In this guide, you will learn how to receive form data using the POST method. When developing web applications, it is crucial to understand how data is sent from the client side to the server and processed there. This guide is based on the popular Node.js express framework. Other technologies will also be briefly discussed to give you a broader understanding.
Main Takeaways
- The POST method is used to send data to the server, and the server must be configured to receive and process this data accordingly.
- You must ensure that the necessary middleware, such as body-parser, is installed and configured to parse the incoming data into a usable format.
- Understanding the distinction between URL-encoded form data and JSON data is important for correctly processing requests.
Step-by-Step Guide
To receive data with a POST handler, start with the following steps:
Step 1: Set Up the Server
First, start your Express server. You can create the basic structure of an Express server by ensuring that the necessary packages are installed. If you don't have an Express project yet, create one with the command npm init and install Express with npm install express.
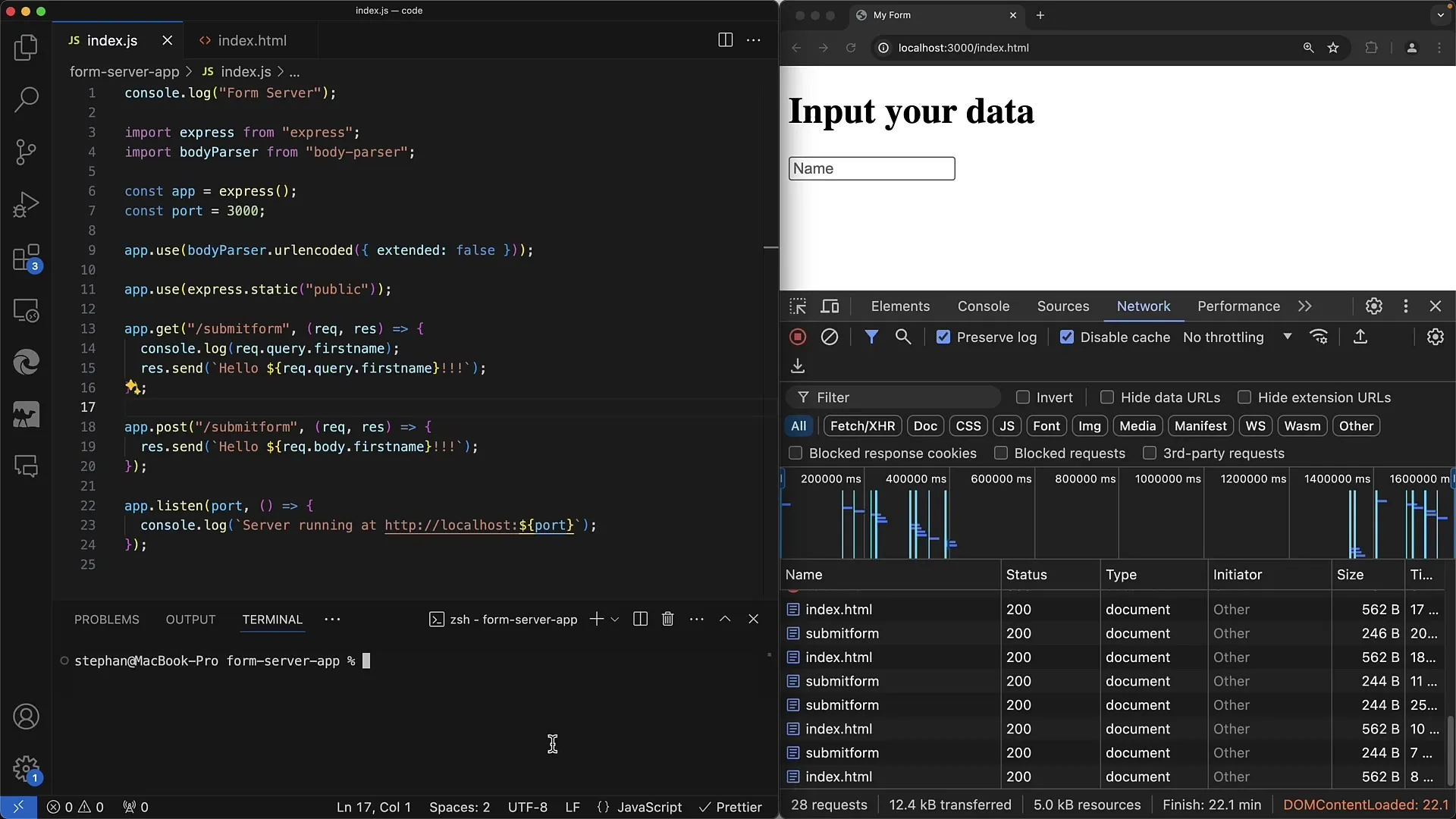
Step 2: Define the POST Handler
Define a POST handler in your server code. This is done using the method app.post(), where you specify the URL to which the POST request is directed and a callback function. In this callback function, you can process the form data.
Step 3: Receive Data in the Body
To receive the data from the body of the POST request, you must use req.body. Here, you access the sent form data. Note that you must use the name of the input in your HTML form to correctly retrieve the data.
Step 4: Set Up Middleware
Don't forget to install and configure the body-parser middleware. This middleware is necessary to parse the incoming data. Use app.use(bodyParser.urlencoded({ extended: true })) to process the URL-encoded data. Make sure to set the middleware before defining the POST handler.
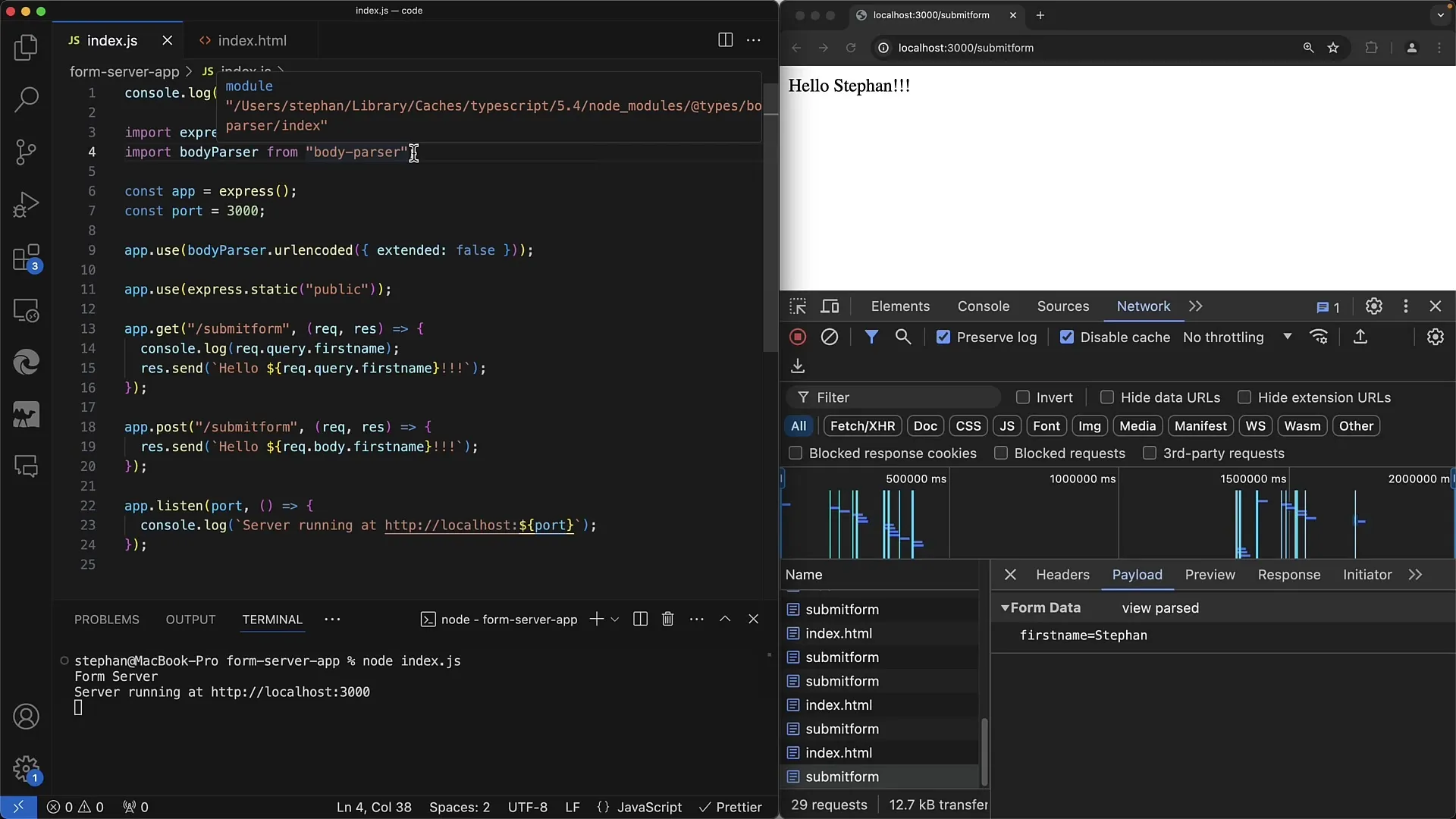
Step 5: Test Form Data
Now you can fill out your HTML form and send the data to the server. Restart your server and test the form by making your entries and submitting the form. Ensure that the server responds correctly and processes the data.
Step 6: Process JSON Data (optional)
If you want to send JSON data, you can do so as well. Make sure to use the appropriate middleware. To process JSON data, use app.use(bodyParser.json()). This is particularly useful if your application has API-like specifications or uses frontend frameworks like React or Vue.
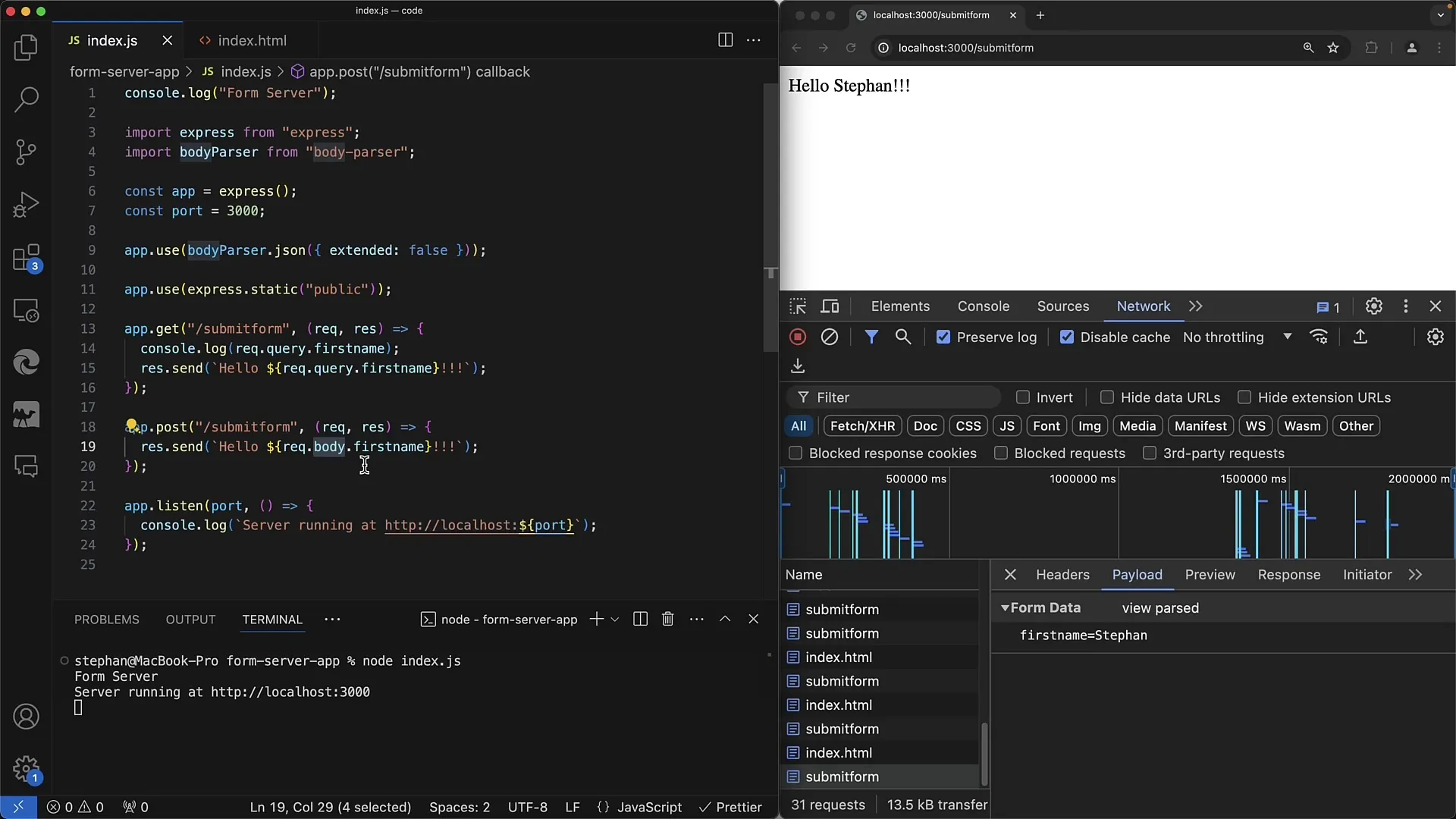
Step 7: Receive Files (optional)
If you plan to receive files via forms, you will need a special library, such as multer. This allows for handling multipart data. Note that these adjustments may vary depending on the server technology used.
Summary
In this guide, you have learned how to receive form data using the POST method in a Node.js Express server. You have learned the steps to set up a server, define a POST handler, and correctly configure the middleware. Understanding these concepts is crucial for the development of functional web applications.
Frequently Asked Questions
What is the difference between GET and POST?GET sends data via the URL, while POST transfers data in the body of the request.
How do you install body-parser in an Express project?Run the command npm install body-parser in your project directory.
Can I also send JSON data with a POST request?Yes, you can send JSON data by configuring bodyParser.json() and ensuring that your frontend application sends data in JSON format.