Programming can sometimes be frustrating, especially when errors occur and the program stops abruptly. It is crucial to learn techniques that help you handle these errors elegantly and efficiently. A fundamental technique in Python is exception handling. With the keywords try, except, else, and finally, you can ensure that your program continues to run reliably even in the face of errors.
Key Insights
- Exception handling in Python allows you to catch errors without crashing the program.
- The keywords try, except, else, and finally have specific roles in error handling.
- With the proper application of these concepts, you can ensure that your code remains stable even under unexpected conditions.
Step-by-Step Guide to Exception Handling
Step 1: Understanding Error Generation Basics
First of all, it is important to understand the types of errors that can occur in your code. A simple example would be attempting to add a string to a number. This causes a TypeError. By experimentally generating such errors, you can get a better sense of when and where you need exception handling.
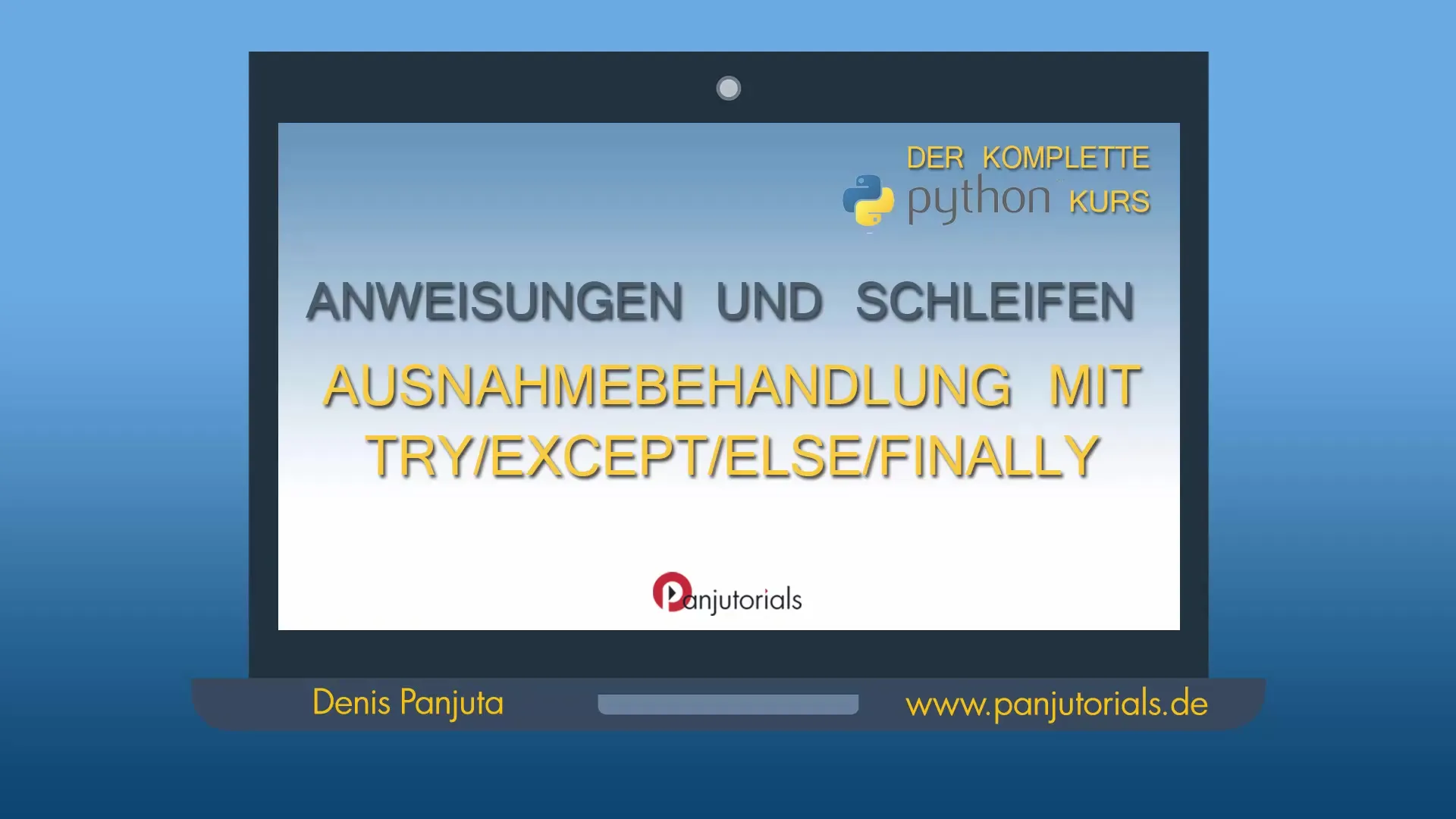
Step 2: Simple Application of Try and Except
To handle errors, you use the keywords try and except. The try block contains the code that could potentially generate an error. If an error is raised in the try block, the corresponding except block takes control. This happens without crashing the program.
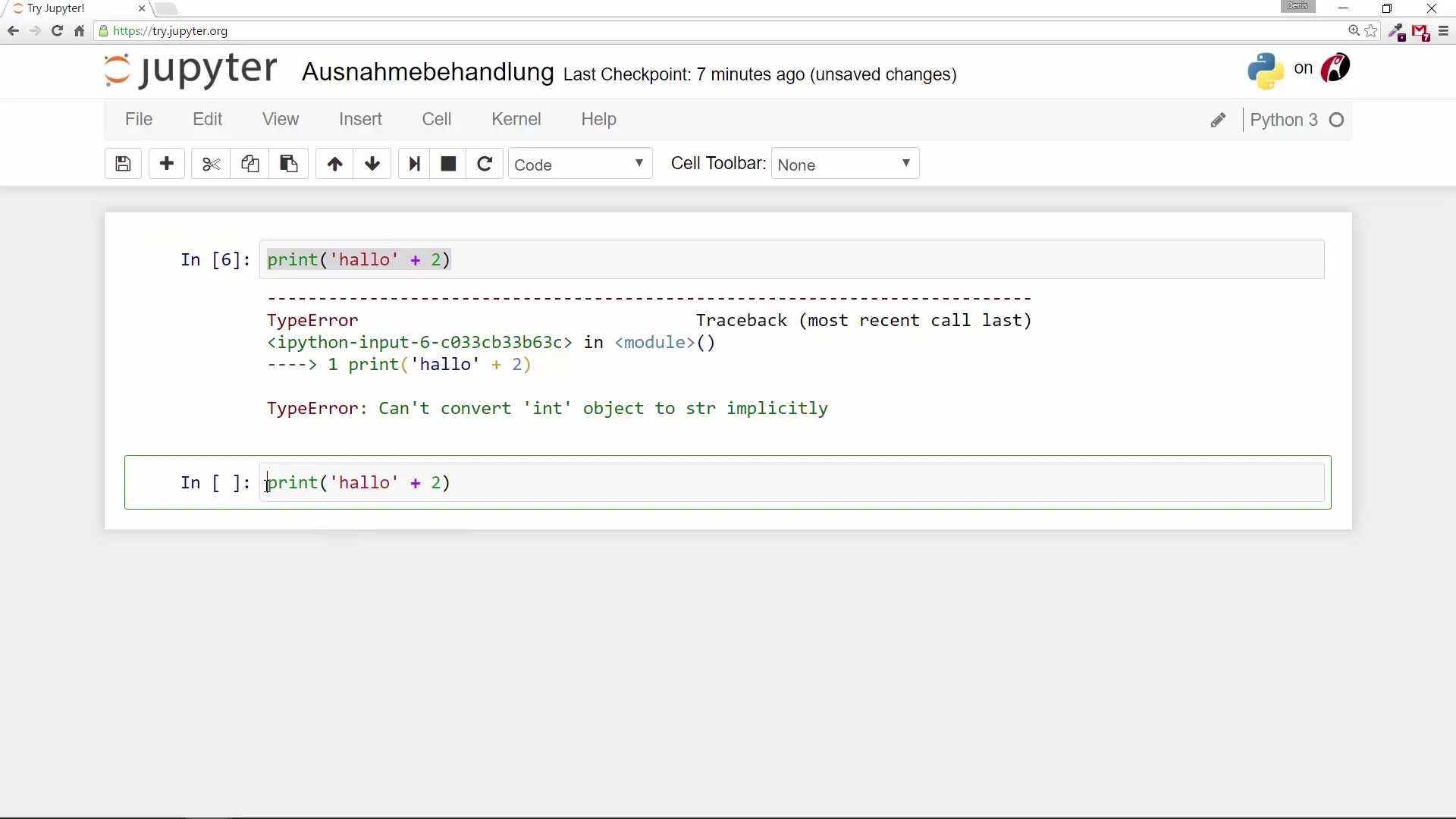
Here’s a simple example:
In this case, the error message is printed via the except block when the code is executed, instead of causing a program crash.
Step 3: Using Finally
The finally keyword is used to ensure that a certain piece of code always runs, regardless of the outcome of the try block. This can be useful for releasing resources or performing final operations that are necessary regardless of the success of the try block.
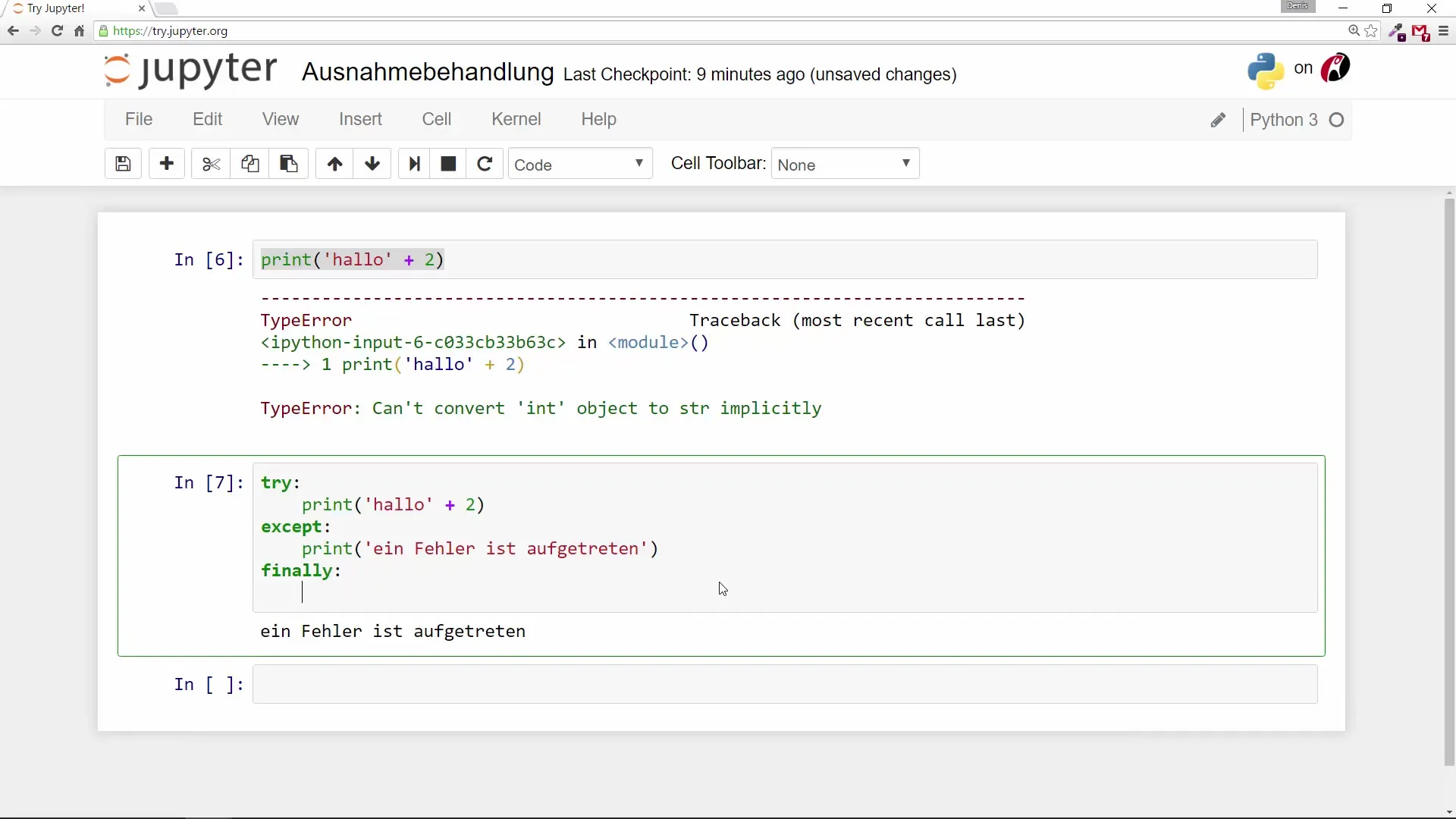
Step 4: Using Else
The else block runs when the try block executes successfully without an error. This allows you to separate code that should only run if the try block was executed successfully.
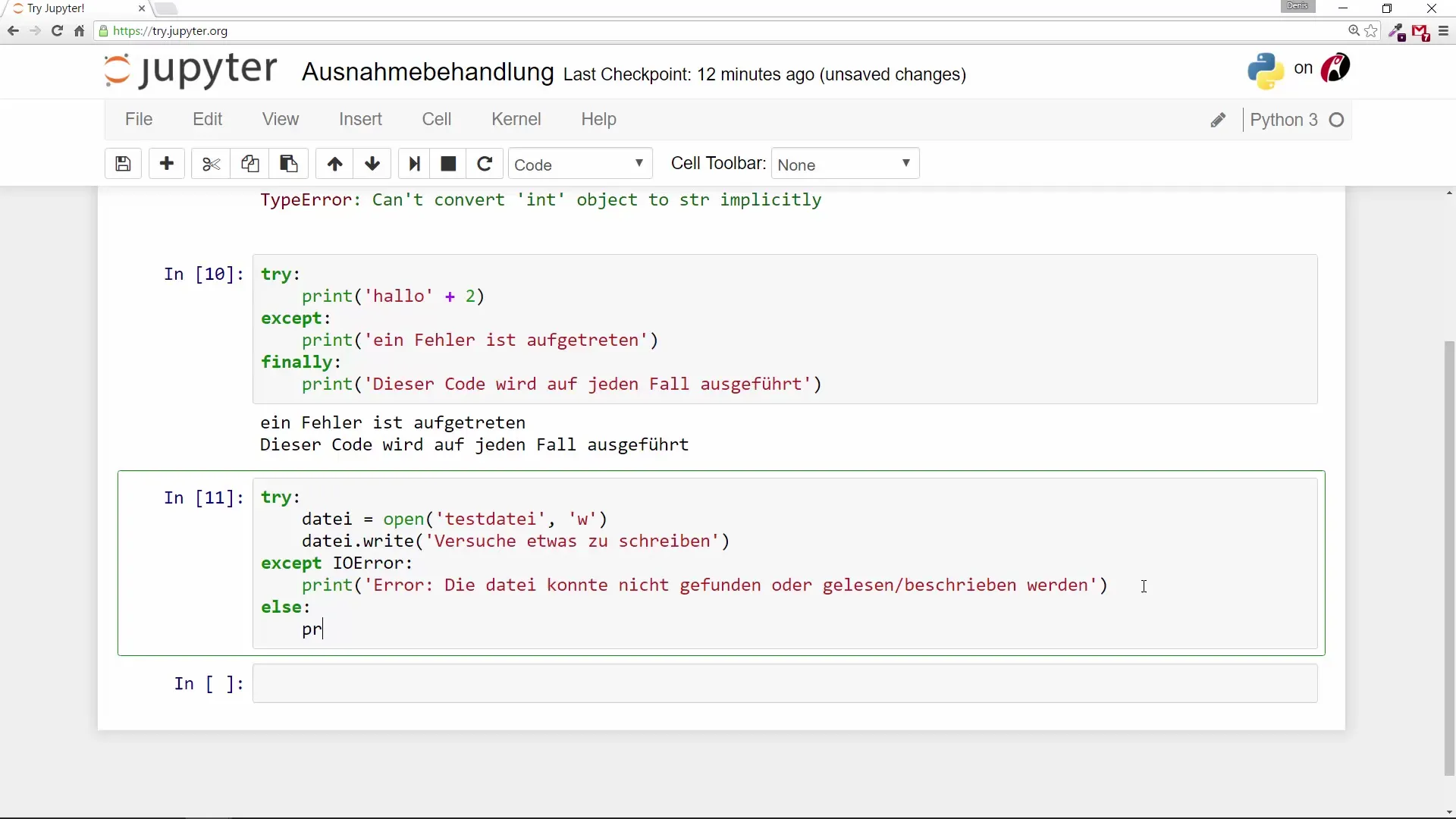
Step 5: Working with Files
A common application of exception handling is working with files. You can use try, except, else, and finally to safely open and edit files.
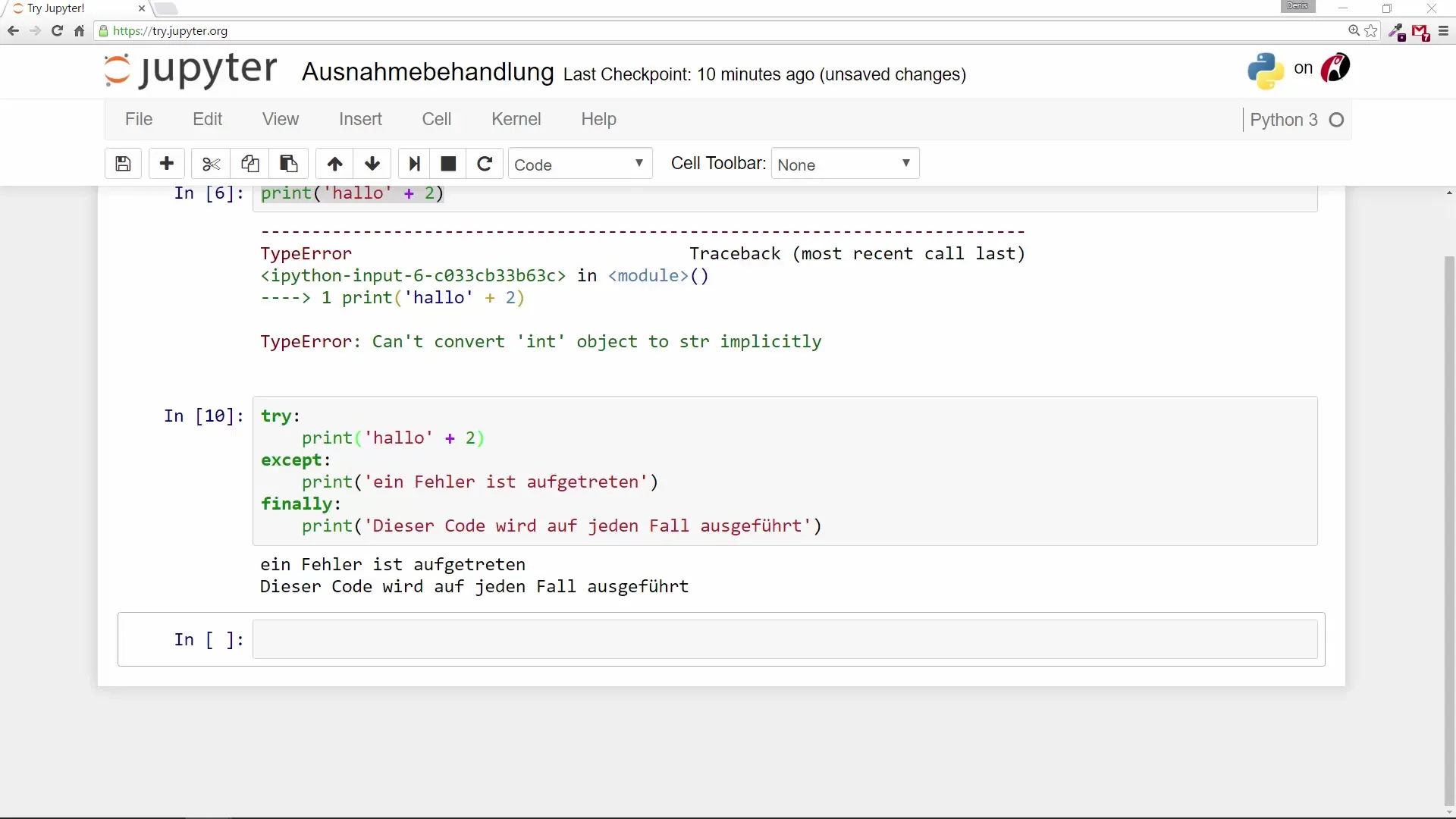
Here’s an example of how you would work with a file:
Step 6: Catching Multiple Errors
You can use multiple except blocks to specifically handle different types of errors. This gives you the ability to respond precisely to various issues.
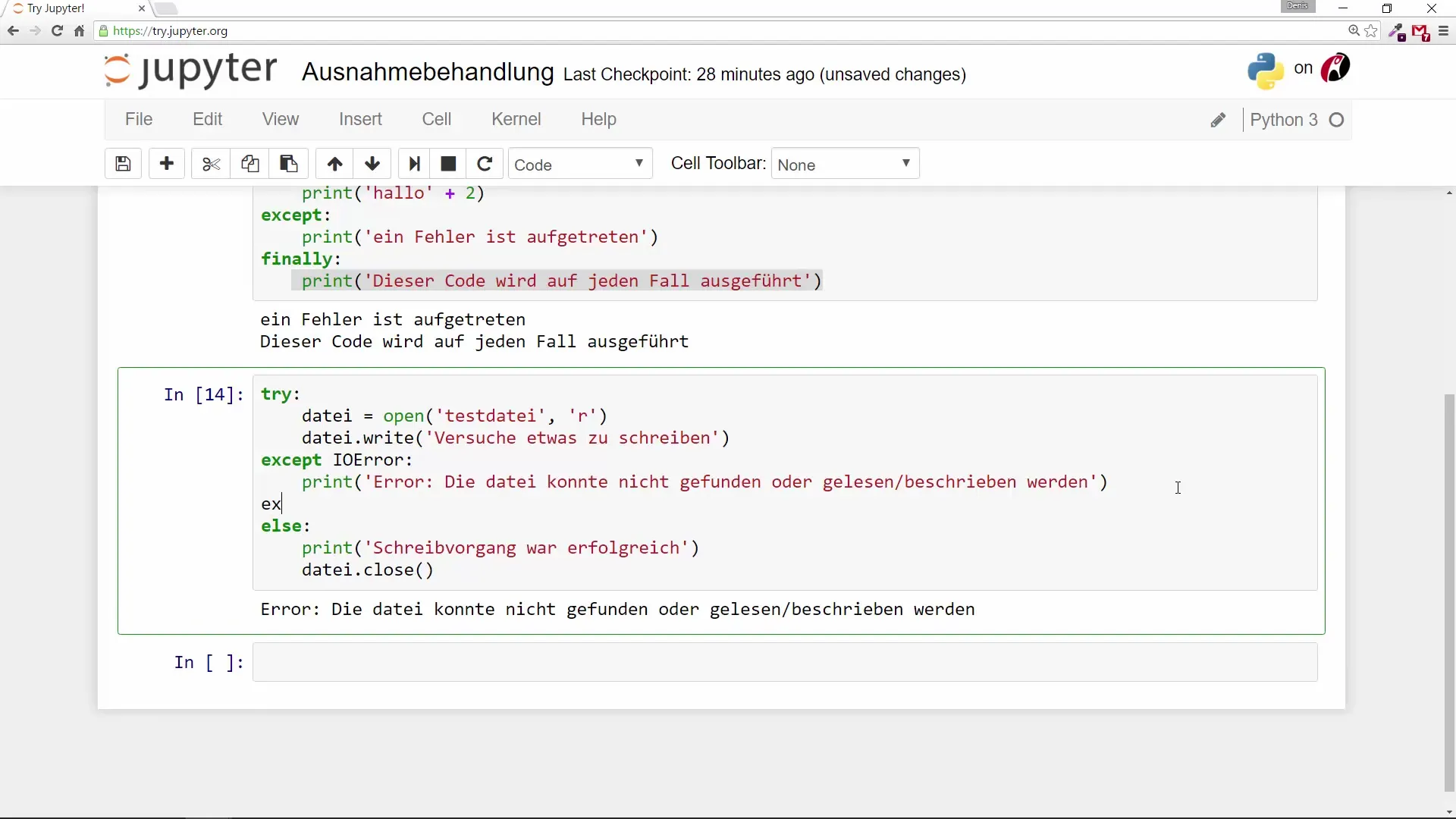
In this example, the error of division by zero is specifically addressed, and there is a general handling of type errors.
Summary
By utilizing the keywords try, except, else, and finally, you can handle errors in your Python code elegantly and effectively. These techniques ensure that your program remains stable, even when something unexpected happens. By appropriately adapting your error handling, you ensure that the user receives clear feedback and that your code runs smoothly.
Frequently Asked Questions
What does the keyword try do?try marks the block of code that is to be tested for an error.
When is the except block executed?The except block is executed when an error occurs in the try block.
What exactly happens in the finally block?The finally block always runs, regardless of the outcome of the try block, to perform cleanup actions.
When is the else block reached?The else block is executed when no error has occurred in the try block.
Can I use multiple except blocks?Yes, you can use multiple except blocks to specifically handle different types of errors.