The use of the Rest-Operator in JavaScript is not limited to arrays but also plays an important role with objects. With this tutorial, you will learn how to efficiently use the rest operator to capture the remaining properties of an object. This is particularly useful when working with complex data structures and only needing a part of the properties.
Key insights
- The rest operator allows you to extract properties from an object and collect the rest in a new object.
- This process encourages a clear and consistent style in your code, especially when working with functions and their return values.
Step-by-Step Guide
If you want to familiarize yourself with the rest operator and object destructuring, follow these steps and practically apply what you learn.
Creating an Object
To apply the rest operator, start by defining a simple object with several properties. In this example, you will create an object with properties a, b, c, and d.
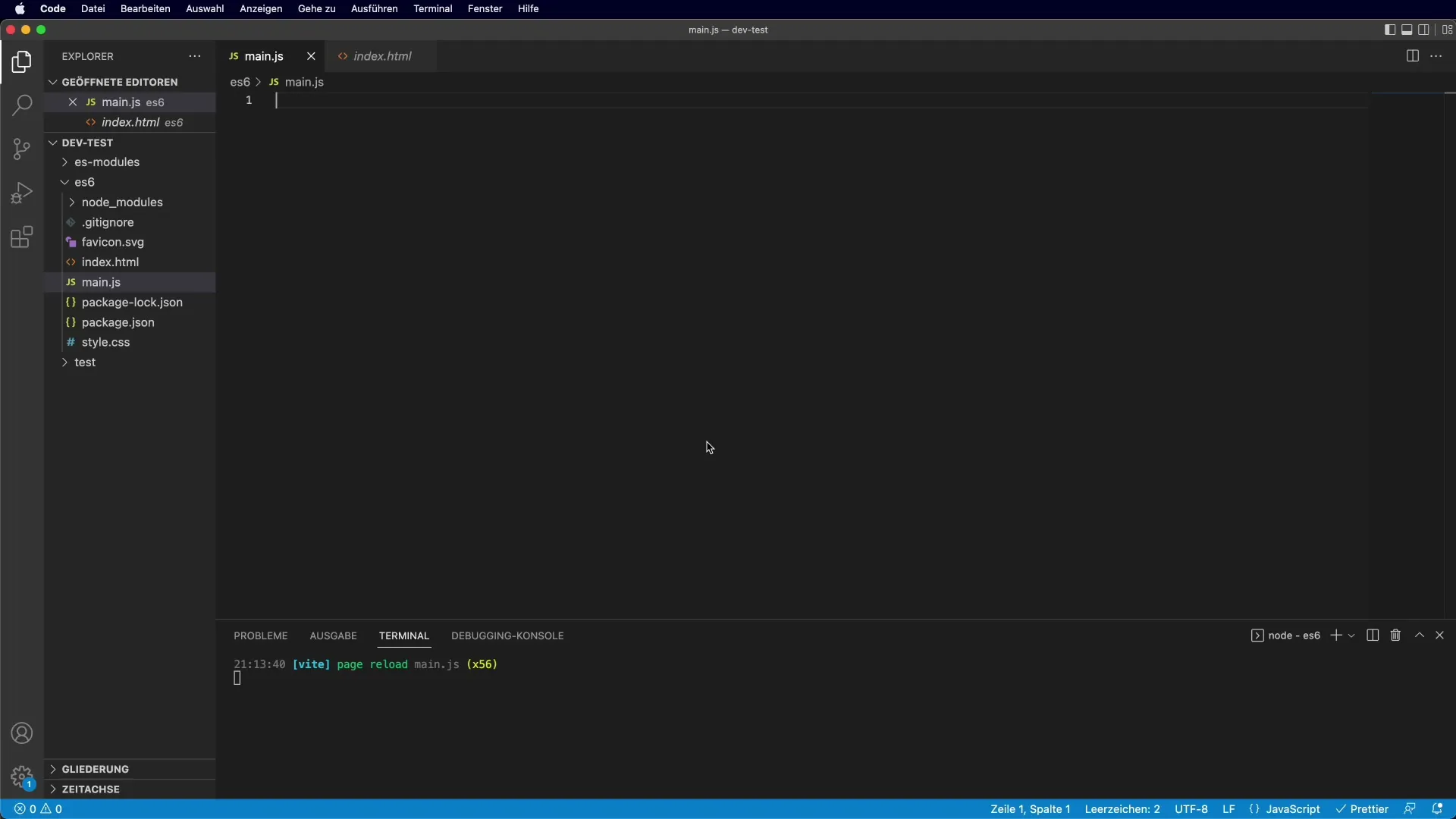
Using Object Destructuring
The next step involves using object destructuring. You want to extract one of the properties of the object. In this case, we will select c.
After running the code, you can check the console to see if the value of c is correctly outputted. This is the first important step before introducing the rest operator.
Extracting with the Rest Operator
Now comes the exciting part: the use of the rest operator. You want to extract c from the object and keep the rest of the properties as a new object. For this purpose, we use the rest operator in the curly braces.
Here, all remaining properties of obj that are not c are placed into a new object rest. You can now see that rest contains the desired properties.
Checking the Results
To ensure that everything works as expected, check the console outputs. You should see the properties a, b, and d in the rest object. This shows that the rest operator worked effectively.
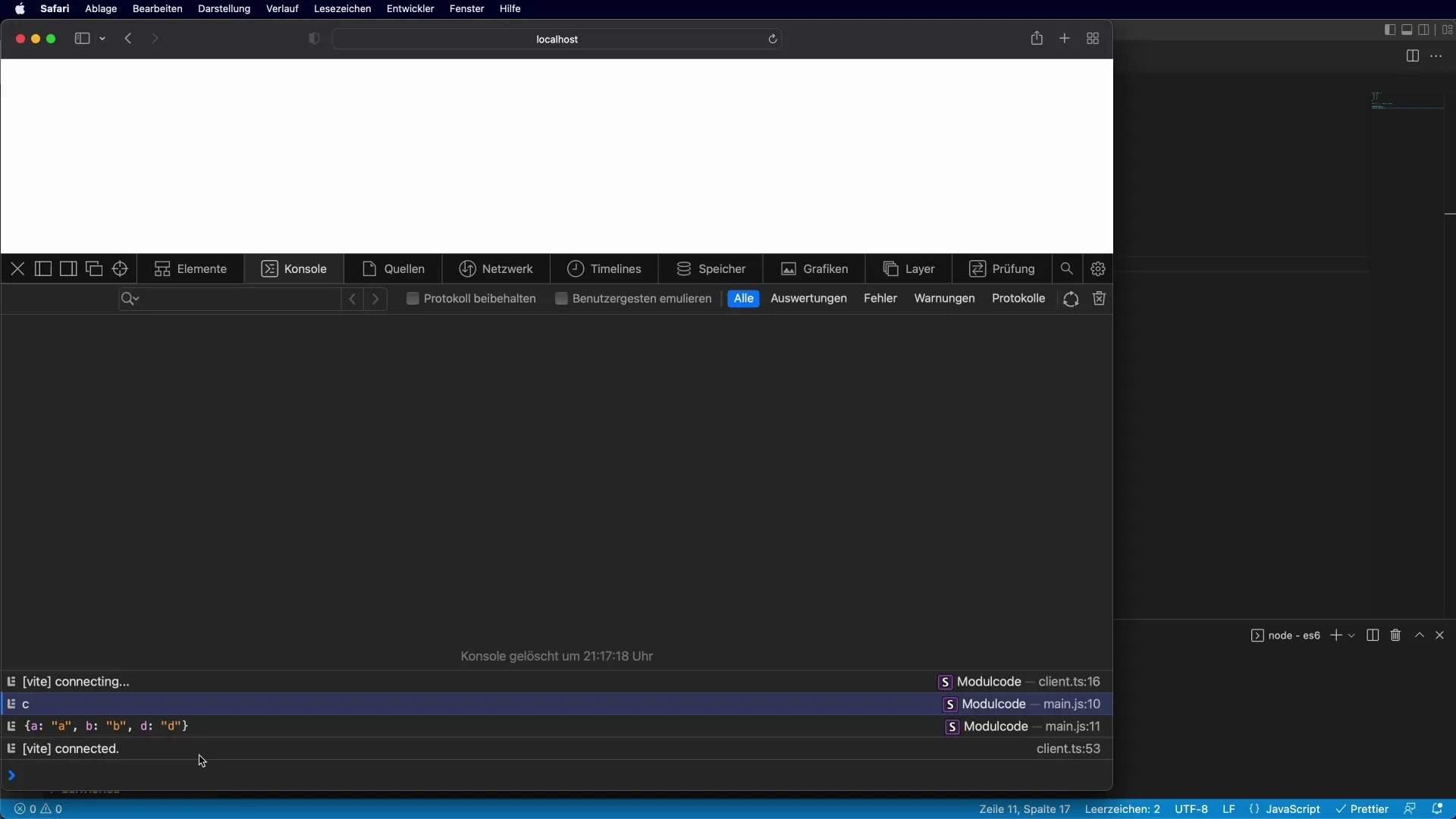
Benefits of Using the Rest Operator
The application of the rest operator has several benefits. For one, it provides a clearer and shorter syntax that makes reading and maintaining your code easier. Additionally, it allows you to extract only the necessary properties while keeping the rest of the data structured and tidy.
In combination with function parameters, the rest operator offers a way to create more flexible and adaptable APIs. This allows you to only query or pass the most relevant data while keeping the remaining information in a compact format.
Summary
The rest operator for objects is a great way to enhance your JavaScript skills. You learned how to analyze an object, extract specific properties, and efficiently collect the rest of the data in a new object. This technique will help you make your code clearer and more effective.
Frequently Asked Questions
How does the rest operator work with objects?The rest operator extracts specific properties from an object and stores the remaining properties in a new object.
Can I use the rest operator in a function?Yes, the rest operator can also be used in functions to handle excess parameters.
Is the rest operator limited to objects?No, the rest operator can also be used with arrays to capture remaining elements.
What does the syntax for the rest operator look like?The syntax uses three dots... followed by a variable name, e.g., const { c,...rest } = obj;.