In this tutorial, you will learn how to receive and process form data using the GET method. You will create a simple HTML form and see how this data is sent to your server via the URL. We will discuss the basics of the GET request and outline the necessary steps in Express.js.
Key Takeaways
- Form data can be sent to the server using the GET method.
- The transmitted data appears as query parameters in the URL.
- You can easily access and process the query parameters of the request.
Step-by-step Guide
First, we start by creating an HTML form. The action of your form is crucial as it specifies where the form data will be sent.
To create a simple form, add the following:
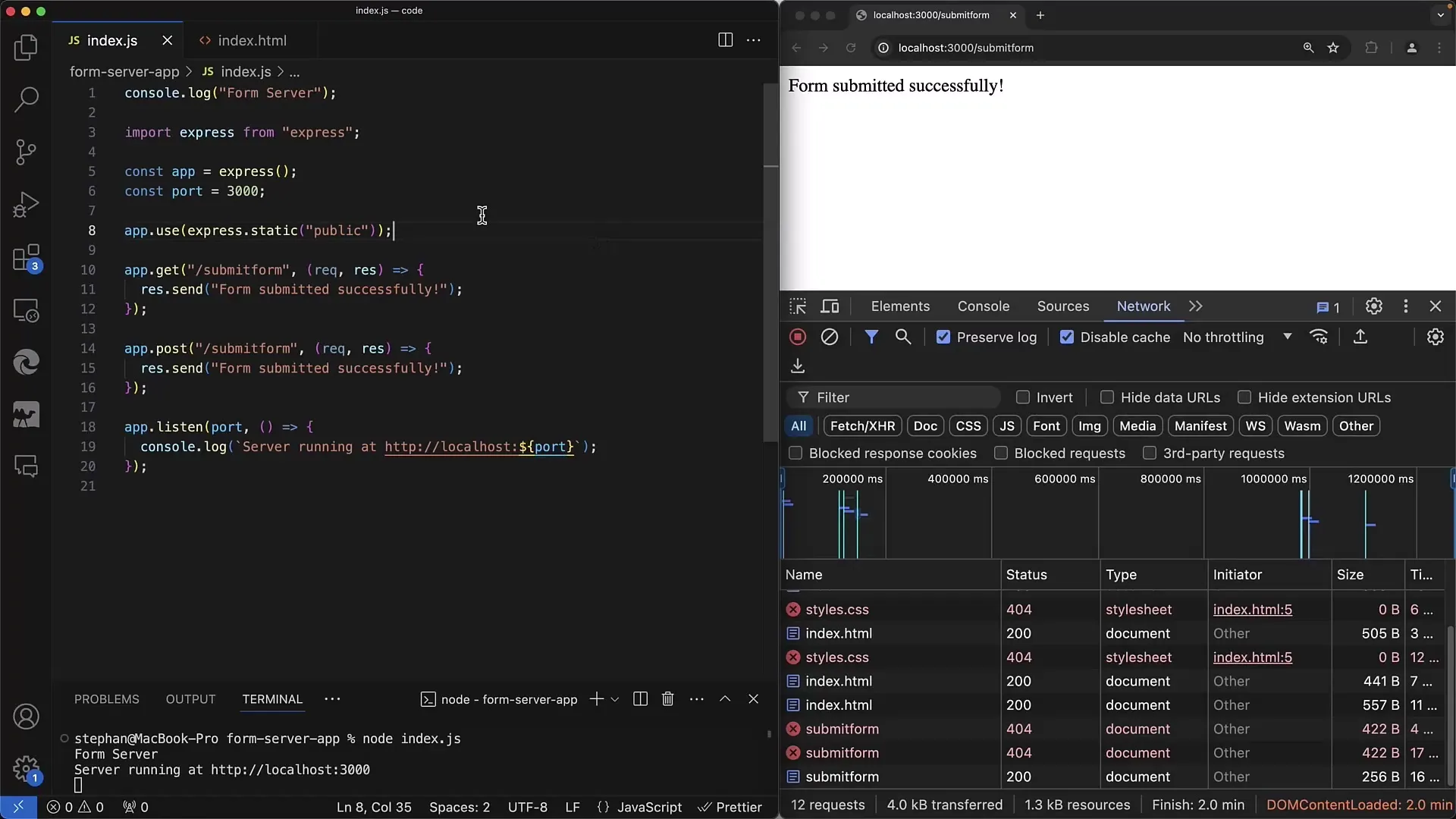
The action of the form contains the path to which the data should be sent, in our case /submitform. There, the GET handler will later be defined in our Express server. For input, we use a simple text field:
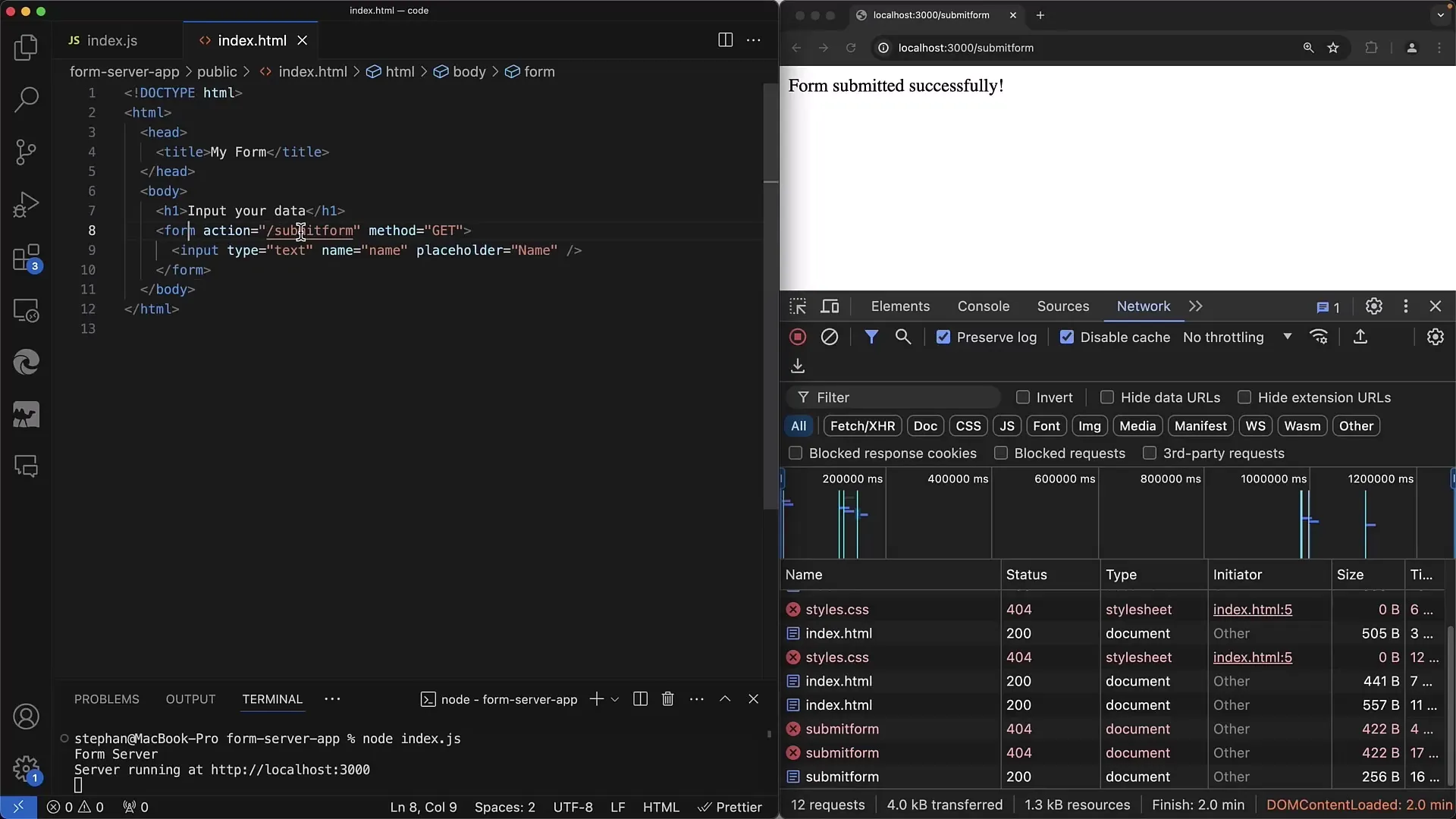
Once the form is set up, we should ensure that the server is ready to receive the data. You need to make sure that you have configured the GET method in your Express server to handle the requests.
Next, refresh the page to ensure everything is working. If you enter something in the text field, you can submit the form by pressing the Return key, even if there is no submission button available.
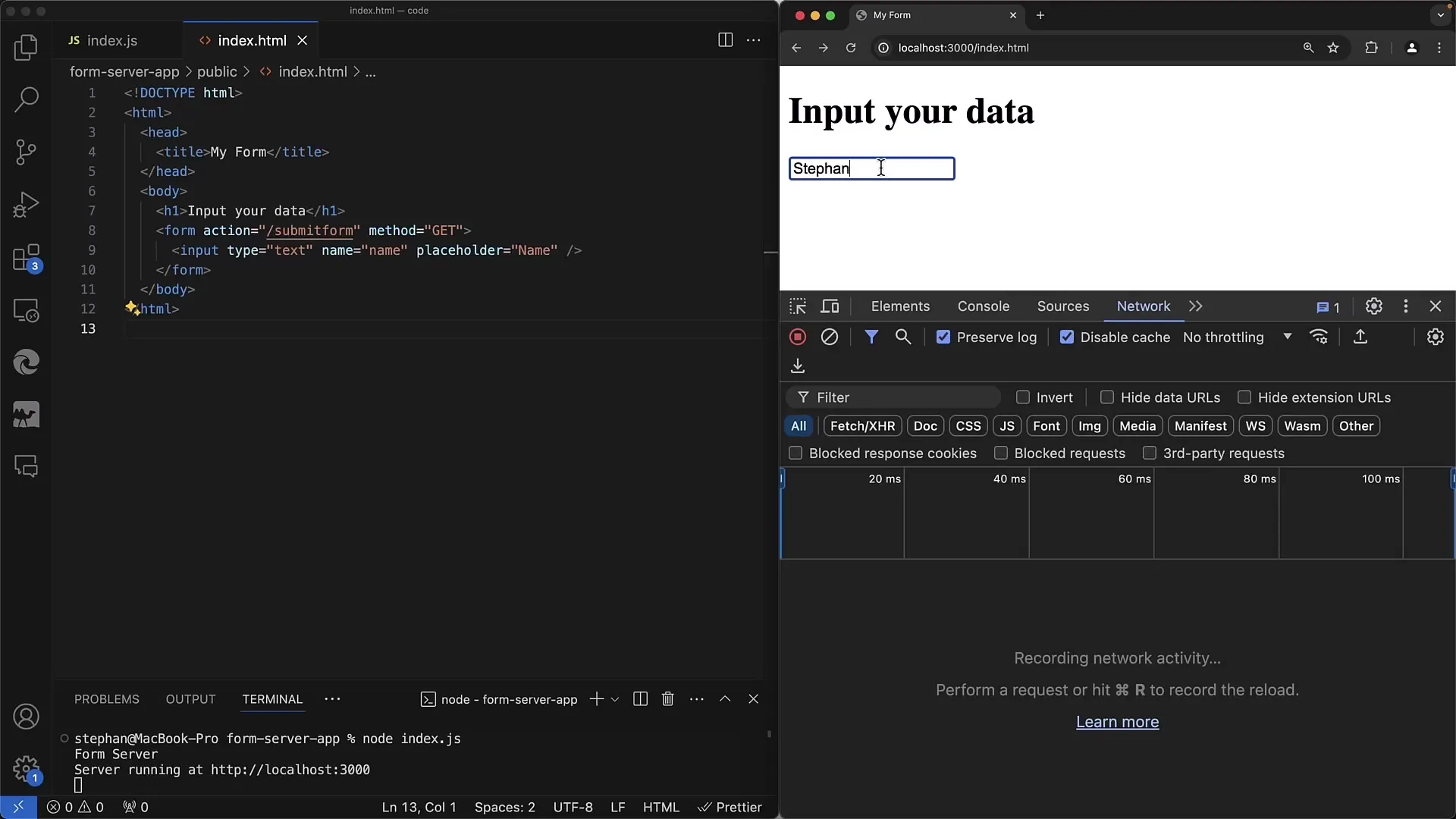
After submitting the form, a response from the server should confirm that the data was successfully sent.
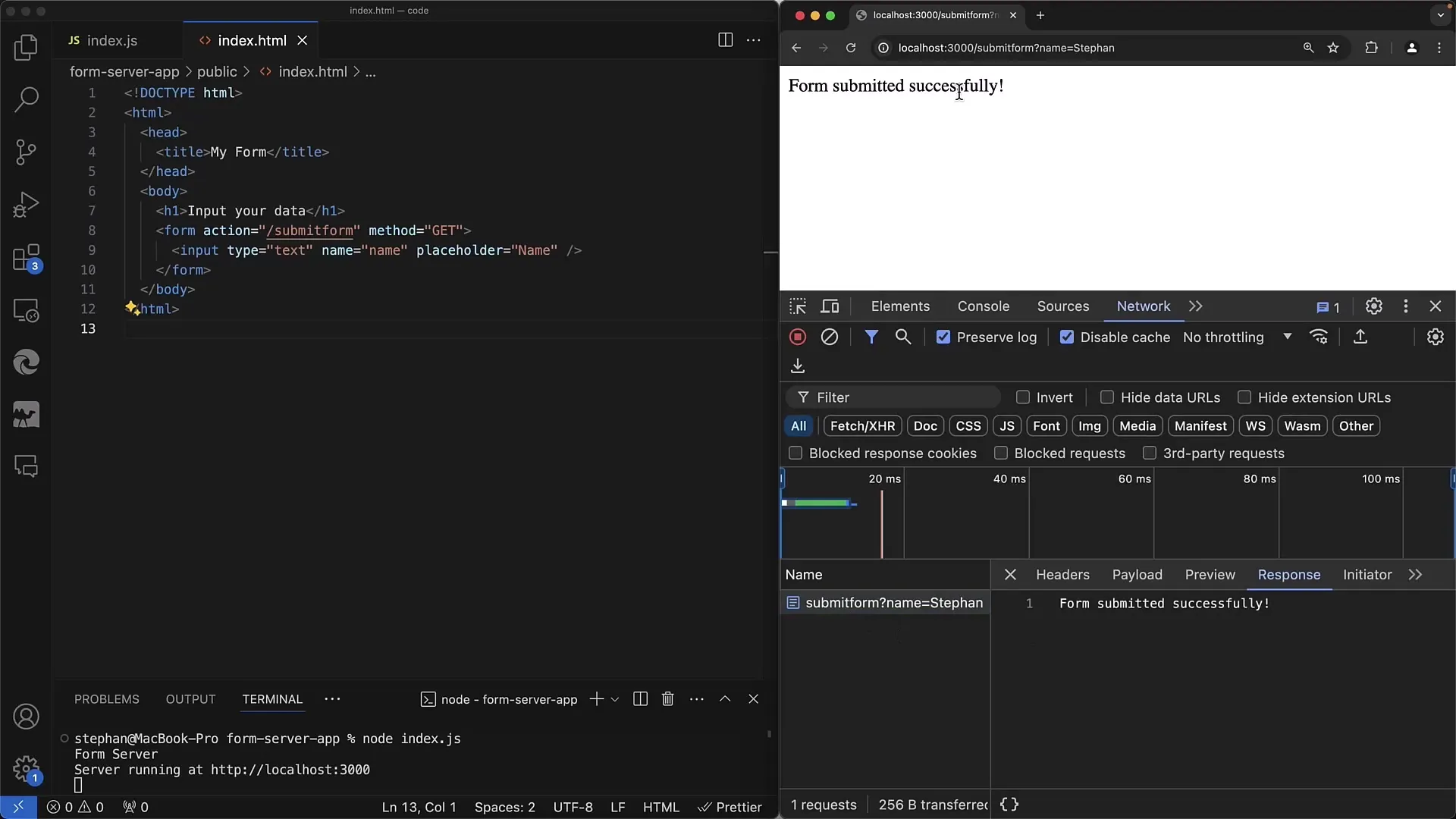
Here you can see the payload that was sent to the server. In our case, the parameter Name was appended to the URL.
In the server code, we will now look at the corresponding GET handler that we need to process the request. The code will be in your index.js file. You define the handler as follows:
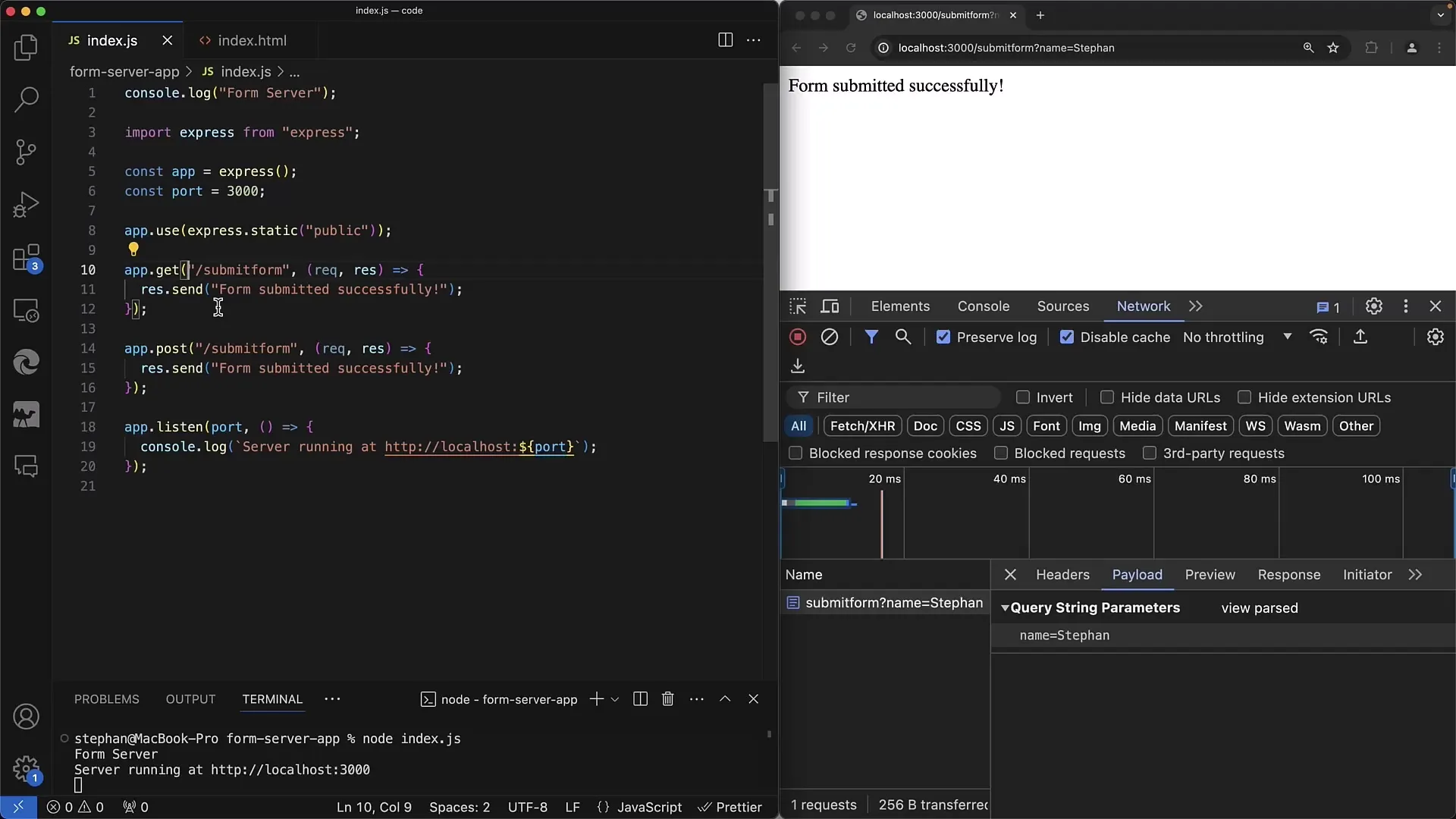
You can now retrieve the query parameters in the server code by accessing request.query. You can also implement this in your GET handler.
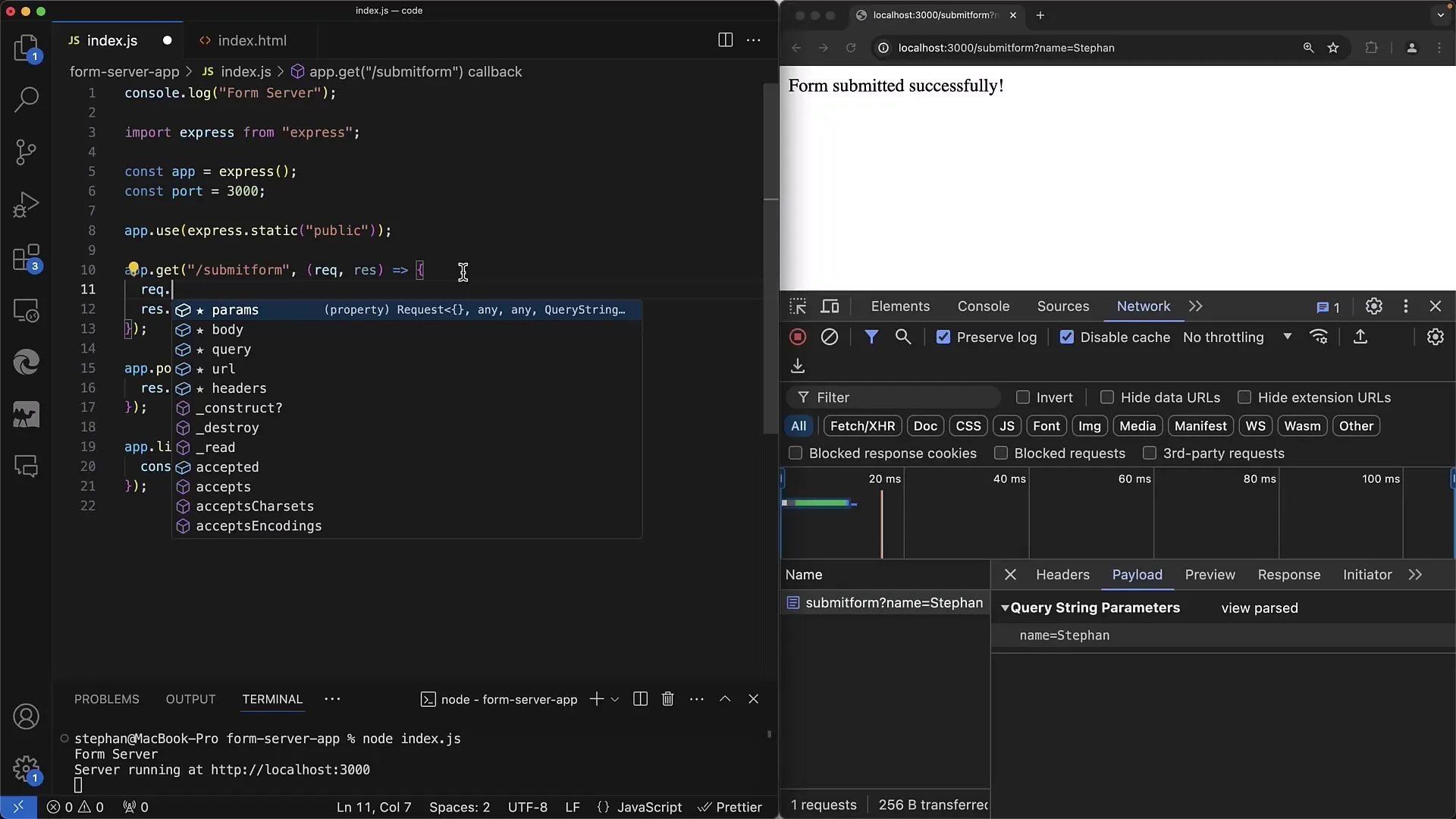
When you resubmit the form, you will see that the parameter is correctly returned. Make sure to restart the server each time you make a change to the server.
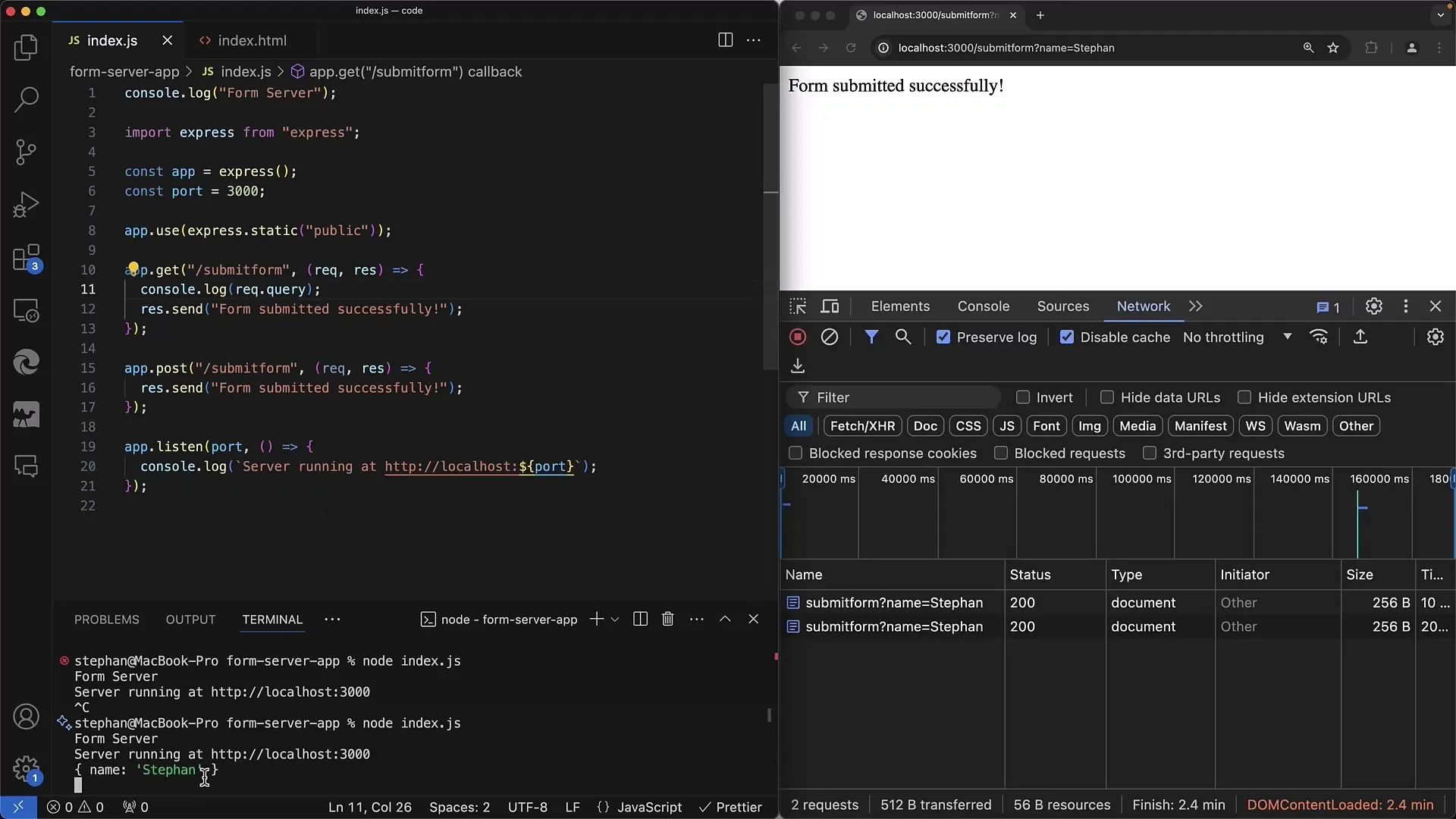
To access the Name, use the format request.query.Name. If you change the name in the form, make sure to adjust the parameter in the server code as well.
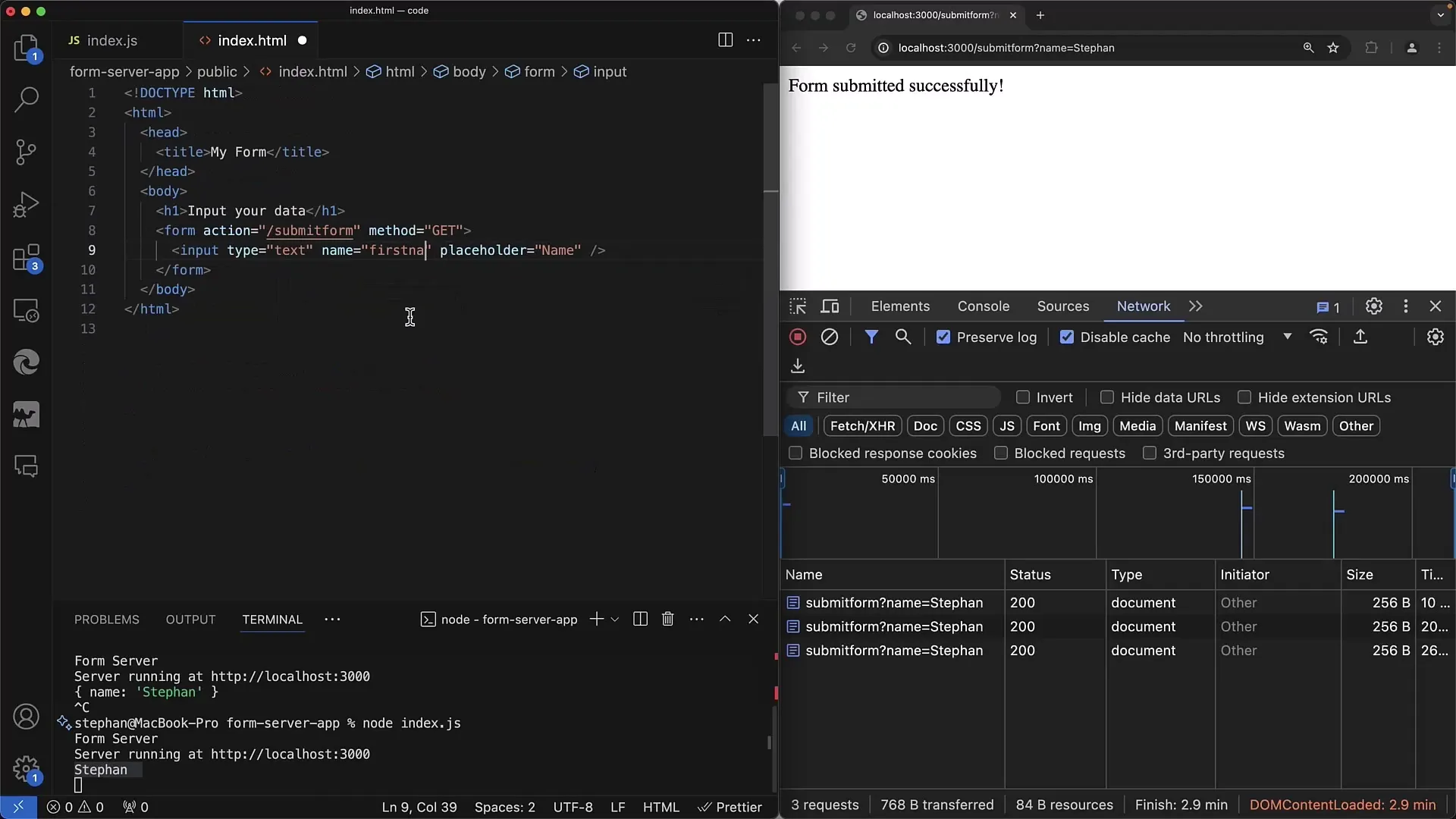
If you change the name in the form to first_name, the requested parameter will look like this:
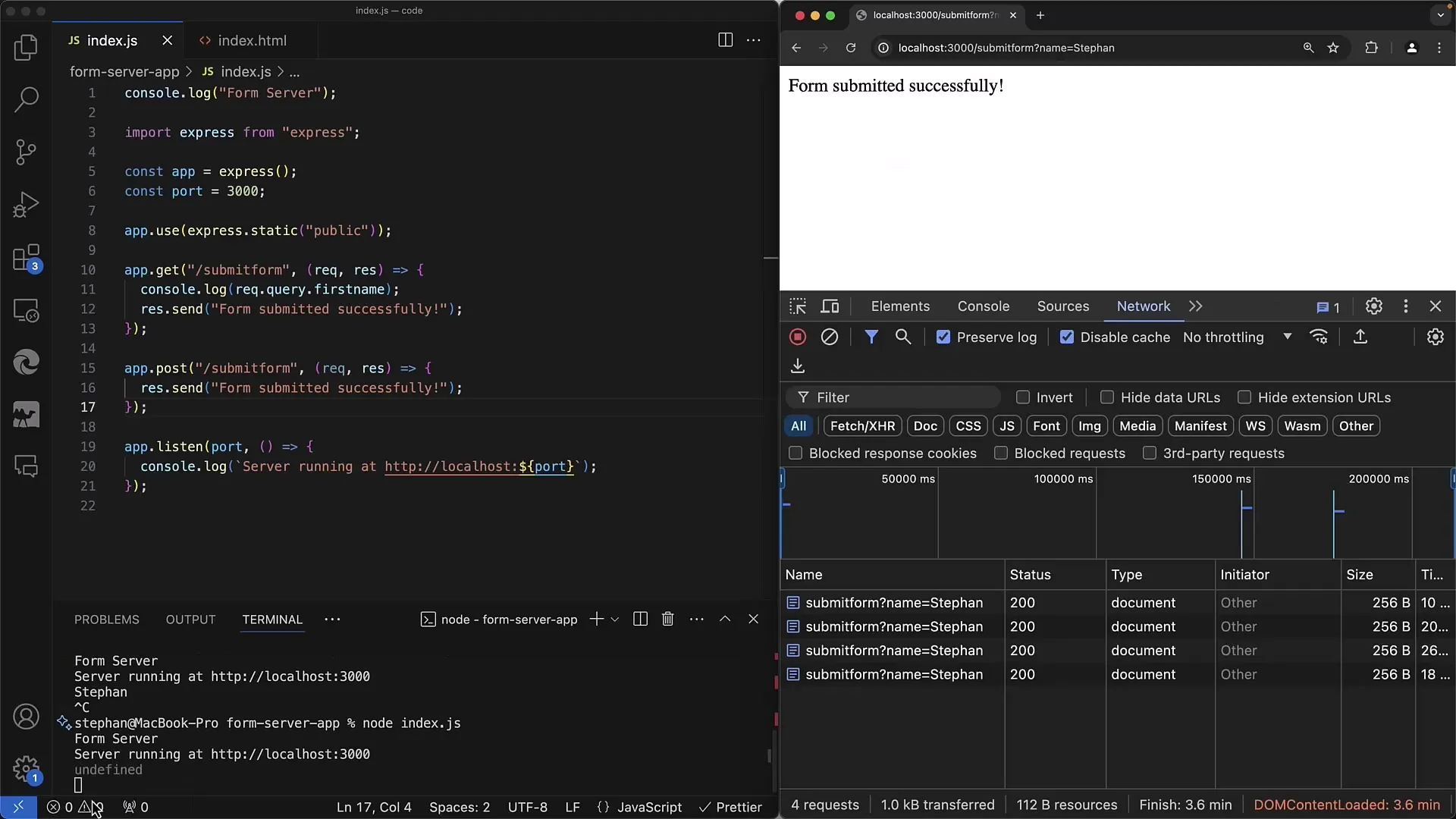
You can see that the server correctly receives and outputs the data:
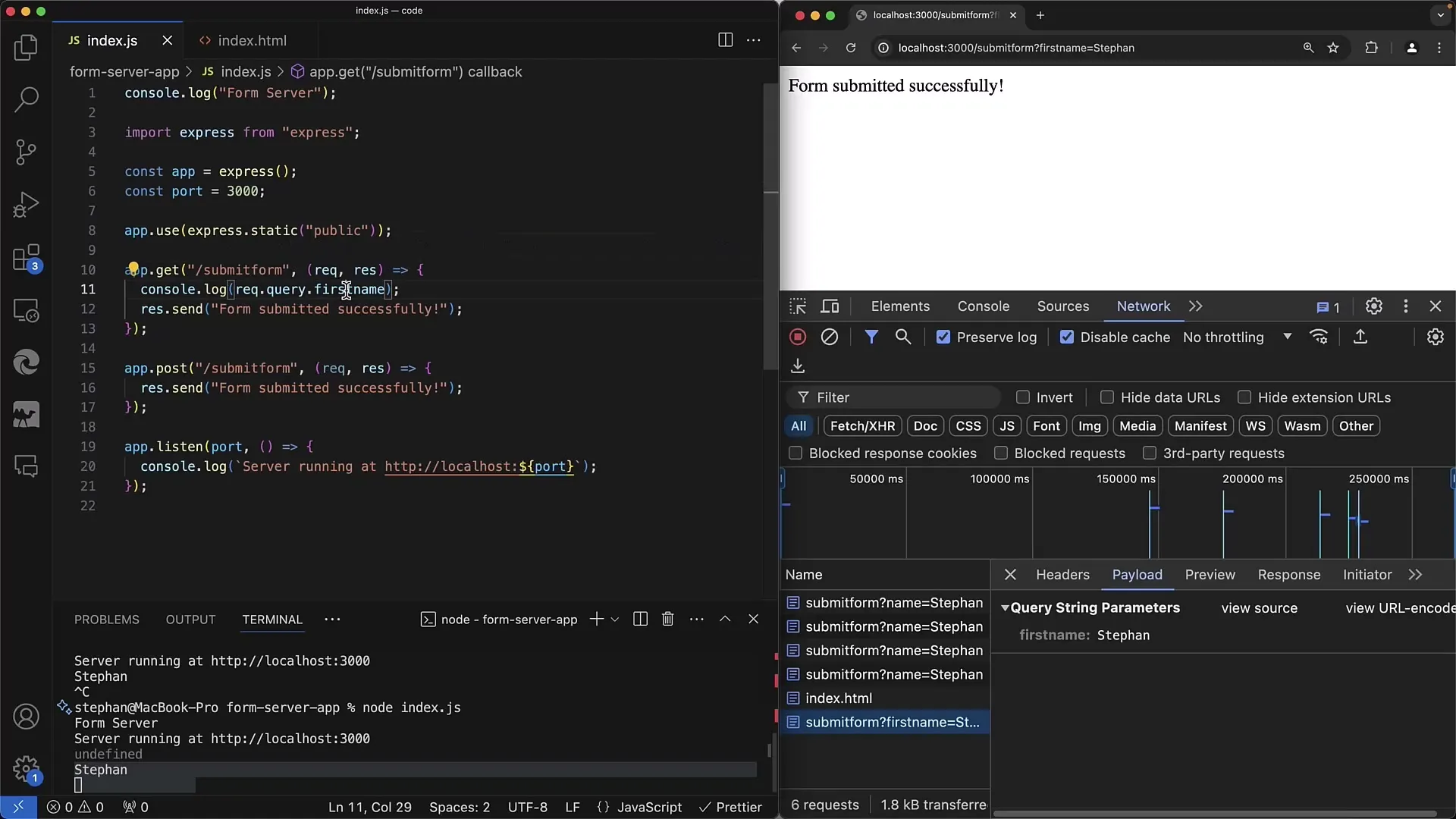
With this foundational knowledge, you can now process the received data, for example, save it in a database, use it elsewhere, or simply return it.
If you wish, you can even send the received data back to the client and act as an echo server.
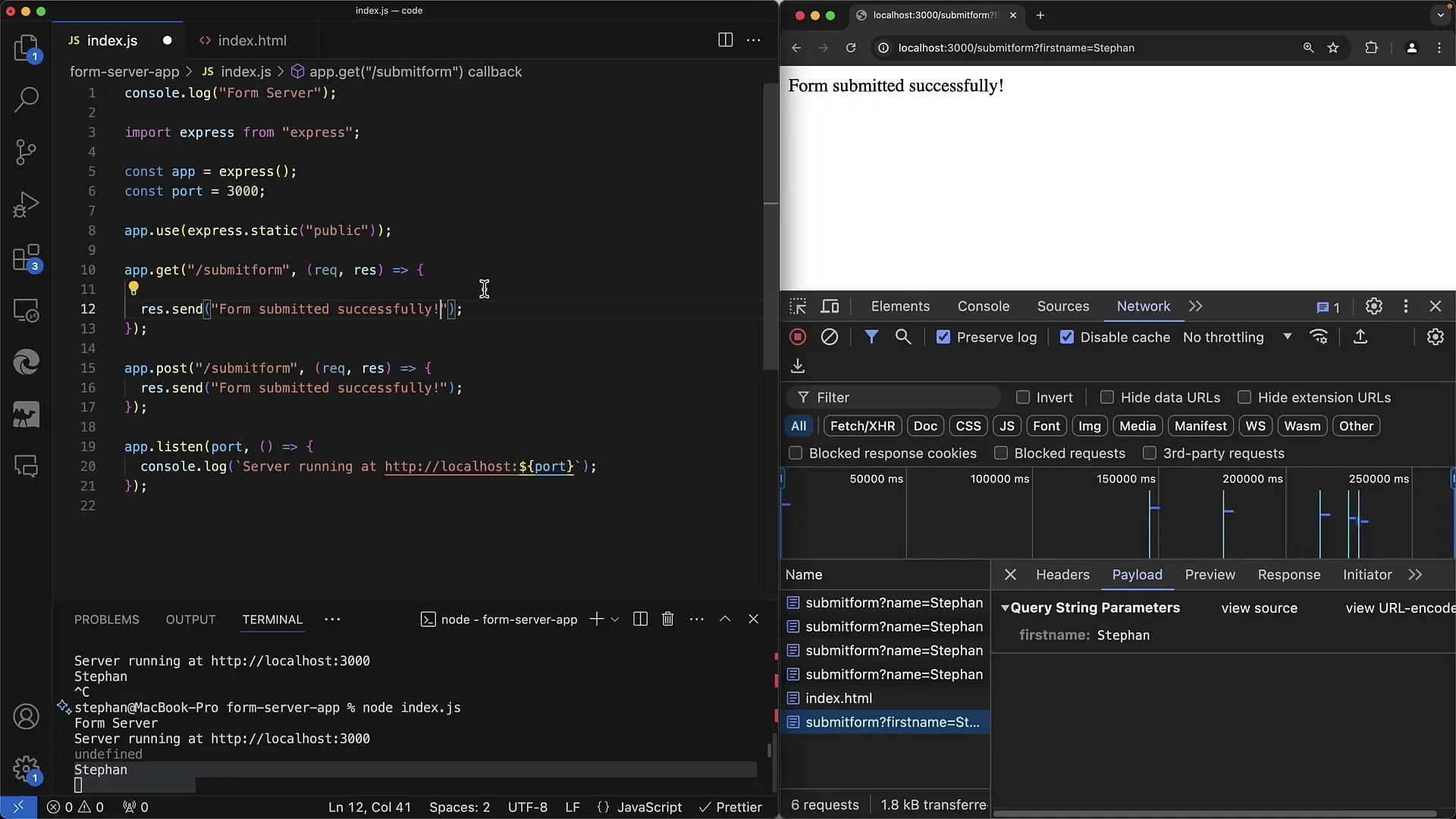
The output could then look as follows:
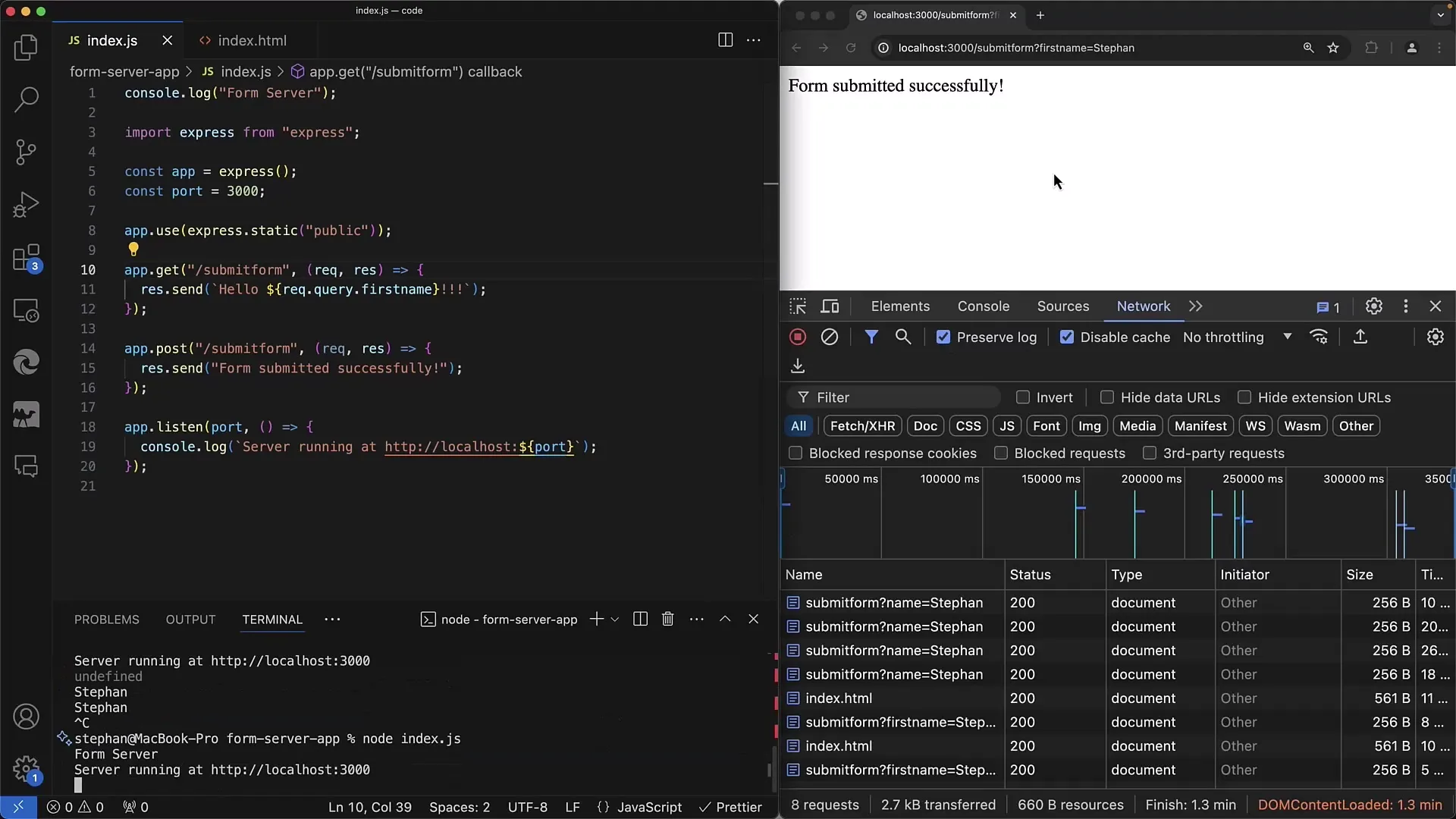
If you enter special characters in the text field, the server will also process and decode them accordingly. You will notice that the output is correct, regardless of the characters entered by the user.
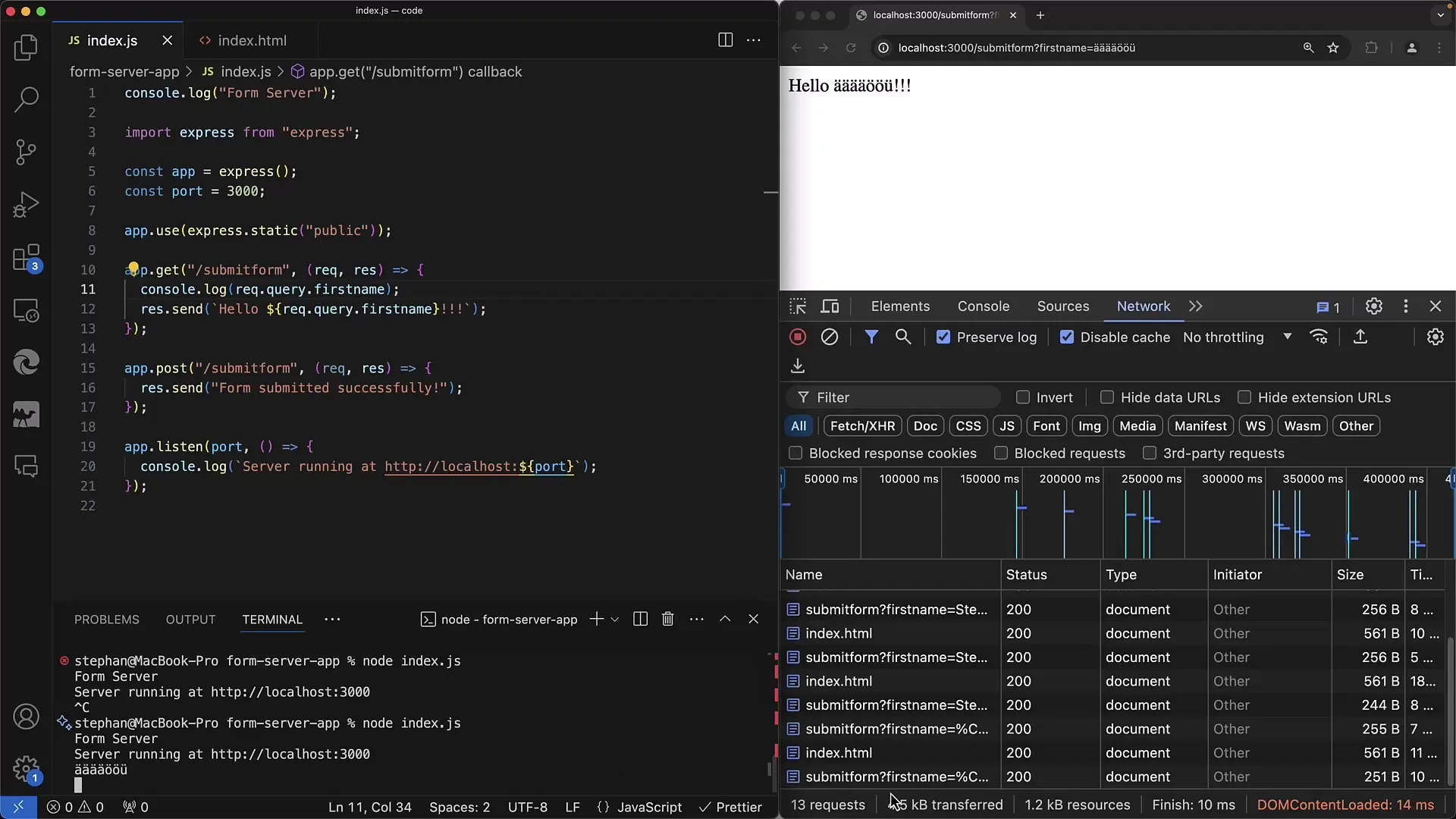
It is important to note that different server frameworks handle encodings differently. When using Express, decoding the query parameters is usually already included, so you don't have to worry about that.
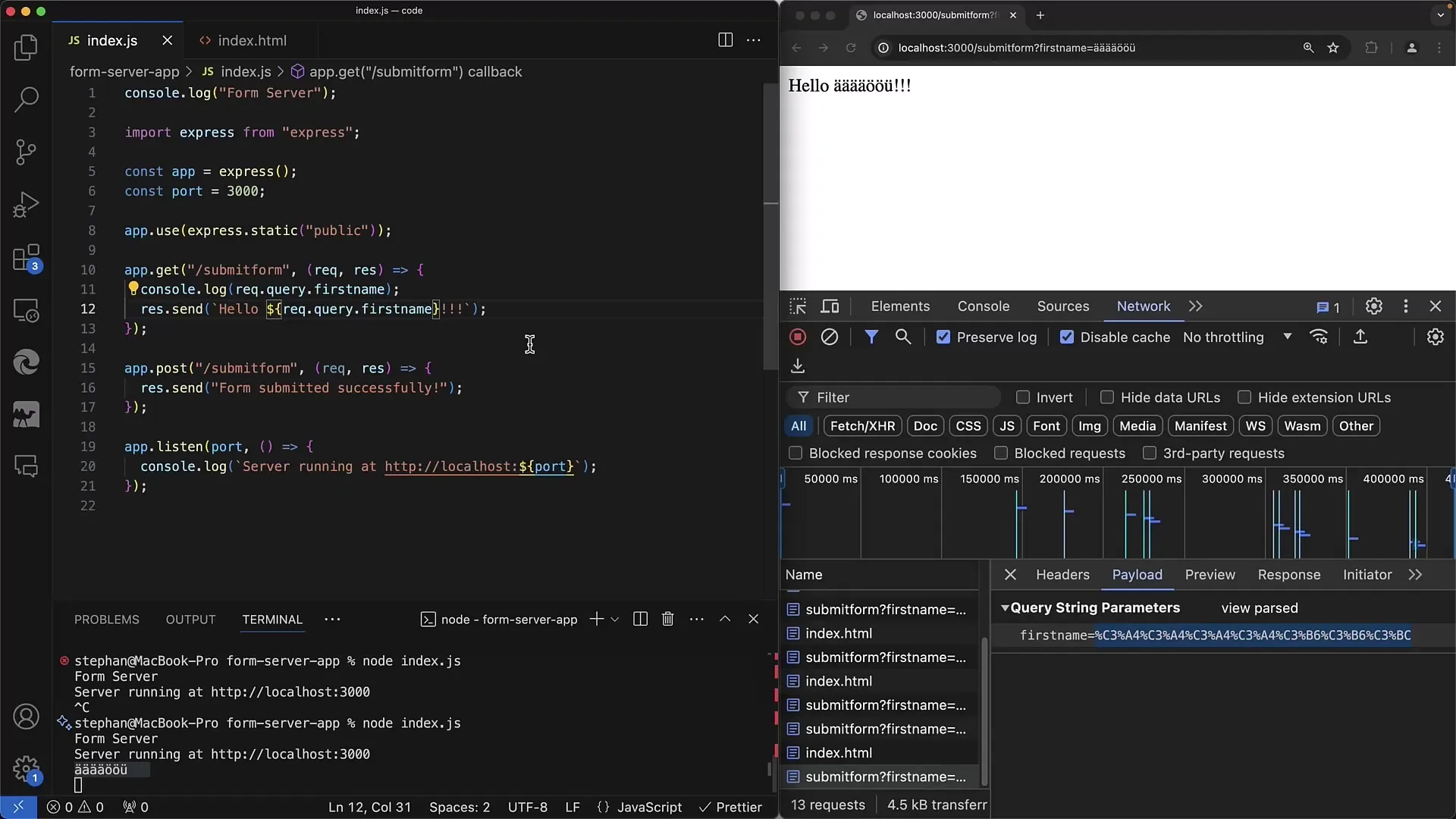
Now you have the basics for processing GET requests, and we have also discussed the use of query parameters in the Express framework. Next, we will focus on the POST method, which requires a different implementation.
Summary
In this tutorial, you have learned how to create a simple web form application using the GET method. The process involves setting up an HTML form, submitting the form data to an Express server, and retrieving query parameters for further processing. You have also seen how to properly configure the server to receive and respond to the form data.
Frequently Asked Questions
What is the difference between GET and POST?GET sends data via the URL, while POST transmits data in the request body.
How can I use multiple parameters in my form?You can add multiple input fields to your form and configure their names accordingly.
How do I deal with special characters in form data?Express automatically handles the decoding of special characters.
Do I need to restart the server every time I make a change?Yes, any changes to the server code require a server restart to take effect.