In this guide, you will learn how to integrate and manage forms in Vue.js. Handling form elements is essential for many web applications. Vue.js offers you flexible and efficient ways to create and control forms. This guide is based on a video tutorial and takes you step by step through the implementation of a simple form with Vue.js, clearly explaining concepts and examples.
Main Insights
- Vue.js enables simple creation and management of forms.
- Vue.js's Composition API provides a structural way to handle state.
- Using v-model simplifies handling bidirectional data binding in forms.
- Event handlers such as @change and @submit are central to interactivity.
Step-by-Step Guide
First, you start with the basic prerequisites. Make sure you have set up a Vue.js application. This is usually done via the command line with npm create vue. Just as explained in the video, you have chosen the right structure for your project.
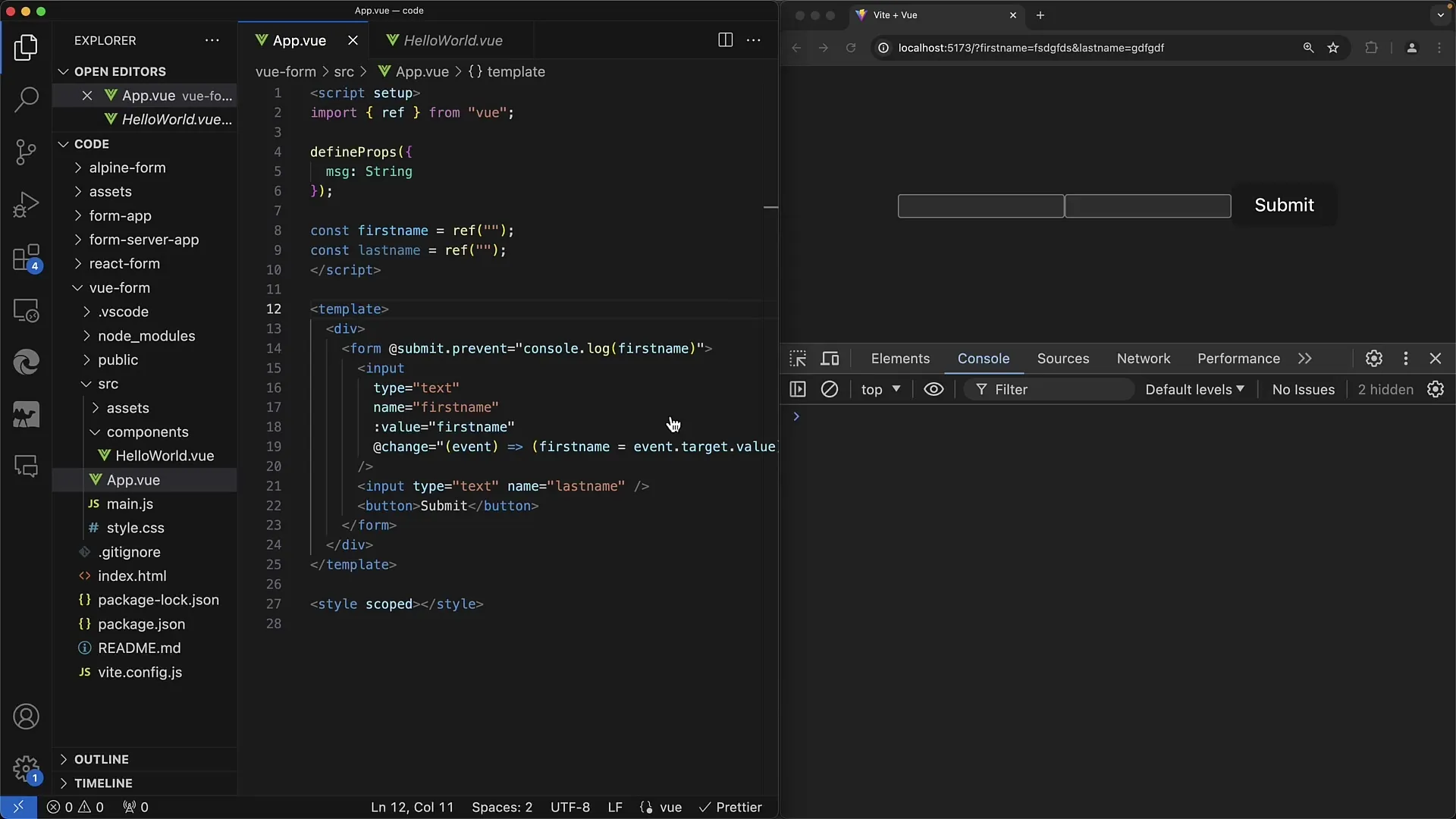
Now that you have the basic structure, you can create a simple component that will contain your form elements. This is where we enter the world of the Composition API, one of the two main methods of developing with Vue.js. Unlike the older Options API, the Composition API allows for a much clearer organization of component state and logic.
In the setup declaration of your component, you use ref to declare state variables. This allows you to directly reference the input value of your form elements. At this point, you can define variables like firstName and lastName, which will later store the input values.
Next, we add a simple text field for the first name. You can bind the value of the input field with the v-model directive, which allows the binding between the user interface and the data. This works very similarly to Alpine.js, as v-model serves the same purpose in most cases.
If you now want to define an event for changing the input value, you can use the @change event. Make sure to call a function every time the value changes to update the state. In this case, the event object is used to get the current value of the input field.
The next element we look at is the form event @submit. To prevent the form from reloading the page, add event.preventDefault() to your submit handler. This is a common practice to control form data processing instead of refreshing the entire page.
When the user submits the form, you can simply output the current state of the firstName value with console.log(firstName). This allows you to check if the input was captured correctly.
We now implement a preview of the entered name directly below the input field. You can use the double curly brace syntax of Vue.js to display the value of firstName as part of the template.
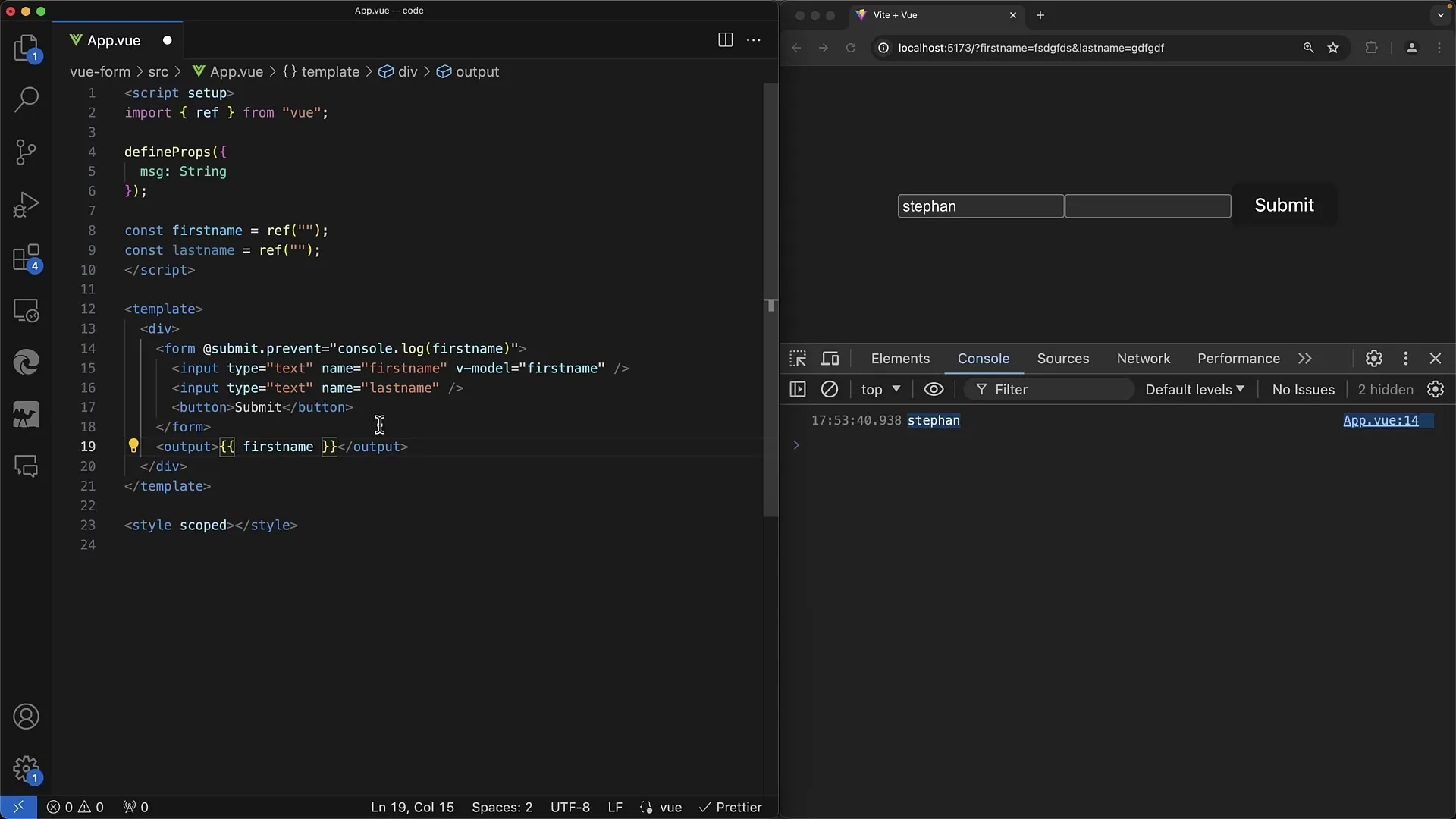
To add the last name as well, simply add another input field with v-model="lastName". Afterwards, you can combine both values to display them correctly.
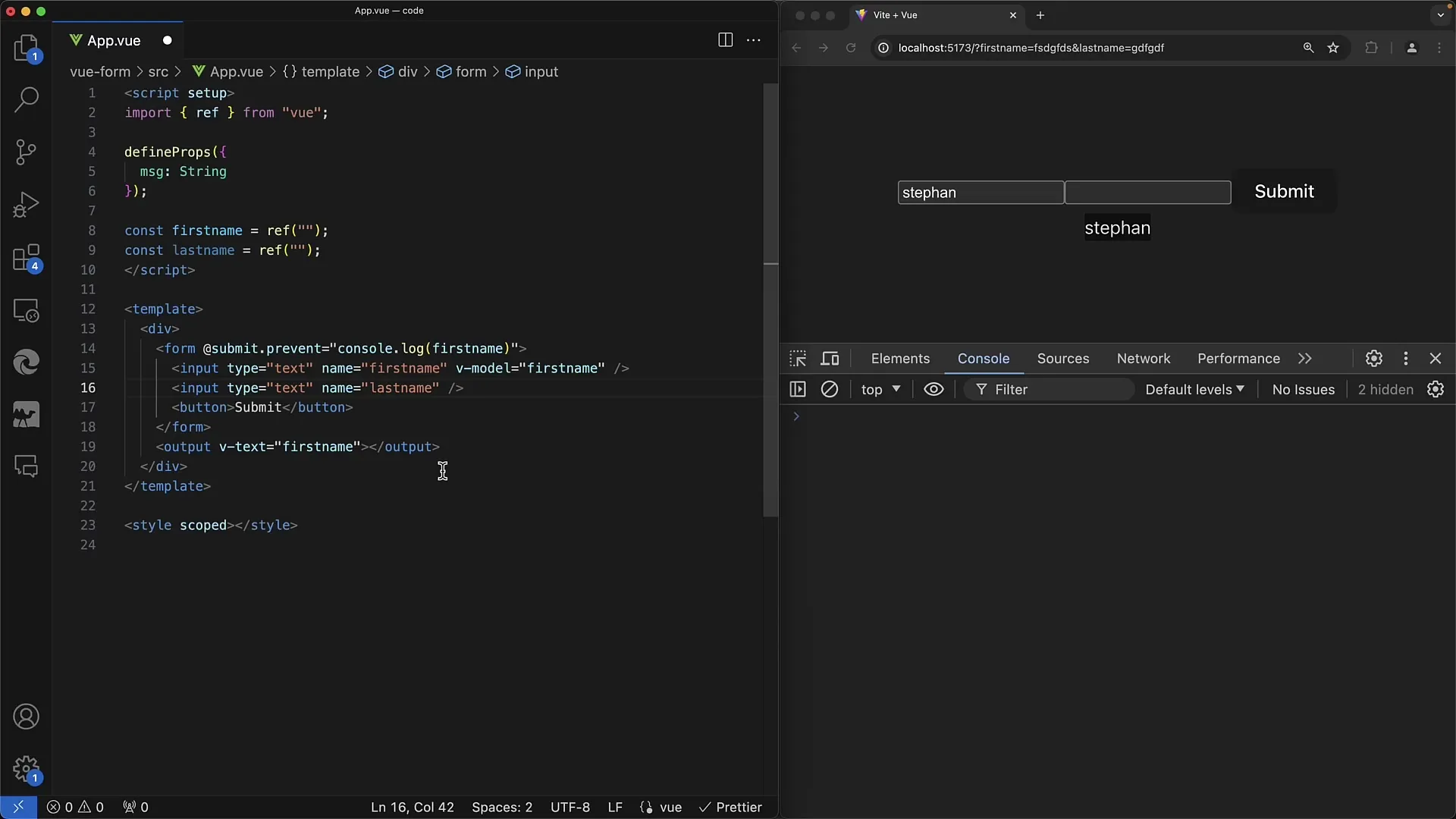
Using v-model greatly simplifies the code as you no longer need additional event handlers to update the values. This makes your component cleaner and reduces potential sources of error.
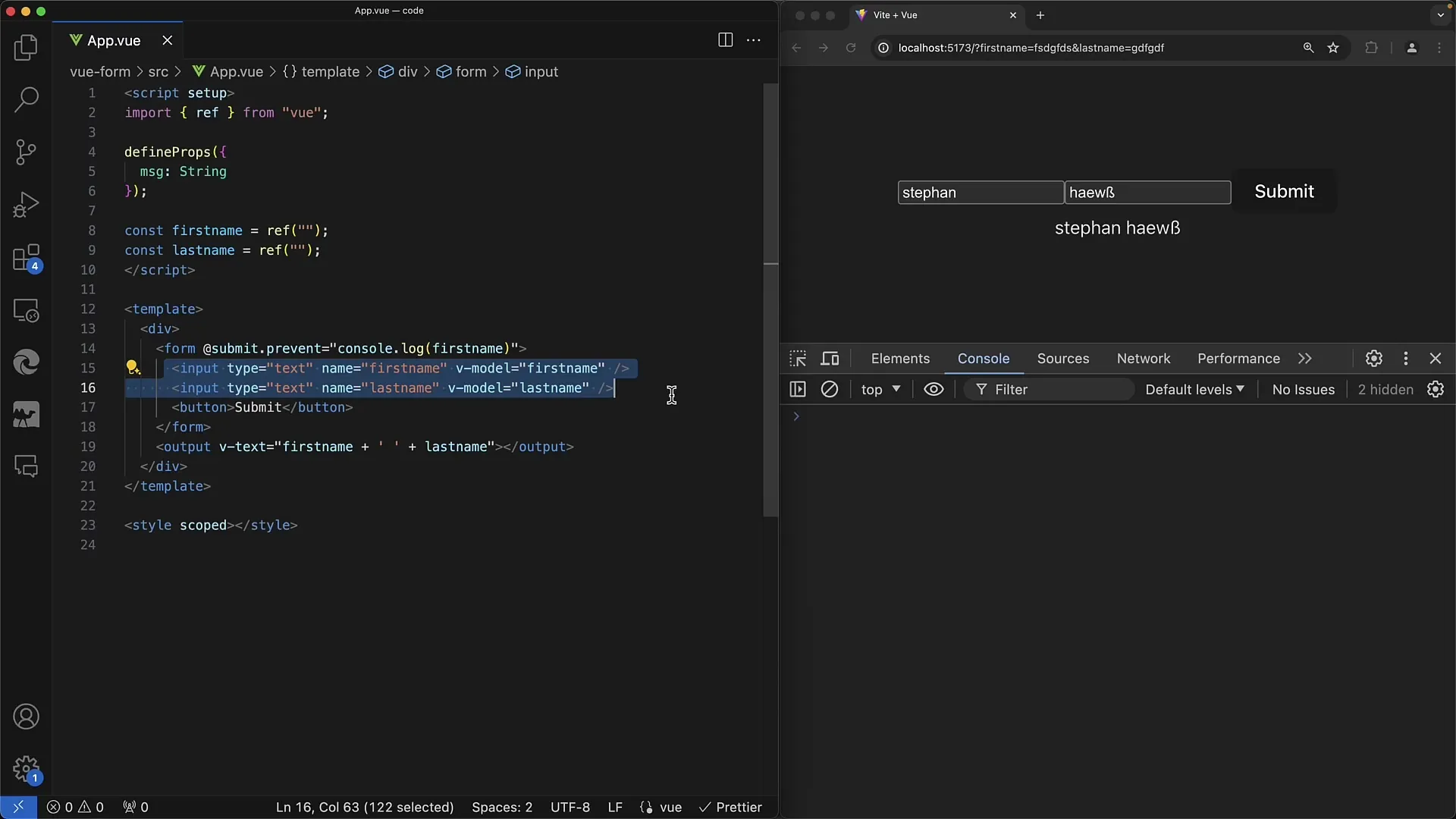
Remember that Vue.js is not only meant for simple text inputs. You can also add more complex form elements like selects, text areas, and checkboxes. Handling them follows the same principles as described above.
As you have seen, the different elements of Vue.js work seamlessly together to provide you with an efficient way to manage form data. For a deeper dive into advanced techniques, I recommend visiting the official Vue.js documentation on form input bindings.
Summary
You have learned how to create and manage forms in a Vue.js application. This includes using v-model for simple data binding, handling events, and integrating the Composition API for clear structure.
Frequently Asked Questions
How does v-model work in Vue.js?With v-model, you bind a form field directly to a variable, enabling bidirectional data binding.
What is the difference between the Options API and the Composition API?The Options API is the older method for creating components, while the Composition API offers a more modular and flexible structure for state management.
How can I prevent the form from reloading the page?Use event.preventDefault() in your submit handler.
How can I work with checkboxes in Vue.js?Use v-model with an array to manage the states of multiple checkboxes.
Can I also use select and textarea fields in Vue.js?Yes, the handling is similar to text fields, often using v-model for data binding.