If you have already created a simple To-do-App in React, you may be wondering how to mark tasks as completed and display them accordingly. In this guide, you will learn step by step how to integrate checkboxes into your to-do list to tick off tasks and strike through the associated text. Let's get started!
Key Takeaways
Integrating checkboxes into your to-do app provides users with the ability to manage tasks and recognize their status. You will learn how to use checkboxes to modify the state of to-dos and display text accordingly.
Step-by-Step Guide
Add Checkbox
In the first step, you add a checkbox to each of your individual to-do elements. To do this, use the HTML element with the type checkbox.
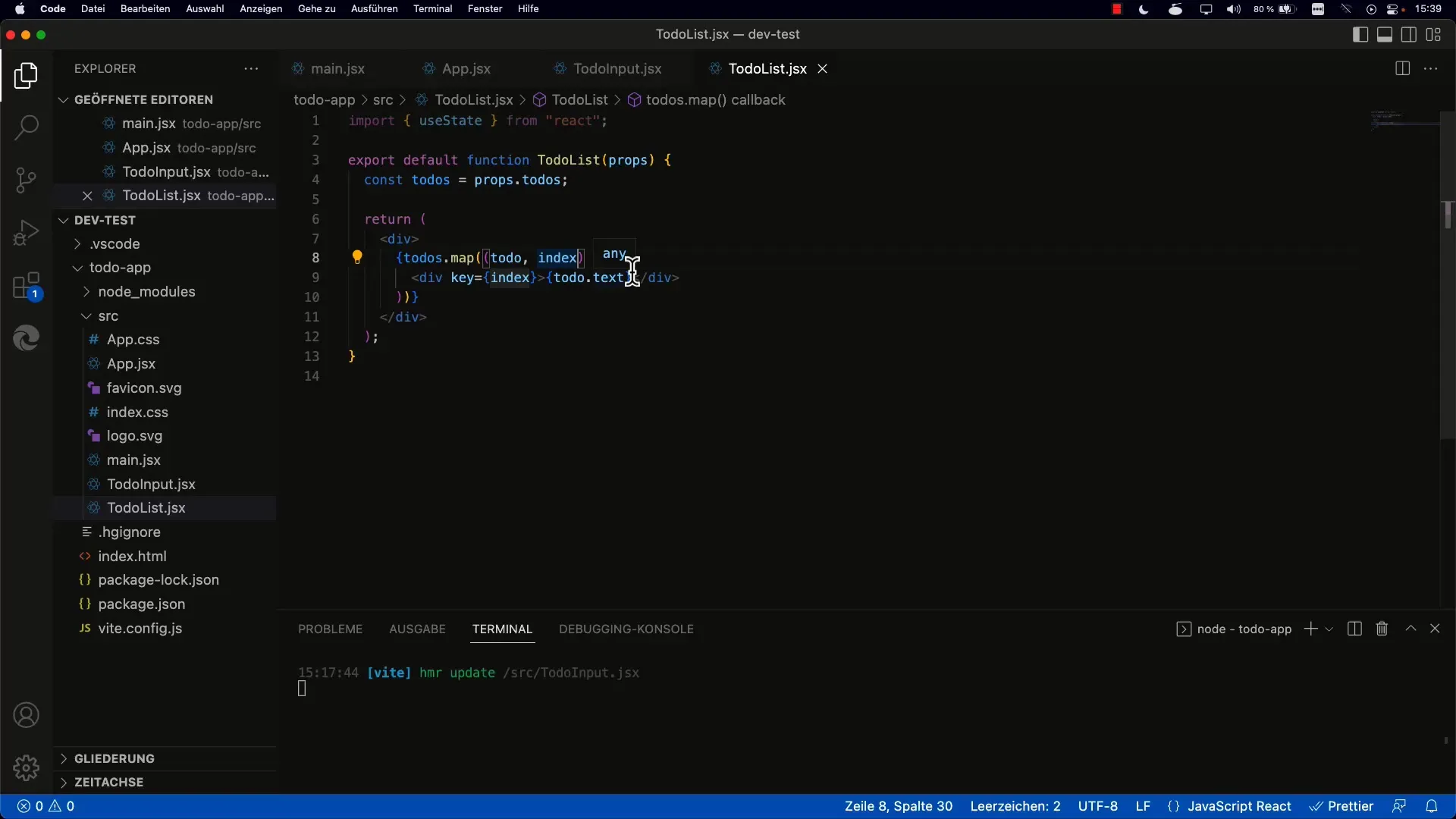
Now you can check if the checkbox works by clicking on it. However, nothing happens yet – the functionality still needs to be implemented.
Handling the onChange Event
To react to changes in the checkbox, you need to use the onChange event. In your checkbox implementation, you can specify the event handler and access the checked property via the event parameter.
Remember to use checked here and not value. With this info, you can query the status of the checkbox.
State Management
The next step is to update the state of your to-do element depending on whether the checkbox is checked or not. Here you need to ensure that you inform the parent component so that the state is managed correctly.
This means you need a function to pass to the checkbox so you can adjust the status. This function is called by the onChange event.
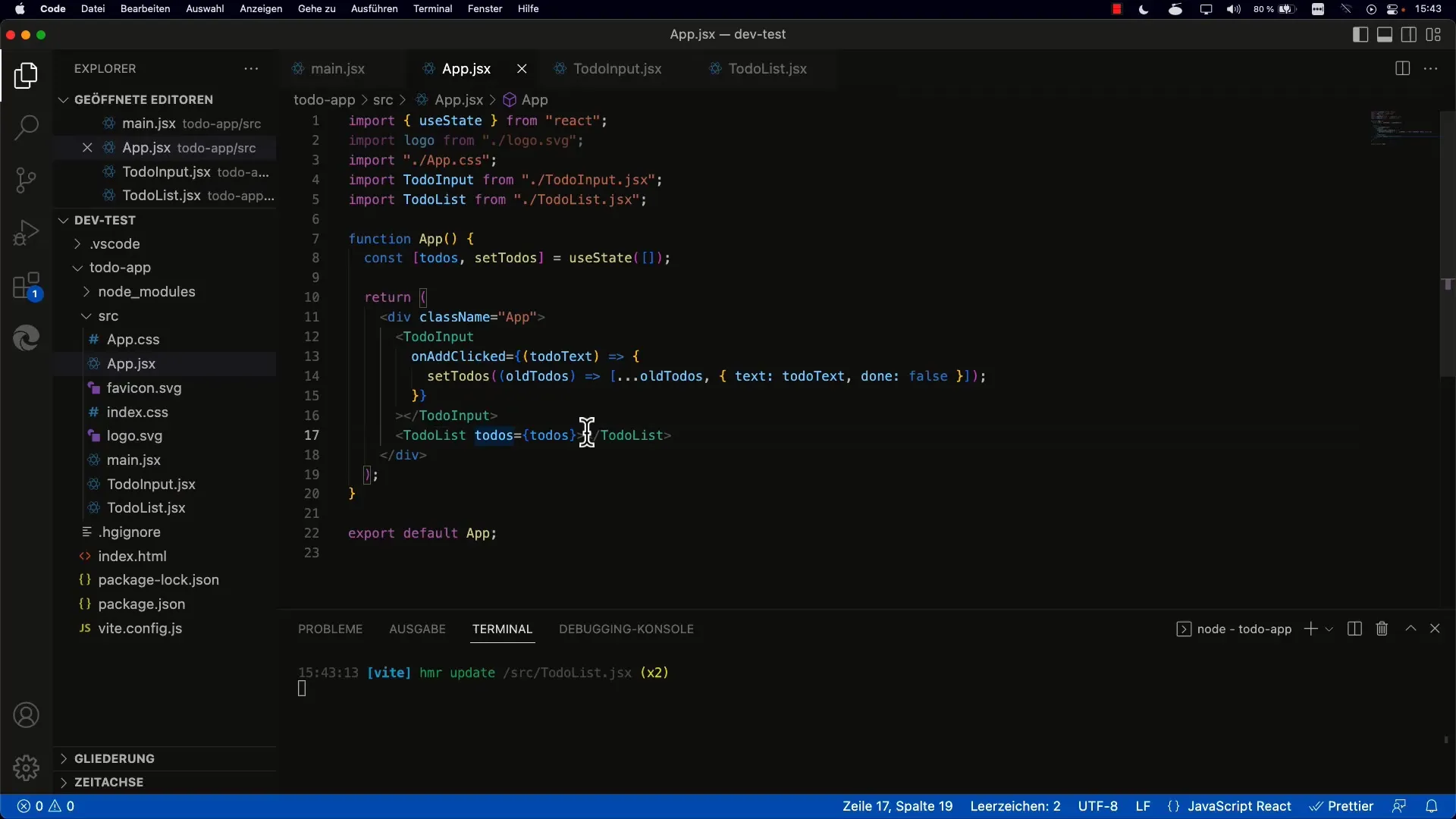
Styling Completed Tasks
Now that your checkbox is functional, let's make sure that the text of the to-do elements is struck through when they are marked as completed. This is where CSS comes into play.
You can use the CSS property text-decoration: line-through; for this. It should only be applied when the to-do elements are marked as completed.
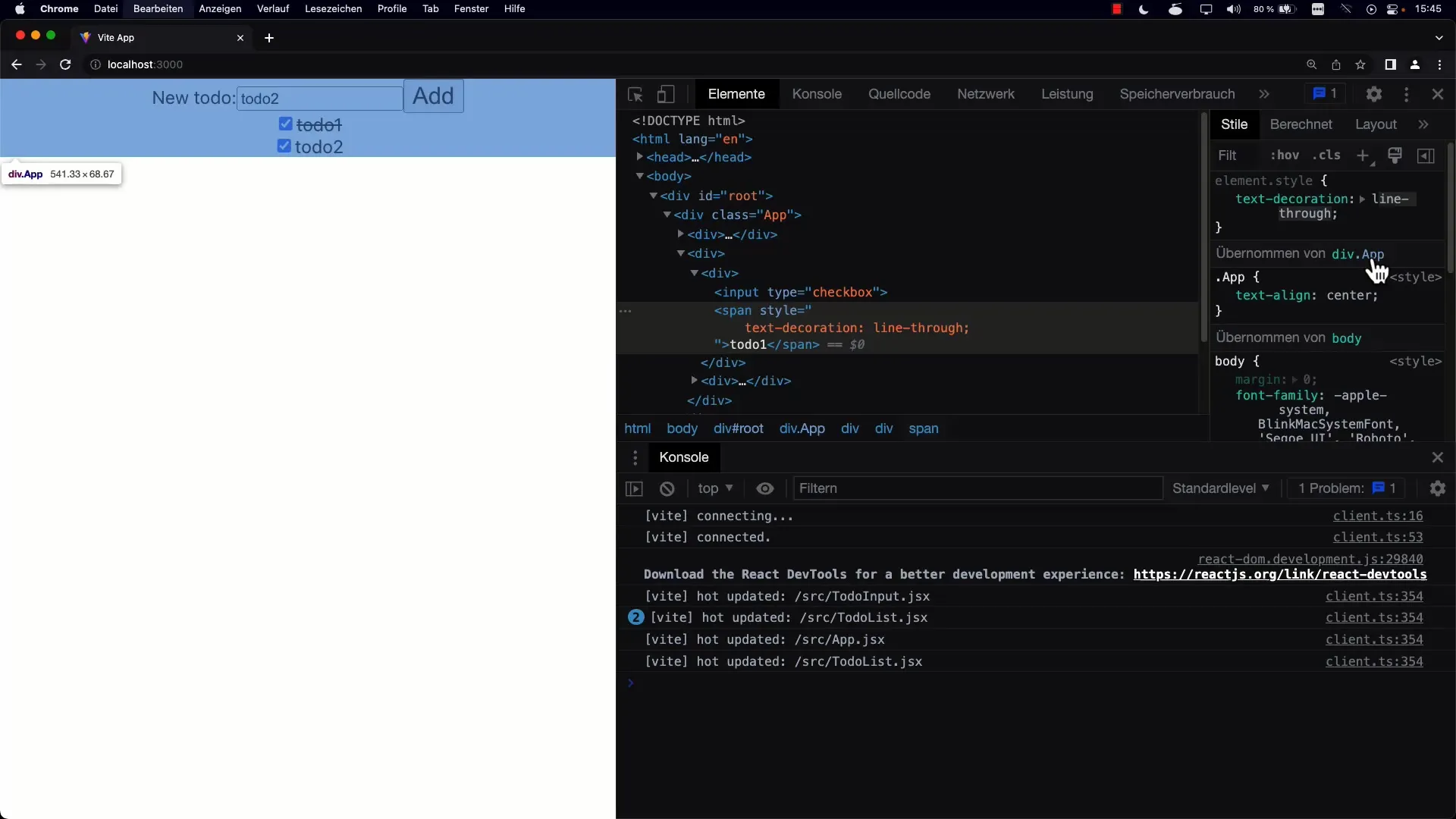
If the to-do is not completed, you can simply maintain the default style.
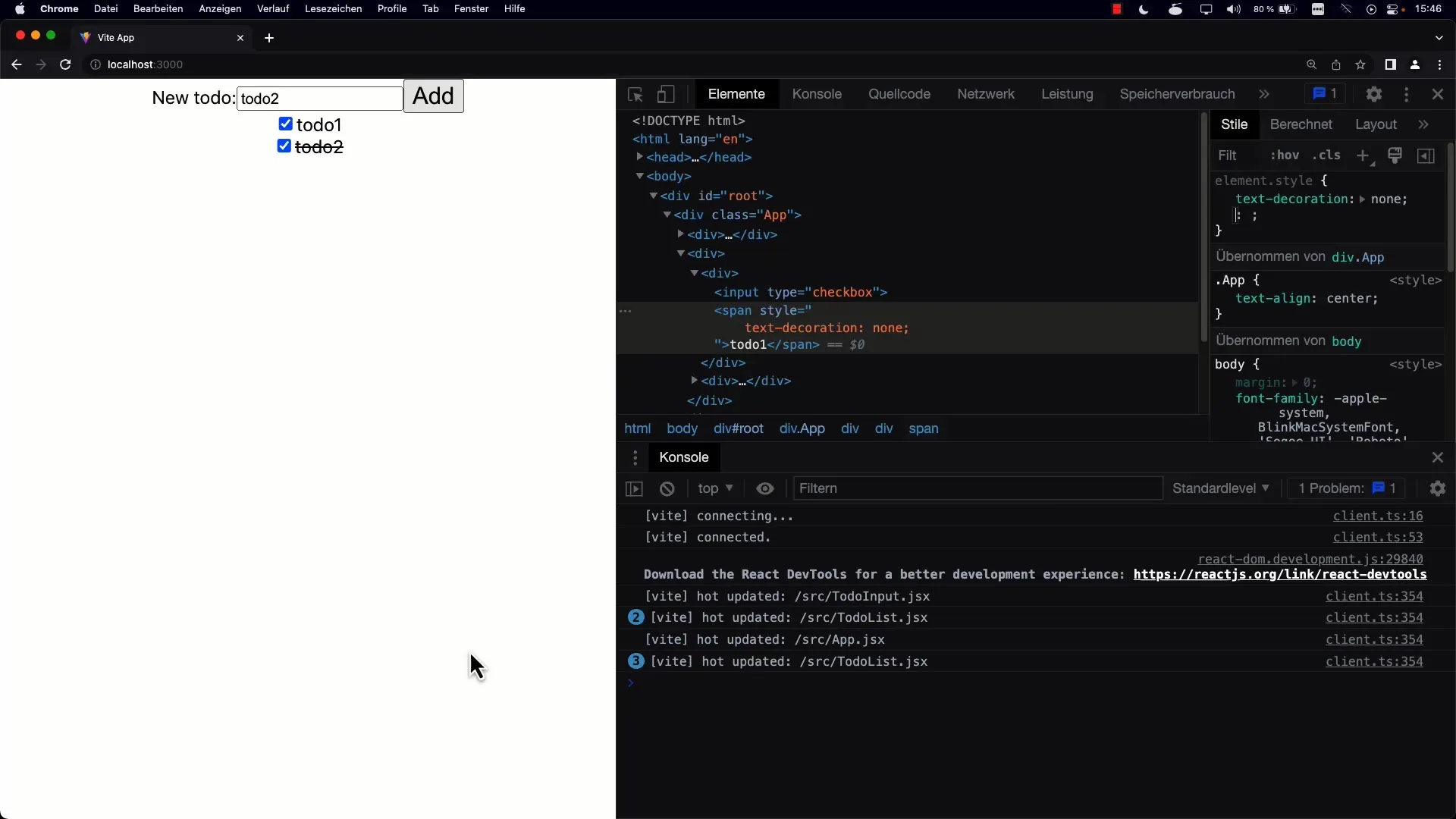
Toggle Implementation
Now you need to implement the to-do elements to retain their state when the page is refreshed. For this, you will use React State Management. You will obtain the current state of the to-dos when the checkbox is checked and update the state of the to-do list.
It is important to make a copy of the existing to-dos and then only modify the status of the respective to-do. You can achieve this by using map to create a new array while leaving the other to-dos unchanged.
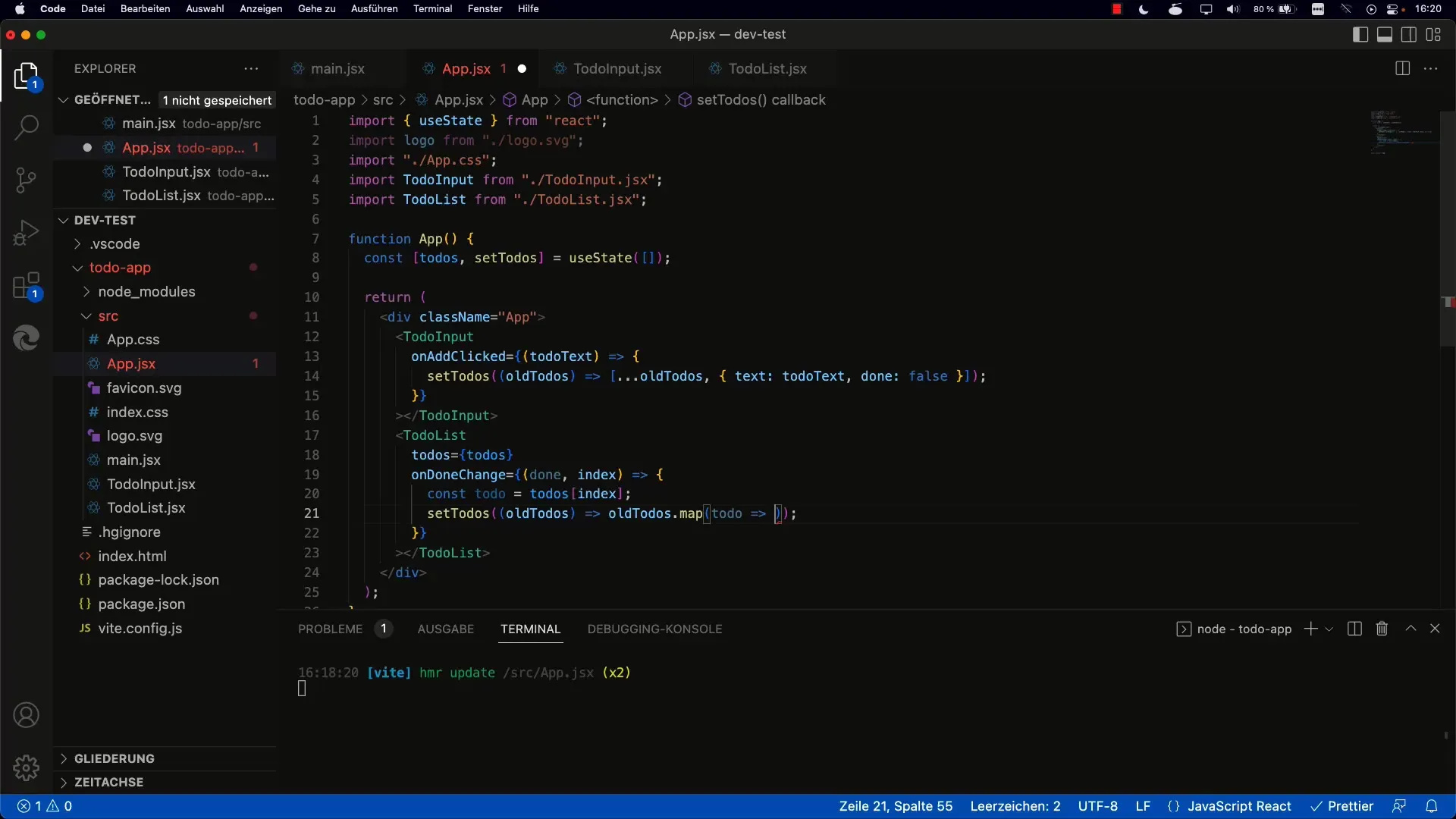
Final Test and Improvements
Once everything is implemented correctly, you can test the application in the browser. You should be able to tick off tasks and see the text change accordingly. Experiment with different to-dos and verify that everything works as desired.
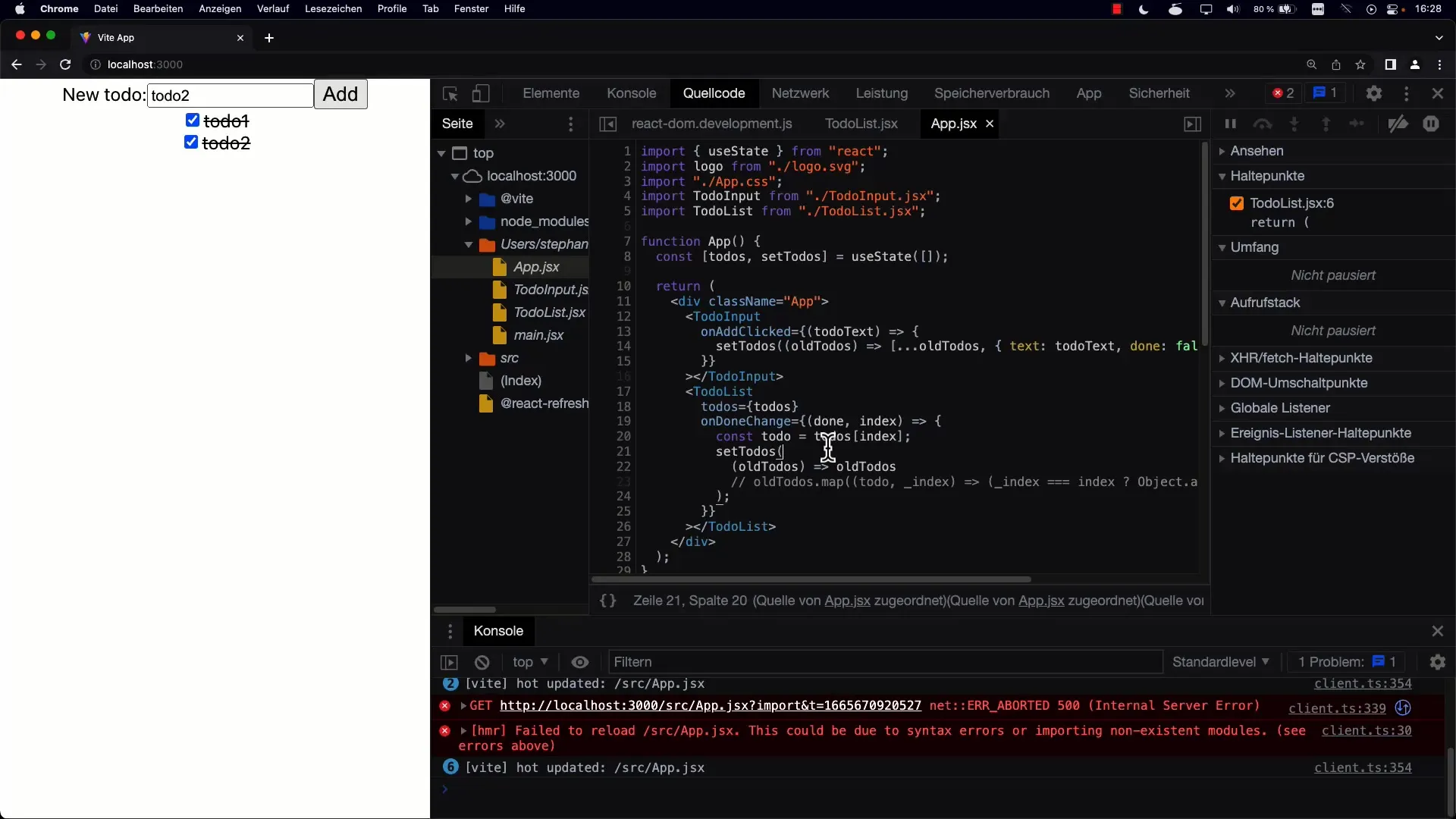
You can also consider improving the styling of your app and possibly implementing additional features, such as deleting completed to-dos or sorting between completed and uncompleted tasks.
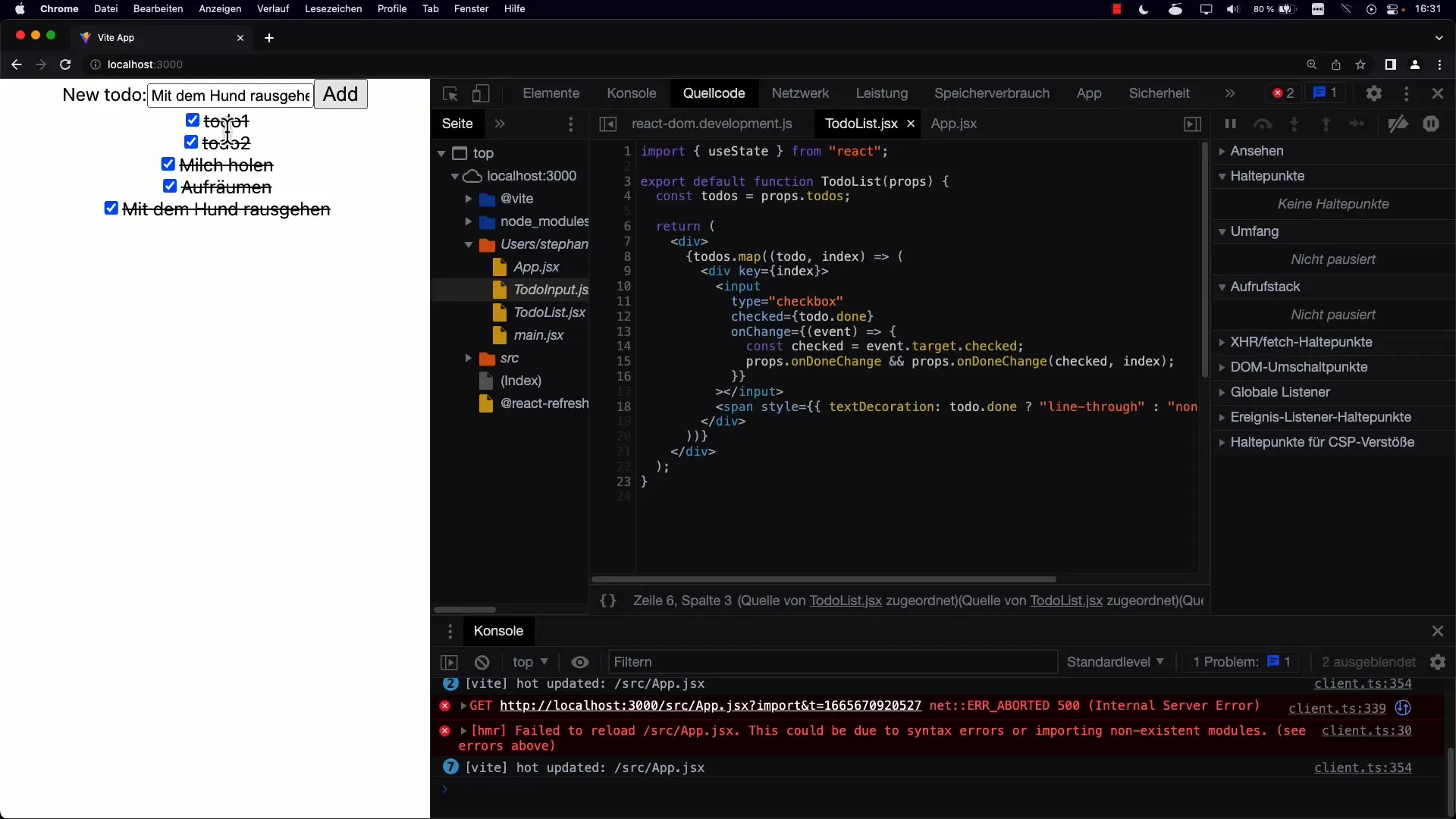
Summary
You have learned how to integrate checkboxes into your to-do app to mark tasks as completed. In doing so, you have made progress in dealing with React's state management, adjusted the styling of text display, and significantly improved interaction with users.
Frequently Asked Questions
How do I implement the checkbox in my to-do app?You can add a element in your to-do item component.
What do I do with the task state?Use the onChange event to retrieve the state of the checkbox and change the state of the to-do accordingly.
How can I display the text of completed tasks?Use CSS with the property text-decoration: line-through to strike through the text when the task is marked as completed.
Can I further improve the to-do list?Yes! You can add functionalities such as deleting and sorting tasks to optimize the user experience.