In this guide, you will learn how to securely and correctly implement password fields in web forms. This is a fundamental aspect of web development, especially when it comes to user input and data protection. Password fields are essential in many applications as they ensure the security of user data. You will learn the key points to consider when implementing a password field and receive a step-by-step guide to make these input fields secure.
Main Takeaways
- Password fields must always be transmitted using the "Post" method to ensure the security of the transmitted data.
- They do not automatically encrypt the inputs, so the use of HTTPS is essential.
- Avoid using "GET" as it makes passwords visible in the URL, posing a security risk.
Step-by-Step Guide
To create a password field in your web form, follow these steps:
Step 1: Create the basic structure of your form
First, you should create the basic structure of your HTML form. Here we define the form and the necessary input fields, including the password field. Make sure to set the method to "post" to securely transmit the entered data.
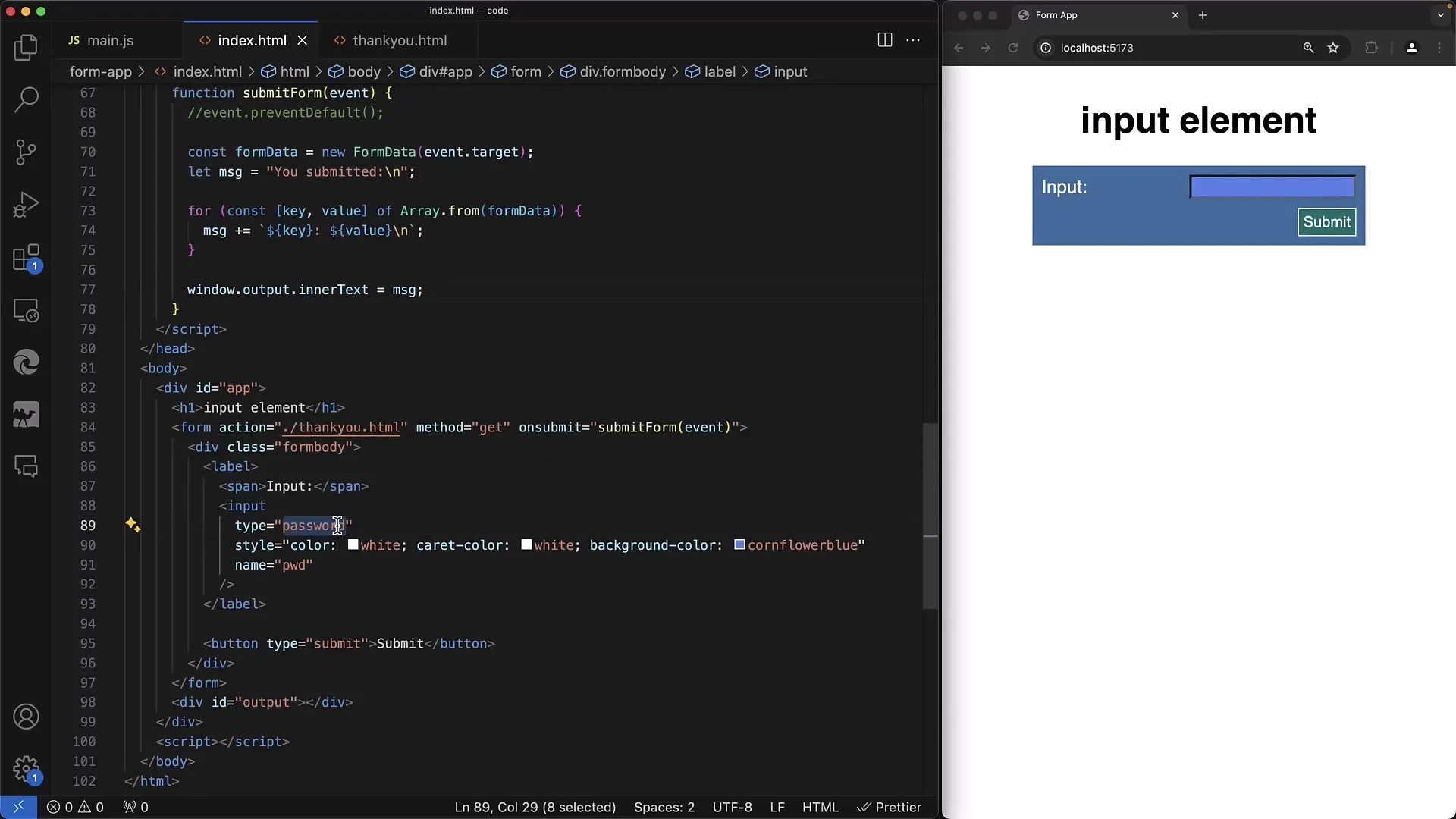
Step 2: Add the password field
To add a password field, use the input tag with the type attribute set to "password". This ensures that the characters entered by the user are not displayed in plain text.
Step 3: Prevent default submission
When the form is submitted, we prevent the default submission to correctly process the entered data. This is usually done with JavaScript, where we use preventDefault to ensure we have control over the submission process.
Step 4: Check the submission method
Check the method used for submitting the form. The submission method should always be set to "Post" to guarantee the security of the provided passwords. When using "Get", information including passwords could appear in the URL and thus be easily accessible.
Step 5: Demonstrate data transmission
After entering the password, demonstrate that the data is not visible in the URL. This can be done through your browser's developer tools. Check the network requests to ensure that passwords are sent in the body of the request and not visible in the URL.

Step 6: Use HTTPS for secure transmission
Ensure that you use HTTPS for your web project. This protects the transmitted data through additional encryption. All your form data, including passwords, will be secured by HTTPS, making it significantly harder for hackers to intercept your data traffic.
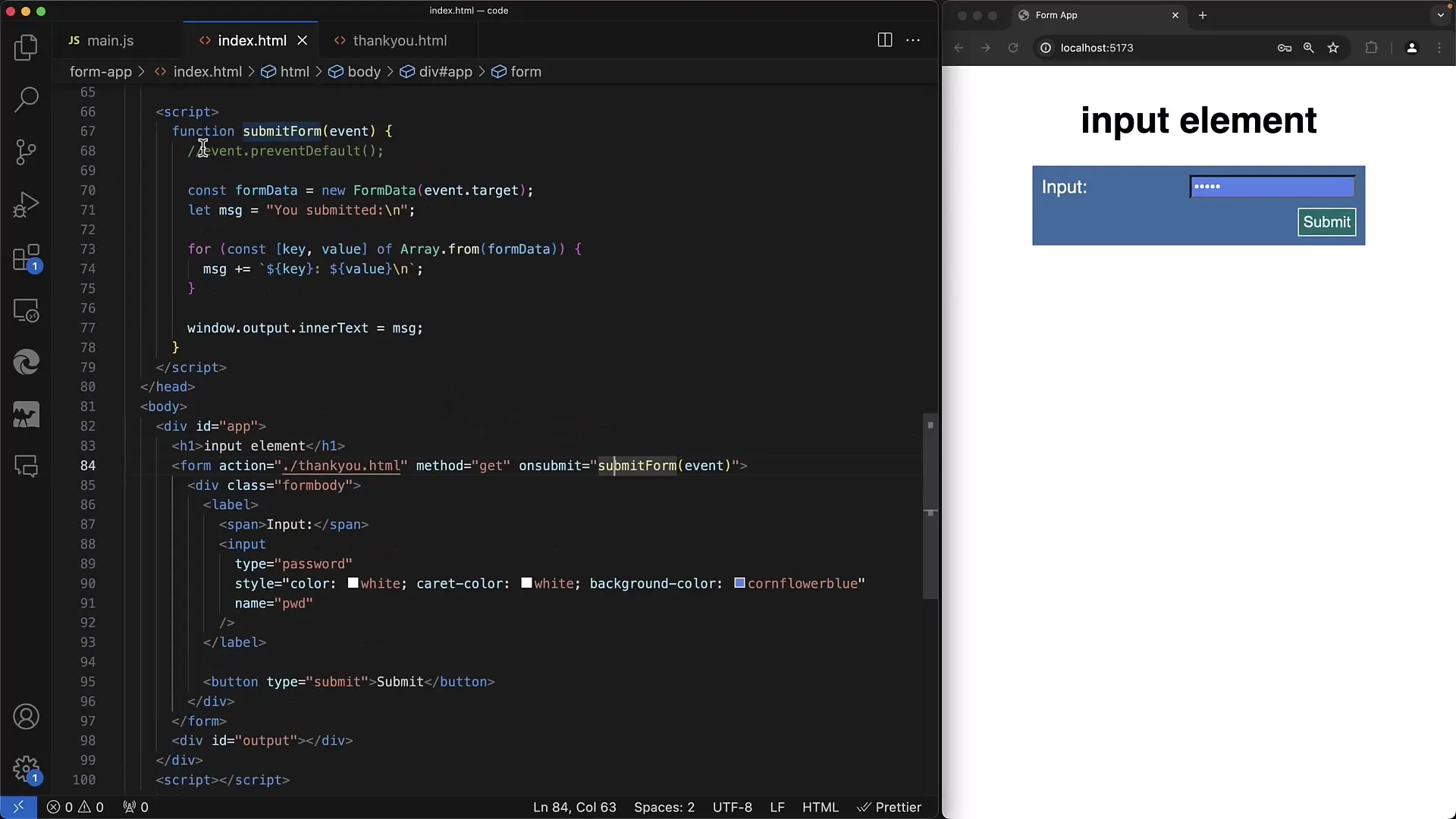
Step 7: Deal with password management
Password fields are also important for password managers, which allow users to securely store their passwords. Remember that it is important to ensure that these managers can process the entered data correctly and securely.
Step 8: Input validation and security
Validating user inputs is another step in this process. You should ensure that users use strong passwords containing at least a combination of letters, numbers, and special characters. Client-side validation is easily accomplished using JavaScript.
Step 9: Avoid simultaneous entries
When prompting for a new password and its confirmation, make sure to compare the two fields. Some basic validations can be performed on the client side to ensure that the entries match before the form is submitted.
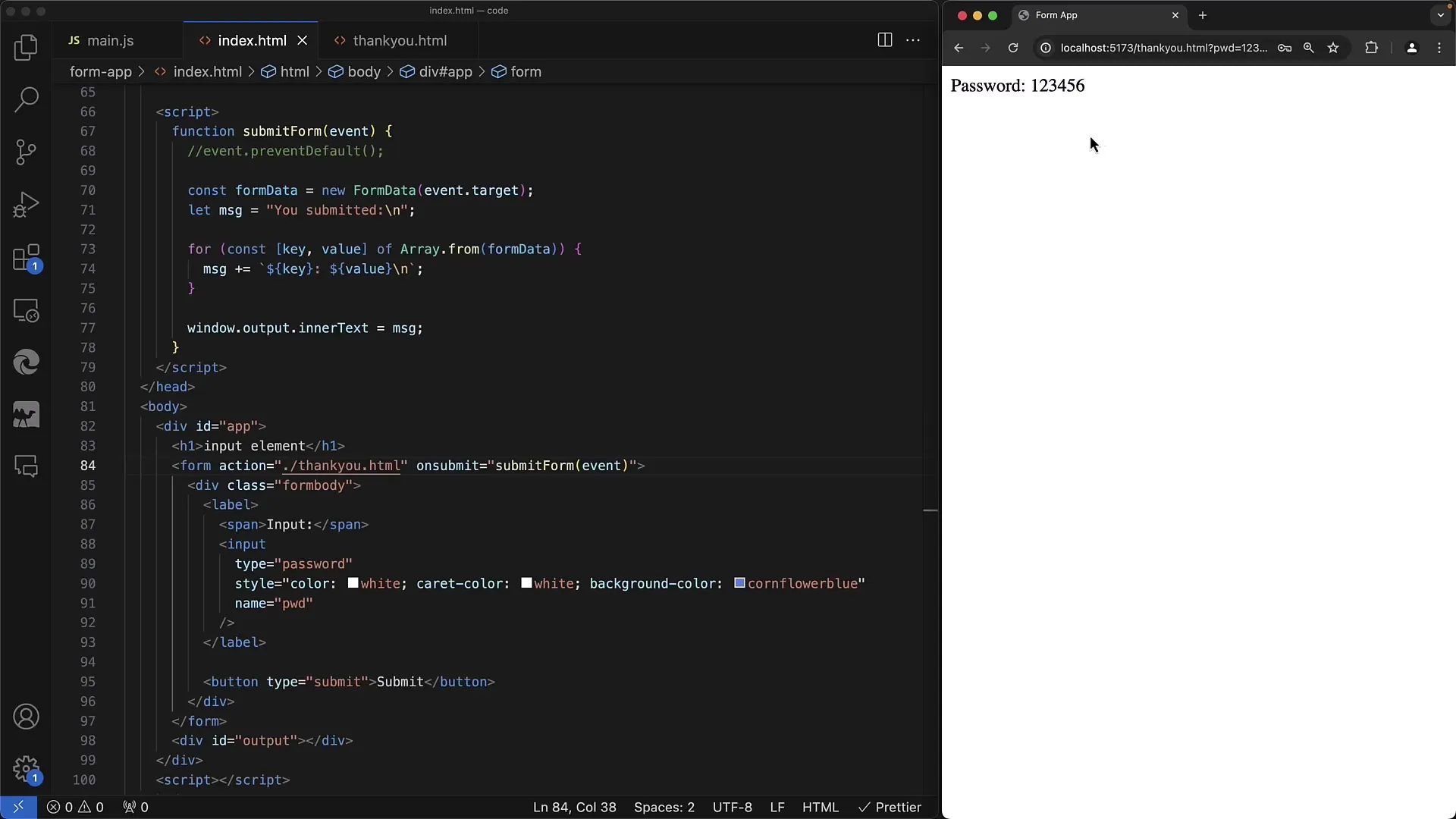
Summary
You have now learned how to set up a password field in a web form and can adhere to the following security guidelines to protect user data. The key points include using the "Post" method, ensuring HTTPS, and validating user inputs. Make sure to support users well when entering passwords and ensure that your implementation meets security standards.
Frequently Asked Questions
What is the difference between "GET" and "POST"?"GET" can transmit data in the URL, which is insecure, while "POST" does this in the request body, which is more secure.
Why should I use HTTPS?HTTPS ensures that the data being transmitted between the client and the server is encrypted, which increases security.
How can I perform input validation in JavaScript?You can retrieve the values of the input fields using element.value and validate your desired conditions in a function.