In this guide, I will show you how to identify and fix an error when removing items from an array using the Chrome Developer Tools. We will go through an existing code together that is not working as expected, and then methodically examine the underlying issues. These steps are not only helpful for solving this specific error, but also for generally dealing with debugging in JavaScript.
Main Insights
- Incorrect removal of array elements can lead to unexpected results.
- Debugging with Chrome Developer Tools enables effective troubleshooting in code.
- Directly manipulating the array during iteration can shift indices and create errors.
- Using alternative methods like filter can be more efficient.
Step-by-Step Guide
Step 1: Understanding the Code
Firstly, it is important to understand the existing code. The array we are analyzing contains the values ["orange", "banana", "apple", "apple", "grape"]. Your goal is to remove all "apple" entries from this array. The current code uses the forEach method to iterate through the array and splice to remove the "apple" entries.
Step 2: Setting a Breakpoint
To find the error, set a breakpoint before the line that executes the splice command. This will give you the opportunity to examine the values of fruit and index as the code progresses.
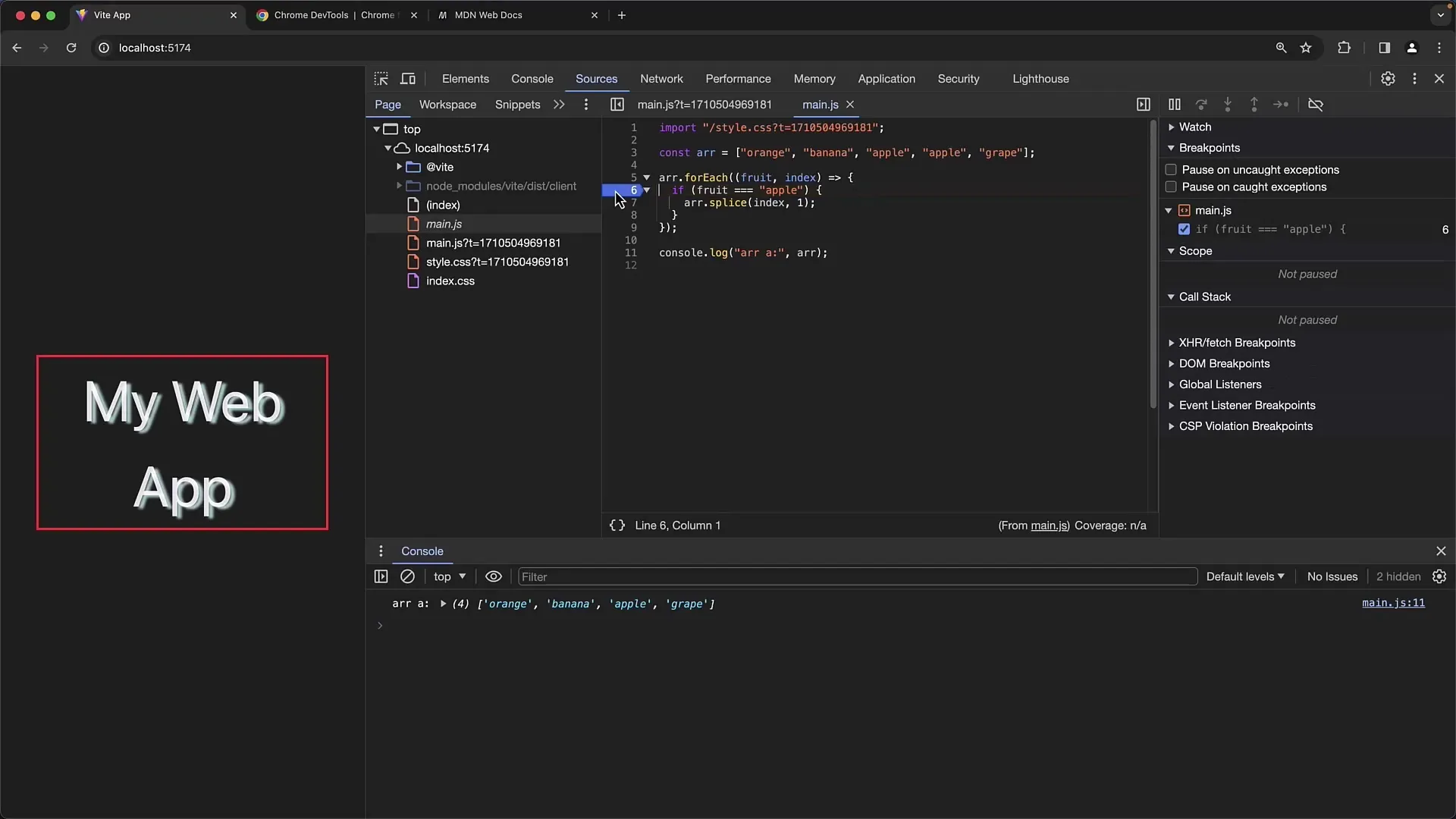
Step 3: Running the Code
Start the code in the Developer Tools and observe what happens when the breakpoint is reached. When you pause the execution, check the local variable fruit. It will show you the current element and index.
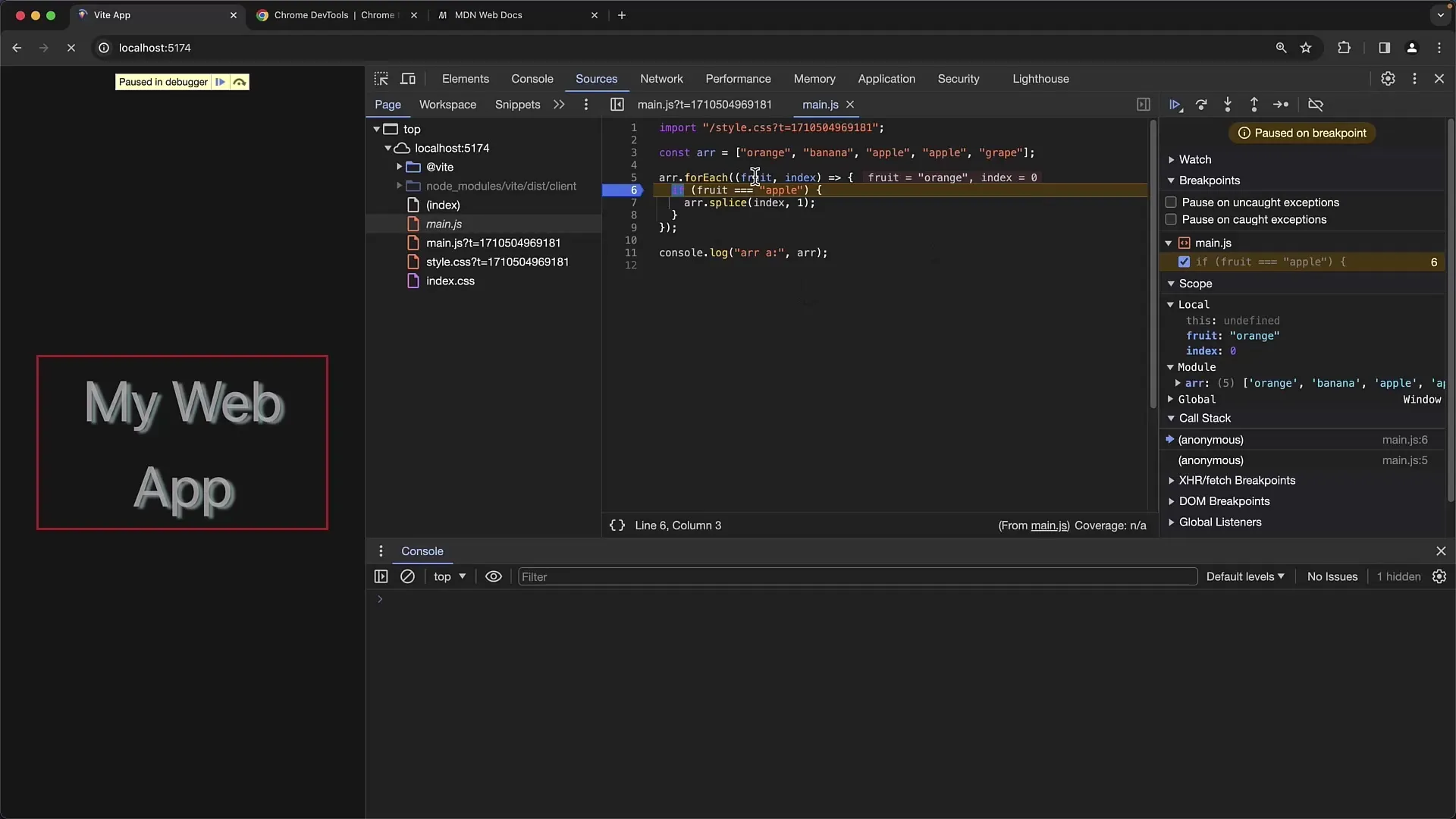
Step 4: Debugging the forEach Loop
As you iterate through the different elements of the array, pay attention to how the indices change, especially when splice is used. The first "apple" entry is correctly removed, but the second "apple" entry is skipped because the indices of the remaining elements have shifted.
Step 5: Error Diagnosis
Understand the problem: Once an element is removed with splice, the last elements of the array shift. During the next iteration, the current element is not referenced correctly, which results in not all "apple" entries being removed.
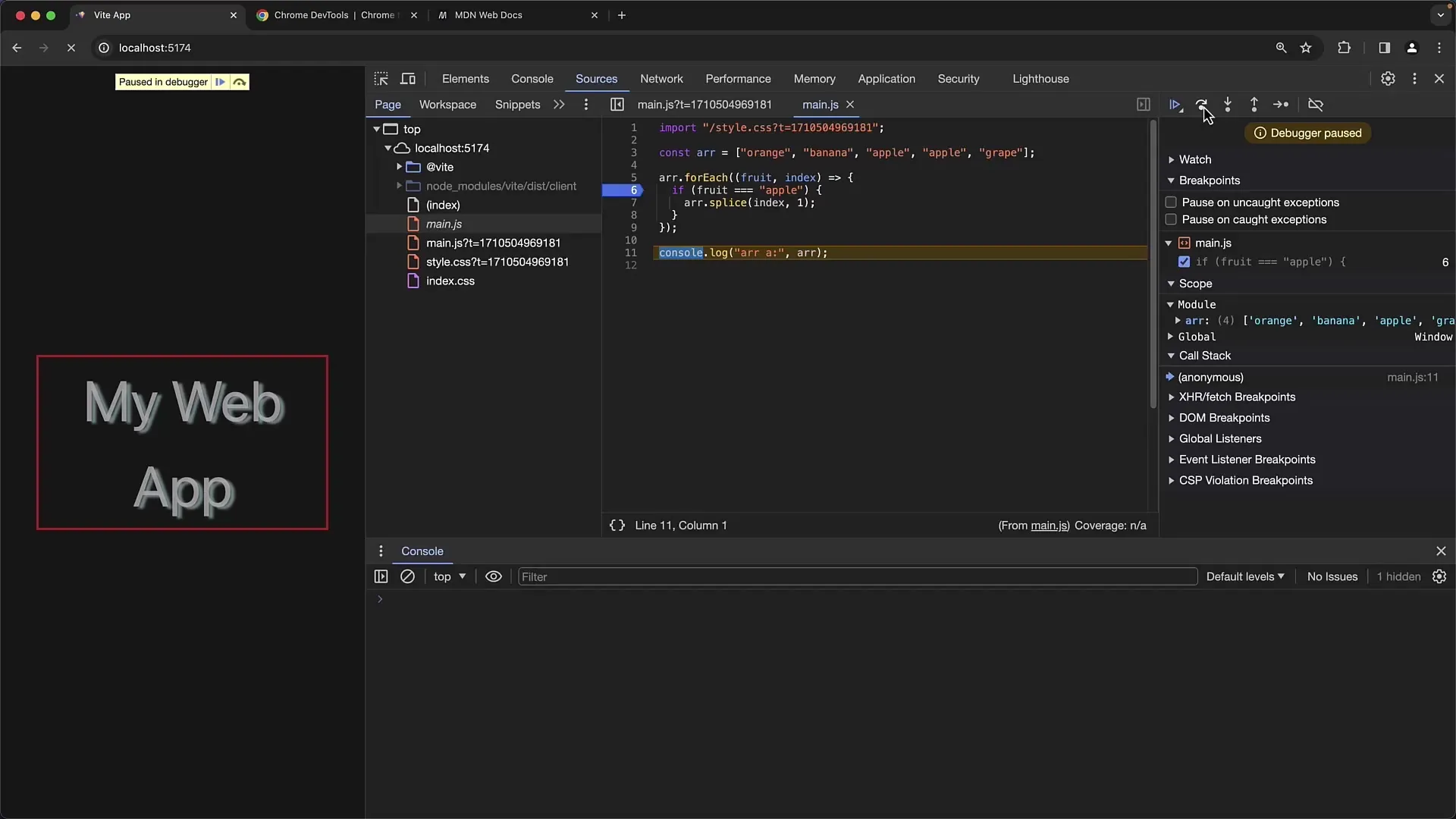
Step 6: Fixing the Code
Now you can fix the error by using a different method. Instead of forEach and splice, you can use the filter method. This way, you create a new array that contains only the desired elements.
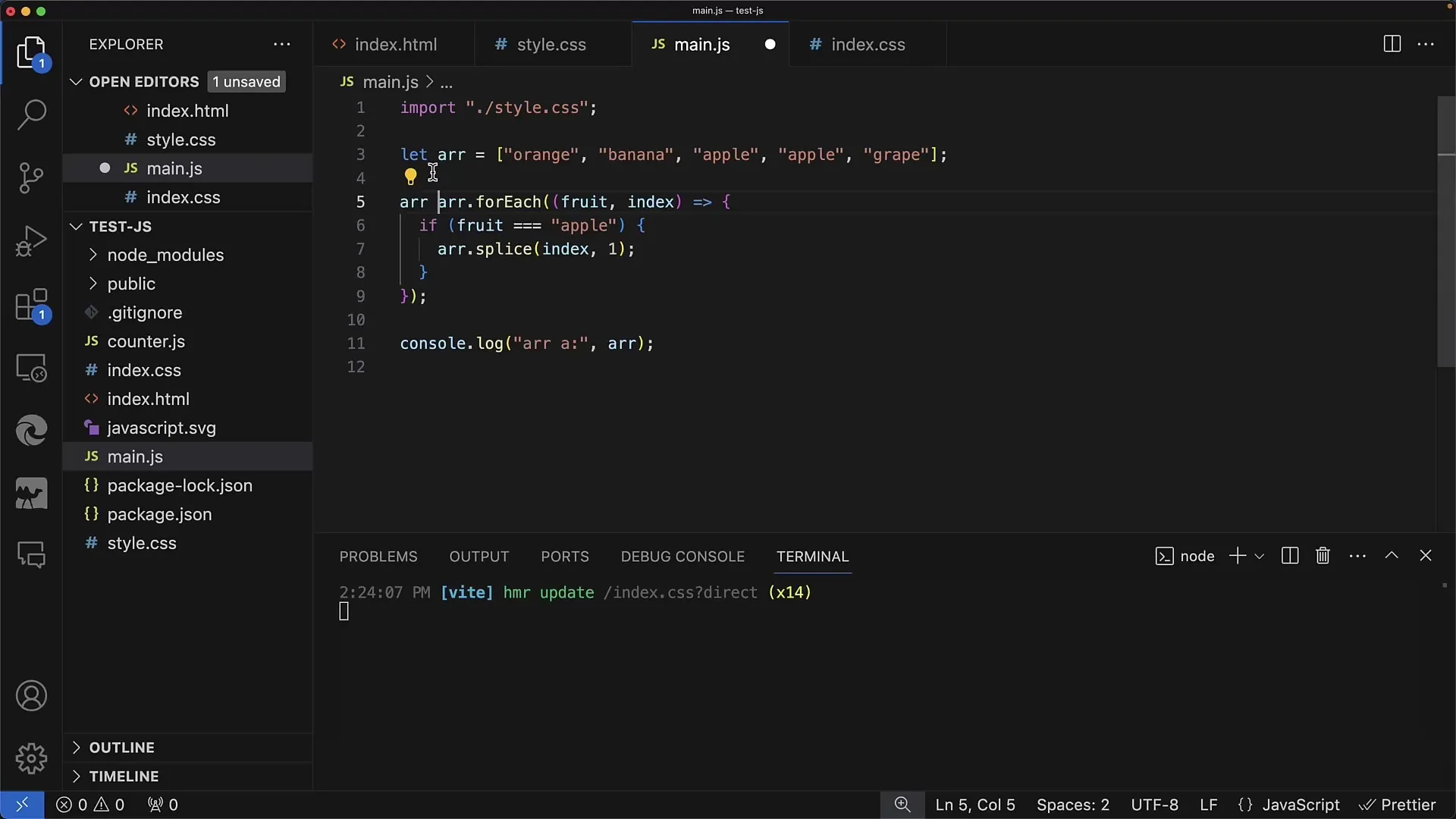
Step 7: Implementing the filter Method
You can simply specify that you want a new array that contains all elements that are not equal to "apple". The code becomes simpler and no index checks are necessary.
Step 8: Retesting the Code
Test the new code and if necessary, set a breakpoint again to ensure that only the desired fruits are included in the array. Check the output and make sure that the "apple" entries have been successfully removed.
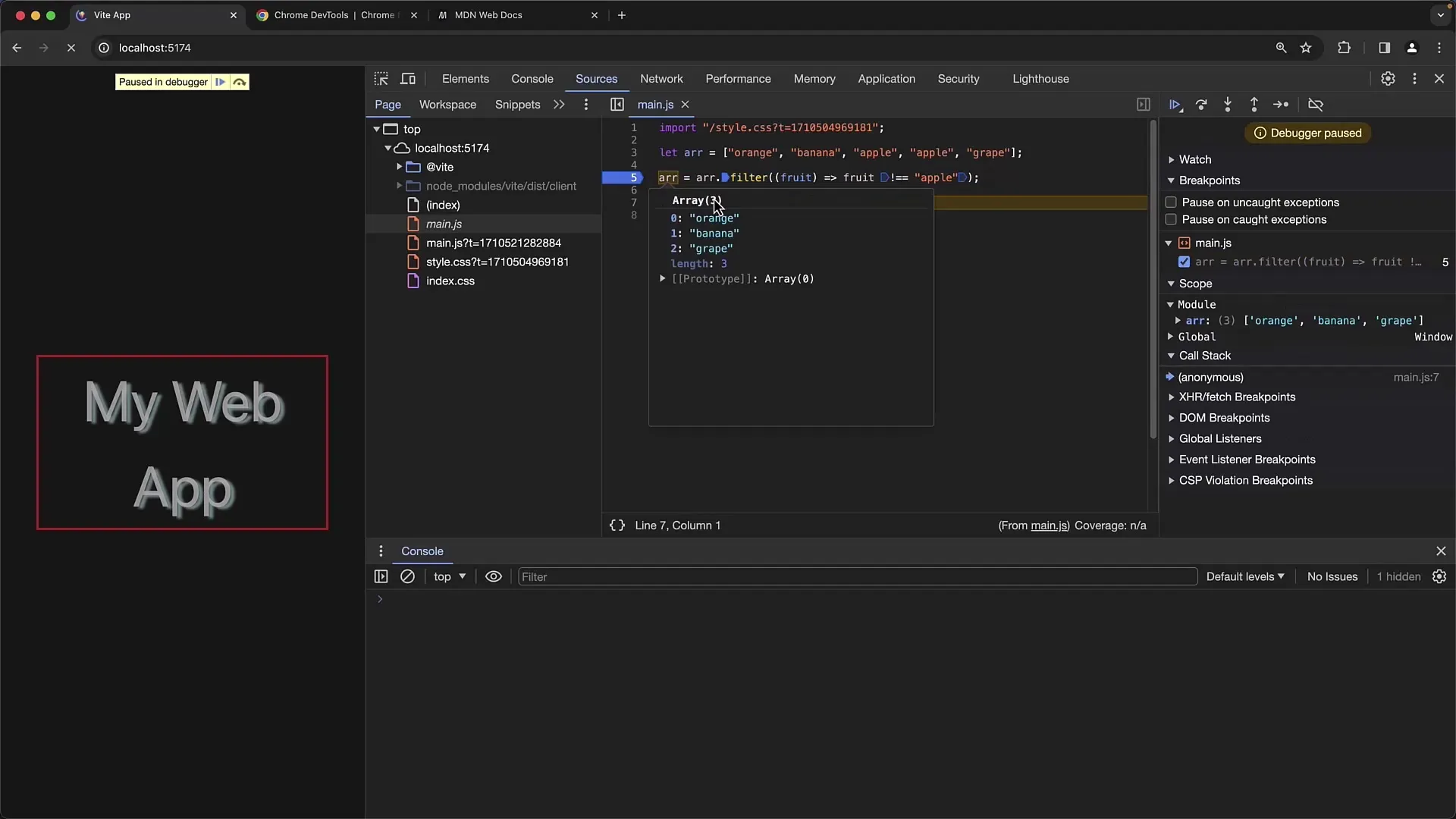
Step 9: Completing the Debugging Process
Make sure you fully understand how the code works and that the bug fixes are reflected in the code quality. Now you know how to proactively avoid errors when manipulating arrays and what tools can help you quickly identify these errors.
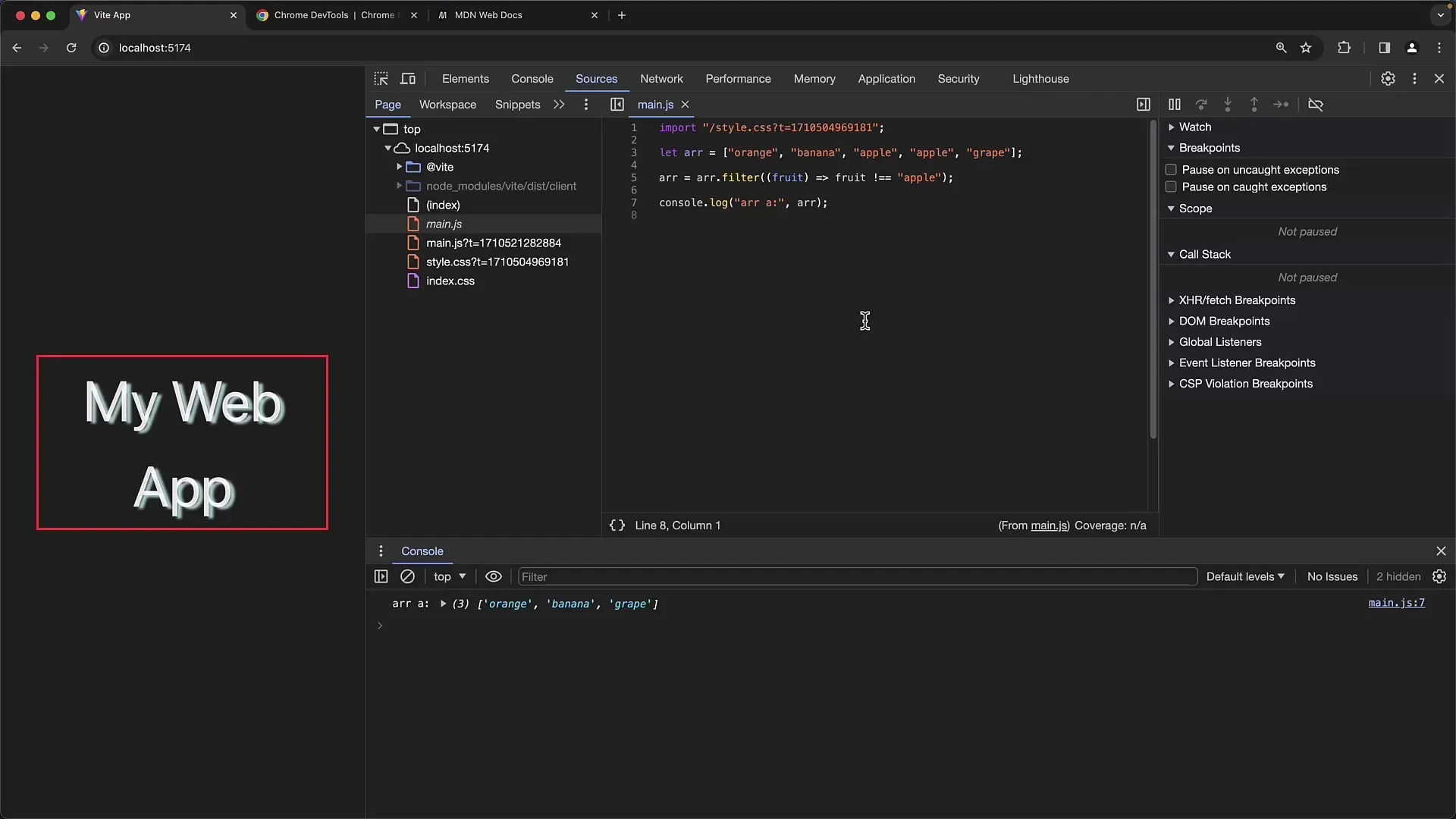
Summary
In this tutorial, you have learned how to effectively debug with Chrome Developer Tools, especially in relation to array manipulation. You have identified important sources of errors and developed approaches to fix the errors.
Frequently Asked Questions
Why doesn't the code remove all "apple" entries from the array?The code uses splice within a forEach loop, which causes the indices to shift and skips some entries.
What is the advantage of using the filter method?The filter method creates a new array and avoids issues with shifting indices, resulting in clearer and less error-prone logic.
How can I better utilize Chrome Developer Tools?Use breakpoints and monitor local variables to understand the current state of your code during execution and to detect problems early.