In the world of JavaScript, Arrays are a fundamental data type that you use frequently. With each new ECMAScript version, the handling of arrays has been improved by various innovative methods. In this tutorial, you will learn to use some of the most useful new array functions introduced from ES6 to ES13. We will look at both new and proven functions that help you work more effectively with arrays.
Key Insights
- find(): Search through an array and find the first element that meets a certain criterion.
- filter(): Create a new array with all the elements that meet the given condition.
- map(): Transform the elements of an array and produce a new array.
- reduce(): Combine all elements of an array into a single value.
- some(), every(): Check if at least one element or all elements of an array meet a certain condition.
Step-by-Step Guide
1. Understanding the find() Method
The find() method is one of the functions introduced in ES6. You use it to find a specific element in an array. The name says it all: with find(), you search through an array, and it returns the first element that meets your criteria.
To use find(), you pass a callback function that defines a condition. This function will be called for each element in the array.
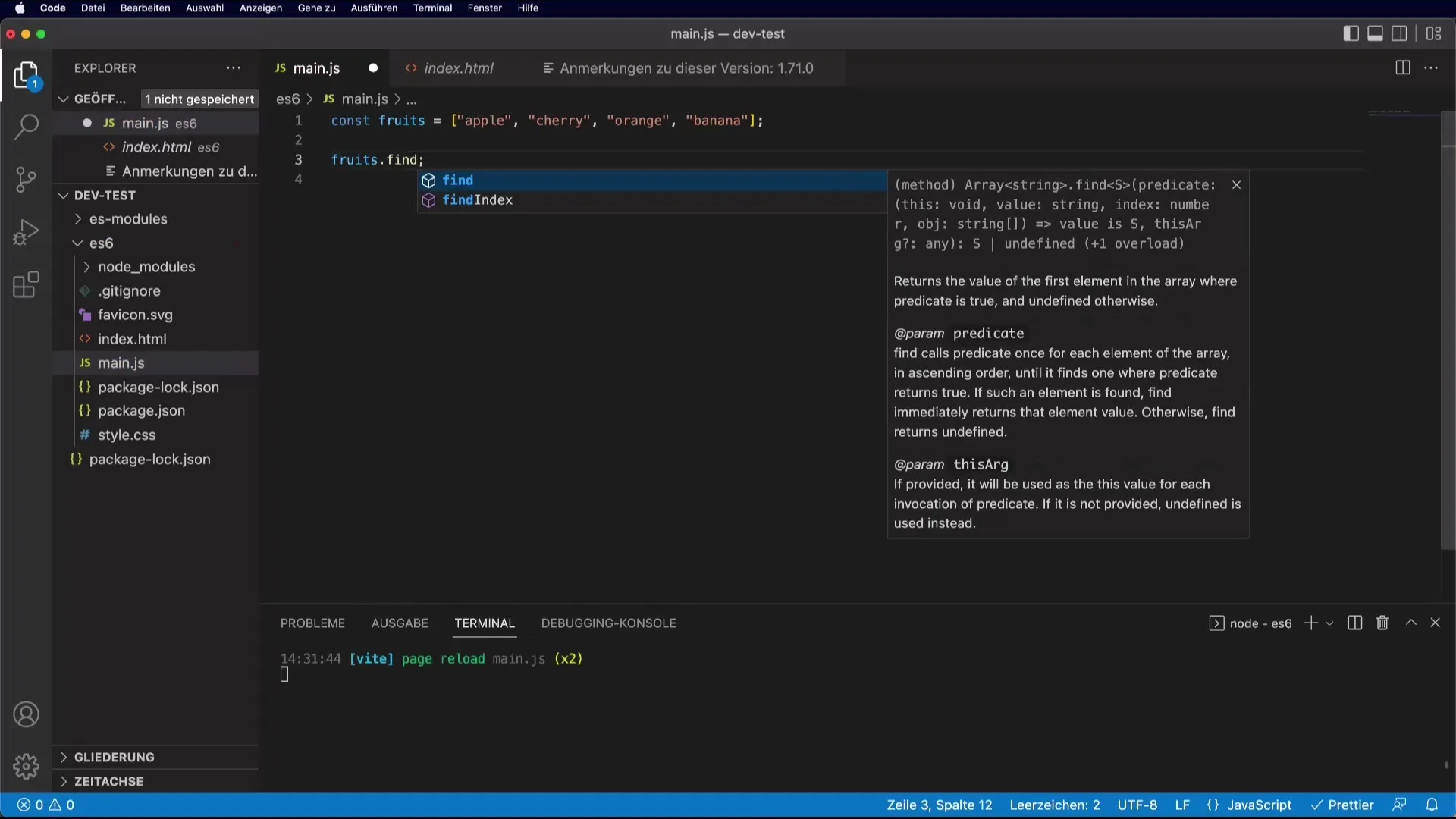
In this example, "Orange" is found and returned. If you search for a different color, the result could be correspondingly different.
2. findIndex() for the Index of the Sought Element
In addition to find(), there is also findIndex(), which returns the index of the first element that meets the condition. This is useful if you need the actual index in the array.
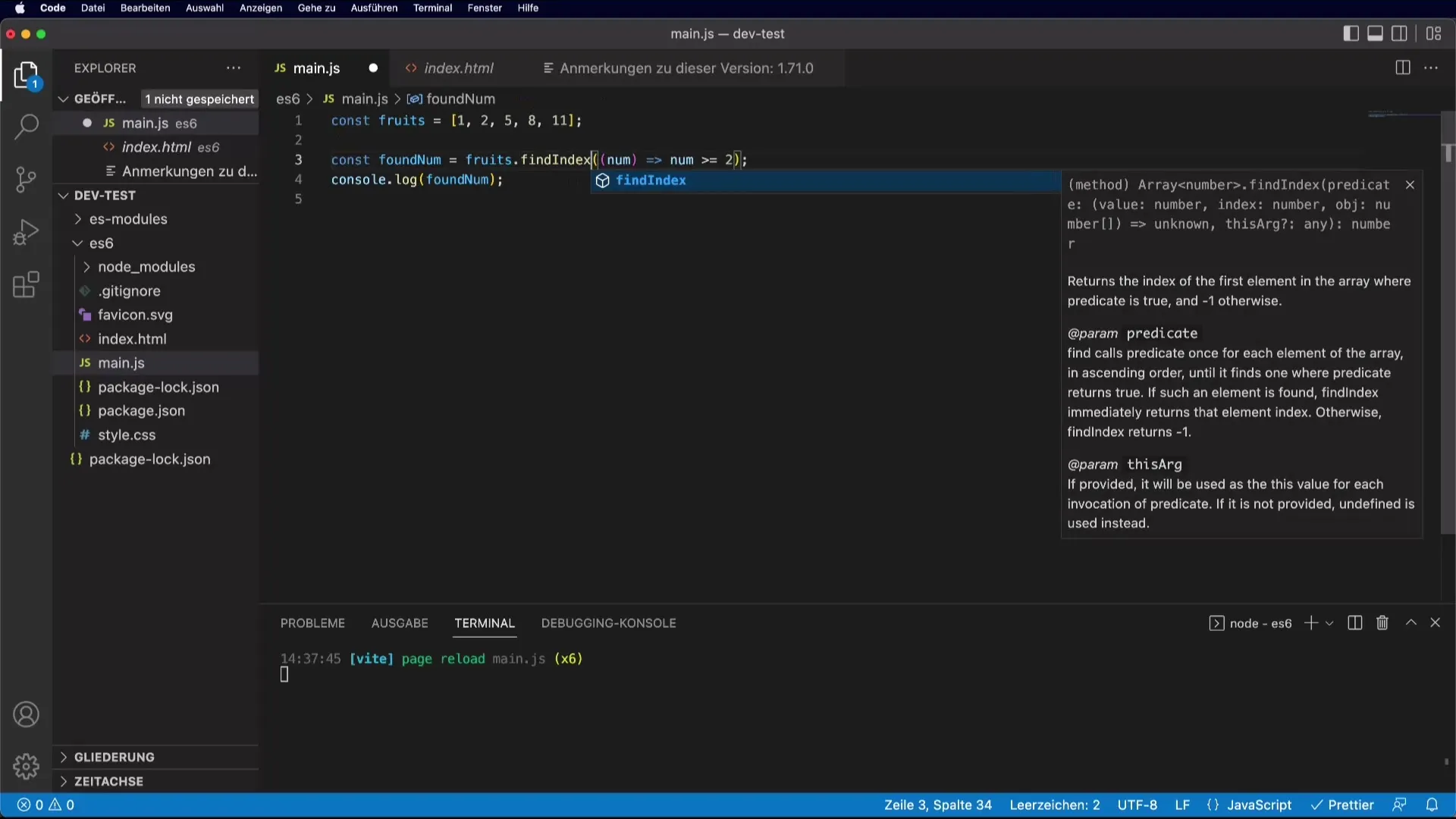
In this case, foundFruitIndex returns the index of "Orange," which is 2, since arrays are zero-based.
3. Filtering Arrays with filter()
The filter() method allows you to create a new array that contains only the elements that meet a specific condition. Here, we use filter() to return all fruits whose length is greater than 5.
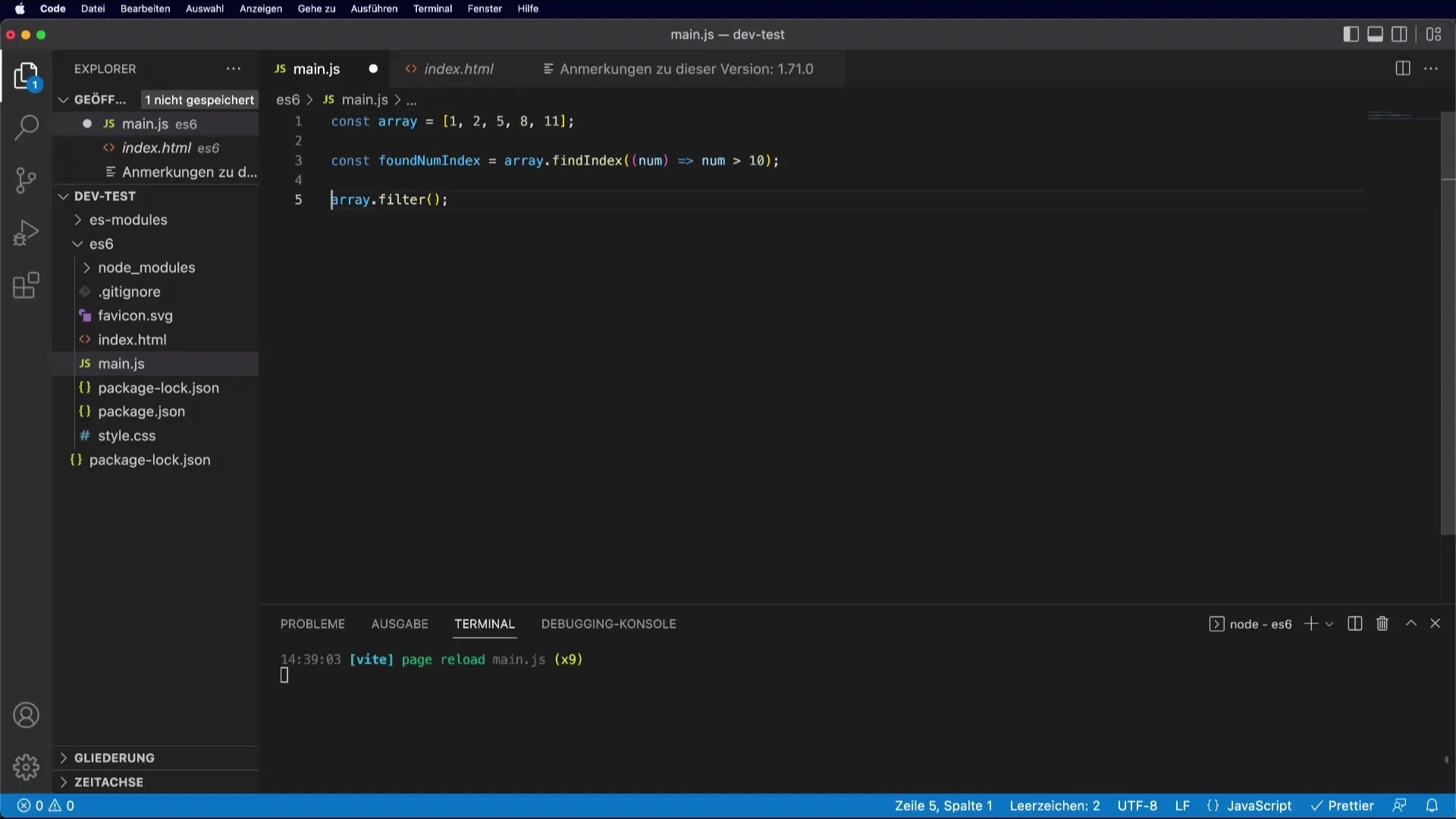
The result then shows all fruits that have more than five letters, e.g., "Banana."
4. map() for Transformations
With the map() method, you can change the values in an array and create a new array. For example, to get the lengths of the fruit names.
Here, you get the lengths of the fruit names as an array: [5, 6, 6, 6].
5. reduce() for Summarizing Values
The reduce() method is one of the most powerful functions that helps you condense all values in an array into a single value.
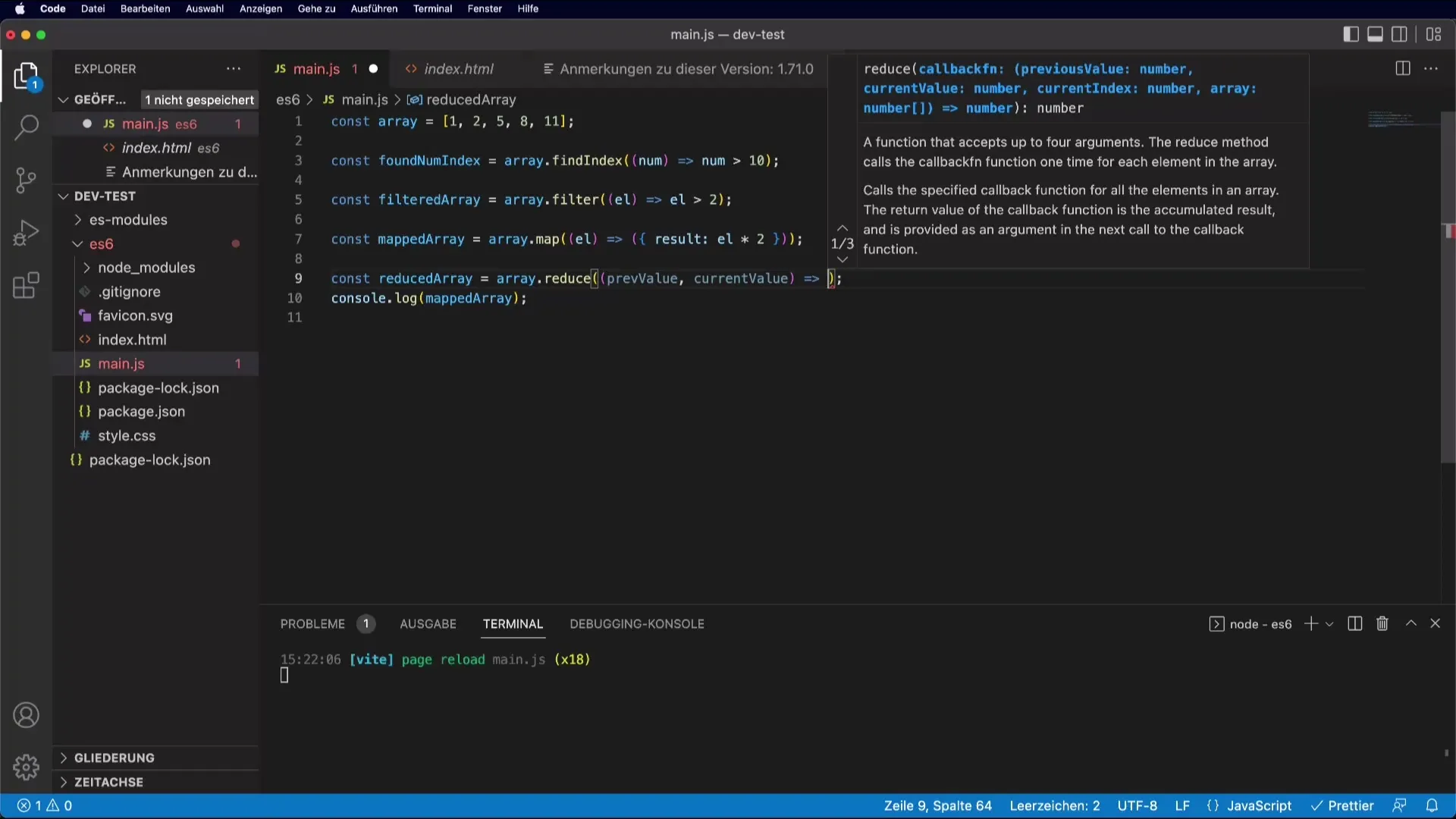
The result is 15, as all values have been added together.
6. Checking Conditions with some() and every()
The methods some() and every() check the elements of an array against conditions. With some(), you ask if at least one of the elements meets the condition, while every() ensures that all elements meet it.
Here, hasLargeFruits indicates whether there is at least one fruit with more than six letters.
7. Array.from() to Create Arrays
This method allows you to create an array from another structure.
This converts an HTML collection into a true array, so that you can apply array methods to it.
8. Check if Something is an Array with Array.isArray()
To check if an object is an array, you use the Array.isArray() method.
The result is true, since fruits is an array.
Summary – Useful New Array Functions in JavaScript
In this comprehensive guide, you have learned how to effectively use various array functions in JavaScript, starting from the well-known find(), filter(), map(), and reduce() to the less commonly used but still useful methods like some(), every(), Array.from(), and Array.isArray(). These functions will make your code more modern and your data processing more efficient. Experiment with these methods in your projects to optimize your handling of arrays.
Frequently Asked Questions
What does the find() method do?The find() method searches an array and returns the first element that meets the specified condition.
How can I find the index of an element?With the findIndex() method, you can find the index of the first element that meets the condition.
What is the difference between some() and every()?some() checks if at least one element meets the condition, while every() ensures that all elements meet it.
When do I use map()?map() is used to transform each element in an array and produce a new array.
How can I check if something is an array?With the Array.isArray() method, you can check if an object is an array.