The development of graphical user interfaces (GUI) is an exciting and fulfilling area in programming. Especially with Python and the Tkinter module, many possibilities open up to create user-friendly applications. This guide helps you understand the basics of GUI programming in Python and allows you to create simple user interfaces independently.
Key insights
- Tkinter is the standard module for creating GUIs in Python.
- GUIs consist of various elements such as labels, buttons, and input fields.
- The execution of a main loop is crucial for the display and interaction of the GUI.
- The Pack method enables simple layout management of GUI elements.
Step-by-step guide to creating a simple GUI
Installing PyCharm
Before you start programming your first GUI, it is important to set up the right development environment. In this case, we recommend PyCharm, a lightweight IDE that is easy to handle.
Download PyCharm from the official website. Visit jetbrains.com and search for PyCharm. Choose the Community Edition, which is sufficient for Python development. After downloading, you can run the installation file and go through the installation process. Make sure to select the 64-bit version and assign the.py file extension to the PyCharm environment. After successful installation, open the IDE.
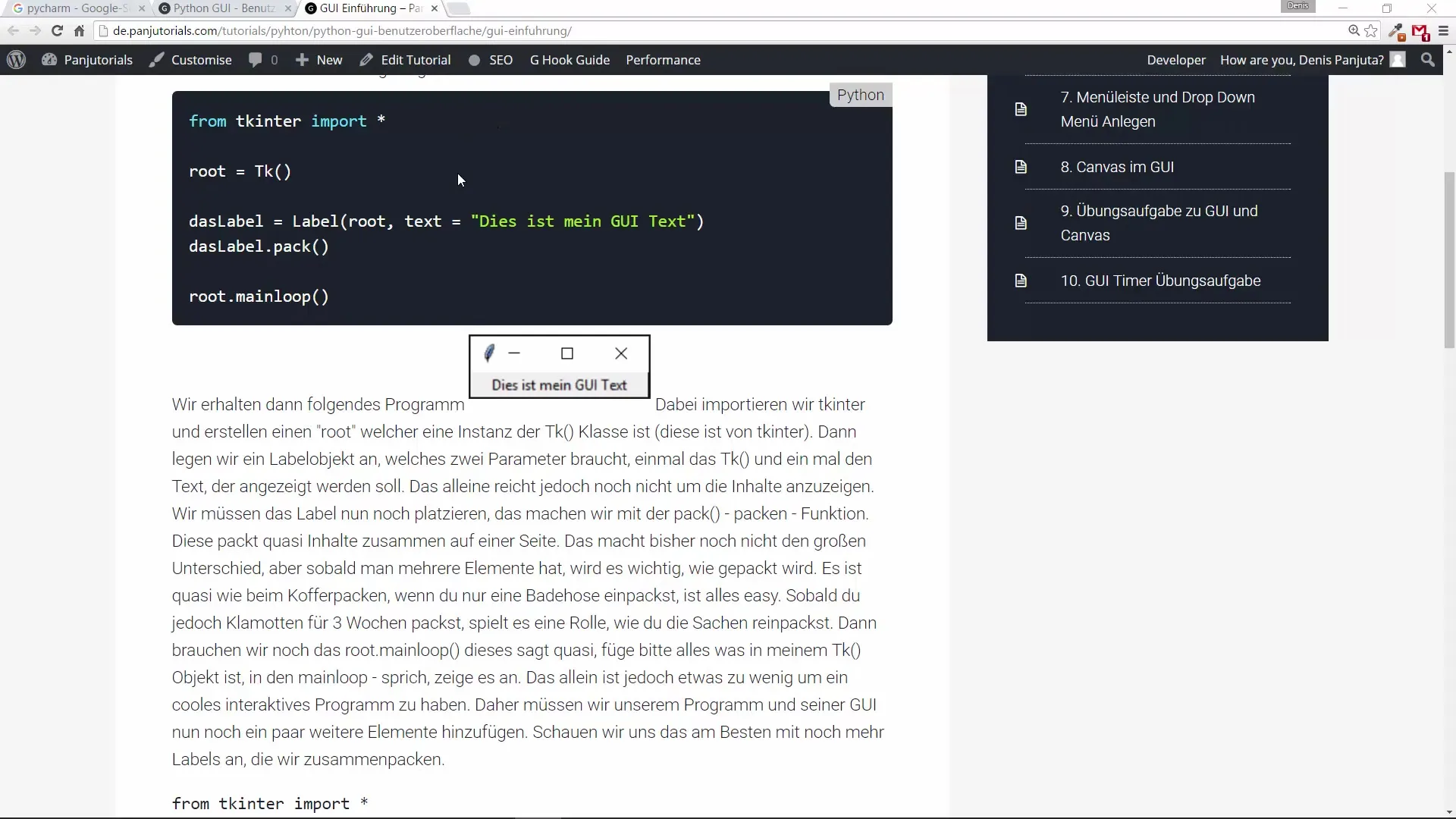
Creating a new project
Once PyCharm is open, create a new project by creating a folder named "Python Course". Inside this folder, create a new Python file, for example, "Video_1.py". This file will be the starting point for your first GUI application.
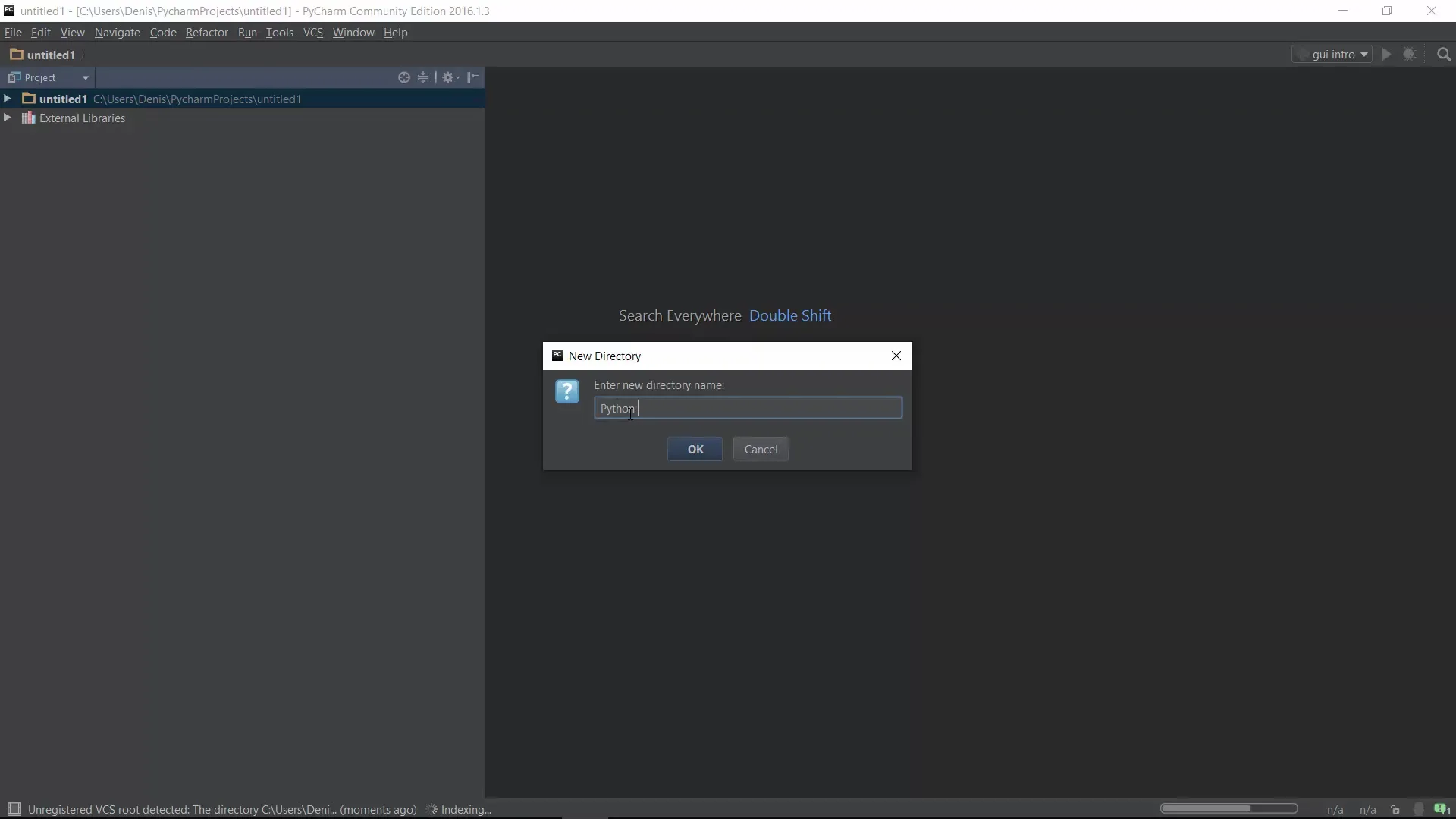
Importing Tkinter
Next, you need to import Tkinter in your Python script. This is done easily with the line from tkinter import *. This module provides you with all the necessary functions and classes to design the graphical user interface.
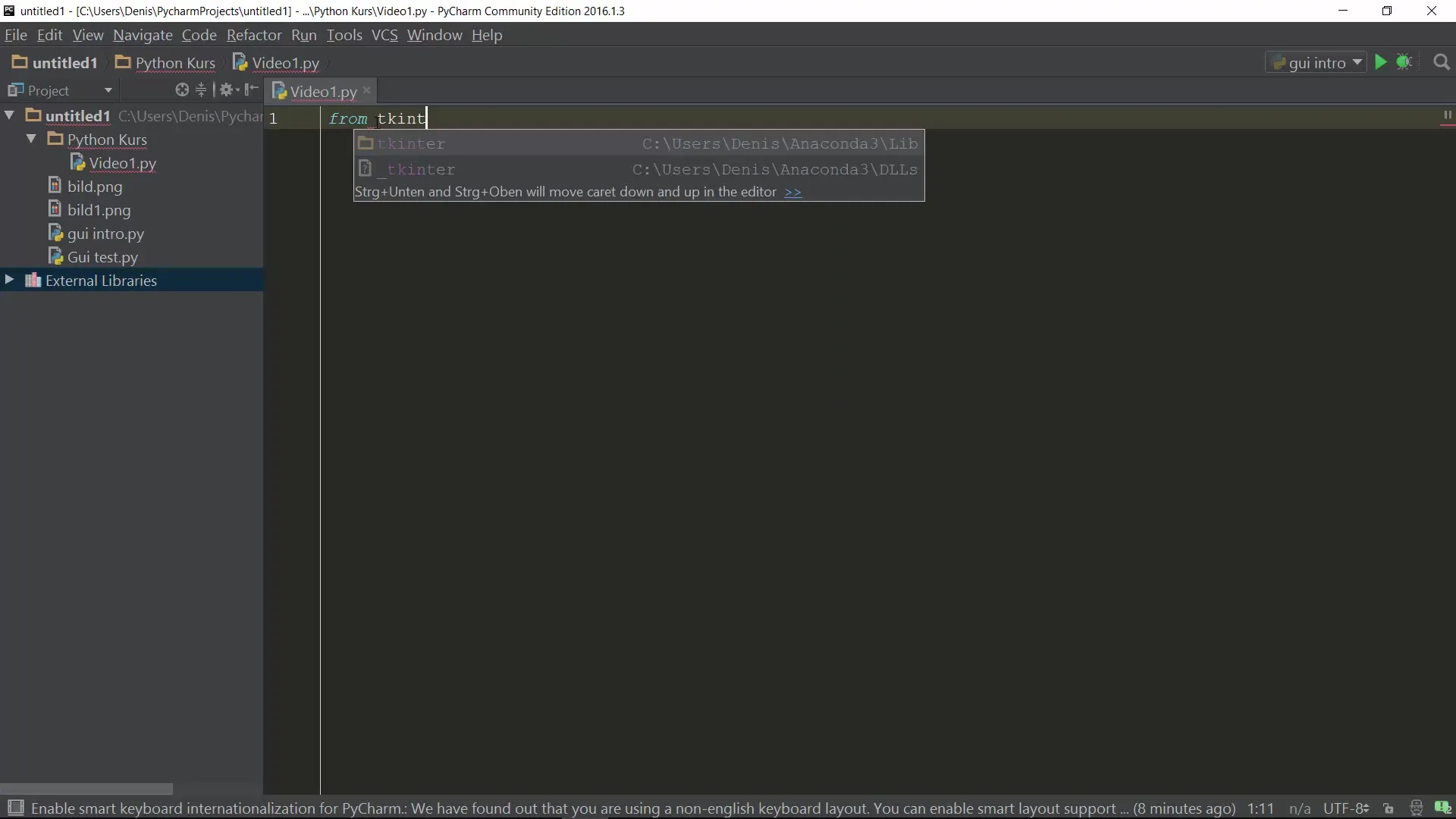
Creating a Tk object
Next, you need to create a Tk object that represents the basis of your GUI. This is done with root = Tk(), where root represents your main window. You can also replace the term “root” with “master”, which is used in many examples.
Adding a label
Now you can add a label to display text. Create a label object with label = Label(master=root, text="This is my first GUI"). Here, our text is defined through the text attribute. Don't forget to add the label to the GUI with the Pack method afterward: label.pack().
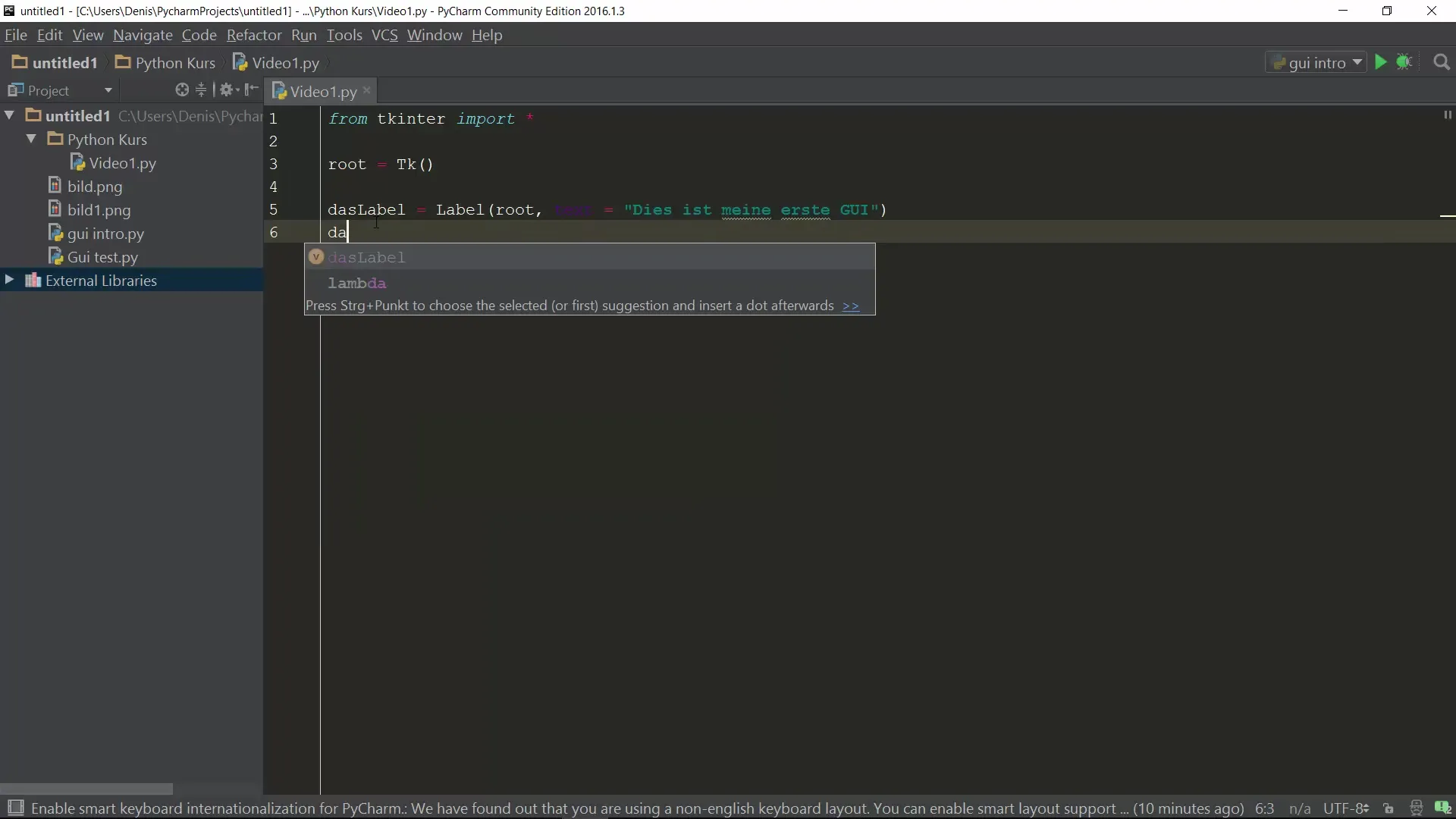
Starting the main loop
To render your GUI and enable user interaction, you need to start the main loop. This is done with root.mainloop(). This main loop ensures that the window remains open and is continuously updated until the user closes the window.
Testing the GUI
After completing all the steps, you can run your program. You can choose the “Run” option from the context menu or use the keyboard shortcut Shift + F10. You should now see a window displaying the text “This is my first GUI”.
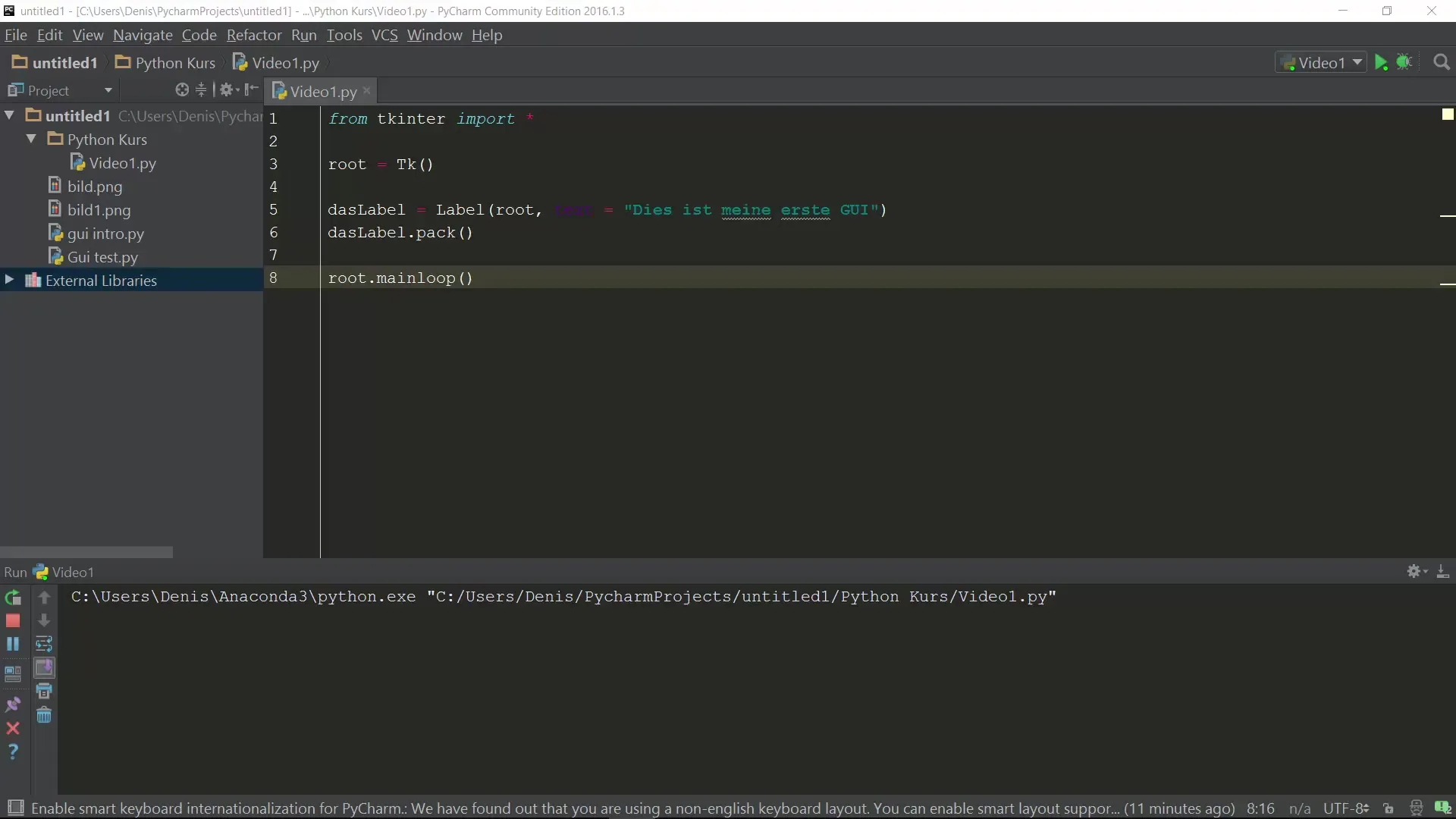
Adding additional labels
To practice, you can add multiple labels. For example, create another label with label2 = Label(master=root, text="Text 2") and add it to the GUI as well. Repeat this step for additional labels.
Making GUI adjustments
When you test the GUI, you may notice that all labels are displayed one below the other. The simple use of the Pack method ensures that these elements are arranged accordingly. If you resize the window, the arrangement remains intact.
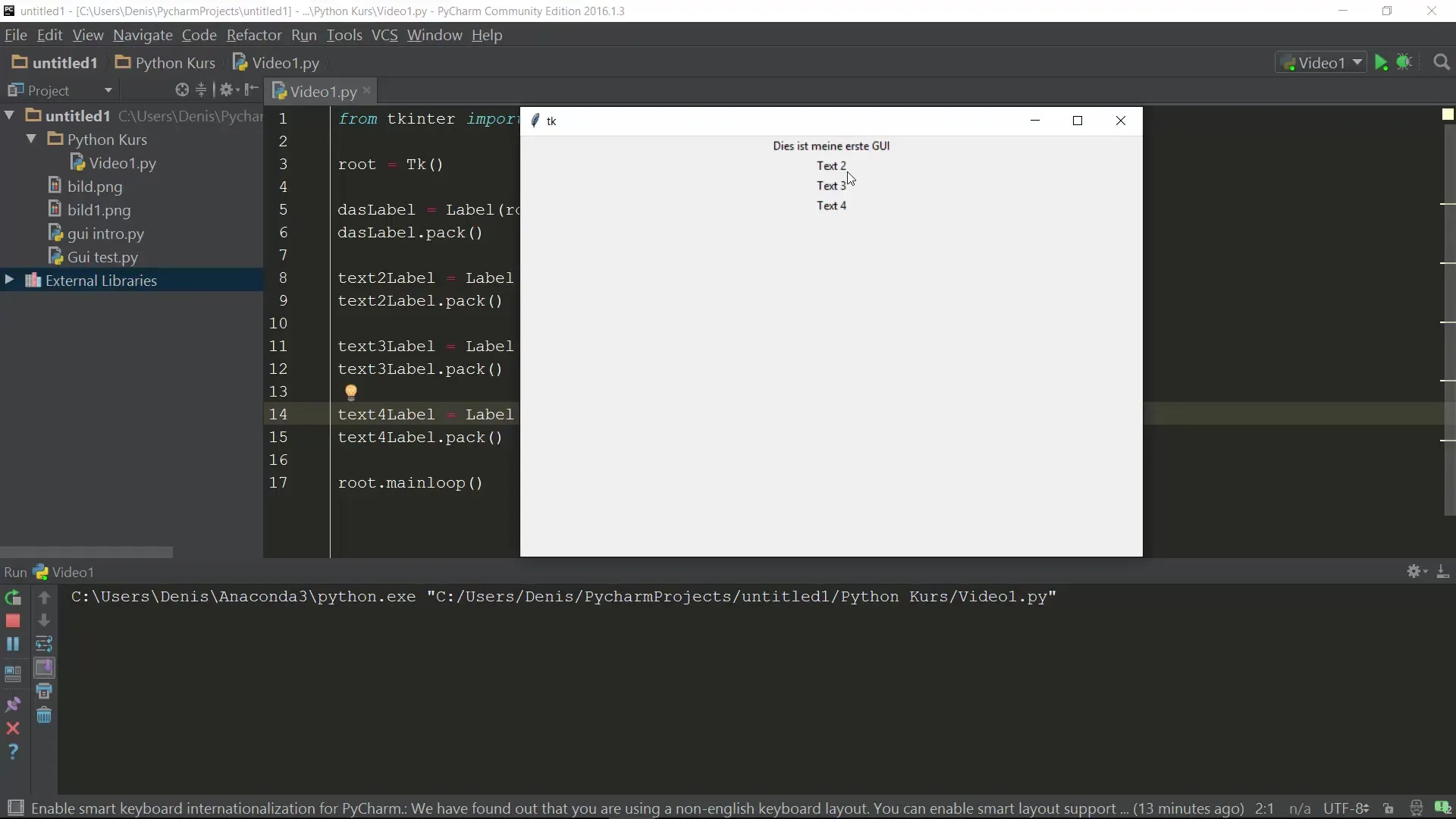
Summary
In this guide, you have learned the basics of GUI programming with Tkinter. You learned how to set up a development environment, create a simple window, display text, and add multiple GUI elements. With this knowledge, you can now start developing more complex applications with graphical user interfaces.
Frequently asked questions
How do I import Tkinter in Python?Use the line from tkinter import * to import the module.
Why is the main loop important?The main loop keeps your GUI visible and responsive to user actions.
Can I add other GUI elements as well?Yes, Tkinter offers many additional elements such as buttons, input fields, and more.
How can I change the font size?The font size can be adjusted through the attributes of the label or other widgets.
Does Tkinter work on all operating systems?Yes, Tkinter is cross-platform and works on Windows, Linux, and macOS.