The understanding of Rechenoperatoren is a fundamental part of programming in Java. You will learn not only how to perform calculations but also how to handle different data types. In this guide, you will turn to the basic operators necessary for mathematical calculations. Let's begin with the essential aspects of these operators.
Key insights
Rechenoperatoren in Java enable basic mathematical operations. You will learn to differentiate between arithmetic and string-based concatenations and understand the consequences of using different data types.
Step-by-Step Guide
1. Understand operators in Java
Java uses various operators to perform calculations. The most commonly used include addition (+), subtraction (-), multiplication (*), and division (/). You will learn how each of these operators works and how they can be applied in your code.
2. Create a Java class for the arithmetic operations
First, you need to create a new class. For example, name this class RechenOperatoren. In this class, you will write your main method, which starts your program.
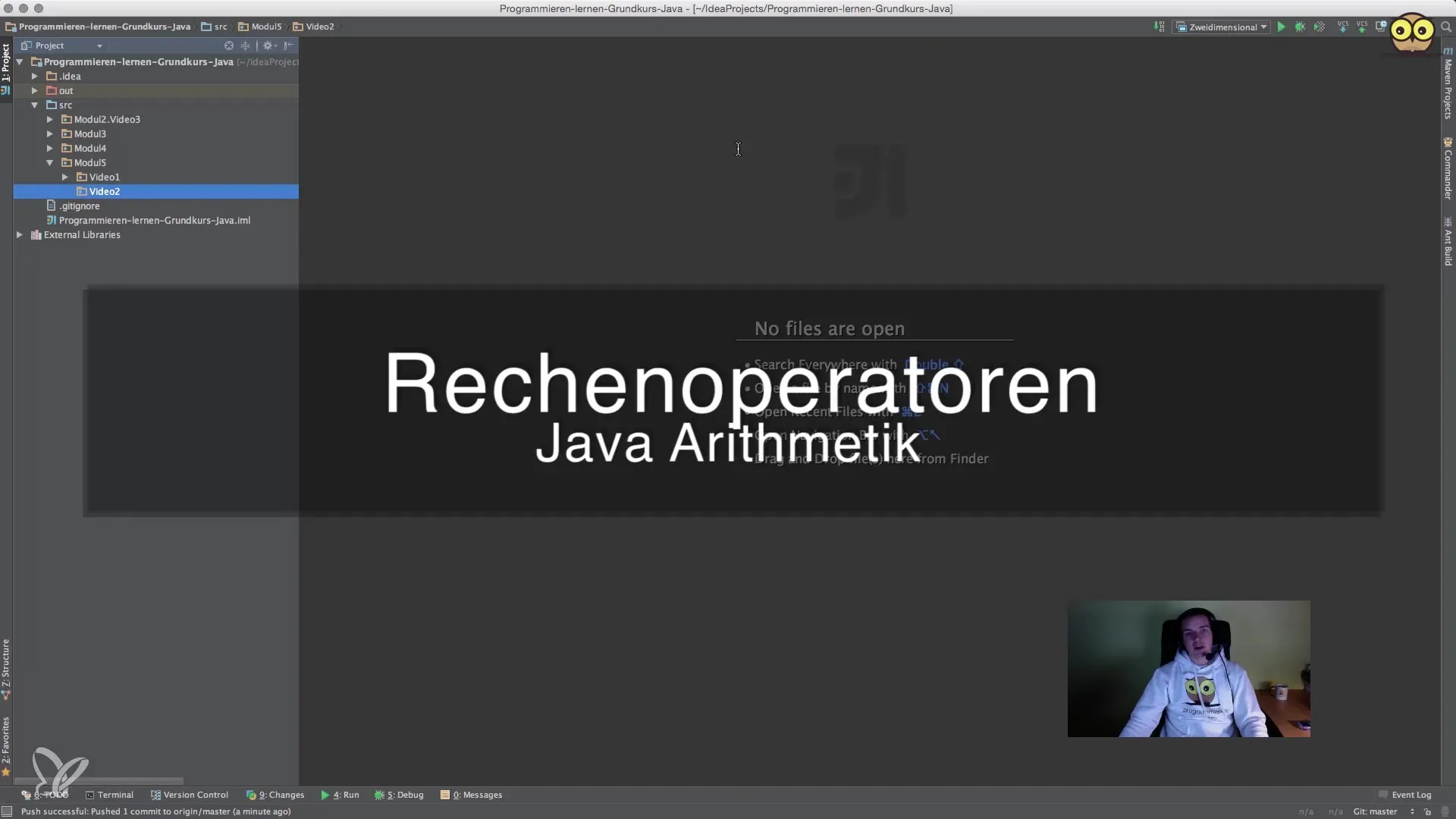
3. Perform simple addition
With the plus sign (+), you can add two numbers together. Create variables for the two operands, for example, int operand1 = 2; and int operand2 = 3;. Then you can calculate a result by adding the two operands together.
Don't forget to output the result using the System.out.print() method.
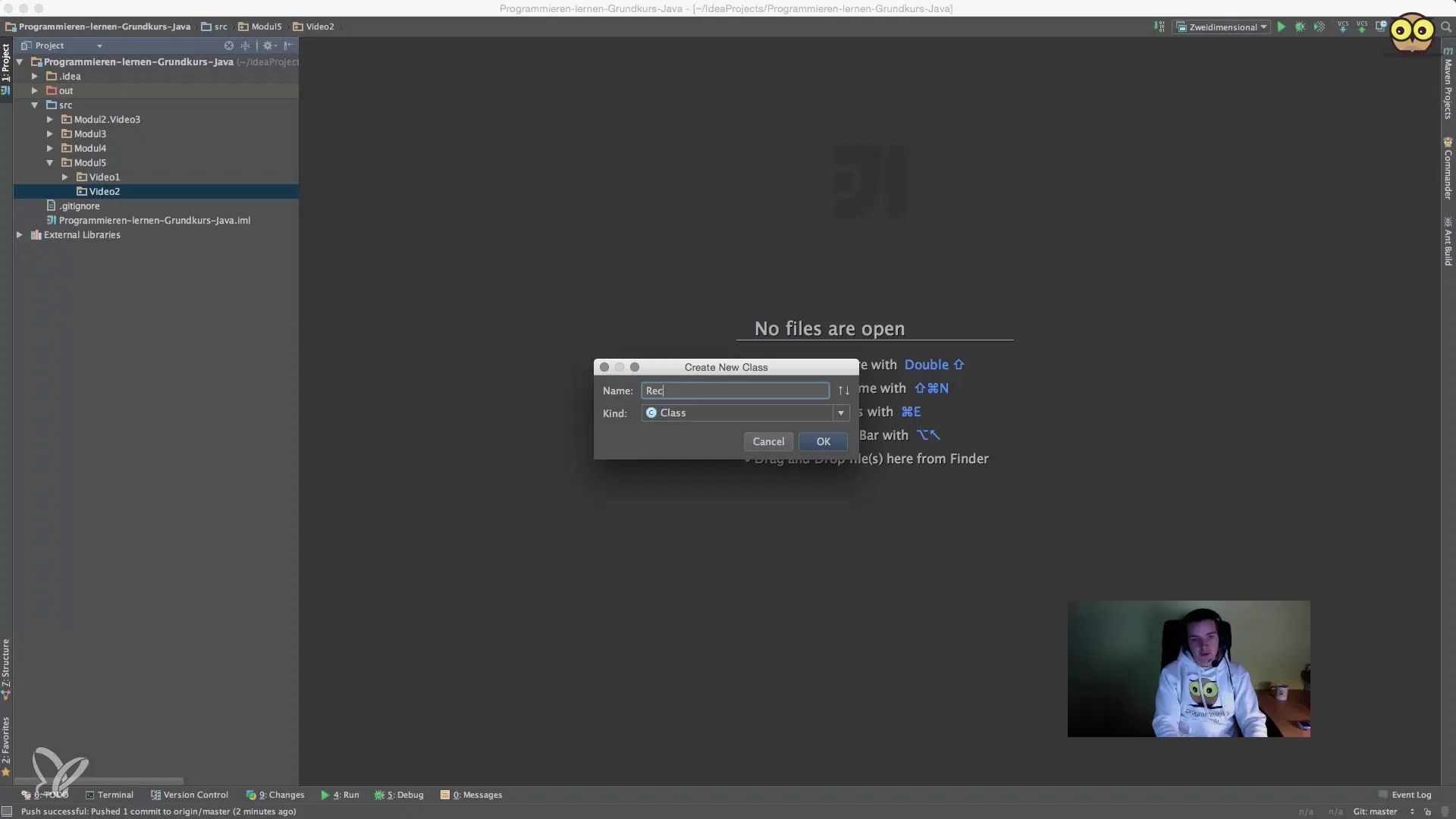
4. Apply subtraction
The minus operator (-) works similarly to the plus operator. Again, define two operands and then perform the subtraction. For example, you can calculate as follows:
And you should print this result as well.
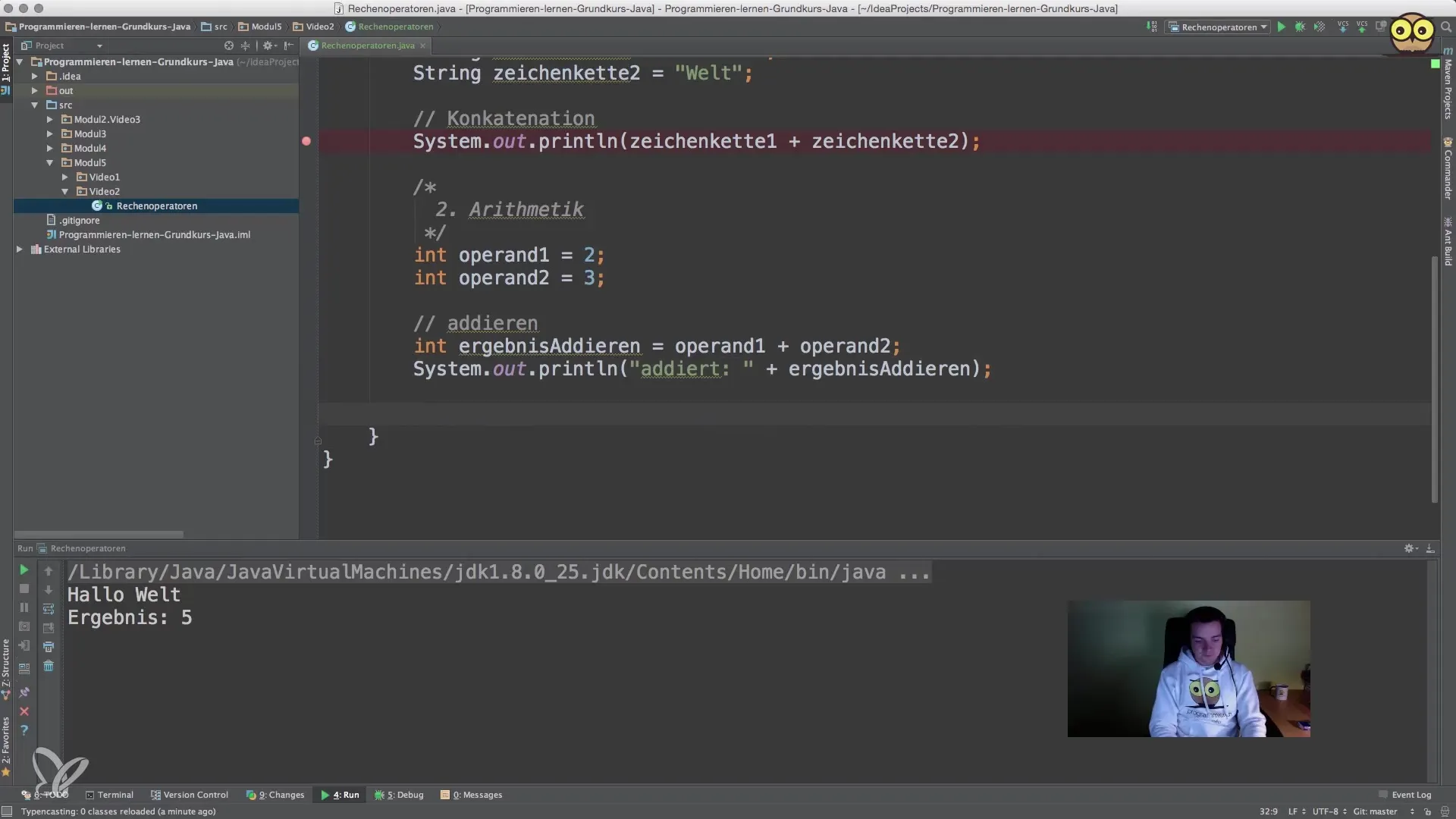
5. Multiplication
Multiplication in Java is performed with the asterisk (*). You can use the previously defined operands to calculate the product.
Be sure to output the result using the print method.
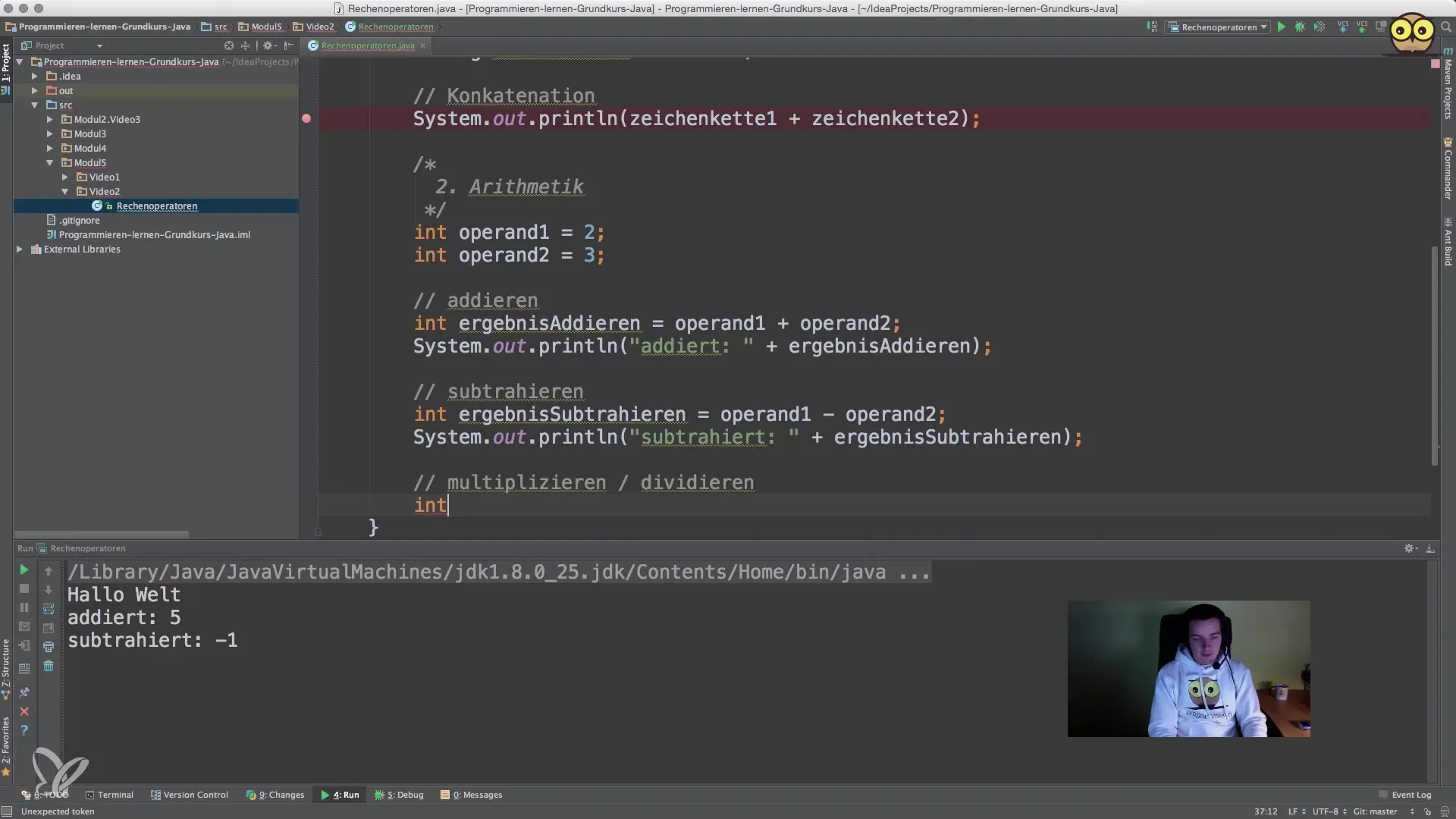
6. Perform division
Divide the values using the forward slash (/). Here, it is important to pay attention to the data types you are working with. Dividing integers often results in an integer value, and decimal values may be discarded. Adjust this by using a double value to achieve more accurate results.
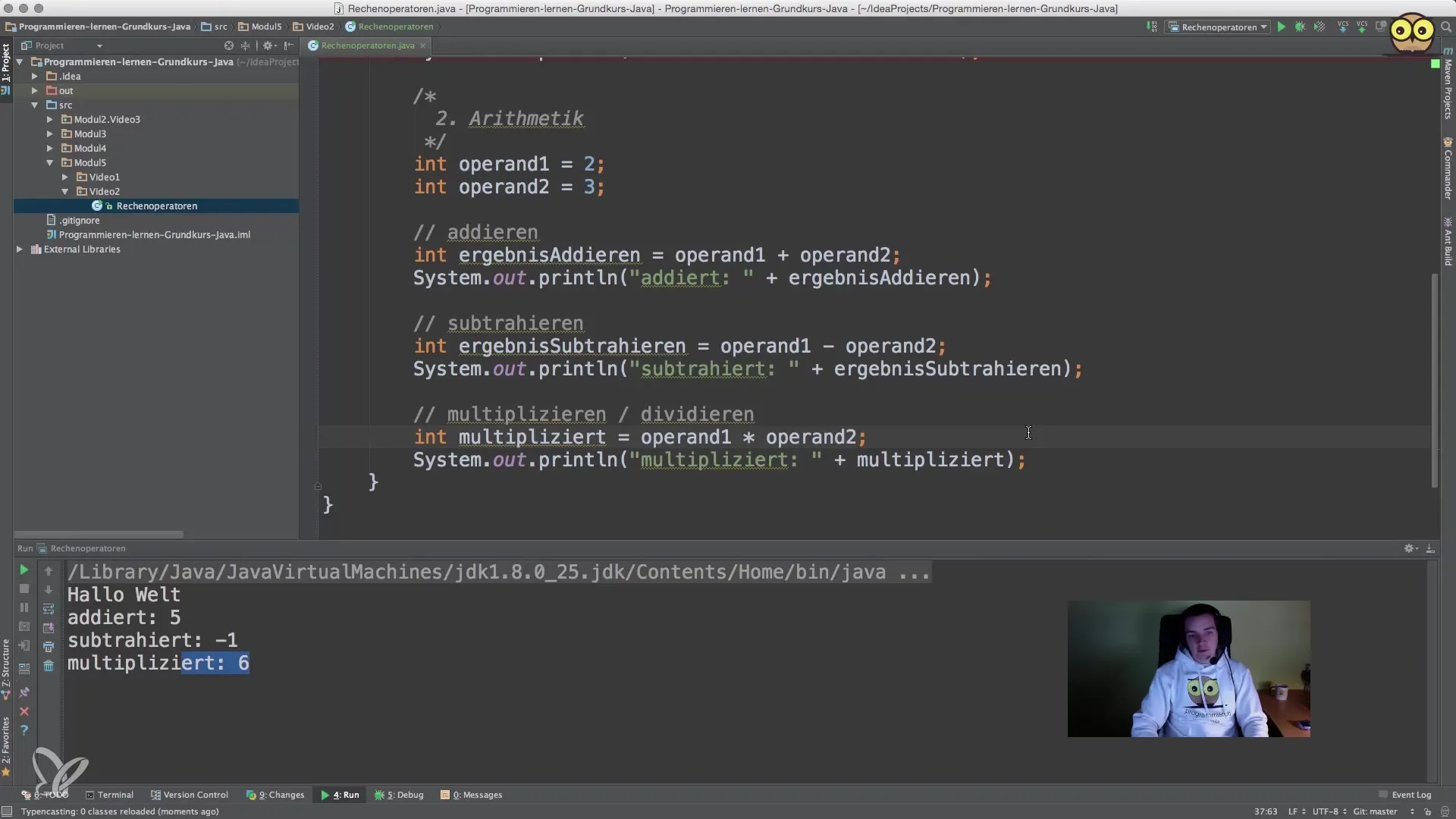
7. Use the modulo operation
With the modulo operator (%), you can determine the remainder of a division. For example, you can use 17 % 4 to see what the remainder is. This technique is useful for determining whether a number is even or odd.
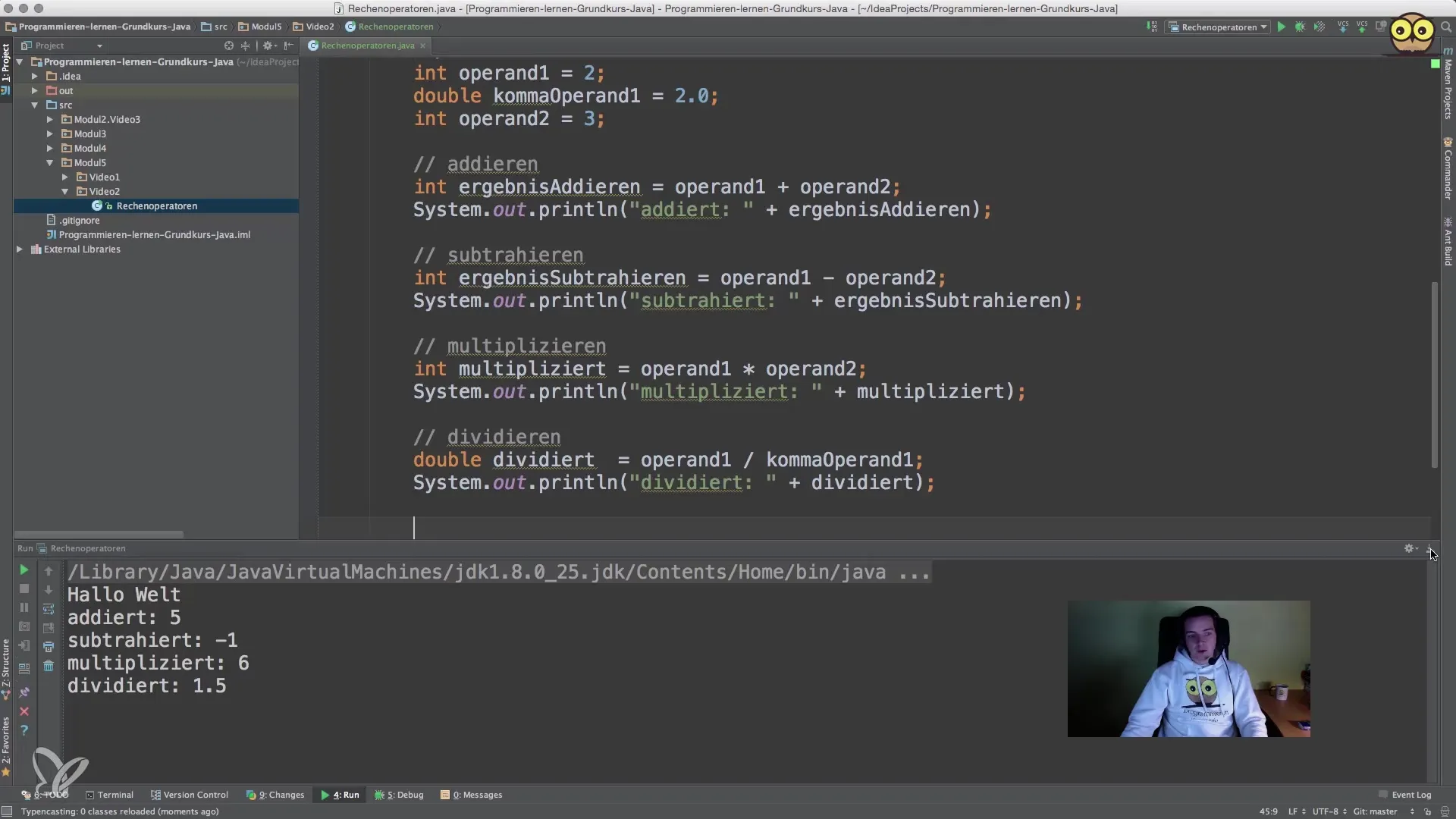
8. Summary of arithmetic operations
To visualize your arithmetic operations, you can implement a type of viewer option in your code that displays the results of the various calculations. This will help you maintain a clear overview of the results.
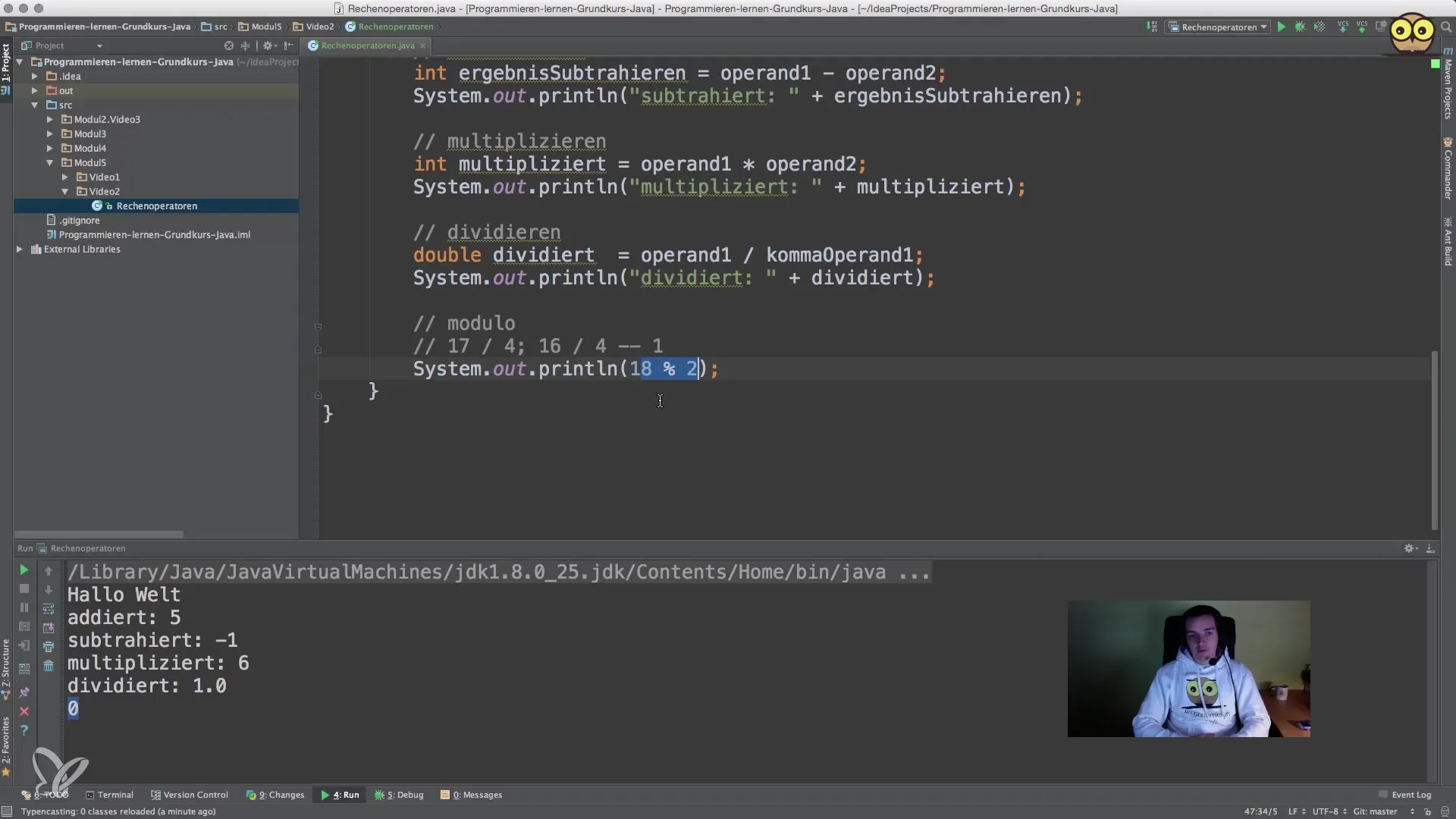
Summary – Arithmetic operations in Java: Basics for beginners
In this guide, you have learned the key arithmetic operations in Java. Understanding the various operators and their correct application is crucial for creating effective programs and avoiding common mistakes.
Frequently Asked Questions
How do I perform division in Java?You use the / symbol. Make sure to choose the data type accordingly to get decimal points.
What does the modulo operator stand for?The modulo operator % shows the remainder of a division and can be used to check even and odd numbers.
Can I add strings?Yes, with the plus operator you can concatenate strings, which is called concatenation.
What happens when dividing integer values?When dividing two integer values, the decimal part is discarded. To get accurate results, use double.
Why does division sometimes yield an unexpected result?This can happen when you divide two integers. Make sure to define at least one of the operands as double to get precise values.