JavaScript provides various methods for declaring variables, and each of these approaches has its own peculiarities. In this article, we will focus on the key differences between var, let, and const to help you make the right choice and avoid potential pitfalls.
Key Insights
The main differences between var, let, and const are:
- var has no block scope limitations.
- let limits the scope to the block in which it is defined.
- const is a specialized declaration for immutable values.
Step-by-Step Guide
1. Introduction to var
var is the oldest method for declaring variables in JavaScript. It allows you to declare variables globally or functionally, but the issue is that it does not consider block scope. This means a variable declared with var is visible beyond the curly braces in which it was created.
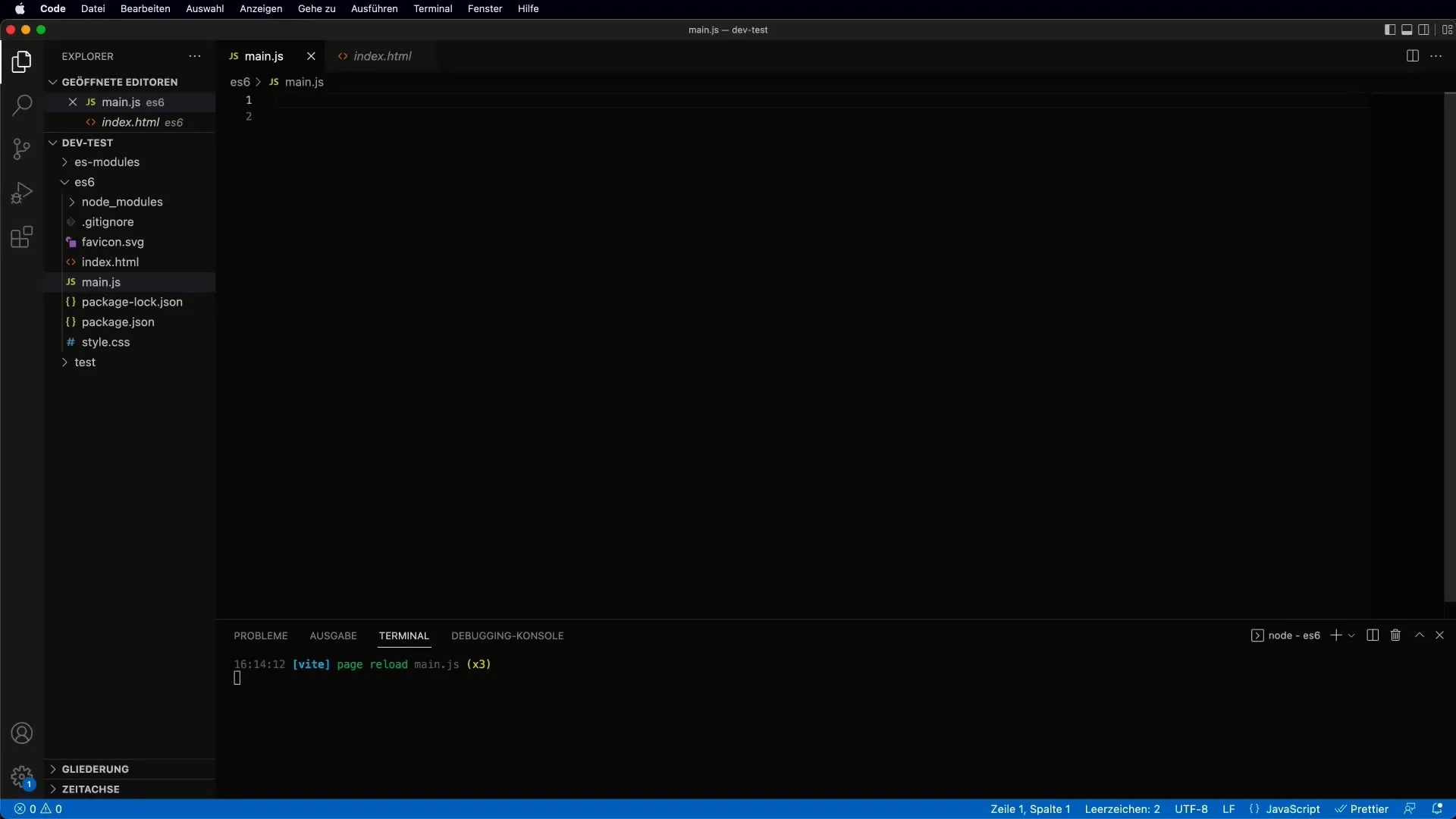
2. Example for var
For example, when you declare a variable W with a value of 0 and then access it, even if the access is before the declaration, the variable will not throw an error but will return undefined.
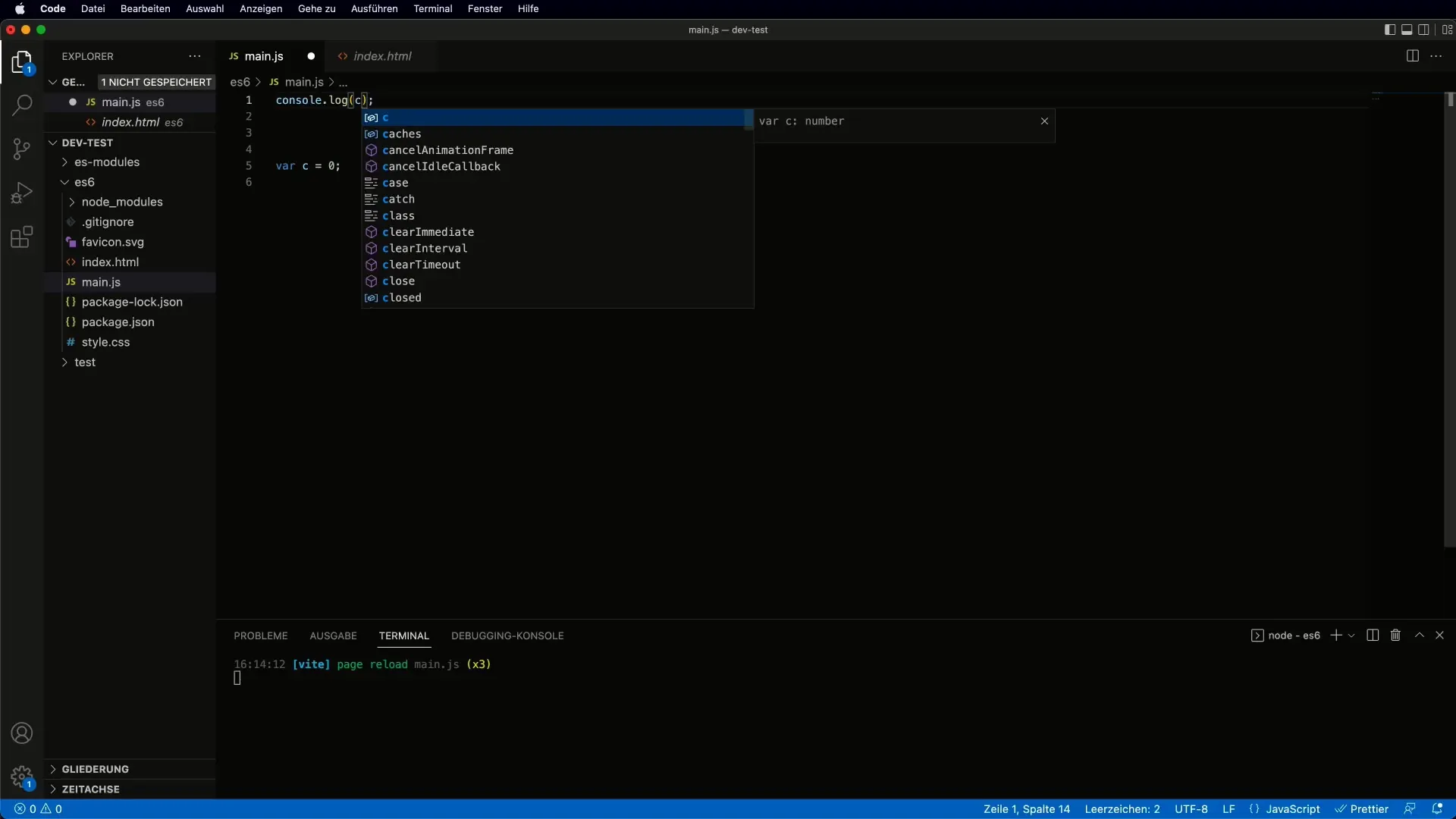
3. Introduction to let
The introduction of let provides a way to declare variables with block scope. This means you can only access let variables within the curly braces in which they are declared. If you attempt to access a let variable outside its block scope, you will receive a ReferenceError.
4. Example for let
If you define a let variable C in a block and then try to access it before it has been initialized, you will find that this is not allowed. The browser will throw an "uninitialized variable" error.
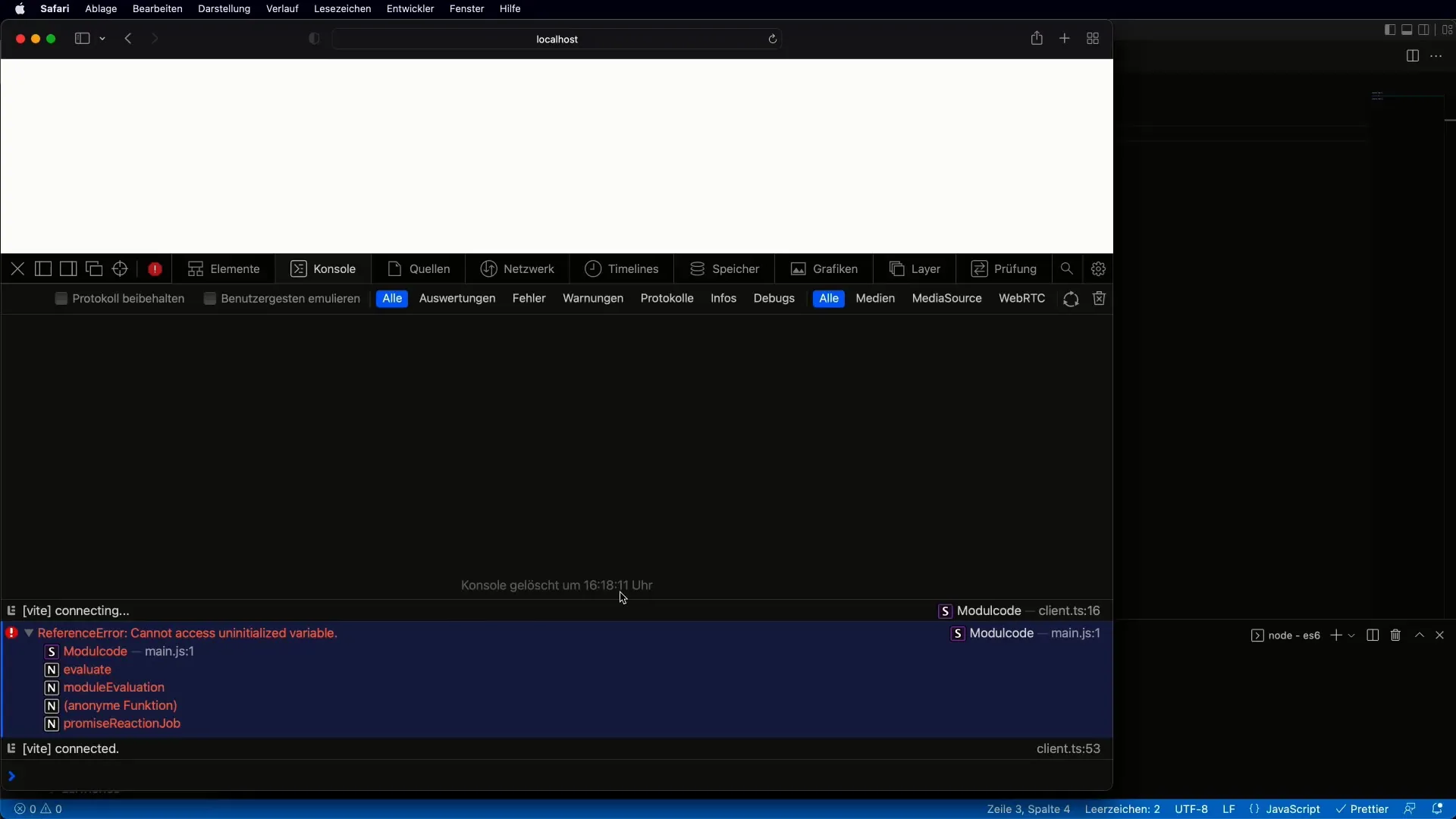
5. The Difference between let and var
The main difference between let and var is block scope. With let, you can define variables within a block without them being visible outside. This creates better encapsulation and prevents accidental overwrites and errors.
6. Introduction to const
const is used to declare constants, i.e., values that cannot be reassigned. This means you define a variable with const once and cannot assign a new value to it afterward. This is particularly useful for values that should not be changed, such as configurations or set values.
7. Example for const
If you try to assign a new value to a const variable, an error will be thrown. However, it is important to note that const only restricts the assignment of the variable, not the content if the variable is an object. Therefore, you can still change properties within the object.
8. Practical Use
In practice, it is advisable to use const by default unless you know you will need to change the variable later. This leads to clean and maintainable code. You should use let particularly for counter and loop variables.
9. Function Declaration and var
Another aspect of variable declaration is function declaration with var. Functions are hoisted, meaning you can call them before their actual definition. However, this can lead to confusion if you do not understand the logic.
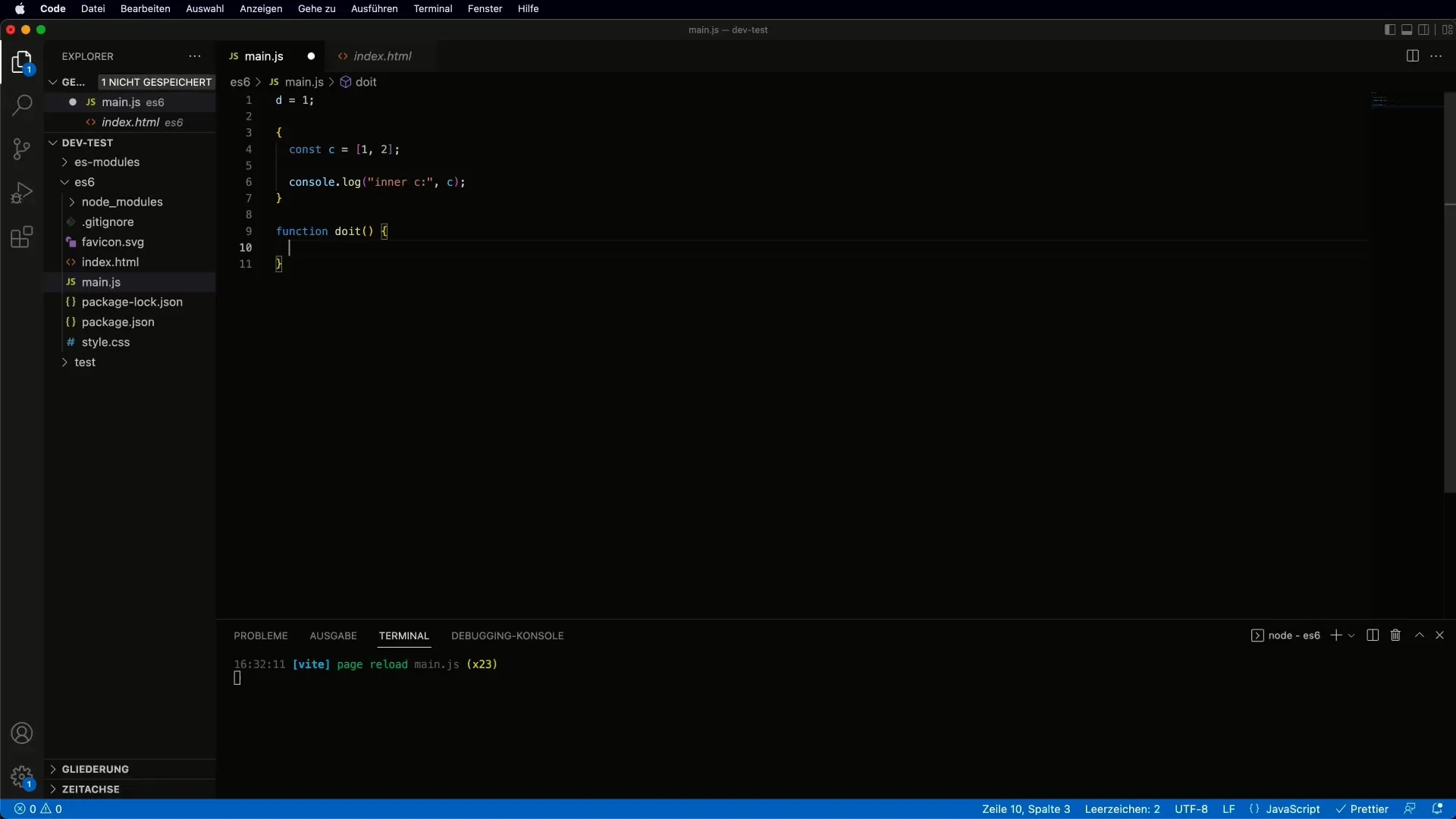
10. Conclusion
In conclusion, the choice between var, let, and const has an impact on the readability and stability of your code. let and const are superior to modern approaches, while var can often lead to unexpected behaviors.
Summary - Variable Declaration in JavaScript: A Guide
In this article, you discovered the differences between var, let, and const. This knowledge is crucial for consciously and effectively working with variables in JavaScript.
Frequently Asked Questions
What are the differences between let and var?let has block scope and is only visible within curly braces. var is hoisted and does not have block scope.
Can I change the values of const variables?No, you cannot change the value of a const variable. However, you can modify the properties of an object declared with const.
When should I use let or const?Use const when the value is immutable, and let when the value needs to be changed.
How does the hoisting effect work with var?Variables declared with var are "hoisted" up in the function, making them accessible even before their definition.
What are the disadvantages of using var?var can lead to issues when used in a block-based context as it does not have the expected block scope.