In this tutorial, you will learn how to create a React project and implement the integration of forms into your application. We will lay the foundation for working with form elements and go through the necessary steps to set up and handle input fields. This will help you develop a better understanding of how forms work in React and what best practices you should follow.
Key Takeaways
- Creating a React project is done through create-react-app.
- You will learn how to implement form elements in React and how to respond to user interactions.
- The structure of a React application is crucial for managing components and inputs.
Step-by-Step Guide
First, you should ensure that Node.js is installed on your computer. This is the foundation you need to create a React project. Open a terminal in the directory where you want to create your project.
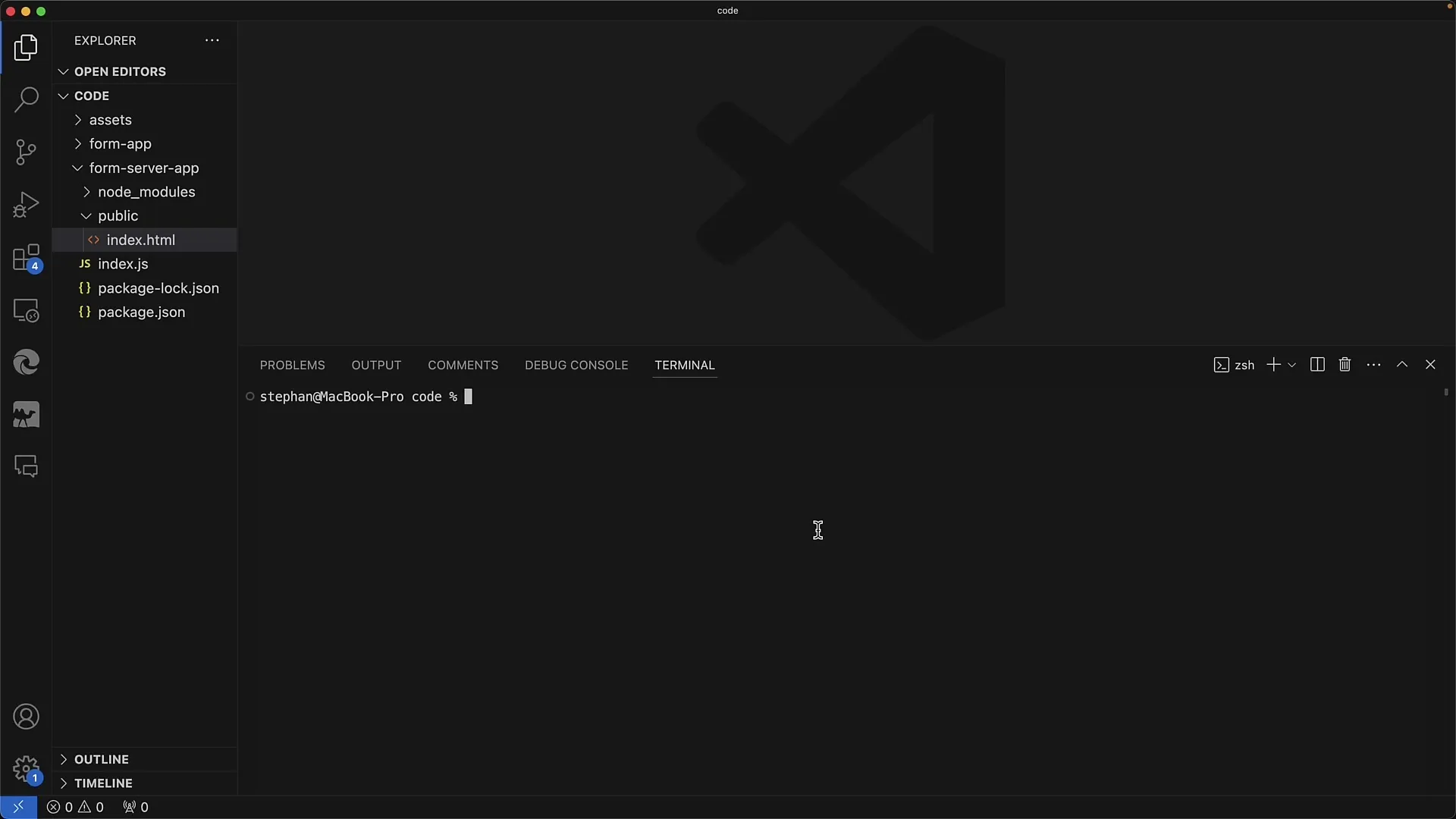
Now you can use the command npx create-react-app my-app to create a new React project. For our example, we will name the project "react-form." It will automatically create a subdirectory.
During the setup, you will be prompted to choose the desired framework. In this case, you should select React. You can also use alternative frameworks like Preact, but for this tutorial, we will focus on React.
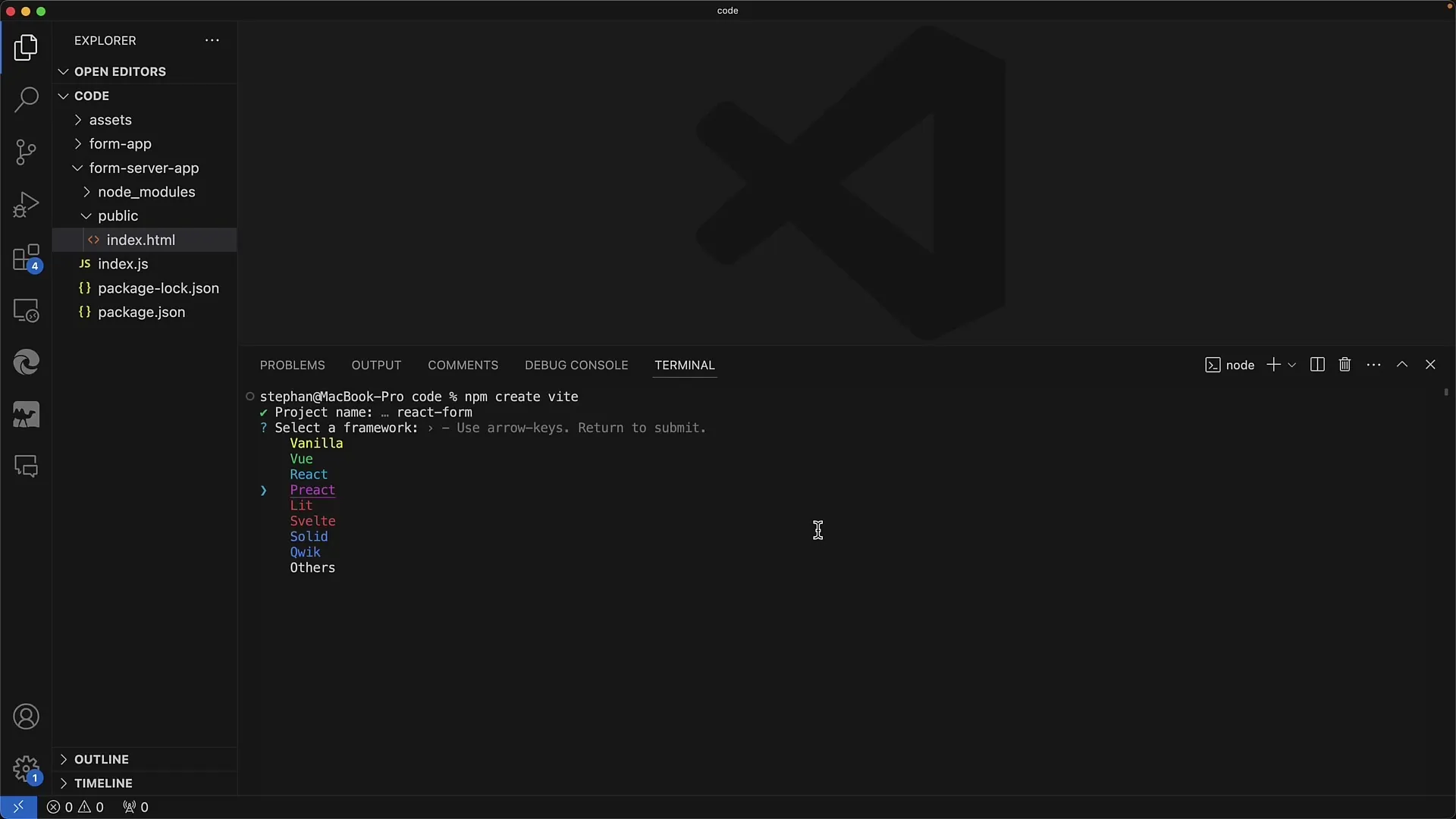
Additionally, you can decide whether you want to use TypeScript or not. For this tutorial, we will use traditional JavaScript.
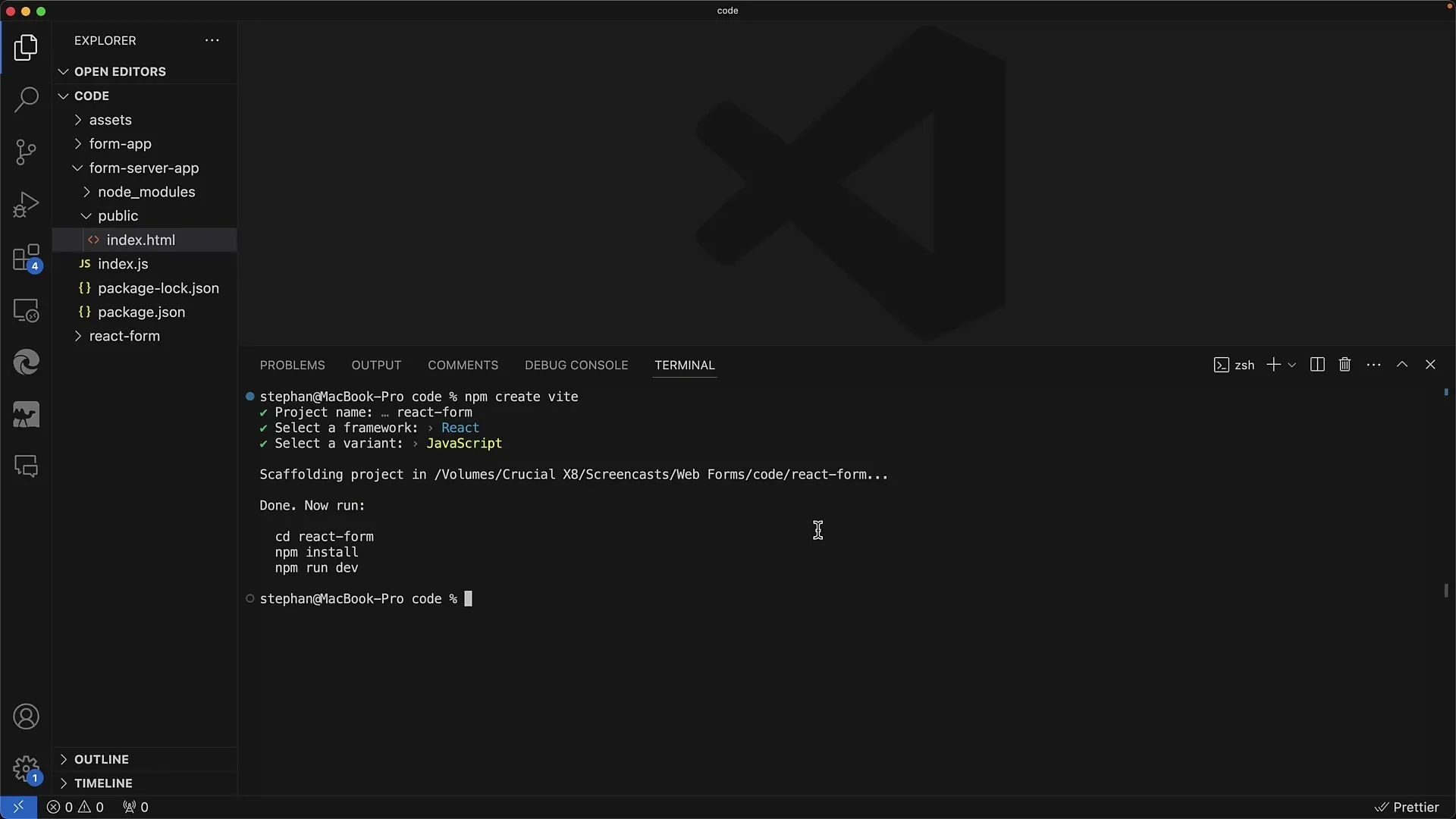
Once the setup is complete, you can navigate to the project directory and install the dependencies using npm install. This ensures that all necessary packages are available.
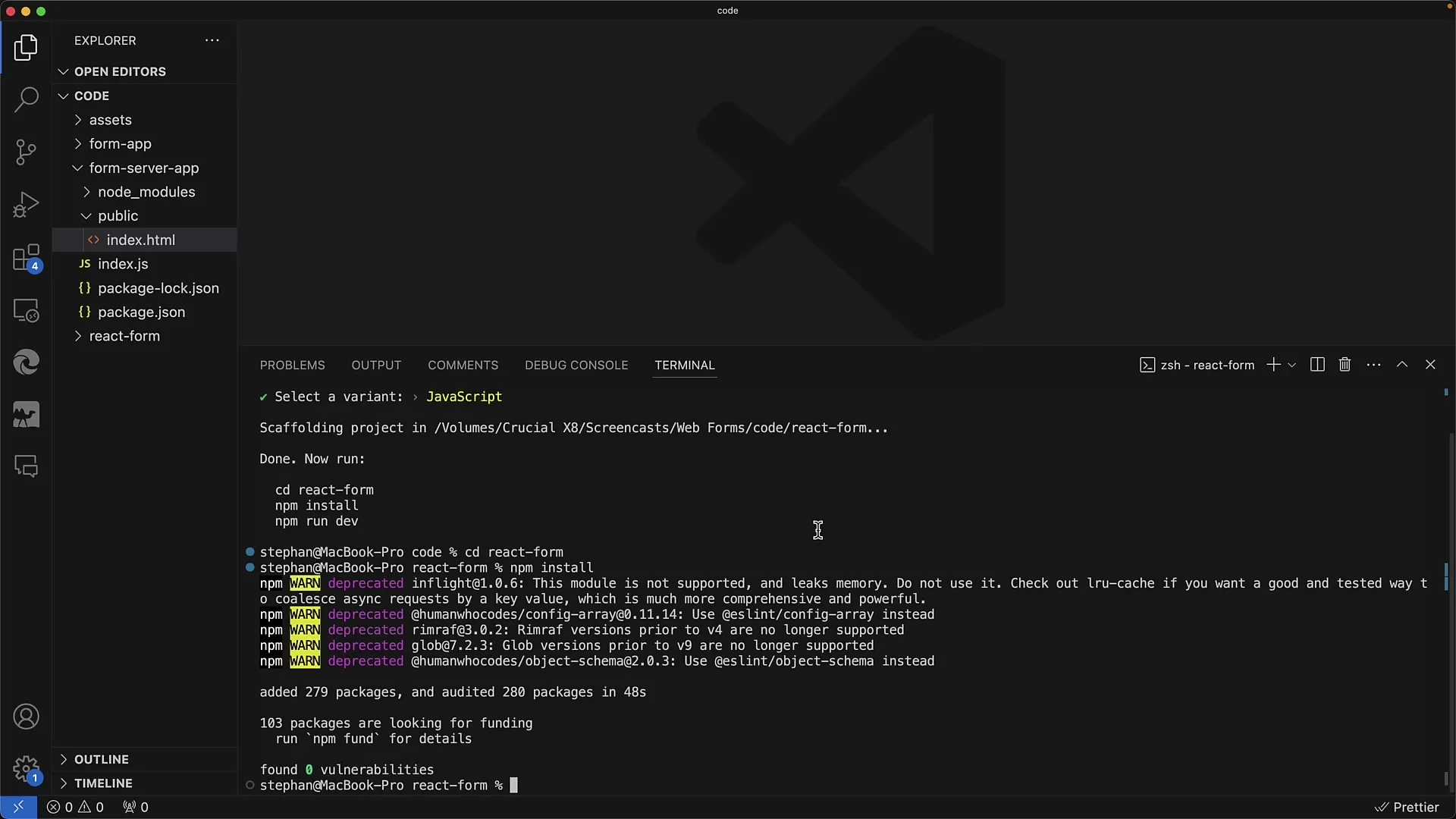
Now you can start the development server by entering npm start. This will open the application in your default browser, typically at http://localhost:3000.
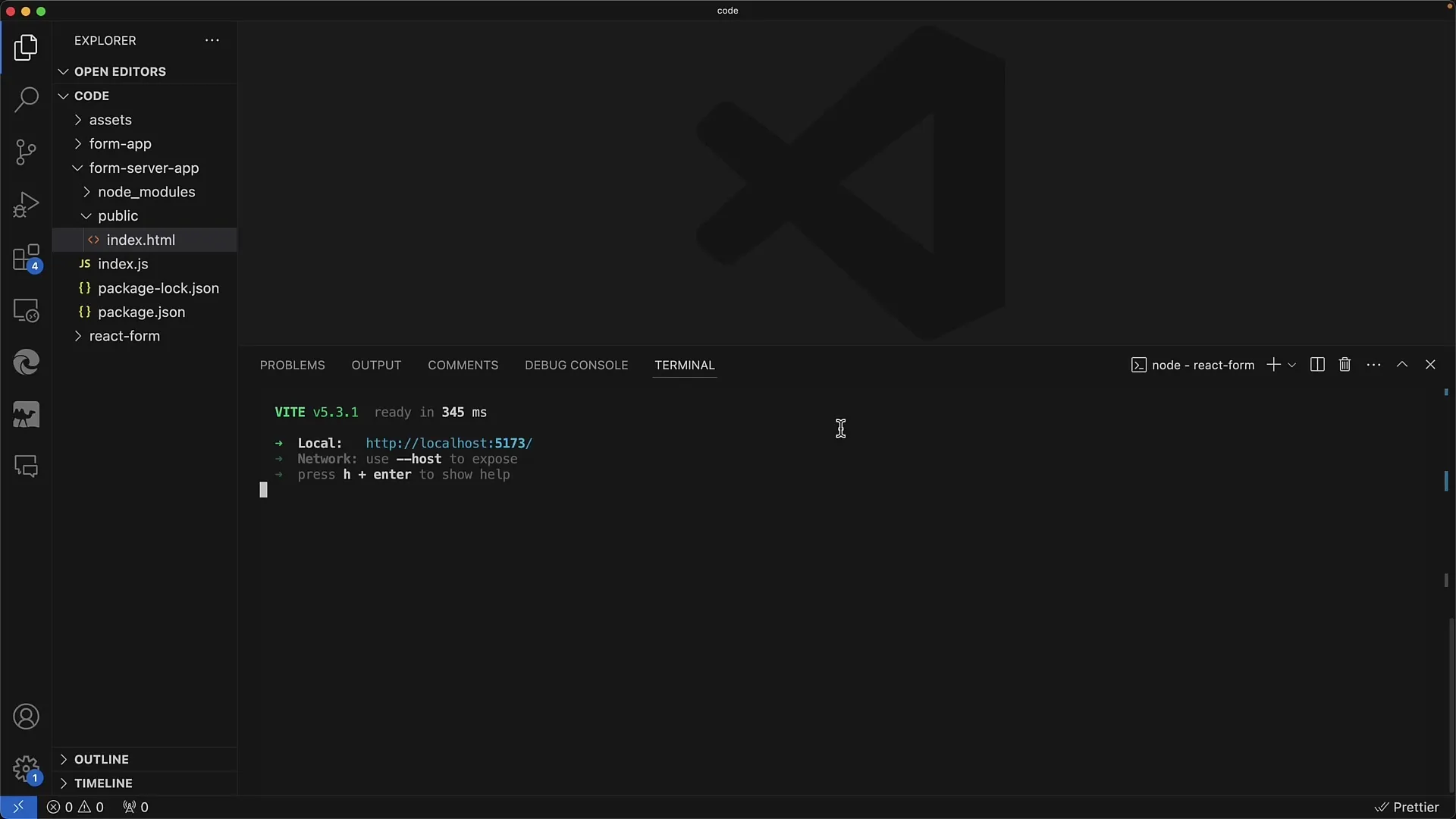
When you open the application, you will see the default view of React. At this point, there are no form elements yet, but that's not a problem. You can now start implementing the basics of forms in React.
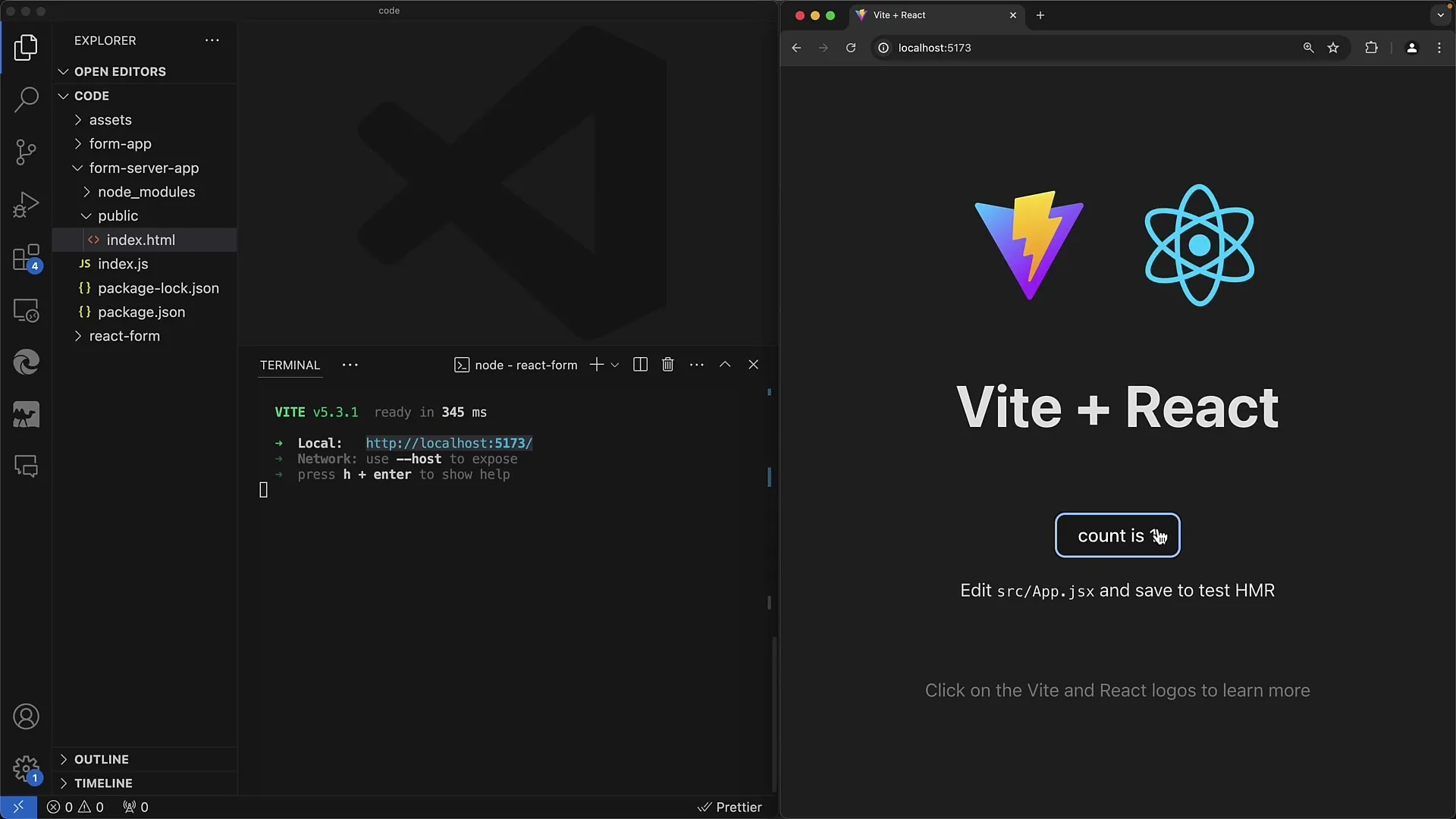
In the src folder, there is a file named App.js, which represents the main component of our application. You can open this file and see that it contains some basic structural elements.
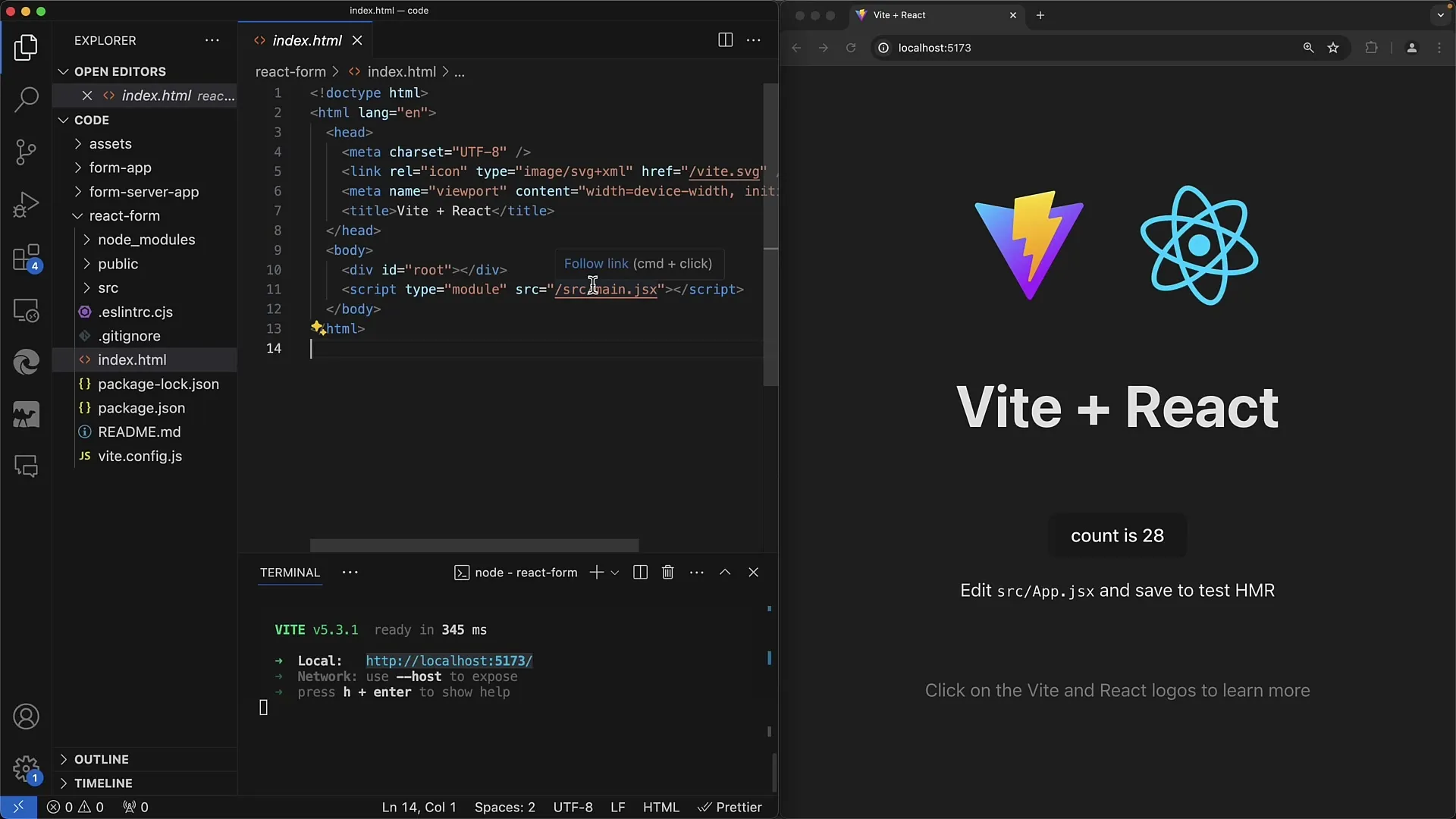
The next step is to clean up the existing code in App.js and make room for our form elements. You can remove anything that is not needed to get a clear view of the code you want to work with.
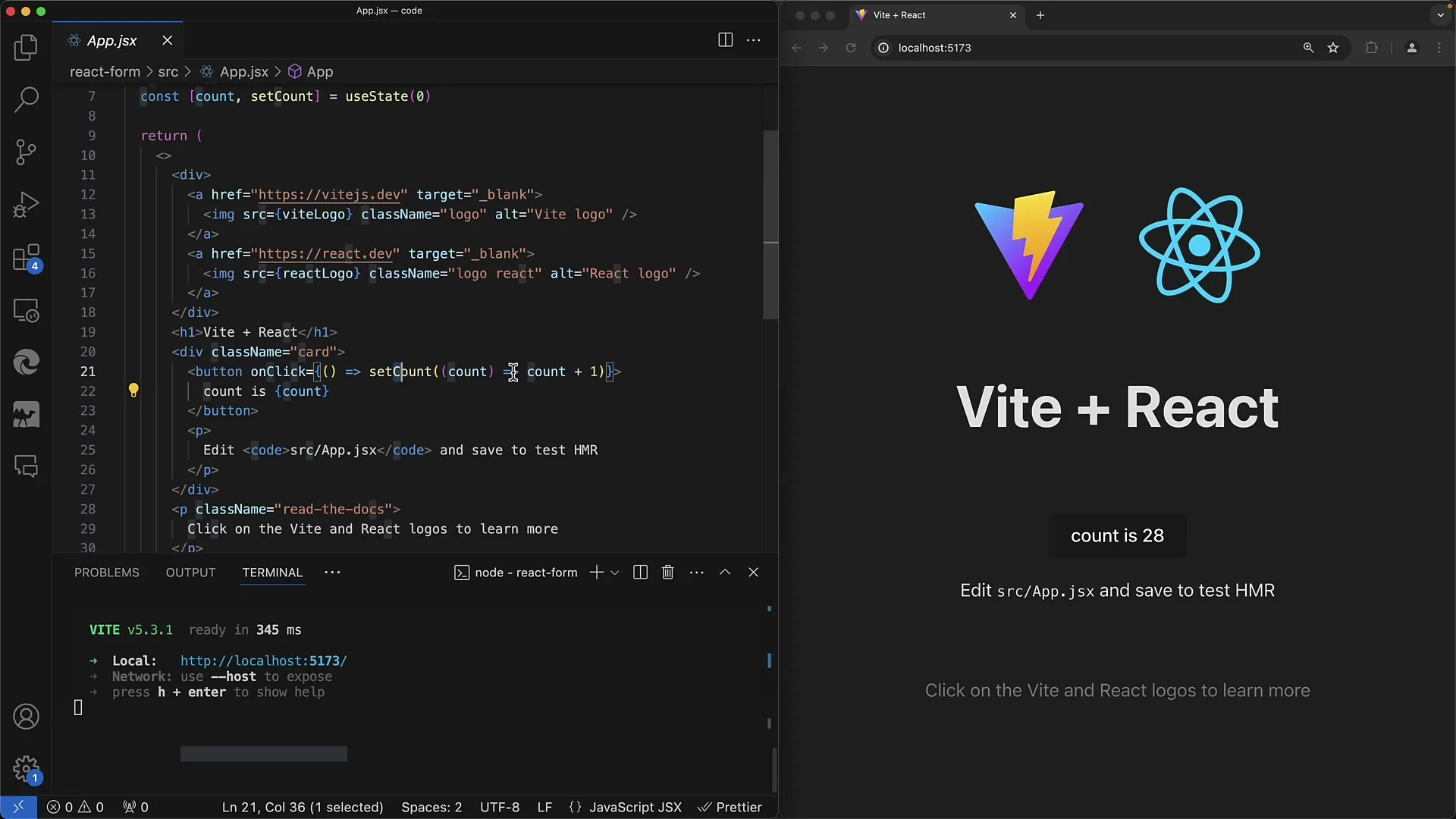
In the next step, you will insert an element into the App. This will help you learn how to react to inputs in React. Start by adding a simple input field.
After adding the input element, you can write functions to respond to events like change or input. To do this, you need to define event handlers that allow you to handle user inputs.
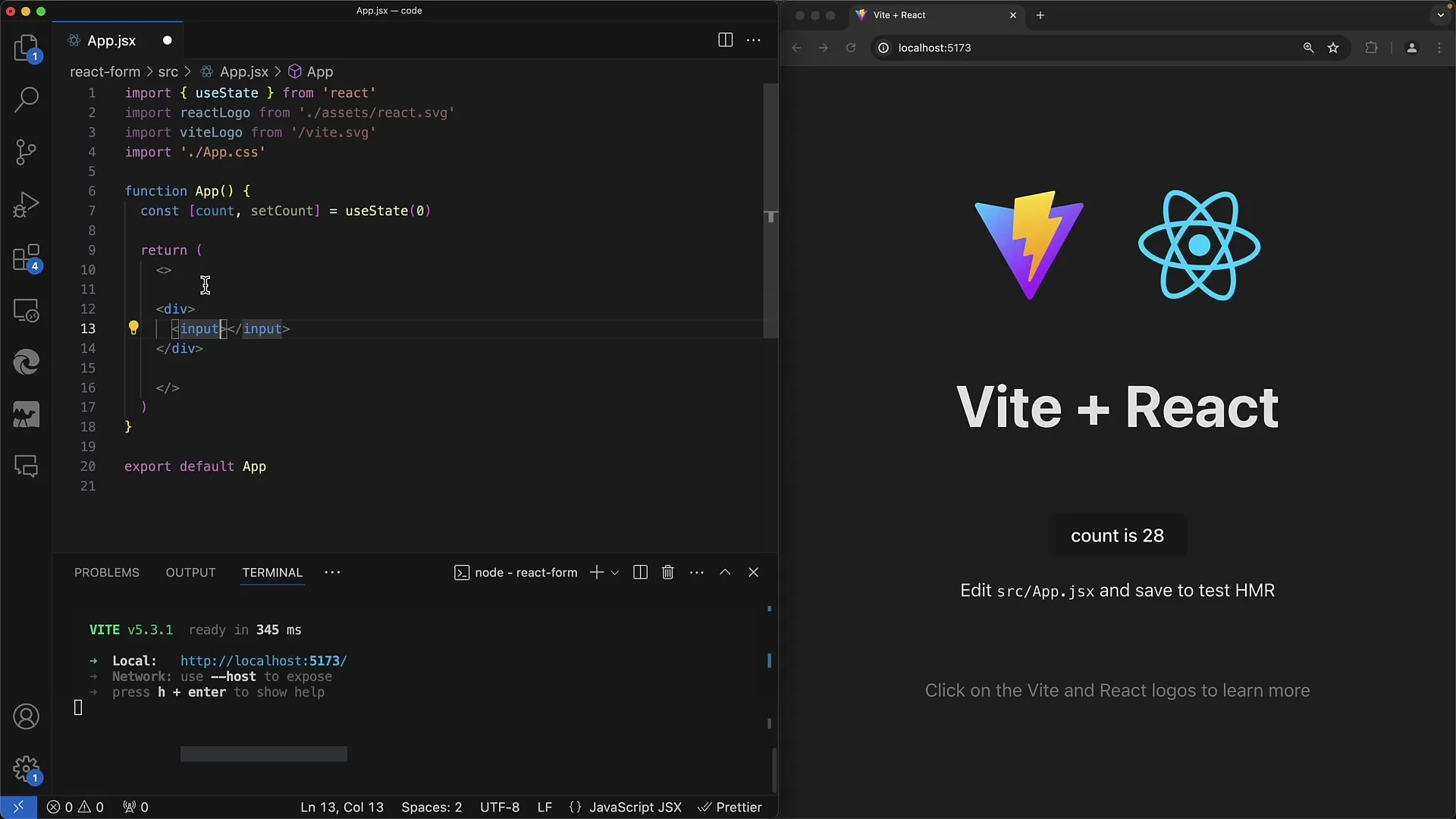
These are the basic steps to create a form in a React application. In the next video, we will delve deeper into the various event handlers and options available to create interactive forms in React.
Summary
In this tutorial, you have learned how to set up a React project and integrate form elements. From the installation to creating the basic components to processing the first input, you have gone through the essential steps to implement a functional form in your application.
Frequently Asked Questions
What do I need to start with React?You need Node.js and npm (Node Package Manager).
How do I create a new React project?To create a React project, you can use the command npx create-react-app project-name.
Can I use TypeScript for my React project?Yes, you can choose TypeScript during the setup of your React project.
Where can I find the main component of my React application?The main component is located in the file src/App.js.
How can I respond to user interactions?You can define event handlers for input fields to respond to events like change or input.