Reading text files is a fundamental skill in C# programming and opens many doors for working with data. Whether you want to store simple configurations or perform complex data analyses, understanding how to effectively read text files is crucial. In this guide, you will learn how to read text files in C# and have various methods available that are tailored to your specific needs.
Key Insights
- To read text files in C#, you need the using System.IO directive.
- You can read the entire file or read it line by line.
- Escape characters enable a user-friendly representation when outputting to the console.
Step-by-Step Guide
First, you need to create a new text file that you want to read later. To do this, open the Solution Explorer in your Visual Studio or a similar IDE. Right-click on your solution in the Solution Explorer and select "Open" from the context menu.
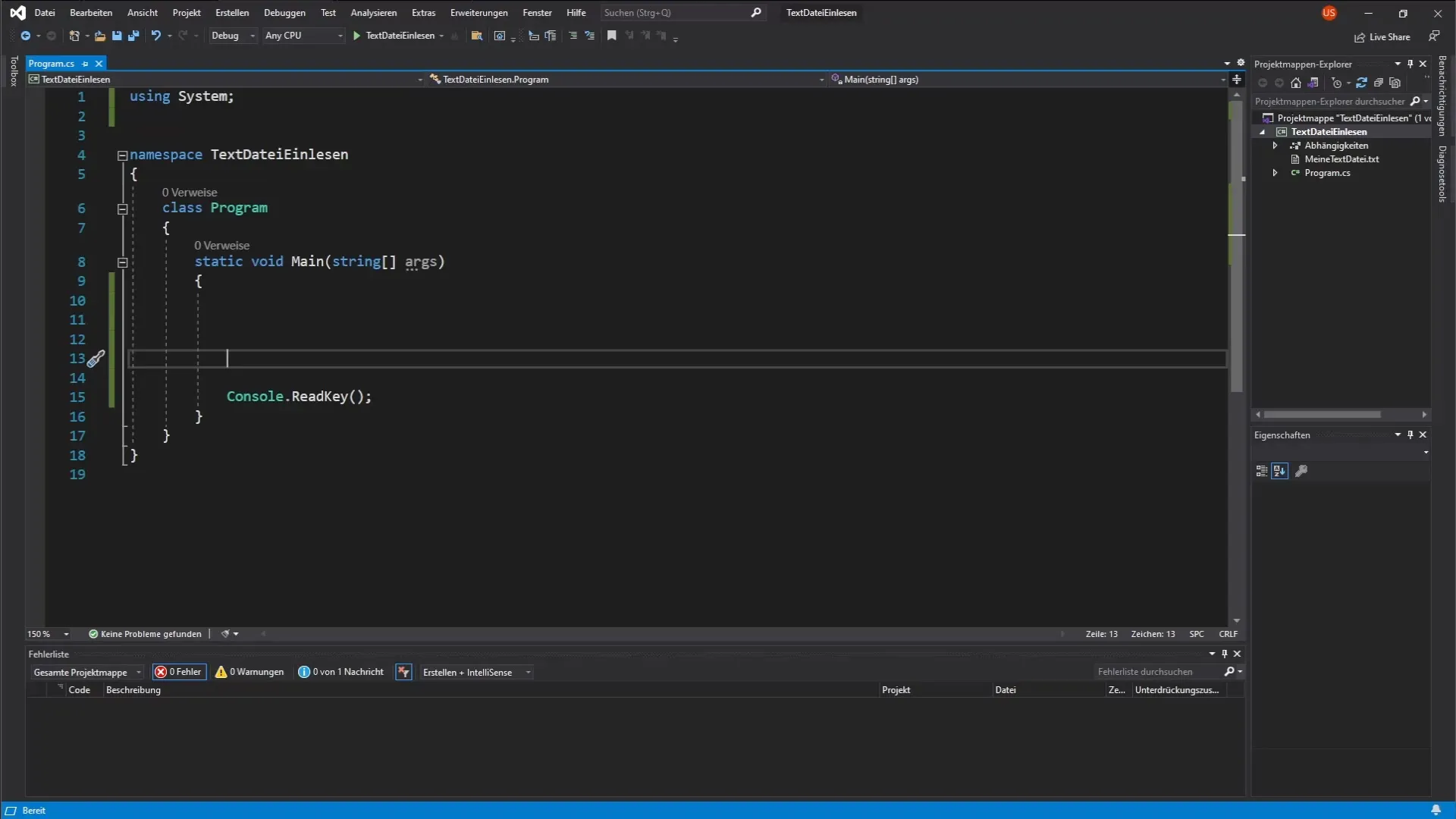
In the file explorer, you can create a new file by right-clicking in a free area. Select "New" and then "Text Document." Assign a suitable name for the text file, for example, "meineTextdatei.txt."
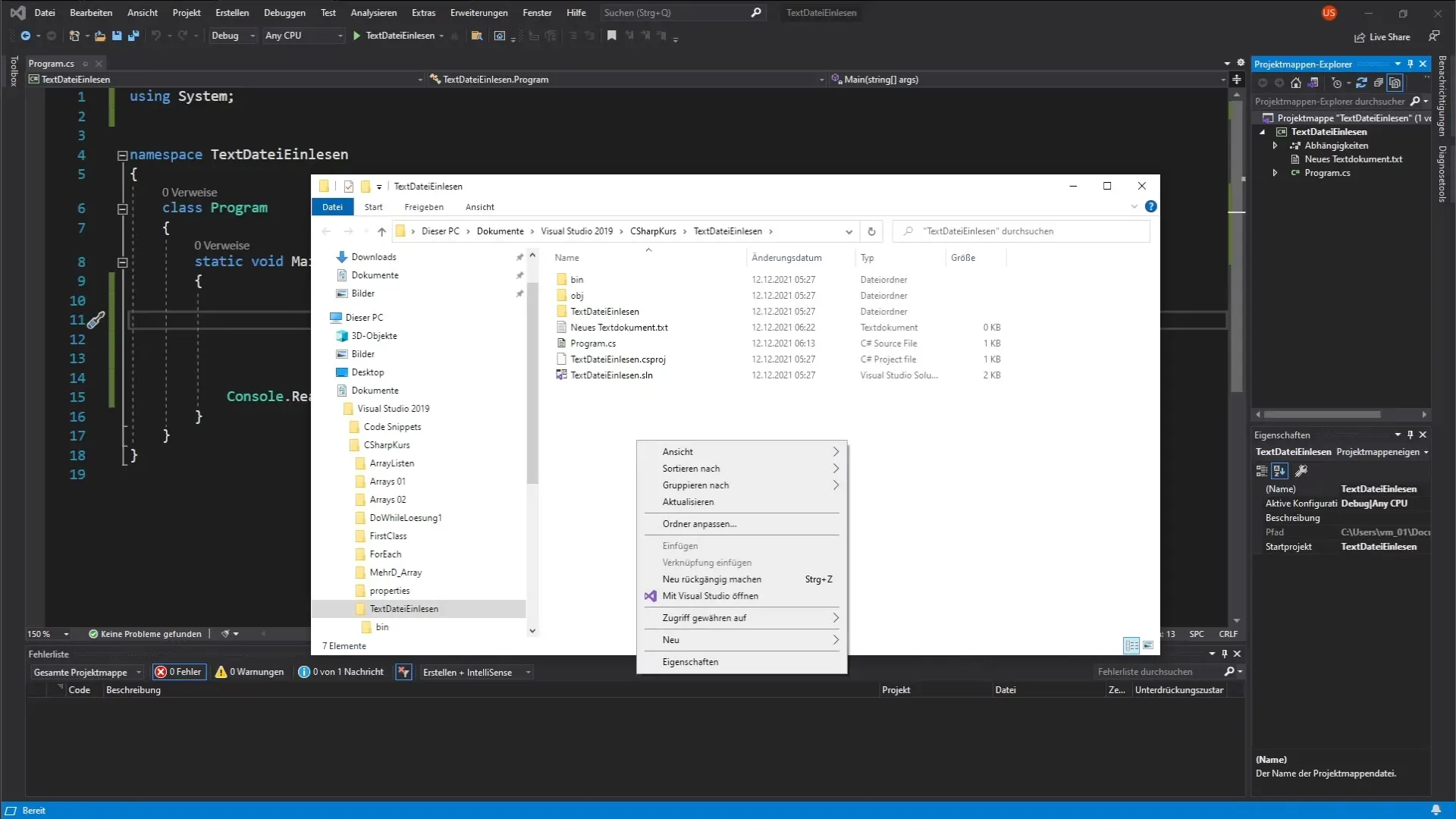
Once the file is created, open it by double-clicking. Add some example lines, such as "Line 1," "Line 2," and "Line 3." Save the file afterward and close it.
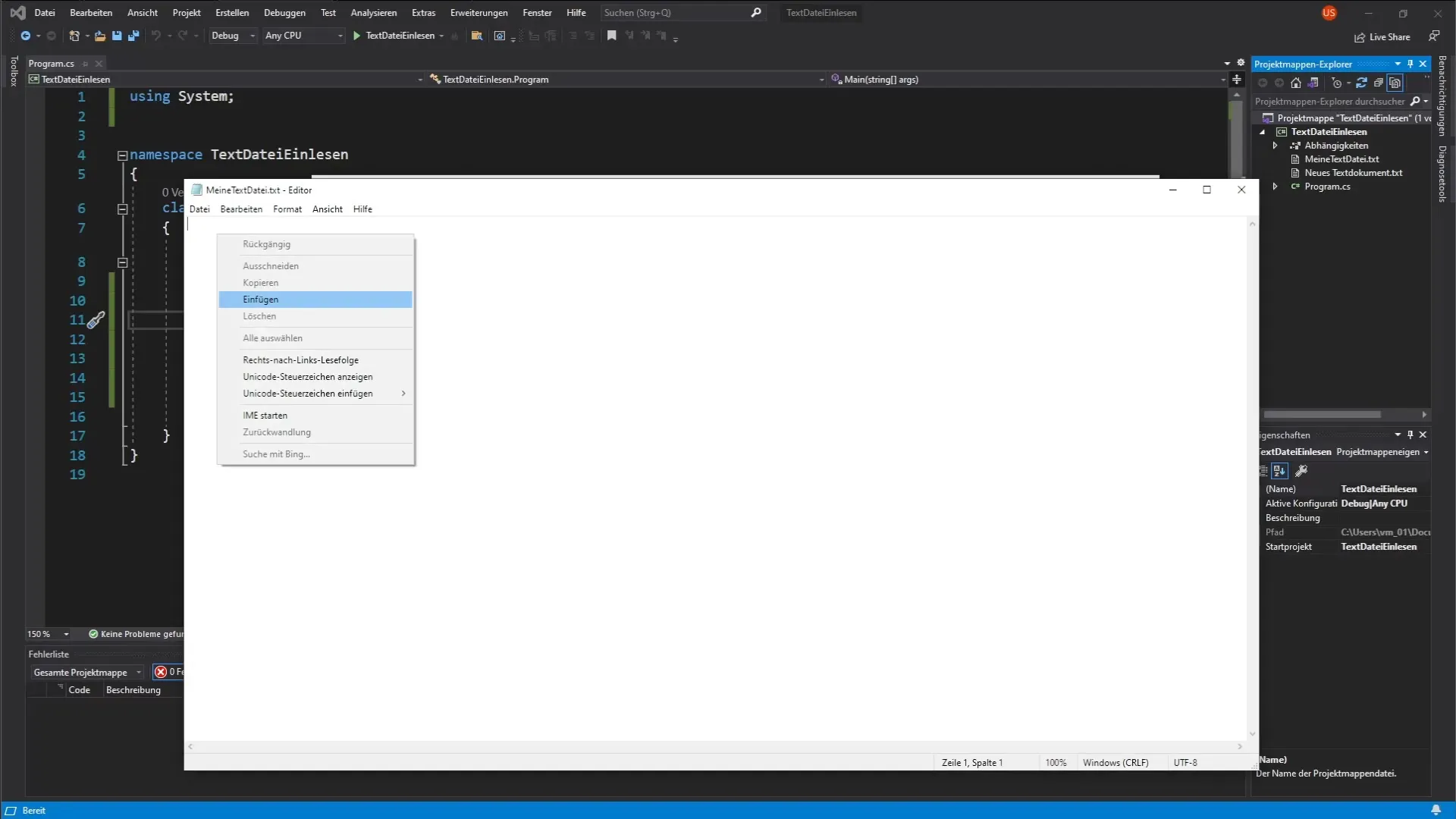
Now it's time to read the file in your C# project. First, you need to add the correct using directive to utilize the functions for reading files.
To read the file, you need the directory where the text file is saved. Navigate back to the folder where you placed the file. Click at the top in the address field and copy the directory path.
Paste the copied directory path into a string variable. This allows you to use the path repeatedly without having to type it multiple times. Ensure that you use the necessary escape characters (\) for directories to correctly represent the backslashes.
Now you can create a new string variable named inhaltTextdatei to store the content of the text file. You use the File.ReadAllText() method to read the entire content of the text file as a single string.
Now output the content using Console.WriteLine() to the console. Start your program, and you should see the lines from the text file on the console.
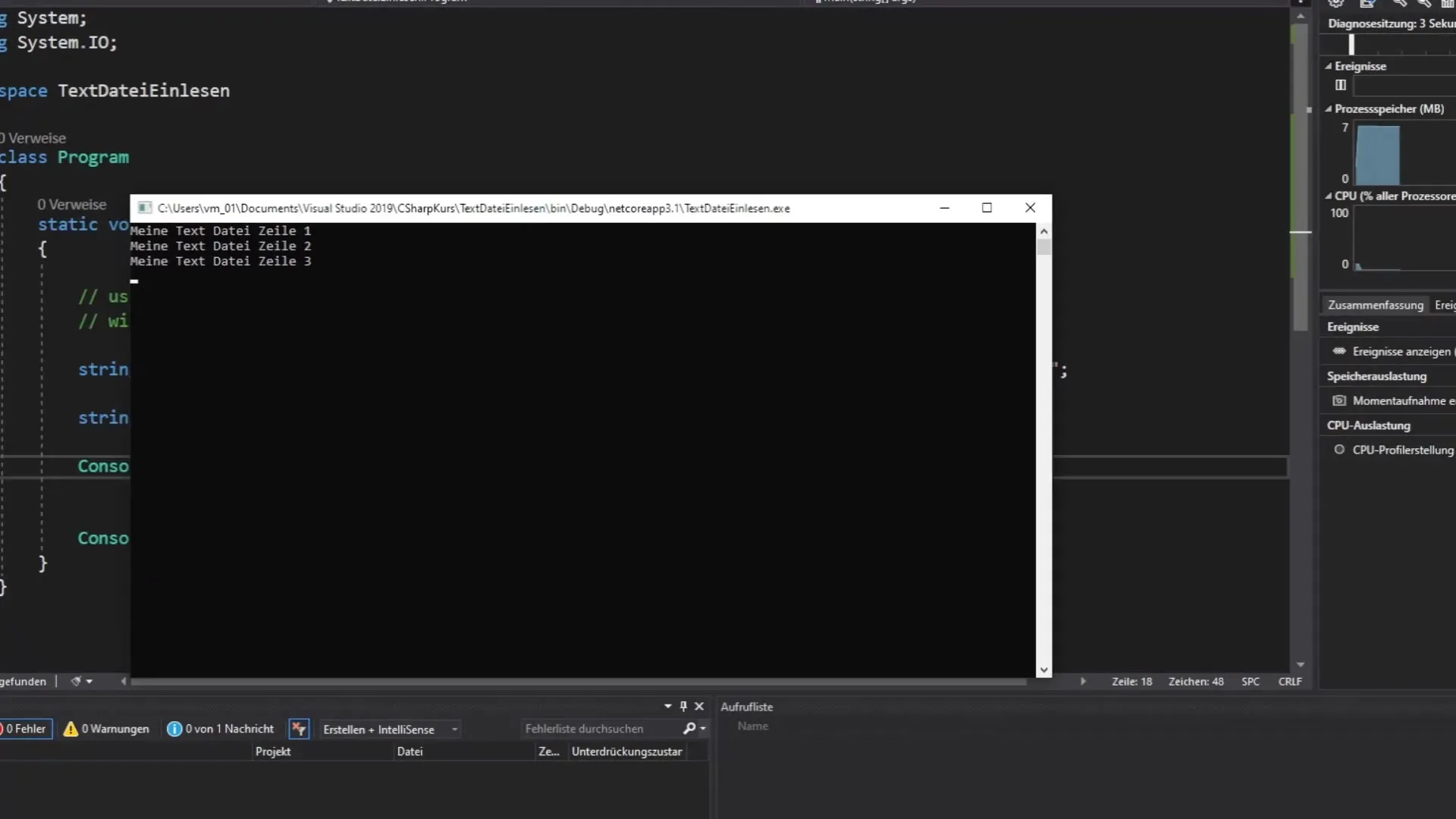
If you prefer to read the text file line by line, you can do that as well. Instead of using File.ReadAllText(), you can use File.ReadAllLines() to get a string[] that contains each line of the text file.
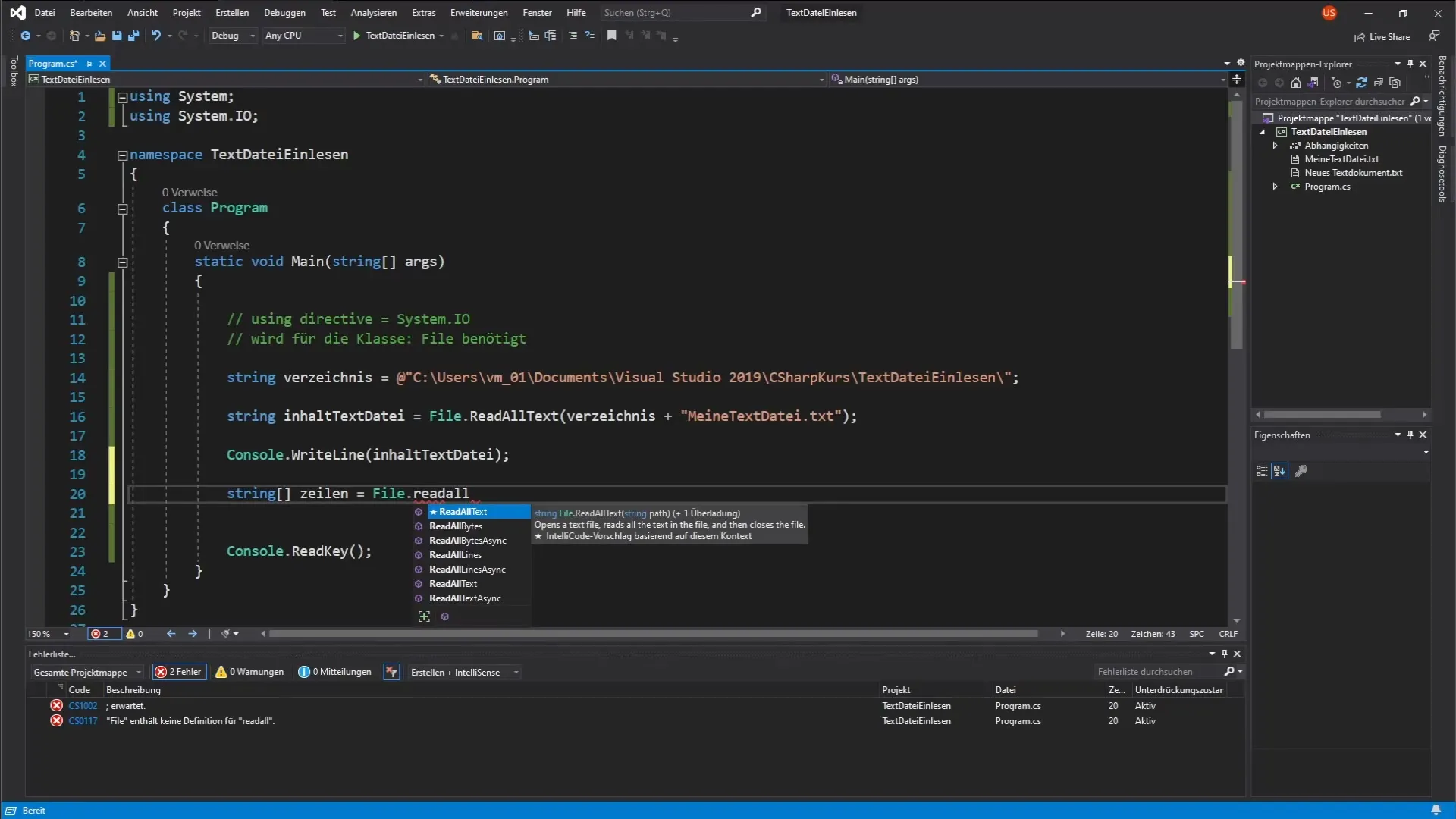
Create a new string array that will store all the lines of the text file. Again, you need to specify the directory accordingly. You can use the previously copied directory path.
Now it’s time to output each line of the text file to the console. For this, use a foreach loop to iterate through the string array.
If you want to format the output, you can use escape characters to present the text in a more appealing way. For example, you can add a tab or use line breaks to improve readability.
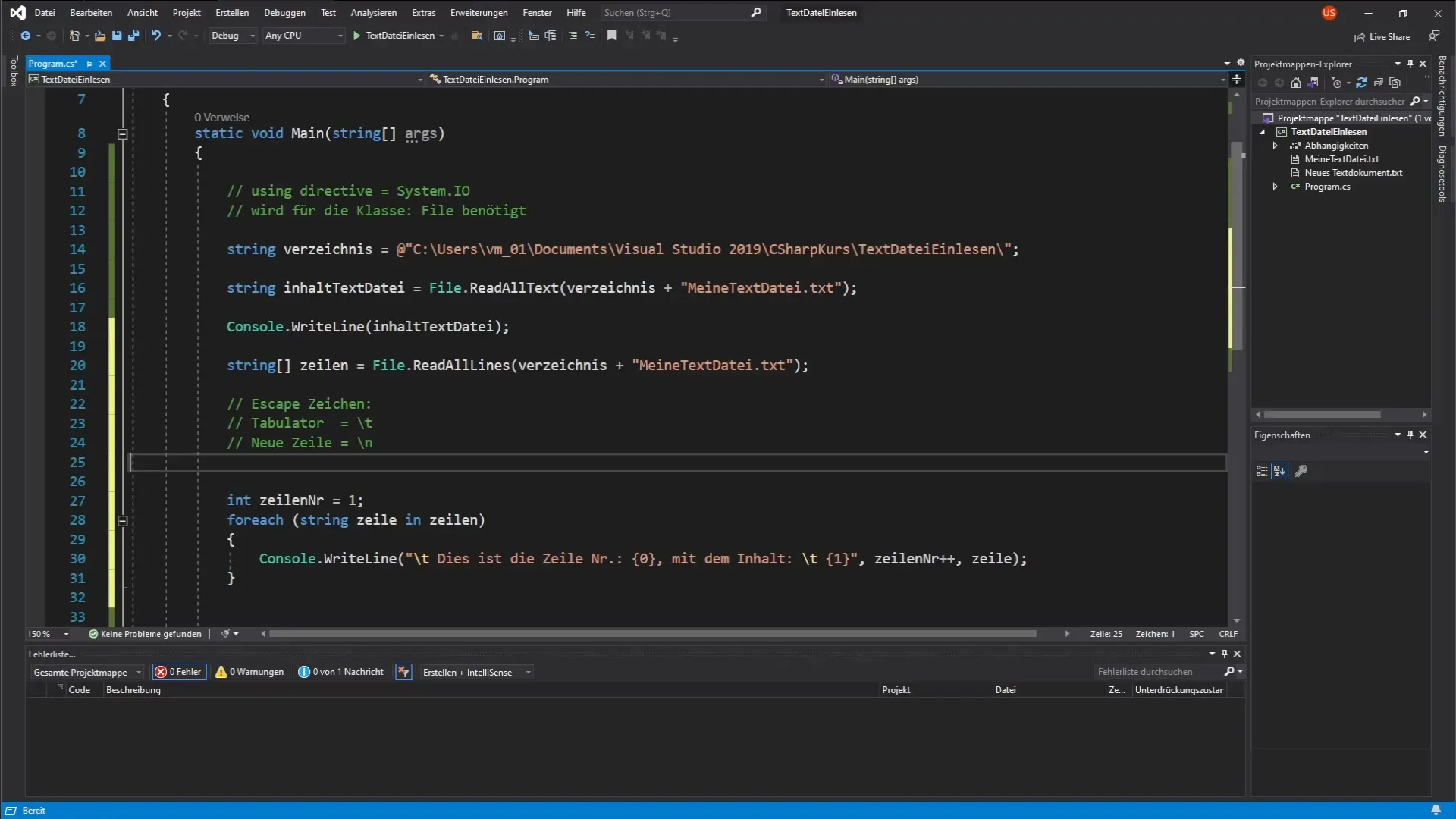
In summary, you have now learned how to read text files in C# both completely and line by line. Escape characters allow you to structure the output, making your console outputs more varied and engaging.
Summary – Reading Text Files in C
Through this lesson, you have learned various methods to successfully process text files in C#. You can now independently apply the approaches for complete and line-by-line reading and try creating and reading your own text files.
Frequently Asked Questions
How do I read a text file in C#?Use the File class from the System.IO namespace and use methods like ReadAllText() or ReadAllLines().
What escape characters can I use?You can use escape characters like \t for tabs and \n for line breaks.
How can I ensure my file path is correct?Make sure to enter backslashes correctly (use one extra backslash, or use the @ syntax).