Typical programs work with data that must be entered and outputted by the user. In this tutorial, we explore how you can process user inputs in Java and effectively design outputs. There are various ways to collect or provide information. In this context, the distinction between outputs to the console and error outputs plays an important role. You will also learn how to read data efficiently, whether through the console or graphical user interfaces.
Key Takeaways
- Java offers several ways to handle data entry and output.
- It is important to separate outputs from errors.
- The Scanner is a useful tool for reading user inputs.
Basic Output with System.out
The first step in working with outputs in Java is to use System.out, which represents the standard output. With the PrintStream class, which represents System.out, you can send outputs to the console.
To create a text output, you can simply use System.out.print or System.out.println. println ends the line after the output, while print does not. If you want the next output to continue on the same line, you use print.
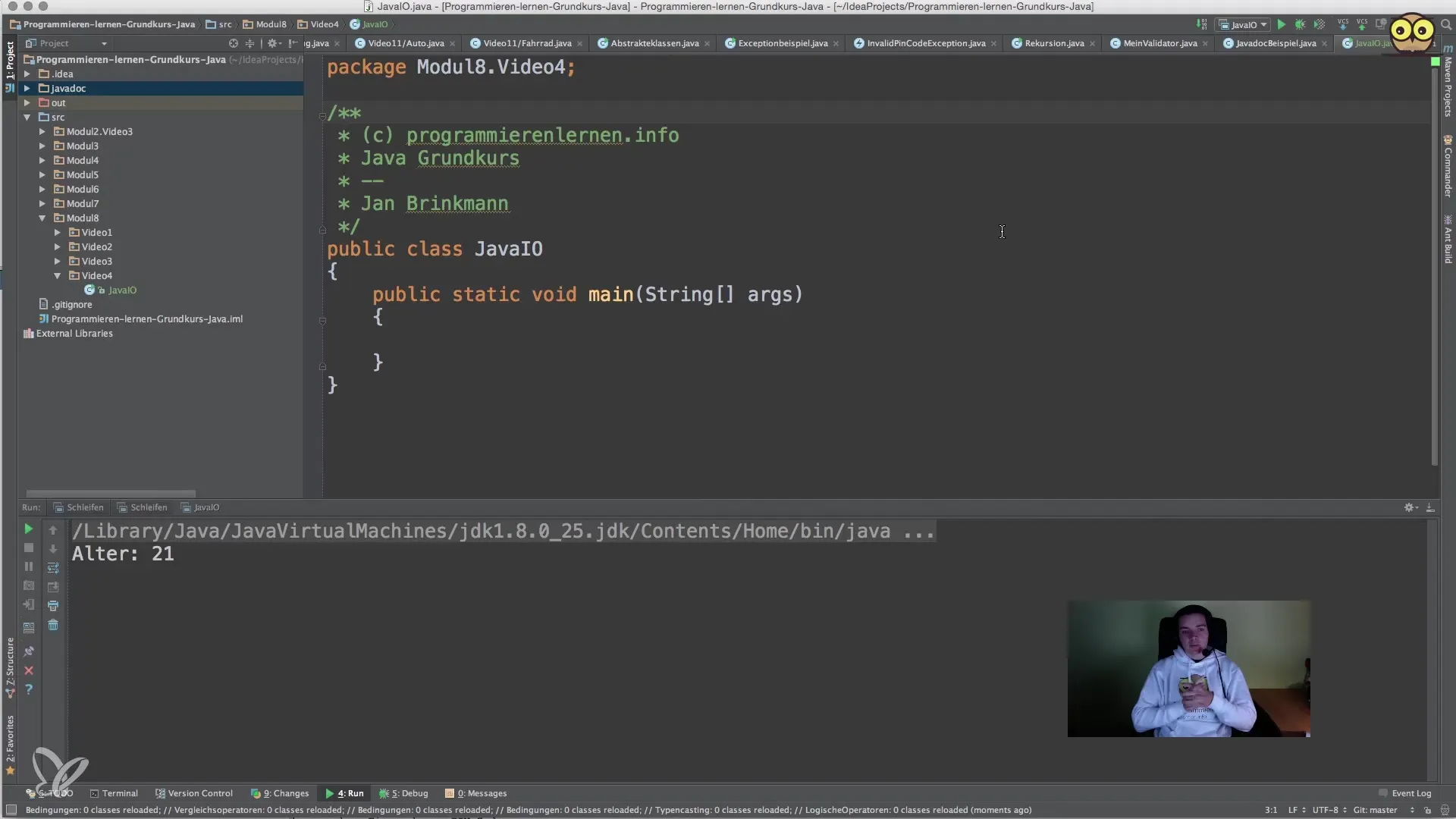
You can run the program like this:
This output will be displayed on the console, and you can modify the program to output more information.
Error Outputs with System.err
For error outputs, it is advisable to use System.err. This has the advantage of clearly separating errors from regular outputs. Errors can thus be visually highlighted, for example, by using a different color in the console (often red).
If an error occurs, you can output a specific error with System.err.print or System.err.println:
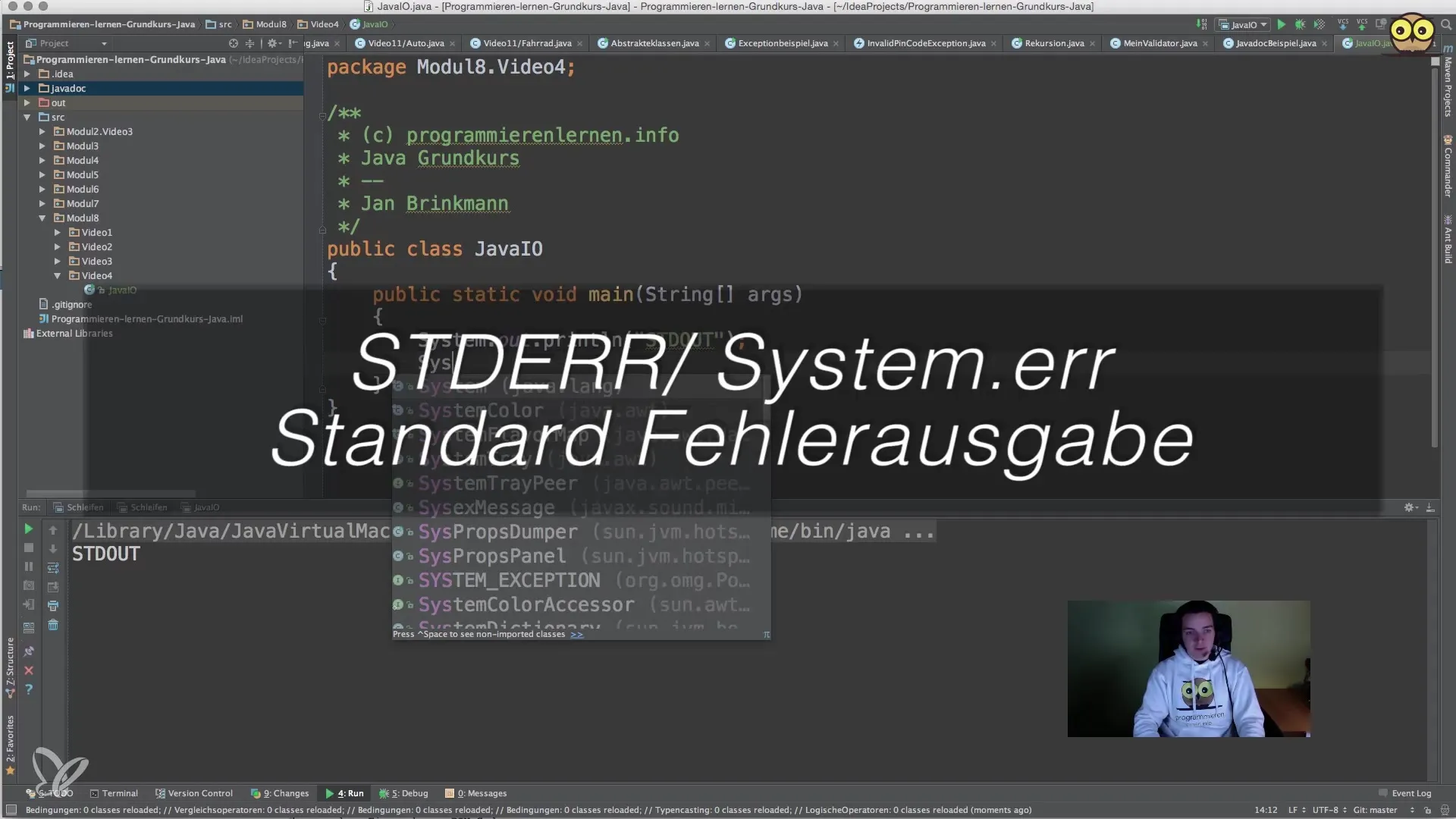
Here is an example of an error message:
By separating regular outputs from error messages, you can improve the readability and traceability of outputs in your program.
Inputs with System.in and the Scanner
To obtain inputs from the user, we use System.in. It is advisable to use the Scanner class from the java.util package. Scanner simplifies reading data, as it automatically recognizes different data types and returns the input as a String.
First, you need to import the Scanner:
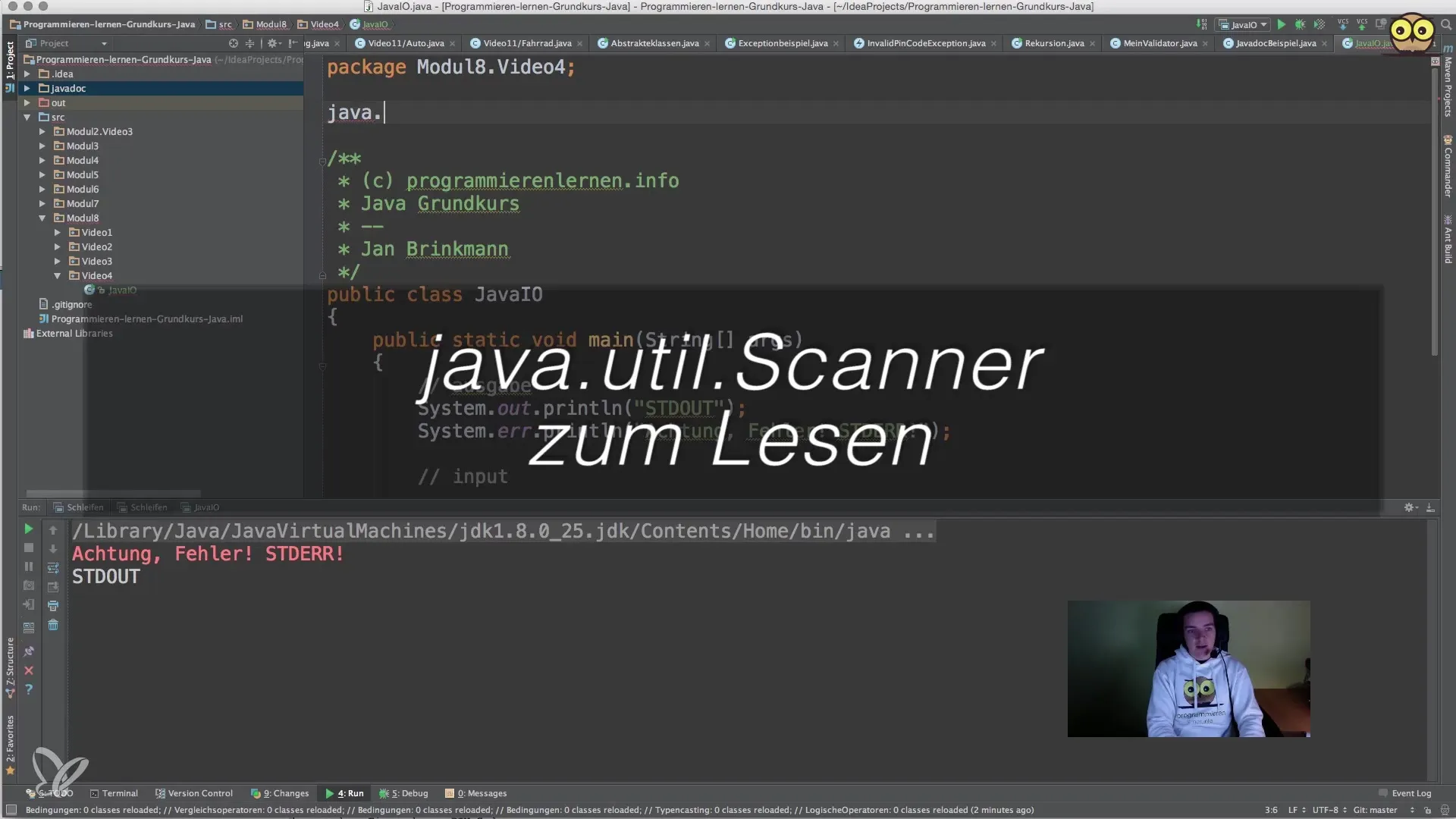
Then you can create an instance of the Scanner and pass System.in:
Now you have the opportunity to read inputs in your program.
Capturing User Inputs
To retrieve data from a user, you can use nextLine(). This allows capturing an entire line as a String. A typical example would be asking for the user's age.
Here is an example of user input:
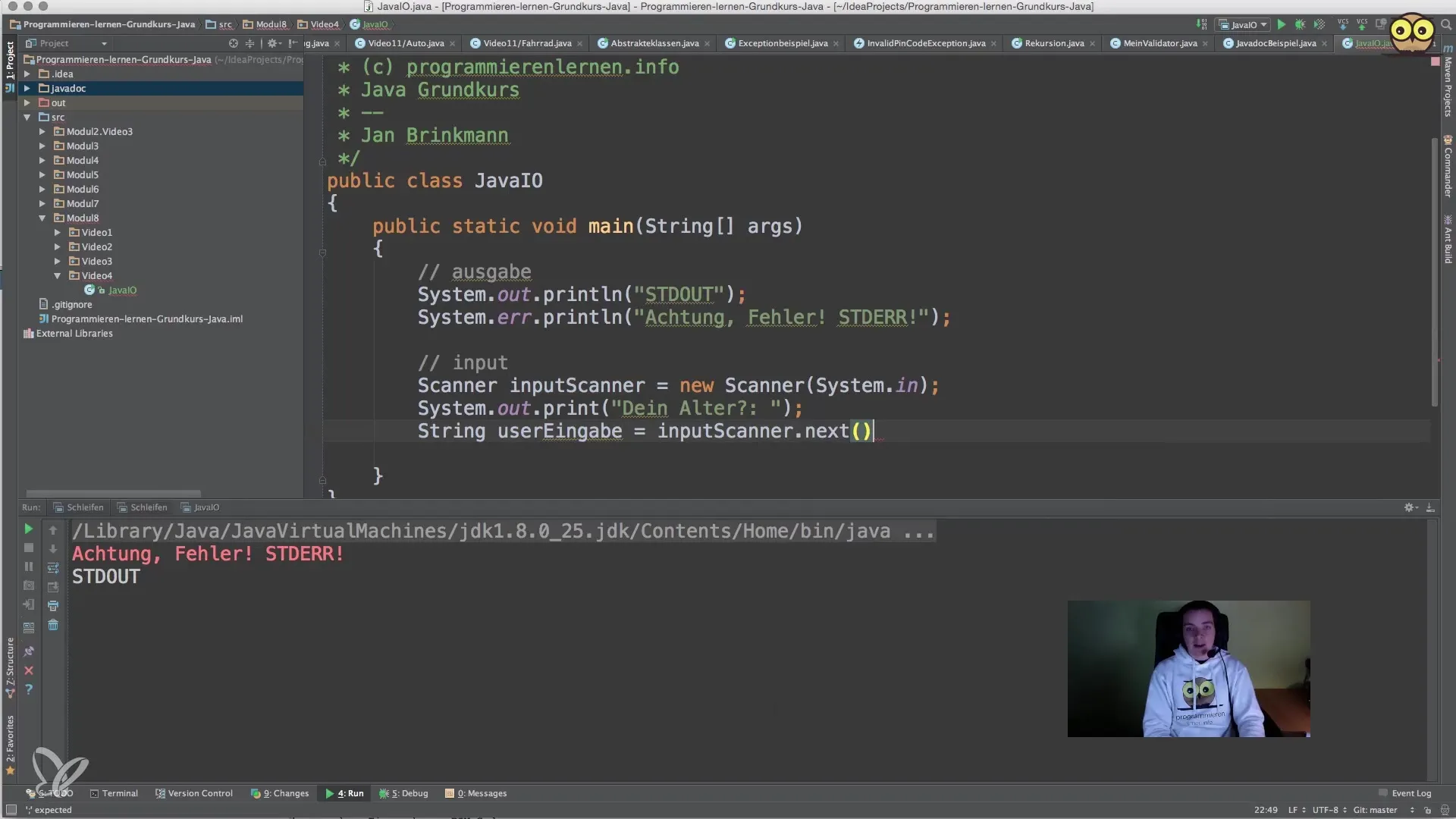
In this example, the user is prompted to enter their age, and then the program outputs the entered information.
User Interactions with Graphical Dialogs
If you want to use a graphical user interface (GUI), you can utilize the JOptionPane class from the javax.swing package. This allows you to capture inputs in a standard dialog window, which can be somewhat more user-friendly.
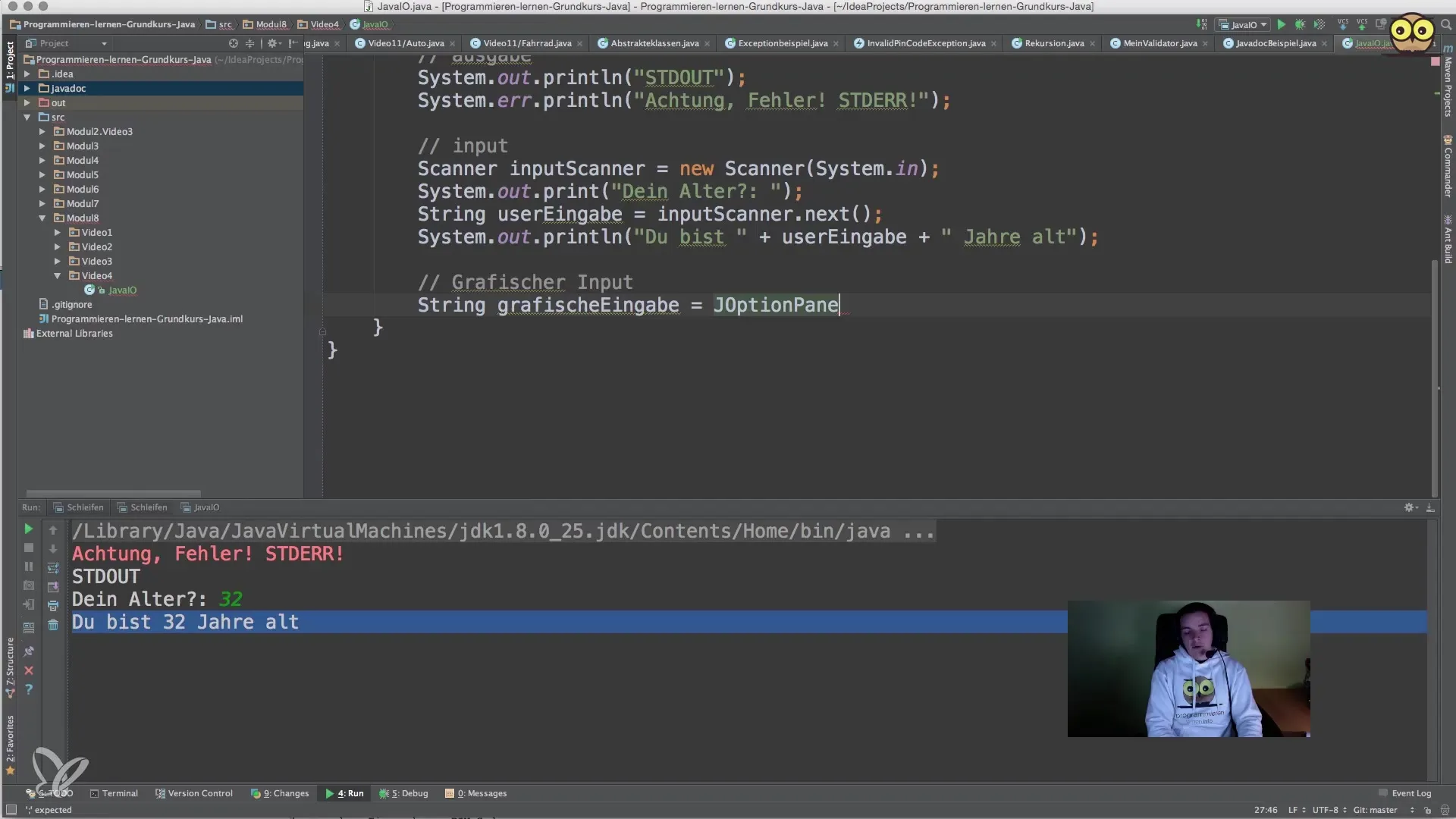
This shows a graphical dialog where the user can enter their age.
Validating User Inputs
It is important to validate the inputs coming from the user. You should ensure that the age is indeed in an acceptable format, such as an integer. You can do this by attempting to convert the string to a number and working with exceptions.
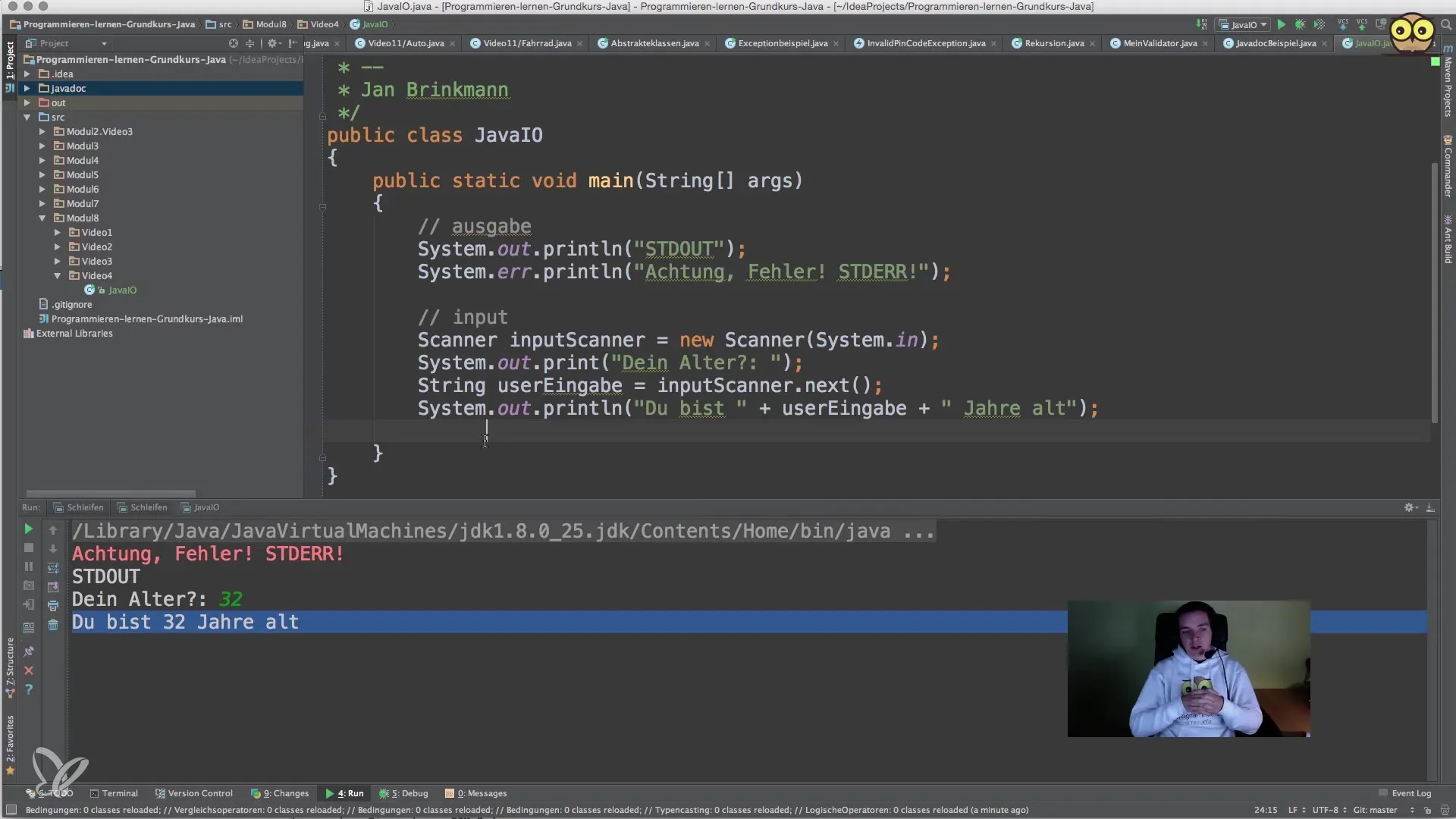
By implementing these validations, you protect your program from unexpected inputs.
Summary – Optimizing Input and Output in Java
You have now learned the fundamental concepts of input and output in Java. The distinction between standard outputs and error messages is fundamental to creating clarity in program communication. Moreover, using the Scanner and the option to implement graphical dialogs offer flexible solutions for data input.
Frequently Asked Questions
Which class do I use for standard output?You use System.out to display outputs in the console window.
How can I output error messages?Use System.err to output error messages to separate them from regular outputs.
How do I capture user inputs in the console?The Scanner from java.util is ideal for capturing user inputs from System.in.
Can I use graphical input dialogs?Yes, through JOptionPane from javax.swing you can use graphical dialogs for input.
Do I need to validate user inputs?Yes, validating inputs is important to avoid errors and unexpected inputs.