Numbers are fundamental building blocks in programming. You encounter them in almost every program, whether you are performing calculations, analyzing data, or simply working with inputs. In this guide, you will learn about a variety of useful functions that will help you effectively work with numbers in Python. Let's dive straight into the practical information!
Key Insights
- The round function allows you to round numbers to a desired number of decimal places.
- With math.ceil, you can round numbers up to the next whole number.
- math.floor rounds numbers down to the next lower whole number.
- The abs function returns the absolute value of a number.
- Through the pow function, you can compute exponents.
- Functionality for conversion to hexadecimal and binary values is also available.
Step-by-Step Guide
First, let's look at the basic rounding functions.
The round function is your first point of contact when it comes to rounding numbers. You can use it to round a numeric value to the next whole number or to a specific number of decimal places. For example, if you enter round(5.6), you will get 6 as a result. On the other hand, if you use round(5.4), you will get 5.
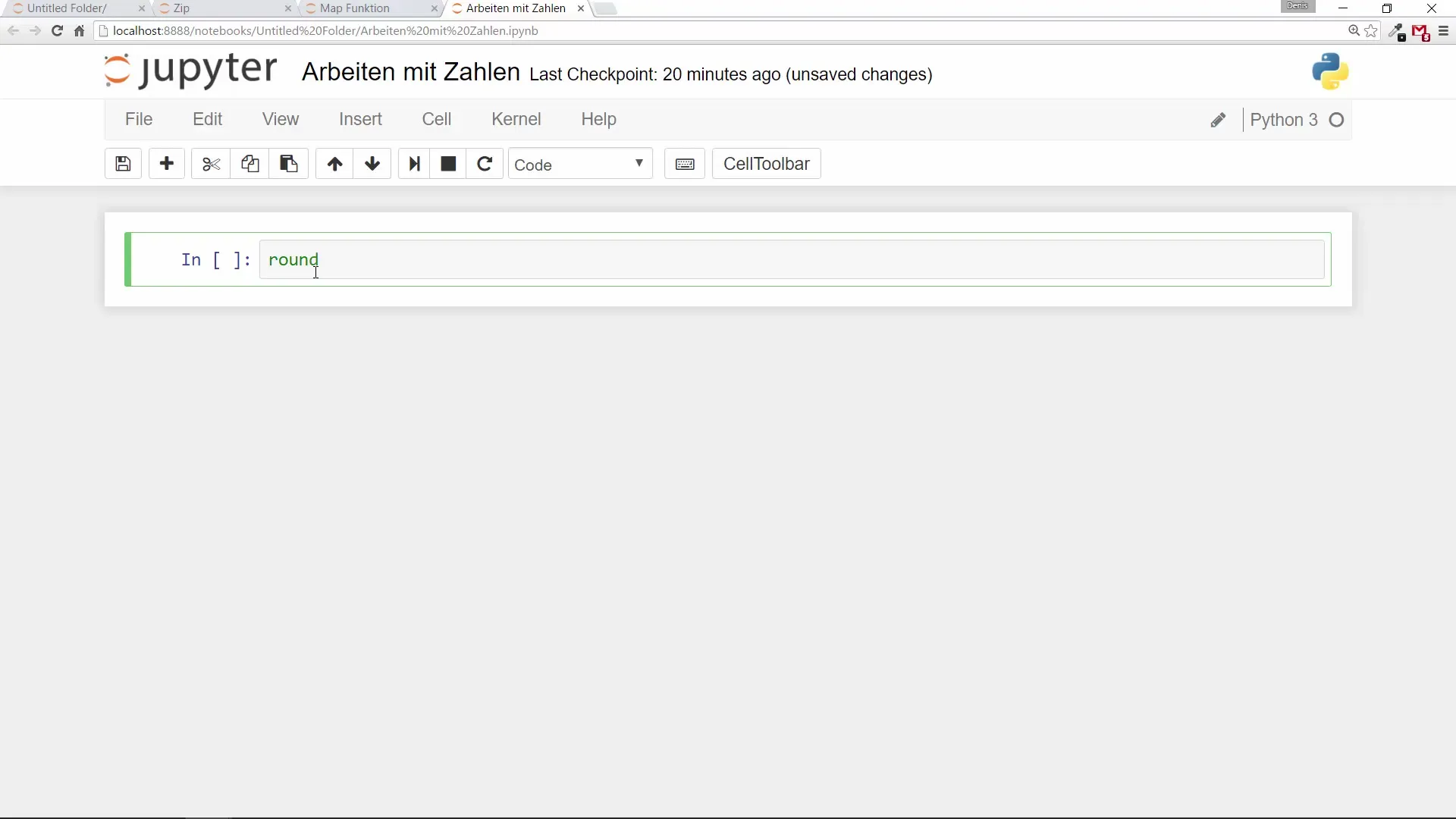
Do you want to round the number to a specific number of decimal places? That's also possible! Just specify the number of decimal places as the second parameter. For example, if you use round(5.41251, 2), it results in 5.41. With this method, you can easily control how precise your results should be.
There are also scenarios where you always want to round a number up to the next whole number. For this, you use the math.ceil function. To utilize this function, you first need to import the math module, which you do with import math. After importing, you can use math.ceil(5.41251), for example. This call will return 6.
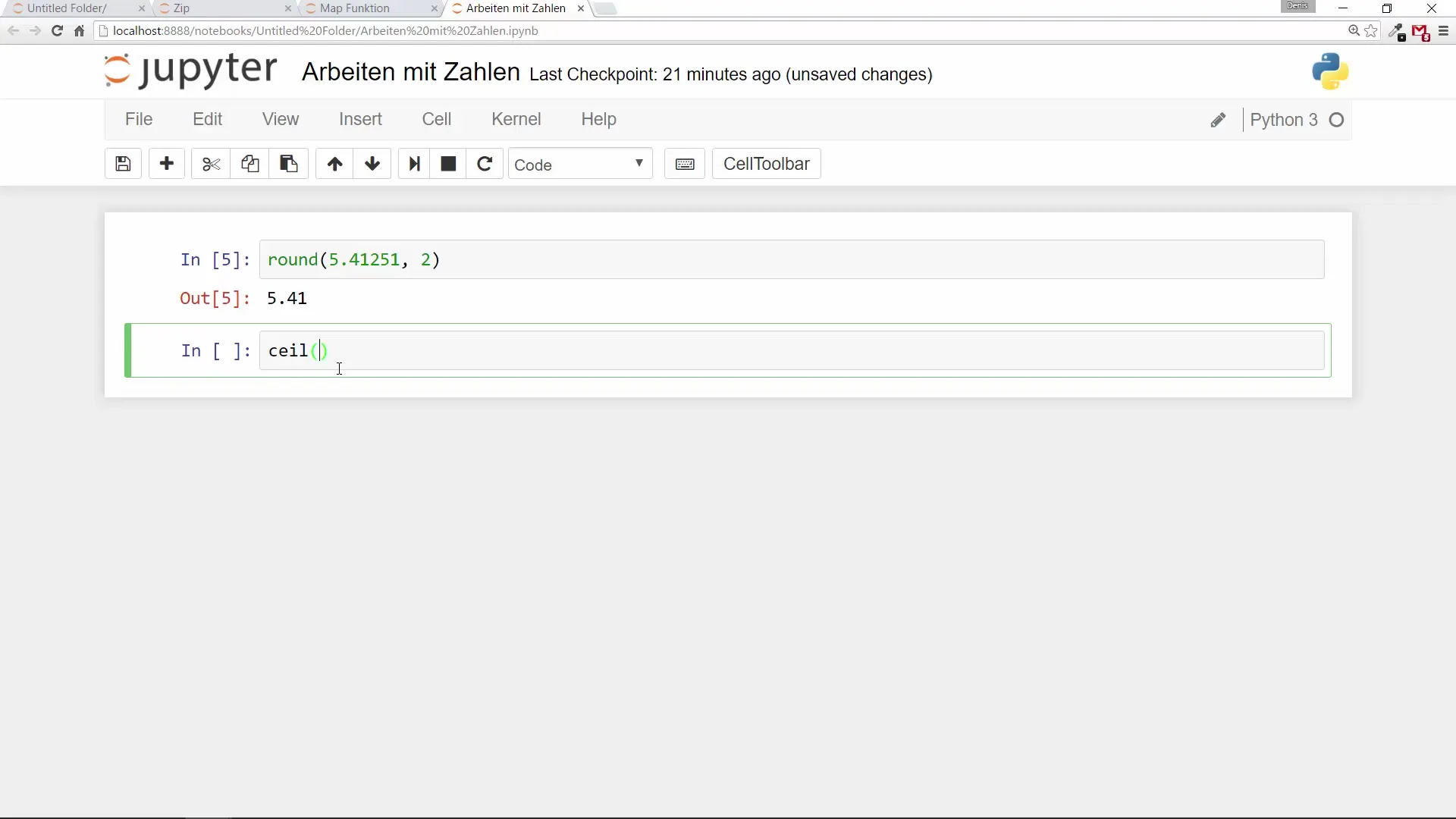
On the other hand, the math.floor function allows you to round numbers down. So if you enter math.floor(5.41251), you will get 5 as a result since this function rounds the number down to the next lower whole number.
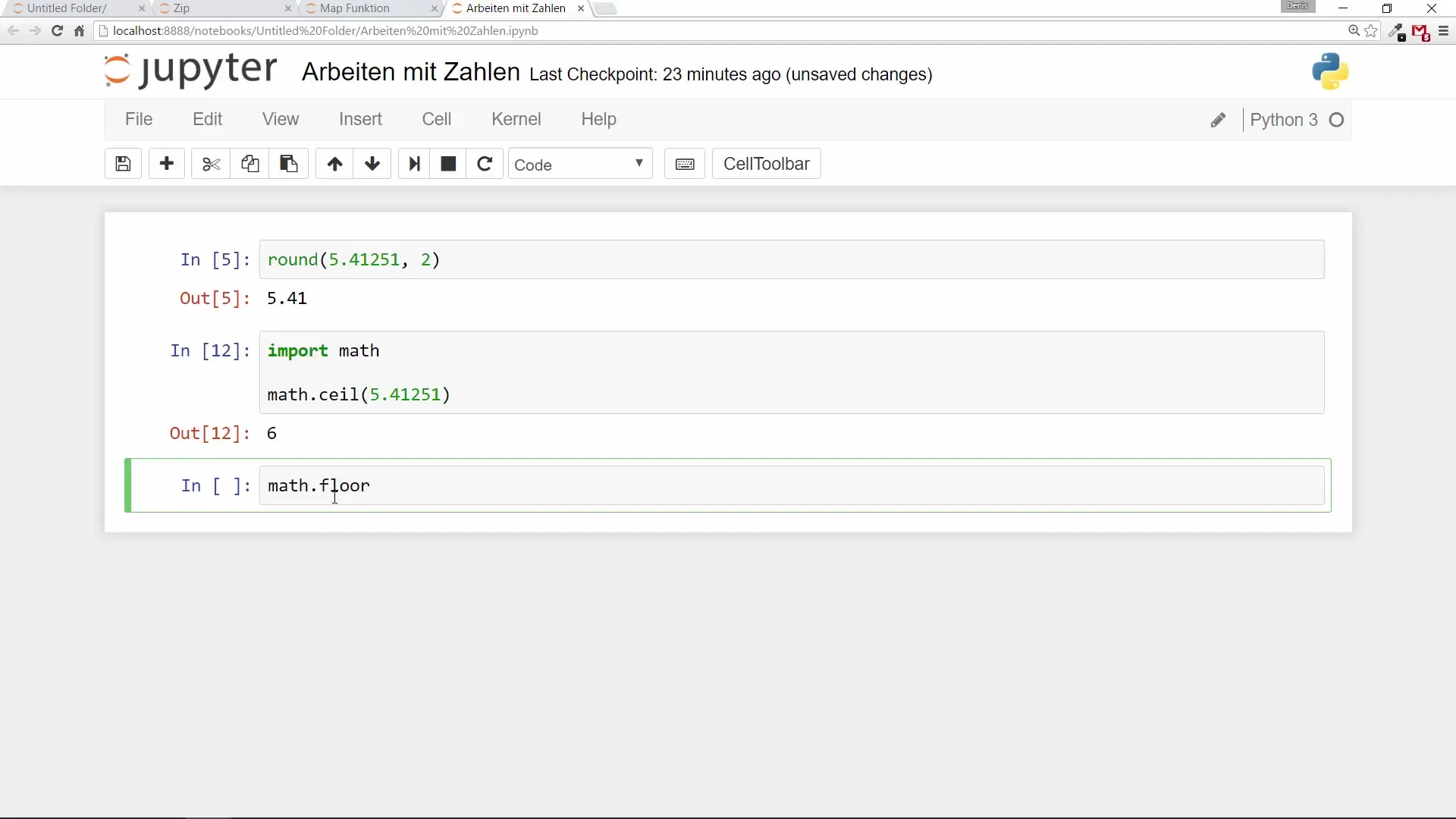
If you are working with negative values, you may need the absolute value of a number. With the abs function, you can use abs(-55), for example, which gives you the value 55. This is particularly helpful when you're unsure whether a value is positive or negative but always need a positive value.
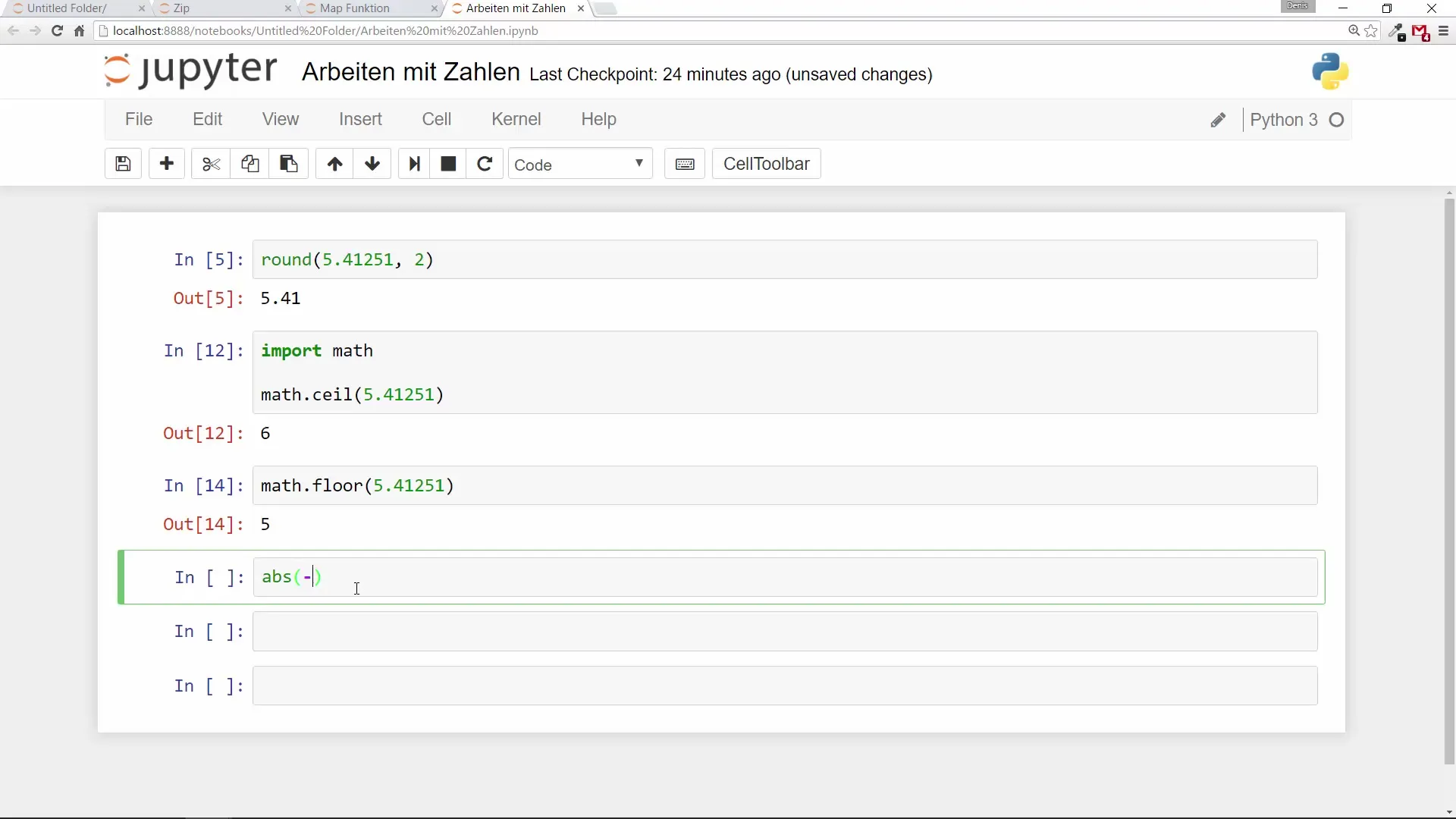
The pow function allows you to compute with exponents. For example, you can use pow(2, 3), which results in 2 raised to the power of 3, giving you the value 8. If you enter pow(2, 10), you will get 1024 as a result.
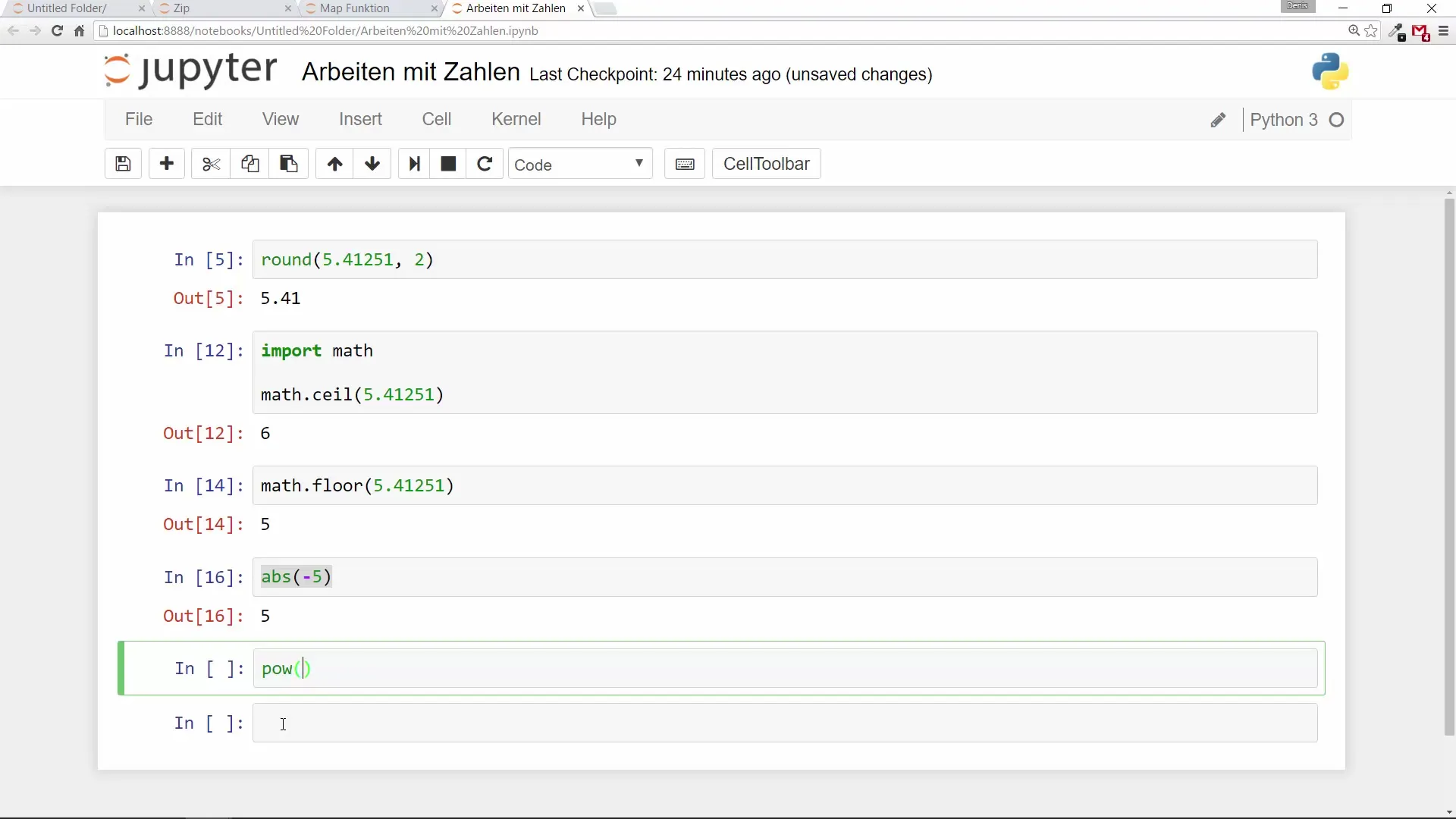
If you want to convert a number into a hexadecimal value, you can use the hex function. For example, when you enter hex(123), you will get 0x7b. This way, you can conveniently work with hexadecimal numbers, which can be necessary in many programming scenarios.
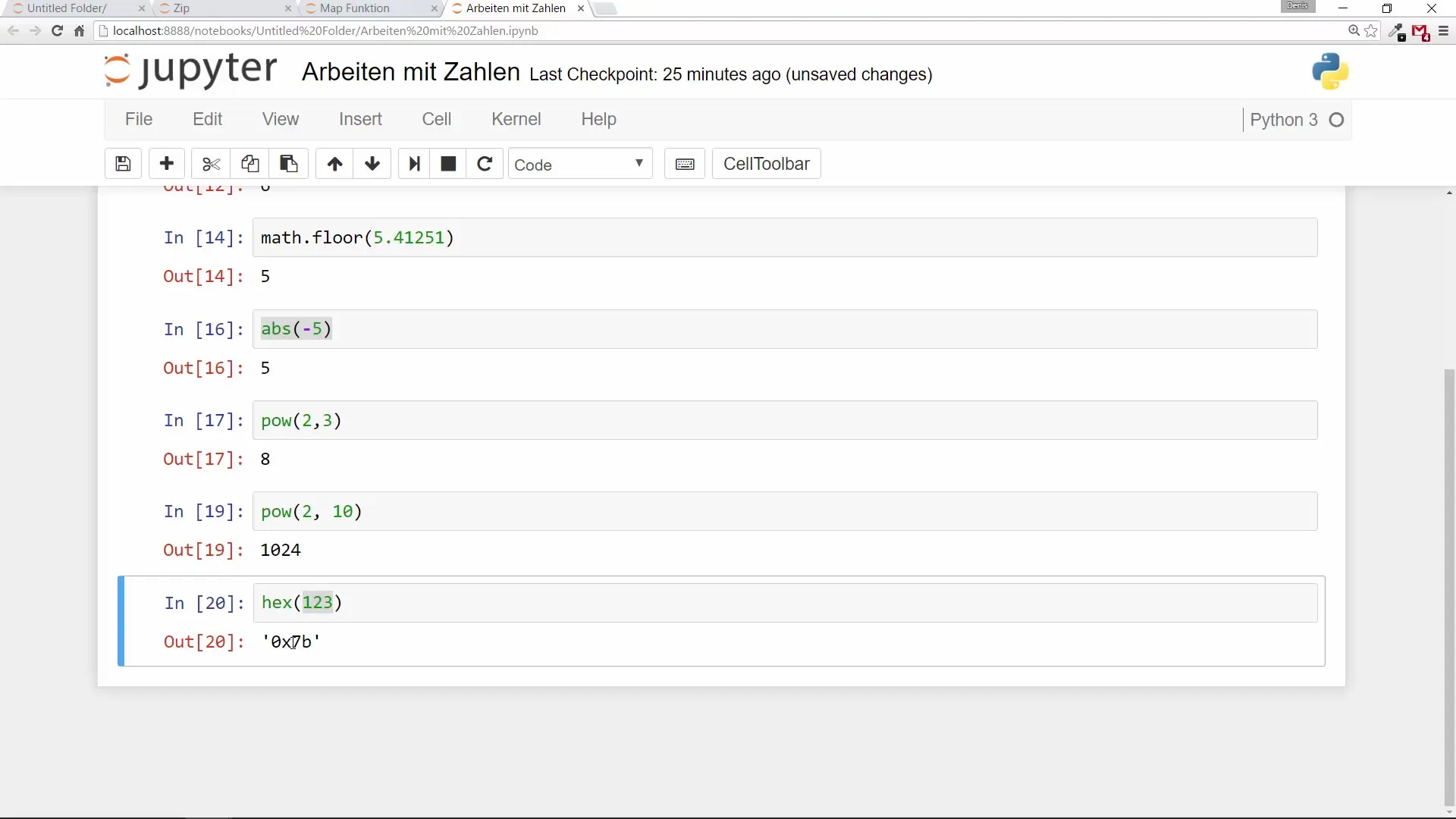
Also important is the ability to convert numbers into binary values. With the bin function, you can convert a number like 123 into the binary value, which gives you 0b1111011. This is especially helpful when you're working with bit operations or need to interpret stored data.
For more complex mathematical functions, such as cosine or arc cosine, you can refer to various functions from the math module. These functions are well documented on the website media.sonic.ch, where you can also find the desired results.
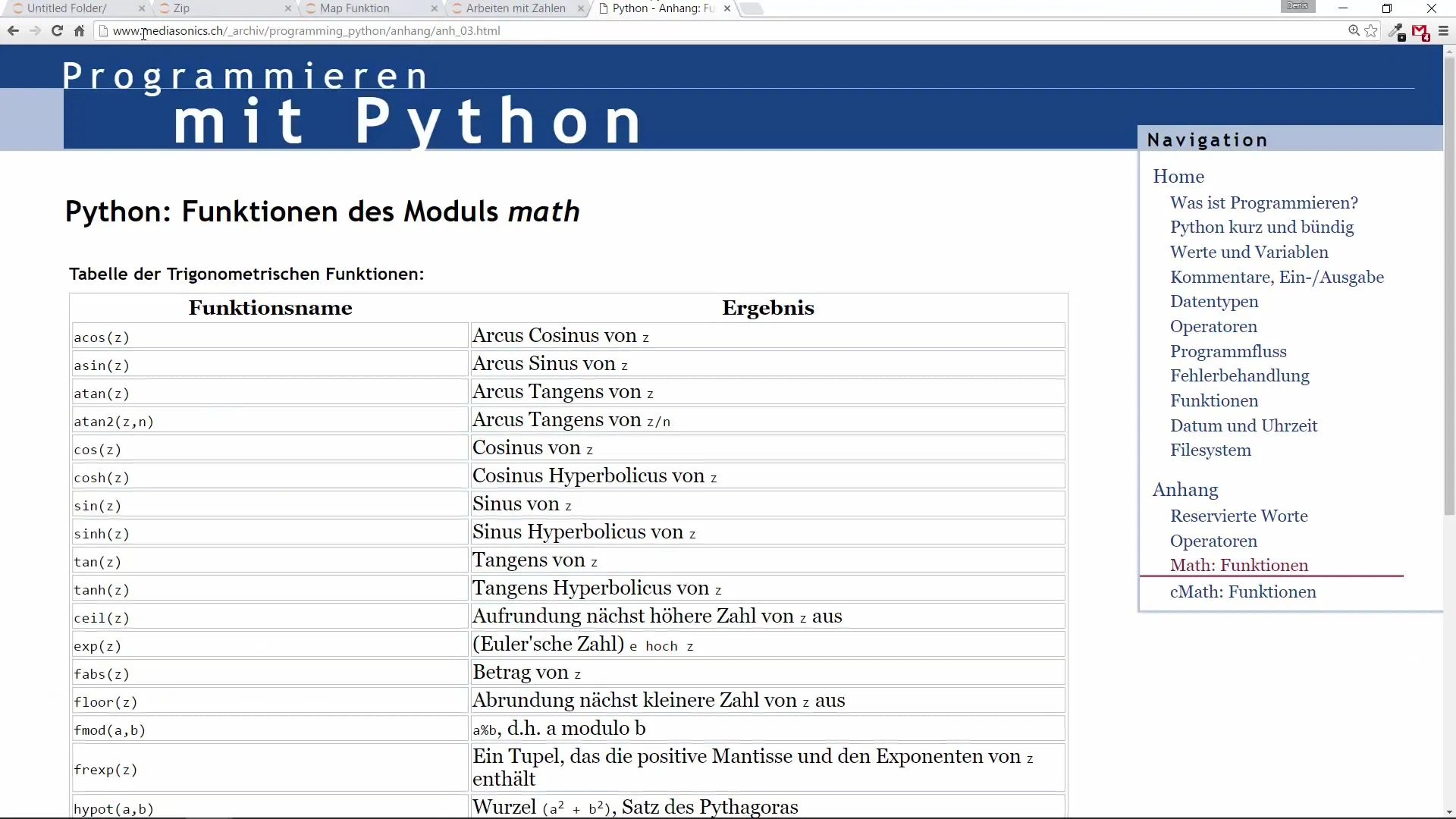
However, there are some functions that specifically require the math module, such as math.floor and math.ceil. Make sure to import the module before using these functions.
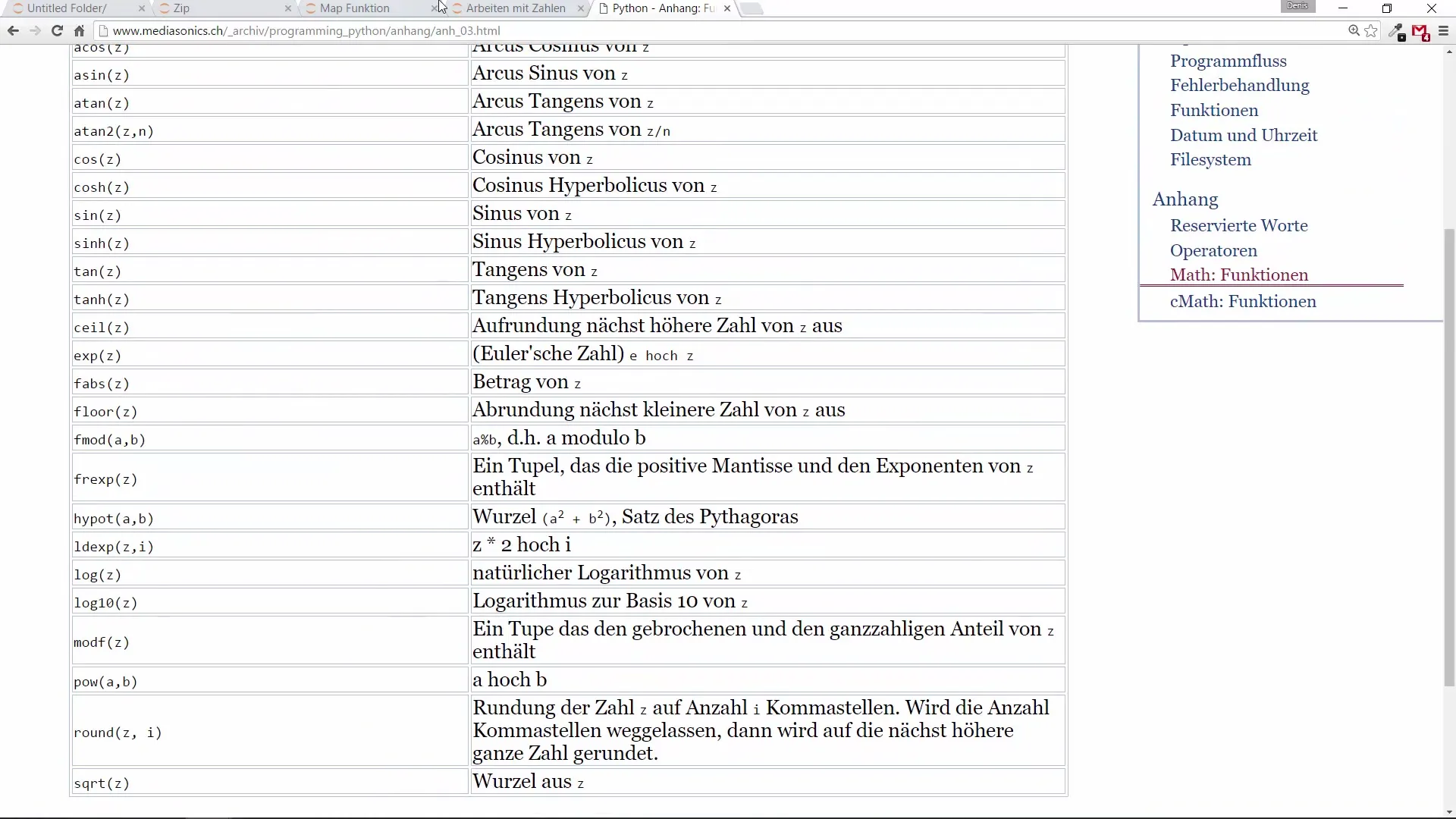
With these basic functions, you have laid a solid foundation for working with numbers in Python. Learning these methods will help you develop more efficient and accurate code solutions.
Summary
In this guide, you learned about the key functions and methods for working with numbers in Python. You now know how to round numbers, round up or down, determine absolute values, calculate exponents, and convert numbers to hexadecimal or binary values. These skills will certainly be helpful in programming.
Frequently Asked Questions
What is the round function?The round function is used to round numbers to the next whole number or to a specific number of decimal places.
How does math.ceil work?math.ceil rounds a number up to the next whole number.
Can I get the absolute value of a number with Python?Yes, you use the abs() function for this.
How do I get exponents with pow?The pow(x, y) function calculates x raised to the power of y.
How do I convert a number to a hexadecimal value?Use the hex() function to convert a number into hexadecimal format.