Destructuring is a particularly helpful feature in JavaScript that allows you to extract values from arrays and objects into variables without writing multiple lines of code. This technique not only improves the readability of your code but also makes it shorter and more elegant. In this guide, you will learn how to effectively use destructuring to optimize your JavaScript code.
Key insights
- Destructuring allows for easy extraction of values from objects and arrays.
- You can assign variables simultaneously, making the code more readable.
- The order of variables matters for objects, but not for arrays.
- You can rename values to avoid conflicts with existing variables.
Step-by-Step Guide
Destructuring Objects
Let's start with objects. Imagine you have an object that contains two properties, x and y. First, you declare your object like this:
Here, you notice that you have to write the property obj twice. This is cumbersome and can be solved more elegantly. At this point, destructuring comes into play.
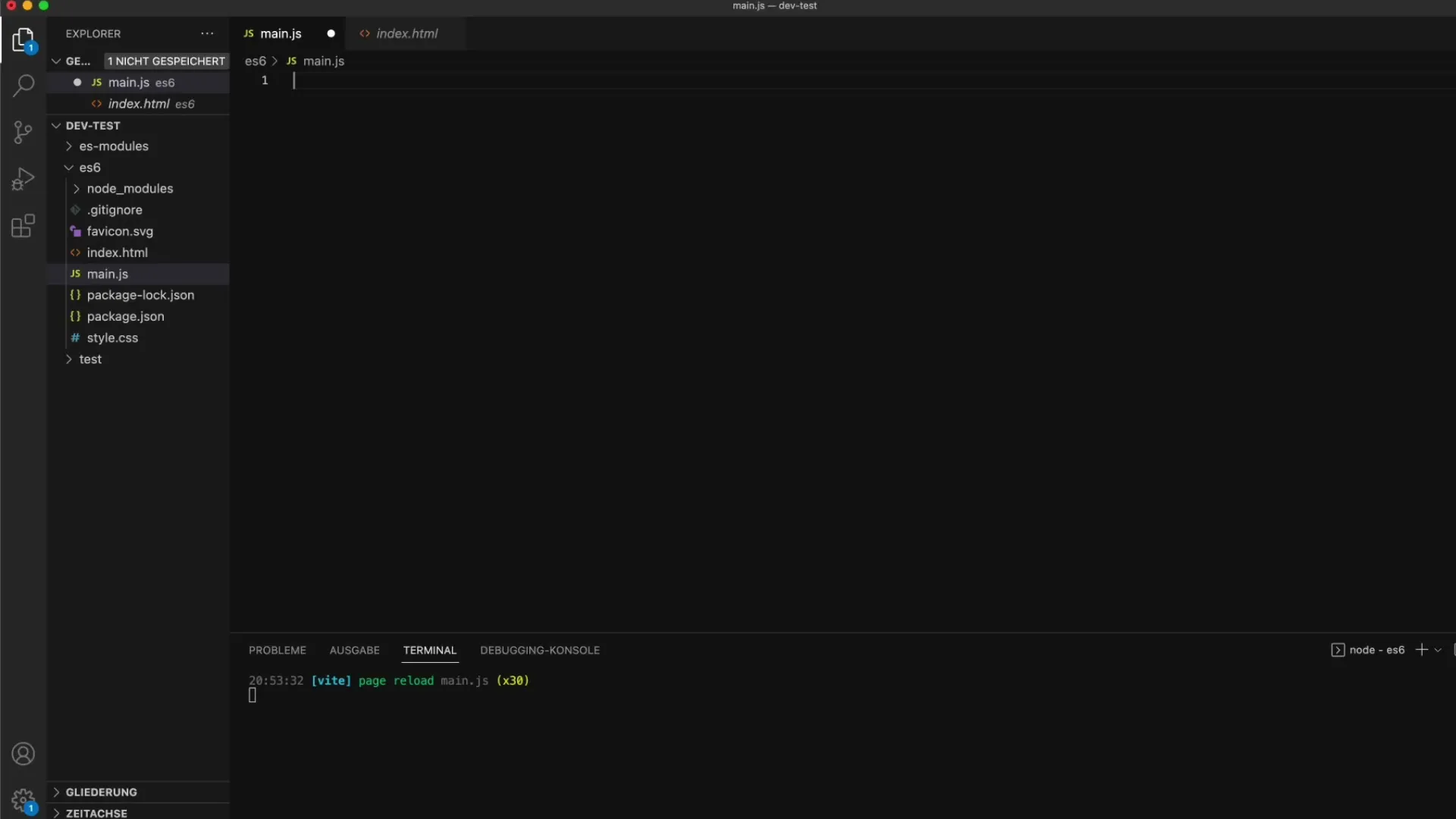
Now you've extracted x and y directly from obj and only need to use the object name once. Let’s check the results.
You will see that the results remain the same. This technique not only saves lines of code but also optimizes readability.
Renaming Variables
When using destructuring, it may happen that the properties in your returned object cause conflicts with already existing variables. In this case, you can rename the variables.
Now you have successfully renamed the properties to x1, y1, and z1 and can use them without conflicts.
Functions with Objects
Destructuring also simplifies dealing with return values from functions.
You receive the values of x and y directly without additional assignments.
Destructuring Arrays
Now let’s look at destructuring with arrays.
Here you have extracted x, y, and z from the array. Note that the order is important here and corresponds to the values in the array.
Skipping Values
It's also possible that you don’t need all values from an array.
In this case, you skip the second value.
This gives you the flexibility to only extract the values you really need.
Conclusion
Through destructuring, you can make your code not only shorter but also more understandable. Whether you work with objects or arrays, you will find that this technique saves you a lot of time and writing effort. You can easily break down the structure of objects and arrays and directly assign the required values. With the renamed variables, you can avoid conflicts and meaningfully name your variables.
Summary - Destructuring in React: A Step-by-Step Guide
Destructuring is a valuable technique in JavaScript that allows you to efficiently extract values from objects and arrays. You have now learned how to implement this helpful approach to make your code clearer and more elegant.
Frequently Asked Questions
How does destructuring work with objects?With destructuring, you can extract properties from objects and store them directly in variables.
Can I rename variables when destructuring?Yes, that is possible. You can rename the variables in the form const { property: newName } = object.
How do I handle conflicts with variable names?Use the renaming syntax when destructuring to avoid overwriting existing variables.
Does destructuring also apply to arrays?Yes, you can use destructuring with arrays by using square brackets.
Can I skip values when destructuring?Yes, that is possible. You can use commas in the assignment to skip certain values.