Understanding the structure of a React App is crucial for effective development and programming. In this guide, you will learn the basics of how a React App is structured and how to integrate it into a website. We will explore the structure of the index HTML file, the corresponding JavaScript file, and the fundamental concepts of JSX as well as ES6 modules.
Key Takeaways
- The starting file for React Apps is the index.html.
- React uses the concept of "root node" as an anchor for all components.
- The createRoot method creates a root node where React elements are rendered.
- JSX is a syntactic extension for JavaScript that allows HTML-like syntax.
- ES6 modules are important for structuring code in React.
Step-by-Step Guide
1. Understanding the index.html
When creating a React App, you cannot bypass looking at the index.html file. It is the entry point for your app code and loads the necessary scripts. In a typical file, you will see a div with the ID root. This DIV serves as the root node for the entire React application.
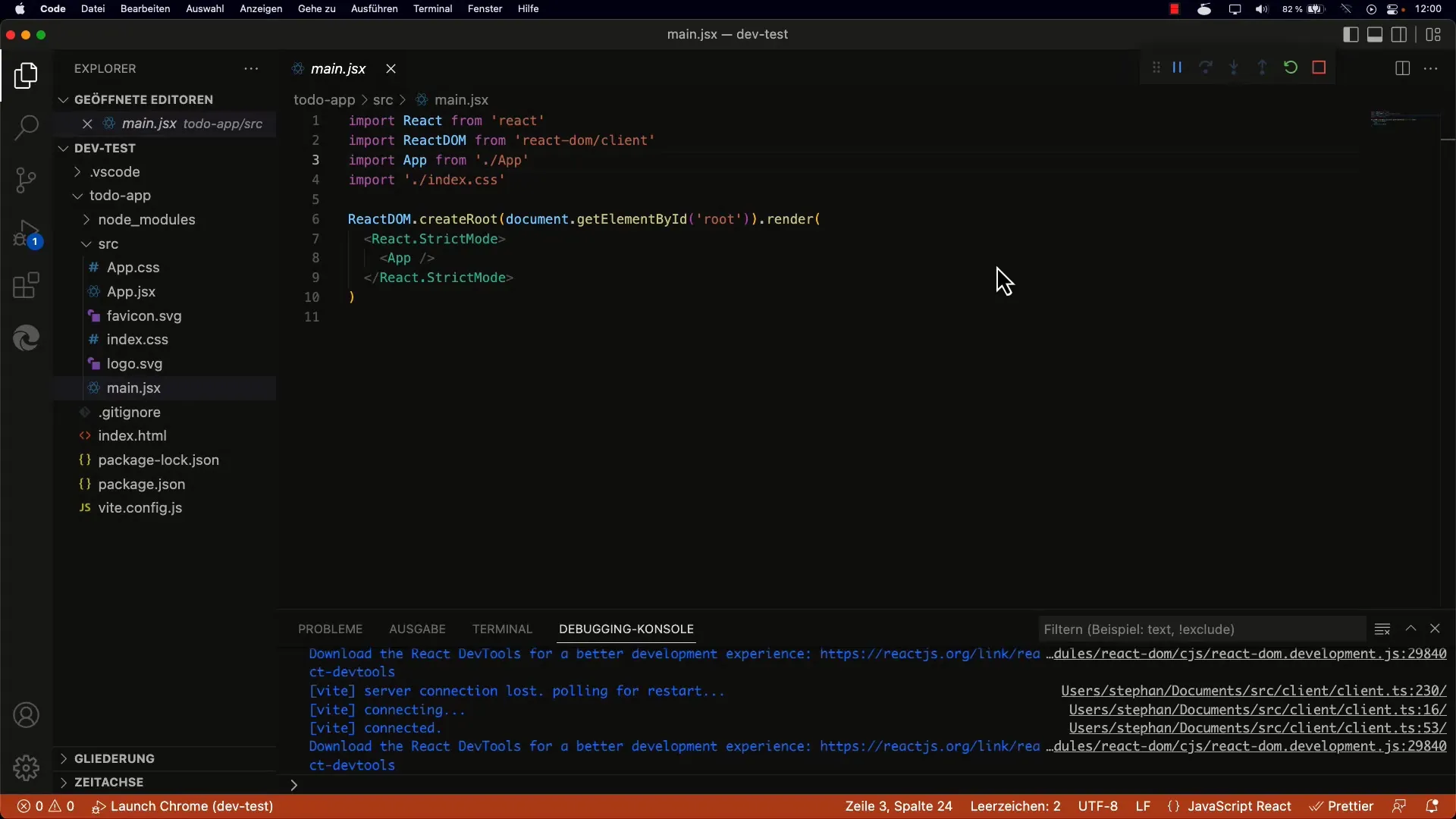
2. Importing the necessary modules in main.jsx
In the main.jsx, which is loaded by index.html, you will see the imports. Here you import React, ReactDOM, and possibly CSS. The modules are necessary to create the app and ensure that you can leverage the best available features. Make sure to import ReactDOM from react-dom/client.
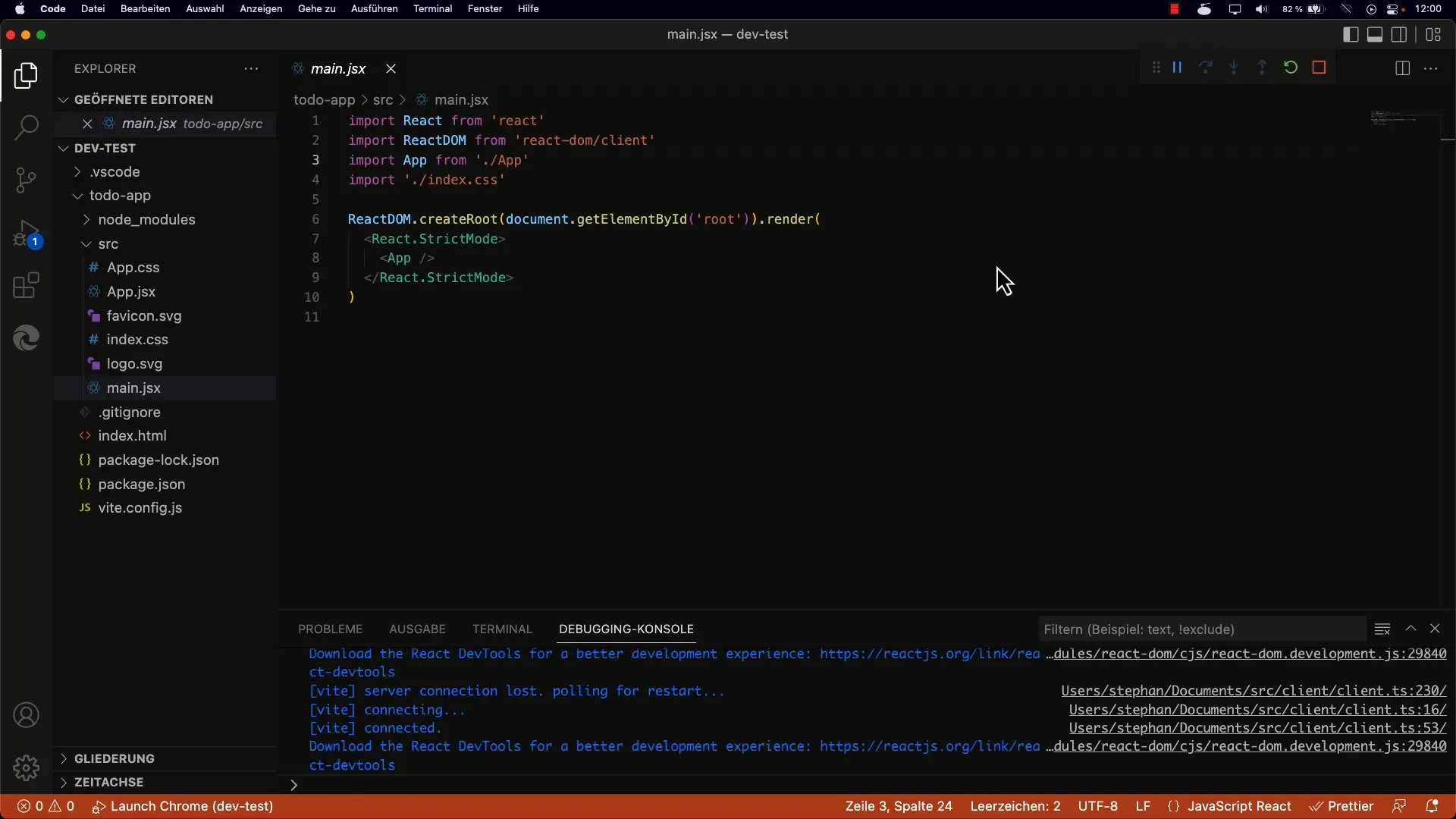
3. Creating the root node
You now need to create a React root node. This is done using the createRoot method. You pass this to the DOM element you defined in index.html earlier. You access the div with the ID root and initialize the root node.
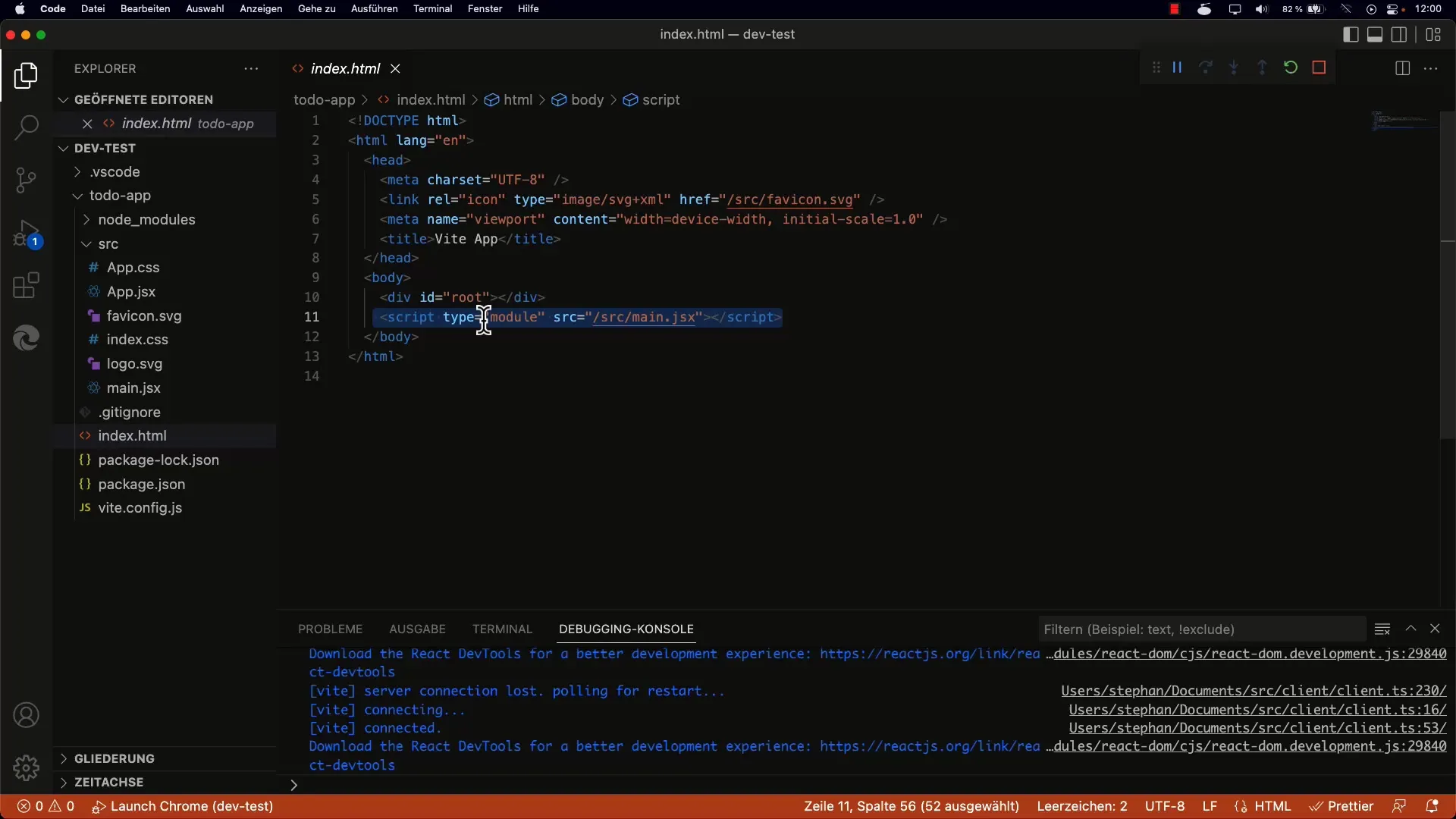
4. Using the render method
After creating the root node, you use the render method. Here you pass the JSX elements that should be rendered. JSX looks almost like HTML, but it gets translated into JavaScript. For example, you could simply render a text node like "Hello World."
5. Understanding the JSX syntax
JSX is a mixture of JavaScript and HTML. This means you write HTML-like code within JavaScript. It allows you to easily and clearly structure your UI. In the example above, you have written a simple text inside a div. This is the first step in creating a React component.
6. Component-based structure
It is recommended to use components to structure your app. Instead of just rendering HTML, you should use React components that you import into your app. This not only improves the readability of your code but also promotes reusability. For example, it is recommended to create an App component and then insert it using JSX.
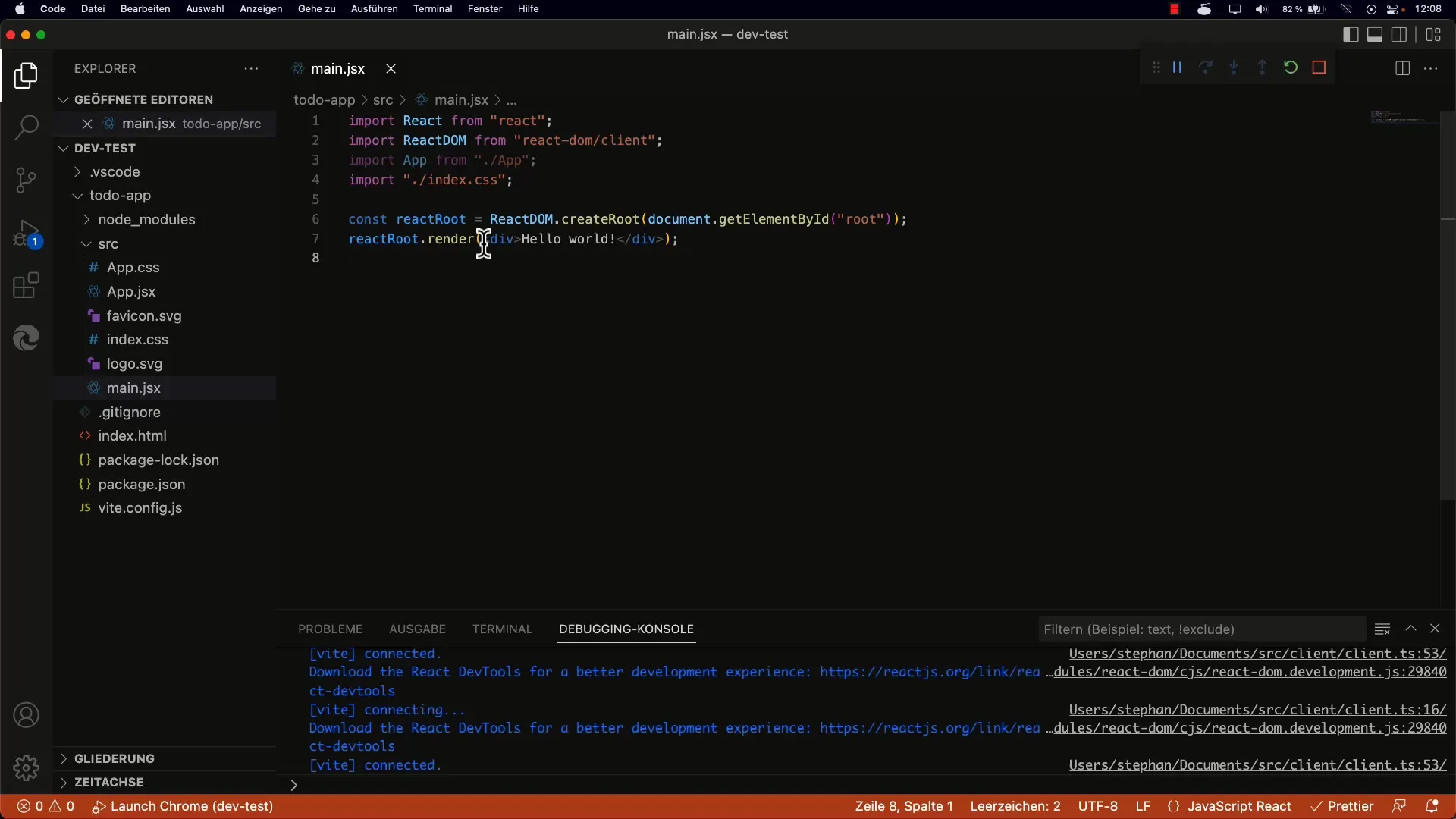
7. Updating the DOM and interactivity
As you progress, you can add interactive elements, such as buttons that change values. React ensures that the DOM is efficiently updated, so that only the necessary parts of the user interface are re-rendered. At this point, you see how the app interface reacts when users interact with it.
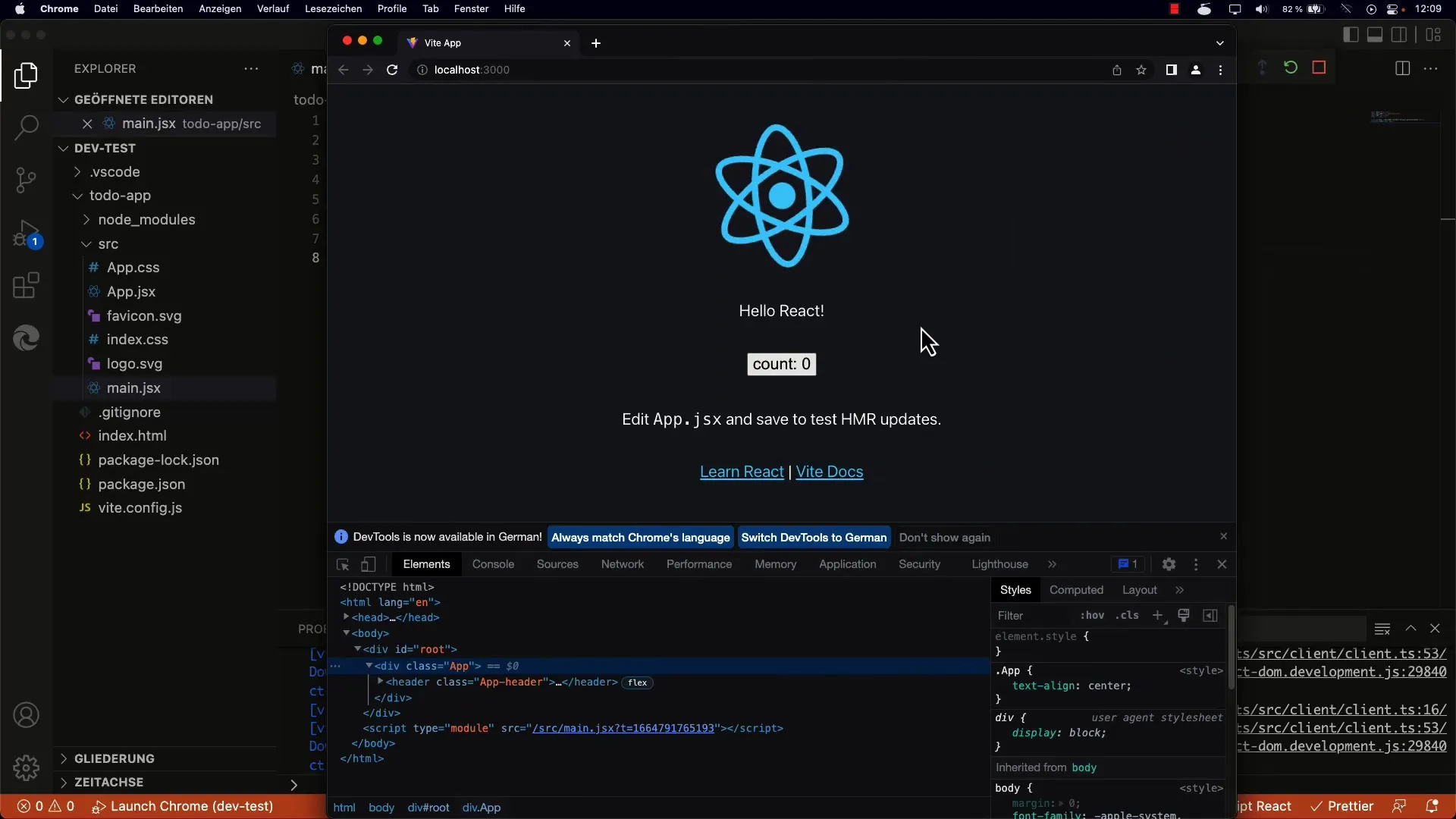
8. Importing the App component and rendering it
Although we have primarily worked with simple HTML in the previous examples, always remember that it is more efficient to divide your UI into components. You can import the App component in main.jsx and then insert it through render. This keeps you within the best practices of React.
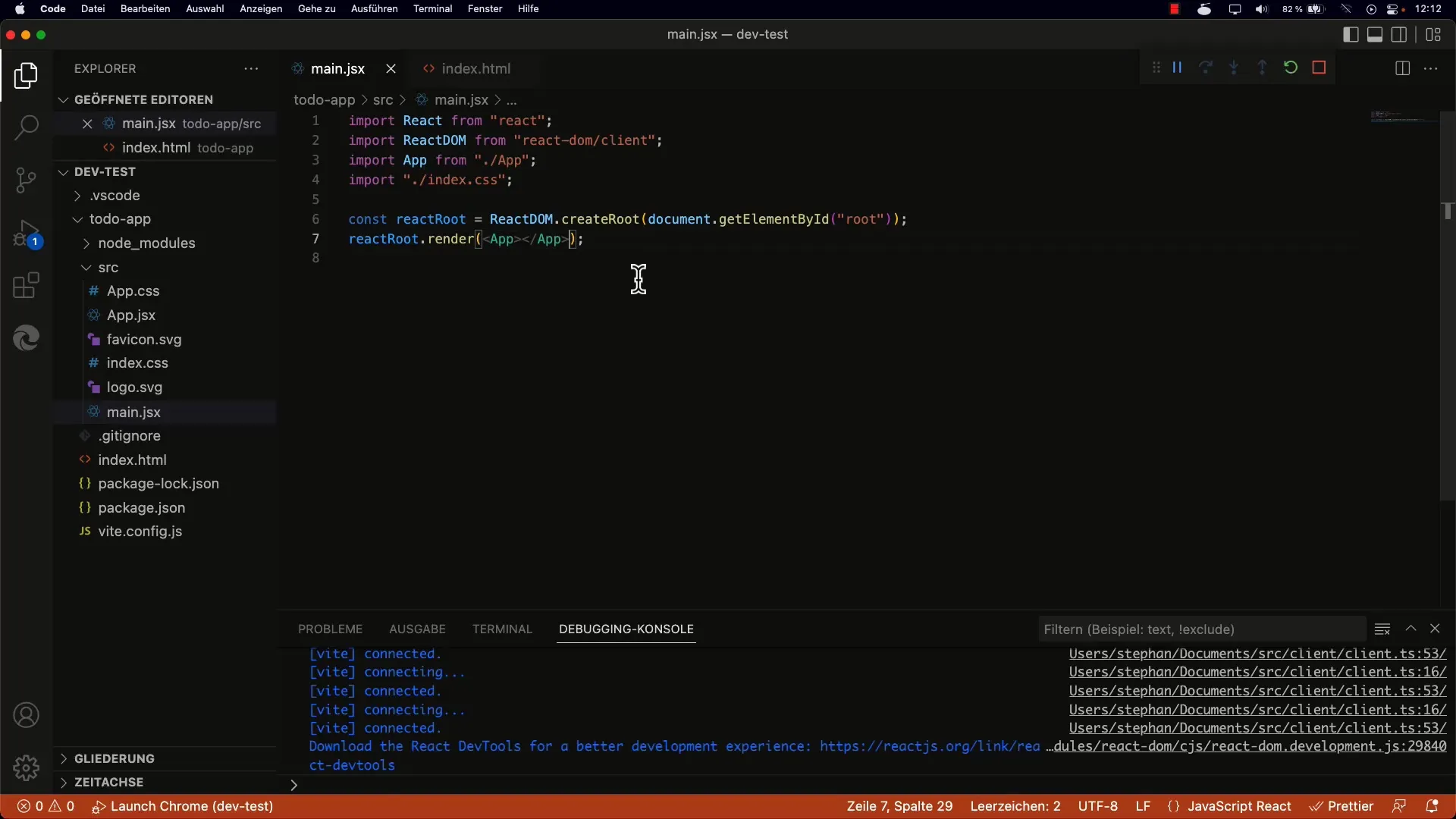
Summary
With the above steps, you have understood the basic structures and functions of a React app. You now know how to create the root node, use JSX, and import components correctly. This knowledge forms a solid foundation for your journey into the world of React development.
Frequently Asked Questions
What is the root node in a React app?The root node is the main DOM element where all React components are rendered.
What is JSX?JSX is a syntactic extension of JavaScript that enables you to use HTML-like syntax in React.
How do I import components into a React app?You can easily import components using the import command in your JavaScript file.
How do I manage rendering of components in React?This is done through the render method of ReactDOM, which allows you to render JSX or other React components for the root node.