Are you looking for a way to determine prime numbers in Python? Great! In this tutorial, I will show you how to write a simple function to display all prime numbers up to a limit entered by the user. We will use Python's enumerate function to enhance the programming fun even more.
Key takeaways
- Understand what prime numbers are and what criteria they fulfill.
- Use loops to determine prime numbers.
- Implement a user query to input the upper limit.
- Apply the enumerate function to iterate through a list of numbers and analyze their properties.
Step-by-Step Guide
Step 1: Basic Understanding of Prime Numbers
Prime numbers are numbers that are only divisible by 1 and themselves. The numbers 0 and 1 do not count and therefore should not be outputted by your program. Before you proceed, make sure that you understand this concept.
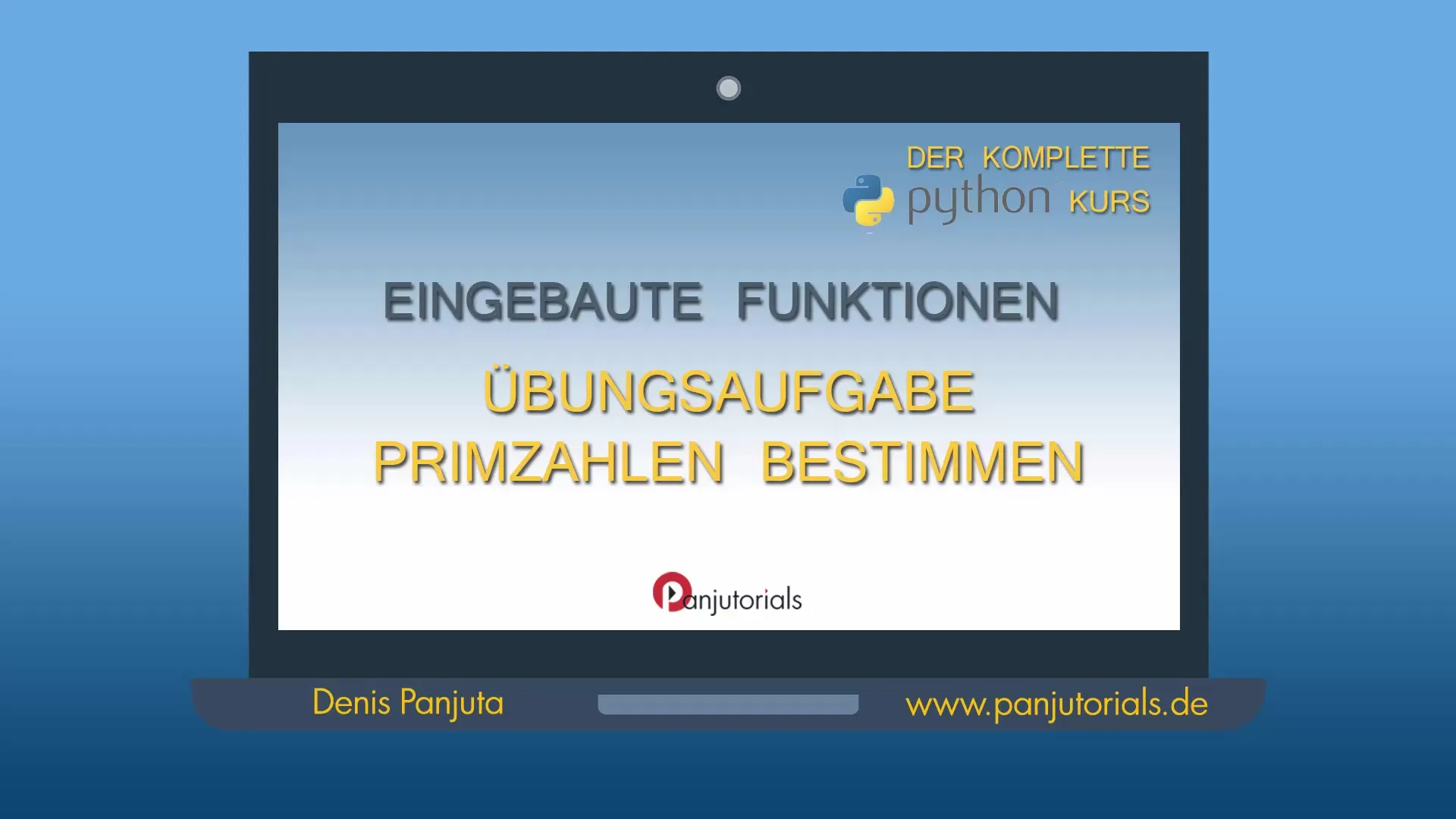
Step 2: Setting Up the Program
Start by creating a new Python file. Name it something like primzahlen.py and open it in your preferred code editor. You will write a function that asks the user for an upper limit.
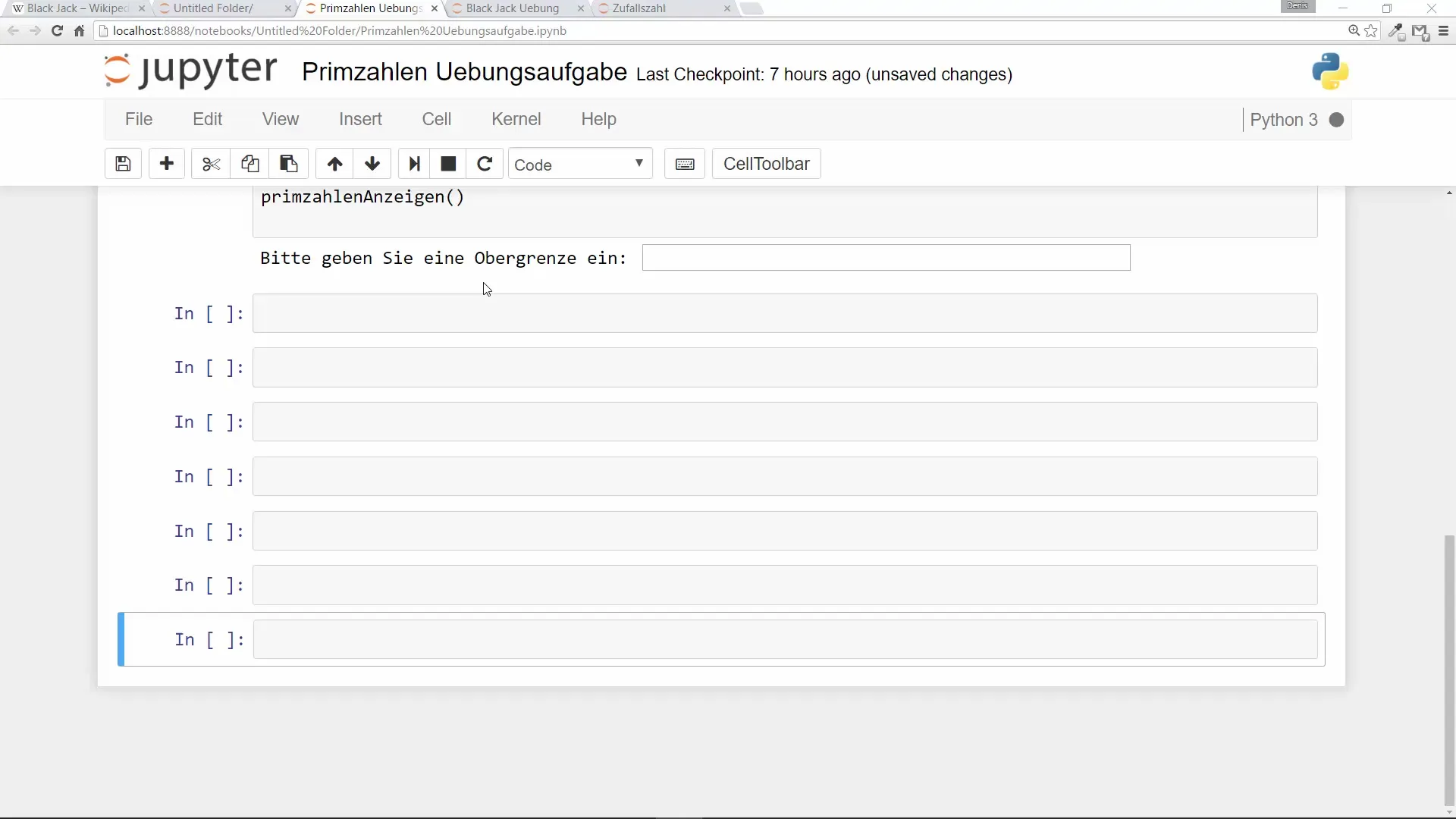
Step 3: Define the Function
Define a function named primzahlen_anzeigen. This function should prompt for user input to set the upper limit. Use a while loop to ensure that the user enters a valid integer.
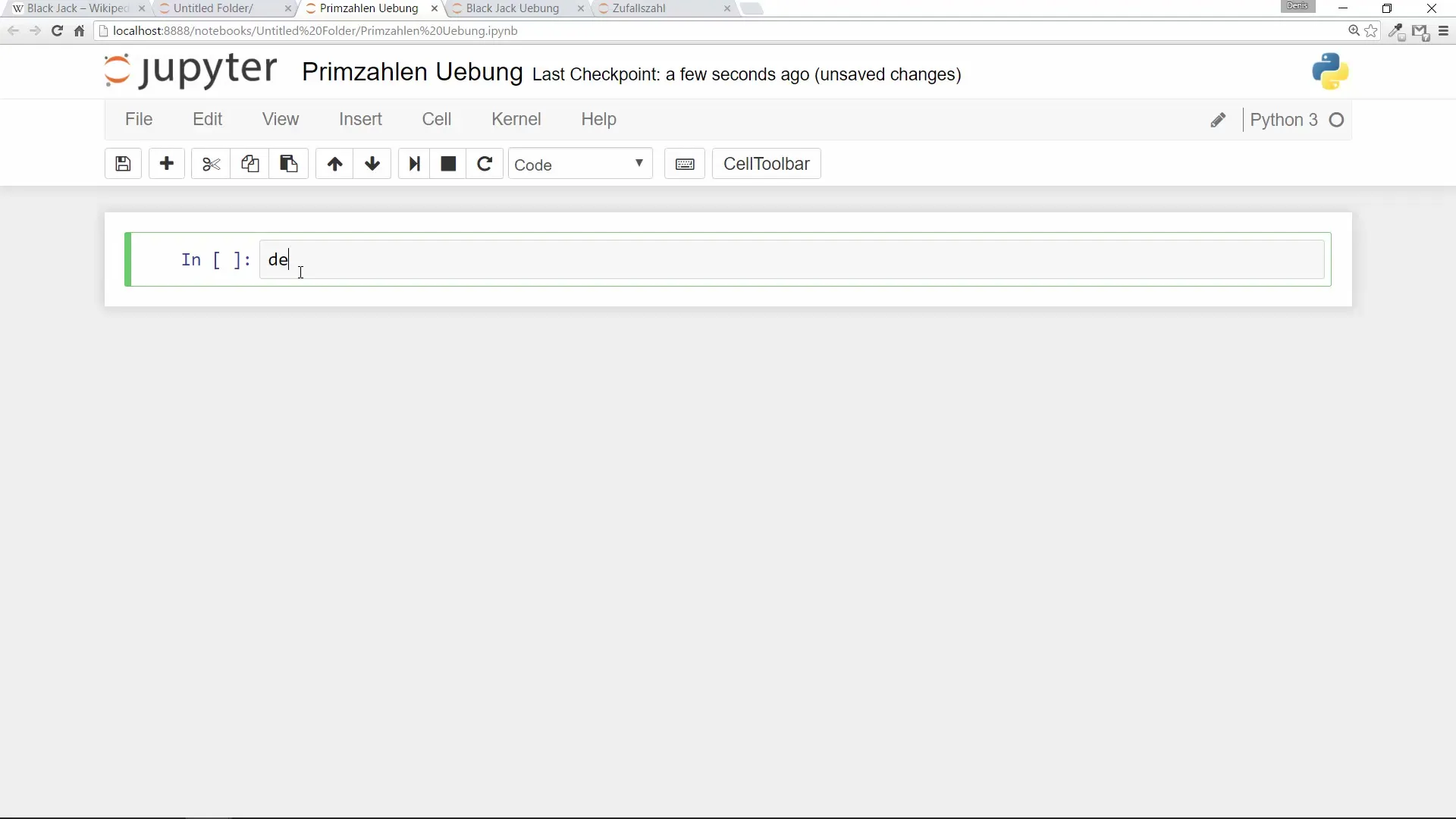
Step 4: Implement Error Handling
Within the while loop, add a try-except block. This block ensures that the user can only make inputs that are actually valid. If an invalid input is made, display an error message informing the user to enter a valid number.
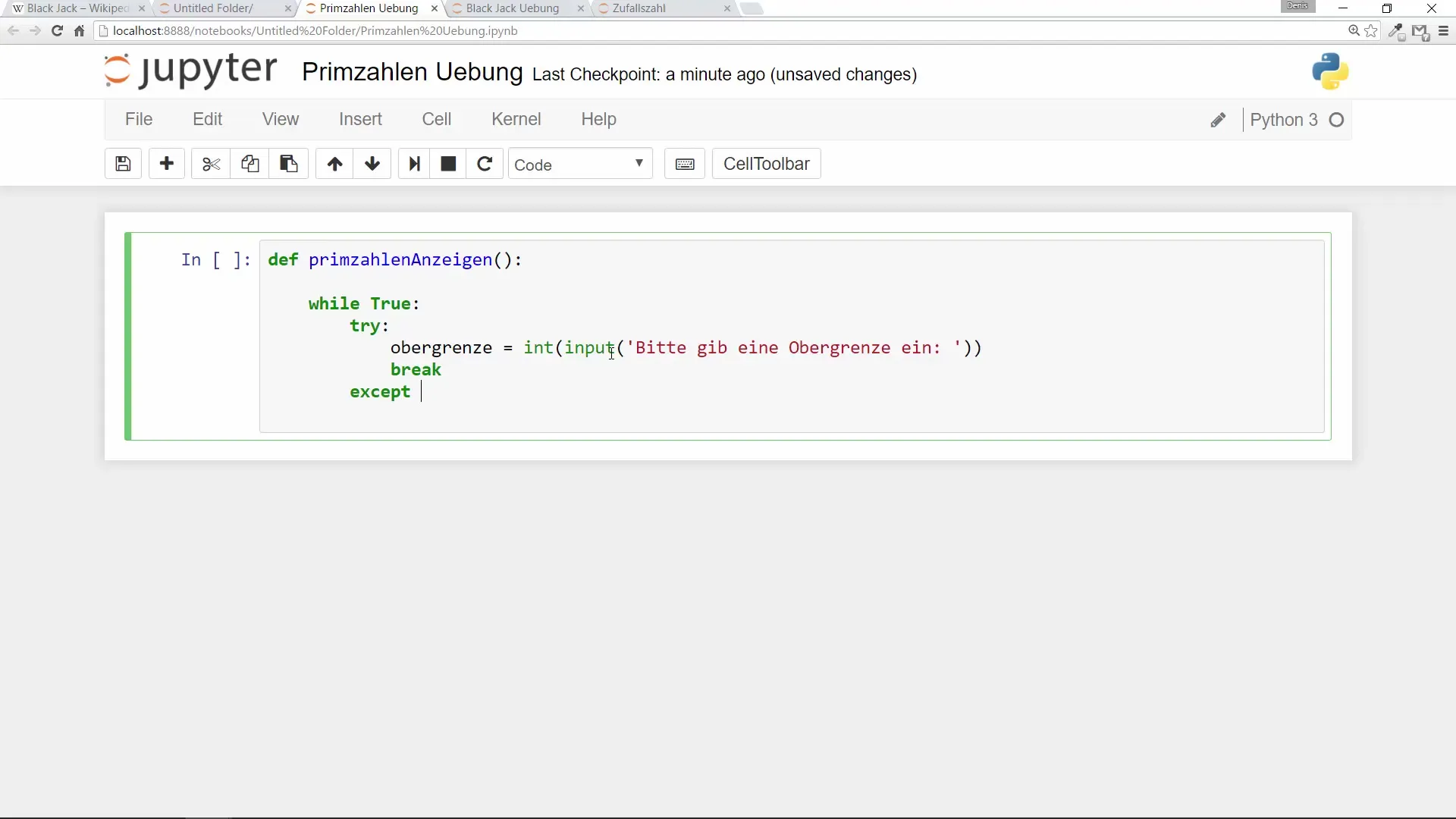
Step 5: Initialization of the Prime Number Collection
Once you have successfully converted the user input into an integer, create a list or array that contains all possible numbers from 2 up to the upper limit, initially marking these as prime numbers. For later processing, set the values for 0 and 1 in this list as False.
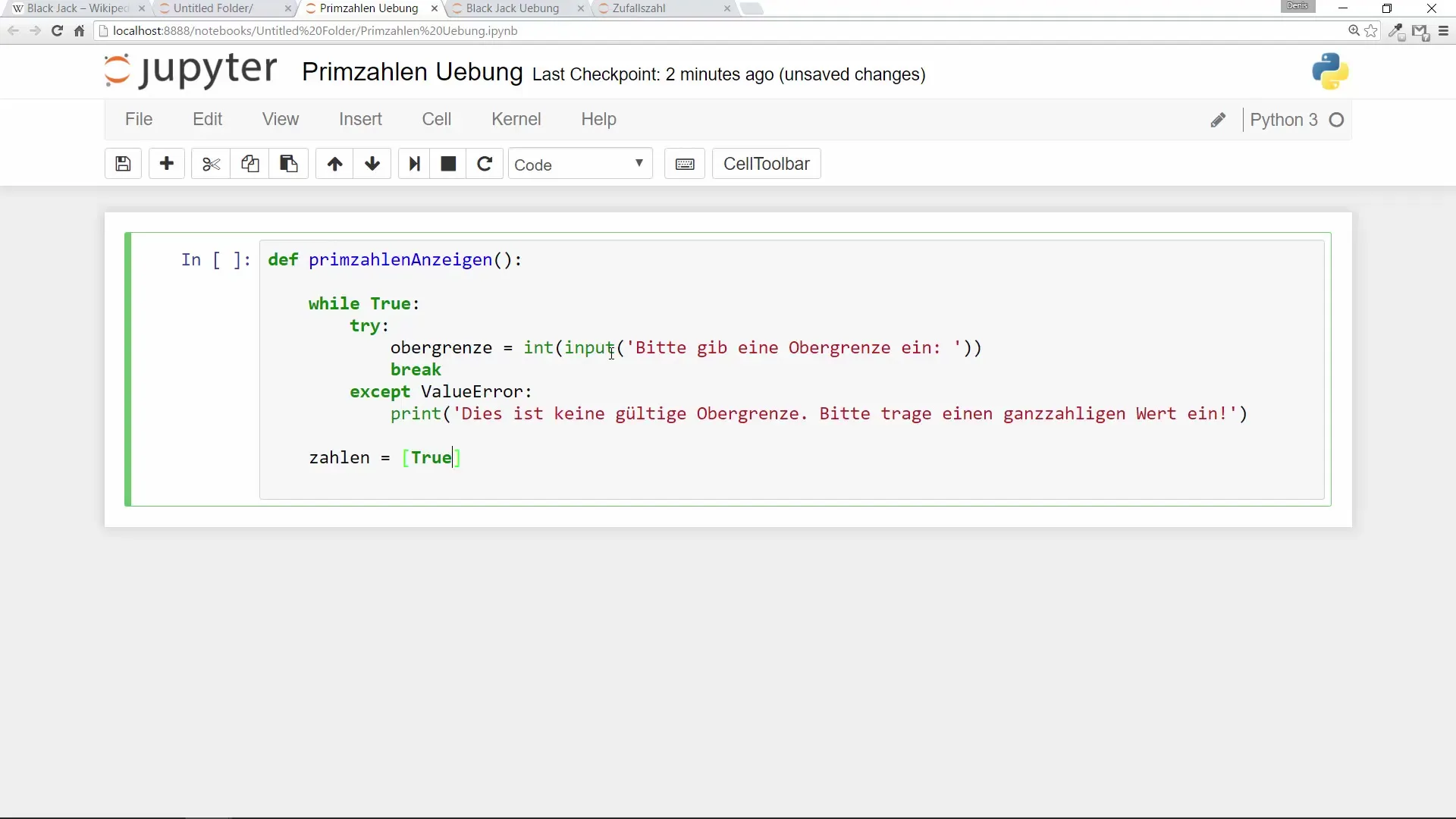
Step 6: Identify Prime Numbers
Now implement a loop that iterates through the numbers from 2 to the upper limit. Within this loop, you should use another loop to mark all multiples of the existing prime numbers. Each number that is no longer considered a prime will now be processed accordingly.
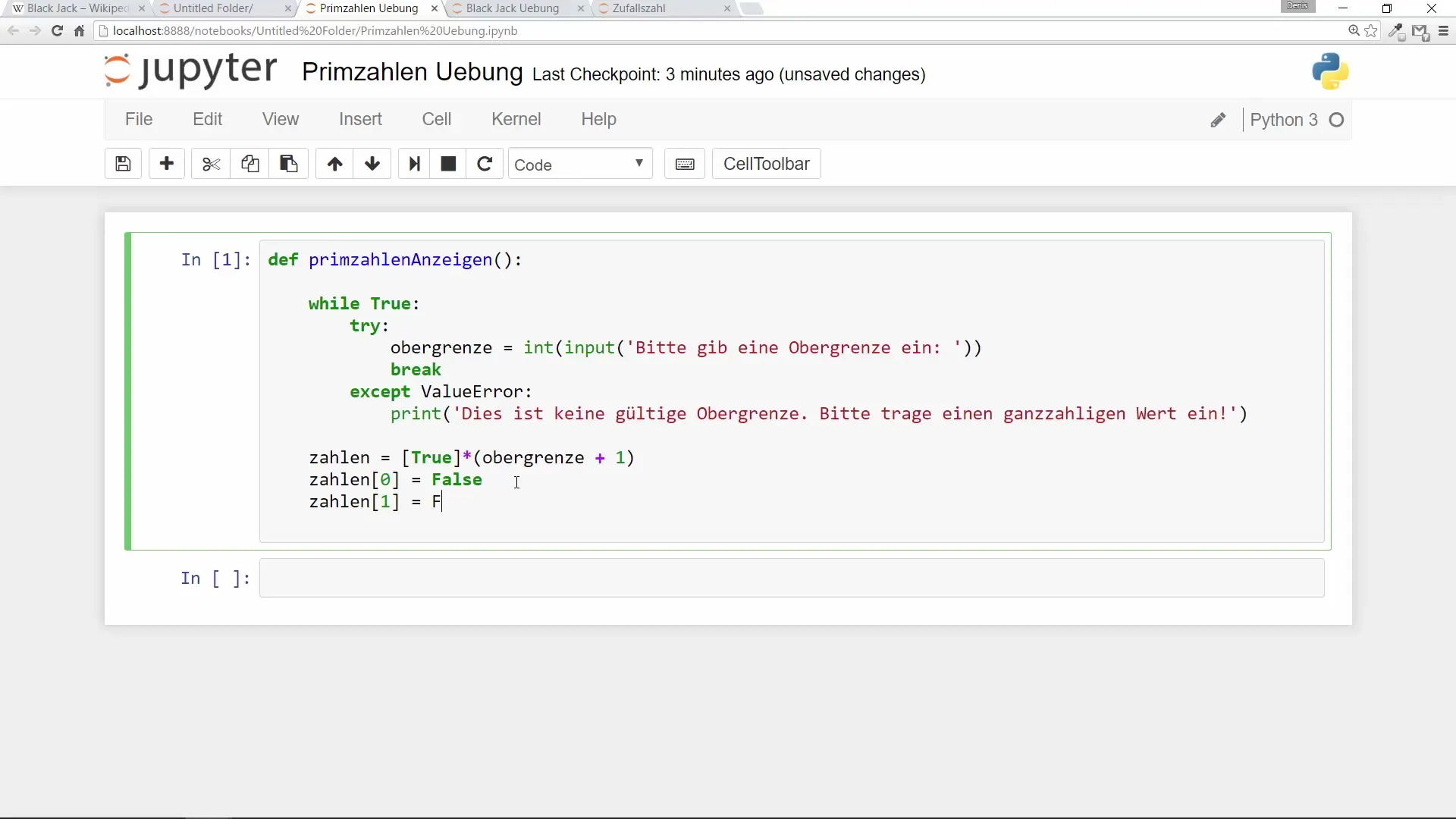
Step 7: Output the Prime Numbers
Once all multiples are no longer considered prime, output the prime numbers. Here you can use the enumerate function to display both the number and its status (whether it is a prime number or not).
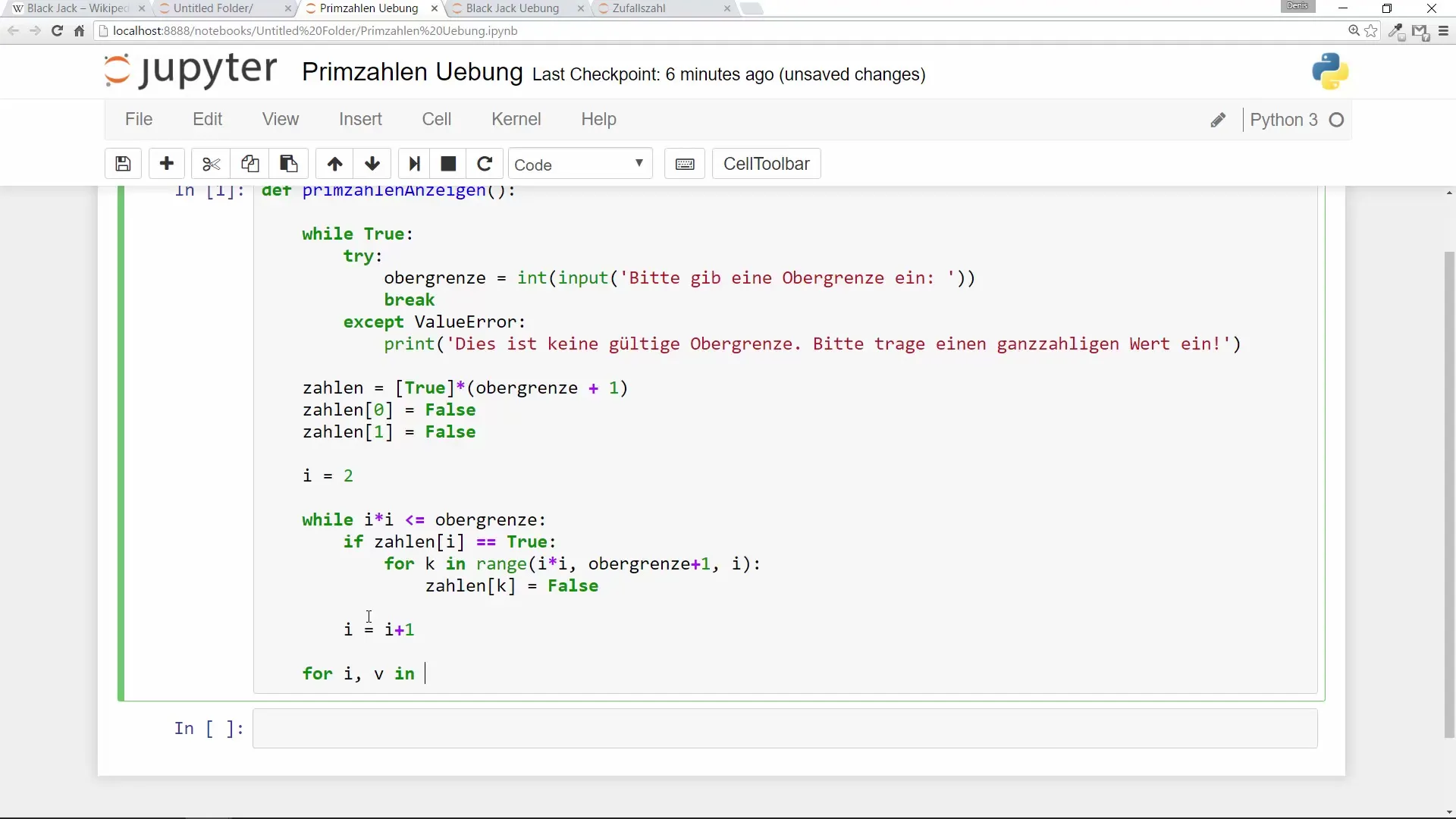
Step 8: Return Value of the Function
While it's optional, it's a good idea to specify a return value, even if you return None in this case. This makes your function more flexible in case you want to add an actual return value in the future. Call the function and run the program.
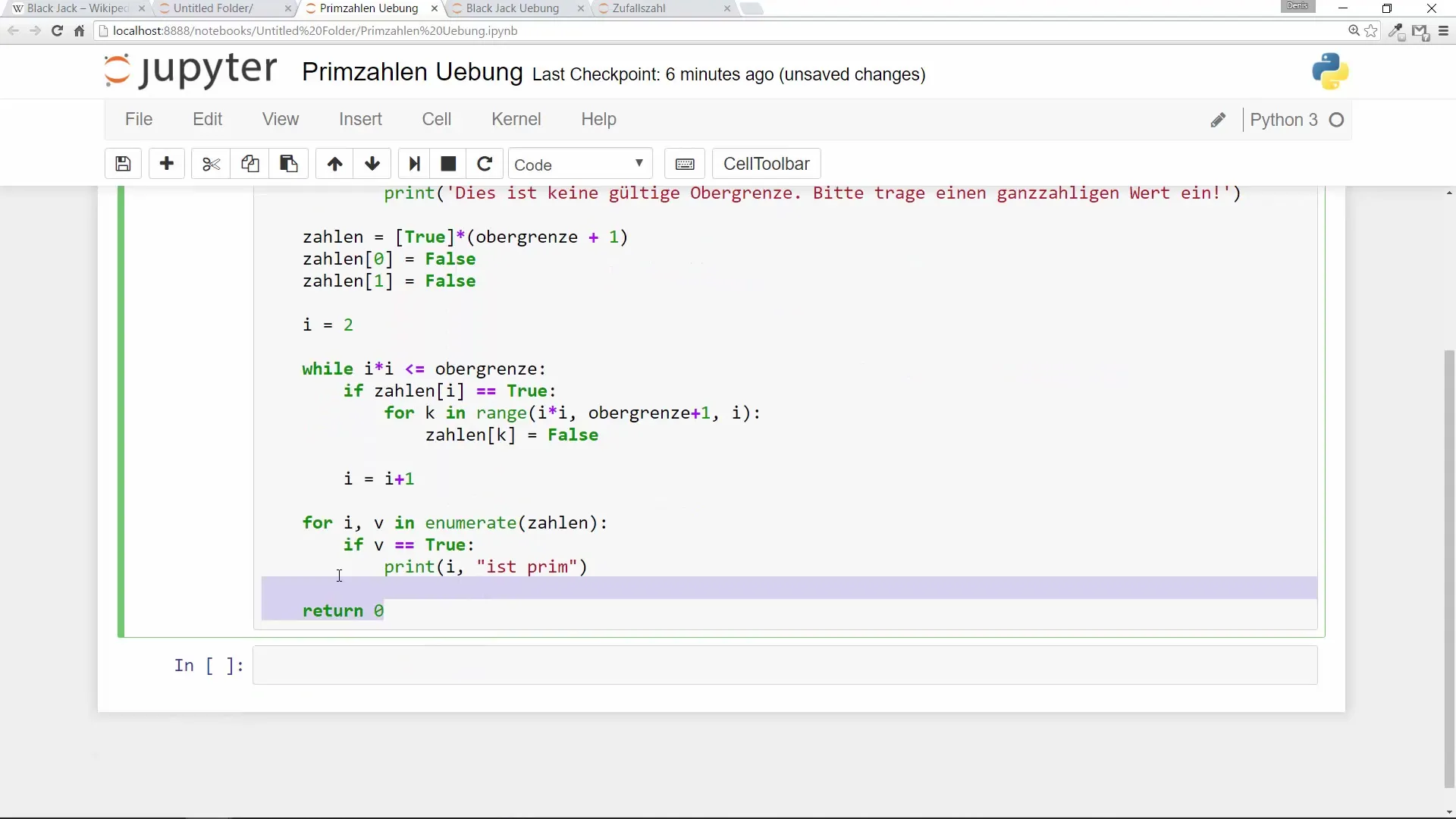
Step 9: Test the Function
Enter an upper limit, such as 17, and watch as the program outputs a list of prime numbers again. Each found prime number should be outputted on a new line to enhance readability.
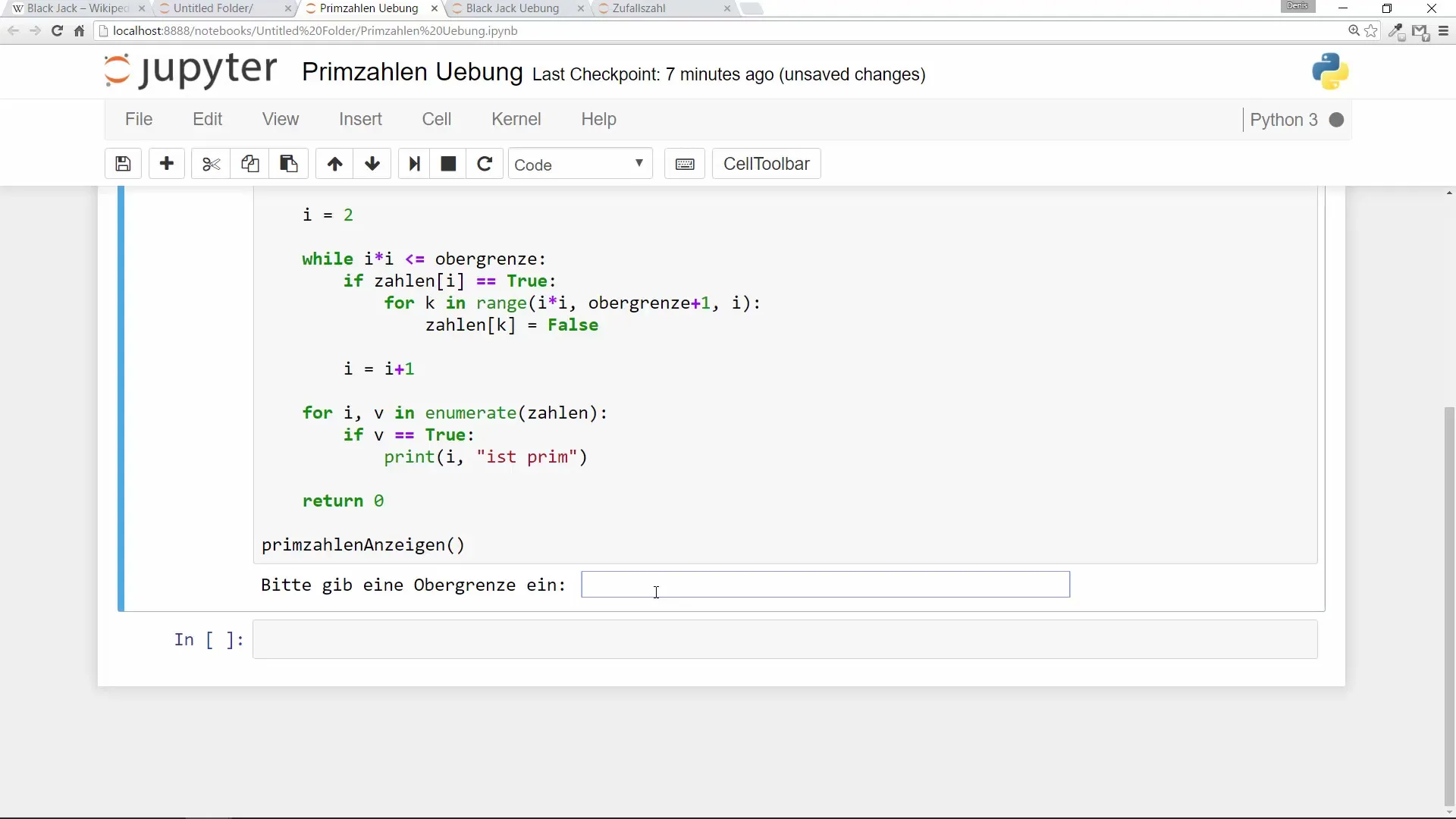
Summary
In this guide, you learned how to create a function to determine and display prime numbers in Python. From user input to console output, each step is crucial for understanding programming and handling numbers.
Frequently Asked Questions
What are prime numbers?Prime numbers are natural numbers greater than 1 that can only be divided by 1 and themselves.
How does error handling work in Python?Using try-except blocks, you can catch errors and display a user-friendly error message.
What role does the enumerate function play?The enumerate function allows you to capture both the index and the value of an object in a loop, simplifying data processing.
Why do we use while loops?While loops are useful to ensure that a condition is met before the program continues, such as during input validation.