Working with React quickly shows how important handling Props is, especially when it comes to considering user-specific inputs. In this tutorial, you will learn how to implement dynamic Props in React and work with input fields to capture user input values. The focus is on the connection between input fields and interactively setting Props, so that you will be able to integrate user-entered values into your application.
Key Takeaways
- Dynamic Props change based on user inputs.
- Input fields need to be updated to effectively reflect changes.
- Managing state in React is crucial for the smooth operation of your component.
Step-by-Step Guide
1. Planning and Setting Up the Project
Begin by creating a new React component that will contain a counter and an input field. You will need to use the useState Hook to manage the state of the counter and the input value. Make sure you have installed all the necessary dependencies.
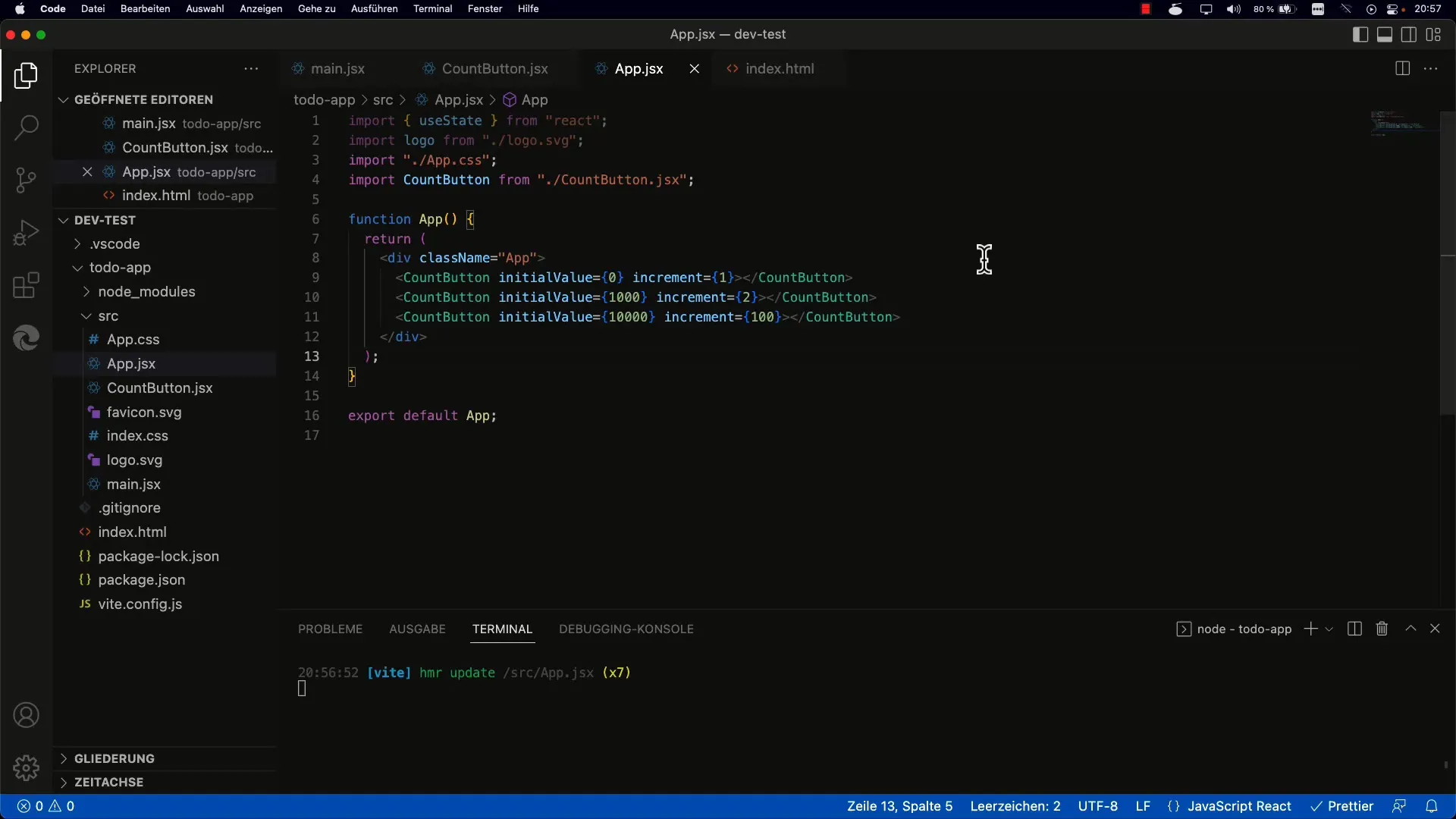
2. Creating the Input Field
In this phase, you will create an input field of type "number". This component will allow the user to specify an increment value. To handle the input, add an onChange handler. This handler ensures that the user's input is registered.
3. Implementing the onChange Handler
The onChange handler is defined to convert the user's inputs into a state. This handler provides you with an event that allows you to extract the current value of the input field. Make sure to convert this value to a number, as it is initially in string format.
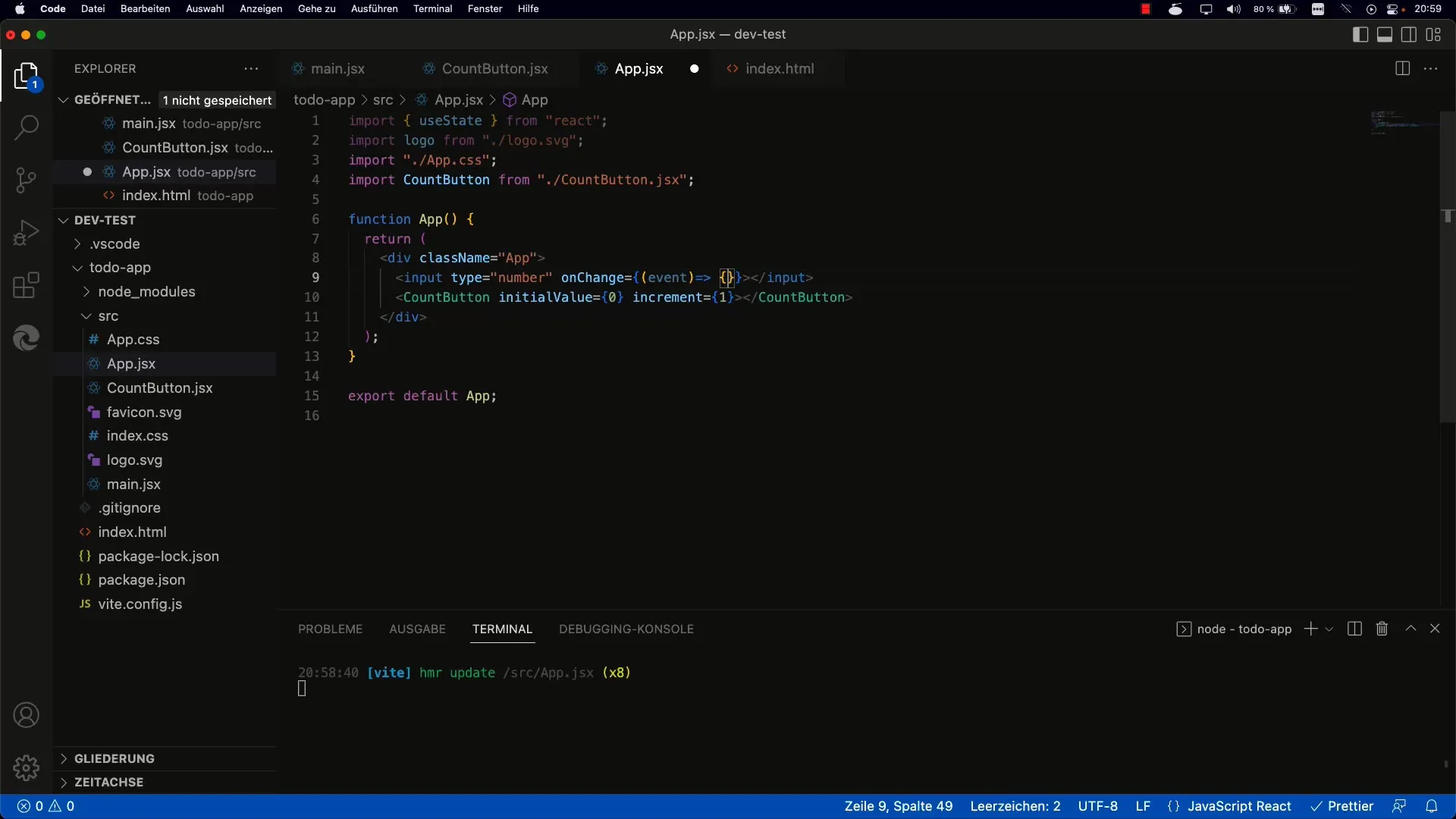
4. Using the useState Hooks
Now is the time to use the state for the increment value. With useState, you define a variable that stores the increment value and is updated with a setter function. The default value can be set to one to ensure that the input field always has a designated initial value.
5. Binding the Increment Value to the Render Function
After setting the state, you now need to update the current increment using the setter function. Replace the log output in the onChange handler with a call to set the value. This change ensures that the counter rendered by the component correctly responds to the new increment.
6. Setting the value Attribute in the Input Field
To ensure the value in the input field is displayed correctly, you need to set the value attribute to reflect the current state. This means setting the attribute to the value of increment. This ensures that the counter always displays the entered increment value.
7. Avoiding Uncontrolled Components
A common challenge is keeping a component's state consistent. If the input field's value is undefined, this can lead to warnings in React. Ensure that the value state is always defined to avoid issues with uncontrolled input fields.
8. Testing the Input Processing
Finally, perform some tests to verify that everything is working correctly. Enter different values into your input field and observe if the counter increments the values accordingly. Also, check if any warnings are output in the console and if the input field behaves as expected.
Summary
In this tutorial, you have learned how to implement dynamic Props in React by using input fields to capture user values efficiently and bind them to your components. You have also learned the importance of actively managing state and ensuring that inputs are processed correctly. With this knowledge, you are well-equipped to create interactive React components.
Frequently Asked Questions
How do I handle uncontrolled input fields in React?Ensure that the value of the value attribute is always defined to avoid warnings.
Can I also use text inputs with the same approach?Yes, you can change the type of the input field to "text" and maintain the same principles.
How can I change the initial value of the input?Set the initial value in useState to the desired starting value, e.g., 0 or 1.