In the JavaScript ecosystem, the module structure has established itself as an essential component, especially with the introduction of ECMAScript 6 (ES6). Here you will learn how to effectively use the new module definitions to make your code modular and maintainable. This guide will introduce you to the fundamentals of working with JavaScript modules, including the use of import and export statements.
Key Insights
- ECMAScript modules enable a clear separation of code.
- Importing and exporting functions and variables improves module clarity.
- The use of "default exports" and "named exports" optimizes the handling of module contents.
- Errors in module imports can be better identified by introducing a strict mode.
Step-by-Step Guide
1. Basics of the Module Structure
You start by loading a module in an HTML file. A typical example begins with an index.html file that is already configured for loading JavaScript scripts.
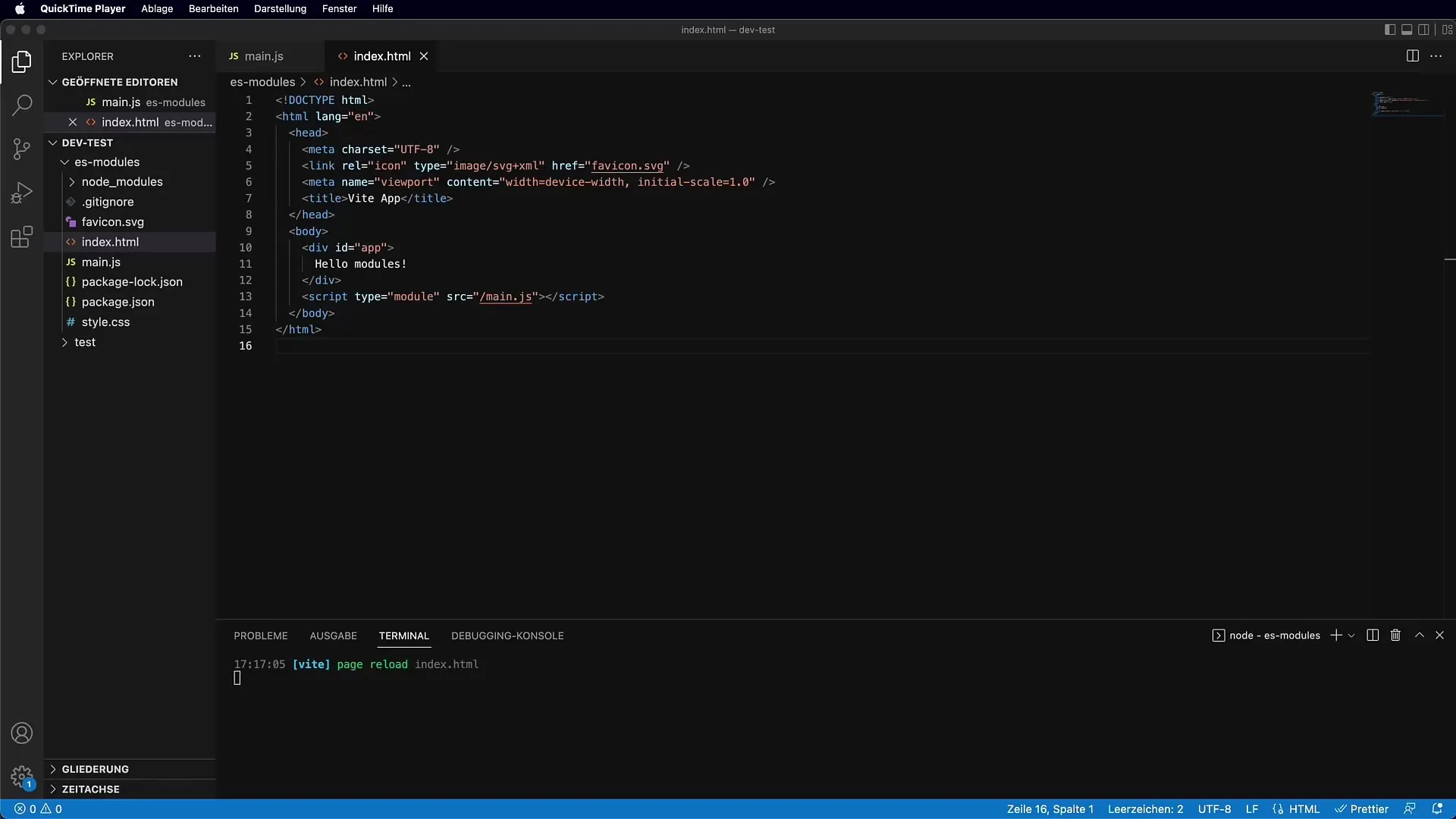
2. Importing a Module
Now that the script is loaded as a module, you can import another file. Create a new JavaScript file, e.g. modOne.js. In your main script (main.js), you can import the module using import.
With this command, you import all the exports of the module under the name ModOne.
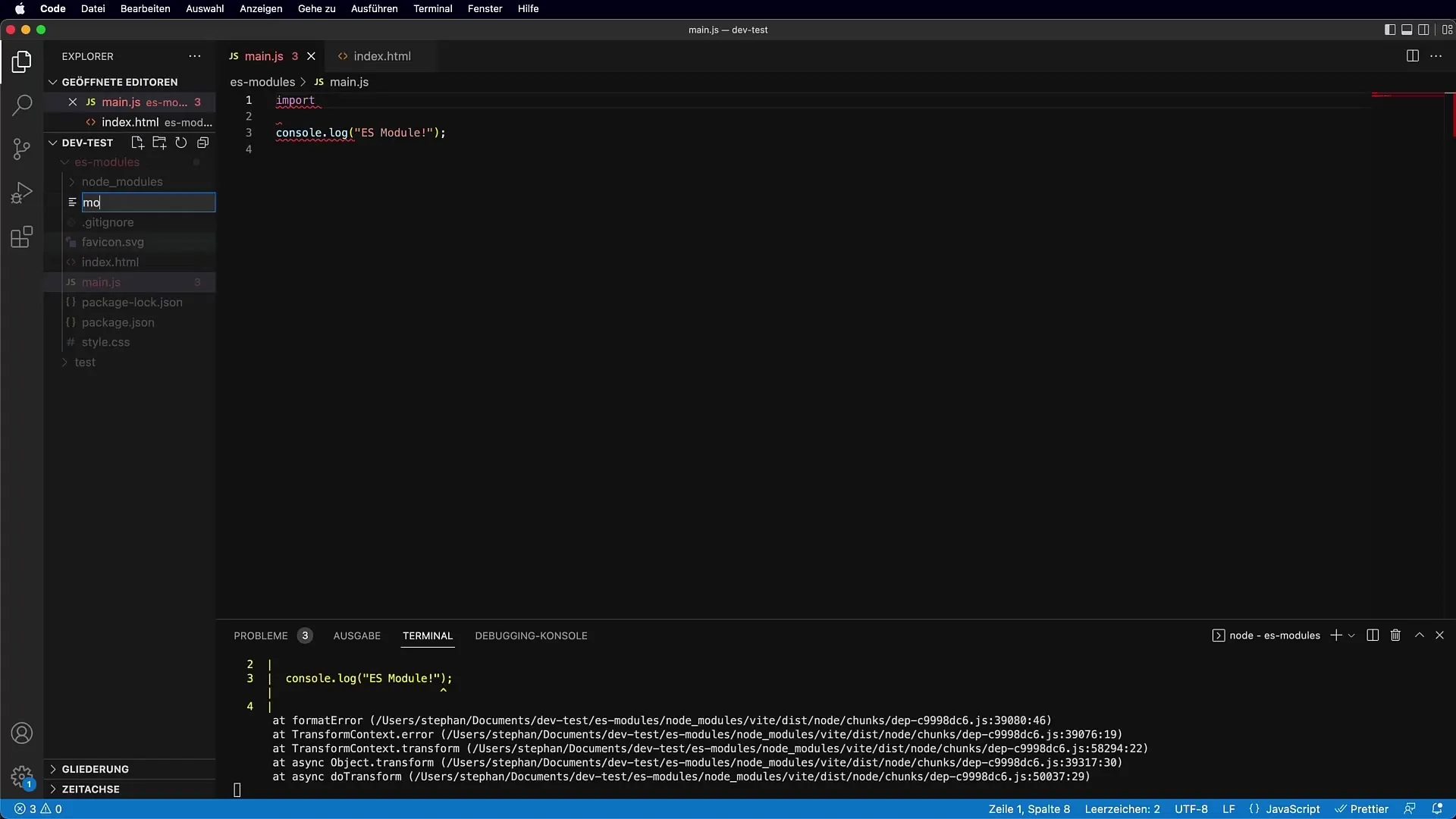
3. Exporting Functions
To make a function available from a module, you need to export it. This is done by adding the export keyword before the function definition.
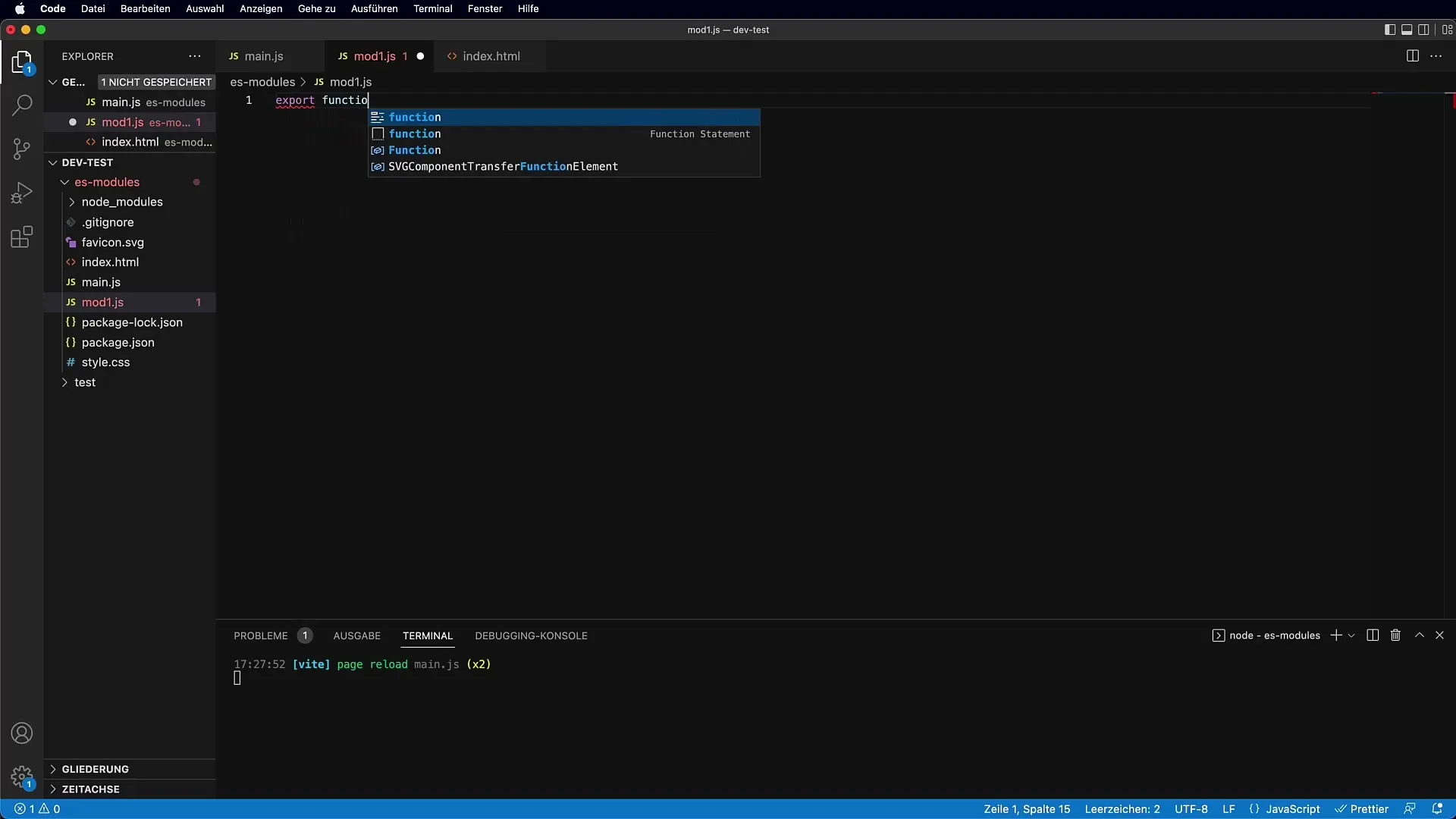
Thus, the function doIt is exported and is now available in other modules.
4. Using Named Exports
If you only want to import certain parts of a module, you can also use named exports. Instead of importing the entire module, it makes sense to import only the required functions.
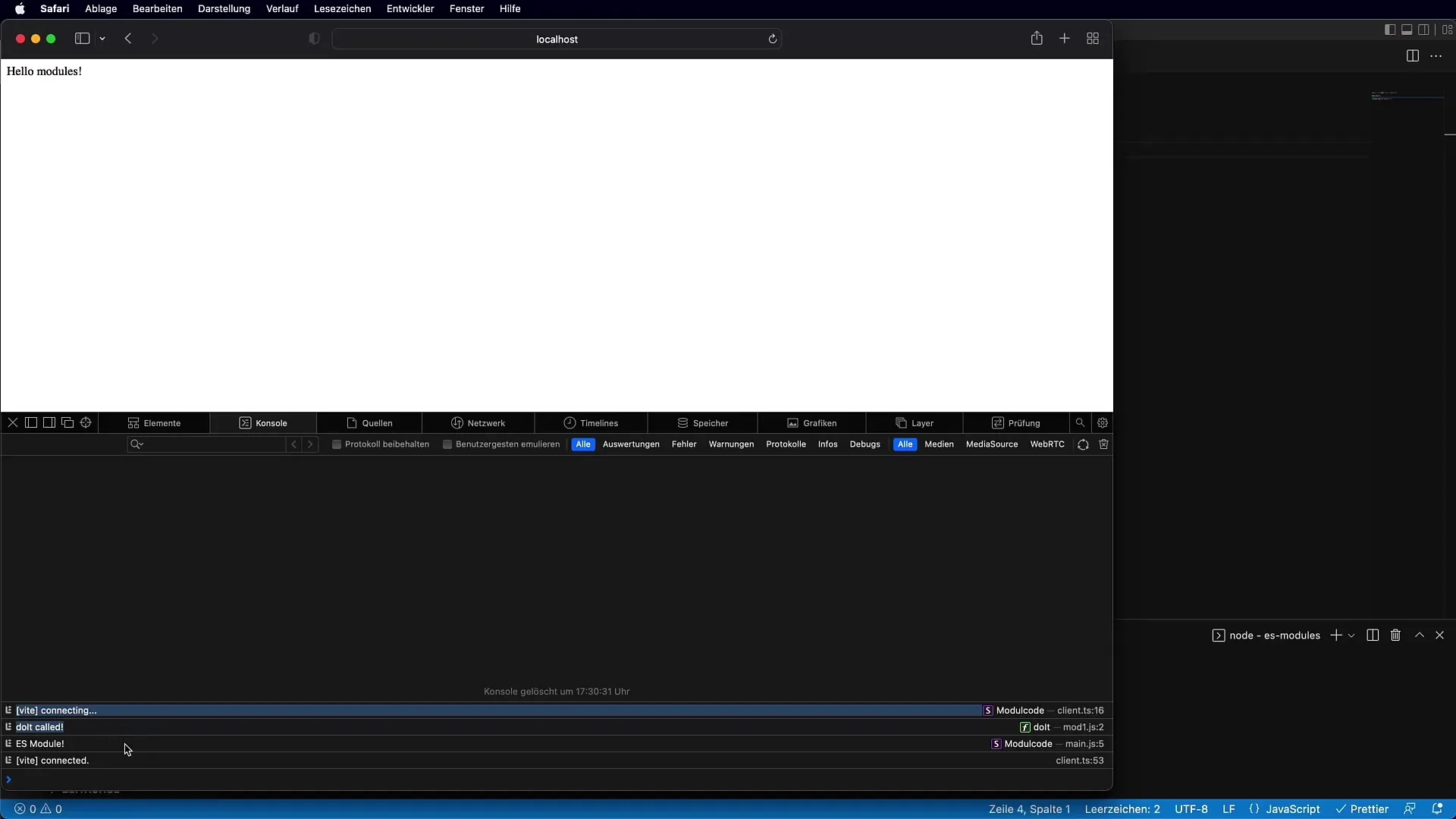
5. Default Exports
An important concept is Default Exports, which allow you to define a single standard export from a module that can then be imported without curly braces.
6. Dealing with Naming Conflicts
If two modules export a function with the same name, you can use an alias when importing to avoid conflicts.
7. Error Detection through Strict Mode
The new ECMAScript modules work in strict mode by default. These restrictions help you detect potential errors early by avoiding problematic syntax and logic.
If you try to declare a function that overwrites the name of an existing import, you will immediately get an error, significantly reducing debugging time.
8. The Importance of Relative Paths
When importing modules, it is important to specify the correct relative or absolute paths. Ensure that the file exists and use the.js extension to avoid execution issues.
9. Summary of Module Usage
To effectively use the functions and features of the new module structure, it is crucial to understand the principles of importing and exporting. This allows you to modularize your code and significantly improve readability.
Summary
Module structures in JavaScript provide an excellent way to keep your code tidy and reusable. You have learned how to load modules, export and import functions, while effectively handling naming conflicts and errors in the code.
Frequently Asked Questions
What are the main advantages of ES6 modules?ES6 modules enable code organization, reusability, and prevent naming conflicts.
How do I import multiple functions from a module?You can import multiple functions by separating them with commas: import { doItOne, doItTwo } from './modOne.js';
What is a Default Export?A Default Export is the main export of a module that can be imported without curly braces.
Do I always have to specify the file extension when importing?Yes, in the browser, it is necessary to specify the file extension to load the file correctly.
Why is Strict Mode important in ES6 modules?Strict Mode helps detect faulty syntax and problems early by applying restrictive rules to the code.