In this tutorial, you will learn how to improve the user interface (UI) of your chat application using the OpenAI API. Up to now, chatting has been quite rudimentary, and through various adjustments, we will optimize the UI to better reflect the state of the art, especially as we know it from ChatGPT. By the end of this guide, you will be able to create a more appealing user experience.
Key Insights
- Actively disabling input fields during response generation.
- Displaying the entire chat history instead of just the last response.
- Improvements in the layout and presentation of the chat.
- Addressing common errors and implementing consistent state management.
Step-by-Step Guide
1. Deactivating Input Fields During Response Generation
Start by ensuring that the input field and the "Send" button are deactivated while the response is being generated by the API. Create a new state using useState for the pending flag.
Set pending to true when the user clicks on "Send," and set it to false once the response has been received. This allows you to control the UI and adjust the input fields accordingly.
When you try this step, you should notice that the input field is disabled during the waiting time for the response and the previous input field is cleared, allowing the user to enter a new message.
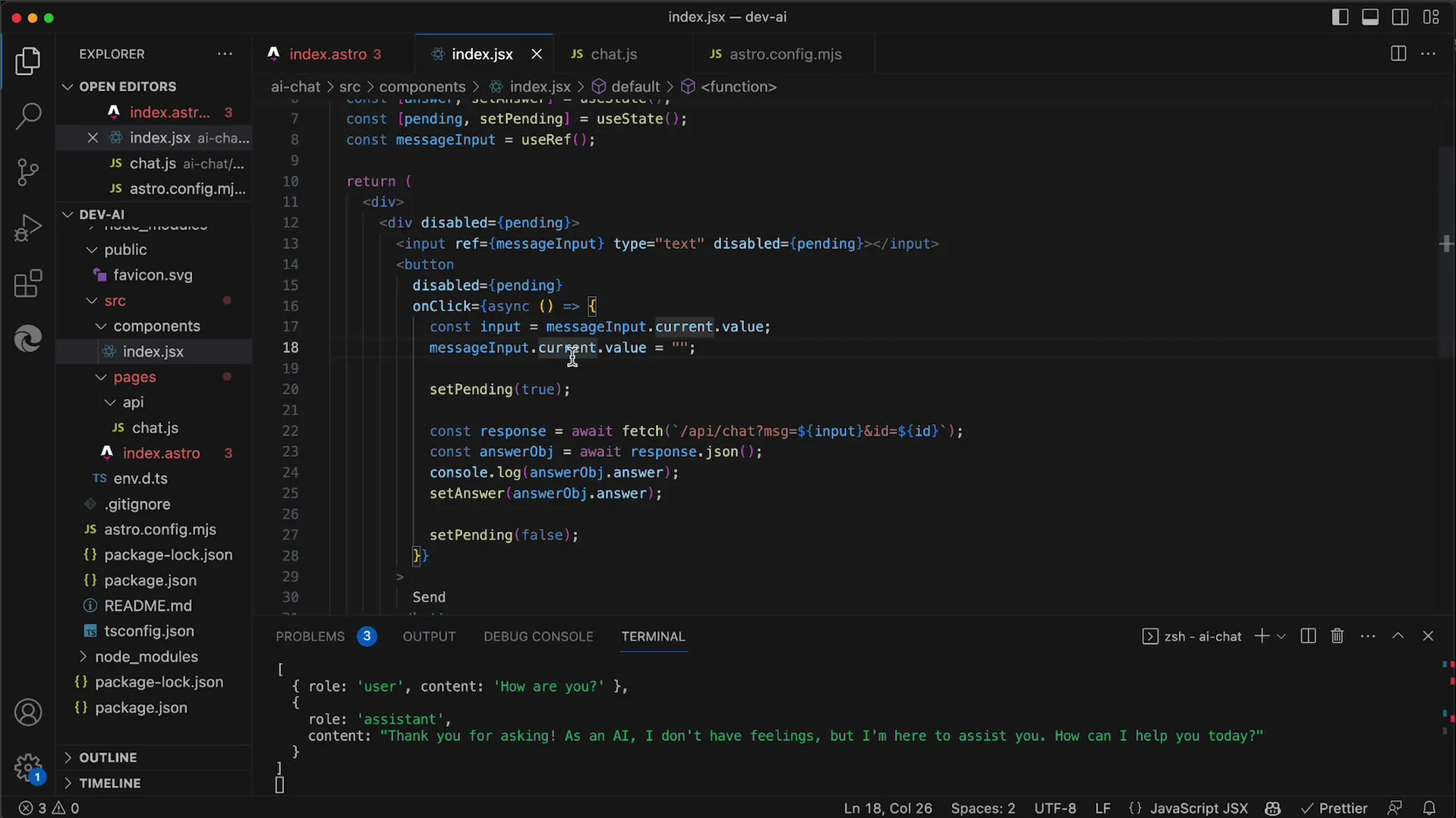
2. Layout Adjustment for Chat History
To create a more appealing layout, you should display the message history below the input field, similar to ChatGPT. To do this, you need to adjust the order of elements in your JSX code so that the history is at the top.
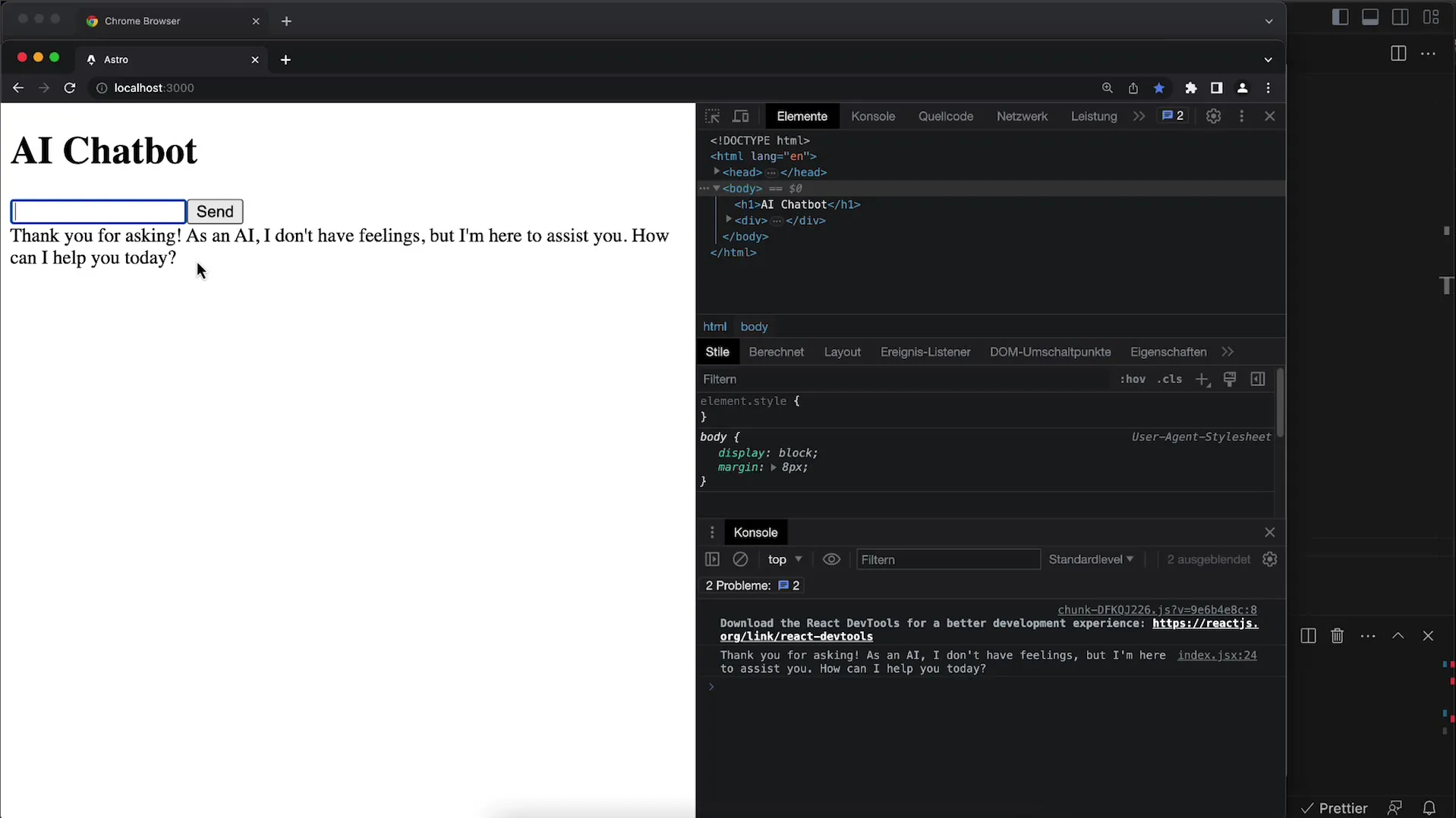
After making this change, test the layout by entering a message and sending it. The history should now be displayed above the input field.
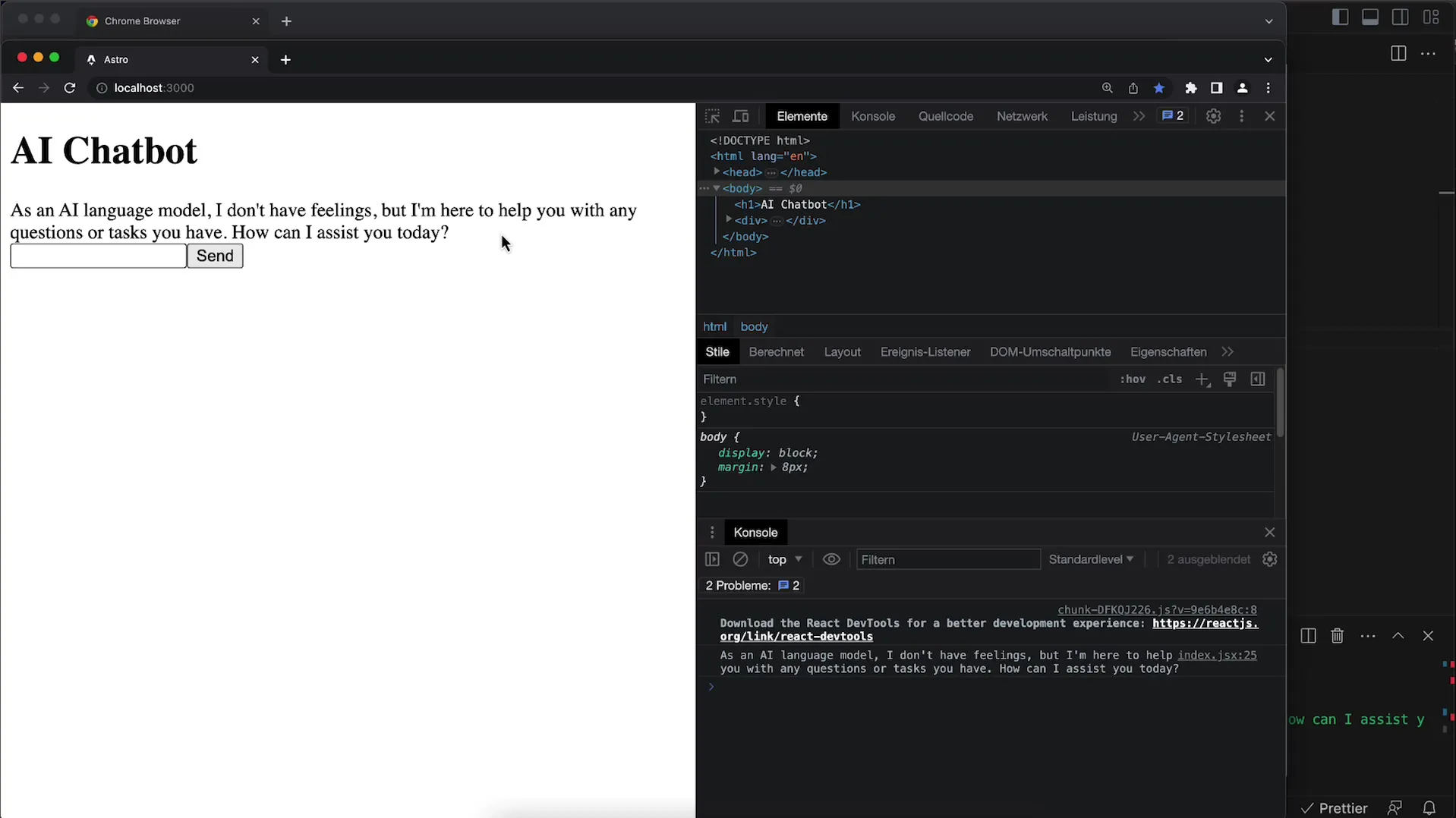
3. Using Flexbox for Better UI Arrangement
To ensure that the input fields and the history are well arranged, use Flexbox. Add display: flex to the outer container and set the flex-direction to column so that the elements are arranged vertically.
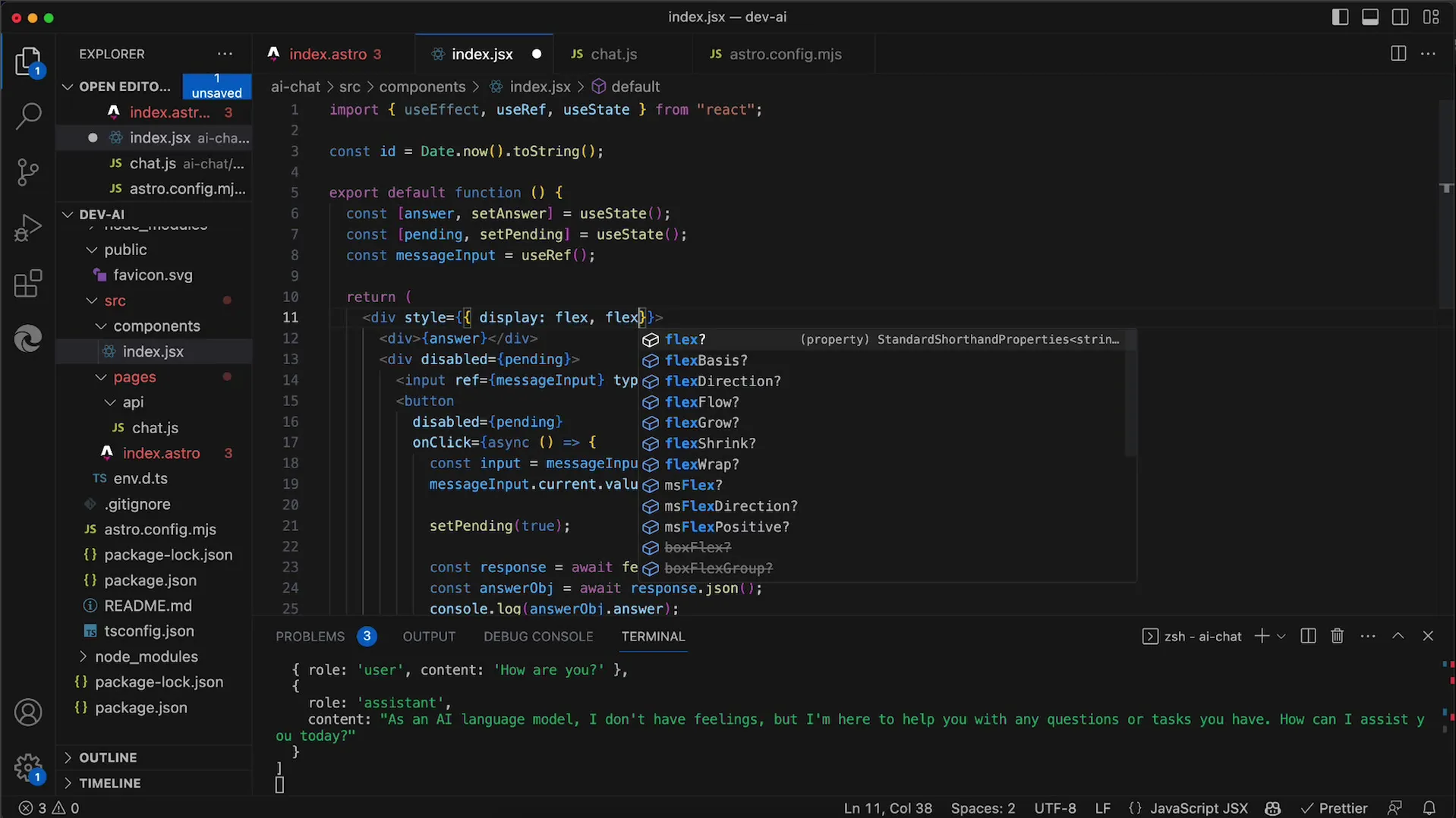
When placing them, you can also ensure there is enough space between the message input field and the history by using flex: 1.
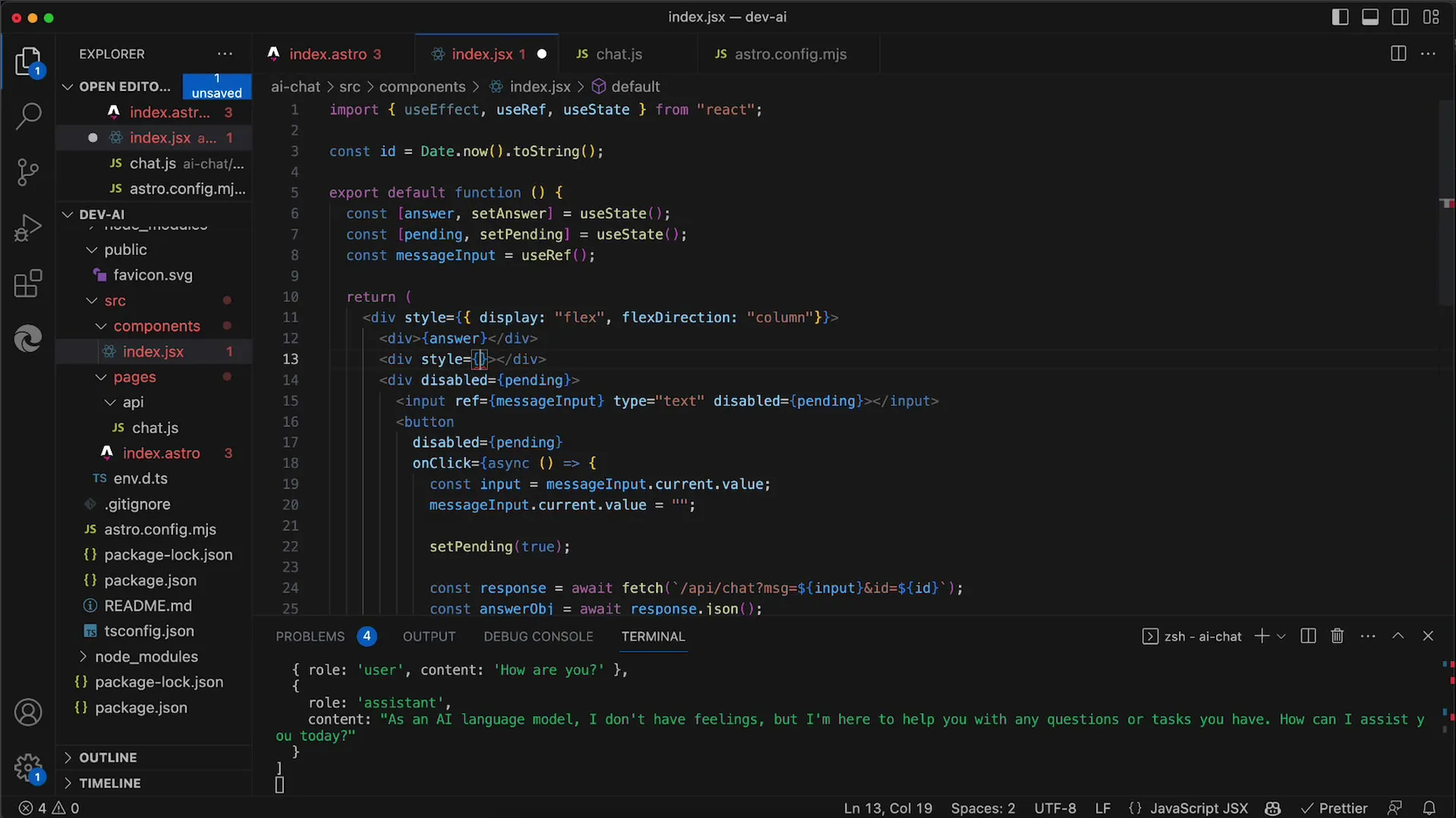
4. Ensuring Full Height for the Containers
Remember that all containers, including body, html, and your main div, need a full height of 100%. Set these attributes in the CSS to ensure your layout is displayed correctly.
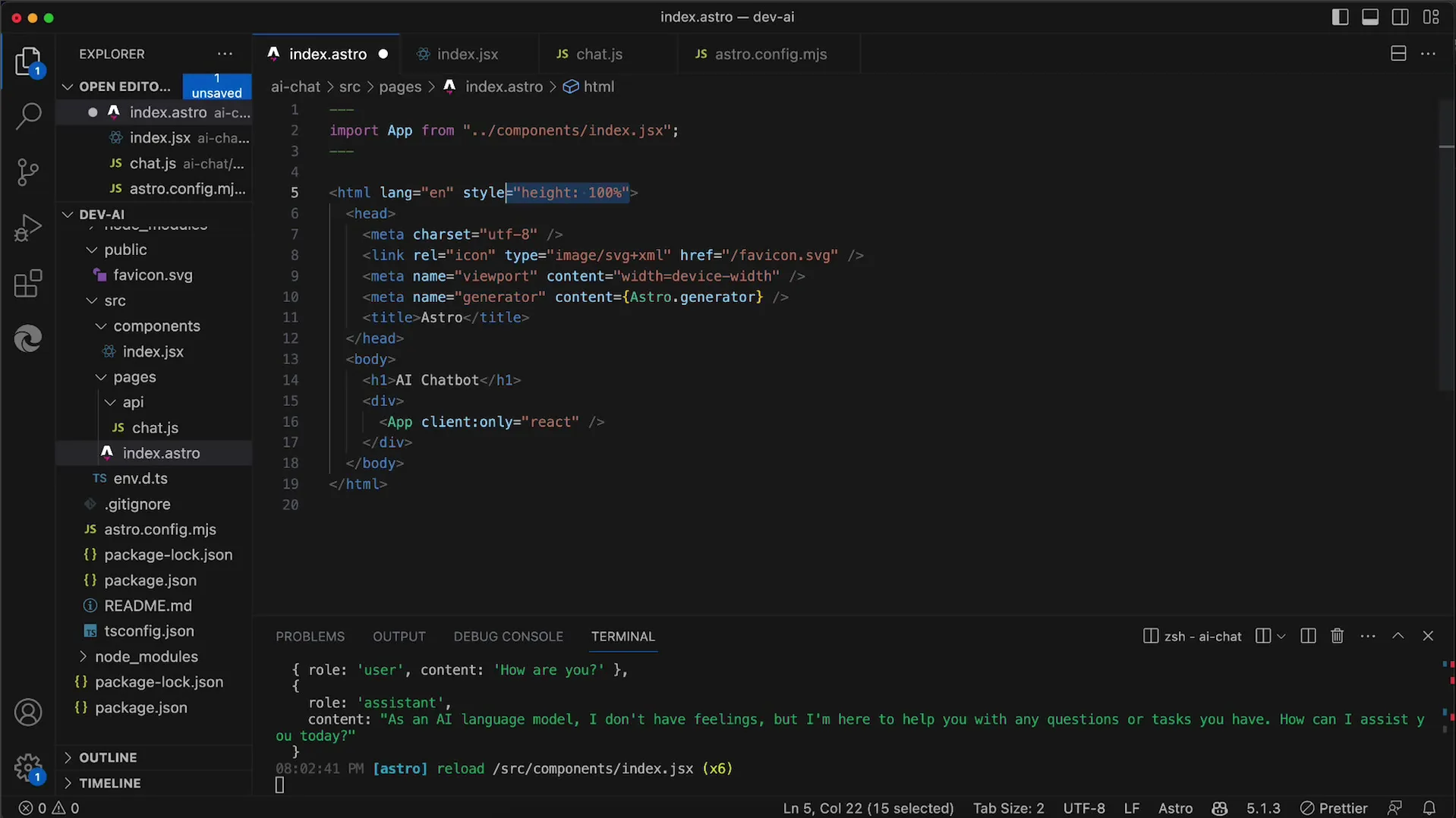
If the layout still doesn't look as desired after these changes, check the margins and set them to 0 to ensure a scrollbar-free view.
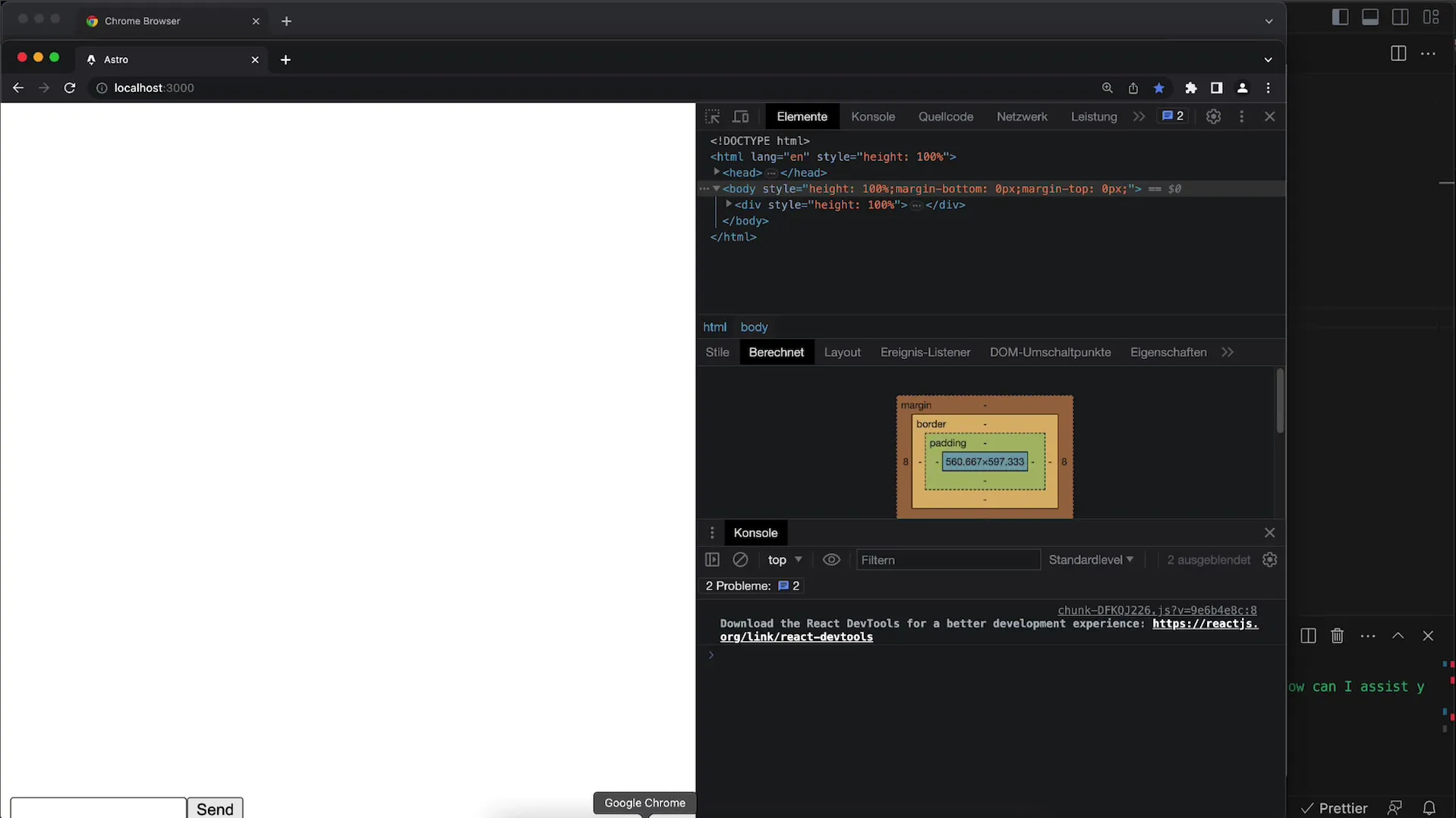
5. Implementing Chat History
To implement the chat history, add another state for messages. This state stores all sent messages. When sending a new message, you need to add it to the messages state.
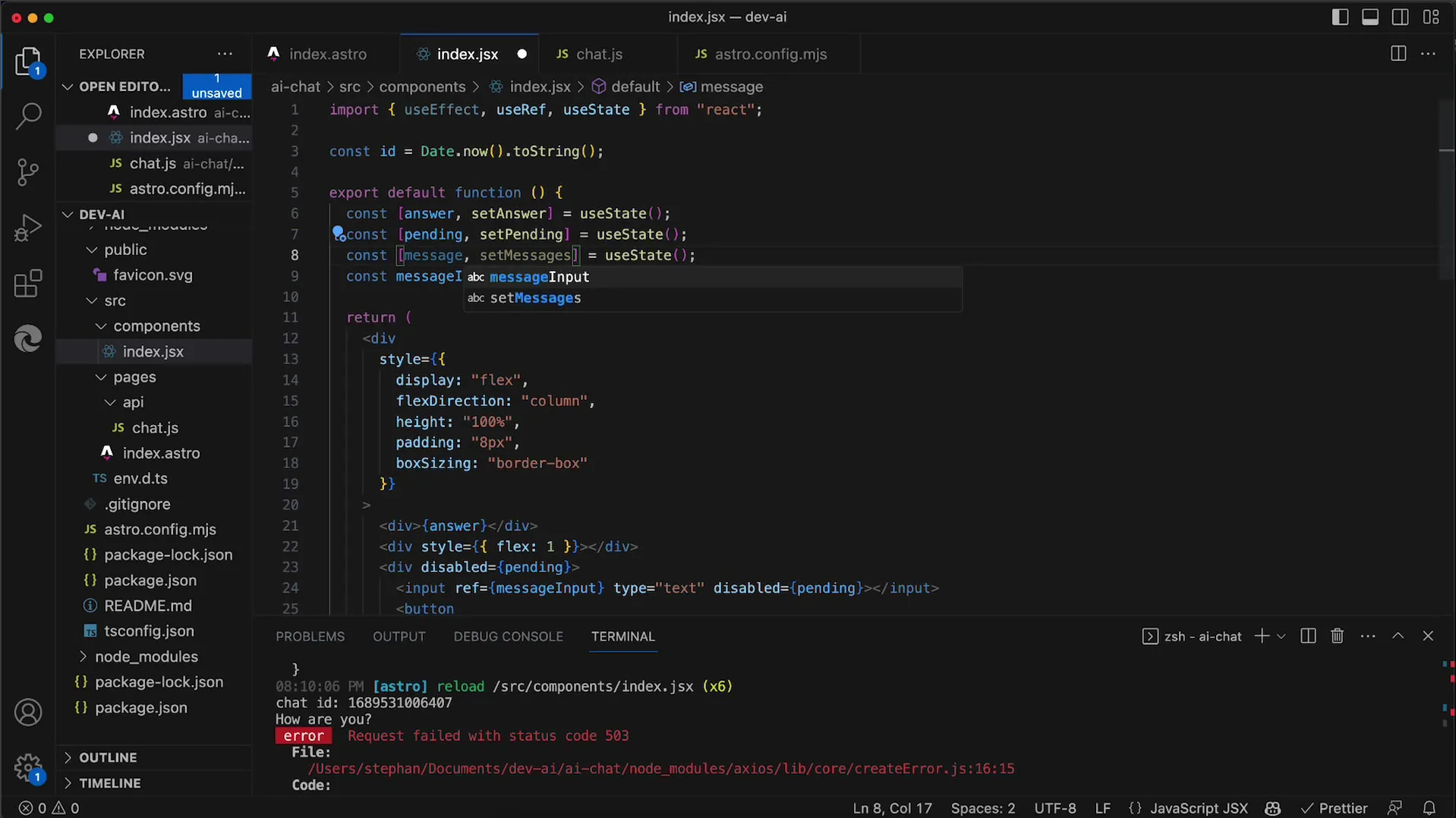
Make sure to use the map function to display each message from the messages array accordingly. This allows for the correct output of each user and API message.
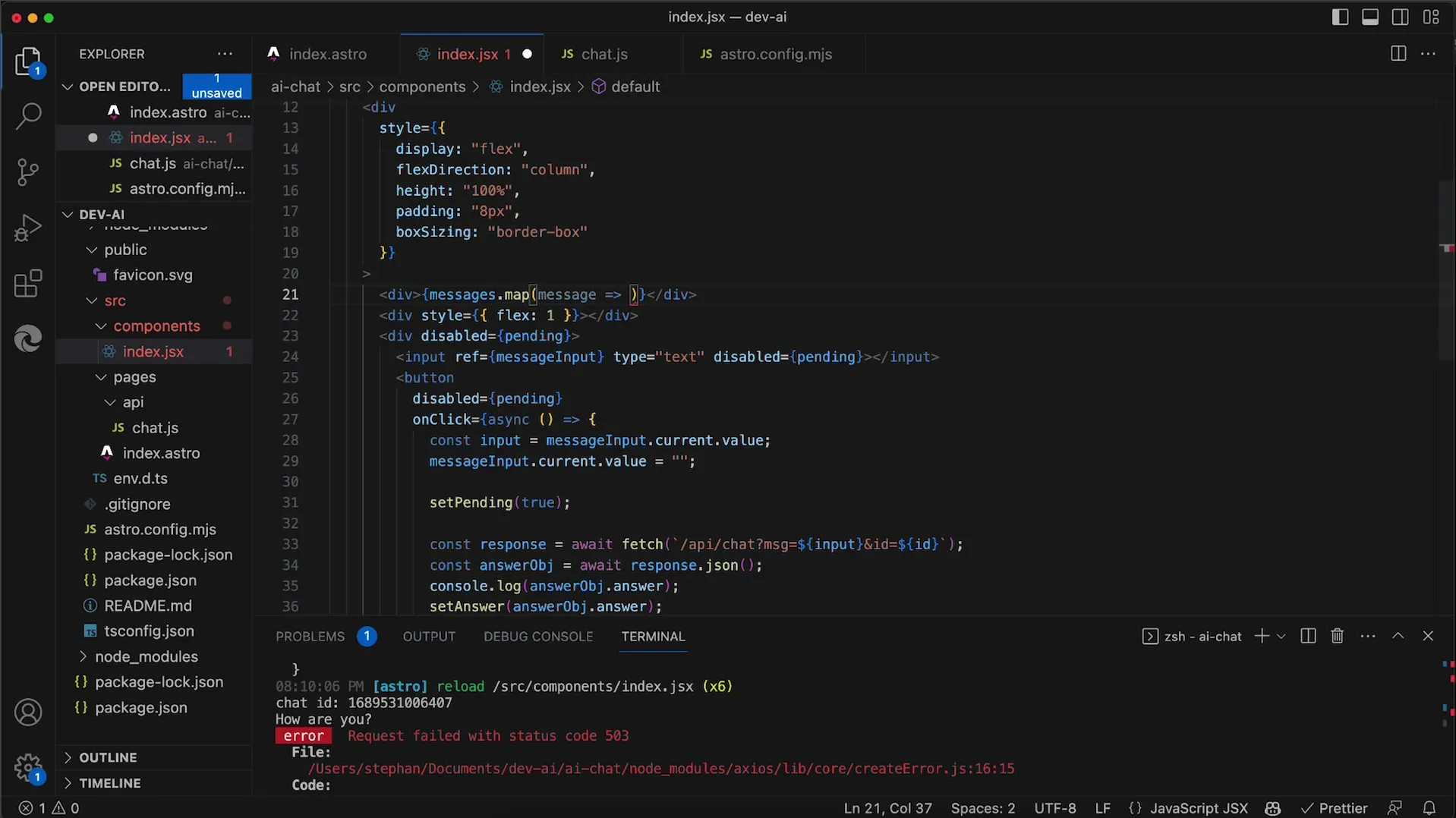
Add error handling
To create a robust user experience, you must handle errors that may occur during use, such as server errors 503. Implement an error handling routine that informs users and, if necessary, attempts to retry the request after a short wait.
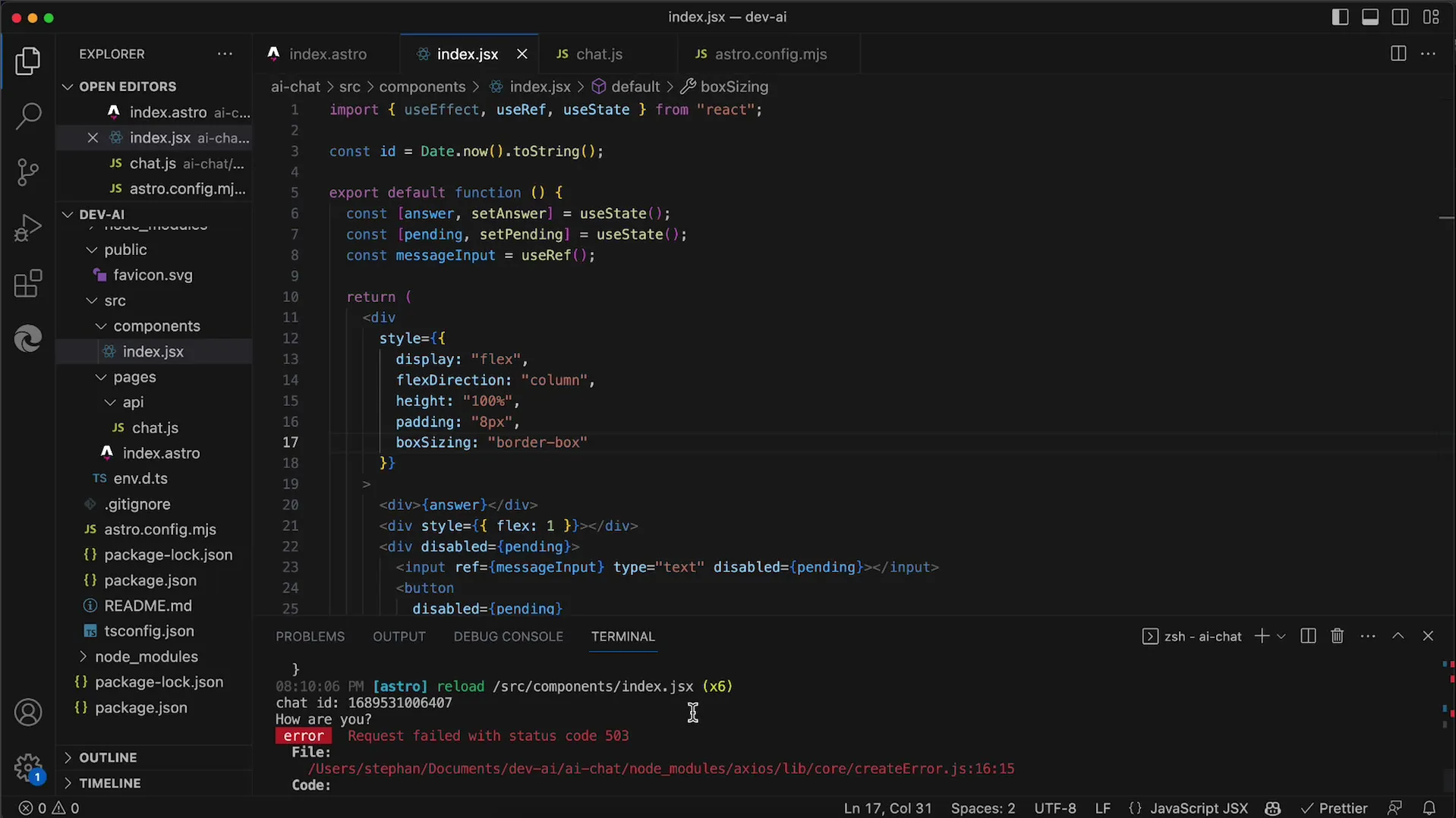
Improvements for message display
Currently, messages cannot be easily distinguished. It would be helpful to present each message with an indication of whether it is from the user or from the AI. Consider how you can adjust the display to create clear distinctions between user and AI messages.
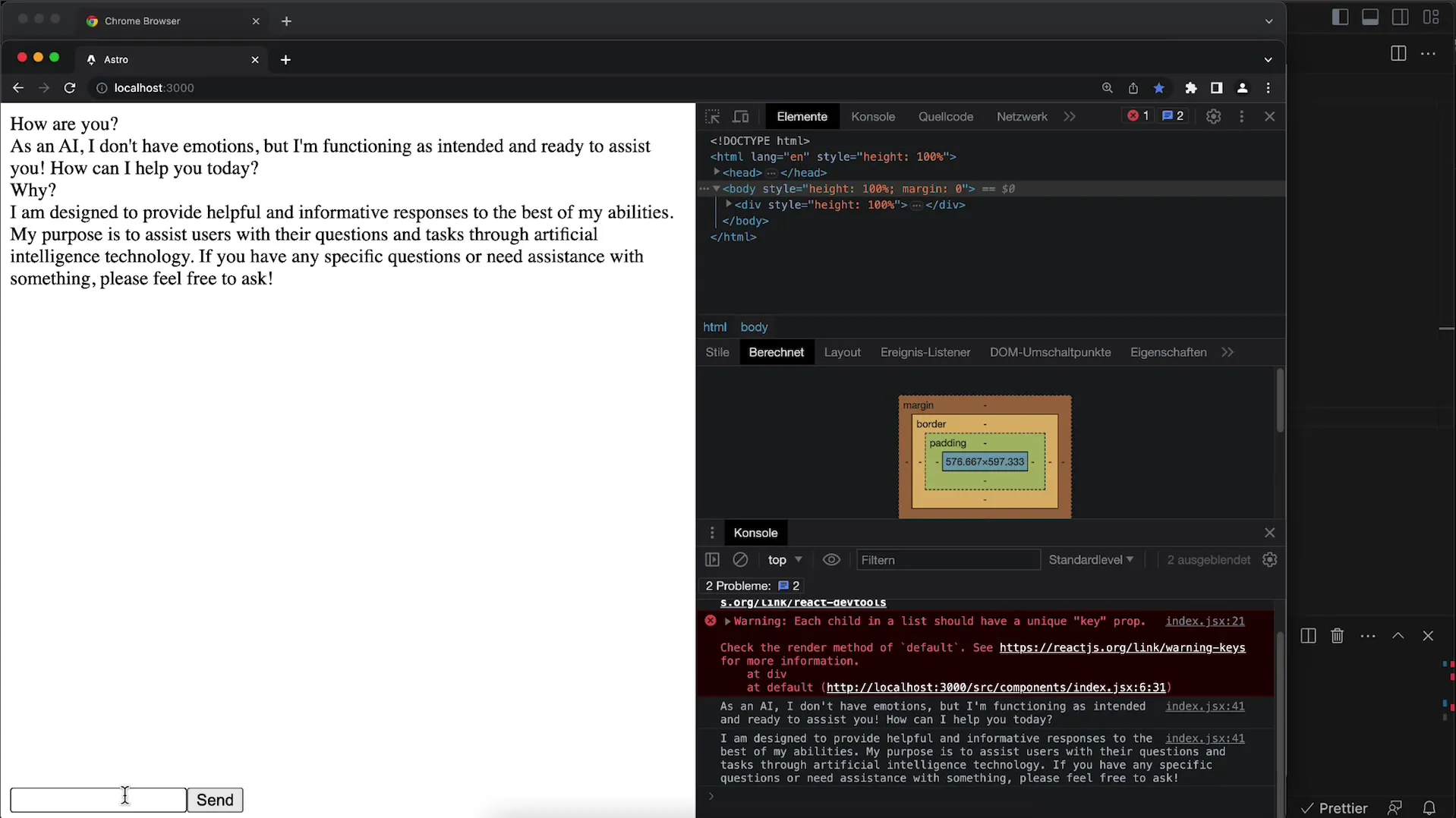
Summary
In this tutorial, you have learned how to significantly improve the user experience of your chat application with the OpenAI API through targeted UI adjustments. From disabling input fields during API responses to implementing a complete chat history - these tips will help take your application to the next level.
Frequently Asked Questions
How can I disable the input fields during the response generation?Use a state management with useState to set the input field and button to true during the waiting time.
How can I implement the chat history?Use an additional state to store all messages and display them using map in JSX.
Why is my layout not displaying correctly?Make sure all containers have a height of 100% and check margins for possible scrollbars.
How can I provide user feedback on errors?Implement an error handling routine that displays a clear message in case of an error and can potentially initiate a retry.