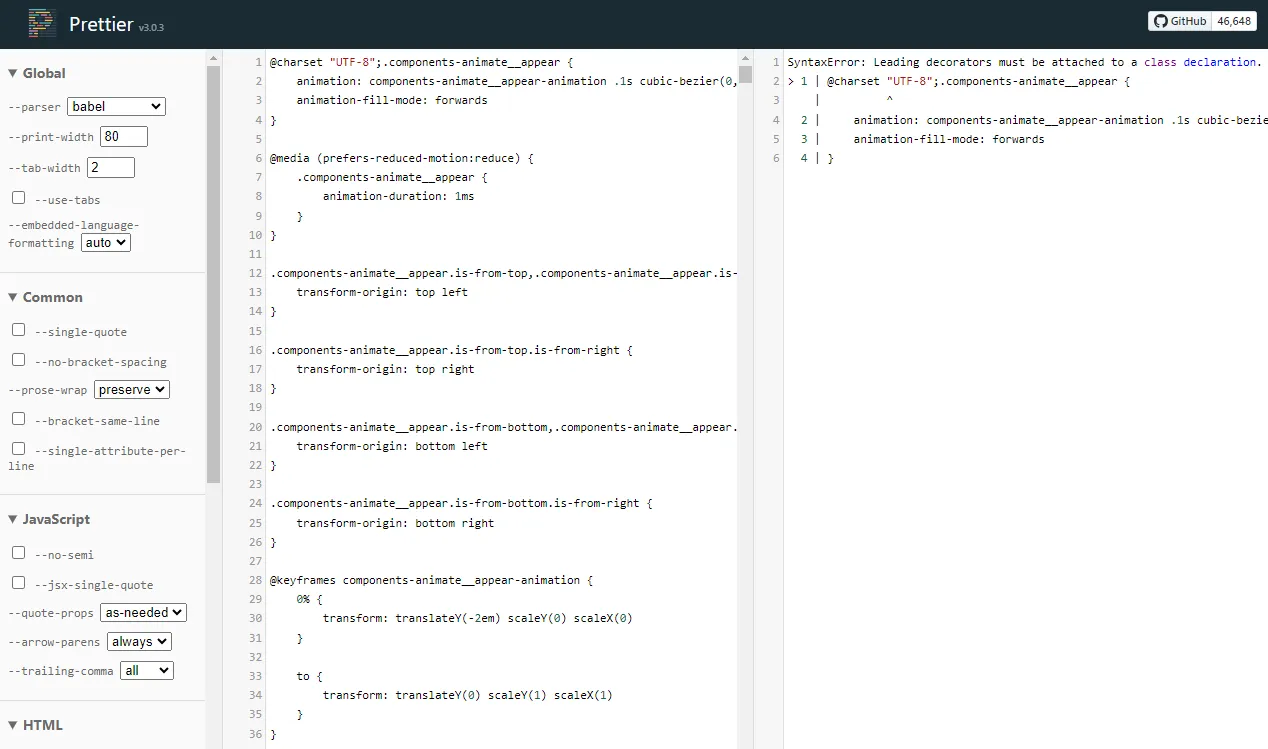
So using a CSS linter helps to ensure that CSS code is more readable, maintainable and error-free, resulting in better quality websites and web applications. It also facilitates team collaboration, as consistent and well-written CSS code is easier to understand and maintain.
Linter tools for JavaScript
There are linter tools for JavaScript, but also directly for JavaScript frameworks such as vue.js, which we use here at TutKit.com. They help you detect different types of errors and style issues, including
- Syntax errors and logical errors.
- Unused variables or functions.
- Invalid or undeclared variables and objects.
- Compliance with coding standards and style guidelines.
- Potentially error-prone constructs and best practices.
ESLint is one of the most widely used linter tools for JavaScript. It provides a comprehensive check of JavaScript code for errors and style guidelines. ESLint is highly configurable and allows you to set your own rules and conventions. You can also extend ESLint with plugins for HTML & CSS linting to check HTML data and CSS files for errors.
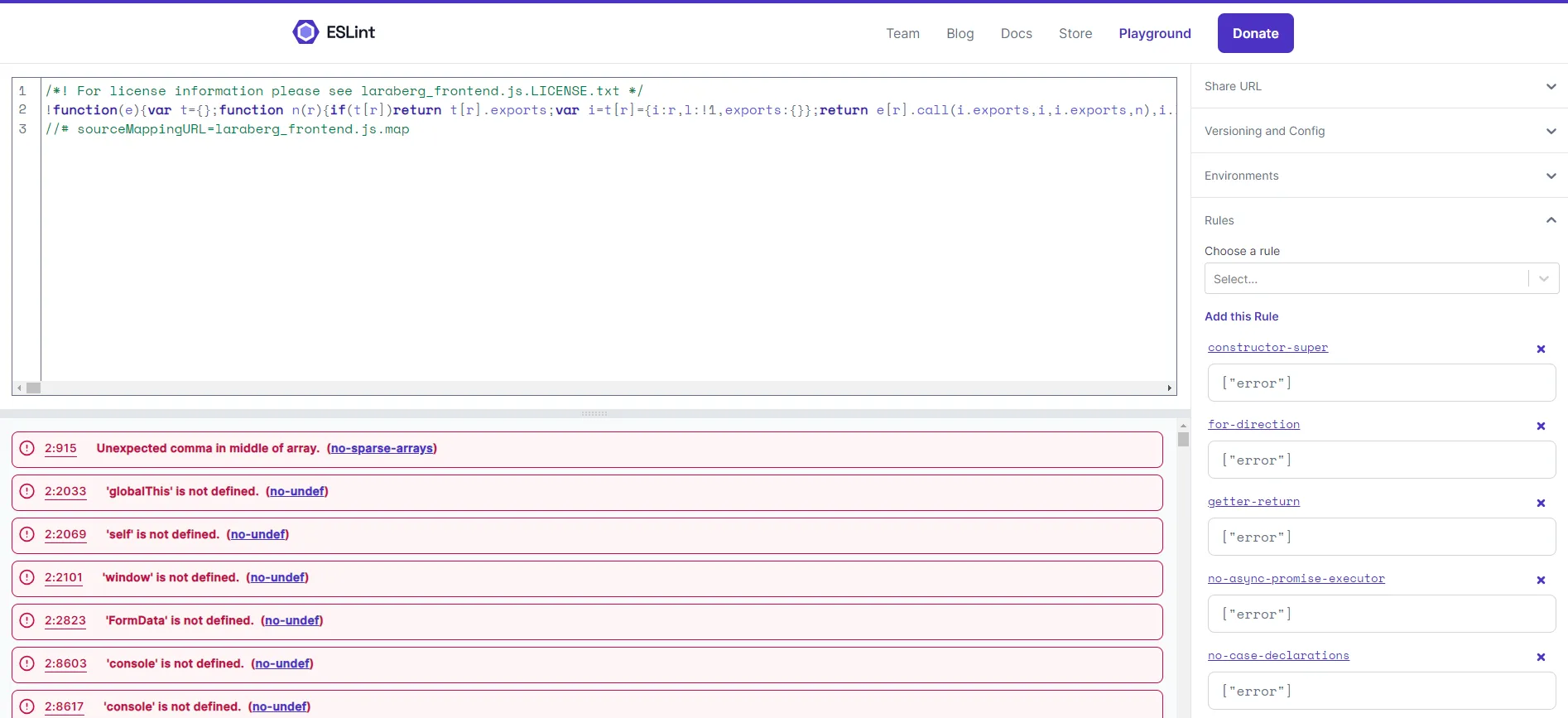
JSHint is a simpler JavaScript linter that checks code for errors and style guidelines. It is less configurable than ESLint, but still useful for basic linting requirements.
Flow is a typing solution for JavaScript that can also be used to detect errors in code caused by missing or incorrect typing.
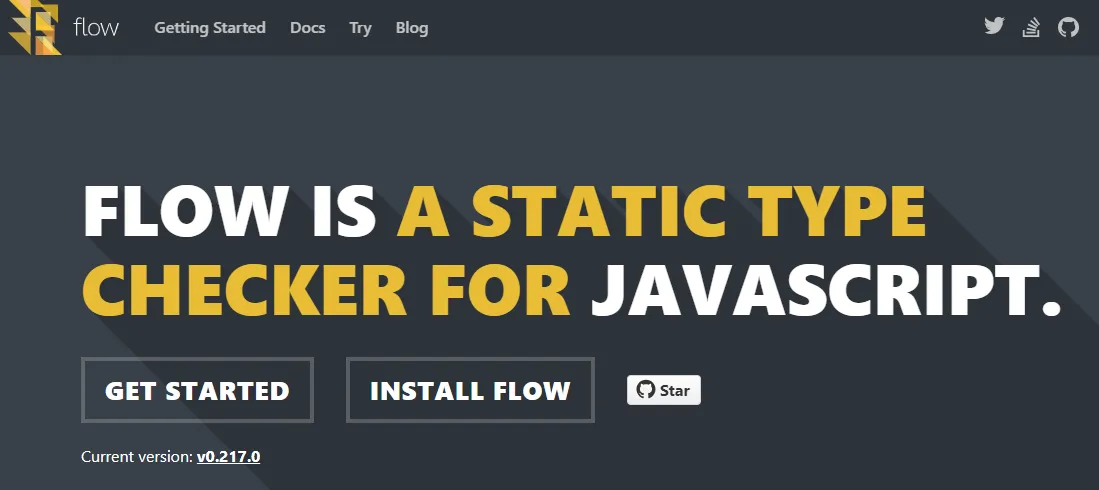
Similarly, StandardJS is a relatively simple linter tool for JavaScript that is based on the idea of promoting a uniform coding style for JavaScript. It enforces a certain set of rules and conventions without configuration.
Linter tools for PHP
There are several linter tools for PHP (Hypertext Preprocessor) that help backend developers identify errors, style issues and best practices in their PHP code and improve code quality, including:
- Syntax errors and logical errors.
- Compliance with coding standards and style guidelines.
- Typing errors and potential security vulnerabilities.
- Code metrics and best practices for code quality.
Check out one of these linter tools for PHP to improve your backend projects:
PHPStan is a static analysis linter for PHP that checks code for type hints, potential errors, and security issues. It offers high accuracy in error detection and can help improve code quality. You can also use it online. What's nice is that on the one hand it is friendly towards outdated legacy code. On the other hand, it is also very useful when using PHP frameworks such as Laravel, on which TutKit.com is also based, or Symfony.
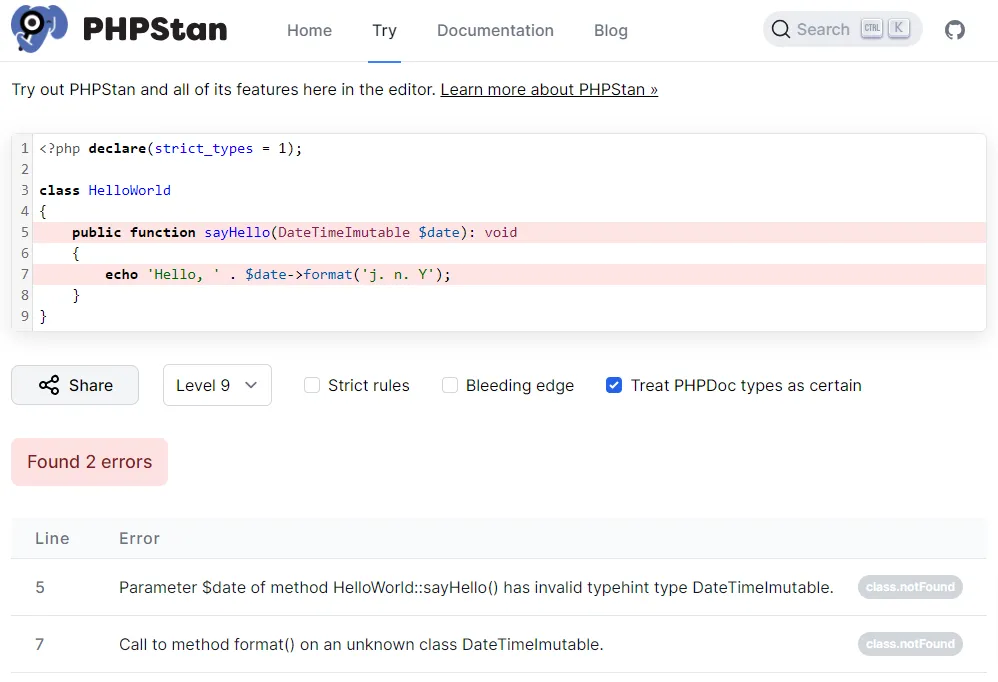
We also use the paid SymfonyInsight tool (for our Laravel project). Although it is not free, it works with gamification and scores etc. to keep developers happy and trigger them to make certain code improvements for more security.
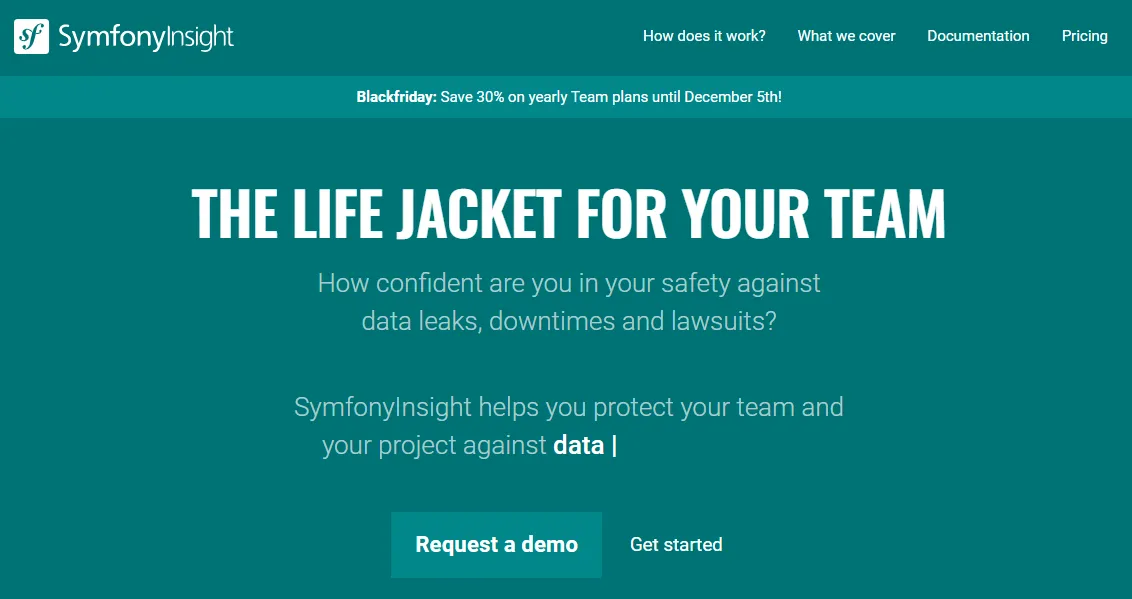
Other php linter you should check out are:
- PHP_CodeSniffer: PHP_CodeSniffer is one of the most commonly used linter tools for PHP. It checks PHP code for compliance with coding standards and offers a variety of predefined or customizable coding standards, including PSR standards (PHP-FIG). PHP_CodeSniffer consists of two PHP scripts: the main phpcs script, which tokenizes PHP, JavaScript and CSS files to detect violations of a defined coding standard, and a second phpcbf script, which automatically corrects coding standard violations. PHP_CodeSniffer is a must-have development tool that ensures your code stays clean and consistent.
- Psalm: Psalm is another static analysis linter for PHP that specializes in typing and security. It provides strong static analysis of code and can also be used to identify type issues.
- PHPMD (PHP Mess Detector): PHPMD checks PHP code for code metrics, DRY (Don't Repeat Yourself) violations, coupling and cohesion, and other potential problems in the code.
- PHPLint: PHPLint is a simple PHP linter that checks for syntax errors and basic coding issues.
- Xdebug: Although Xdebug is typically used for troubleshooting and debugging, it can also be used to check PHP code to gather code coverage and runtime information.
- PHP-CS-Fixer: PHP-CS-Fixer is primarily a code formatter, it can also be used to check and adjust coding standards to improve PHP code.
Our developers in the team use PhpStorm for backend development. The connection of external php linters is easily possible there.
This overview of other tools for quality assurance of php projects is also useful.
Linter tools for Python
Python is the most popular programming language of all, with 28 percent user interest measured by the search volume on Google for tutorials according to the PYPL index (PopularitY of Programming Language)
This means that there is also a whole range of linter tools for Python that you can use to check your Python code for
- Syntax errors and logical errors.
- Unused variables or functions.
- Compliance with coding standards and style guidelines.
- Type checking and static analysis of types (in projects with type annotations).
Just check one of the following linter tools for Python, which you can use to improve your projects depending on your focus:
- pylint: pylint is a widely used linter tool for Python. It checks Python code for errors, style guidelines and coding standards. Pylint is highly configurable and can be adapted to the specific requirements of a project.
- pyflakes: pyflakes is a simple and lightweight linter tool that checks Python code for errors and potential problems. It mainly focuses on static code analysis.
- Black: Black is mainly a code formatter, it can also be used as a linter tool to ensure that Python code has consistent formatting and indentation.
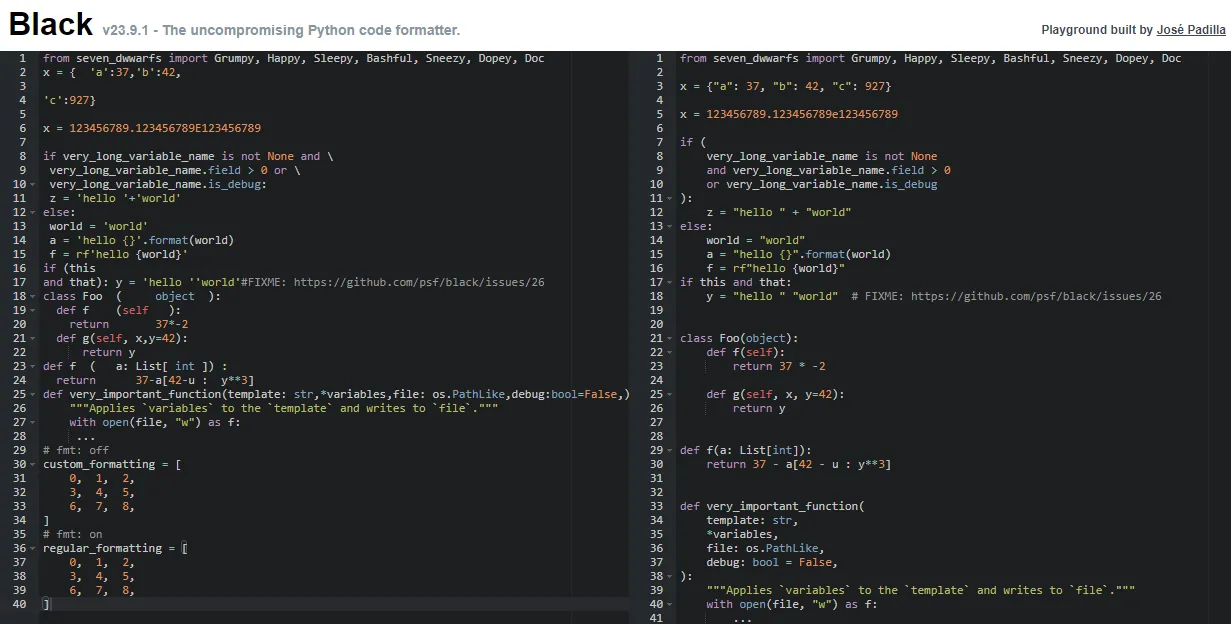
Other Python linters include mypy, isort, Prospector and also Bandit, which is specifically designed for security vulnerabilities in Python.
Linter tools for Laravel
There are linter tools and code review tools designed specifically for the Laravel PHP framework to check Laravel applications for bugs and style issues. We are very grateful for this, because as an agency we specialize in Laravel.
Larastan: Larastan is a static analysis linter for Laravel applications based on PHPStan. It enables static type checking of Laravel-specific code to detect errors and problems related to Laravel.
Laravel Shift: Laravel Shift is a service that checks Laravel applications for updates, security vulnerabilities and outdated packages and recommends how to update or fix them.
PHP Insights: PHP Insights is a code analysis tool designed specifically for Laravel. It checks Laravel applications for coding standards, performance issues, code complexity and other code quality metrics.
Laravel Shift Linter: This is a special linter from Laravel Shift that specializes in style guidelines and best practices for Laravel development. It checks the code for compliance with Laravel conventions.
Laravel IDE Helper: Although Laravel IDE Helper is not directly a linter, it is a helpful tool that makes Laravel-specific code visible in your development environment such as PhpStorm, making it easier to develop and understand Laravel code.
Laravel Debugbar: While this package is not a linter, it is useful for helping developers review and optimize the performance of Laravel applications. It displays detailed information on request and response time as well as database query performance.
Here are more tools specifically for the Laravel ecosystem: https://madewithlaravel.com/
Linter tools for MySQL
Compared to other programming languages, there are generally fewer traditional linter tools for MySQL and SQL, which are used in the same way as for source code in programming languages. SQL is a query language for database manipulation, and the way SQL queries are written and optimized is different from other languages.
However, there are tools and approaches to check SQL code for performance and security:
MySQL Shell (mysqlsh): MySQL Shell provides some checking functions that can help check SQL queries for syntax errors and some basic issues.
MySQL Query Analyzer: MySQL has a built-in query analyzer that can be used to identify slow or inefficient queries and analyze the performance of queries.
Code Reviews: A best practice for reviewing SQL code is to have other developers or database administrators perform code reviews. This can help uncover potential problems and opportunities for improvement in SQL code.
SQL Performance Tuning Tools: There are third-party tools and services that specialize in optimizing SQL queries and identifying performance issues. Examples include Percona Toolkit and Query Analyzer services.
SQL linter plugins: Some developer IDEs and database management tools offer linter plugins that can check SQL queries for syntax errors and some common issues.
While there are no specific linter tools for MySQL that match the traditional understanding of linting tools, the above approaches and tools are helpful to check SQL code for performance issues, security vulnerabilities and syntactic errors. Identifying and fixing problems in SQL queries is important to optimize database performance and ensure that database operations are performed efficiently and securely.
Linter tool for CSV
There are also linter tools and validation tools specifically designed for CSV (Comma-Separated Values) files to ensure that the data in the CSV files is correctly formatted and valid. Here are some examples of linter tools and validation tools for CSV files:
- csvlint is a command line tool and online platform that can be used to check CSV files for syntax errors and validity. It displays errors and warnings in CSV format and allows users to validate and analyze the file.
- csvkit is a suite of command line tools for working with CSV files in Python. It includes the csvclean tool, which allows you to check CSV files for duplicates and inconsistent data.
- OpenRefine is an open source software used for data cleansing and transformation. It also offers functions for validating and checking CSV files for inconsistent data.
- CSVLint is an online service for validating CSV files. You can upload a CSV file and the tool will check it for syntax errors and inconsistencies.
These tools can be helpful to ensure that CSV files are correctly formatted and valid, especially if you are using CSV files for data exchange or data processing.
Linter tool for XML files
There are linter tools and validation tools specifically designed for XML (eXtensible Markup Language) to ensure that XML documents are correctly structured and valid. Here are some examples of linter tools and validation tools for XML:
XMLLint is a command line tool that is part of the libxml2 package and is used to check XML documents for syntax errors and validity. It is widely used and can be used on various platforms.
There are many online XML validation services that can be used to upload XML documents and check them for validity. One example is this Online XML Validator.
Furthermore, the typical editors also offer extensions for XML validation.
Linter tools for validating sitemap.xml
There are also linter tools and validation tools specifically designed for sitemap.xml files to ensure that they conform to sitemaps protocol standards and are formatted correctly. Here are some examples of linter tools and validation tools for sitemap.xml files:
Google Search Console: Google offers you a built-in sitemap validation tool in Search Console. You can upload your sitemap.xml file and have it checked for errors or problems. The submission is then always linked to the indexing request to Google.
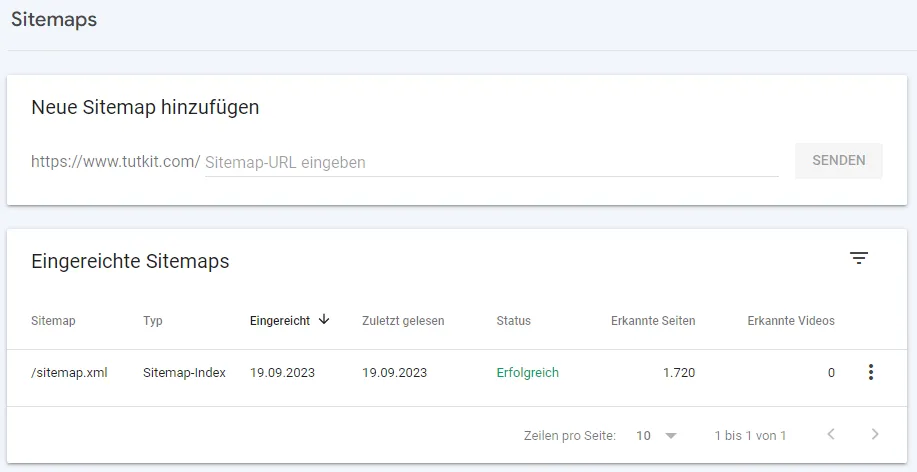
There are various online XML sitemap validation services that can be used to check sitemap.xml files for syntax errors and validity. These include the XML Sitemap Validator (online tool). Here you enter a URL to your sitemap and find out whether it is valid or where there are errors.
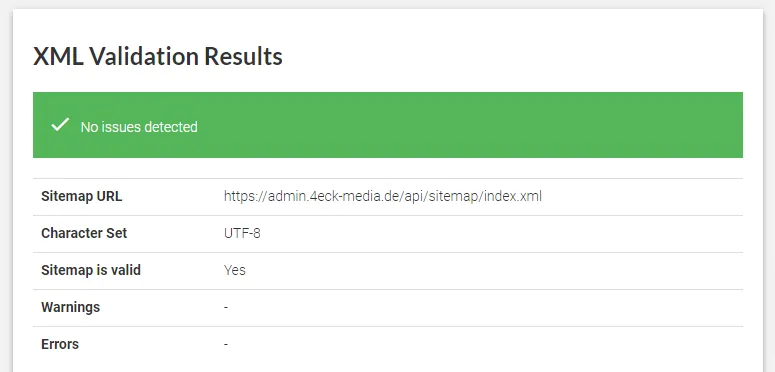
With XMLLint, which I mentioned earlier, you can simply insert the code of your sitemap.xml file and check the validity directly.
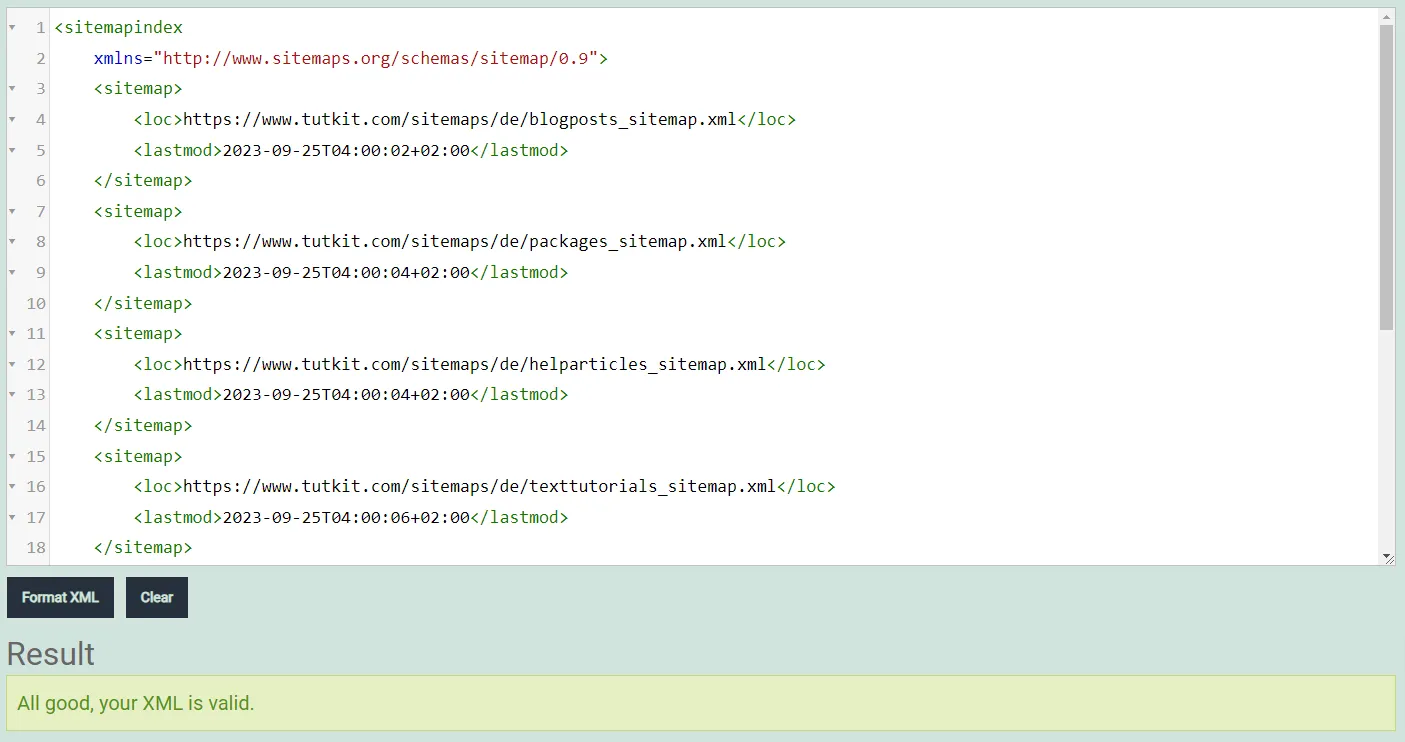
Screaming Frog SEO Spider: This paid SEO tool offers a function for validating sitemaps. You can import your sitemap.xml file into the tool and have it checked for errors.
Using sitemap.xml validation tools is important to ensure that search engines like Google and Bing can interpret your sitemap correctly. Invalid or incorrect sitemaps can cause search engines to have problems crawling and indexing your website. It is therefore advisable to check your sitemap.xml file before use or when making changes to ensure that it complies with the sitemaps protocol standards.
Linter tool for structured data
There are linter tools and validation tools specifically designed for structured data. Structured data is information that is in a standardized format and is used to help search engines and other applications better understand the content of a web page. Here are some examples of linter tools and validation tools for structured data:
Google Structured Data Testing Tool: This tool from Google allows you to validate structured data on a website. It helps to ensure that the structured data complies with Google's recommended standards.
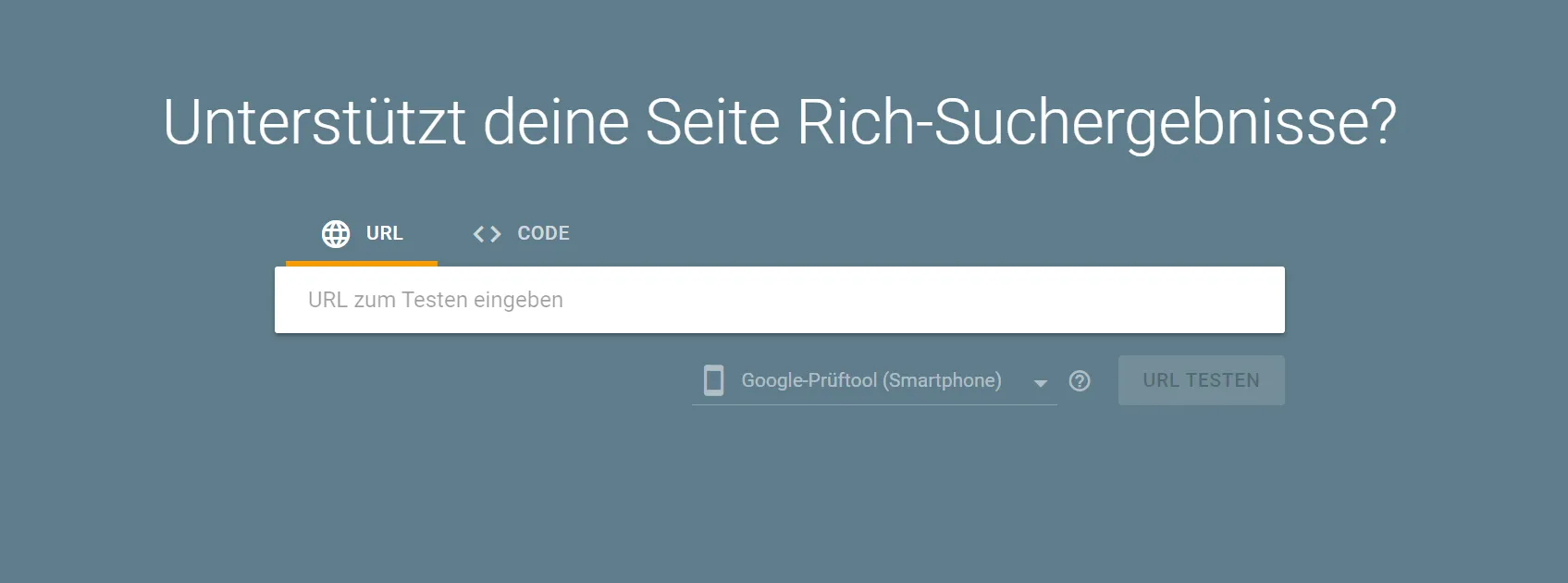
Structured Data Linter: This online tool allows you to check structured data in various formats, including JSON-LD, Microdata and RDFa. It displays warnings and errors in structured data markup.
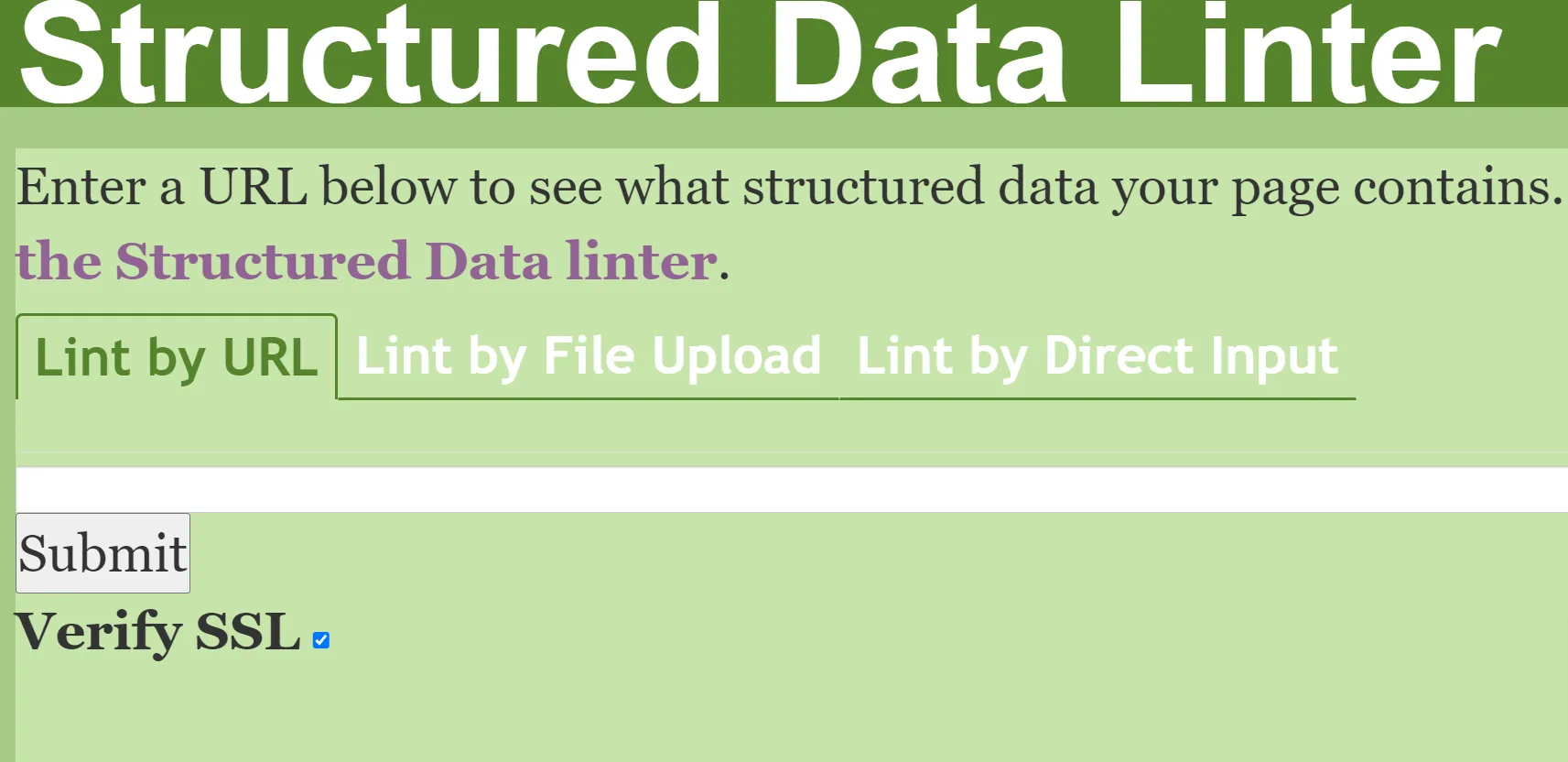
Schema.org Generator: This tool helps to create structured data in JSON-LD format by helping you to select schemas and create markup code. It is useful for generating structured data for specific content. Here in the example, it is immediately clear that isbn is not recognized as a property. After researching, it becomes clear that the correct markup is gtin13 instead of isbn .
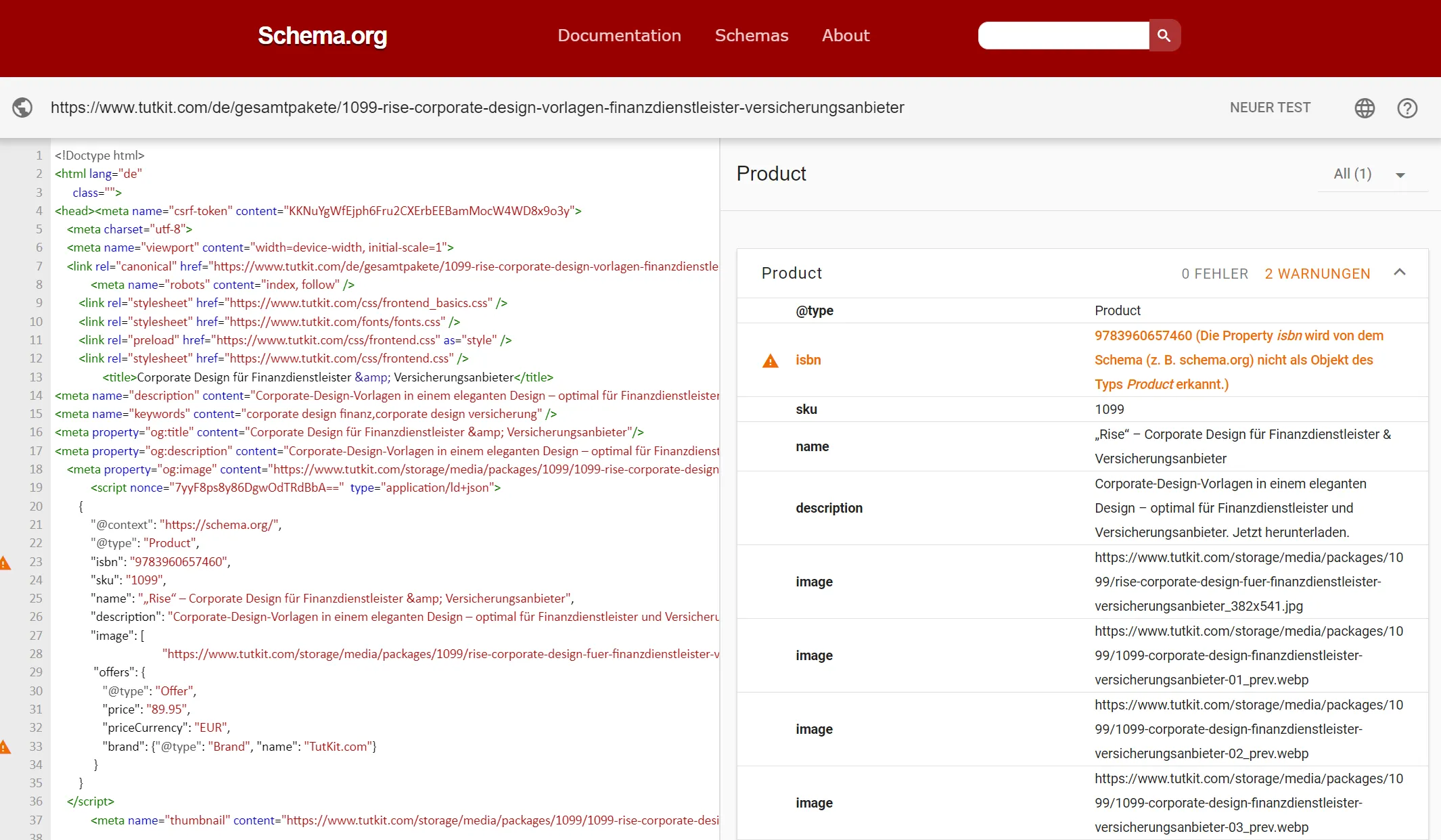
These linter tools and validation tools are helpful to ensure that structured data on your website is formatted correctly and created according to the standards and recommendations of search engines and Schema.org specifications. This helps to improve the visibility and understanding of content by search engines and optimize rich snippets in search results. So use them!
Linter extensions for editors and development environments
For most editors and development environments, there are also options for activating the linter tools mentioned above. You can find the most important links here:
Sublime Text:
https://www.sublimelinter.com/en/latest/
Visual Studio Code:
https://marketplace.visualstudio.com/search?term=lint&target=VS&category=All%20categories&vsVersion=&sortBy=Relevance
Adobe Dreamweaver:
https://helpx.adobe.com/de/dreamweaver/using/linting-code.html
Atom:
https://atomlinter.github.io/
PhpStorm:
https://www.jetbrains.com/help/phpstorm/linters.html
https://www.jetbrains.com/help/phpstorm/php-code-quality-tools.html
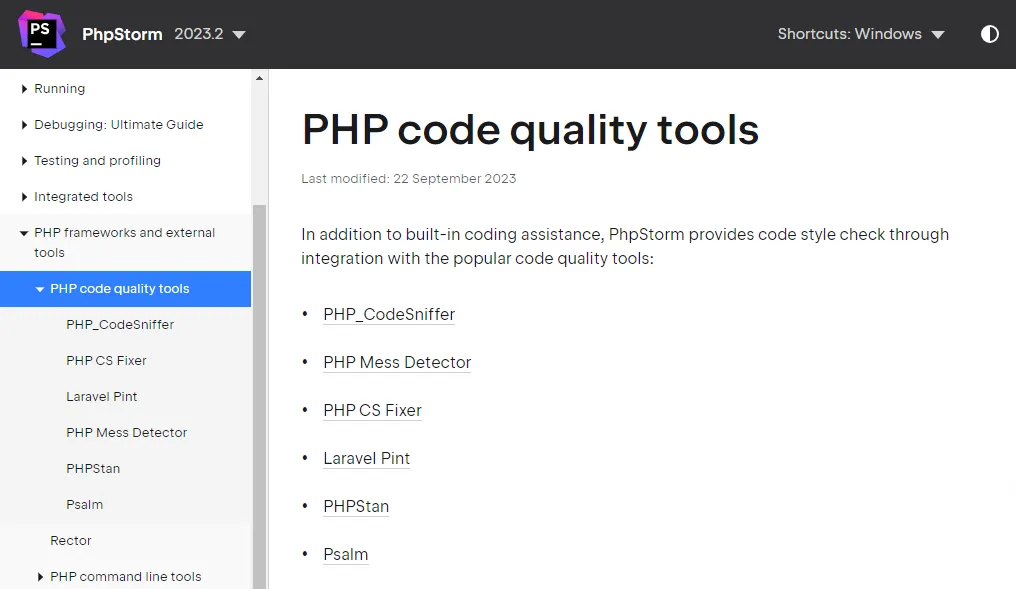
Does the use of linter tools also help with SEO measures?
The use of linter tools and search engine optimization (SEO) are not normally directly related. Linter tools are development and code review tools used to identify errors and style issues in source code, while SEO focuses on optimizing websites and content for search engines like Google to improve visibility and ranking in search results.
Nevertheless, incorrect or inefficient code practices on a website can have an indirect impact on SEO:
- Load times: Poorly optimized code can increase the load times of a website, which in turn negatively impacts the user experience. Slow load times can impact SEO, as search engines like Google favor websites with faster load times.
- Mobile optimization: Mobile optimization is an important factor for SEO. If the code is not responsive or has display issues on mobile devices, this can have a negative impact on SEO rankings.
- Content and structure: Although Linter tools mainly target the code itself, they can also point out structural issues or missing metadata that can impact SEO. For example, they can point out if important meta tags such as the title or meta description are missing.
- Usability: Clean and efficient code can help improve the usability of a website. When users experience the website positively, this can indirectly lead to a lower bounce rate and longer time spent on the website, which in turn can improve SEO.
Overall, linter tools and SEO are different aspects of website development and optimization, but they can influence each other if inefficient code or structural issues affect the performance or user experience of a website. It is therefore important to pay attention to both code quality and SEO optimization factors in order to create a well-functioning and highly visible website.
My conclusion:
Developers use linter tools to ensure that their code meets the set quality standards and conventions that apply in a project or in the developer community. If your programming language or framework wasn't included in the list of linter tools above, do some research. There is bound to be a lintering tool for your preferred programming language.
As a result, the use of linter tools helps to detect errors at an early stage, make the code more readable and better and ensure the overall code quality, reliability and maintainability of software and web projects. Linter messages are therefore an important part of the development process and help to ensure the reliability and maintainability of software projects.