In this tutorial, you will learn how to implement a function using the OpenAI API that allows a virtual sales assistant to add a product to the shopping cart. Even though we won't be implementing a complete shopping cart, we will lay the groundwork so that you can integrate these functions yourself later on. The key lies in a functional call that allows the AI to respond correctly to customer inquiries. Let's get started!
Main Insights
- The function add product to cart is created to add a product to the shopping cart.
- You need to adjust the system prompt accordingly for the AI to use the new function.
- A check for undefined is required to ensure that the product is correctly captured.
Step-by-Step Guide
First, we will create the function that can add the product to the shopping cart. This is a function call that the AI will use when a customer requests to buy a product.
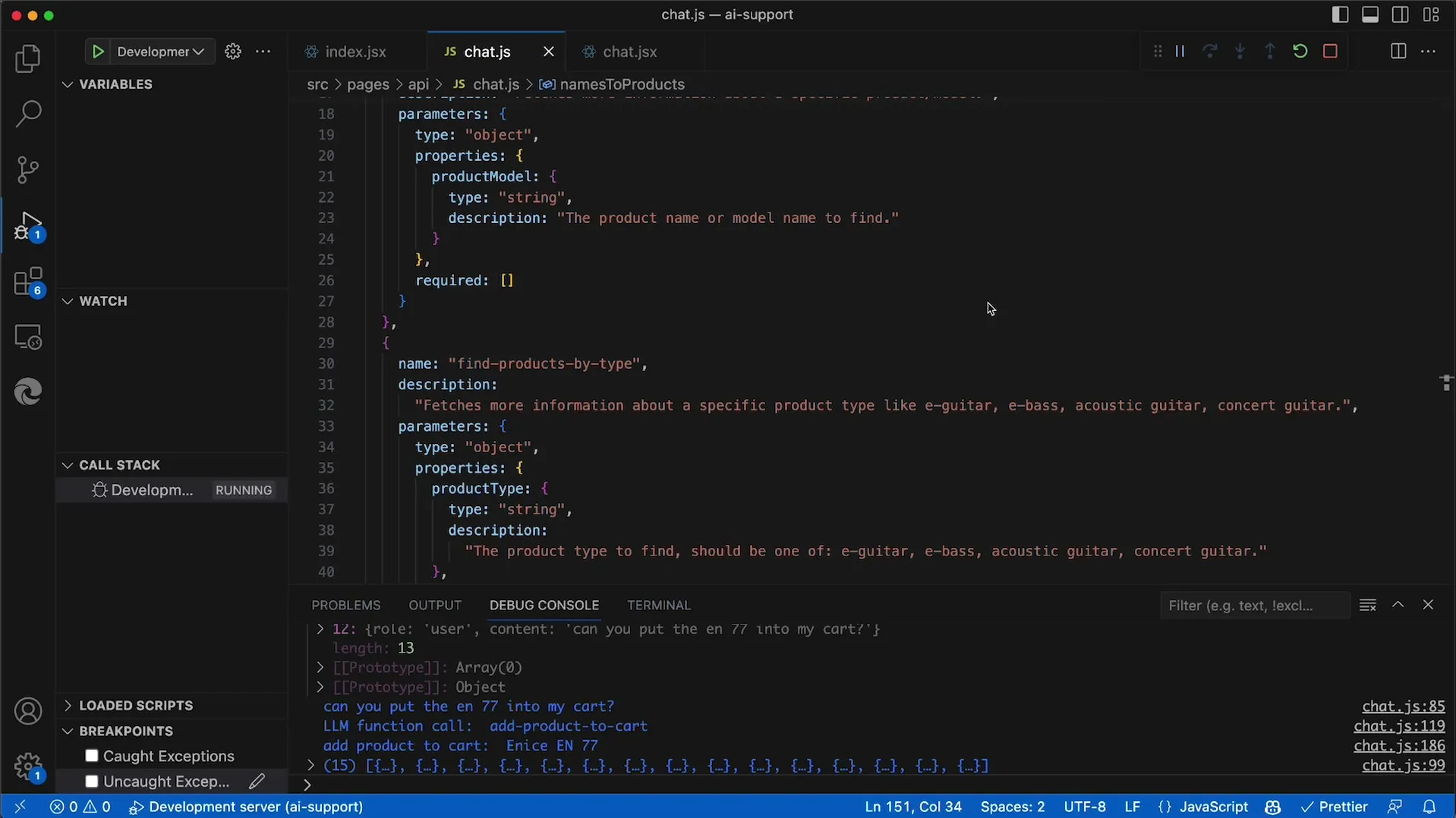
The function is called add product to cart. Its purpose is to add the product with the specified name to the customer's shopping cart.
The function has a parameter named productName, which is of type String. This parameter contains the name of the product to be added to the cart.
To get the AI to use this function, we need to adjust the system prompt. Add the sentence instructing the AI that when a customer requests to add the product to the shopping cart, it should do so accordingly. The last sentence in this context could be something like: "If the customer wants to buy a product, please add it to the shopping cart. You do not need to ask for login or payment information."
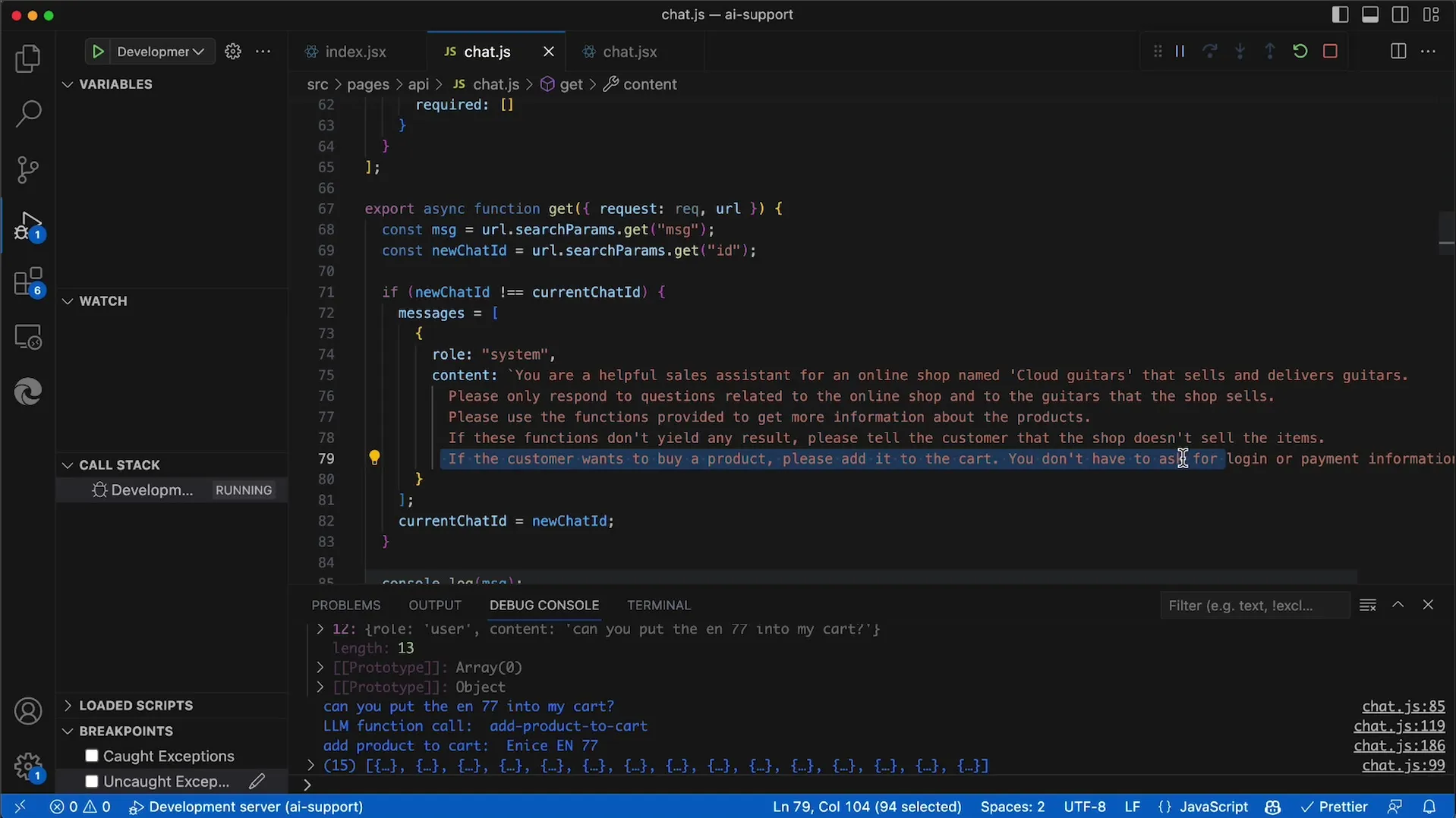
Furthermore, you need to expand the Function Call Handler to process the new function accordingly. Check if the productName from the requests actually exists.
You can use a simple if statement to determine if productName is undefined. If it is not undefined, you can create a console output confirming that the product has been added to the shopping cart.
If the product is not available, the AI will output a message stating that the product could not be found. This logic is essential for providing relevant feedback.
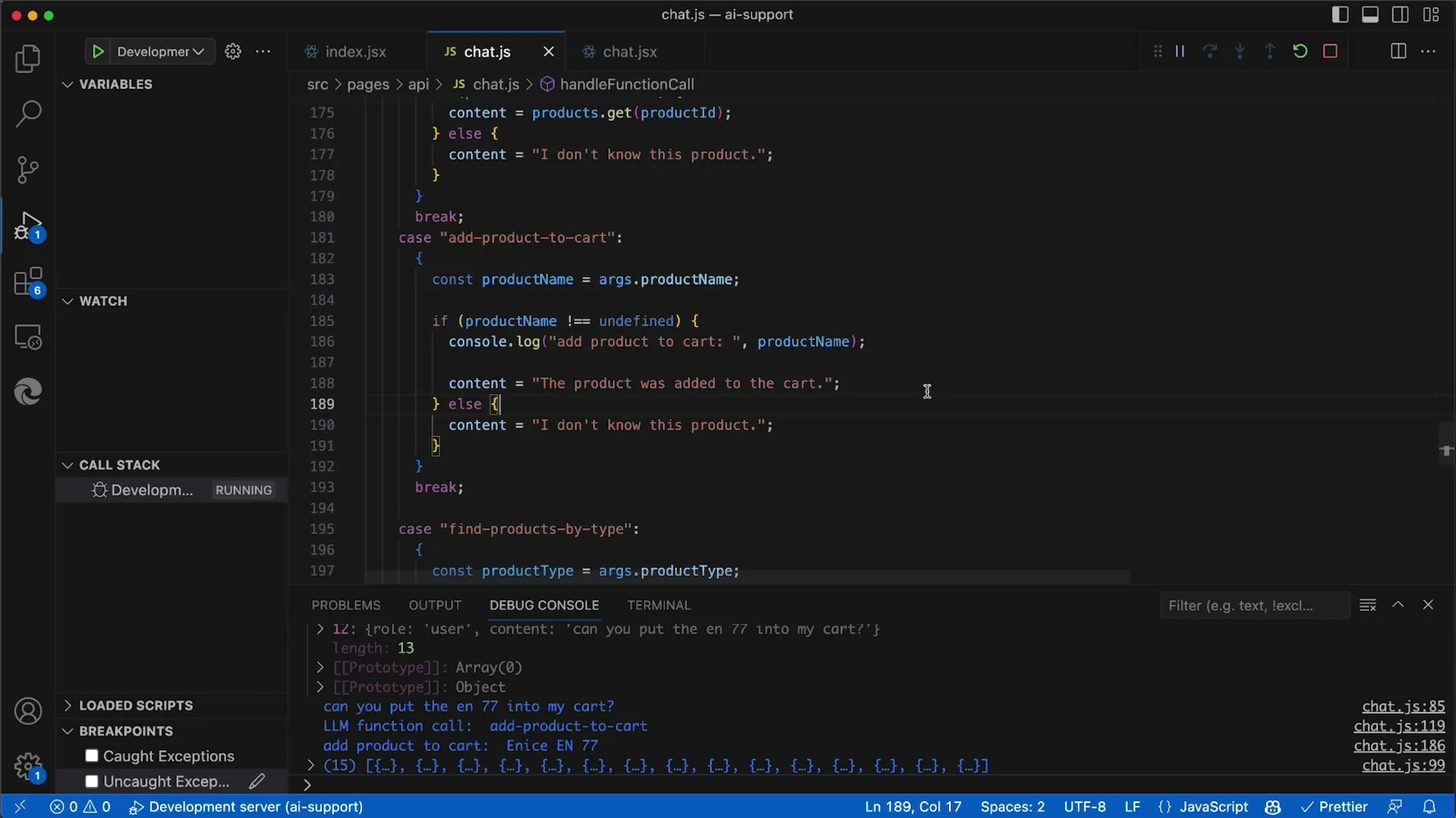
Now, let's test the new function. We make a request to check the availability of a product.
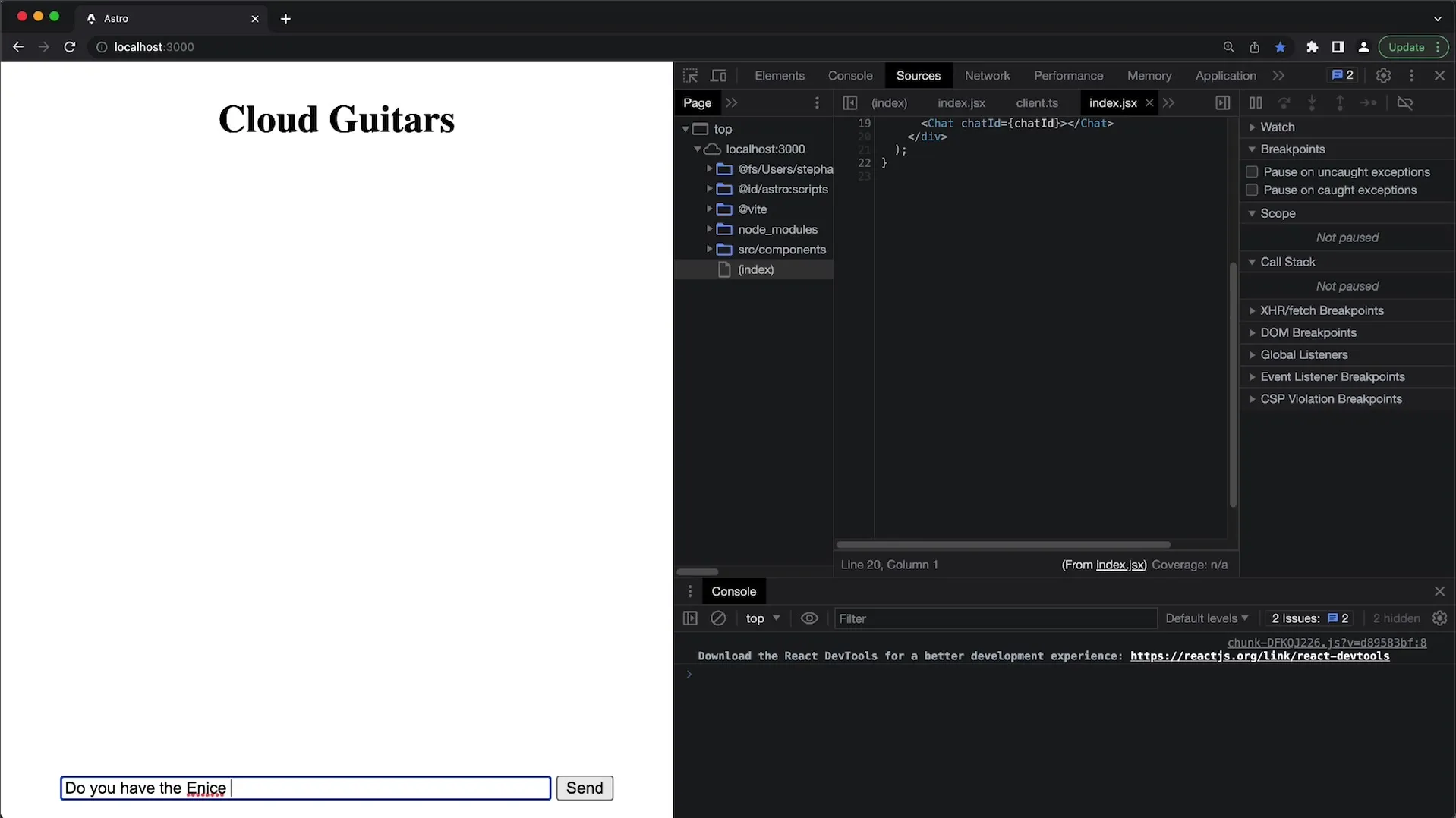
For example, you ask: "Do you have the ECE EN 77?" The AI should respond that the product is available. Now ask again: "Can you add this to my shopping cart?"
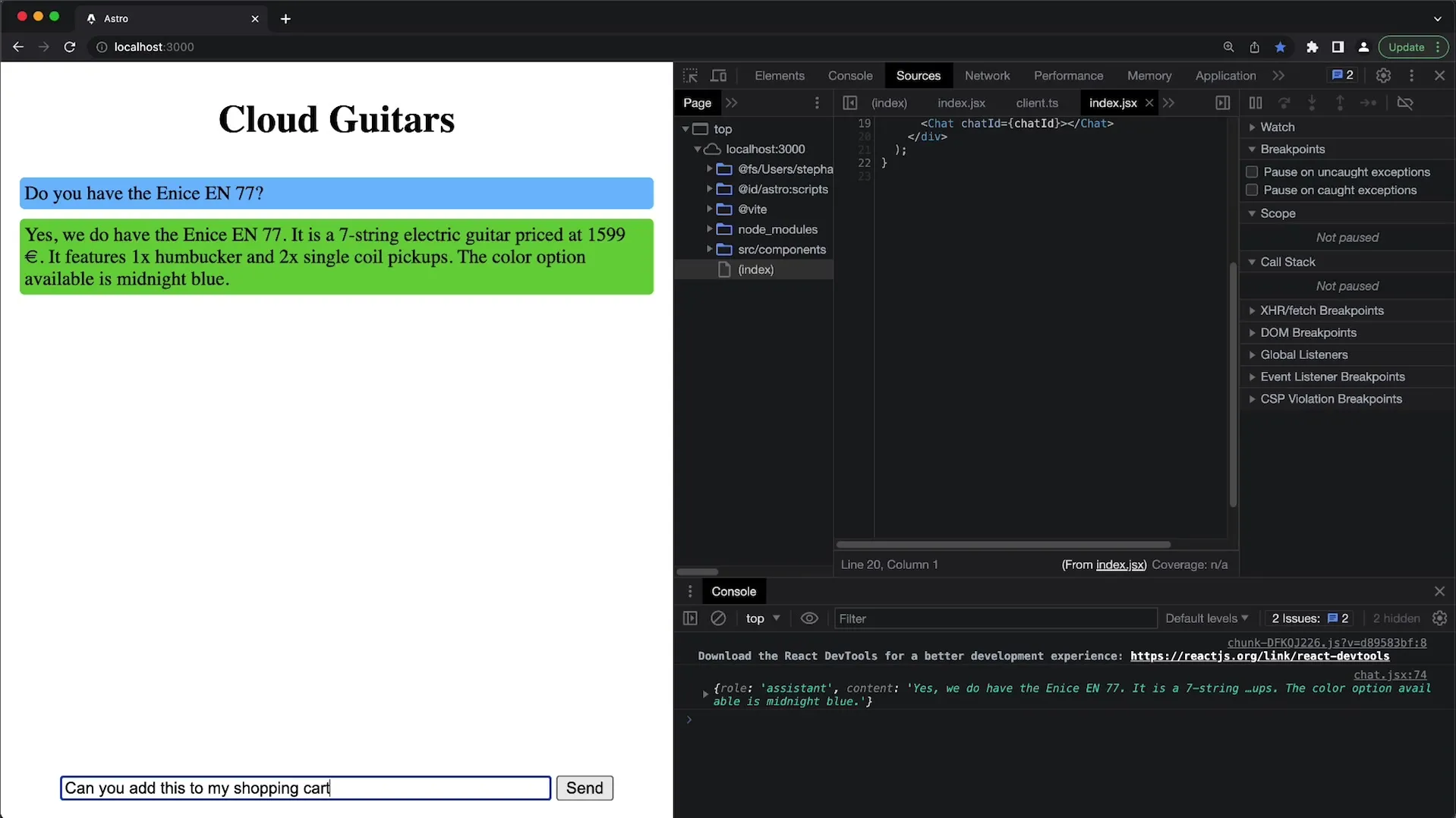
The expected response from the AI would be: "I have added the ECE EN 77 to your shopping cart." This confirms that the function was called correctly.
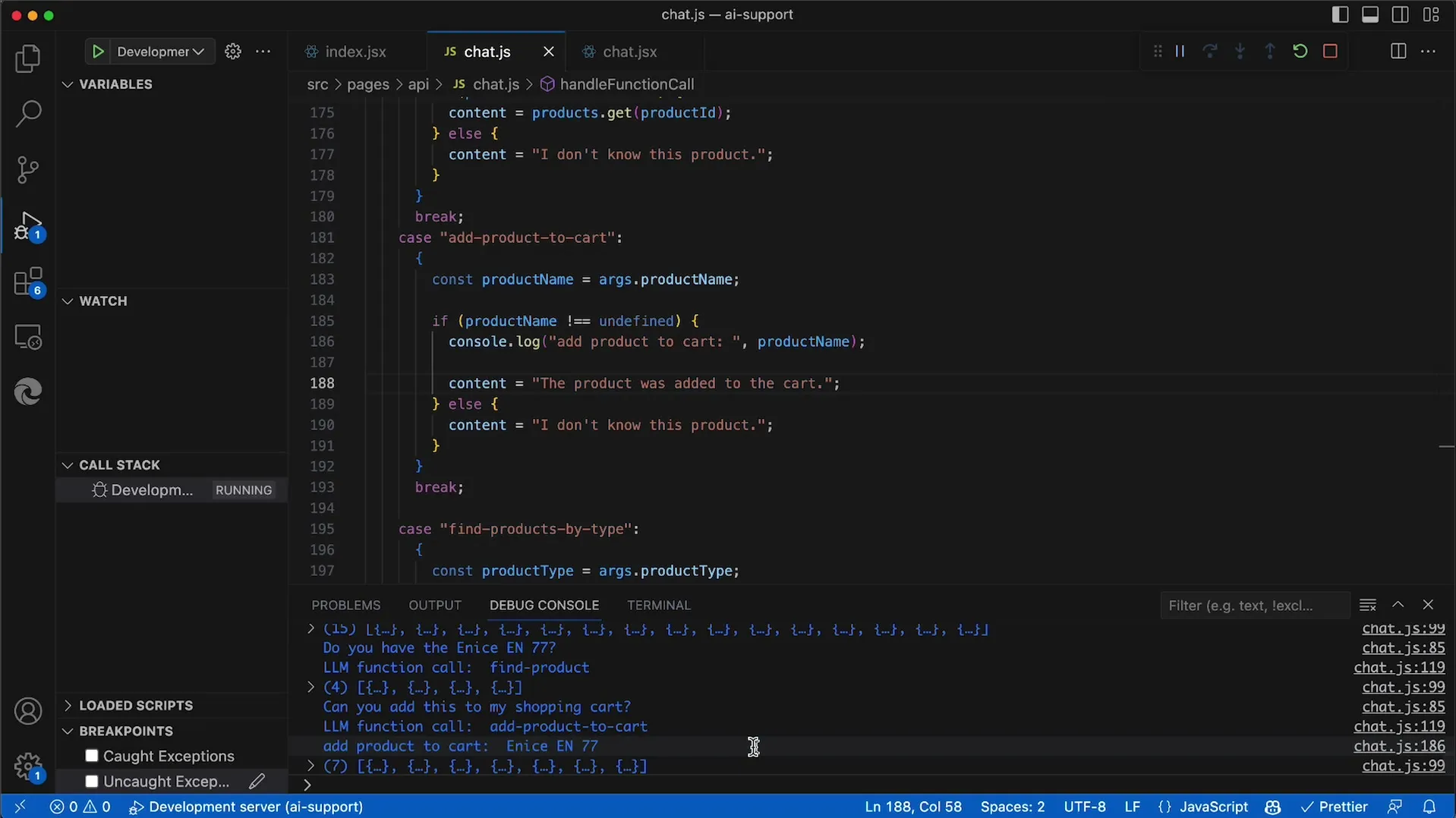
To ensure that the functionality works properly, you should also check the server outputs and make sure that the console outputs confirm that the product has been added.
Note that we are only simulating adding the product to the shopping cart; implementing this in a database is not part of this tutorial. However, you must ensure this in your application.
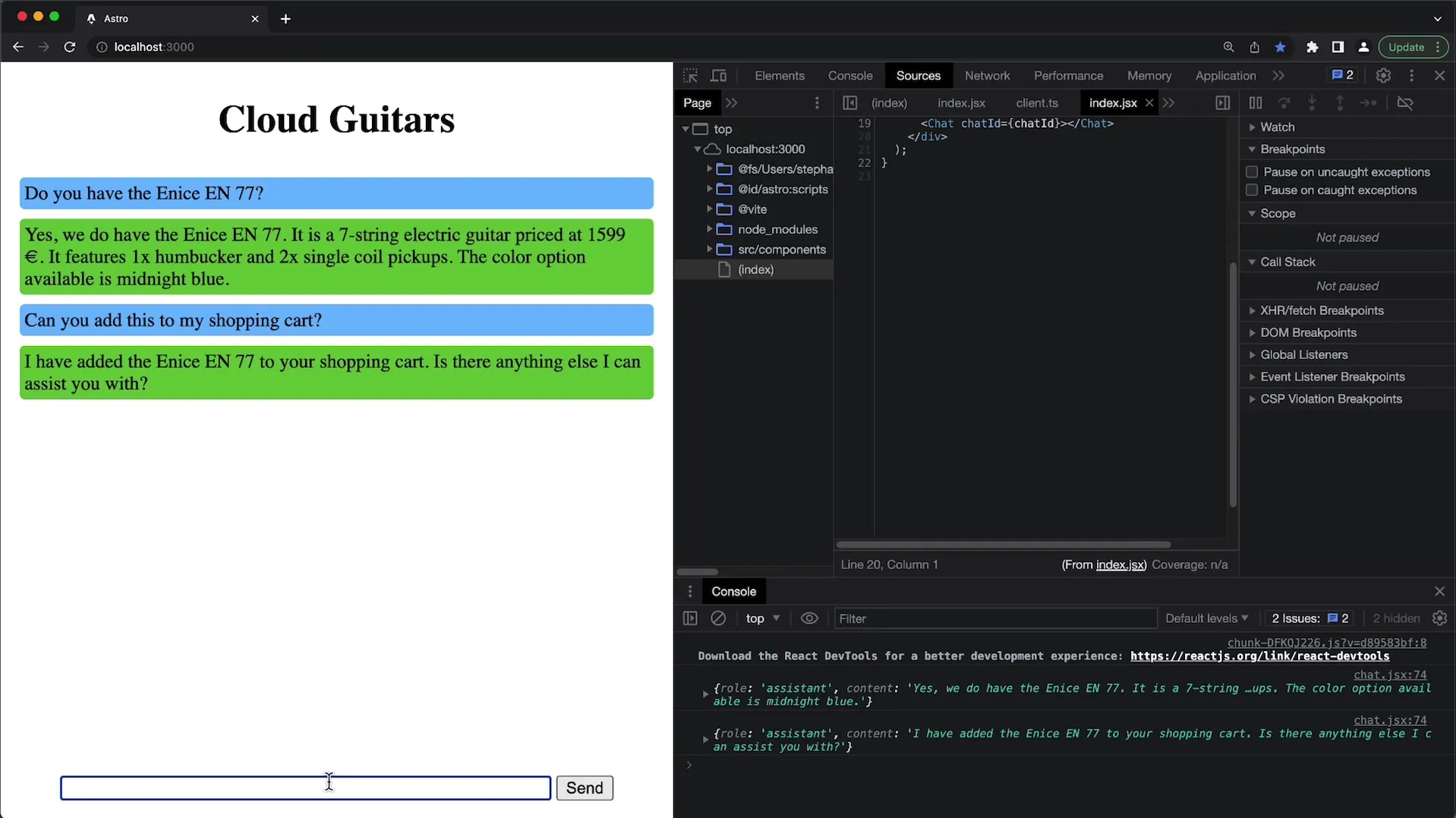
Another important topic is the search function within the system. We have found that the search may not be robust enough as it requires exact matches. Therefore, you should consider how to implement a fuzzy search to also recognize variants or slightly different search queries.
Apply by varying the requests and checking if the system also responds to different inputs. This way, you can ensure that you can further improve the functionality.
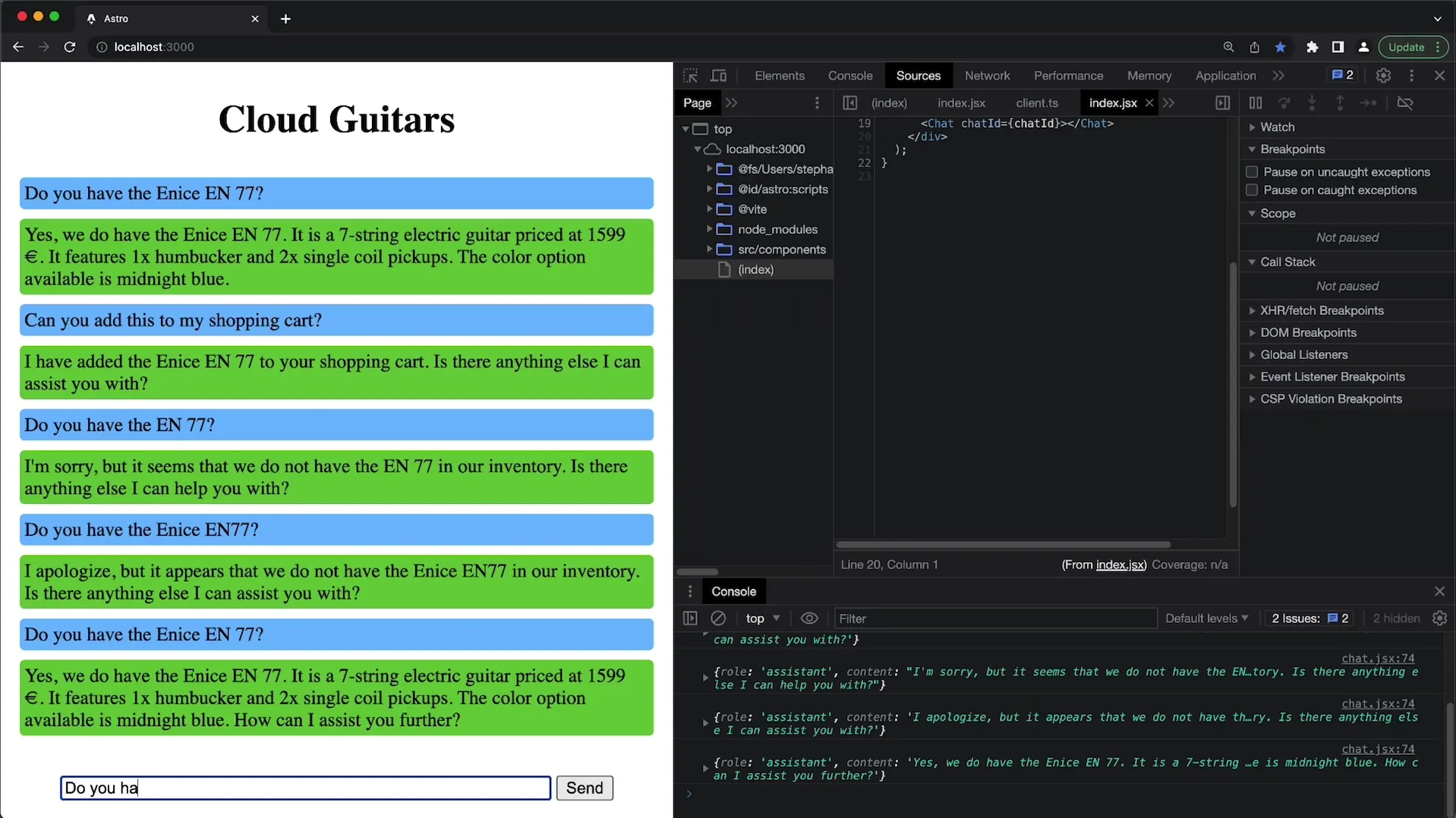
This implementation can always be adjusted and expanded. In the next video, we will focus on how a better search function can be implemented to optimize customer inquiries.
Summary
In this guide, you have learned how to implement a function for integrating a product into the shopping cart via the OpenAI API. You have gone through important steps and adjustments to ensure that the AI works optimally and responds to customer inquiries.
Frequently Asked Questions
How do I implement the function add product to cart?You create the function with the parameter productName and adjust the system prompt.
Do I need a database for the shopping cart?Yes, in order to permanently store the products, a database connection should be made.
How can I optimize the search function?Implement a fuzzy search to also recognize similar products.