Welcome to my WebForms Masterclass! In this course, you will learn everything you need to know about web forms – from the basics to integration in modern web frameworks. Forms are a crucial element for any website as they enable users to input and submit data. In this guide, I will give you an overview of the course content, present the key insights, and guide you step by step through the creation of web forms.
Key Insights
- You will learn the basic functionality of HTML forms.
- Various form elements like Input, Select, and Textarea are covered.
- The course demonstrates how to process and validate form data with JavaScript.
- You will learn how to validate forms without JavaScript.
- The integration of forms in modern UI frameworks like React and Vue.js is discussed.
Step-by-Step Guide to Creating Web Forms
Step 1: Basics of an HTML Form
Understanding the structure of an HTML form is important. Every form in HTML starts with the -tag. Within this tag, you place various form elements that allow users to input information. A simple form could look like this:
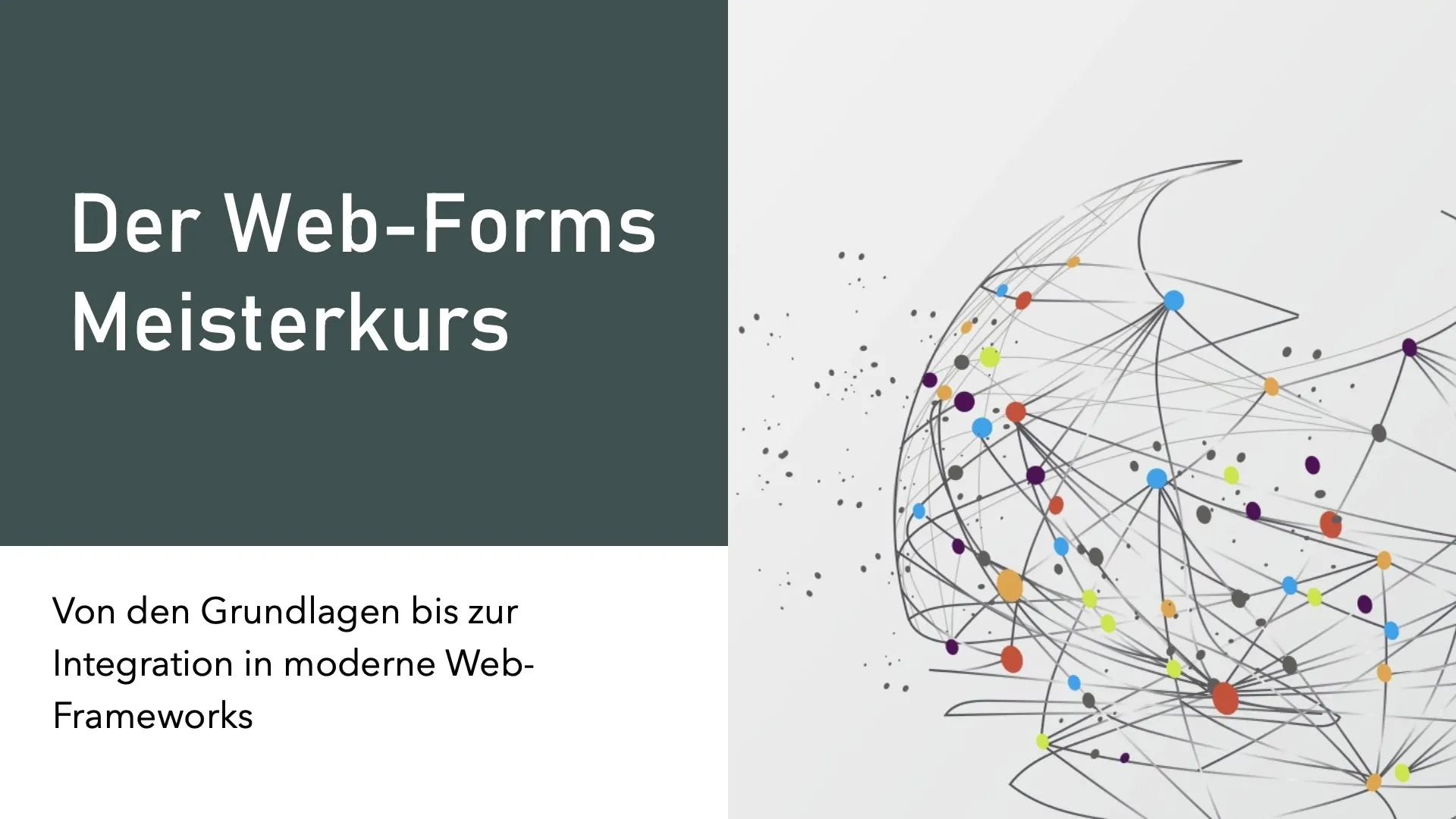
The -tag also has important attributes you should know. These include action, which specifies where the form data should be sent, and method, which defines the type of transmission (e.g., POST or GET).
Step 2: Form Elements
The primary element of a form is the input field. This is usually done with -tags. There are various types of inputs you can use, such as text fields, checkboxes, and radio buttons. Here’s an example of using a text field:
In addition to <input>, there are other form elements like <select> for dropdown menus and </select><textarea> for multiline text. Each of these elements has specific attributes you can use to make your forms more user-friendly.
Step 3: Submitting Data to the Server
To send the entered data to the server, you use the action attribute of the -tag. Here, you specify the URL to which the data should be sent. The method attribute determines how the data will be transmitted. When using POST, the data is sent in the HTTP body, while GET transfers the data in the URL.
Step 4: Accessing Form Data with JavaScript
Another crucial aspect is accessing form data via JavaScript. You can do this by accessing the elements of the form and retrieving their values. Here’s how you can get the value of a text field with JavaScript:
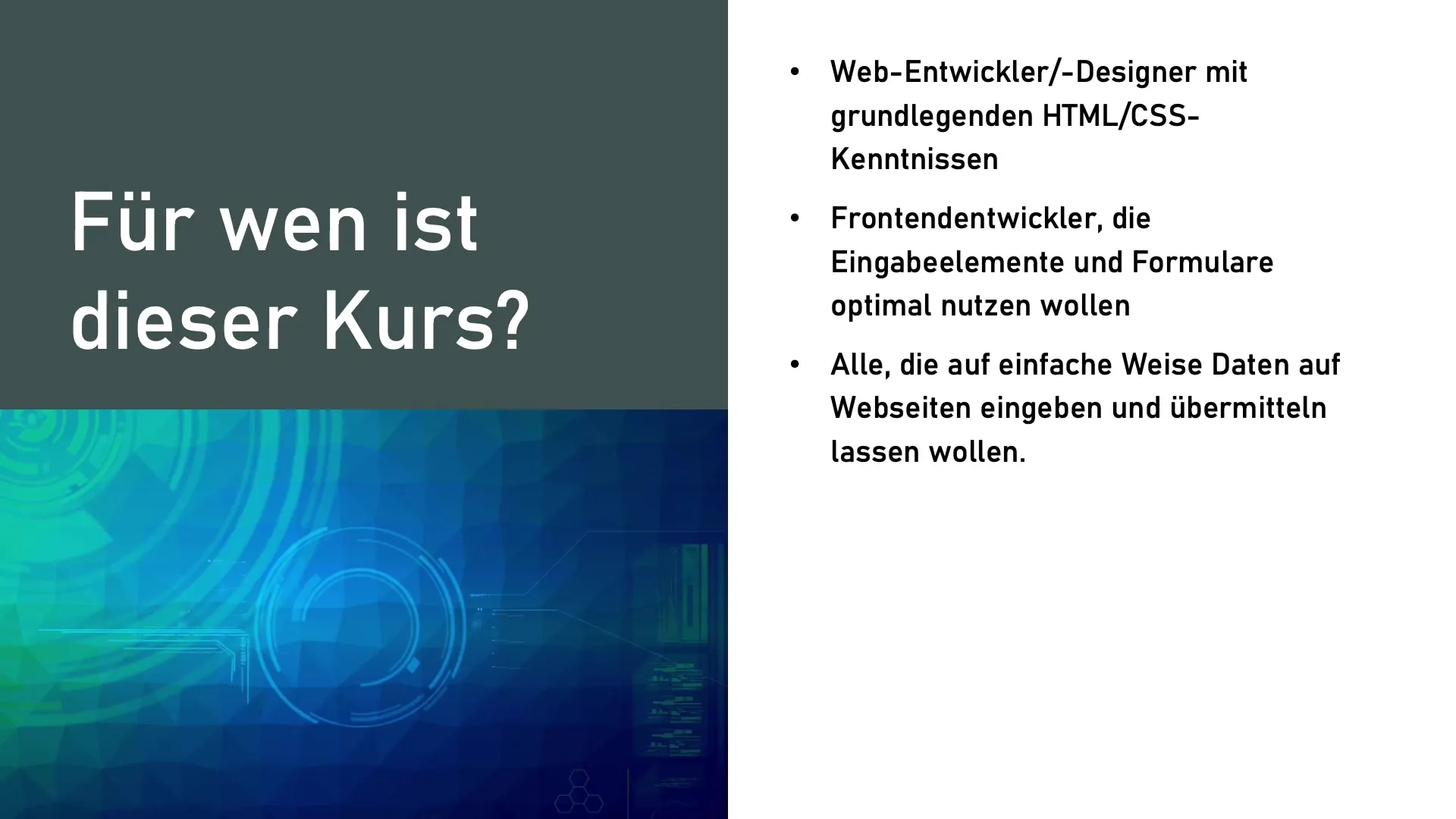
With JavaScript, you can also validate form data before sending it to the server. For example, you can ensure that the field is not empty or meets certain formats.
Step 5: Form Validation without JavaScript
Form validation can also be done without JavaScript by using HTML attributes like required or pattern. These attributes allow you to define basic validation rules directly in the HTML code. Here’s an example:
If a user submits the form and the inputs are invalid, the browser automatically displays an error message, helping the user to correct the inputs.
Step 6: Integration in Modern UI Frameworks
Finally, we will explore the integration of forms in modern UI frameworks like React and Vue.js. These frameworks offer special components and methods to facilitate and optimize the handling of form data. For example, in React, you can manage the form's state with hooks and react to inputs in real-time:
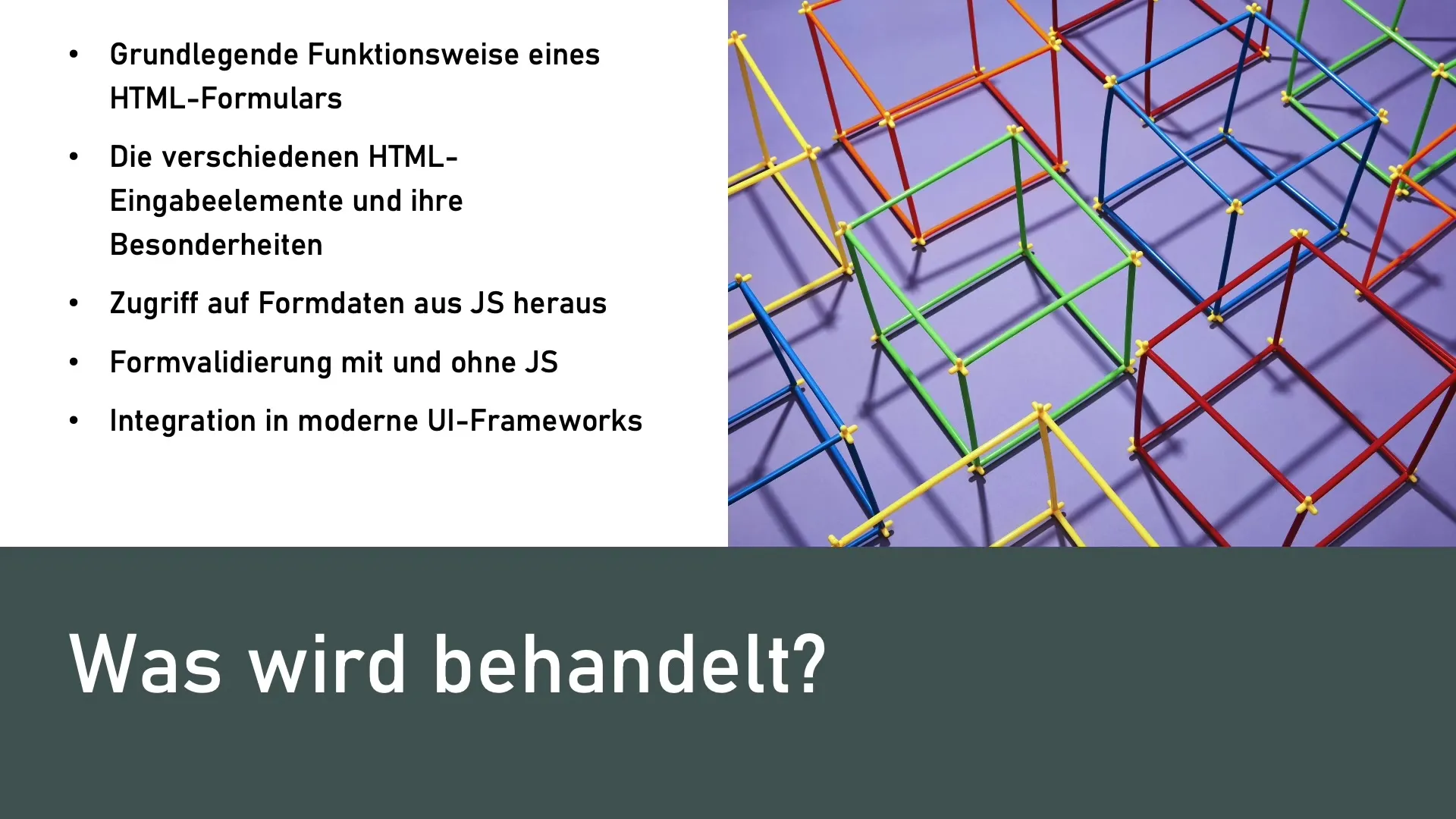
Implementing forms in these frameworks can help you create dynamic and reactive user interfaces that offer a better user experience.
Summary
In this guide, we have covered the key aspects of creating web forms. You have learned how to build a simple HTML form, the types of form elements available, how to submit entered data to the server, and how to validate with and without JavaScript. Additionally, you have gained insight into integrating forms into modern UI frameworks.