Creating components in React is an essential skill that I want to teach you more about here. You will learn how to create simple, function-based components and what things you should consider. Understanding components will greatly simplify the development of complex user interfaces for you. Let's get started right away!
Key Takeaways
- There are two main types of React components: class-based and function-based.
- Function-based components are simpler and less error-prone.
- JSX is used to describe and render the UI.
- Components should represent small, reusable parts of your application.
Step-by-Step Guide to Creating React Components
1. Component Basics
To create a React component, you need basic knowledge of JSX and the structure of a function in JavaScript. A component is actually just a function that returns JSX. Let's create a simple, function-based component.
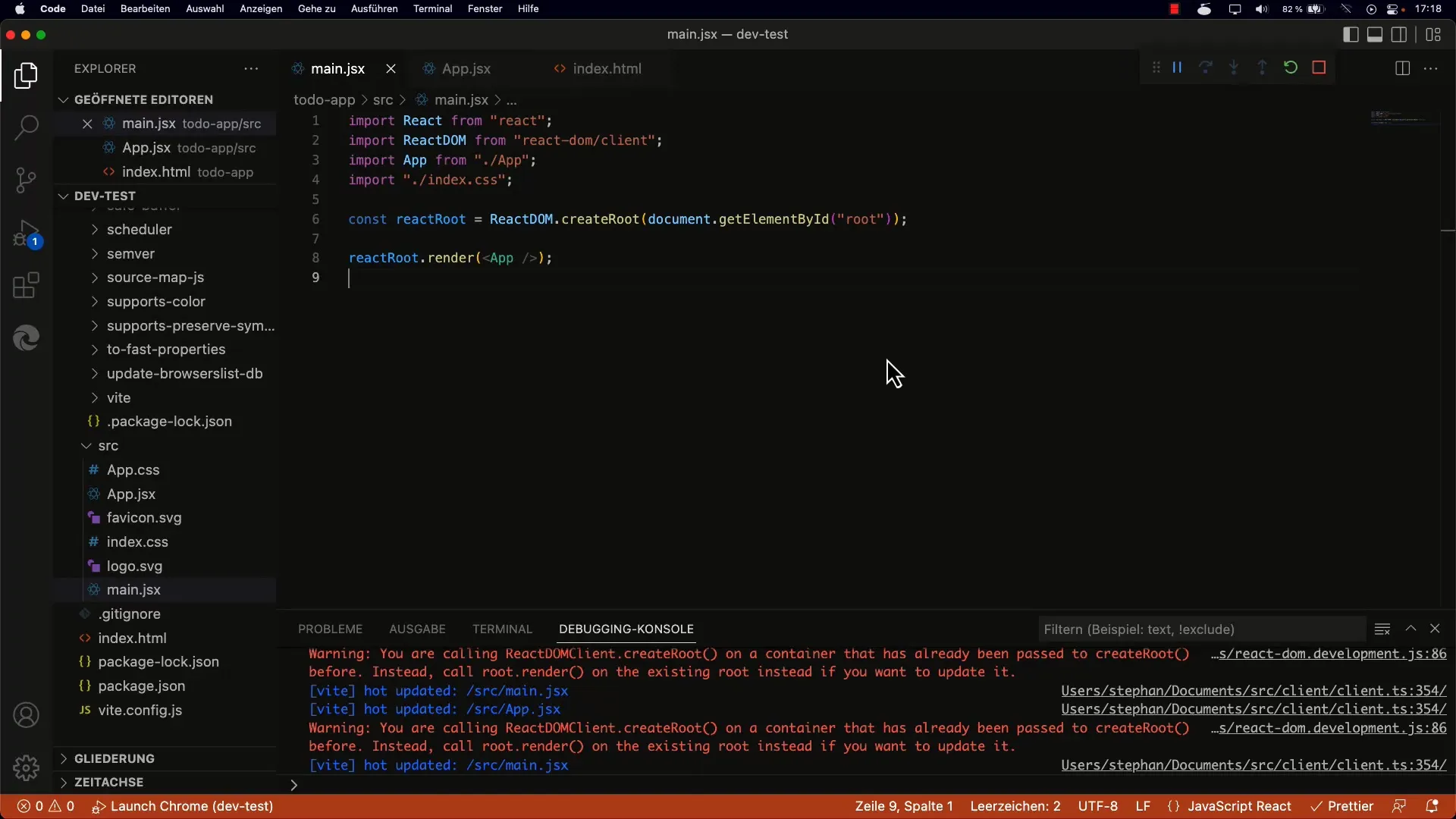
2. Component Definition
You can create a new component named Comp1. First, define this function in the main.jsx file. The function will initially be empty as it does not return anything yet.
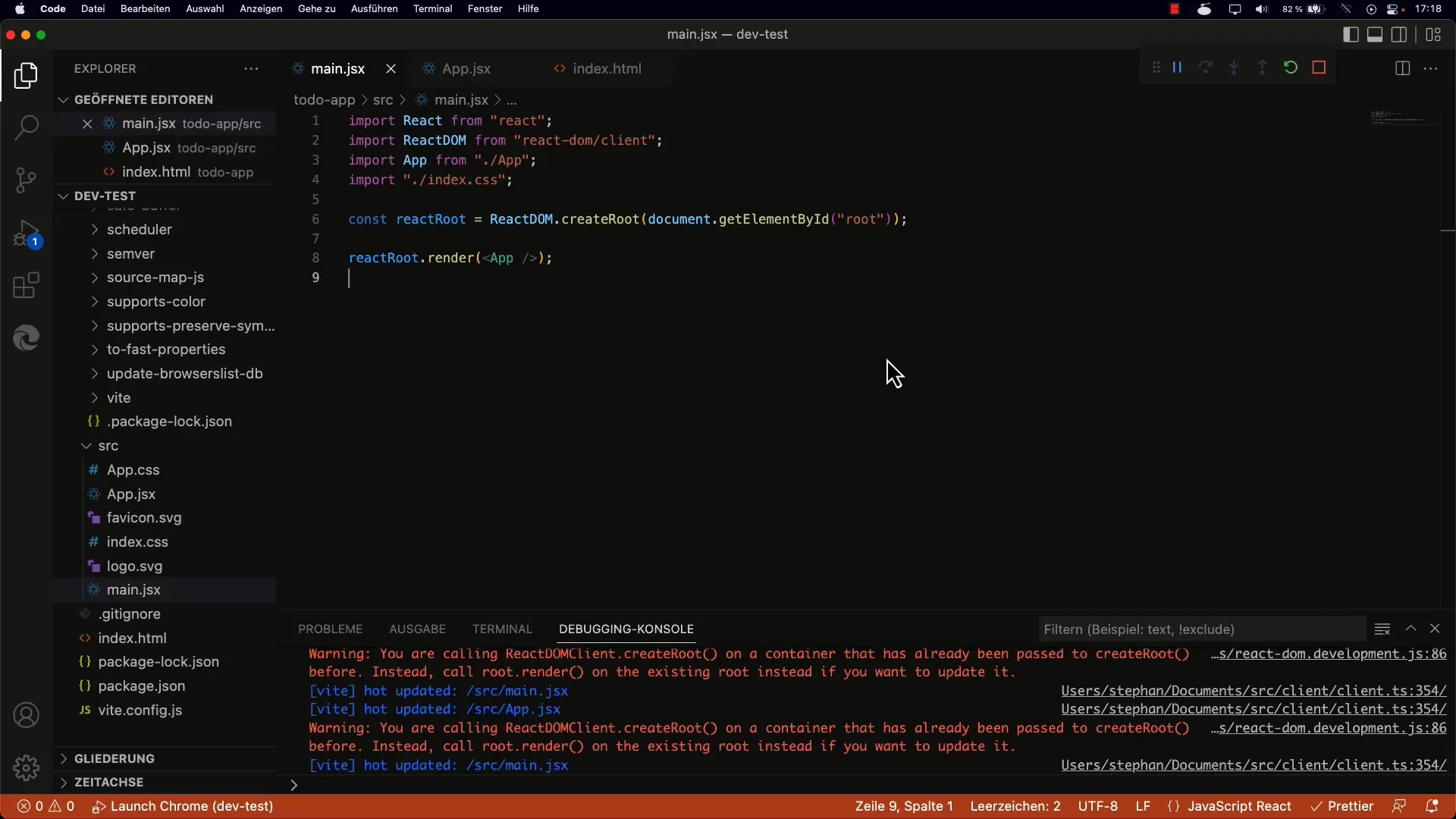
3. Using the Component
Now that you have defined a component, you need to decide where you want to use it. Instead of the existing App component, simply insert your new Comp1 component.
4. Component Return Value
A component should always have a return value. For example, you can return null, which means the component should not render anything and thus not create a DOM element. This is useful when you only have certain conditions under which something should be rendered.
5. Rendering Content
To return something visible in your component, you can use JSX.
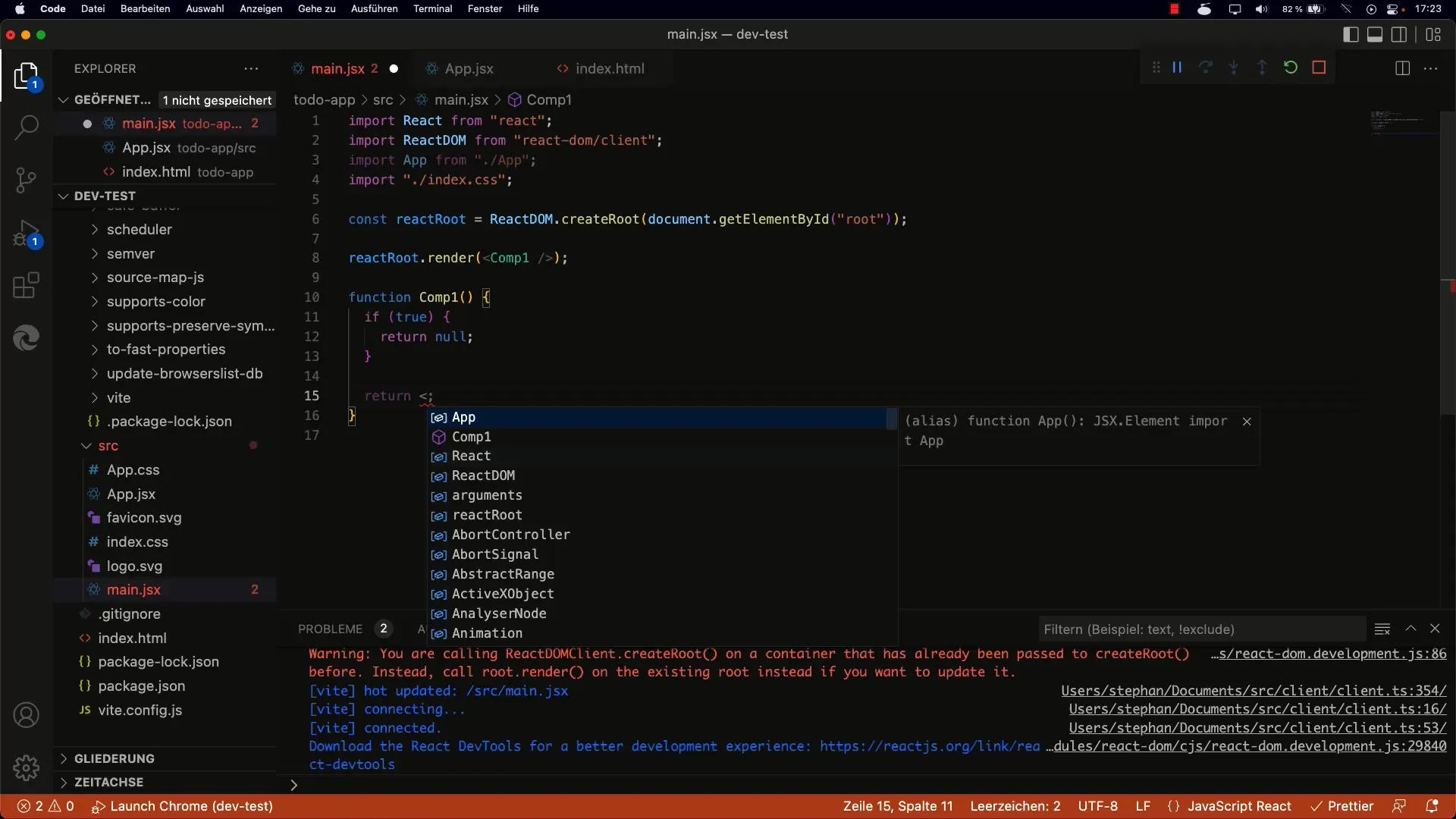
6. Troubleshooting by Reloading
If you encounter errors while testing the component, it can be helpful to reload the page to ensure that all changes are correctly applied. This is especially relevant when working with Hot Module Reloading.
7. Clean Code and Naming Conventions
When defining your functions, it is common practice to capitalize the first letter. This helps you differentiate between standard HTML elements and components you create.
8. Moving the Component to a Separate File
To improve the structure of your code, you should move the Comp1 component to a new file named Comp1.jsx. This makes your code more organized and prevents potential errors from multiple roots.
9. Importing the Component
After creating your new file, import the Comp1 component in your main.jsx. Make sure to name the component correctly to avoid confusion.
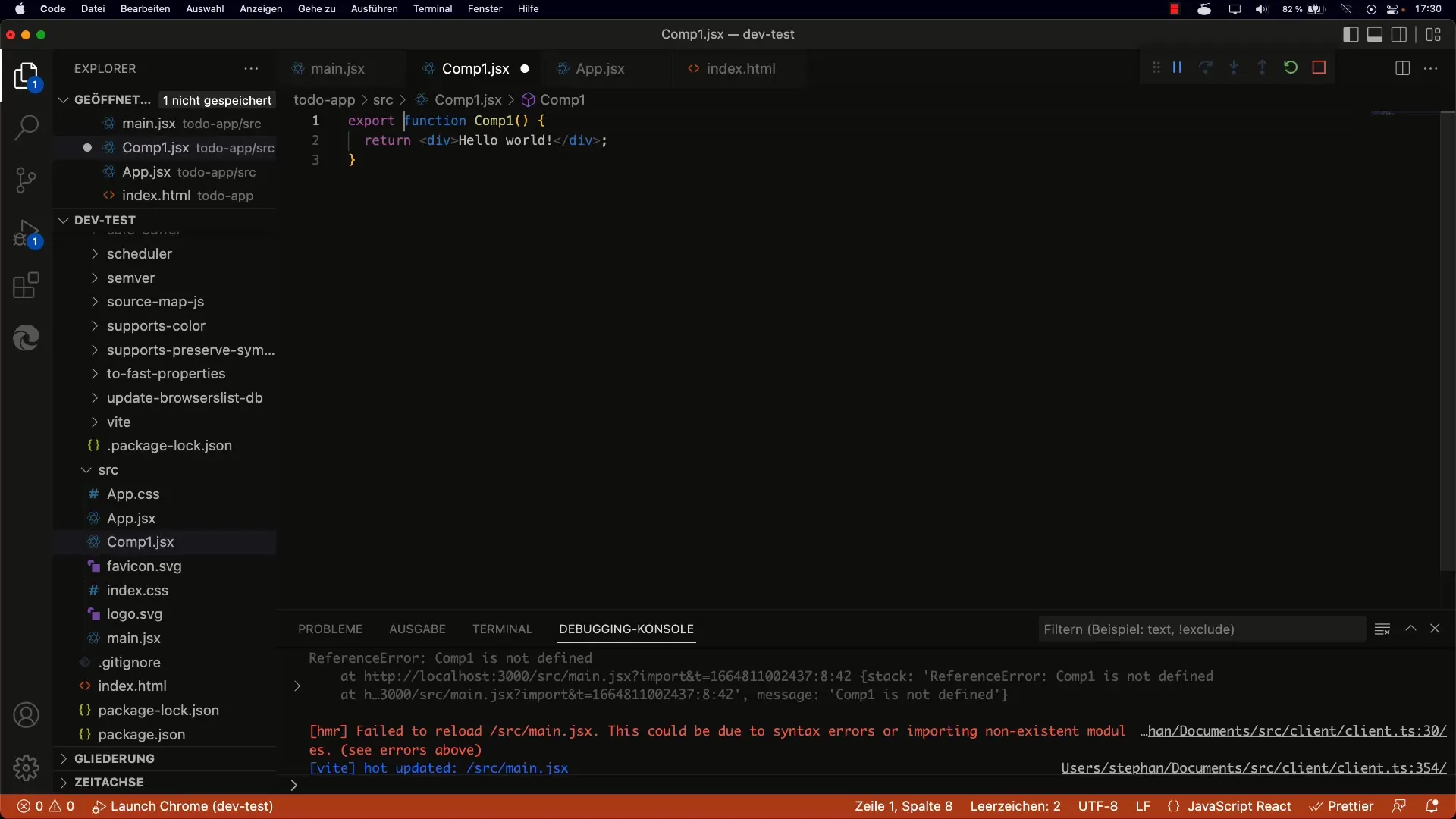
10. Using the Imported Component
Now you can use the imported Comp1 component in your main.jsx. Regardless of the changes you make to Comp1, the app should be rendered correctly without errors.
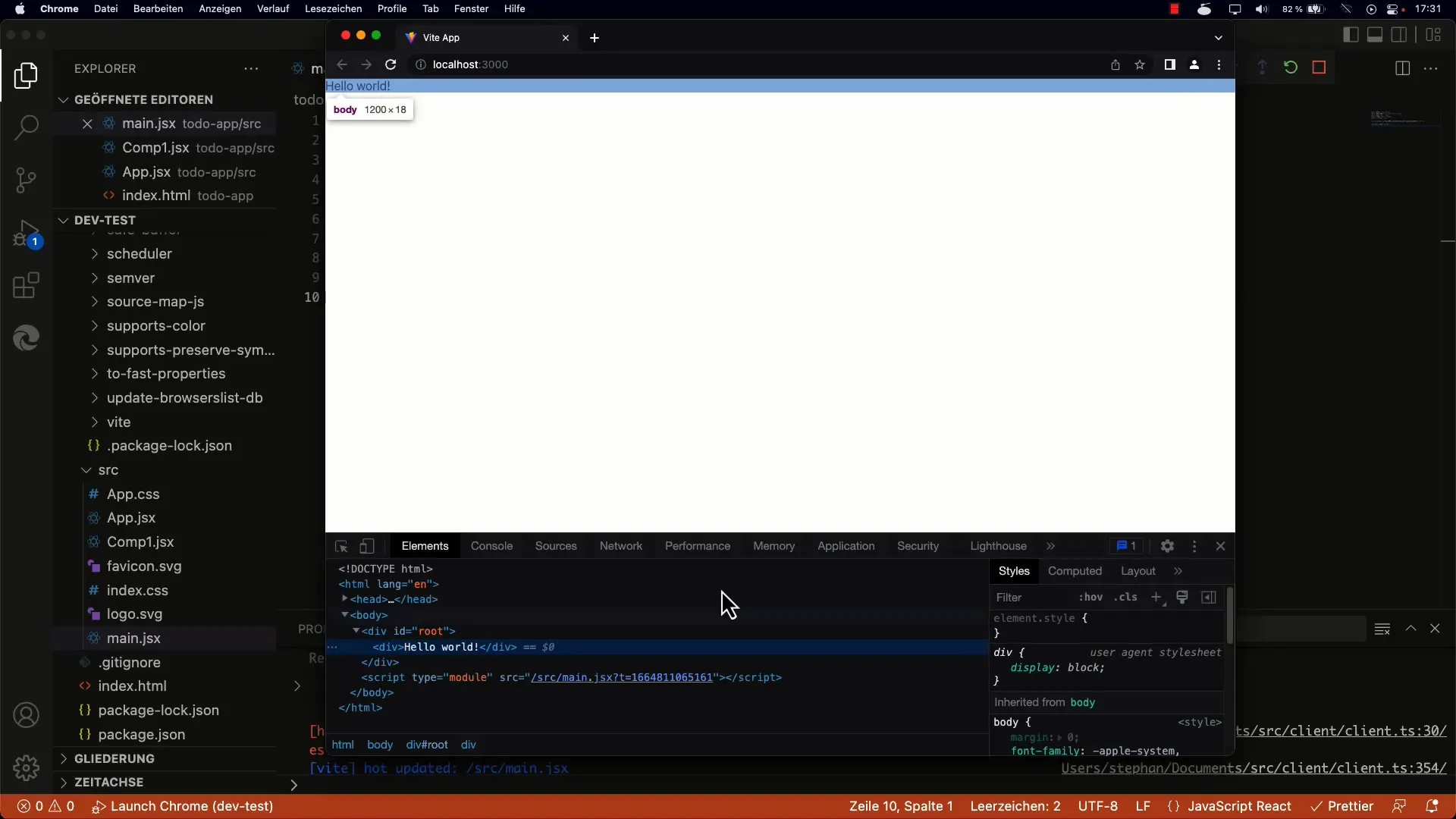
11. State Handling Definition with Hooks
To make components interactive, you need to understand how to use state with hooks. The next exciting feature is the State Hook useState, which helps you manage state in functional components.
Summary
You have now explored the basics of creating and using functional React components. Using JSX and understanding the structure of components are crucial for your development. Ensure a good structure for your code by moving components to separate files. Remember that using hooks is an important part of React development, especially if you want to work with state.
Frequently Asked Questions
How do I create a React component?You create a React component by defining a function that returns JSX.
What are the differences between class-based and function-based components?Function-based components are simpler and require less boilerplate code compared to class-based components.
How do I return nothing from my component?By returning null, no DOM element will be rendered.
What does JSX mean?JSX is a syntax extension for JavaScript that allows writing HTML-like syntax within JavaScript.
Why should I move components to separate files?This improves the clarity of your code and reduces the risk of errors, such as multiple roots.