The goal of this guide is to show you how to create a graphical user interface (GUI) in Java that responds to user input. We will specifically look at using JFrame, buttons, and text fields to enable seamless interactions. It is important that the interface is not only appealing but also provides functional feedback to the user.
Key Insights
- When developing graphical applications with Java, JFrame should be configured sensibly.
- The exit operation is crucial for properly closing the program.
- Adding user inputs, such as buttons and text fields, enhances the interactivity of the application.
- Implementing the WindowListener enables handling of window events, such as closing the window.
Step-by-Step Guide
1. Creating the JFrame
First, you should create a simple JFrame and configure the most basic parameters. It is important to give the window a size and make it visible. In your main program, you can proceed as follows to create the frame:
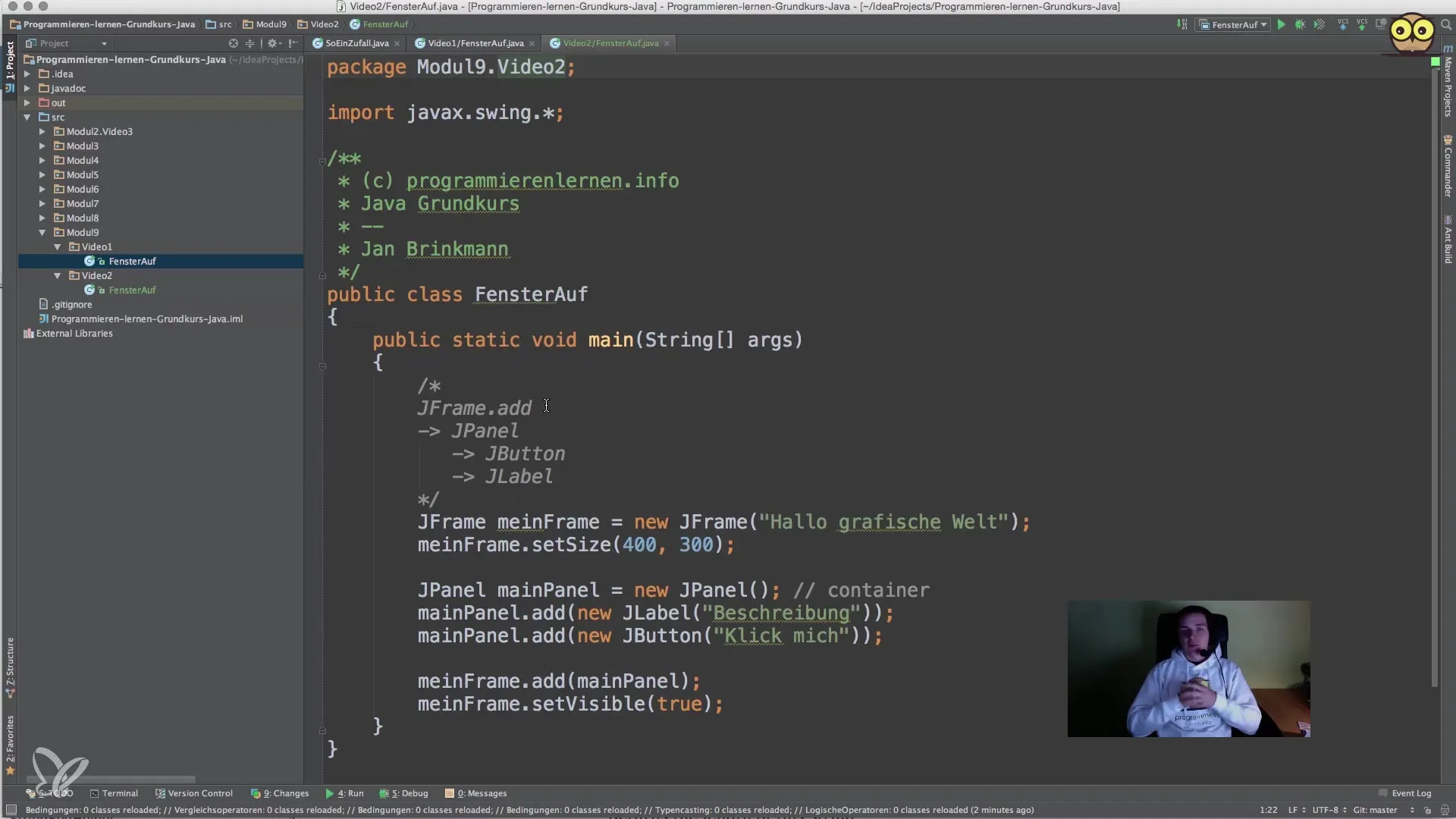
2. Setting the Close Operation
A common problem when using JFrame is properly closing the program. To ensure that the program actually terminates when closing the GUI, the DefaultCloseOperation must be set correctly. Here, we use JFrame.EXIT_ON_CLOSE to completely close the program when the window is closed.
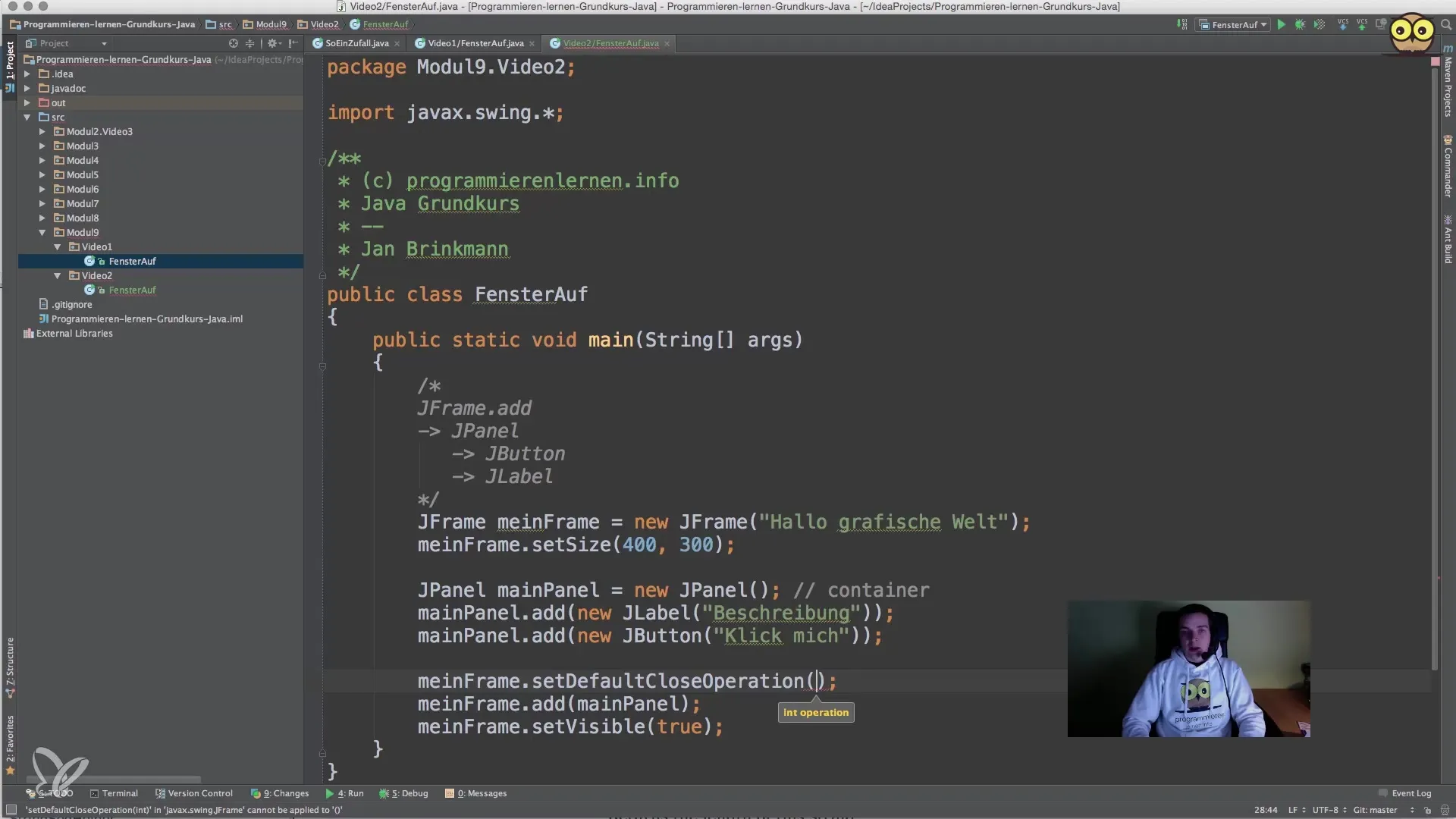
3. Using Panels
A JFrame consists of a content pane that contains most of the graphical elements. Instead of using add directly, it is often clearer to explicitly set the content pane. This way, you can maintain control over where your components are placed.
4. Creating a Custom JFrame Class
Instead of instantiating a new JFrame every time, it makes sense to create your own class that inherits from JFrame. This allows for clearer management and adding additional features.
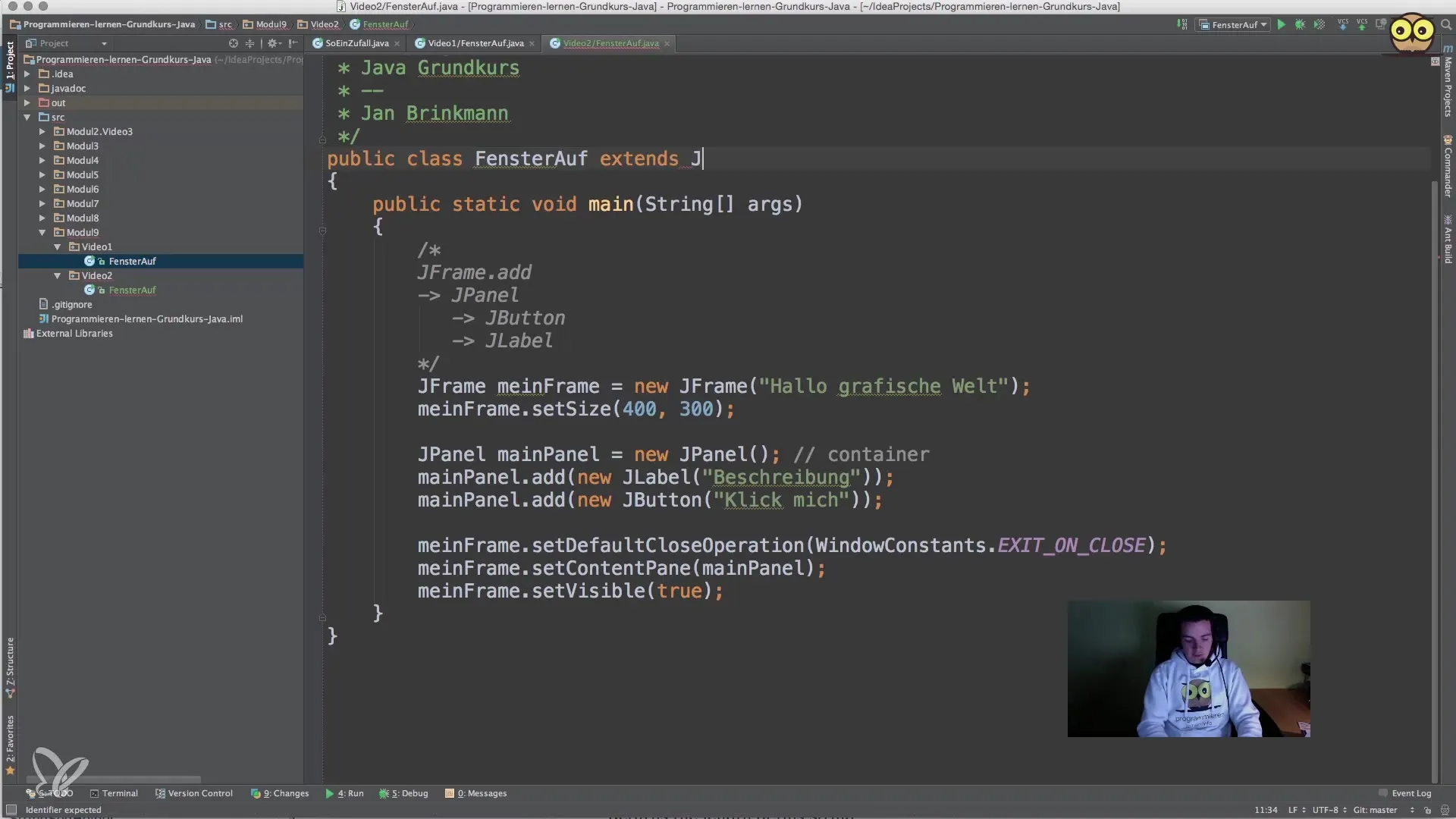
5. Implementing the Constructor
Once you have created your custom JFrame class, you need to implement the constructor to set the window title correctly. This is achieved by calling super(title), which invokes the constructor of the superclass and sets the window title.
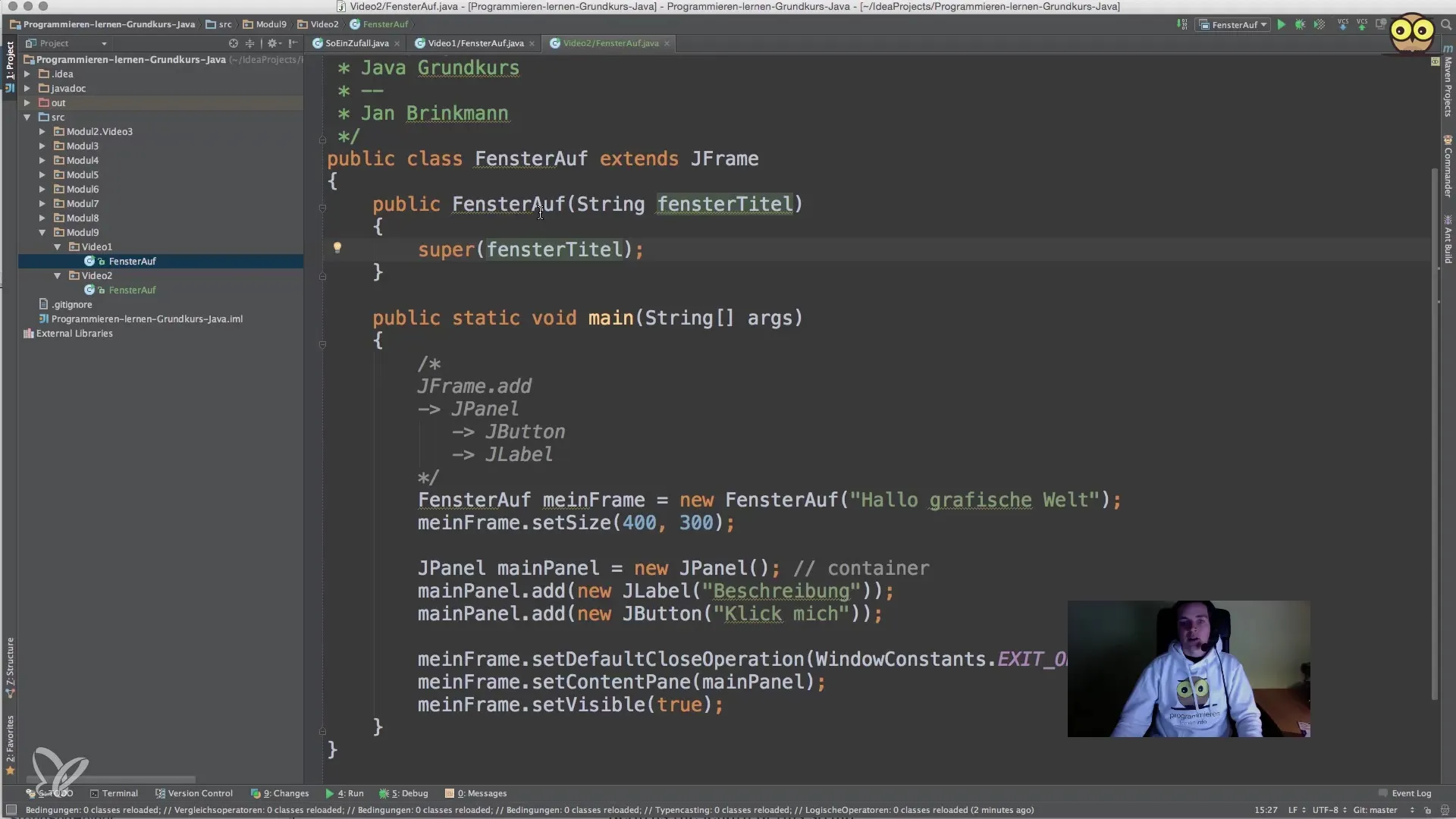
6. Adding a Panel
Within the JFrame class, it is helpful to create one or more panels to structure the user interface. Create a new JPanel and set it as the content pane. This will make handling layouts and other user interface elements easier.
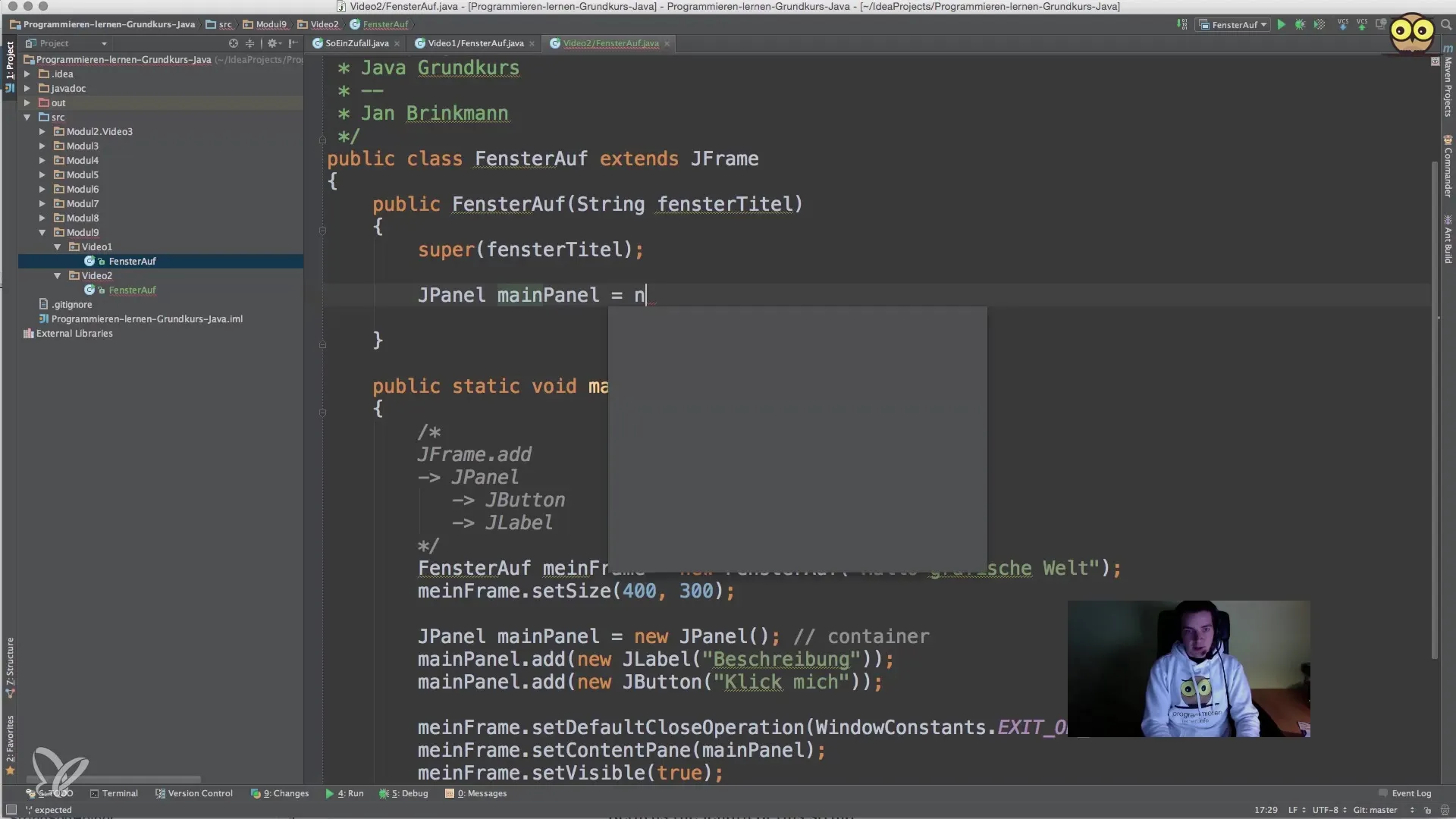
7. Implementing the WindowListener
To respond to window events, implement the WindowListener interface in your JFrame class. You will need to define various methods such as windowClosing to customize the default behavior when closing the window. Serious applications exit the program cleanly by calling System.exit(0).
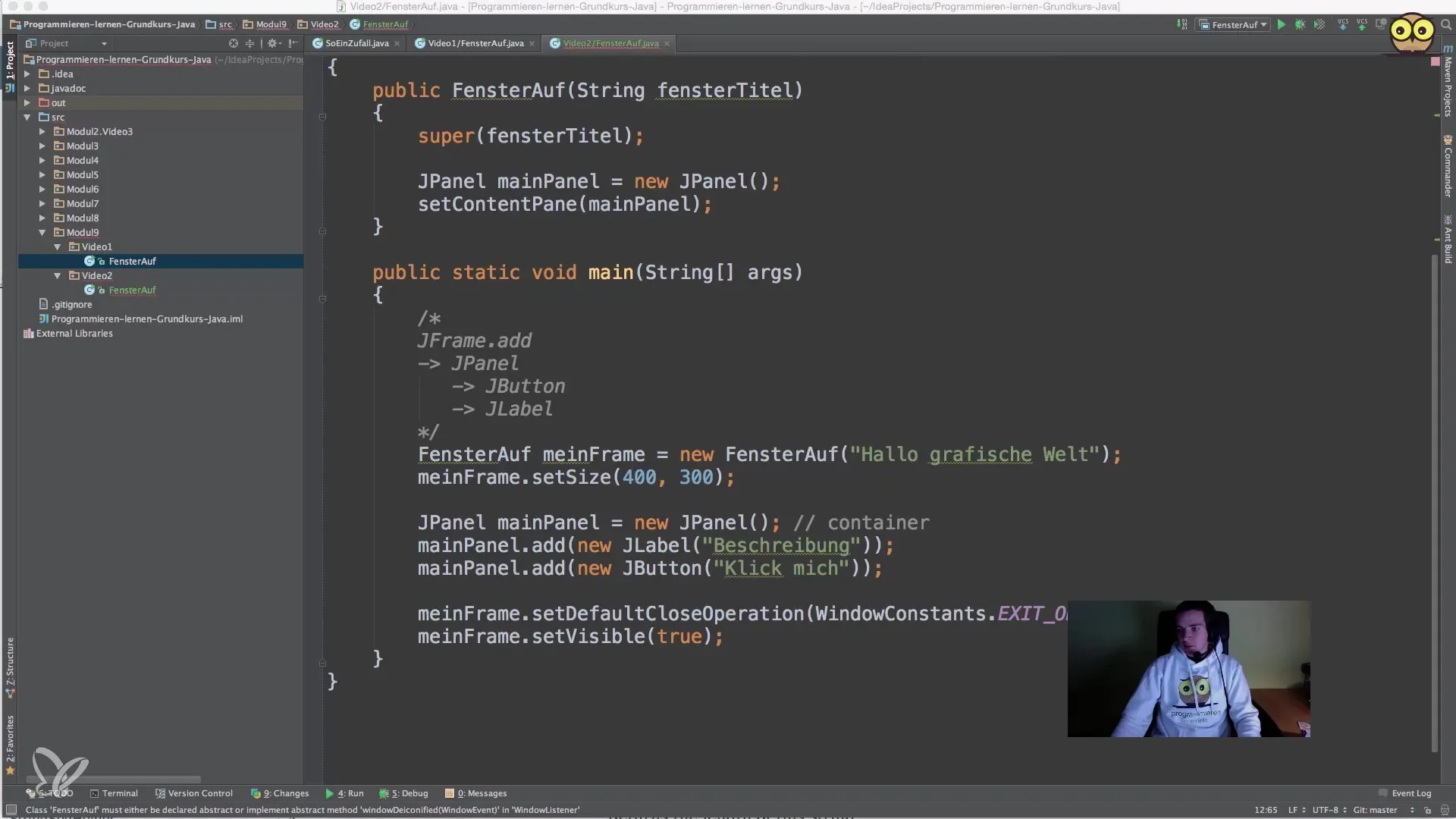
8. Adding User Input
To increase interactivity, we add a text field (JTextField) and a button (JButton) to the GUI. In this case, using attributes within the class is crucial since this access is needed throughout the lifecycle of the instance.
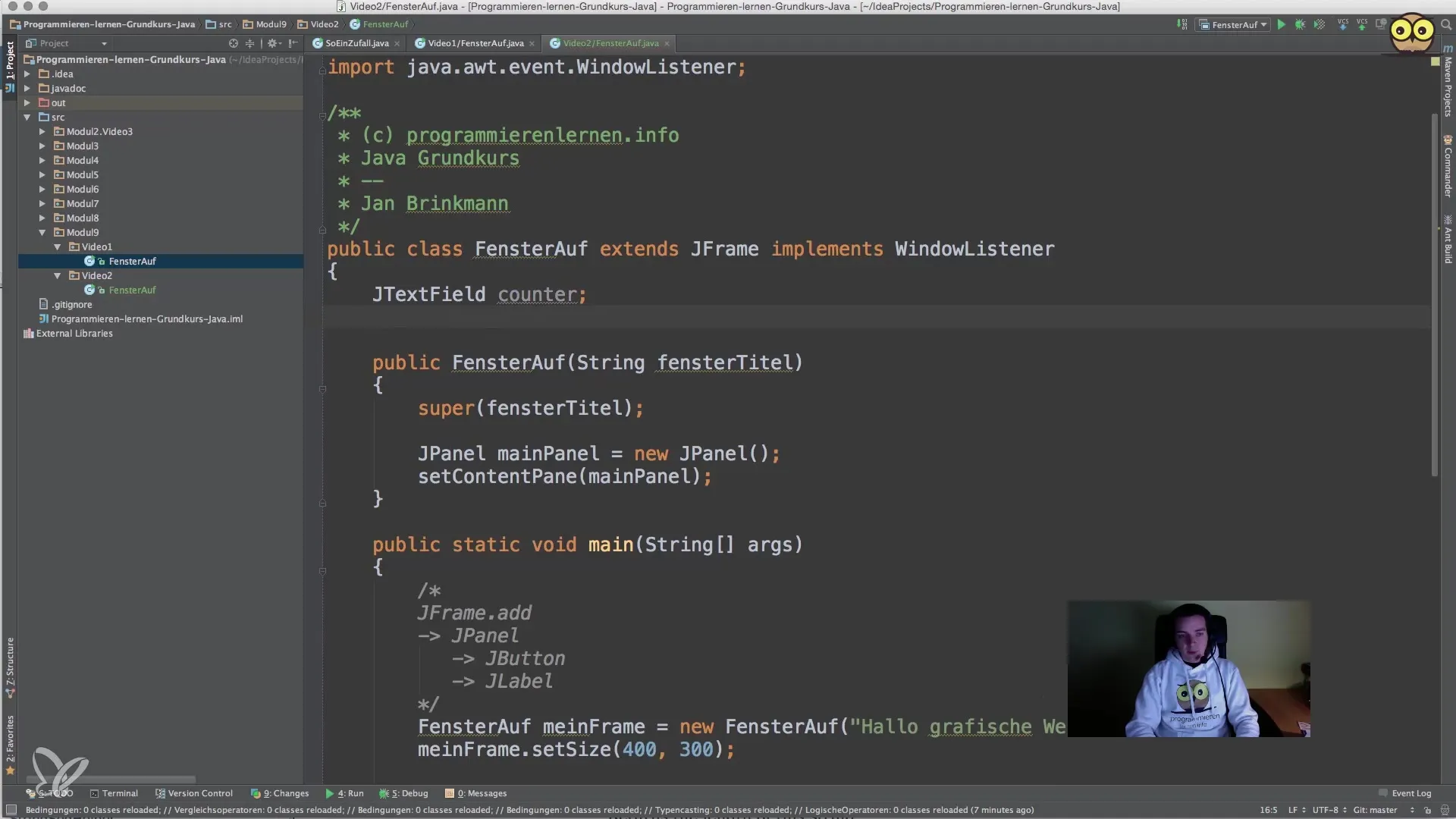
9. Interactive Functionality of the Button
Now that we have a button, it’s time to assign an action to it. This is usually done through an ActionListener that responds to the button click. You can implement the logic to increase the value in the text field when the button is clicked.
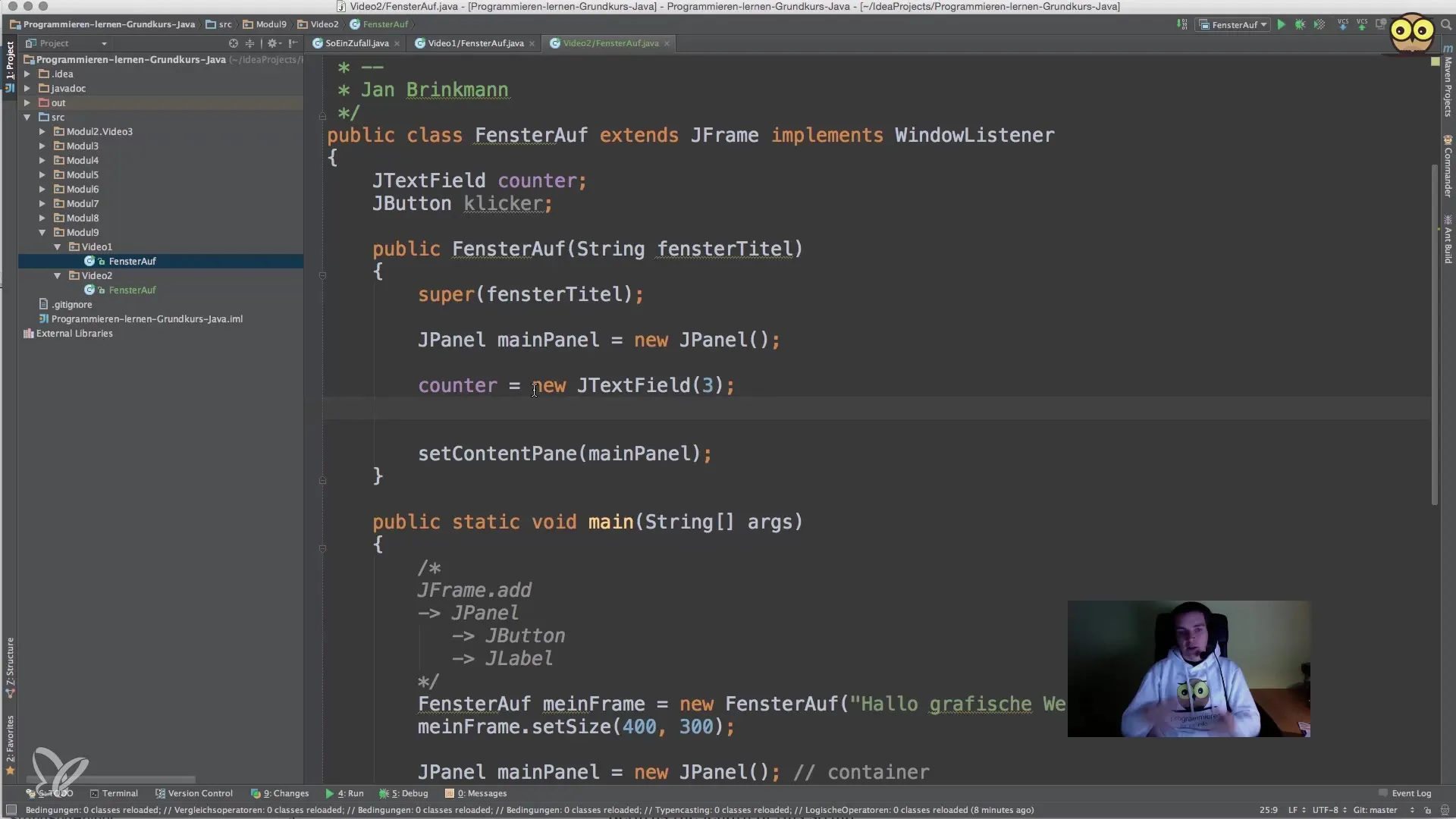
10. Finalizing the Application
After you have added all components, make sure you save the changes and test the application correctly. This means you need to ensure that clicking the button updates the counter correctly and that the window closes properly. Start the application and test the interactions yourself.
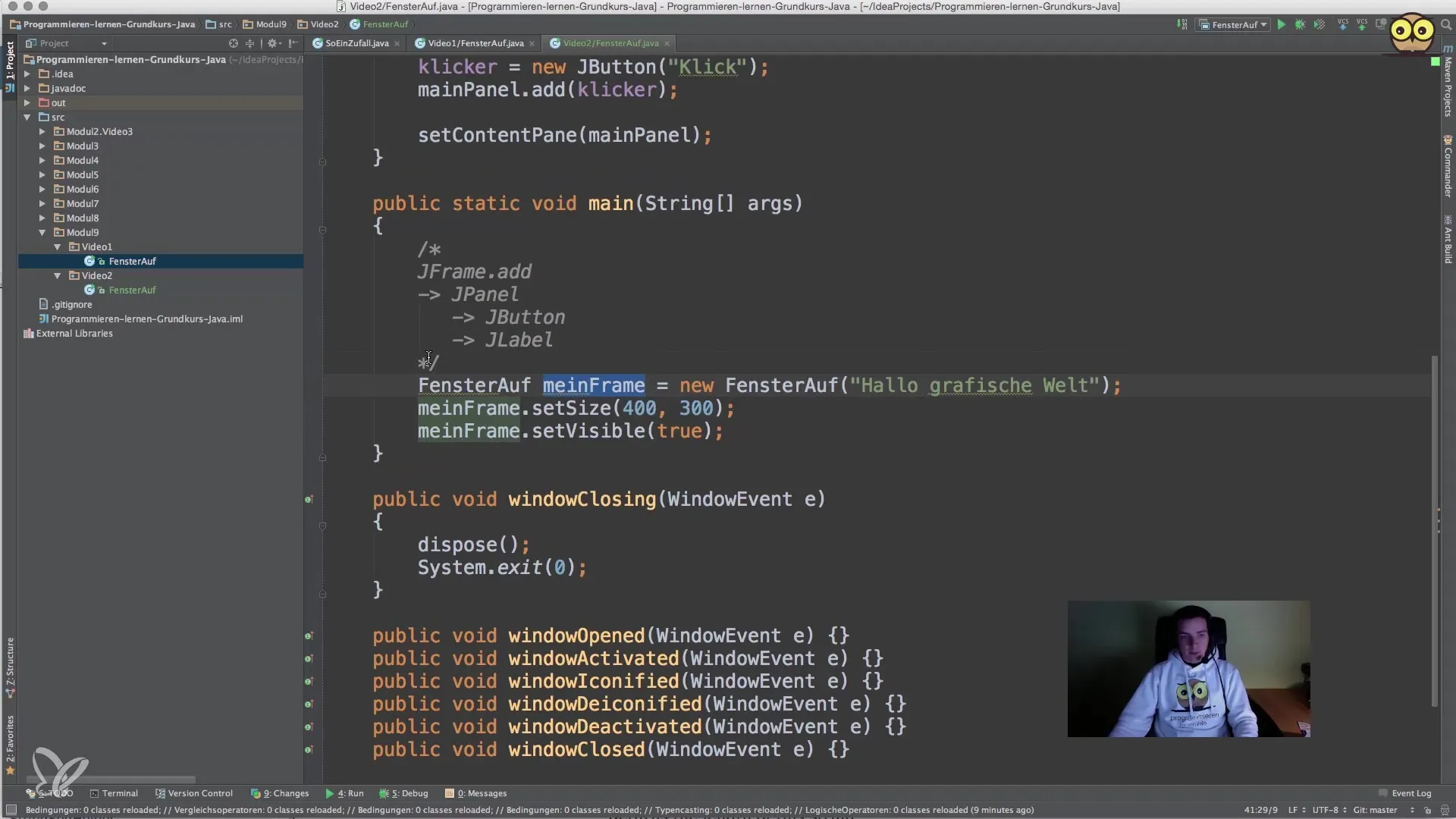
Summary – Implementing User Interactions in Java with JFrame
In the steps above, you learned how to create a graphical application in Java that effectively responds to user input. From configuring the JFrame to implementing interactive elements, you have gained the tools needed to develop engaging software.
Frequently Asked Questions
What is JFrame?JFrame is a class in Java Swing that provides a window for graphical user interfaces.
How can I ensure that my application stops when closing the window?Set the DefaultCloseOperation to JFrame.EXIT_ON_CLOSE.
What is a WindowListener?A WindowListener is an interface that allows responding to events from windows and their status changes.
How do I add buttons and text fields to my GUI?Create instances of JButton and JTextField and add them to the content pane.
How do I implement the ActionListener for a button?Use addActionListener() and implement the actionPerformed() method to define the necessary logic.