In today's tutorial, I will show you how to receive form data on a server using Node.js and Express. Together, we will set up a simple server and go through the necessary steps to create forms that send data to this server. This guide is aimed at anyone with a basic understanding of JavaScript and Node.js who wants to expand their skills in web development.
Key Takeaways:
- Basics of creating a simple Node.js server with Express
- Setting up the project directory and installing required packages
- Creating an HTML file for displaying and using the forms
- Server-side processing of sent data
Step-by-Step Guide
First, we need to set up a server to process the incoming form data. Therefore, we start by creating a new Node.js project.
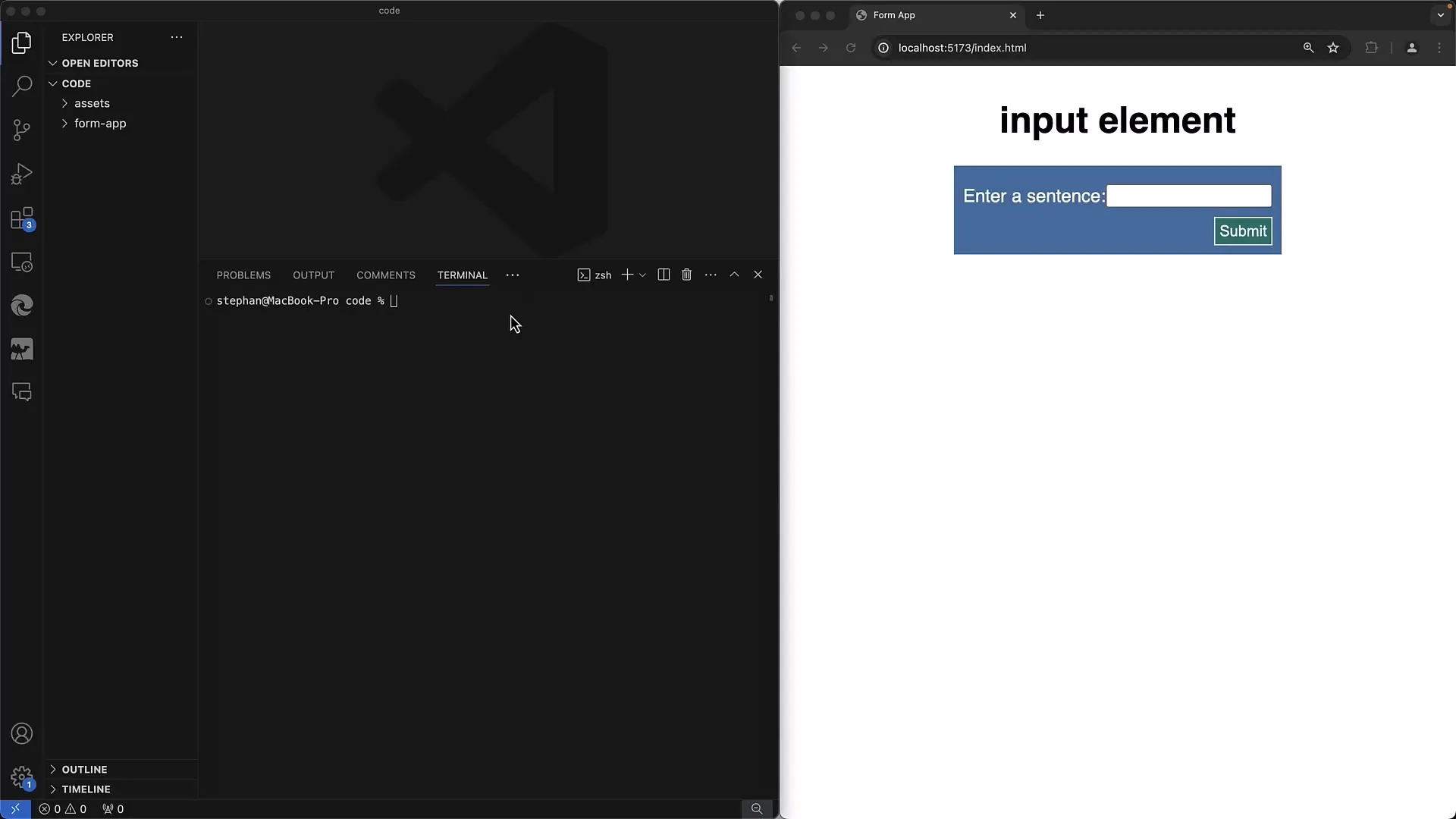
Open your editor, for example, Visual Studio Code, and open a terminal. Alternatively, you can use a regular terminal. Make sure Node.js is installed on your computer.
Navigate to your main directory and create a new subdirectory for your server app. I suggest naming the directory "FormServerApp".
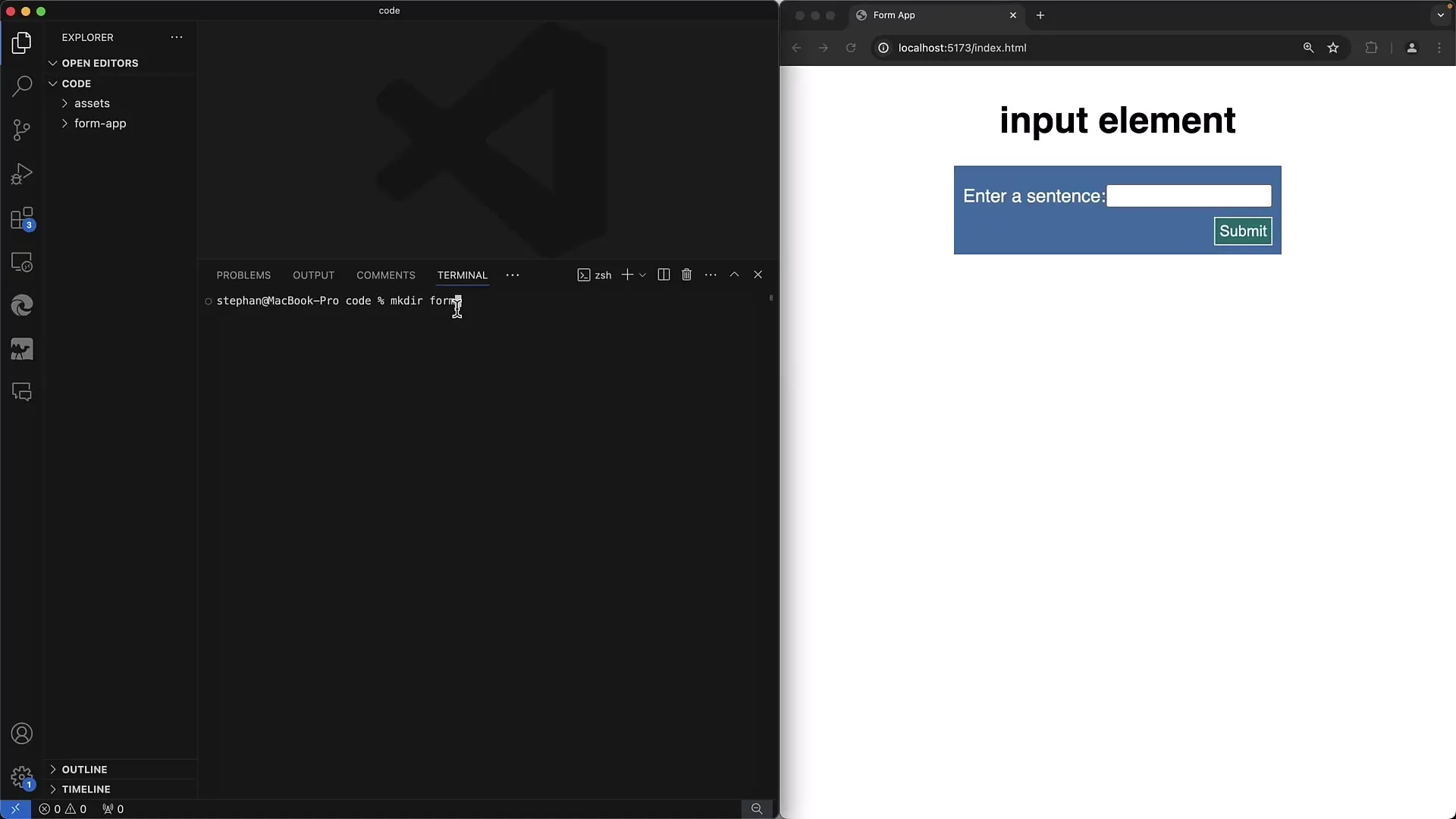
Change into the newly created directory and initialize a new Node.js project with the command npm init. You will be prompted to enter some information, such as the project's name, version, and entry point file. You can either accept the default values or enter your own.
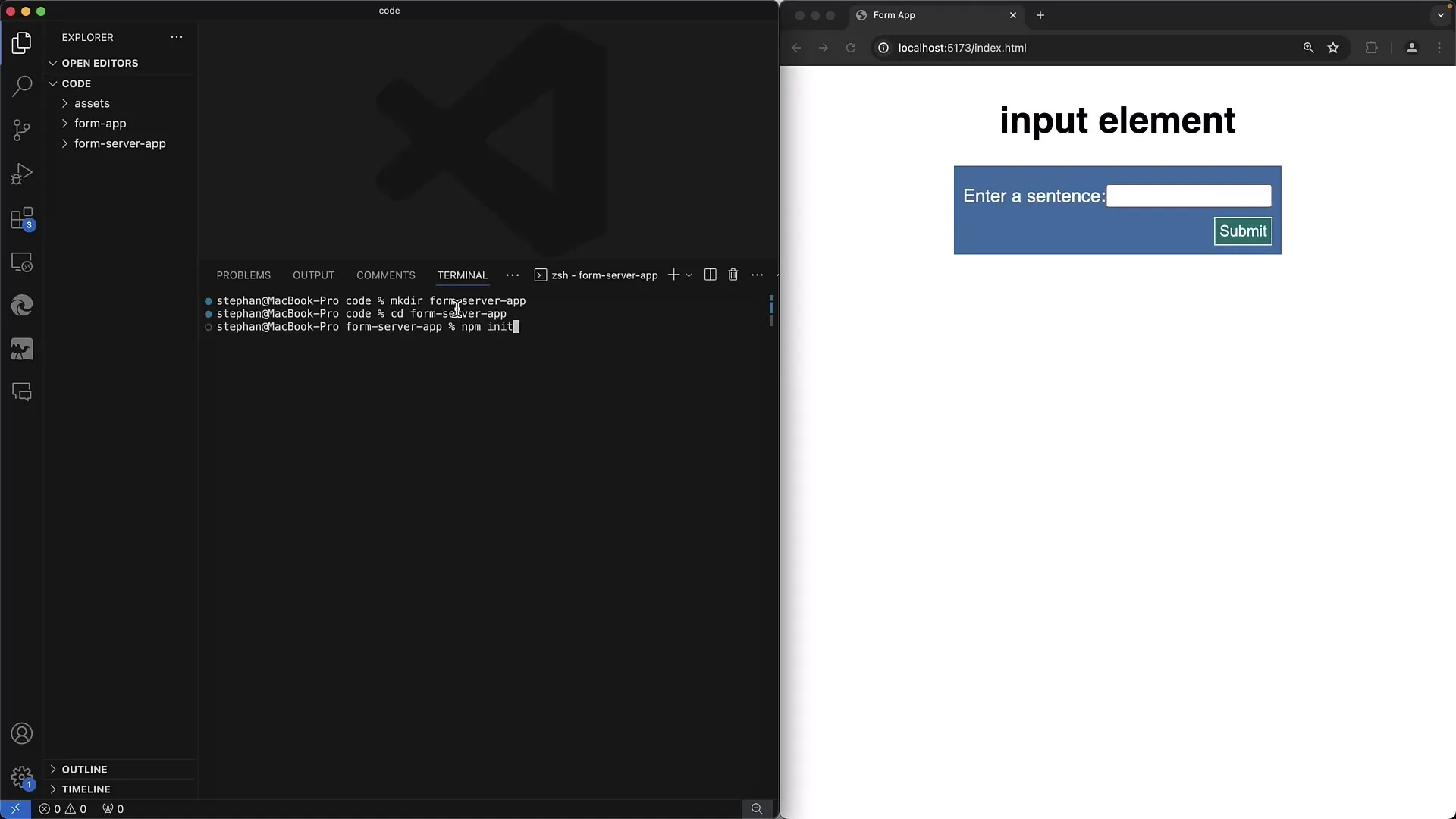
After creating the project, you will see a package.json file in the directory. This file contains all the metadata of your project. Now, we need to install Express, so we run the command npm install express.
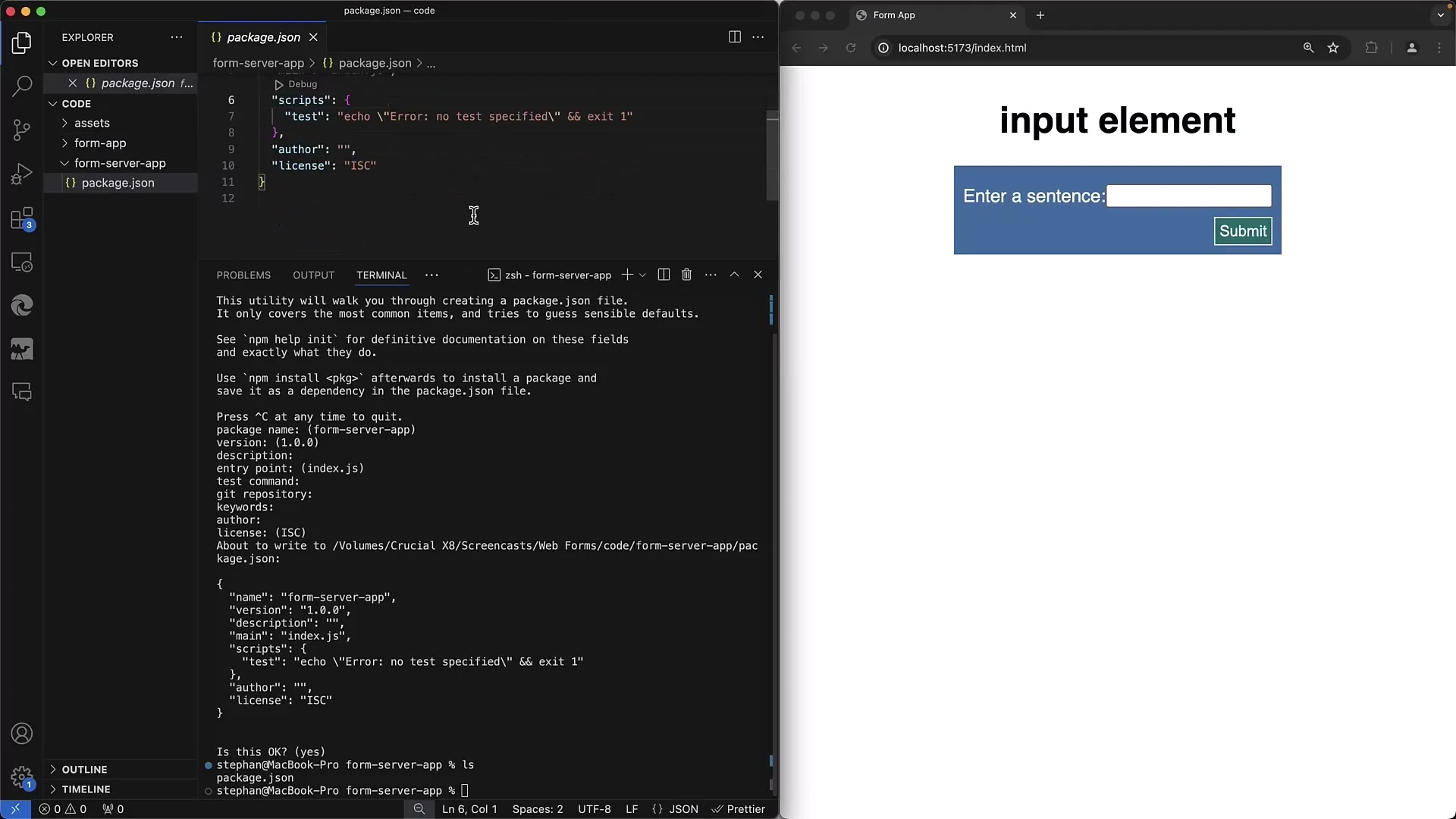
Once the installation is complete, check in the package.json if Express is listed under the dependencies. It is crucial to ensure that the installation was successful before proceeding.
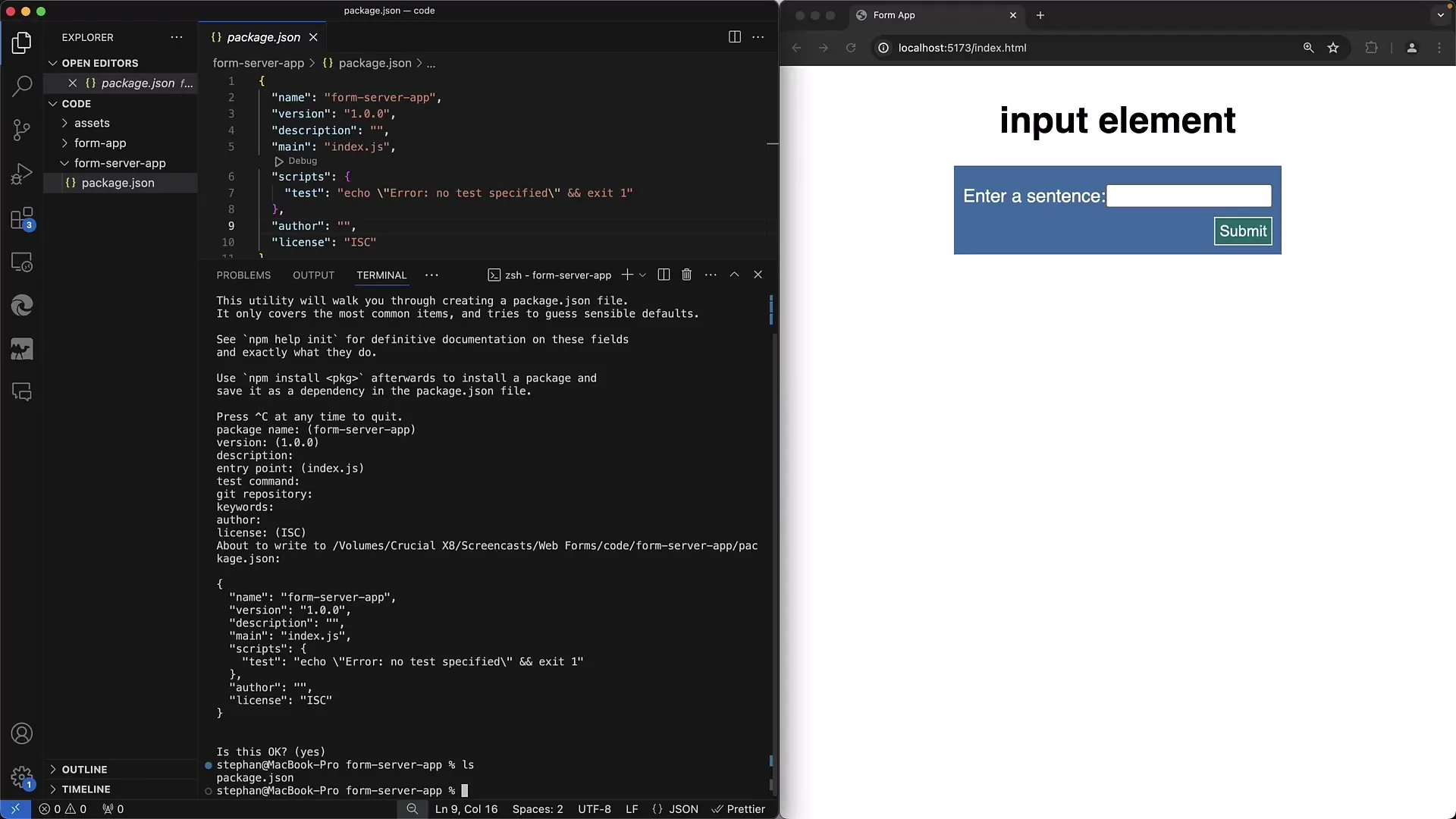
Next, we create a new file named index.js, which will serve as the entry point of our application. This file will contain the main logic for our Express server.
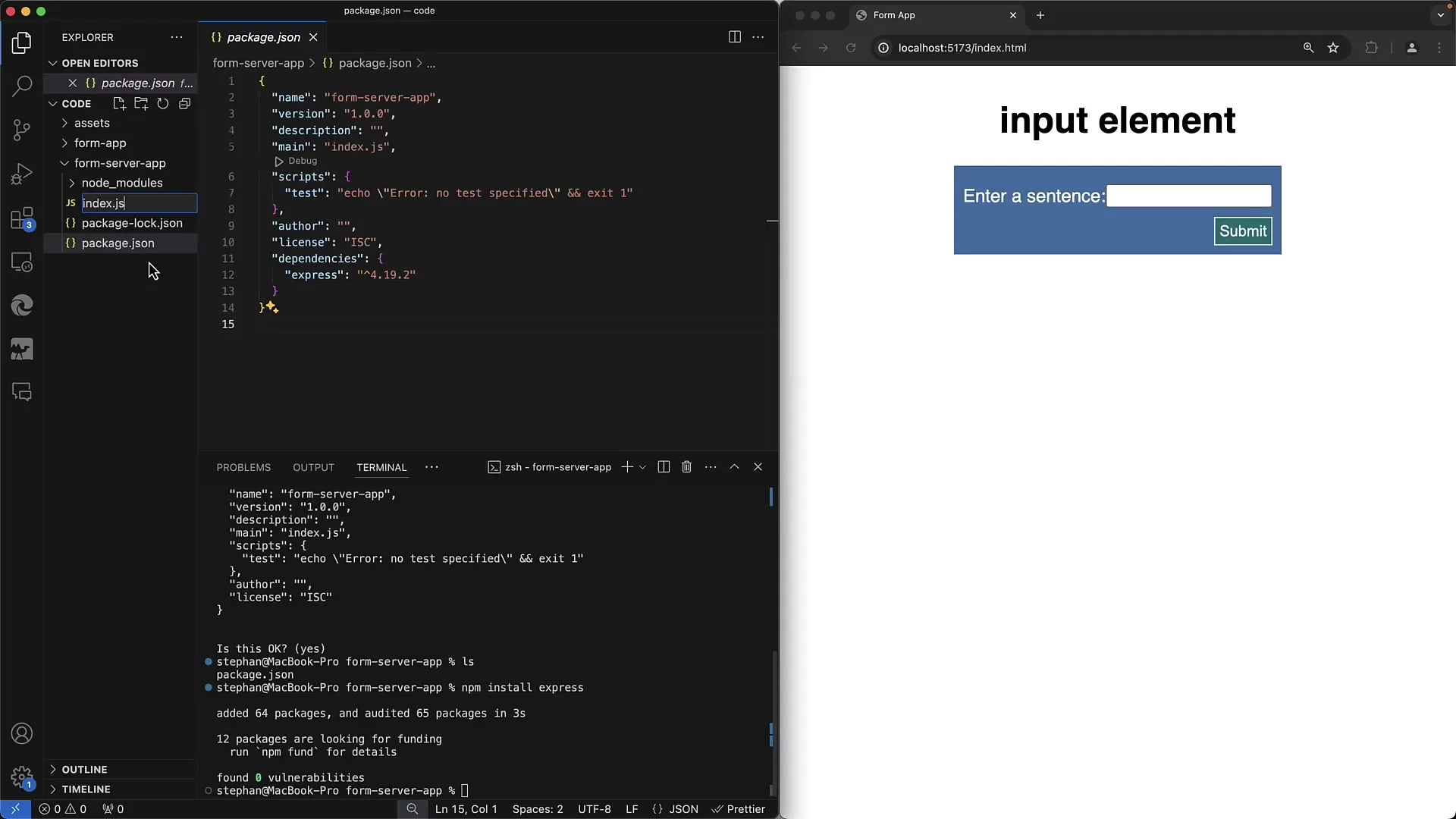
At the beginning, you can perform a quick test by adding console.log("FormServer"); to the index.js file and running it with node index.js to ensure everything is working properly.
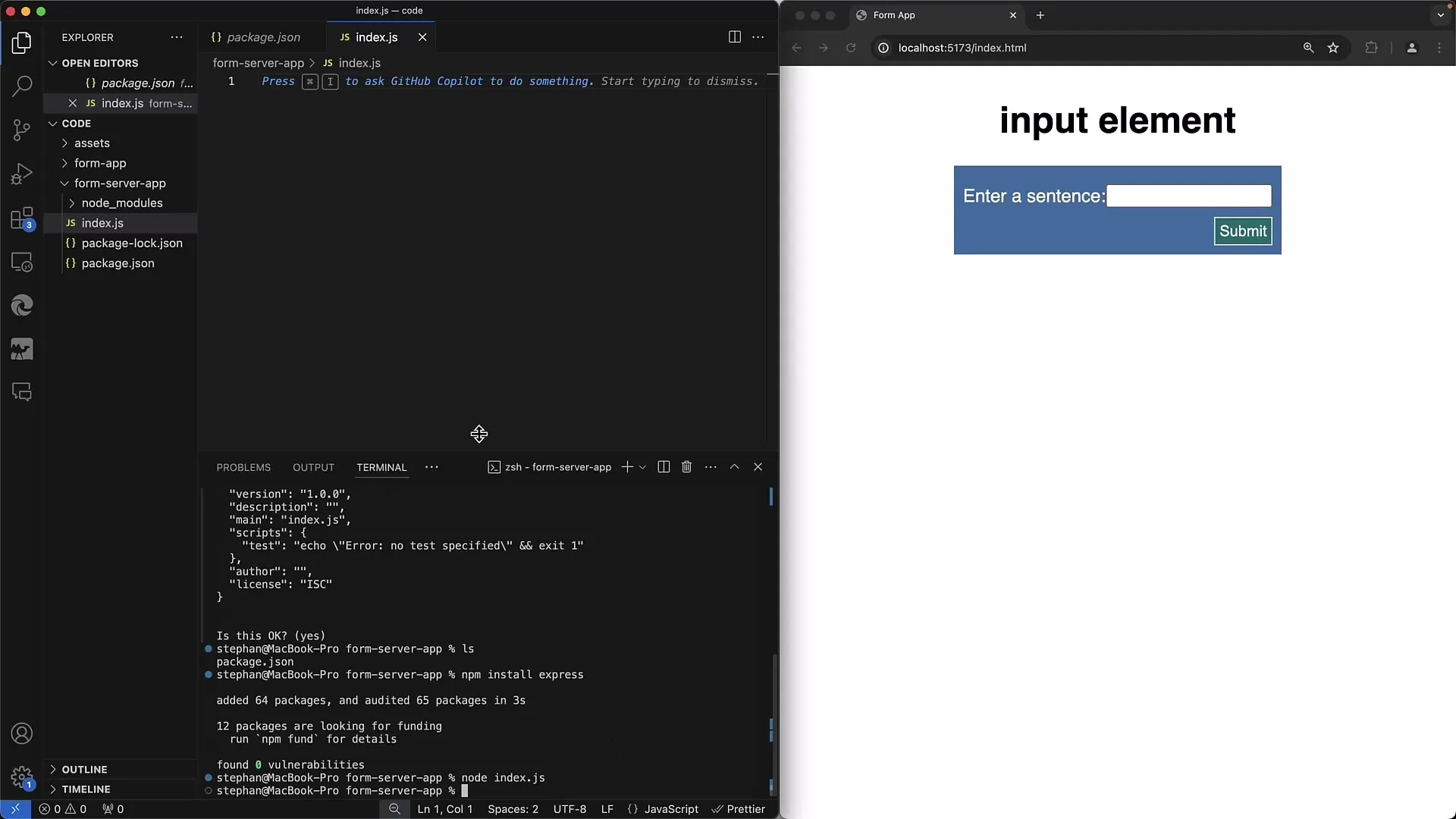
Now is the time to use Express in your index.js file. Add the necessary code to import Express and create an Express app. Here is a simple code to initialize an Express application and listen on a port.
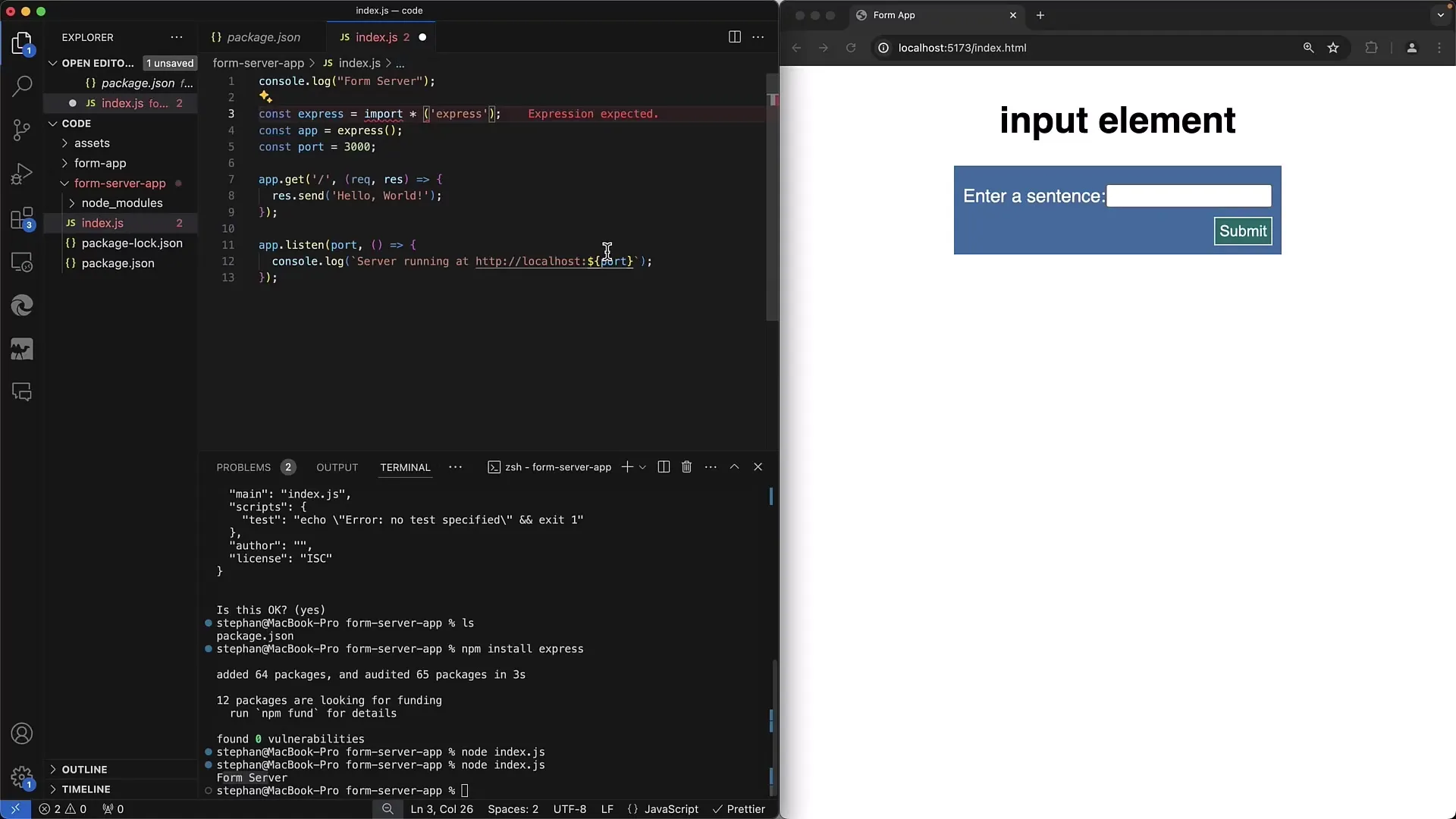
Make sure to run the server on a specific port, for example, 3000. Check if the application works correctly by visiting localhost:3000 in your browser. You should see the output "Hello World".
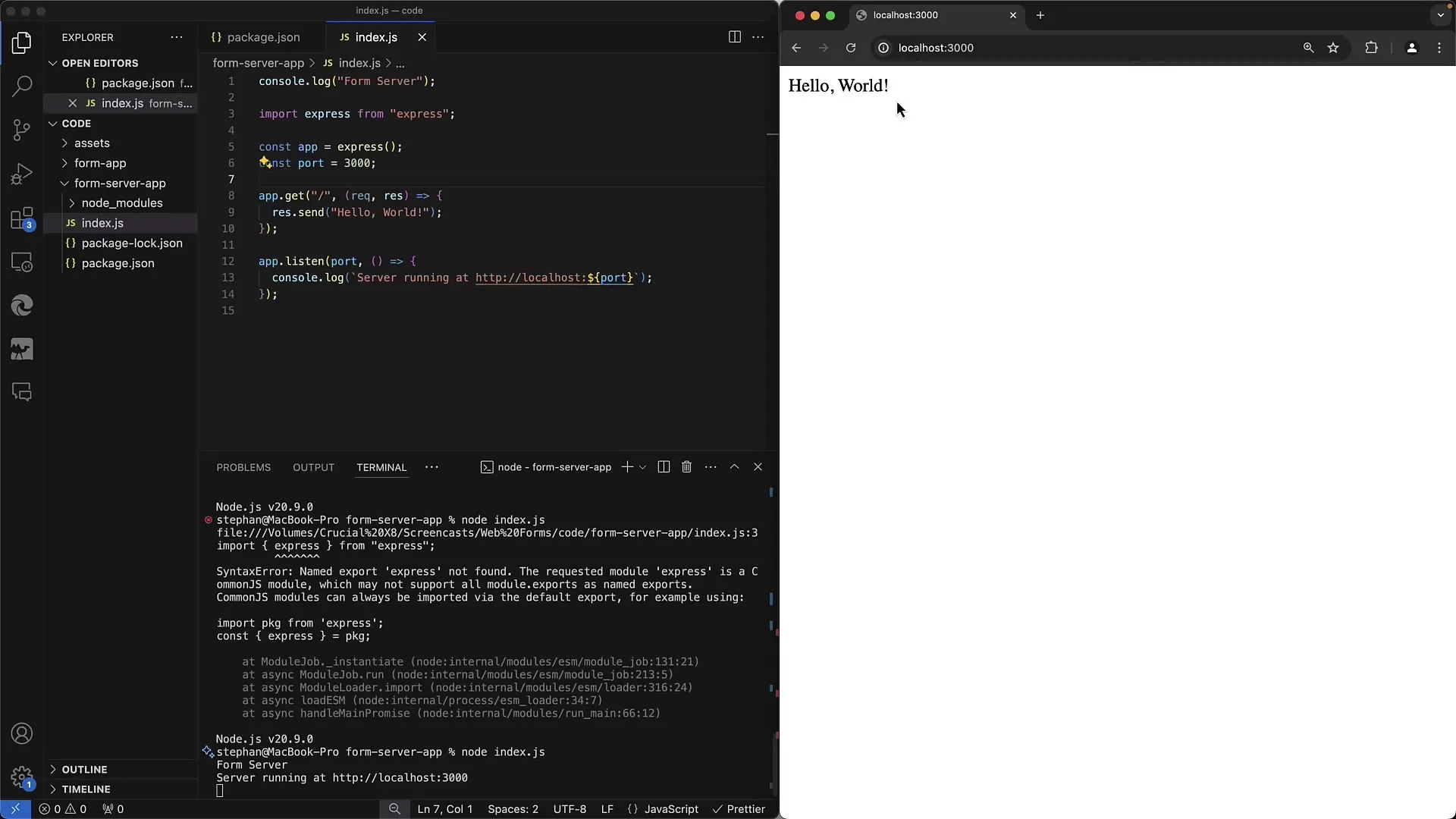
To support forms in your application, we now need an index.html file that contains the HTML structure for our form. First, create a new folder named "public" and place the index.html file inside it.
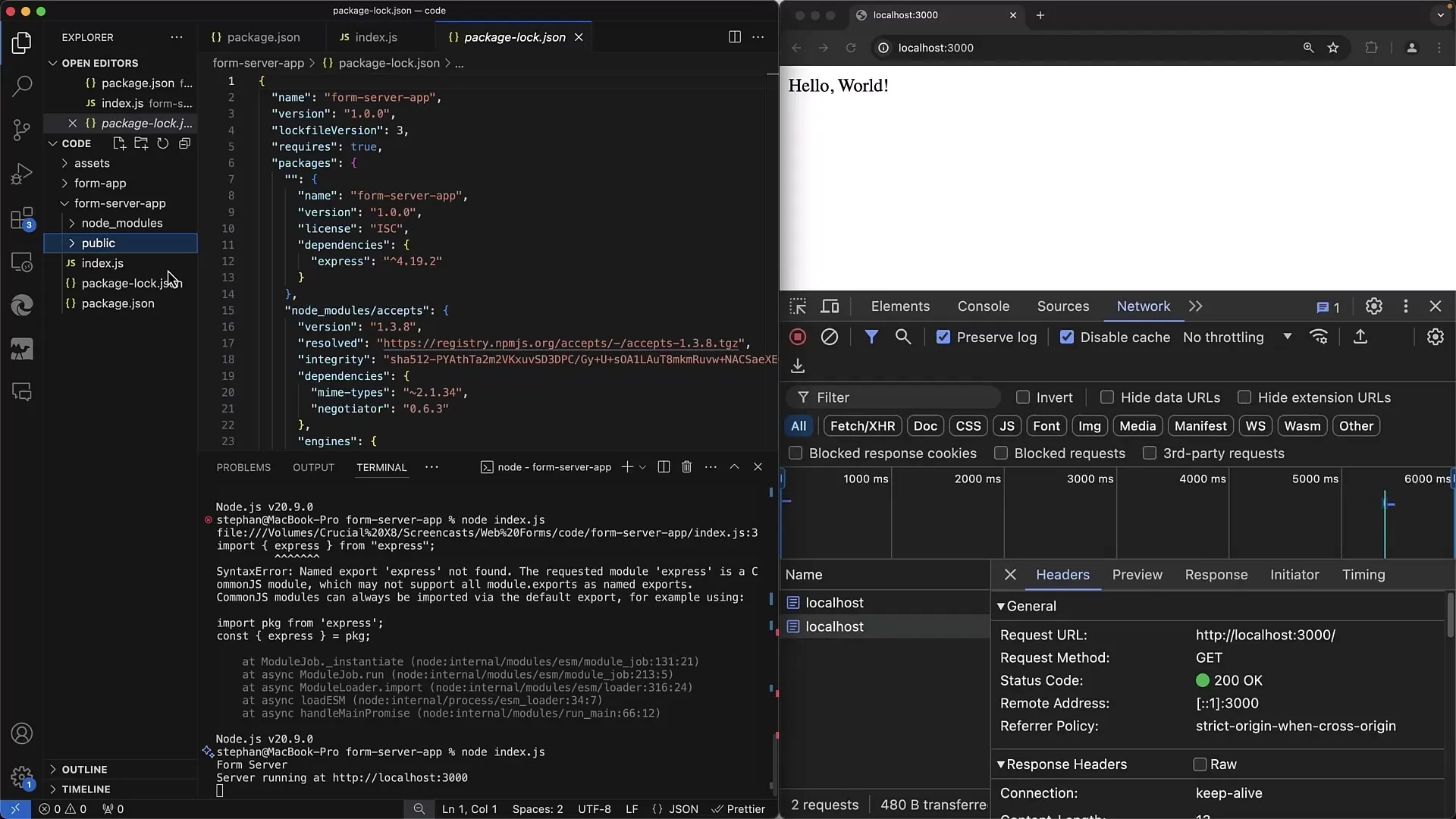
In the index.html file, you can simply insert a basic HTML structure with a form. The form will later send the submitted data to the server.
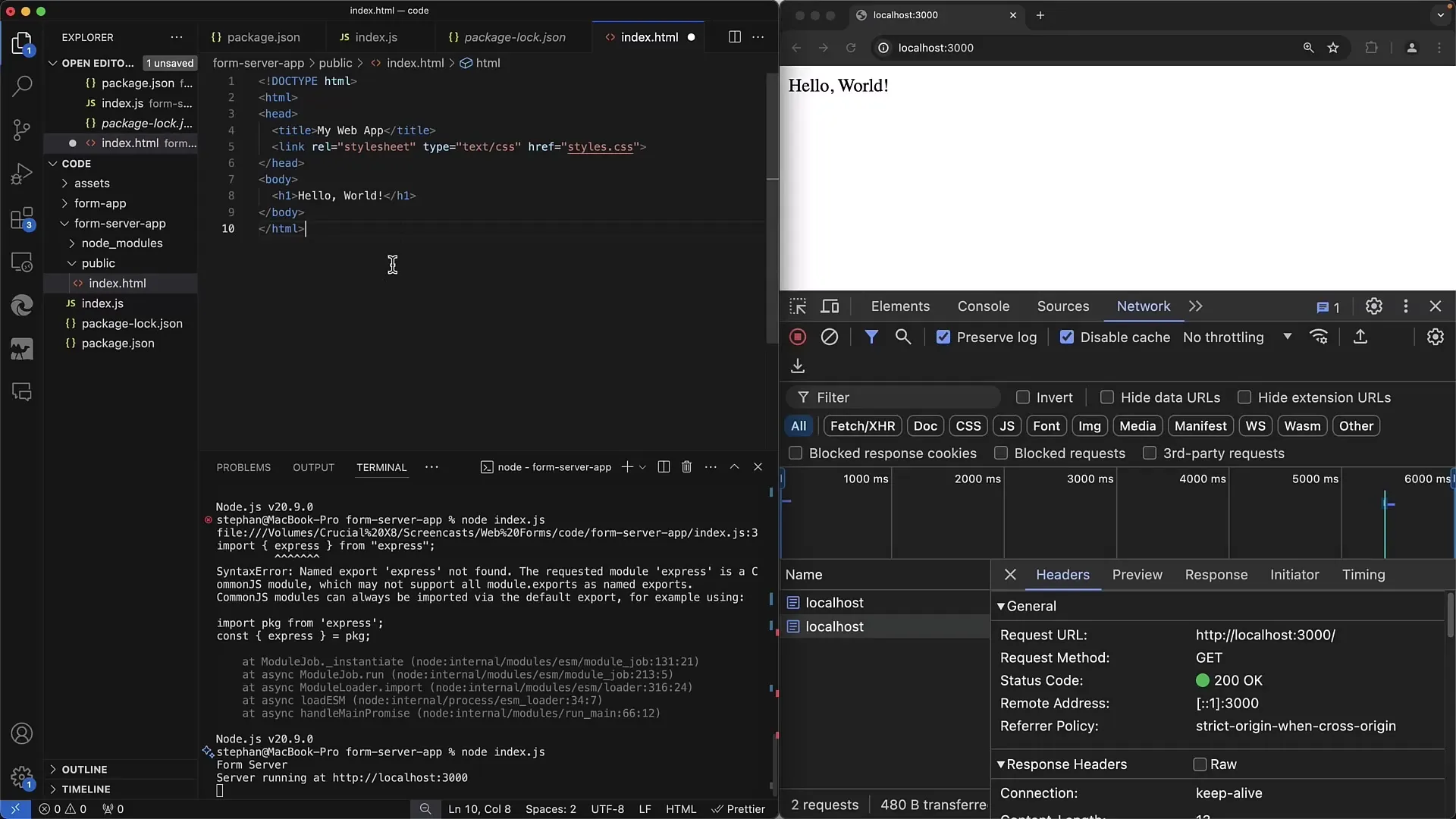
To serve the static files (HTML, CSS, JS) via the Express server, use the method app.use() to set the "public" folder as a static directory. This way, the browser can request the index.html file when you visit localhost:3000/index.html.
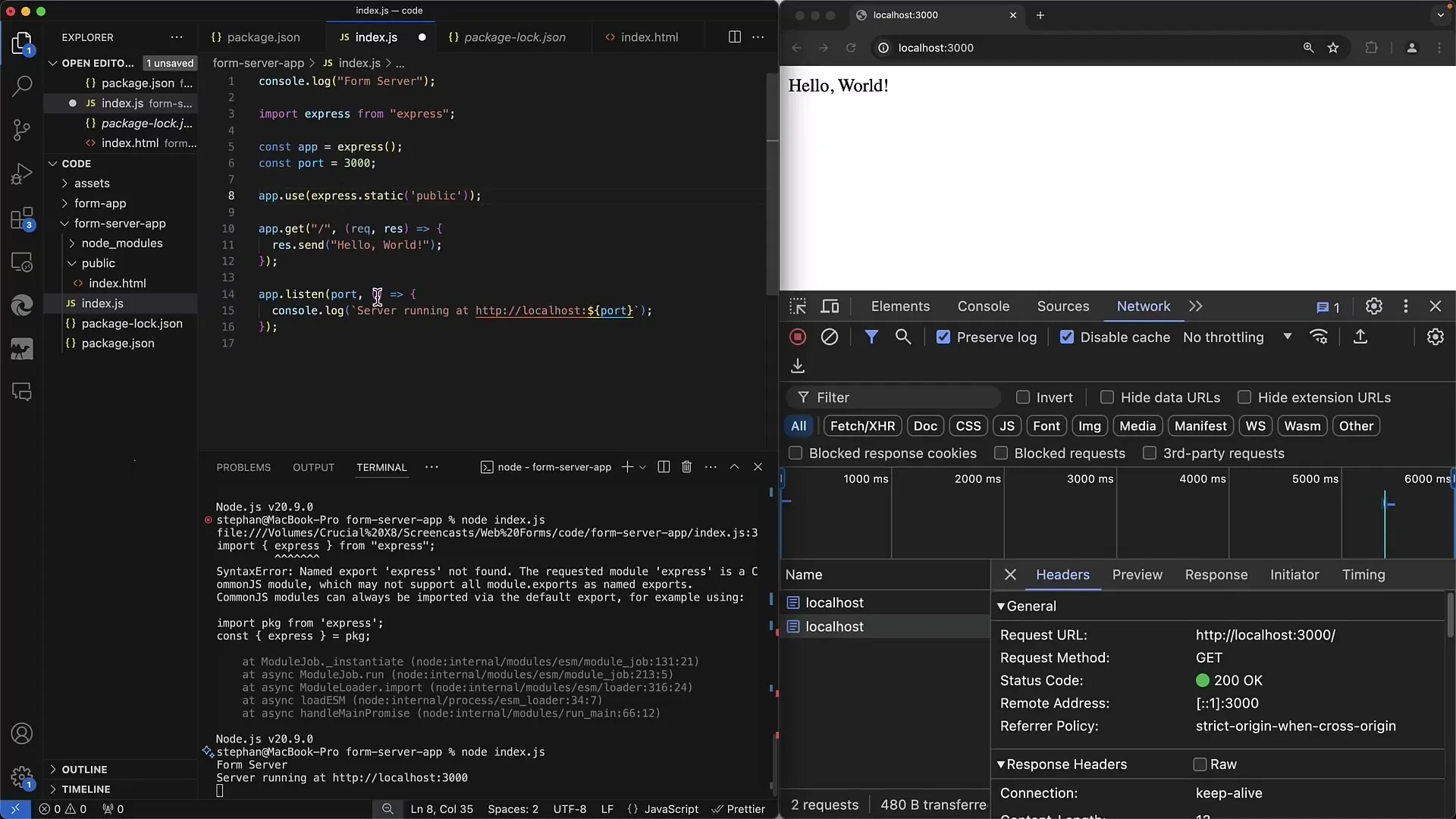
Now that you have started the server and loaded the index.html in the browser, you should be able to display the form correctly. However, when you fill out and submit the form, nothing will happen yet, as we have not implemented the server-side logic.
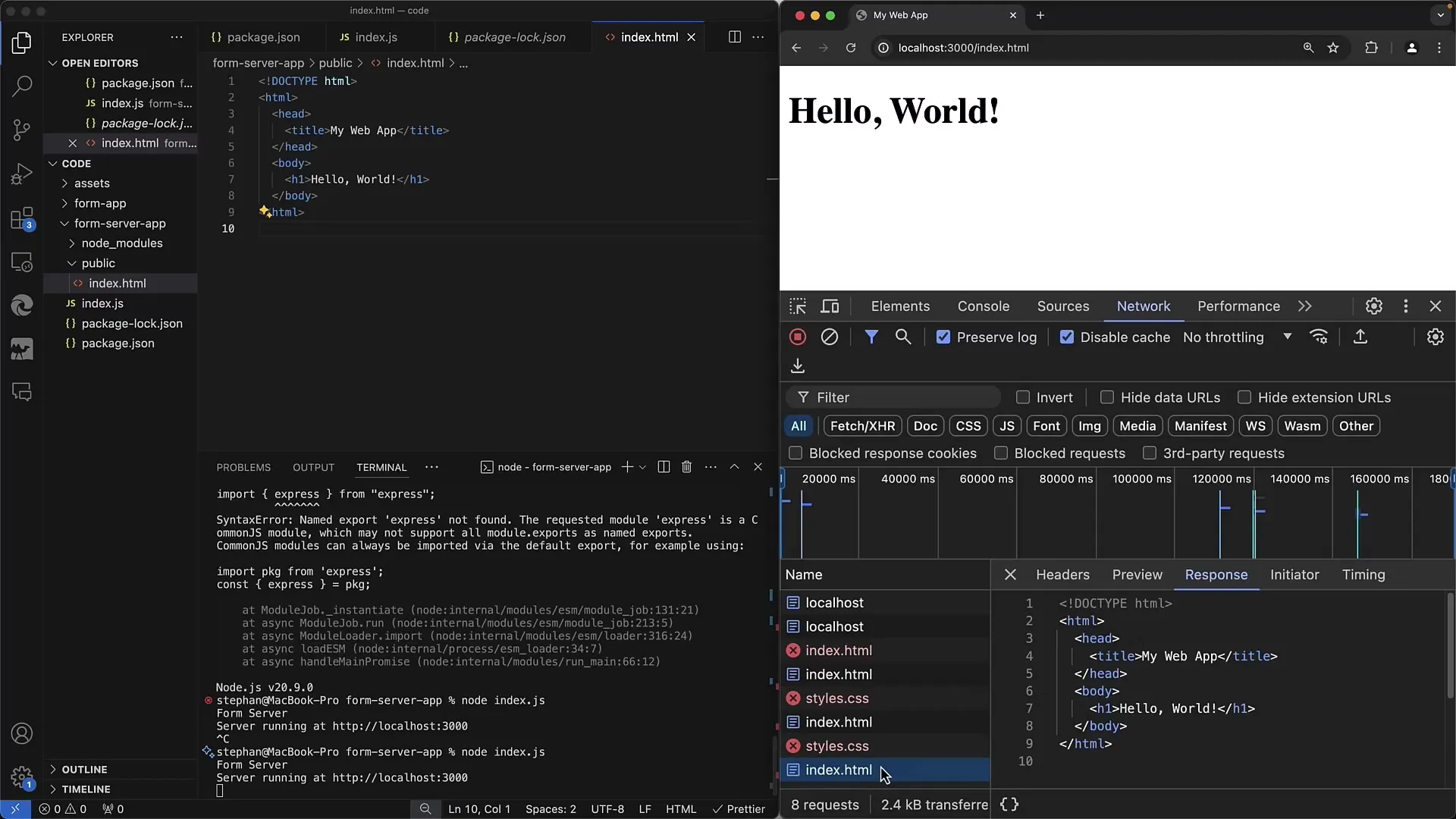
In the next step, we will focus on processing the form data on the server side. This means we need to add a route that receives and processes the data sent by the form. Get ready for the next steps to further develop your web application and add functionalities!
Summary
In this tutorial, you have learned how to set up a simple server with Node.js and Express. You have learned the basics of creating a Node.js project, installing dependencies, and delving into HTML forms. These steps are crucial for handling web forms on the server side.
Frequently Asked Questions
How do I install Node.js?Node.js can be downloaded and installed from the official website.
What is Express?Express is a flexible Node.js web application framework that provides versatile features for web and mobile applications.
How can I send my form data to the server?You can create an HTML form and send the data to the server-side endpoint using Fetch or AJAX.