Strings are a fundamentally important part of programming in JavaScript. Handling strings plays a crucial role, whether you use them for simple outputs or more complex data processing. With the introduction of ECMAScript 6 (ES6) and subsequent versions, numerous useful methods have been added that significantly ease working with strings. In this tutorial, we will show you how to effectively utilize these new functions.
Key Insights
In this tutorial, you will learn about the new string methods from ES6 to ES13. You will see how you can write much more efficiently with functions like startsWith(), includes(), endWith(), repeat(), and replaceAll(). Additionally, we will use the spread operator to convert strings into arrays.
Step-by-Step Guide
Defining a String
Before you can start using the new methods, you first need to define a string. Create a variable that contains the string you want to work with.
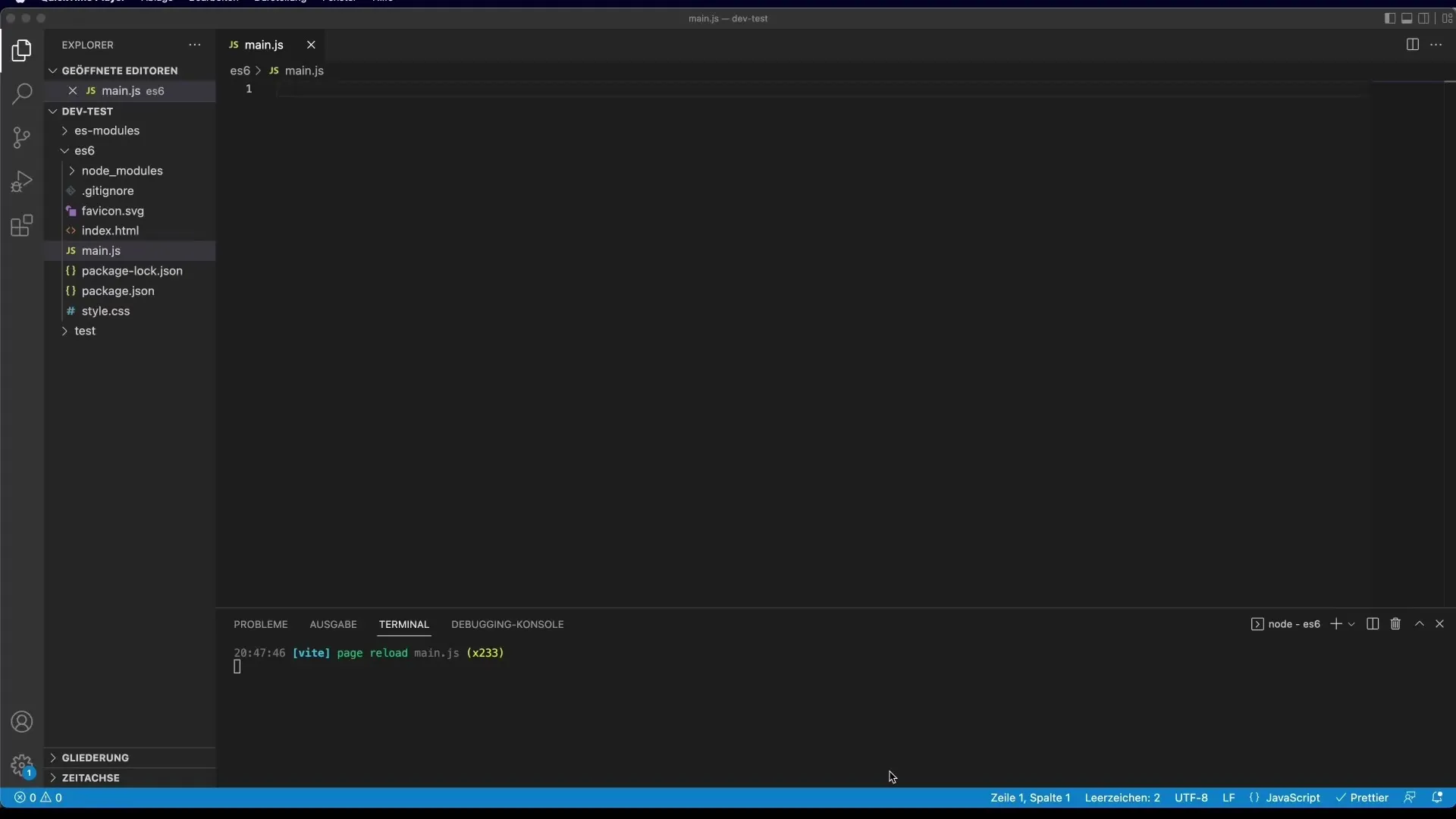
Checking if a String Starts with a Certain Text
One of the new functions is startsWith(). This method checks if your string starts with a specific substring.
If you are searching for something that is not at the beginning, the result will be false.
Checking if a String Contains a Certain Substring
With the method includes(), you can check if a specific substring is contained within your main string. This method is shorter and more understandable than the traditional method with indexOf().
If you are searching for a substring that does not exist, the method will return false.
Checking if a String Ends with a Certain Text
Similar to startsWith(), there is also the function endsWith() to check if your string ends with a specific substring.
Repeating a String
The method repeat() allows you to repeat a string multiple times. This is useful when, for example, you need a specific text or a line for visual effects.
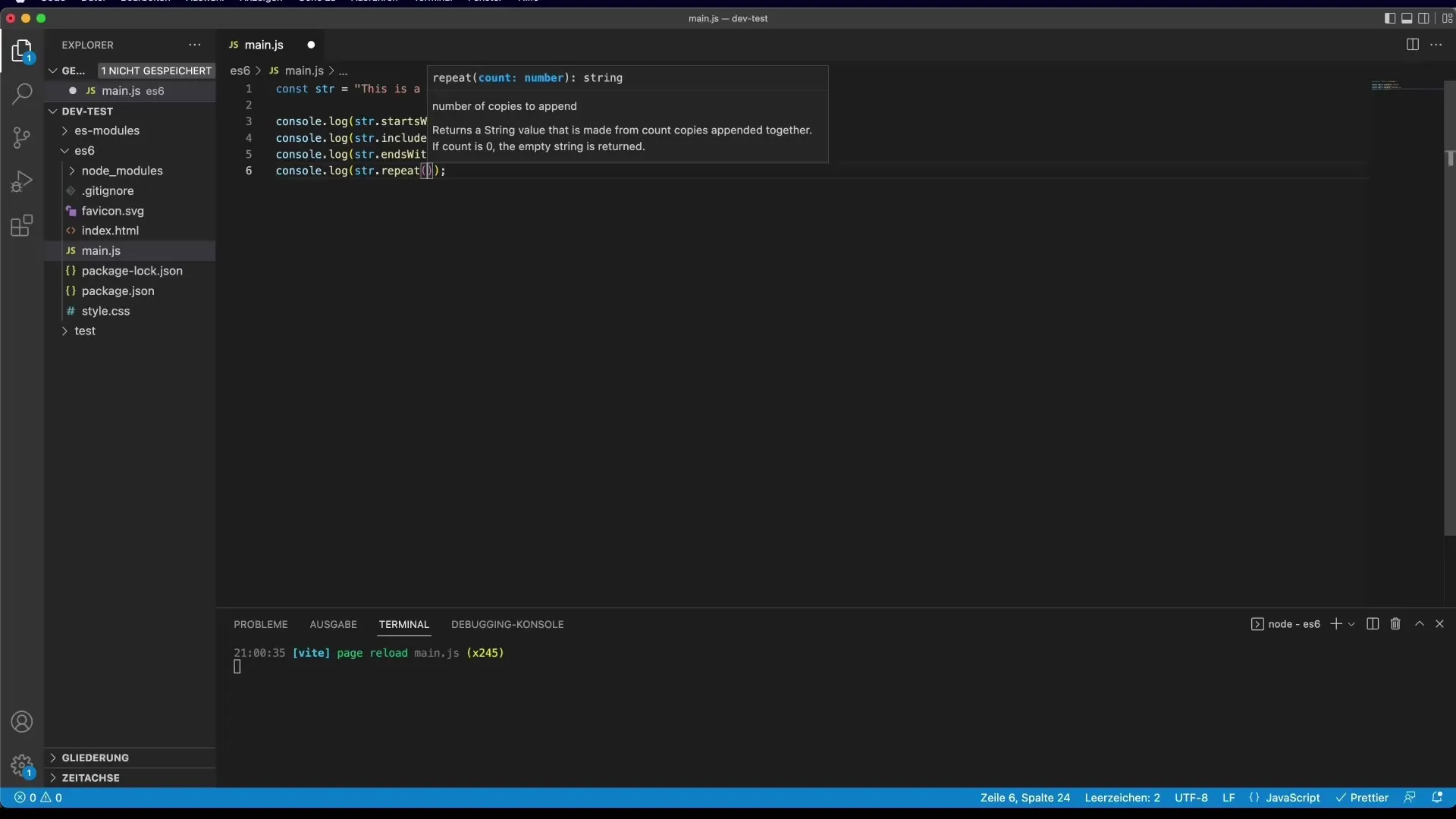
Replacing Substrings
With the new replaceAll() function, you can replace all occurrences of a substring without having to use a complex RegExp.
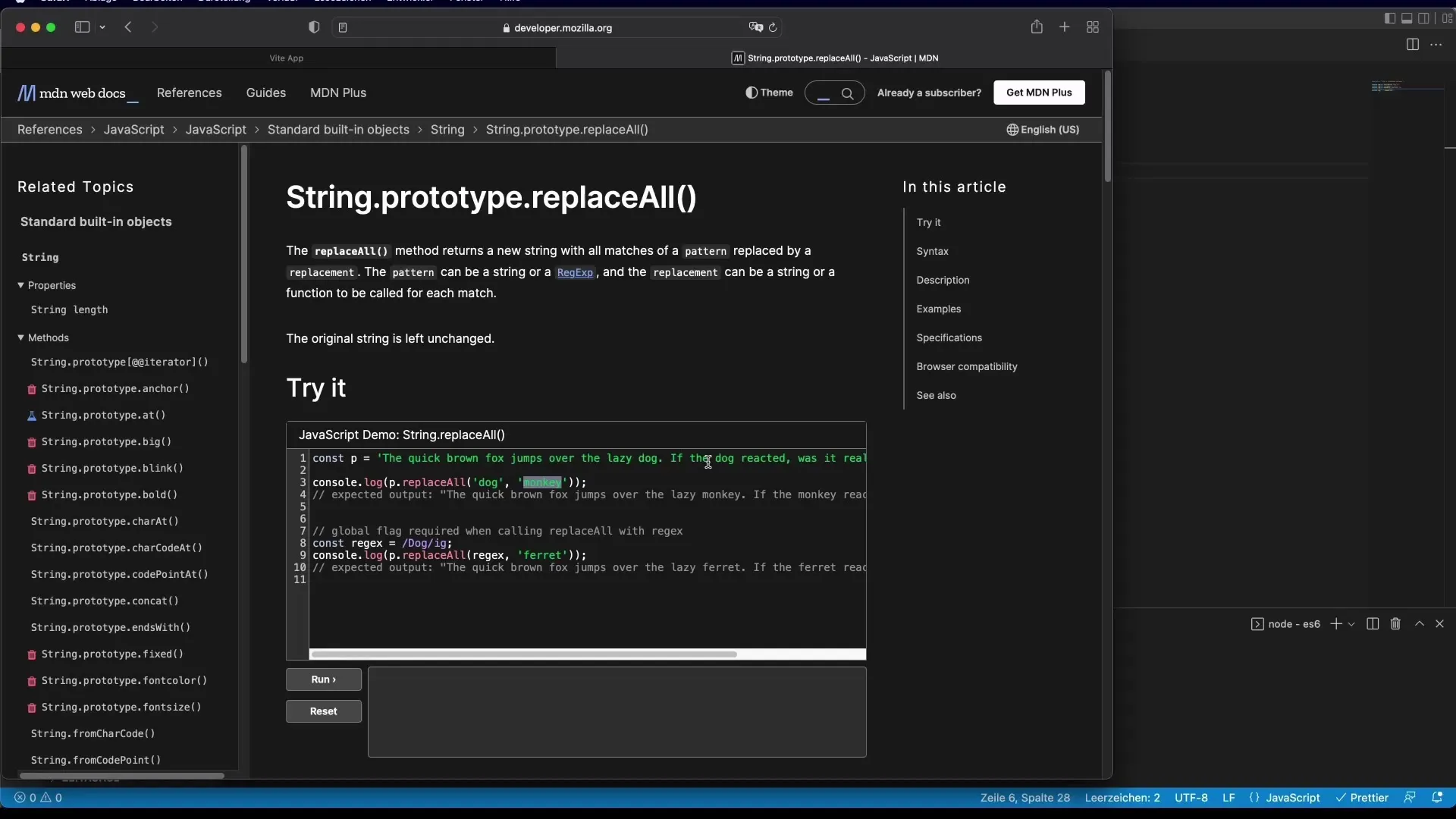
In contrast, the older replace() method only replaces the first occurrence, which can be less helpful in many cases.
Using the Spread Operator with Strings
The spread operator (…) can also be used with strings to convert them into arrays of individual characters. This can be a useful technique if you want to handle each letter of a string separately.
More Useful Functions
There are many more useful string functions that you can find in the official documentation from Mozilla Developer Network (MDN). These include methods like slice(), split(), toLowerCase(), and trim(). These methods have already been available in earlier versions of JavaScript but are still very useful.
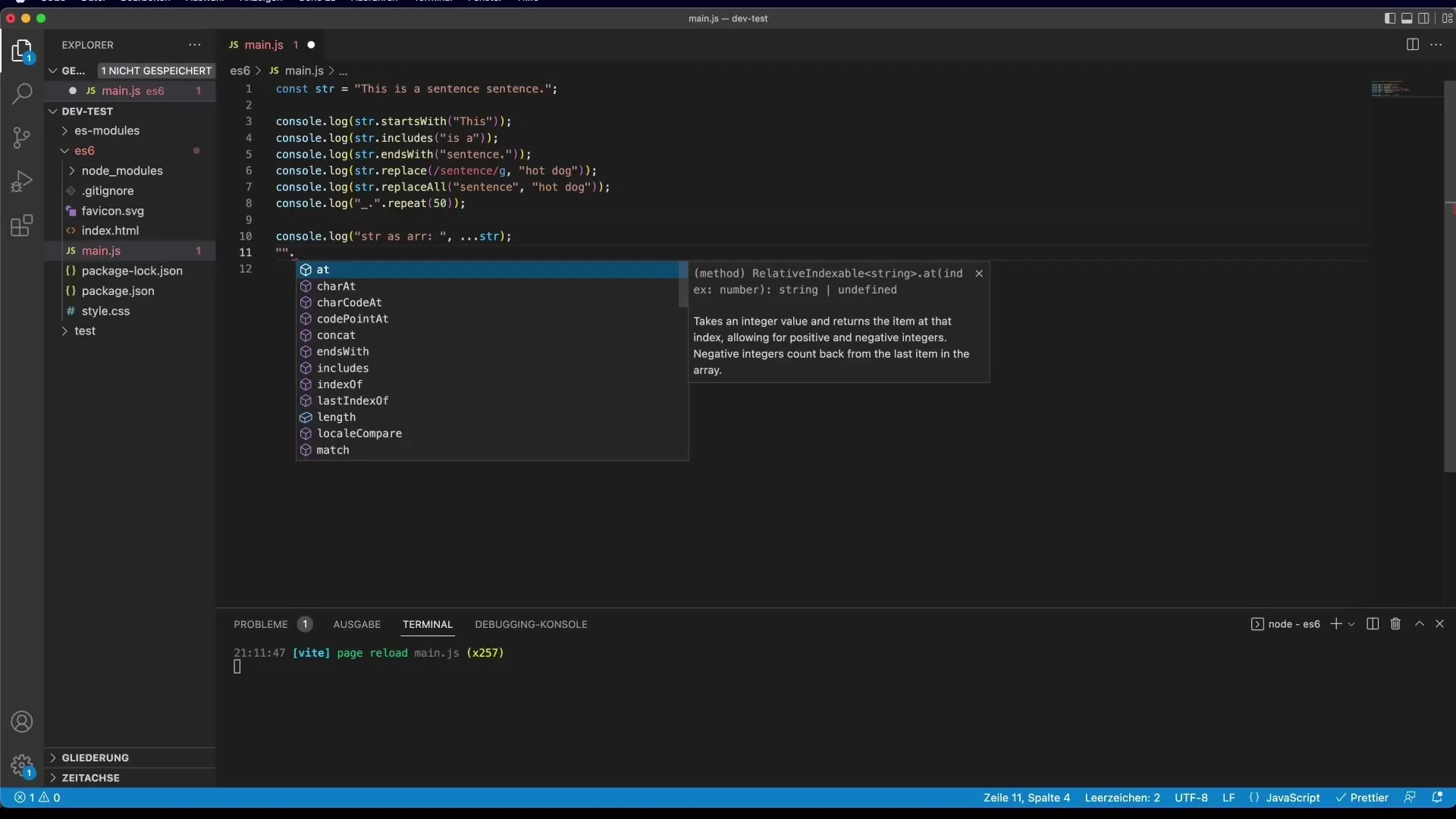
Summary
In this tutorial, you have learned about the new and useful string functions in JavaScript that help you program more effectively. Methods like startsWith(), includes(), endWith(), and replaceAll() improve the readability of your code and make it shorter. It is recommended to consult the official MDN documentation for a more comprehensive list of functions.
Frequently Asked Questions
Which version of JavaScript uses these methods?The mentioned methods are available in ES6 and higher versions.
Can I use replaceAll() in older browsers?replaceAll() may not be supported by older browsers. Check the browser compatibility on MDN.
How can I convert strings into arrays?Use the spread operator (…) or the method split() for this conversion.
Where can I find more information about JavaScript strings?The official MDN documentation provides comprehensive resources about JavaScript strings.