In this tutorial, you will learn how to read and process data entered by users from forms using JavaScript. Form elements are an essential part of every website as they allow users to input information. It is often thought that complex frameworks are needed for this. In this post, we show you how to successfully process form data using simple JavaScript.
Key Takeaways
- You will learn how to create forms, access form data with JavaScript, and prevent default form behaviors using preventDefault to gain control over user data inputs.
Step-by-Step Guide
Start by creating a simple HTML form in your document. Make sure to successfully add different input fields and a submit button.
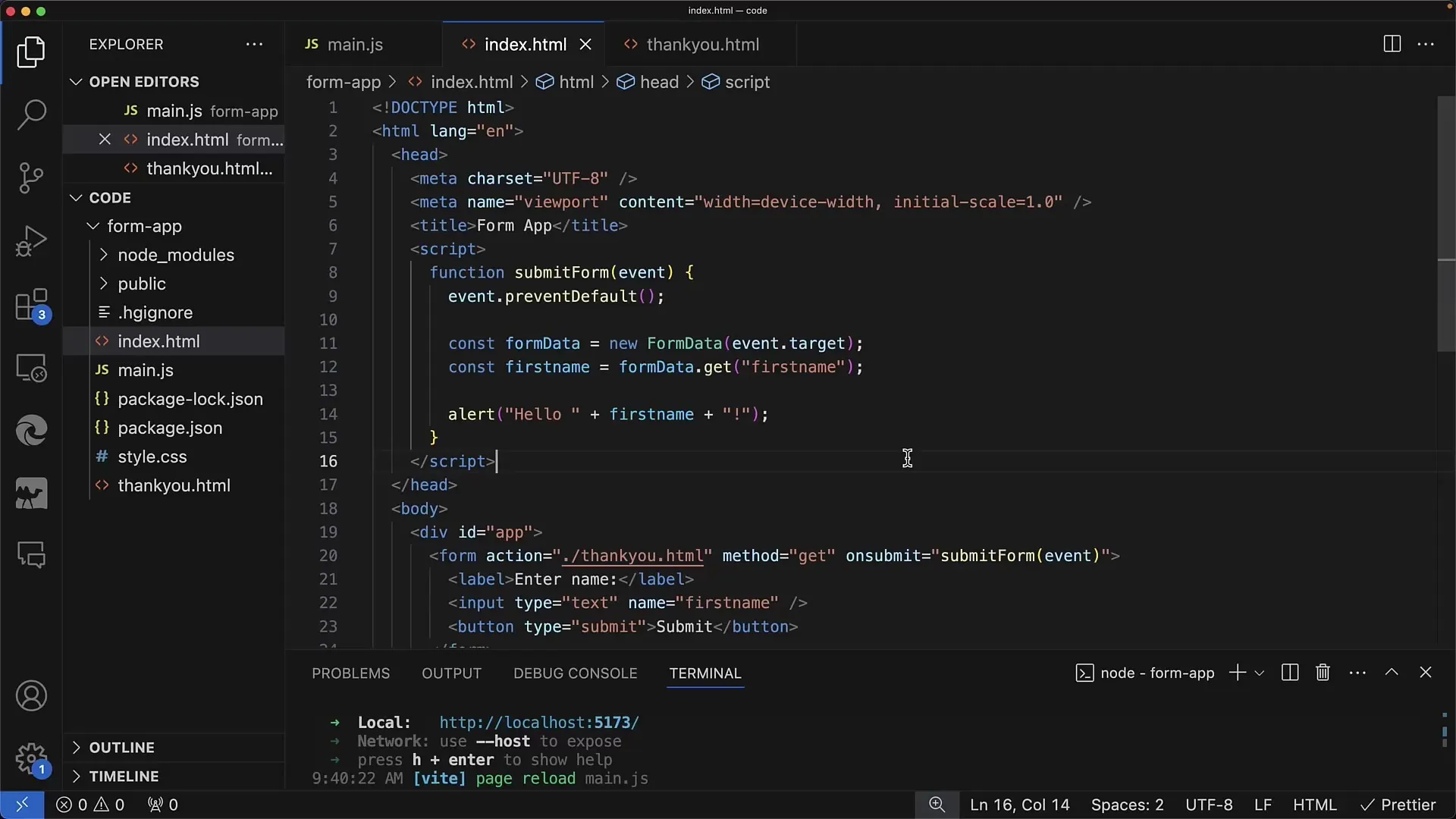
Once your form is visible in the browser, you can test it. Enter a name in the input field and press the Enter key or the submit button. If everything works correctly, you should see an alert with your entered name.
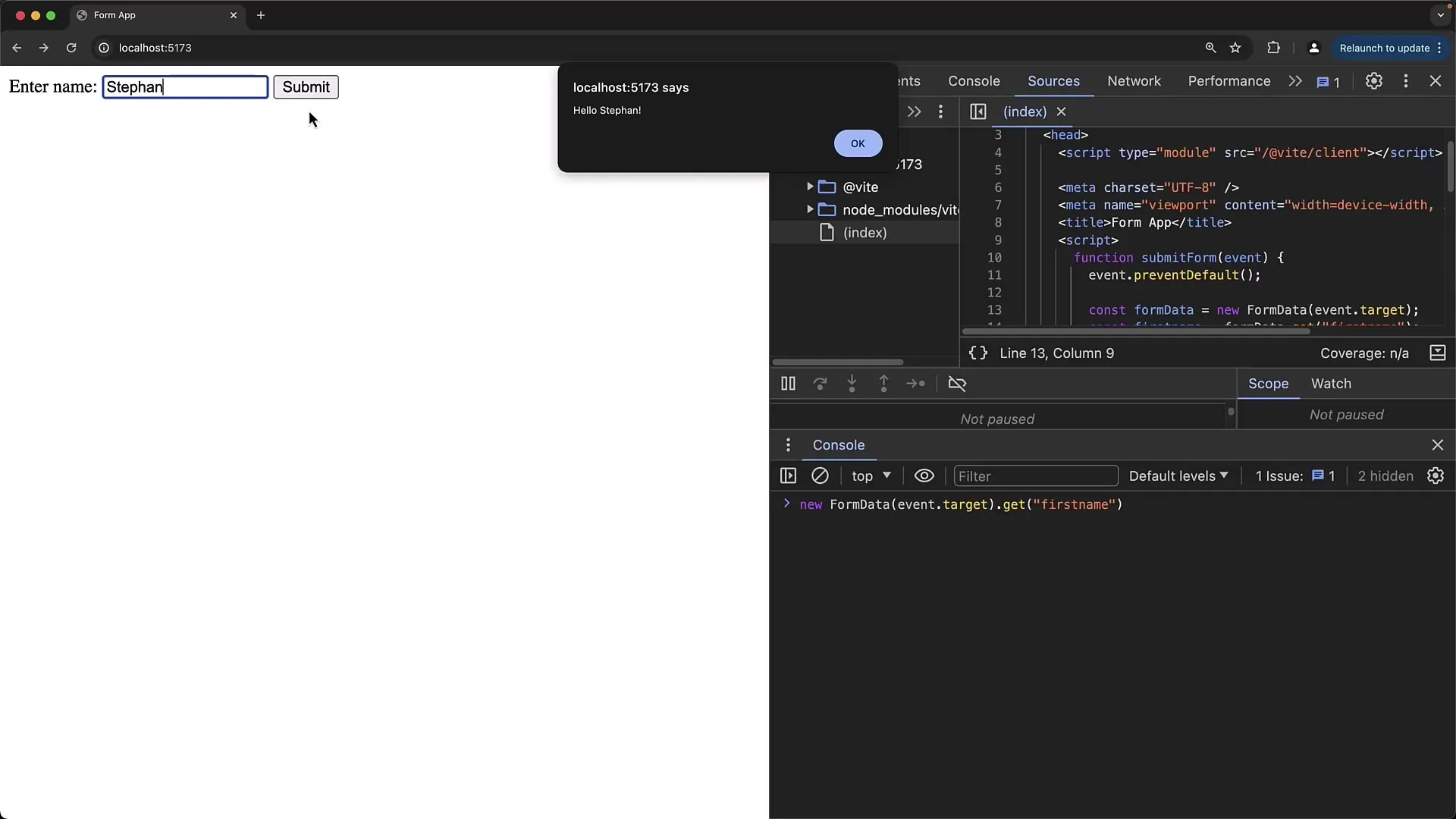
To achieve this, we will add a script tag to your HTML file. In this script, define a global function named submitForm that accepts an event object as a parameter.
In the script, you can set the onsubmit event handler for your form. This means that the submitForm function will be called when the form is submitted. Note that the event object is always available when you are in an event handler.
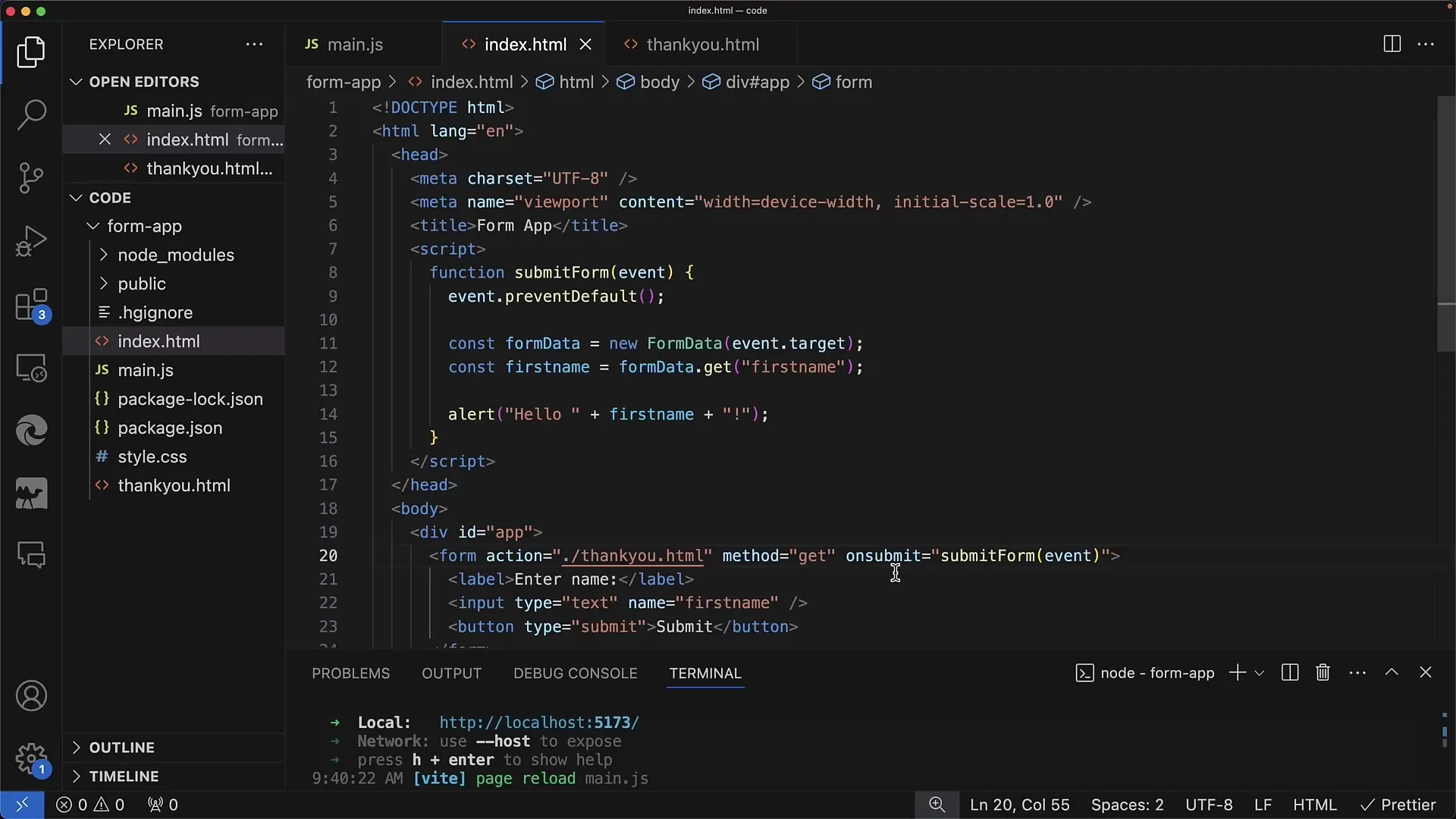
One of the important steps is to prevent the default action of the form by calling event.preventDefault(). This is important because we do not want the form to reload the page after the data is submitted.
Now we can start reading the form data. The form data is available in event.target, making it easy to access the entered information.
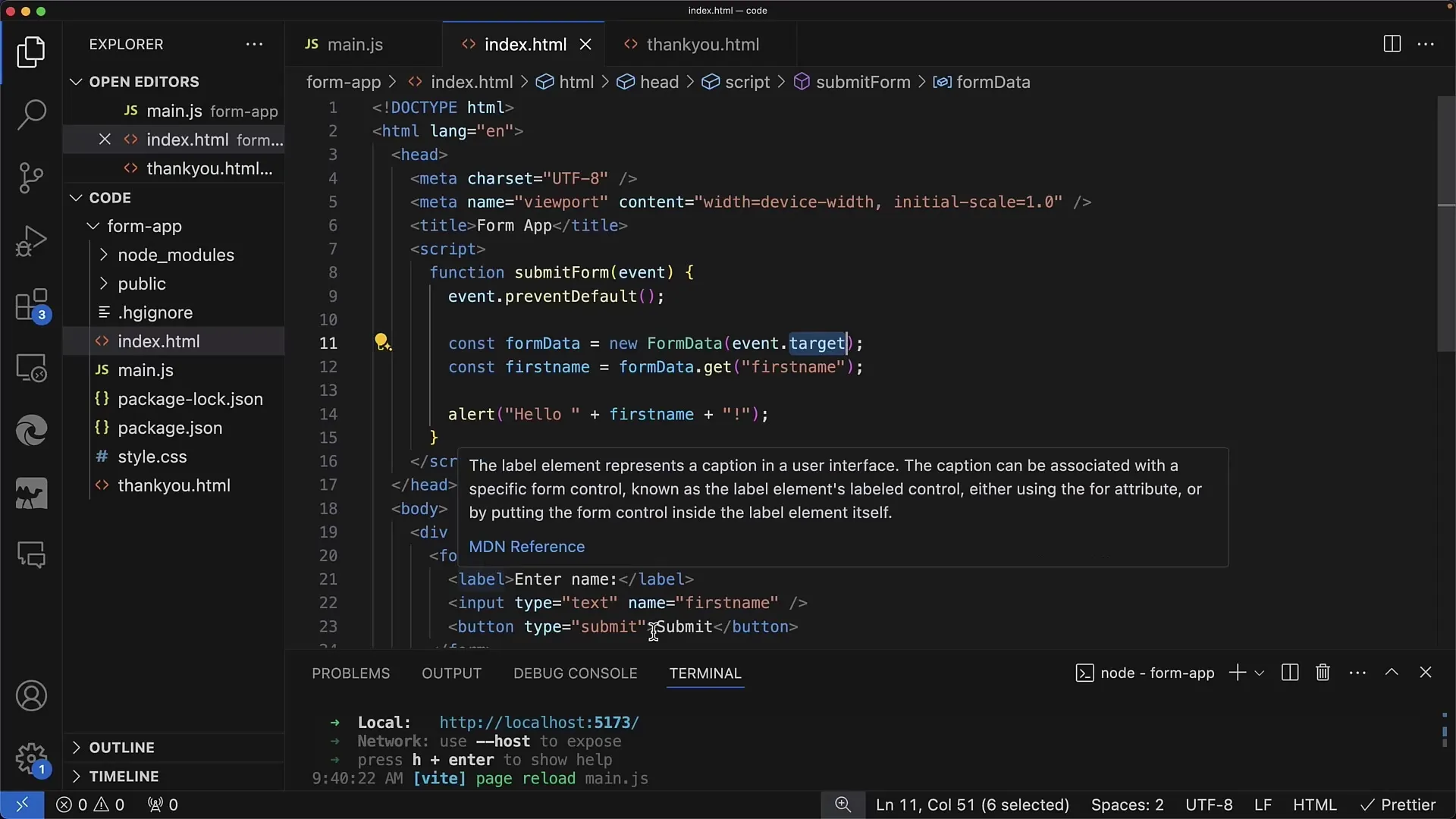
To get the form data, create a new object using the FormData constructor and pass the form element as a parameter. After creating the object, you can access the input values using the get method.
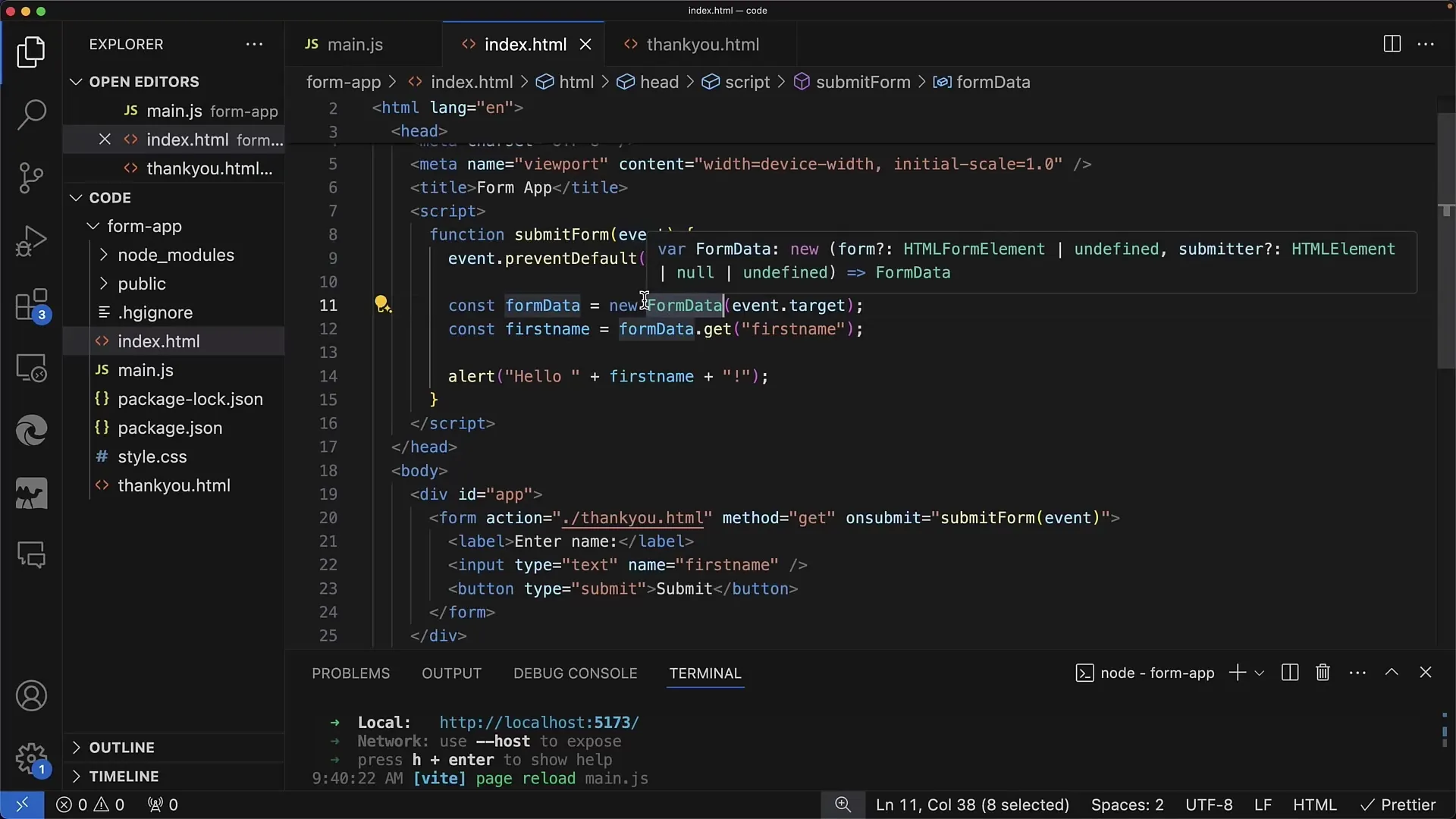
For example, with formData.get('firstName'), you will get the value of the input field with the name 'firstName'. You can then use this value to create a message that will be displayed to the user.
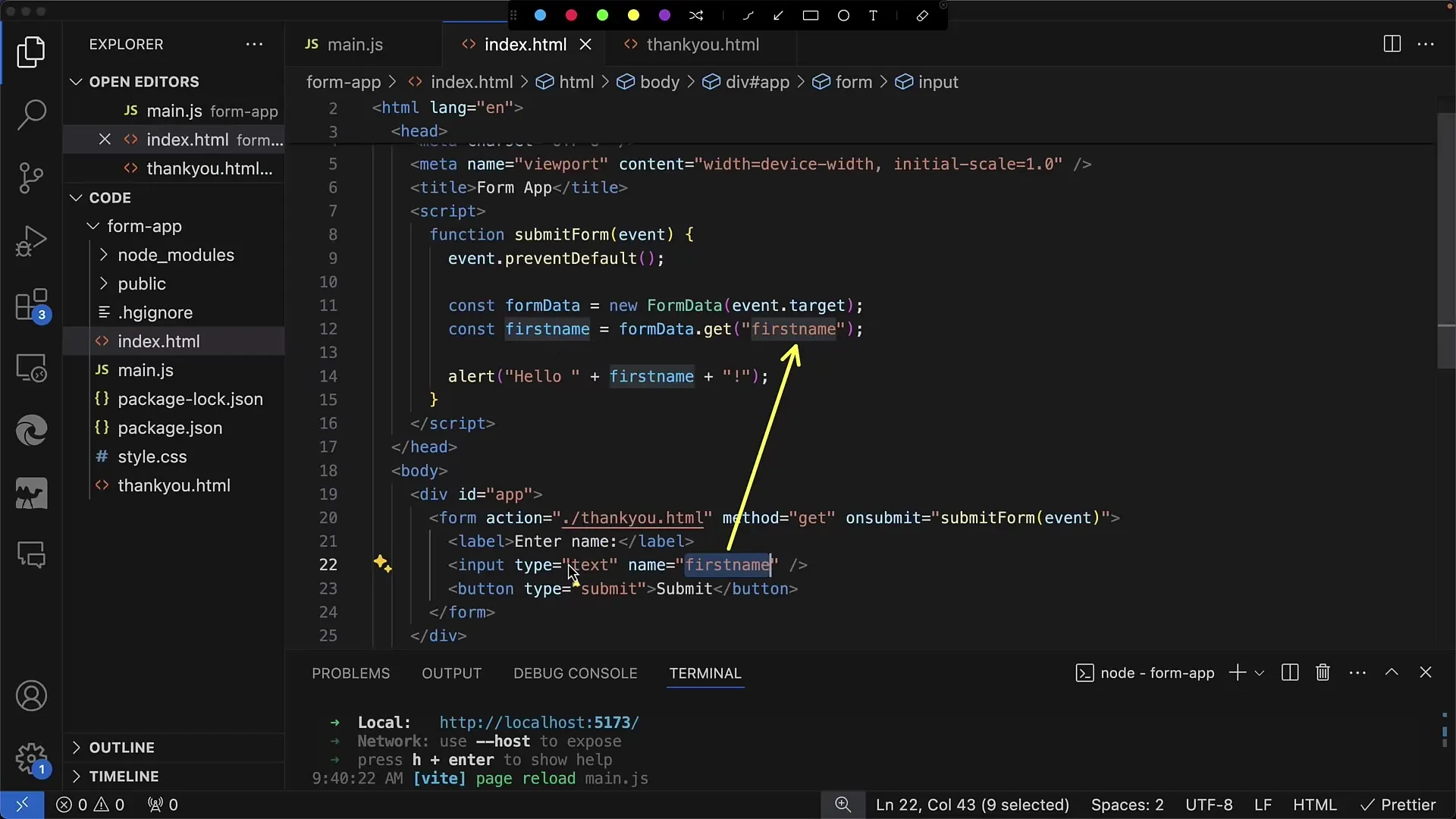
If you want to expand the form by adding another input field, follow these steps: Add an additional text field for the last name with the name 'lastName'. Then you can take similar steps to retrieve its value.
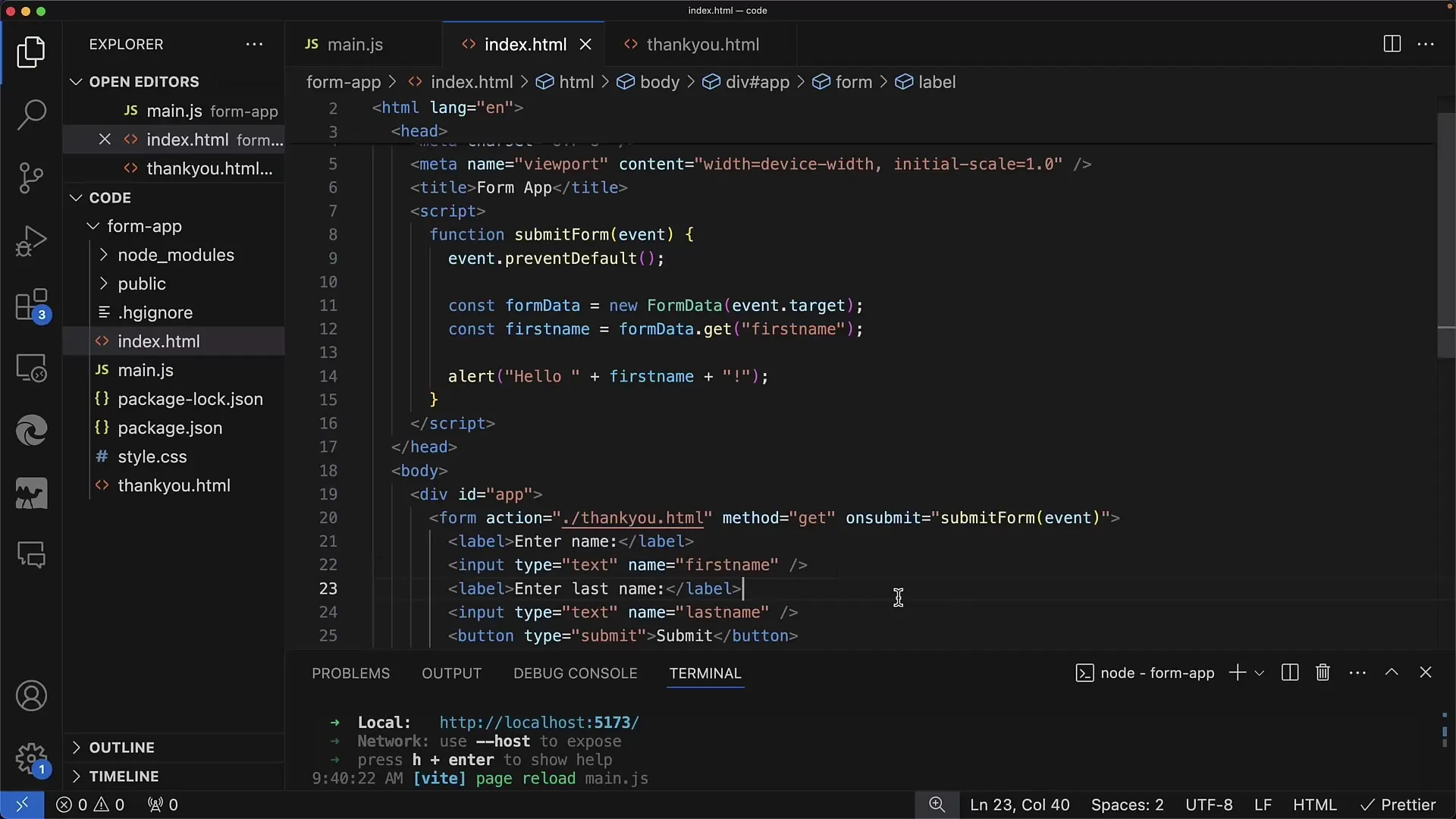
With both values, you can then generate a personalized message. For example: "Hello [First Name] [Last Name]!"
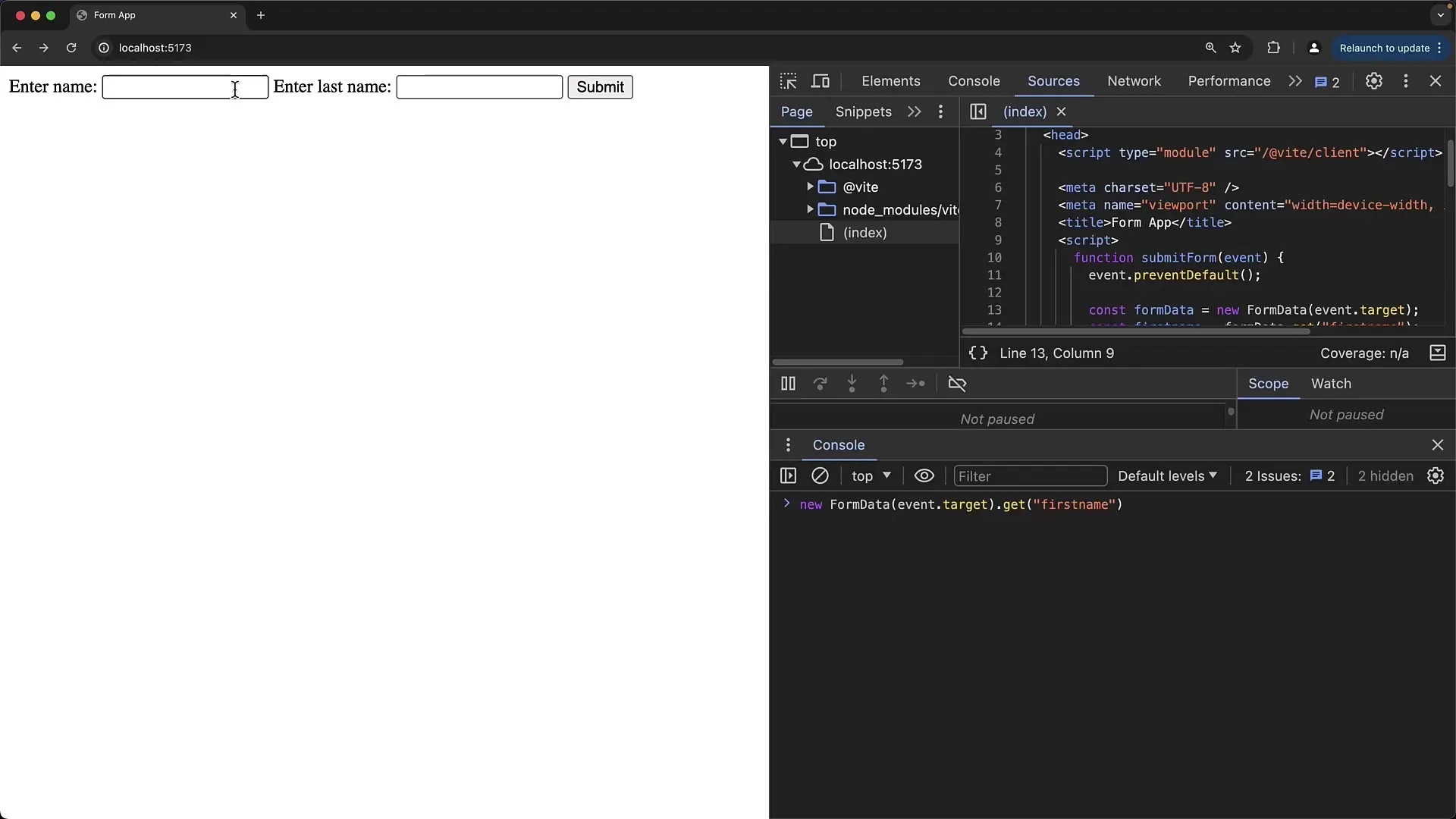
To no longer display the message through an alert but to directly integrate it into the HTML, you can add a new section in the DOM that displays the message as text. To do this, create a div with a unique ID and set its innerText to the resulting message.
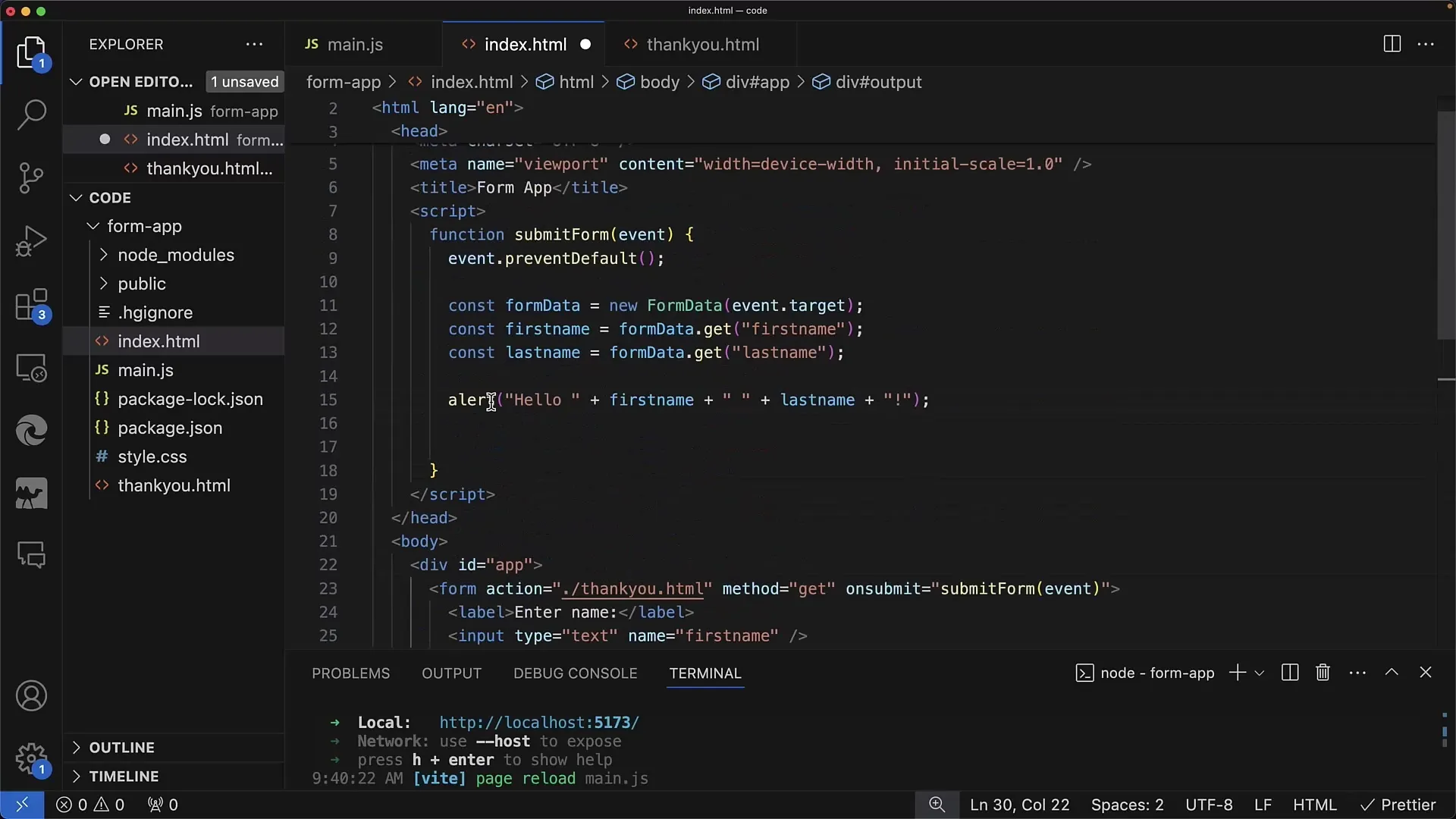
You can now try out different variations by outputting the data in the console or using it for further JavaScript actions, such as making a POST request via fetch.
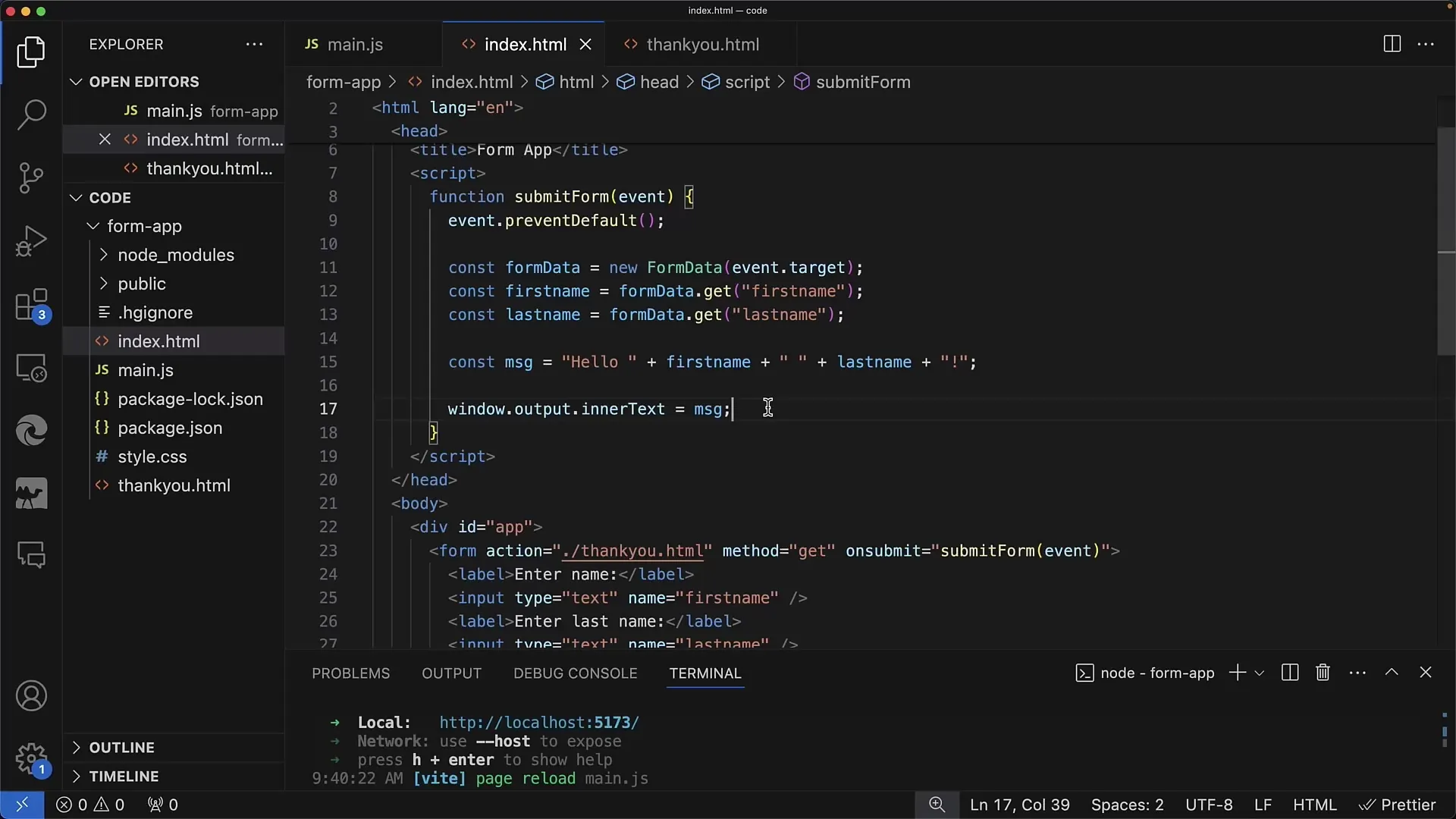
This is the most basic way you can read and use form data with JavaScript. There are many other ways to enhance this, such as adding validations or further processing the data on the server.
Summary
In this tutorial, you have learned how to read form data using simple JavaScript functions. You have explored the importance of event.preventDefault and seen how to insert data into alerts as well as directly into the document. With this foundation, you are well equipped to design more complex forms and handle their data.
Frequently Asked Questions
How do I access the form data?You can access the form data by creating a new FormData object with new FormData(event.target).
What happens if I don't call event.preventDefault()?Without event.preventDefault(), the browser's default behavior will be executed, which can result in the page reloading.
Can I have multiple forms on one page?Yes, you can have multiple forms on one page, and each form can have its own onsubmit function.
How can I use the form data for a Fetch request?You can convert the form data into a JSON format and then send it to the server using the Fetch API.
Are JavaScript frameworks necessary for form processing?No, it is not necessary to use frameworks. You can perform form processing entirely with pure JavaScript.