If you work with CSS, you know the challenge of applying the same properties to different selectors. This is where Sass comes into play, allowing you to use the @extend function to make this task significantly easier and more maintainable. This guide provides you with a detailed overview of how @extend works and how you can effectively use it in your projects.
Key insights
- The @extend function allows you to inherit CSS properties from one selector to another.
- This makes the CSS code cleaner and easier to maintain.
- With @extend, you can avoid redundant code and develop a more modular CSS setup.
Step-by-Step Guide
Step 1: Introduction to Inheritance
Imagine you have various CSS classes that require similar styles. For example, let's say you have the.alert class used for warning messages, the.message class, and an ID #warning. You now want to apply the same style to all these selectors. A traditional CSS syntax might look like this:
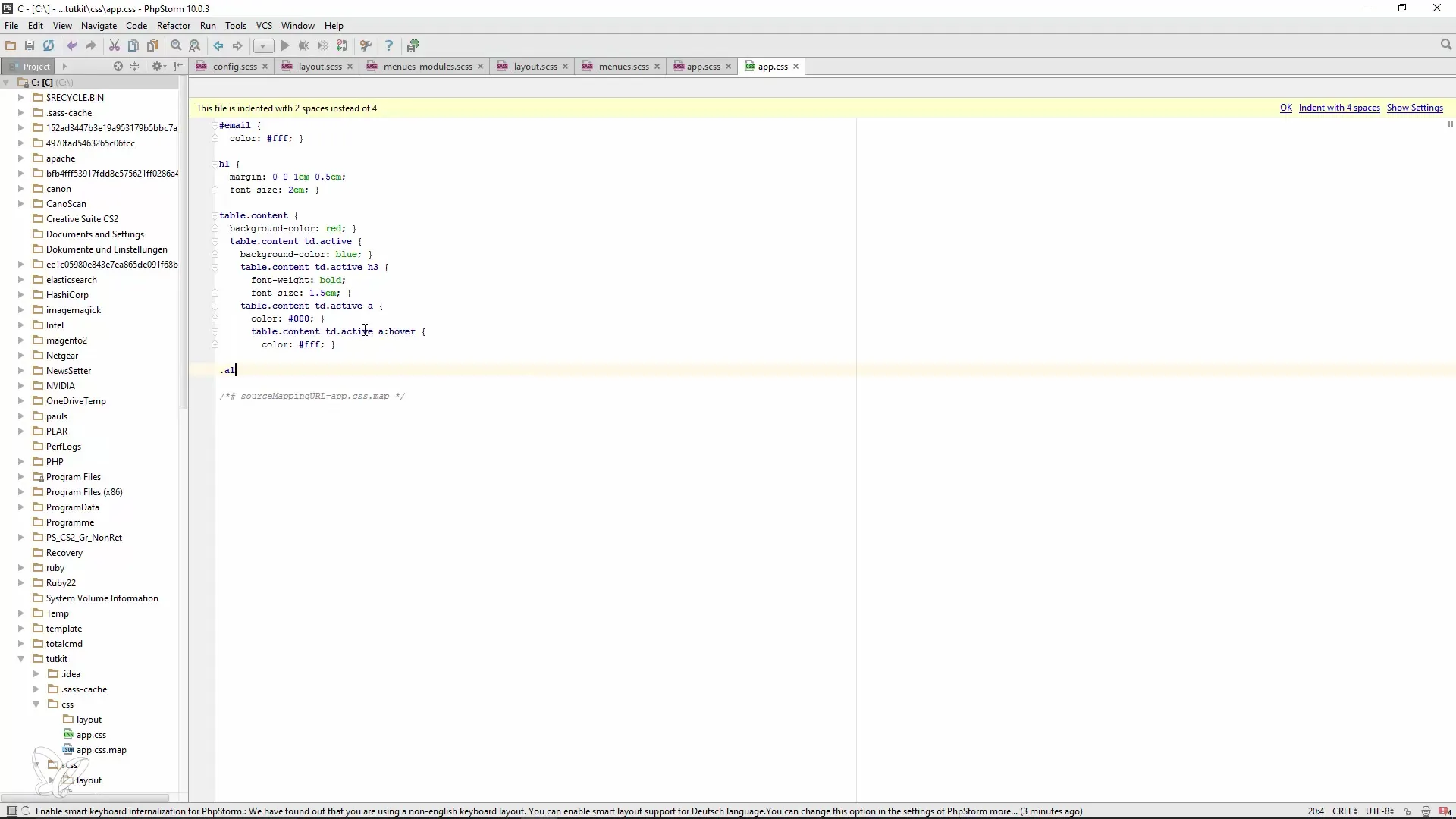
Here you define the properties of color and padding directly in your selectors. This works, but it quickly becomes cluttered, especially in large projects.
Step 2: The Problems of Classic CSS
Imagine your CSS code grows and encompasses hundreds to thousands of lines. If you need to make a change to one of these classes later, it becomes time-consuming and error-prone to search through all the CSS code and make changes to all affected selectors.
The most common approach is to override CSS properties, which bloats the code even more and causes increasing complexity. At this point, the @extend function gains its importance.
Step 3: Introducing the @extend Function
With the @extend function, you can solve the problem described above by inheriting properties from one selector to another. This results in your CSS remaining much more compact and understandable.
Imagine you are working in a modular system where you create different modules, such as menus and their properties. Here, you define the basic properties once and then inherit them to other modules.
Step 4: Applying the @extend Function in Code
Let’s start writing your CSS code. First, define the basic properties for the.alert selector.
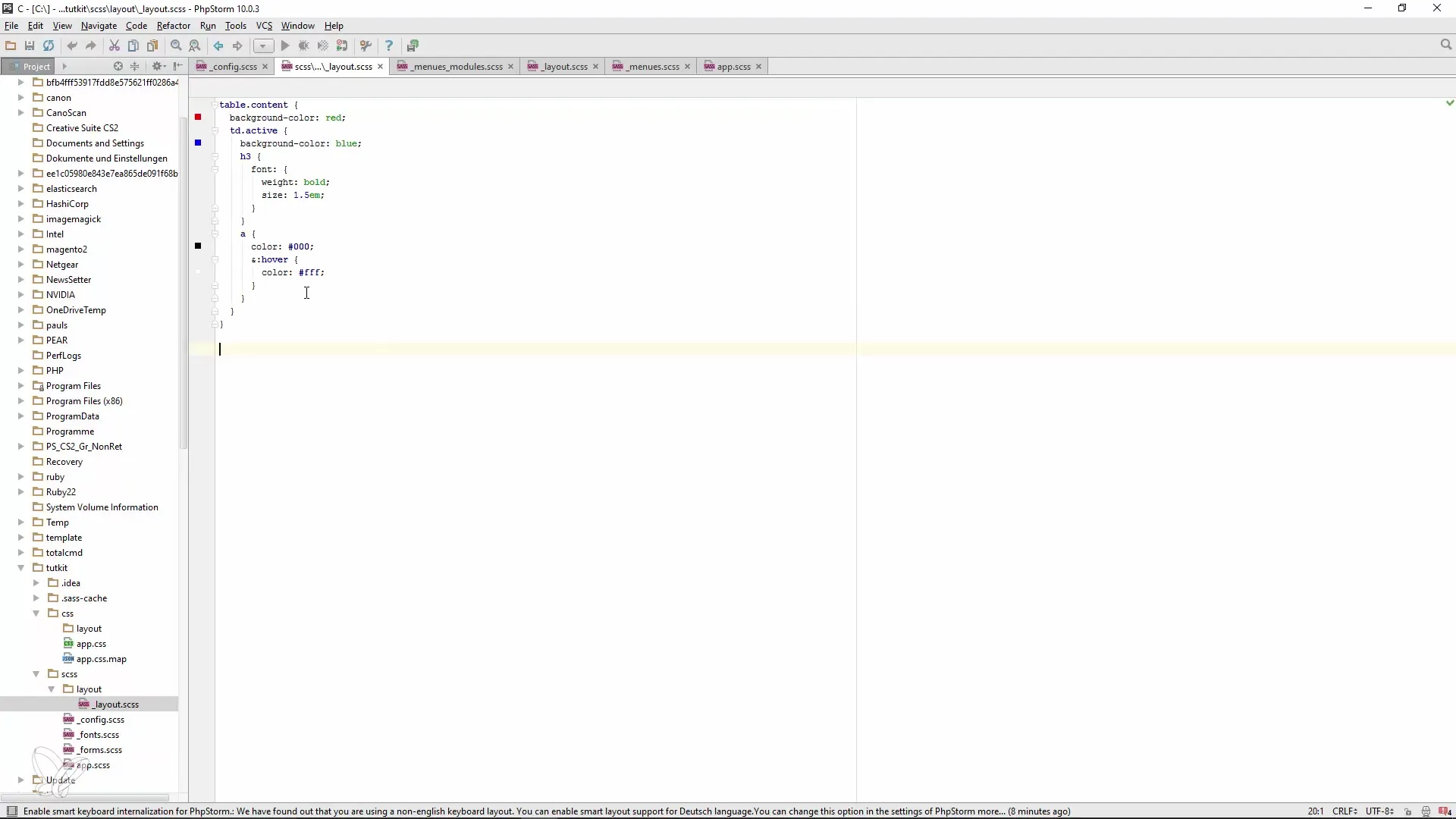
Once you have done that, you can use the @extend function to apply these properties in other selectors.
Here you create the.message class and use the @extend function to inherit the properties from.alert. This is done as simply as:
Step 5: Making Efficient Changes
Now you have the ability to modify properties without having to sift through your entire code. If, for example, the message should require different padding, you can easily adjust it without affecting other selectors.
This not only gives you more control over the CSS code but also makes it easier to make changes to style properties.
Step 6: Reviewing the Generated CSS Code
After implementing the @extend function, it is sensible to review the generated CSS code. You should ensure that everything works as desired. You can do this by looking at the CSS file generated by Sass.
You will see that the @extend function correctly transfers the properties and makes adjustments where necessary. This makes your code significantly more maintainable and provides better clarity.
Step 7: Creating Modular CSS Structures
An important aspect of @extend also comes into play when creating modular structures. Define basic properties, such as fonts, colors, and spacing in a separate module. You can then pass these to other selectors like h1, p, or div.
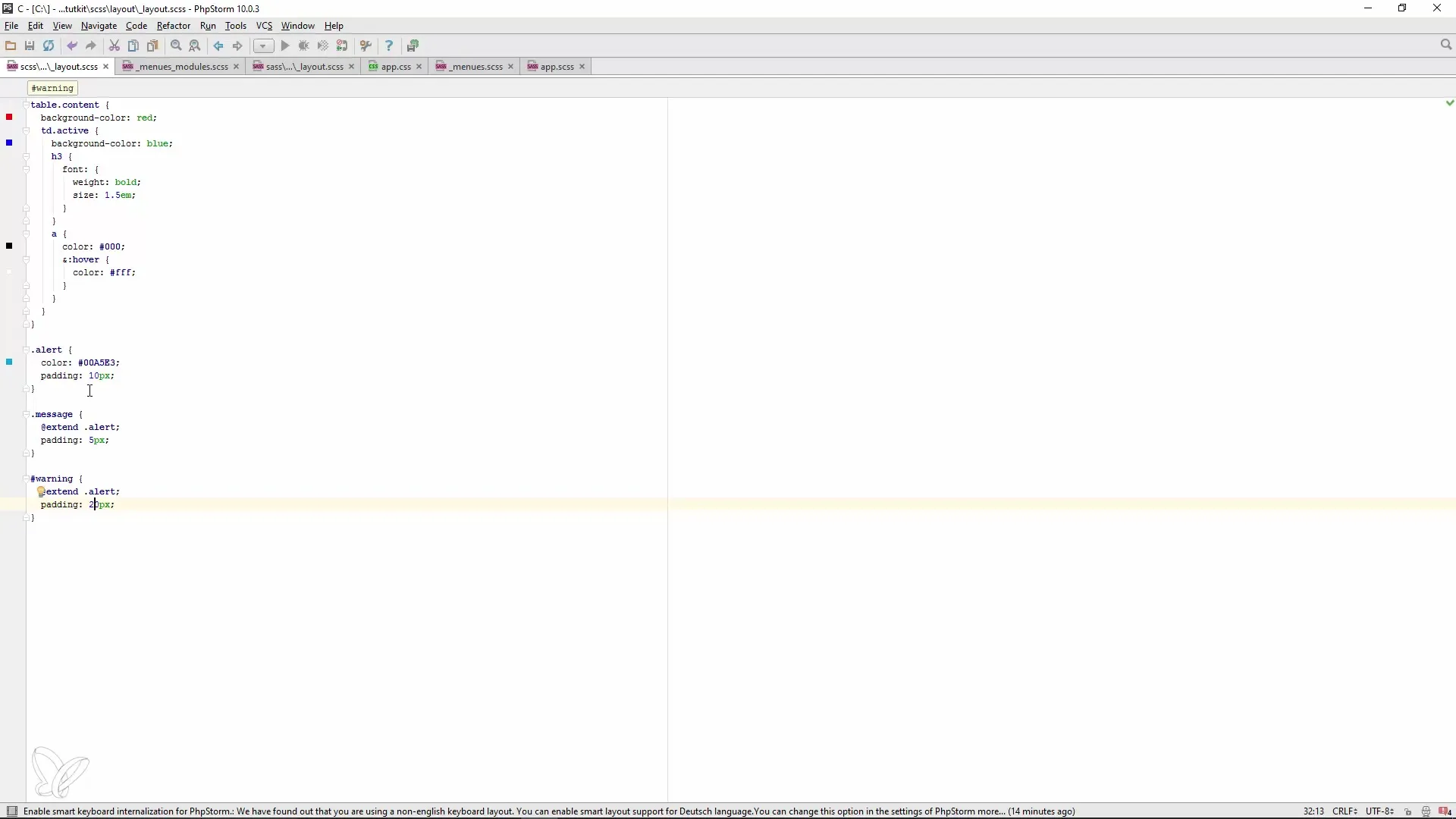
The result is a very lean and well-structured CSS code. You will notice how much easier it is to work with such modular approaches.
Summary
Using the @extend function in Sass allows you to effectively inherit CSS properties and make your code cleaner and more maintainable. You can avoid redundant code and significantly simplify the maintenance of your projects, especially with large and complex CSS structures.
Frequently Asked Questions
How does the @extend function work in Sass?With @extend, a selector can inherit CSS properties from another selector.
Why should I use @extend?It makes the code more maintainable and reduces redundancy, making CSS maintenance easier.
Are there any drawbacks to using @extend?In certain cases, it can lead to a larger CSS bundle when many modules are loaded.
What is the difference between classic CSS syntax and Sass?Sass allows you to structure and reuse code more efficiently, while CSS is simpler but does not offer the same capabilities.
How do I integrate @extend into my existing project?You can simply inherit the properties of one selector into another selector via @extend, as described in the tutorial.