Generating random numbers plays a significant role in programming. Whether you're developing a game, creating simulations, or simply experimenting with random numbers, it's important to know how to generate them effectively. In this tutorial, I will show you how to generate random numbers with JavaScript. We'll walk through the process step by step.
Key Takeaways
- With JavaScript, you can generate random numbers in the range of 0 to 1.
- To obtain a random number in a specific range, some calculations are necessary.
- Using the Math.floor() method allows you to extract integers from random numbers.
Step-by-Step Guide
To generate random numbers, we will work with the Math class in JavaScript. First, I will explain the basics and then move on to specific examples.
First Contact with Random Number Generation
Start by creating a variable to store the random number. In JavaScript, we use the Math.random() function to generate a random number between 0 (inclusive) and 1 (exclusive).
Here's how you do it in your code:
With this line in your script, you generate a random number. Let's try this out in practice.
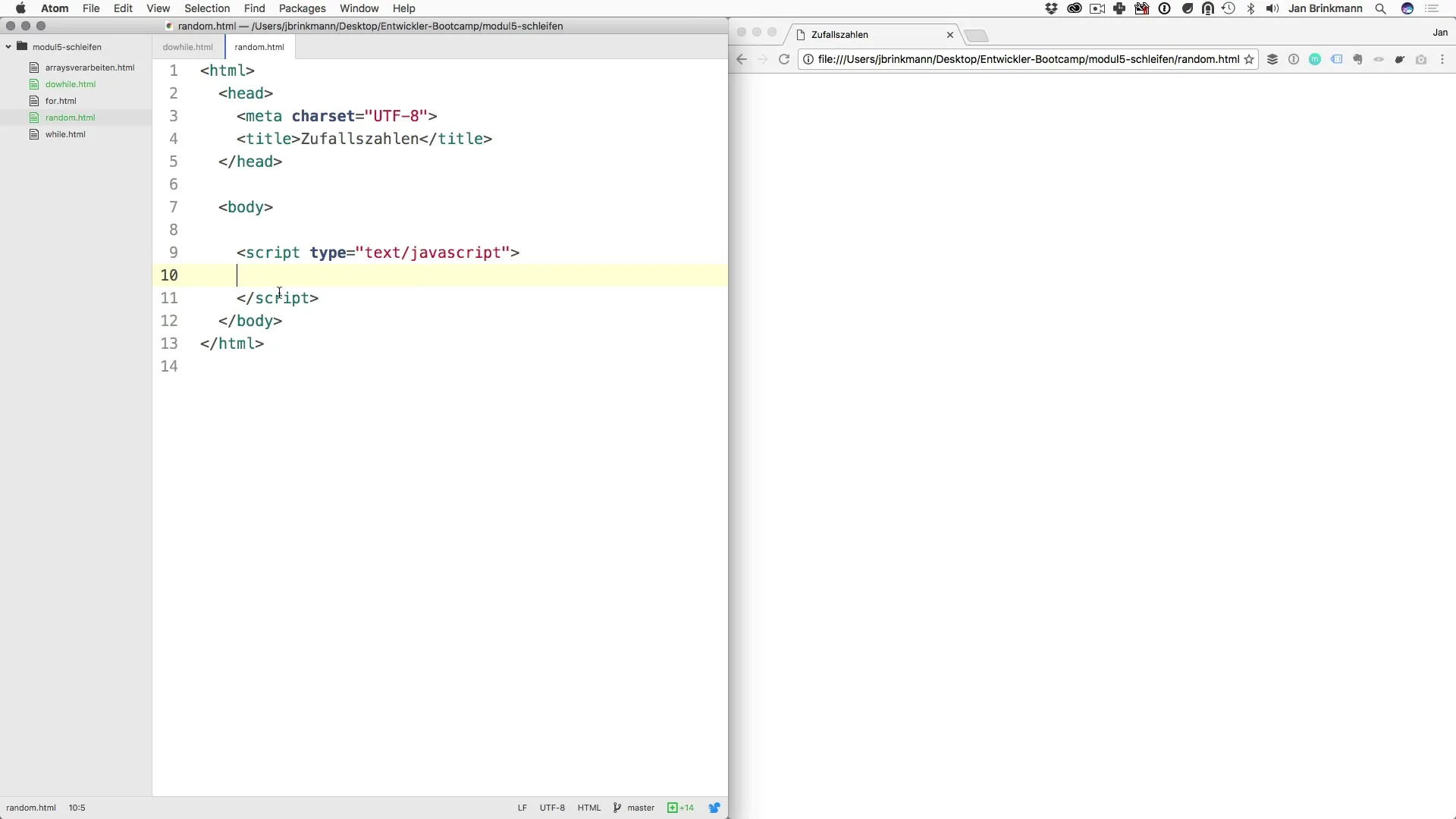
Challenge: Defining a Specific Range
The next challenge is to generate a random number in a specific range, for example, between 1 and 10. The simplest way to achieve this is to multiply the random number by the upper limit while ensuring that we add the correct offset.
Rounding Random Numbers
The next issue is that the values generated by Math.random() are decimal numbers. To obtain integers, we use the Math.floor() function.
Here, Math.floor() ensures that the number is rounded down, allowing you to receive only integers.
Random Numbers in Another Range
If you want to generate random numbers between 1 and 100, you can apply the same logic.
This line generates random numbers between 1 and 100. It’s important to note that due to this method, the result will never be 101 or higher.
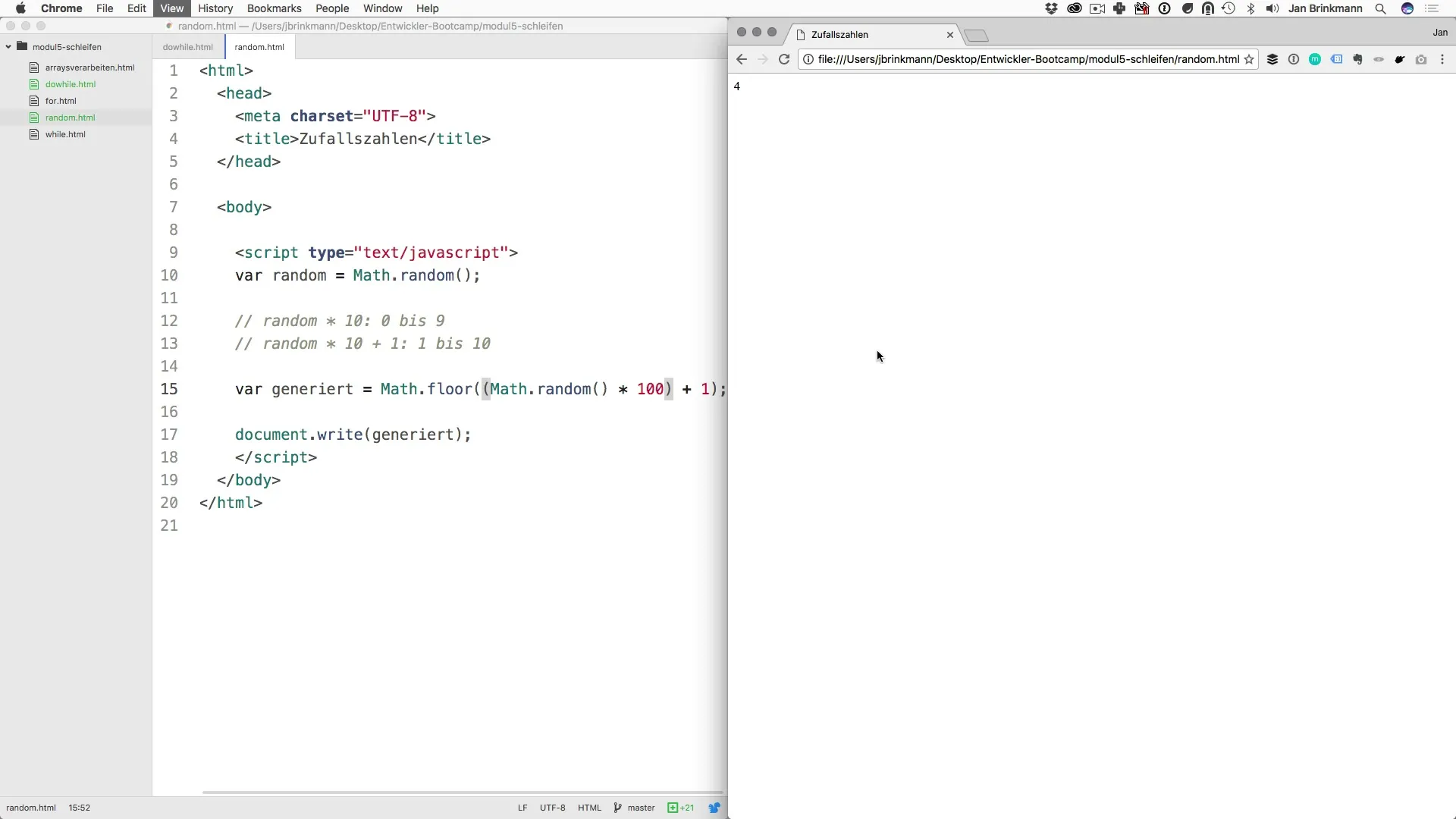
Summary – Generating Random Numbers in JavaScript: A Practical Guide
In this guide, you have learned how to generate random numbers with JavaScript. You can produce random numbers between 0 and 1 and adjust these to obtain whole numbers in specific ranges. Using Math.random() in combination with Math.floor() provides you with the flexibility you need. Use these techniques to realize your own exciting projects!
Frequently Asked Questions
How can I ensure that random numbers are truly random?The Math.random() function in JavaScript uses a pseudo-random number generator that is sufficient for most purposes.
Can I also generate random numbers in other ranges?Yes, you can adjust the multiplication and addition to generate random numbers in any desired range.
Are there differences between methods in different programming languages?Yes, each language has its own methods for generating random numbers, but the basic concept often remains similar.