In this guide, you will learn how to reset a chat using the OpenAI API without having to restart the server. There are often situations where you simply want to forget the previous chat history and start over. In this process, a chat ID plays a crucial role, as it allows resetting the chat history on the server. The following tutorial will show you how to implement this function.
Main Insights
- To reset the chat, you can generate a new chat ID.
- The chat ID is created when the page is reloaded and sent to the server.
- The server compares the new chat ID with the current one and resets the chat history if they are different.
Step-by-Step Guide
First, you already have a functional chat application, but there is a small issue: you cannot reset the chat history yet. To enable this, we need to introduce an ID that is generated every time the page is reloaded.
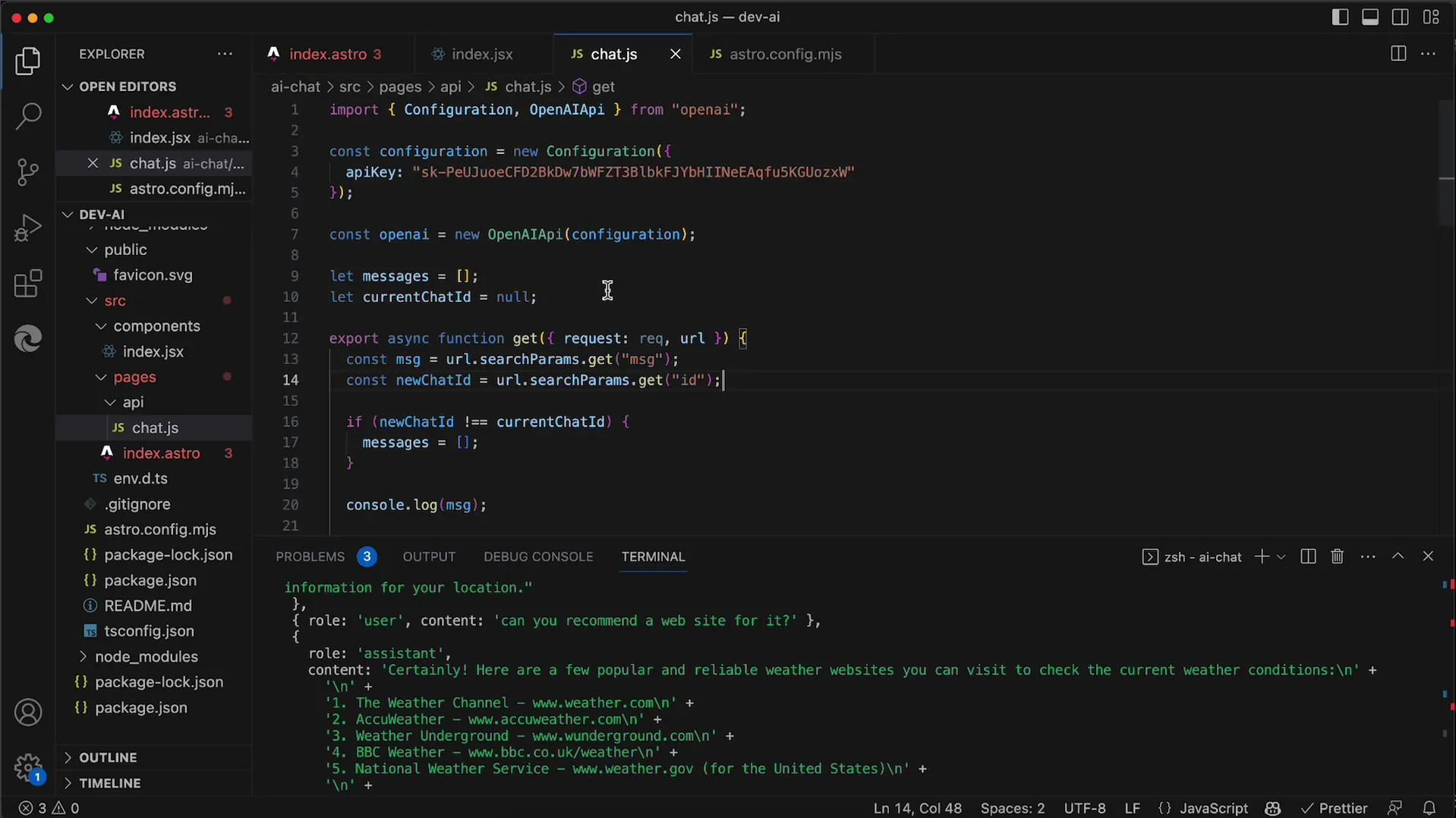
You then need to pass this new chat ID to your server. When the server receives a new ID, it can reset the chat history. This is done through a simple comparison: if the new chat ID does not match the current chat ID, the message list is reset.
To implement this, you must ensure that the client can generate a random ID. You will pass the ID with the search parameter ID. So let's take a look at the index.jsx file of your React component.
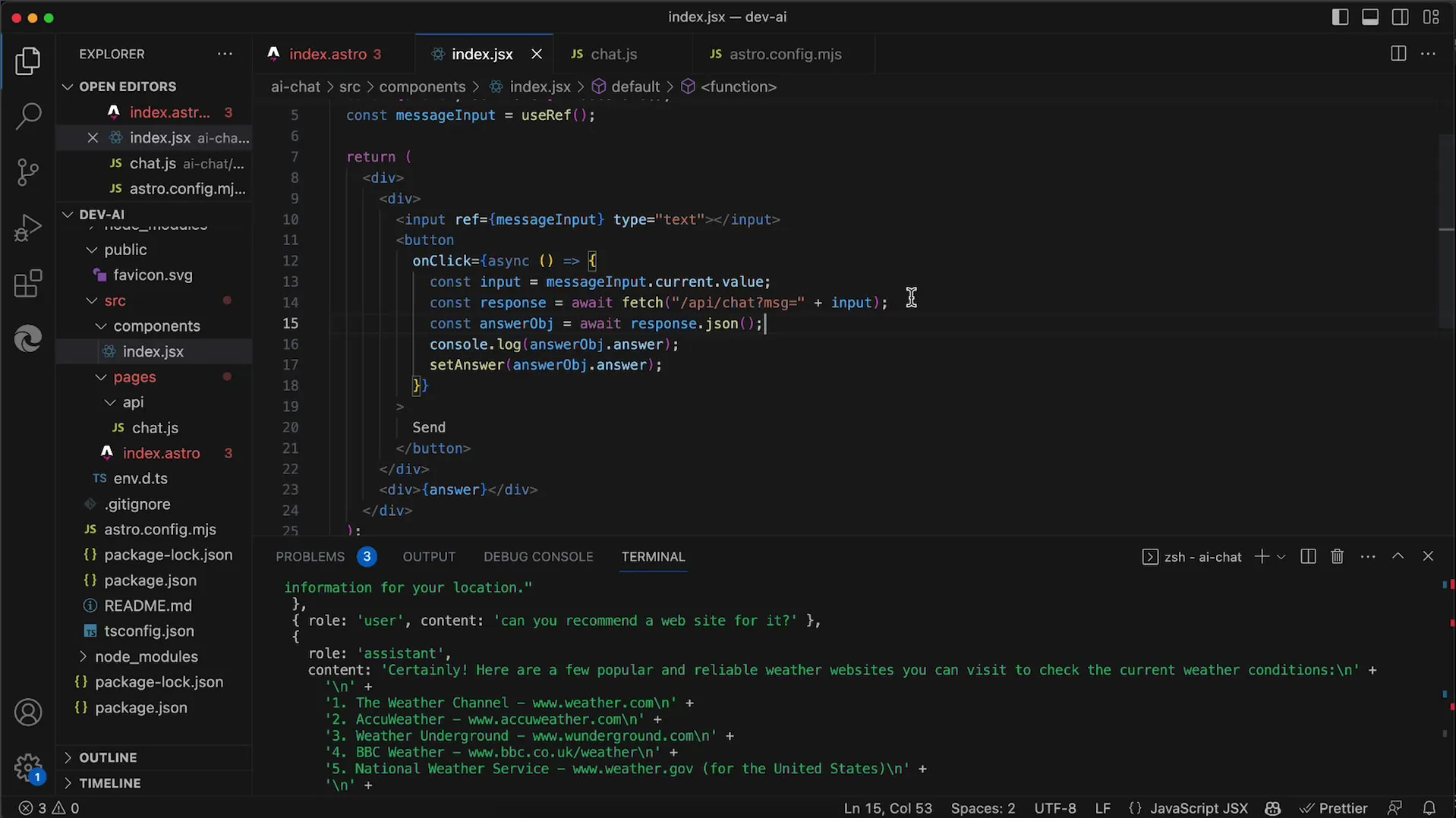
In this file, you have already passed the necessary message (MSG). Now you can also pass the ID. You can effectively do this using template strings to make inserting variables easier.
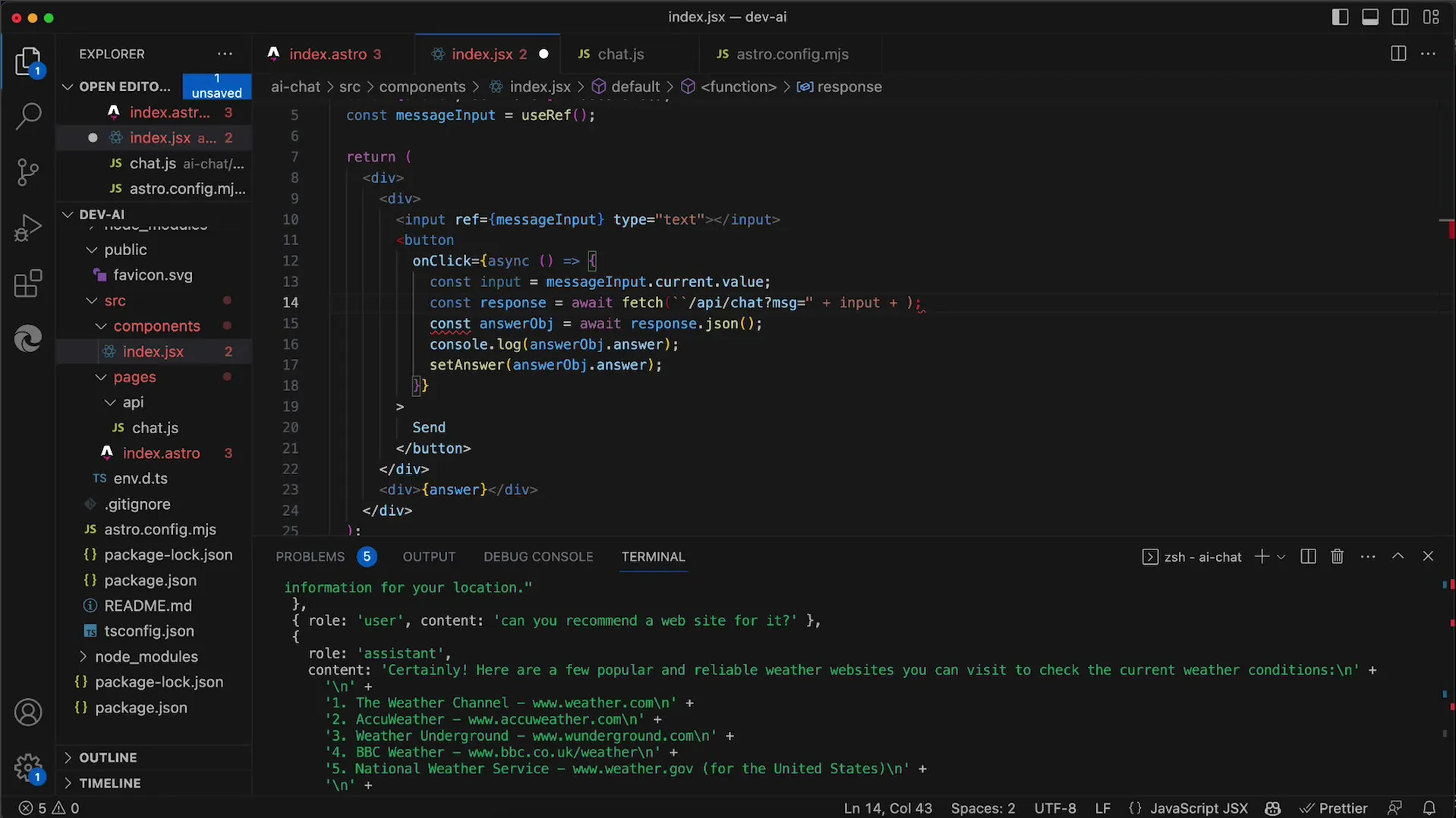
So, convert the code into a template string and insert the variables accordingly. It could look like this: MSG, that's your input field, and the ID equals your new ID.
Now you need to think about generating the ID. A simple method is using the current date. You can simply define a constant that uses the current time in milliseconds since 1970. To do this, you can use Date.now() and then convert this time into a string.
Now, every time the page is reloaded, we will send a new ID. This should work as desired. Let's test this and send a message to see if the data is correctly transmitted to the server.
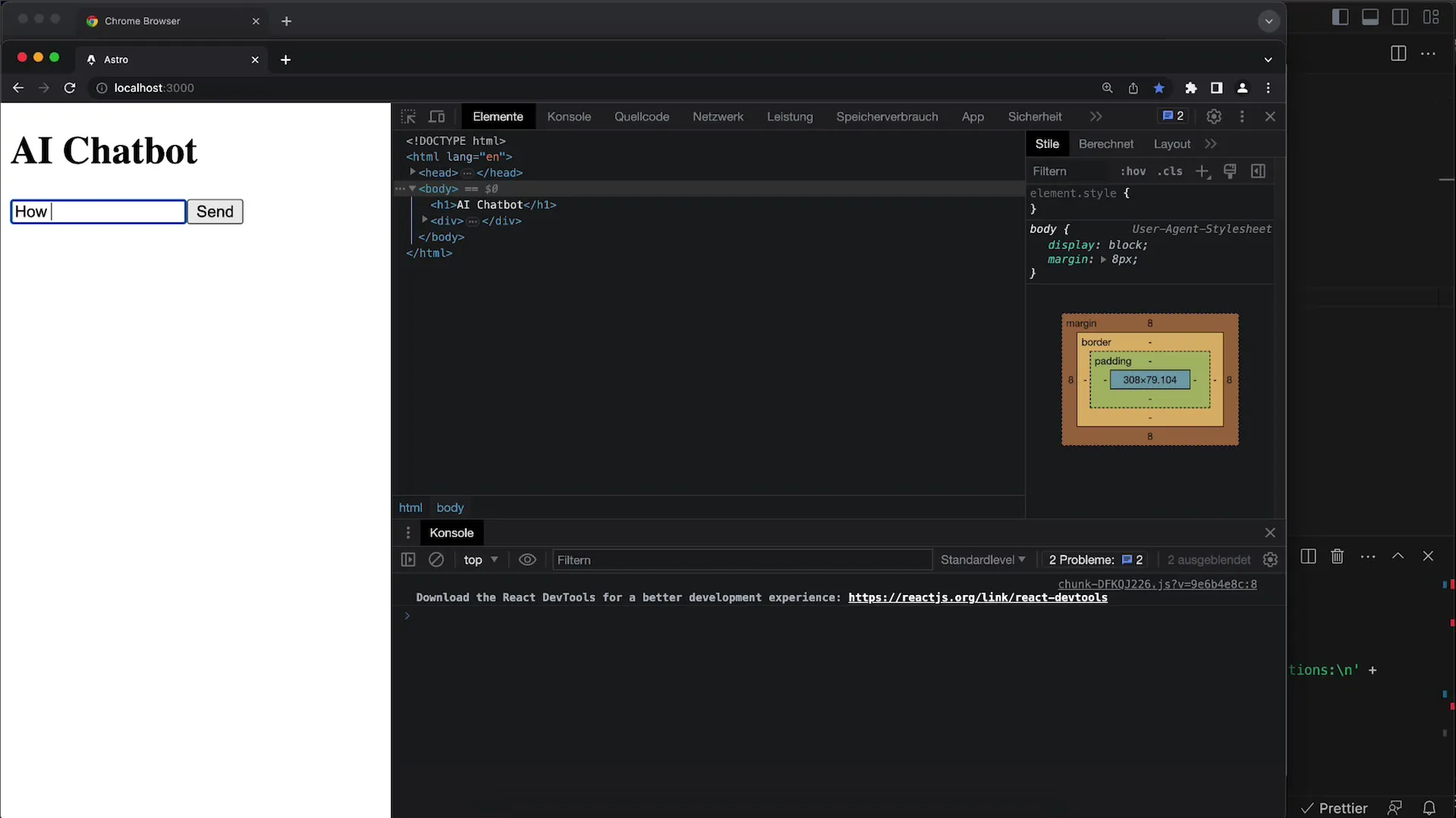
If you send the message "how are you," you will see on the server that there are two messages: one from the user and one from the assistant.
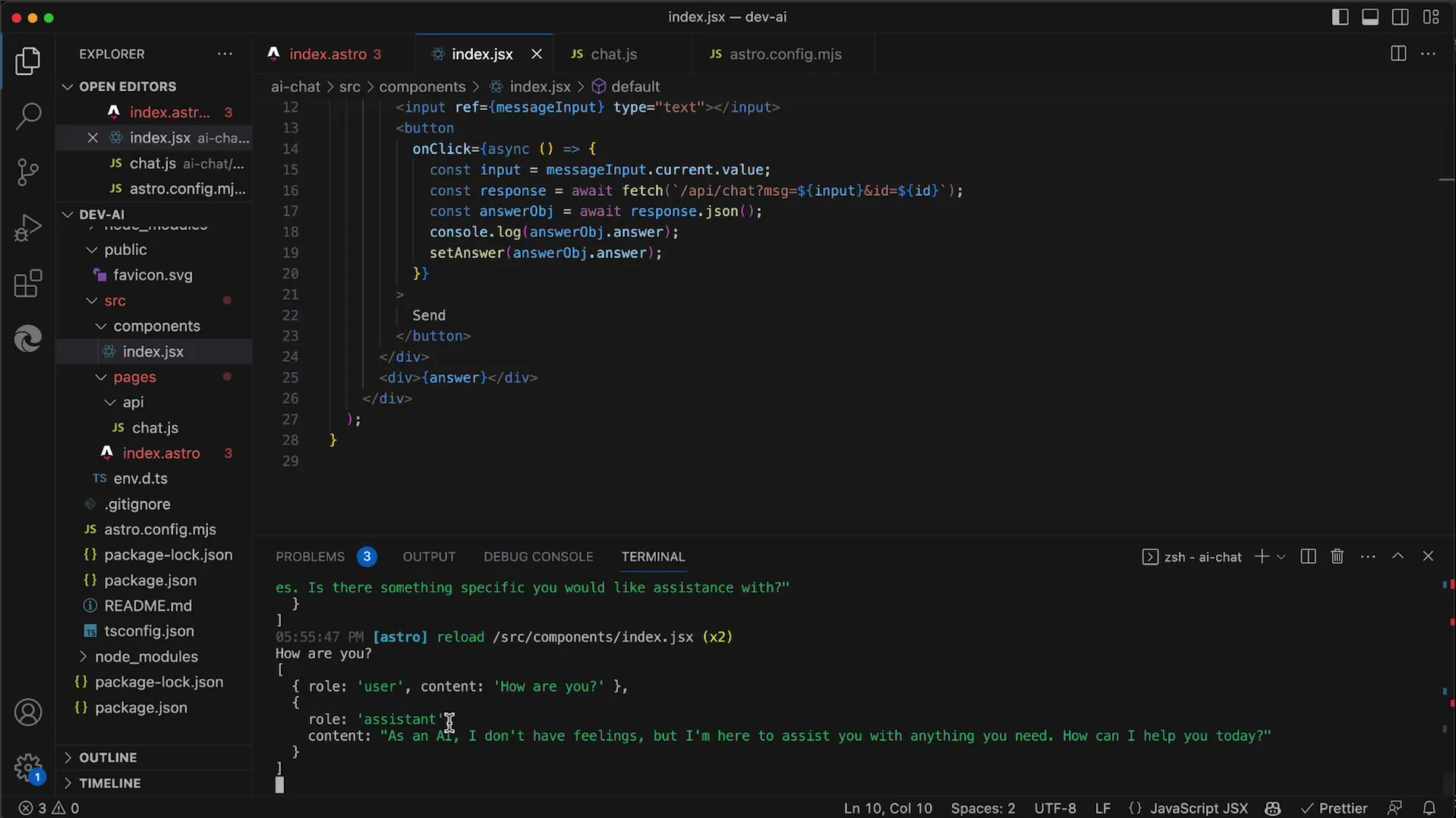
However, if you send another question, such as "why does it not have feelings," the history should be reset accordingly. Only the current question and its answer should be displayed there.
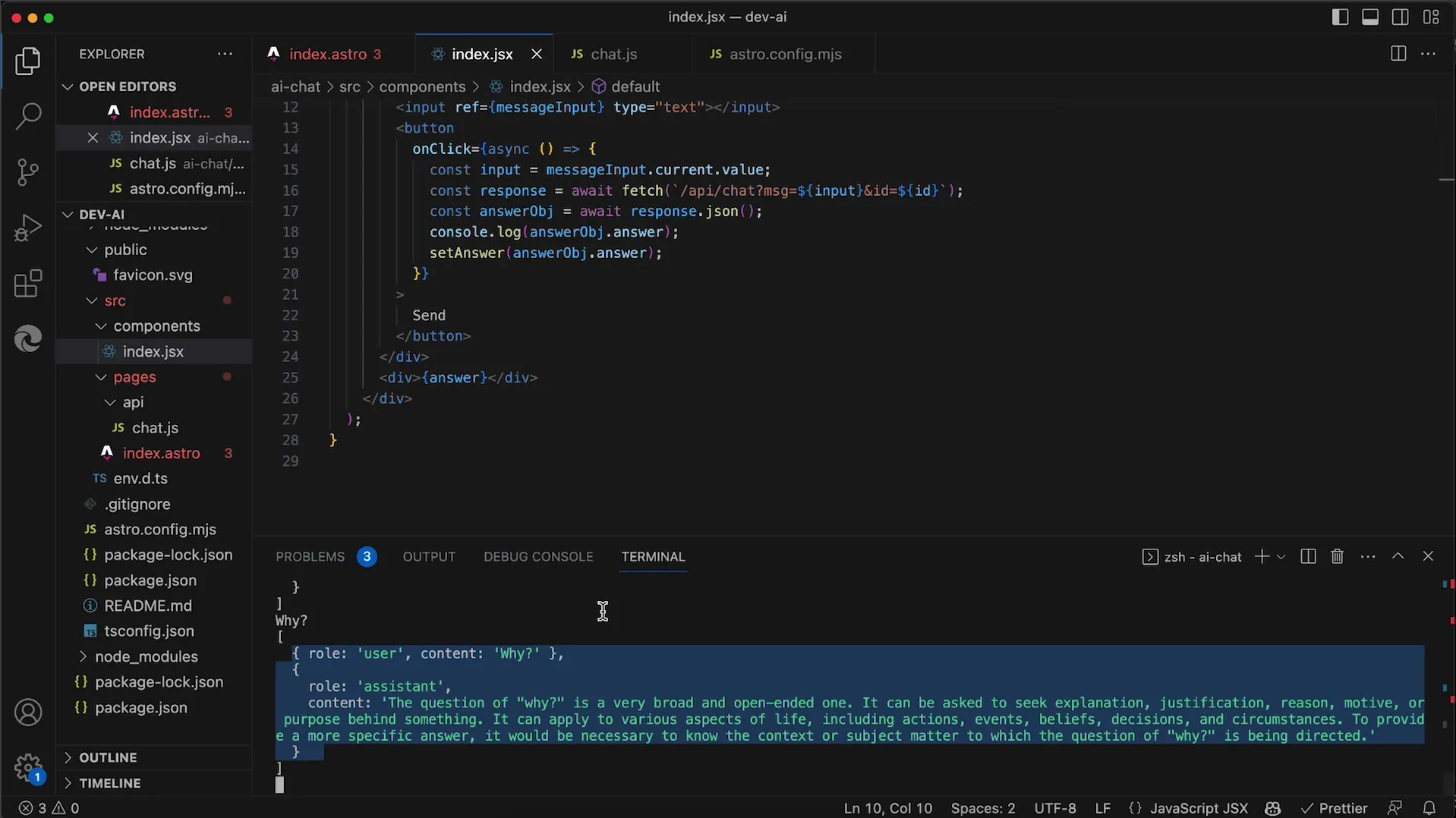
This means that when we check the IDs - there should be no conflict, e.g., the new chat ID being the same as the current chat ID - the logic should be simplified to ensure a smooth process.
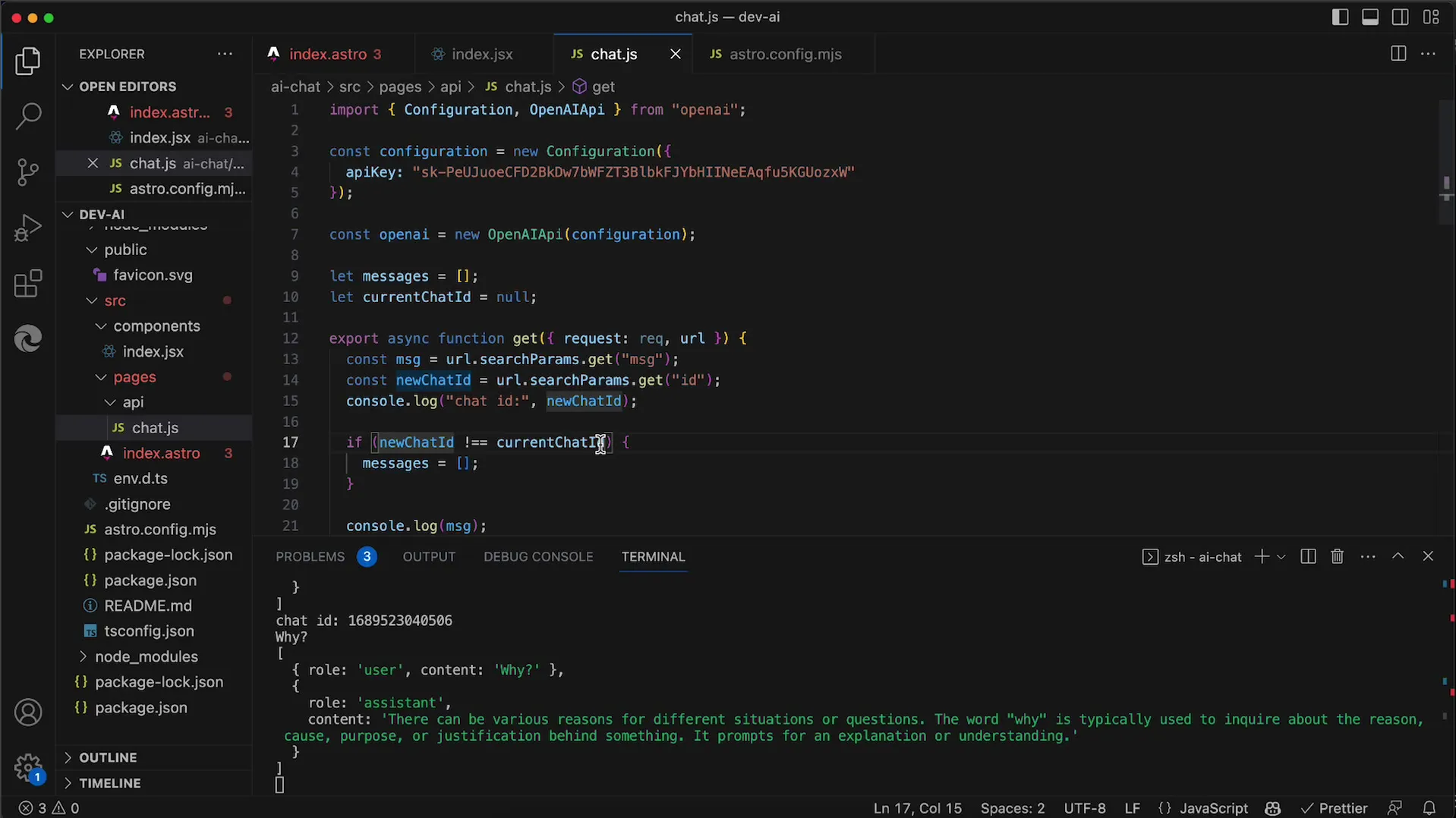
To ensure that it works, run the test again. Reload the page and send the message once more. Now four messages should be saved in the array.
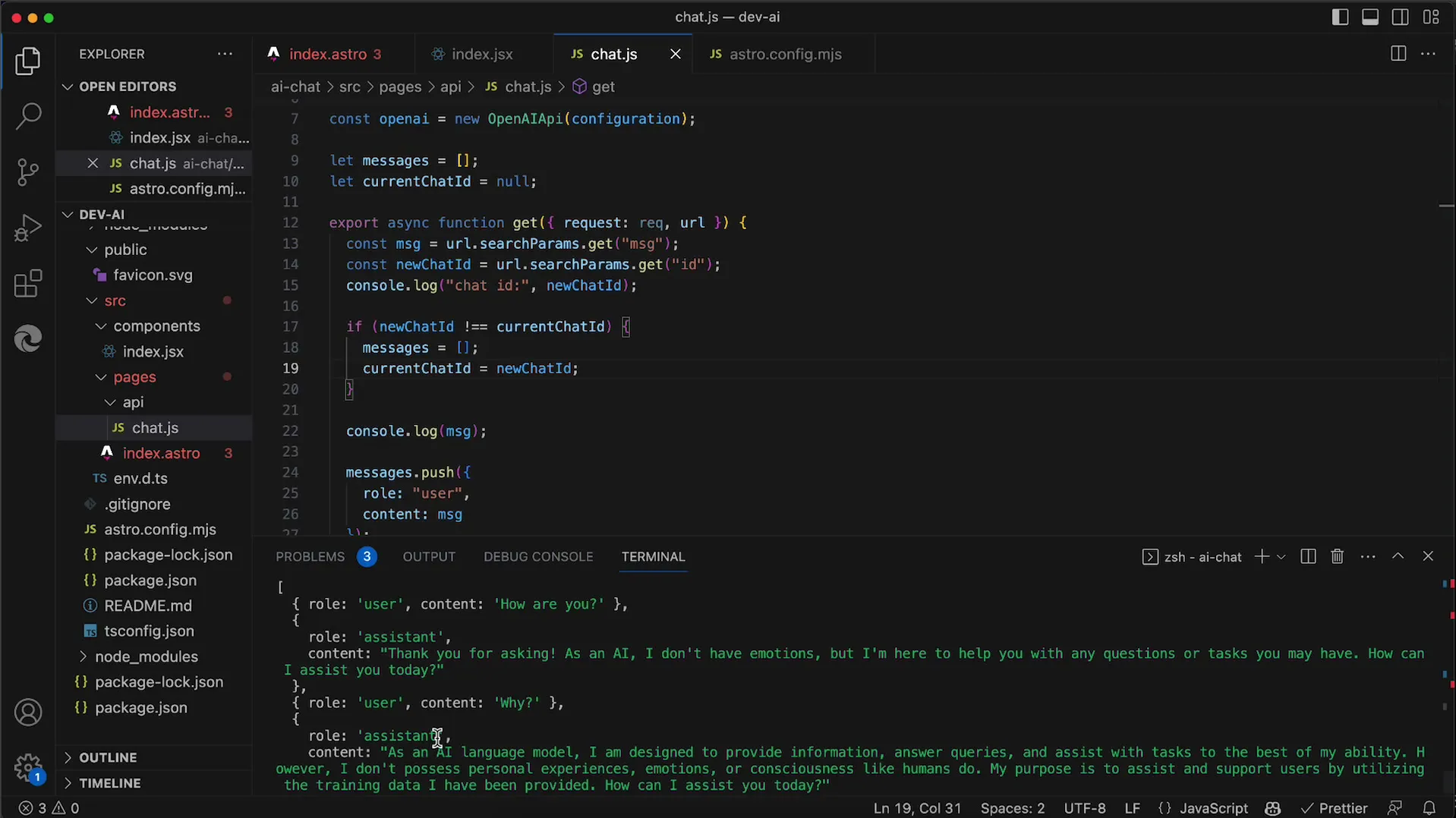
The array shows the total number of messages sent, which means we have successfully reset the history. However, this only happens when you reload the page. Alternatively, you can also implement a button that triggers the chat reset, but for our purposes, reloading the page is sufficient for now.
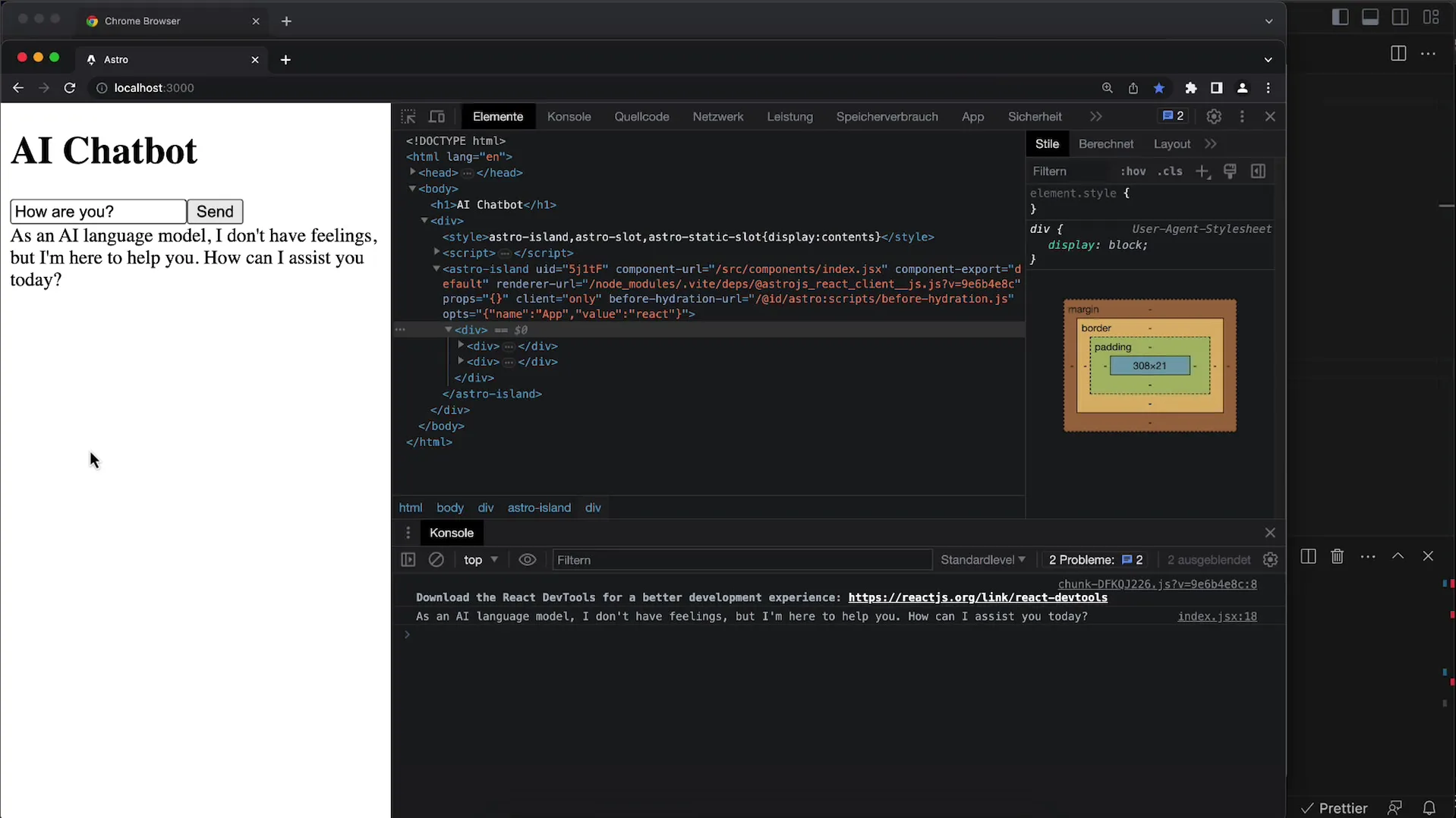
However, please note that the input field will not be automatically cleared after sending a message. Also, the complete chat history is not visible so far, which means that you only see the last question and answer. We will address this in future tutorials.
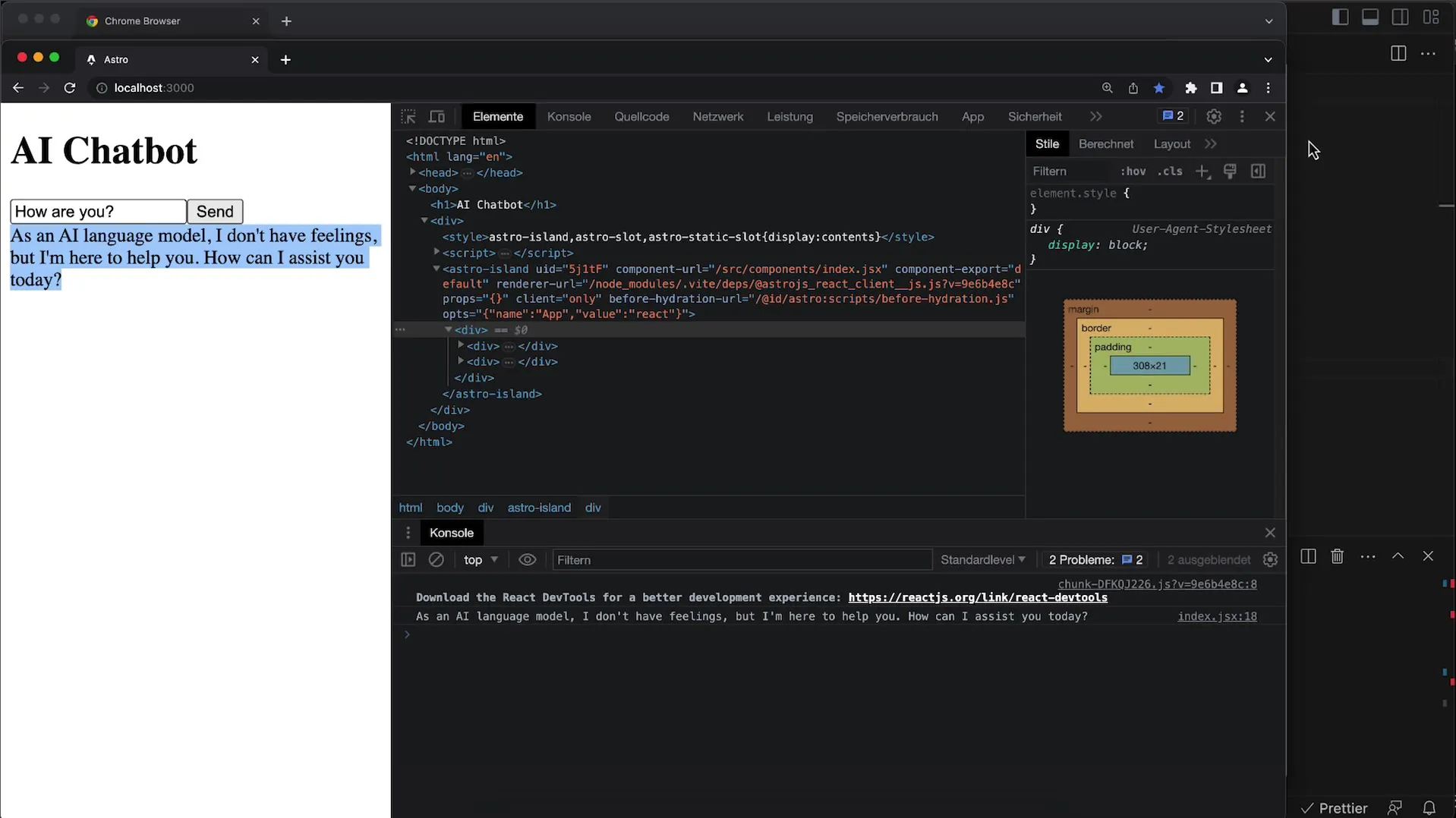
Summary
In this tutorial, you have learned how to implement the chat history reset function in your chat application. By introducing a new chat ID when reloading the page, the history was successfully reset. There are still some improvements that we will implement in the next steps, such as clearing the input field and displaying the full chat history.
Frequently Asked Questions
How can I reset the chat?By reloading the page, the chat history will be reset.
How is the new ID generated?The new ID is generated from the current time in milliseconds.
Can I reset the chat without reloading the page?Yes, you can also implement a button that resets the chat.
What happens if the IDs are the same?If the new ID is the same as the current ID, the history will not be reset.
Do I have to manually delete the input field?Yes, currently the input field is not automatically cleared after sending a message.