In this tutorial you will learn how to manage the state of checkboxes in JavaScript. Checkboxes are an important component in HTML forms, as they allow users to select or reject options. Below I will show you step by step how to address checkboxes, query their states, and set them programmatically. Whether you are a beginner or have advanced knowledge in JavaScript, this guide will help you improve your skills.
Key Takeaways
- You can query the state of a checkbox using the checked property.
- The addEventListener pattern allows for easy handling of changes.
- Programmatically set checkboxes do not trigger a change event.
Step-by-Step Guide
First, you need to ensure you have an HTML page with a checkbox. I assume you are creating a simple form with a checkbox to accept the terms and conditions:
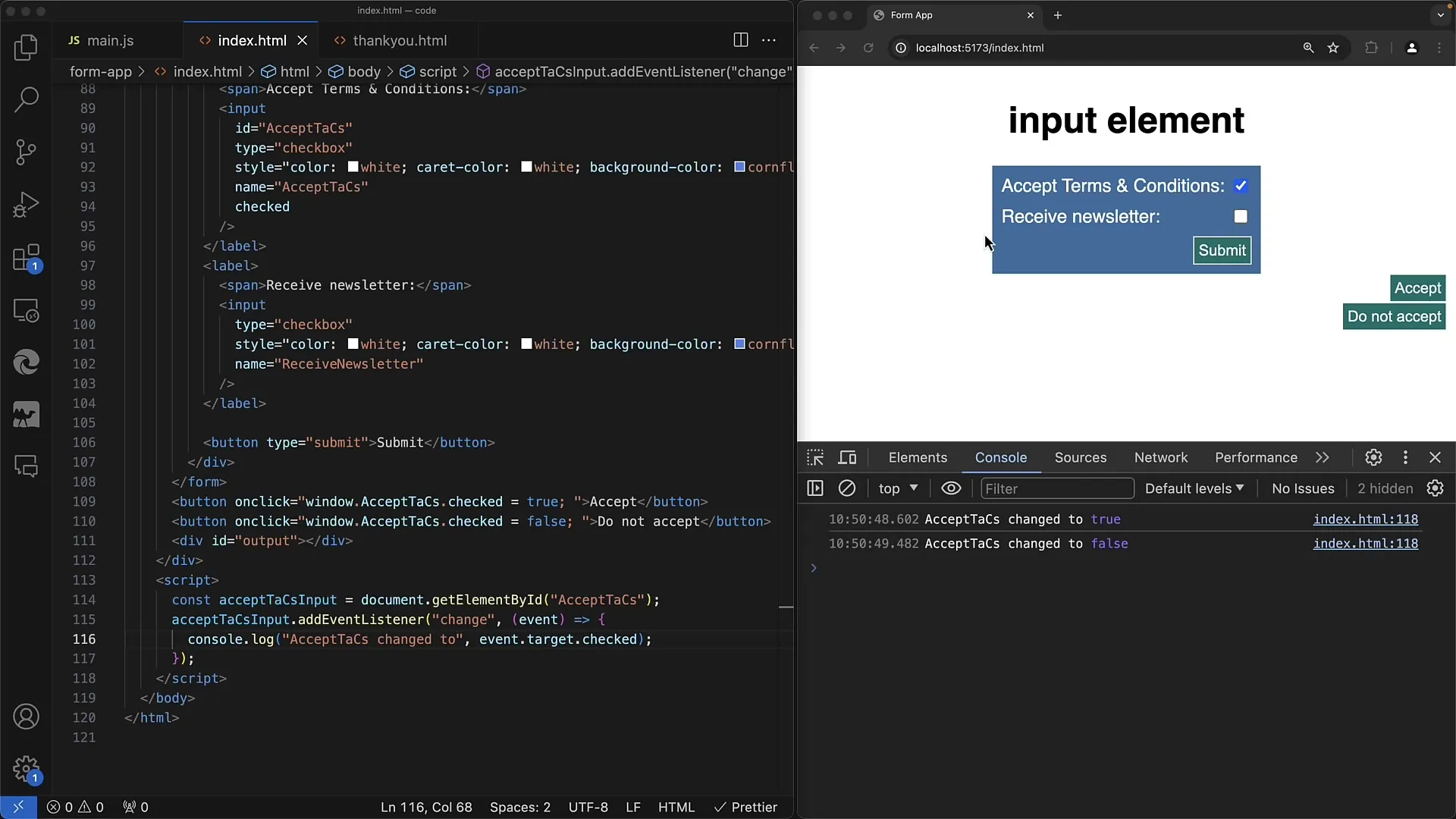
Step 1: Retrieve Checkbox Element with JavaScript
In the first step, the checkbox is retrieved by its ID. In this example, we refer to a checkbox with the ID acceptTerms.
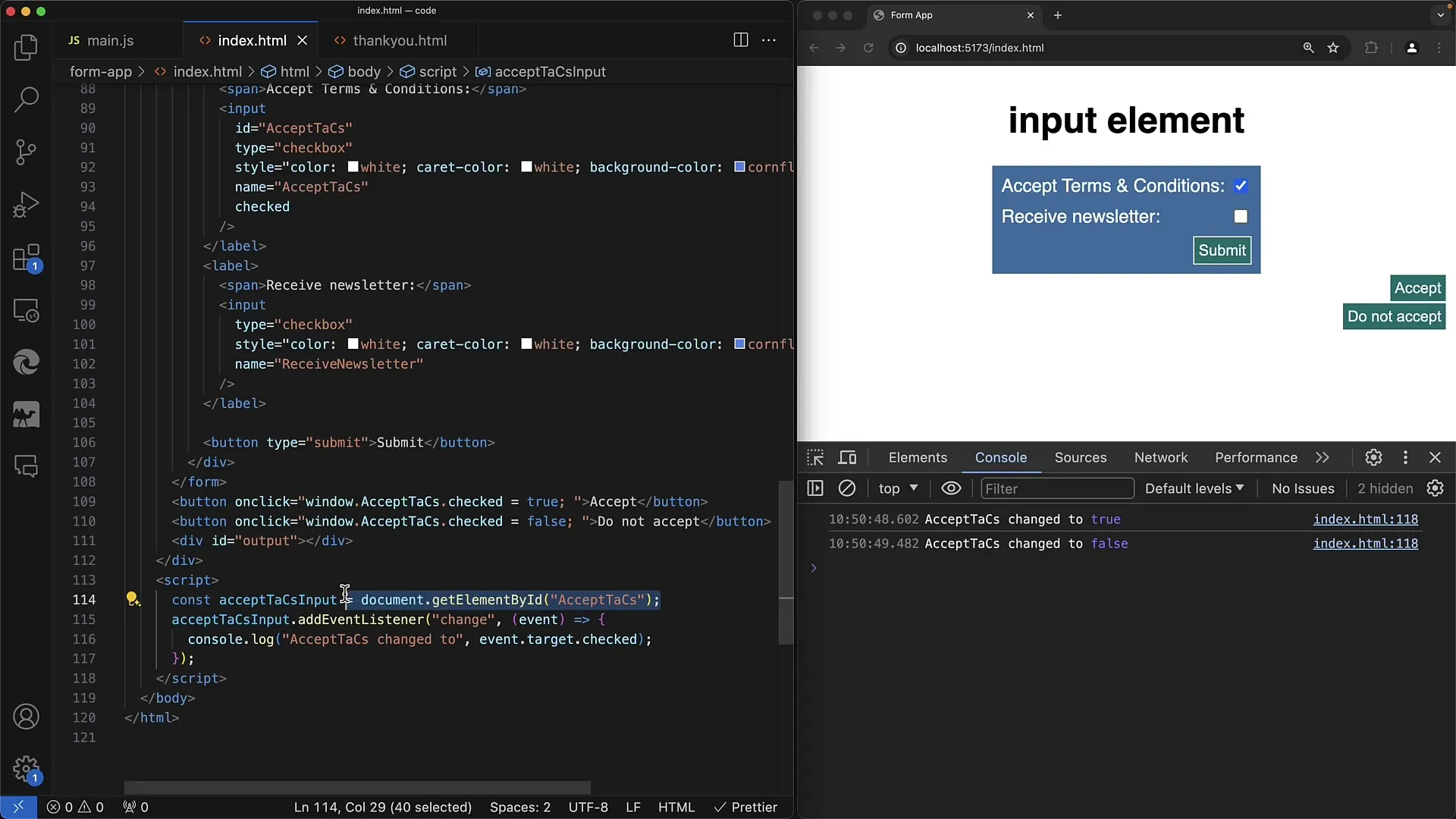
Here we use document.getElementById("acceptTerms") to access the input element.
Step 2: Add Event Listener
To monitor the checkbox state, we add an event listener. This is done using the addEventListener method and the change event. This way you can react to changes in the checkbox.
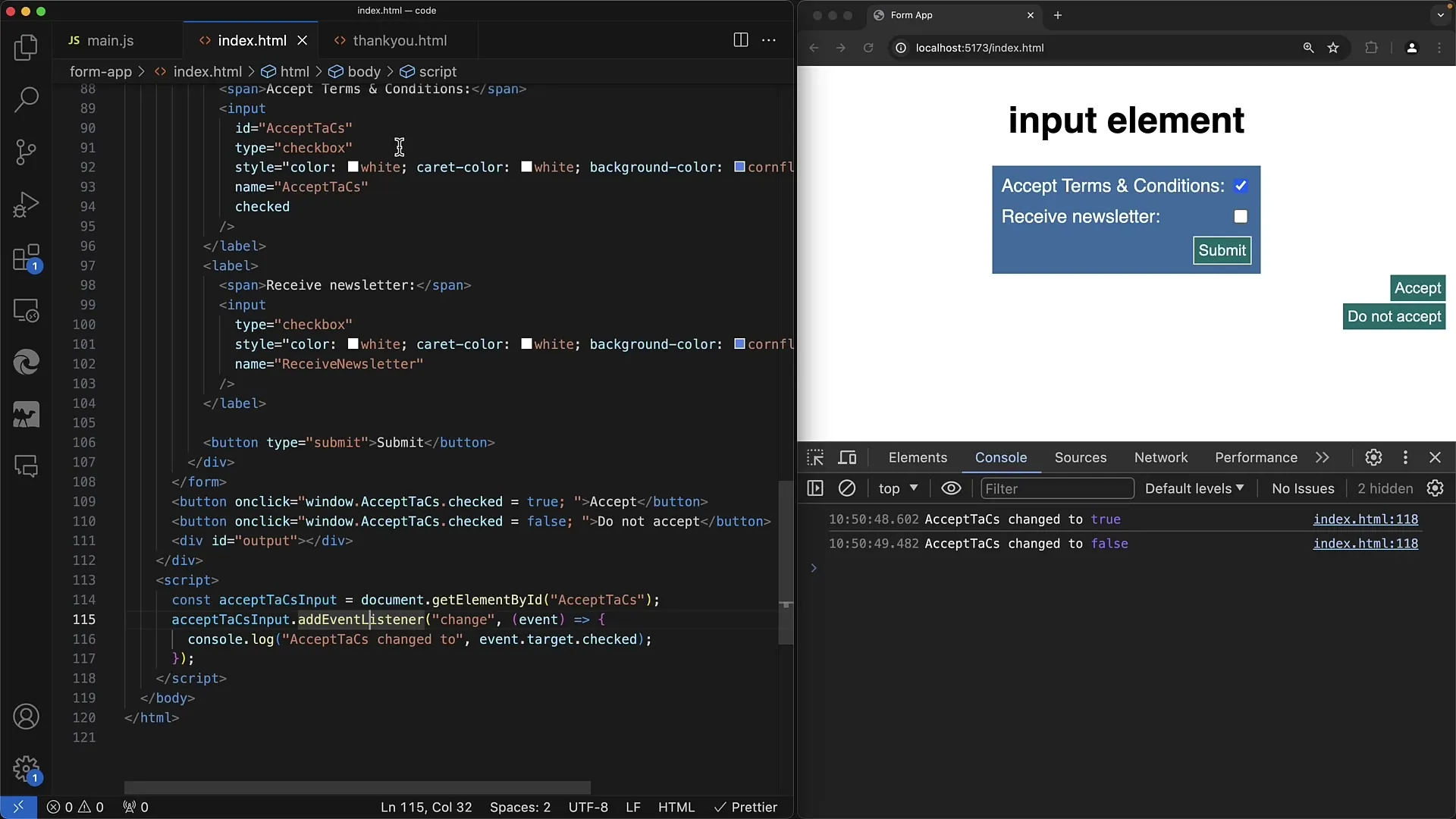
By using an arrow function or a normal function (both are possible), you can output the current state of the checkbox when a change occurs.
Step 3: Check the Checkbox State
In the event listener, you now check the state of the checkbox. Instead of using event.target.value - which does not give you the desired result in this case - you access the checked property.
If the checkbox is activated, checked returns true, otherwise false. Understanding this behavior is crucial to effectively use the checkbox.
Step 4: Example of Output
You can test the functionality by reloading the form and activating or deactivating the checkbox.
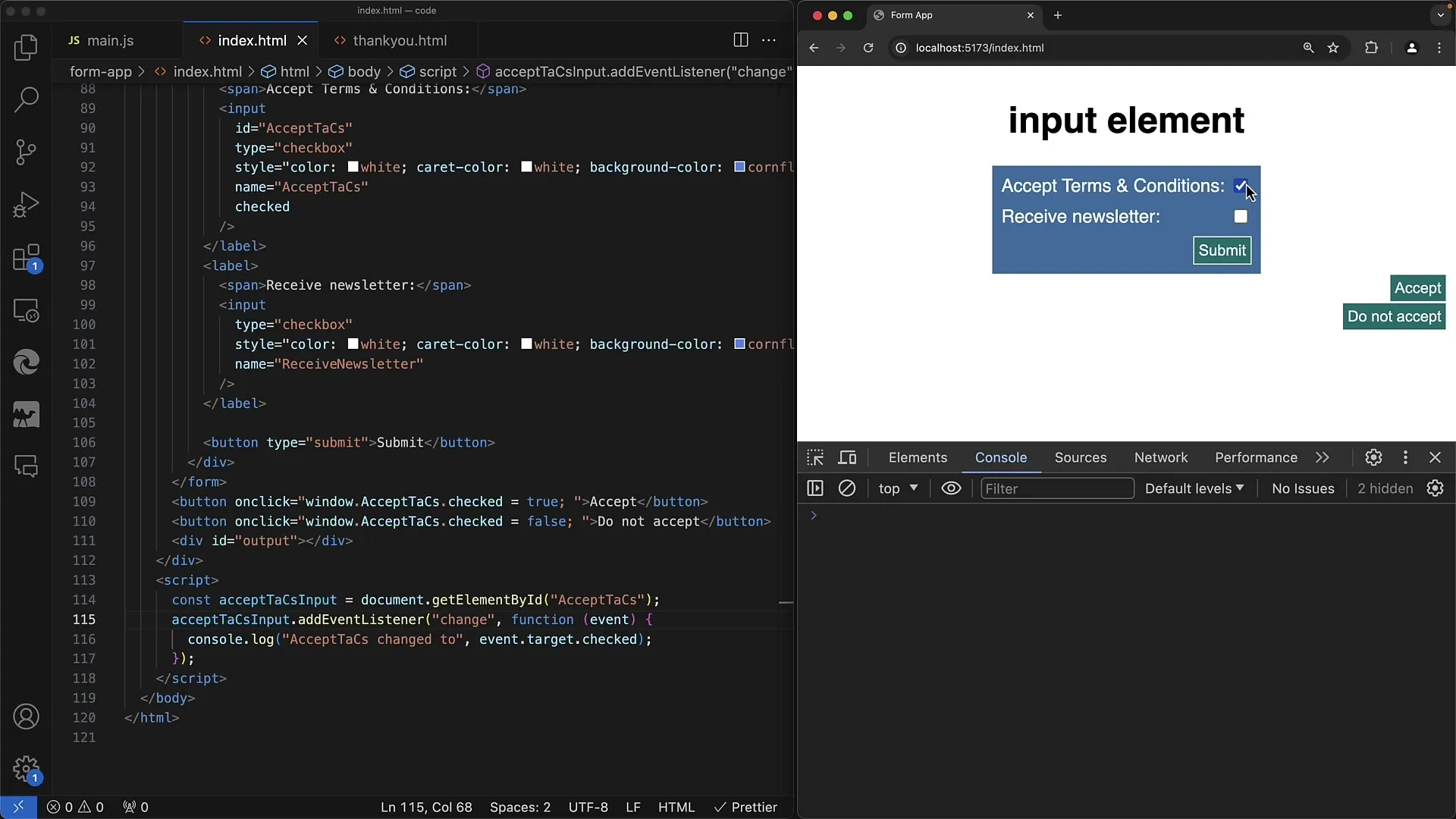
When you uncheck the checkbox, you should see the output accept Terms and Conditions change to false, and when you check it again, true appears. This way you can verify if the checkbox is working correctly.
Step 5: Checkbox Value in HTML
If you display the checkbox initially in the HTML code, you can set the default value with the checked attribute.
A checkbox defined as follows is displayed as activated by default. Through JavaScript, you can also dynamically adjust this property.
Step 6: Programmatically Setting the Checkbox
In this step, I will show you how to programmatically change the state of the checkbox. To do this, we will add two buttons, one for "Accept" and one for "Do Not Accept".
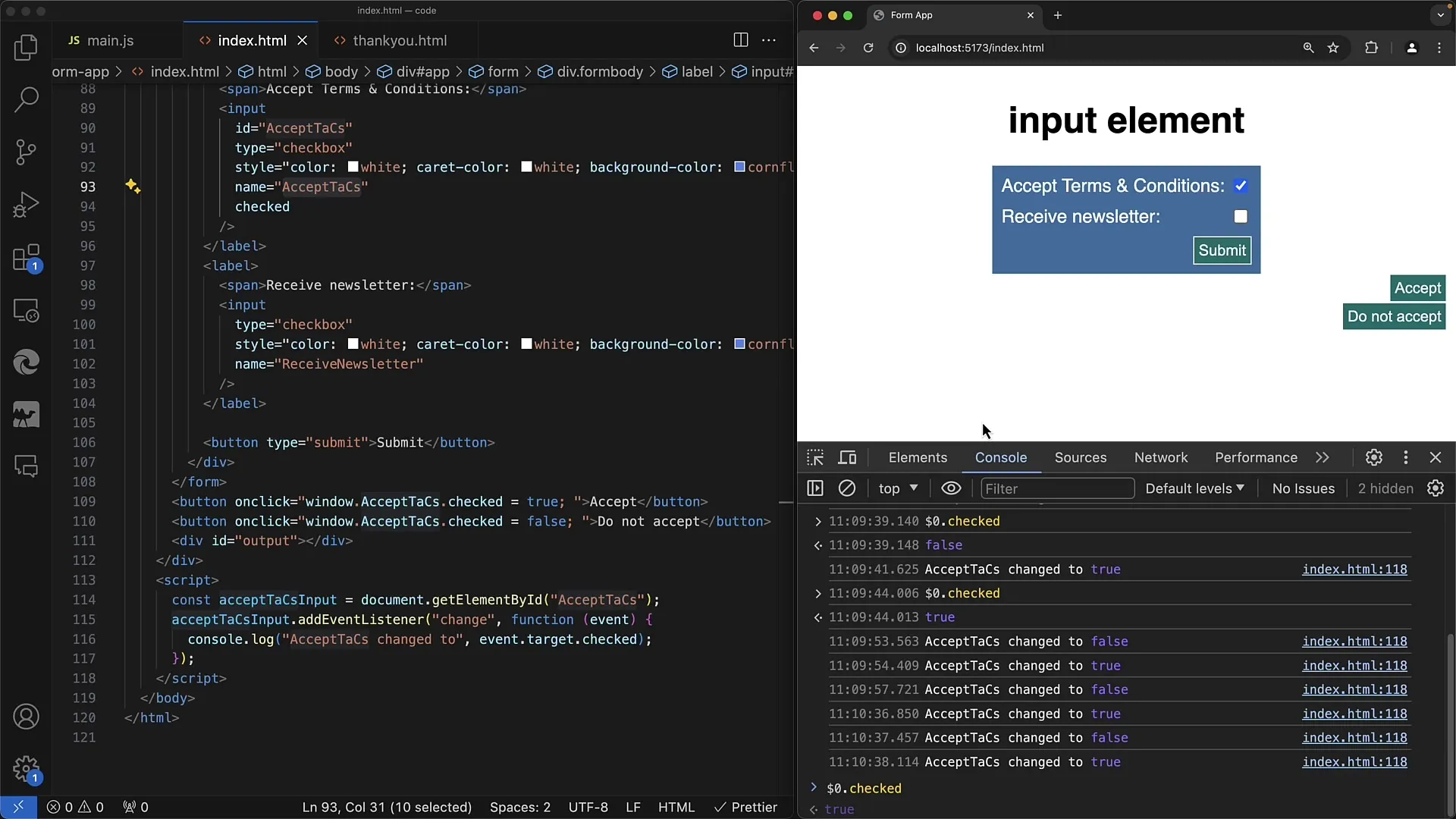
With these buttons, you can change the value of the checkbox directly, without triggering a change event. Keep in mind that this is not a user action; therefore, there is no notification of a change.
Step 7: Behavior of the Change Event
Since you are changing the checkbox through code, there is no change event triggered. This is important to understand in order to avoid logical errors in your code. If there is no user interaction, the change listener will not be activated.
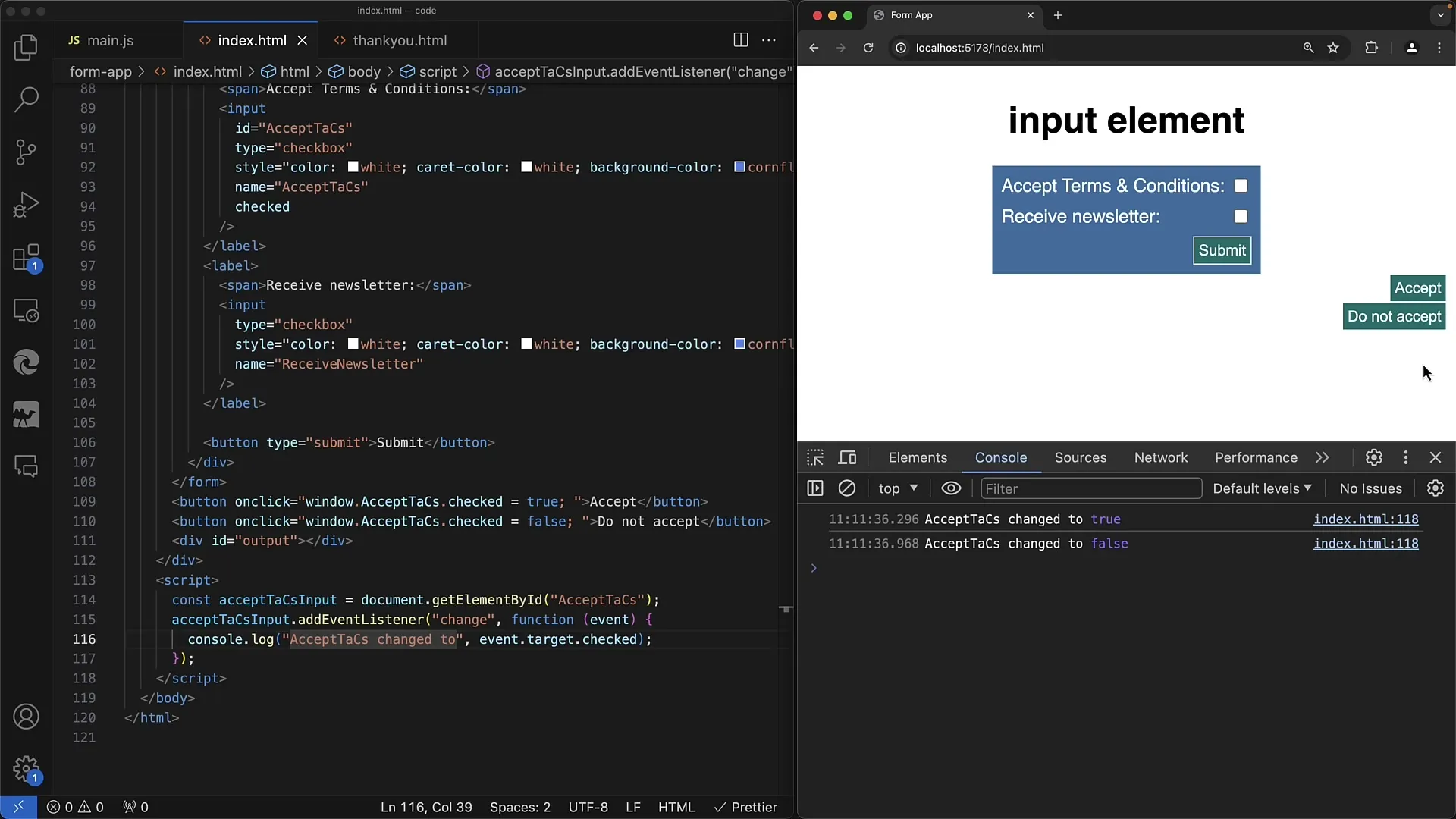
This means that when you click on the "Accept" button, nothing happens, no event is triggered. User actions, on the other hand, trigger the listener and thus changes in the display or logic of your application.
Step 8: Conclusion and Future Applications
Now you have learned how to manipulate checkboxes both in Vanilla JavaScript and programmatically. These basic techniques are applied similarly in many frameworks such as React or jQuery, but the specific implementation may vary.
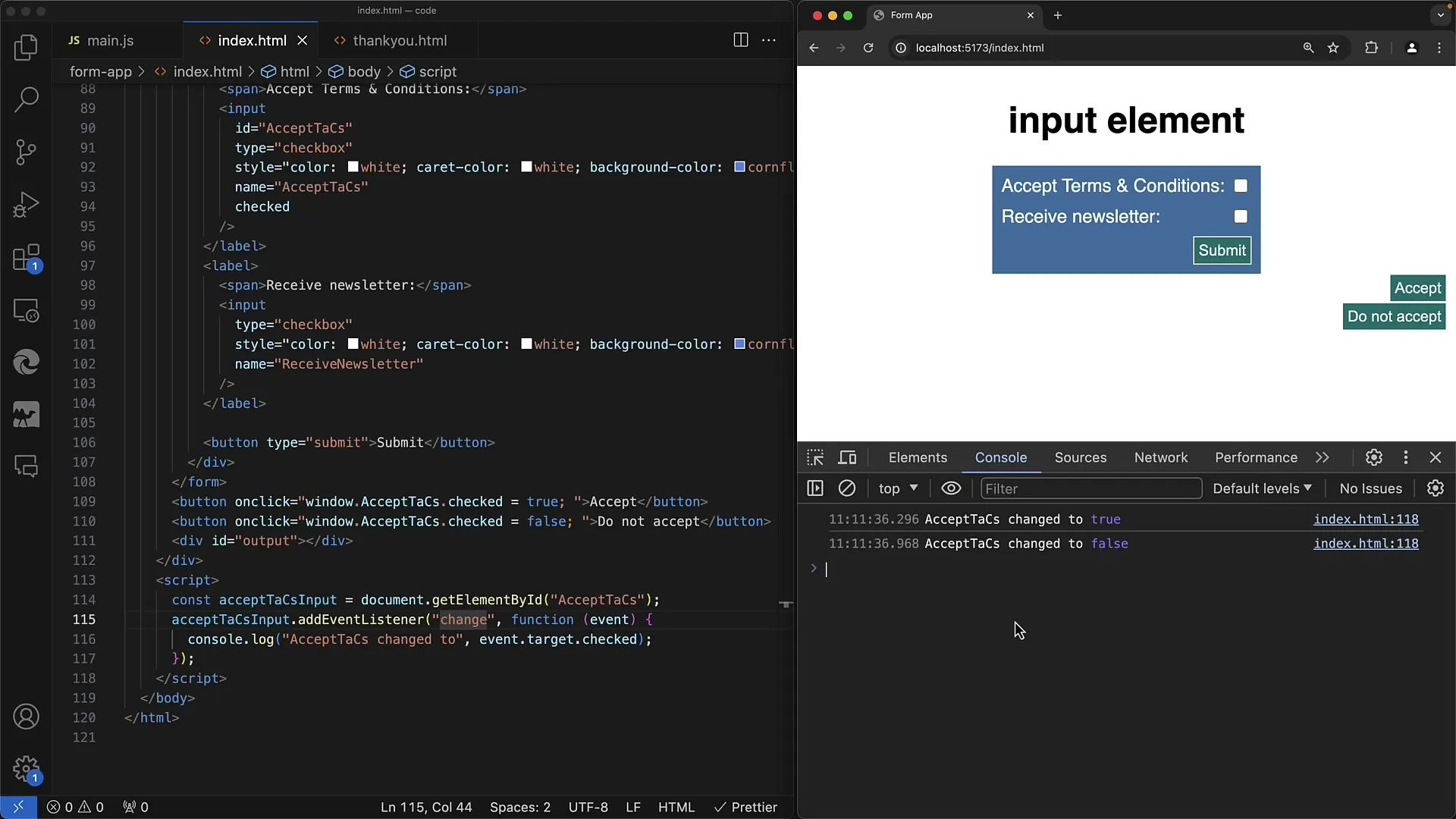
In future tutorials, we will explore how these concepts are implemented in different frameworks and what additional features you can use to enhance user interaction.
Summary
In this guide, you have learned how to check and programmatically set the state of checkboxes using JavaScript. With these skills, you will be able to create user-friendly forms that meet the needs of your application.
Frequently Asked Questions
How can I query the value of a checkbox in JavaScript?Use the checked property of the checkbox element.
What happens when programming a checkbox with JavaScript?When programmatically setting the checkbox, no change event is triggered.
Can I enable the checkbox in HTML by default?Yes, use the checked attribute in the HTML tag of the checkbox.