Organizing to-dos is a fundamental skill for managing tasks, both in everyday life and in software development. In this tutorial, you will learn how to create a React App that groups to-dos into two categories: not done and done. This allows you to have a clear view of your to-dos and helps you effectively manage completed tasks.
Key Takeaways
- Filtering and grouping to-dos in React is a simple yet effective method of task management.
- It is important to assign a unique ID to each to-do to avoid identification issues.
- Splitting the lists enables an improved user experience by clearly separating past and current tasks.
Step 1: Component Structure
First, you need to ensure that you have the basic structure of your to-do list in a React component. The idea is to group the to-dos into two different sections. You start with the not done to-dos, followed by the done ones.
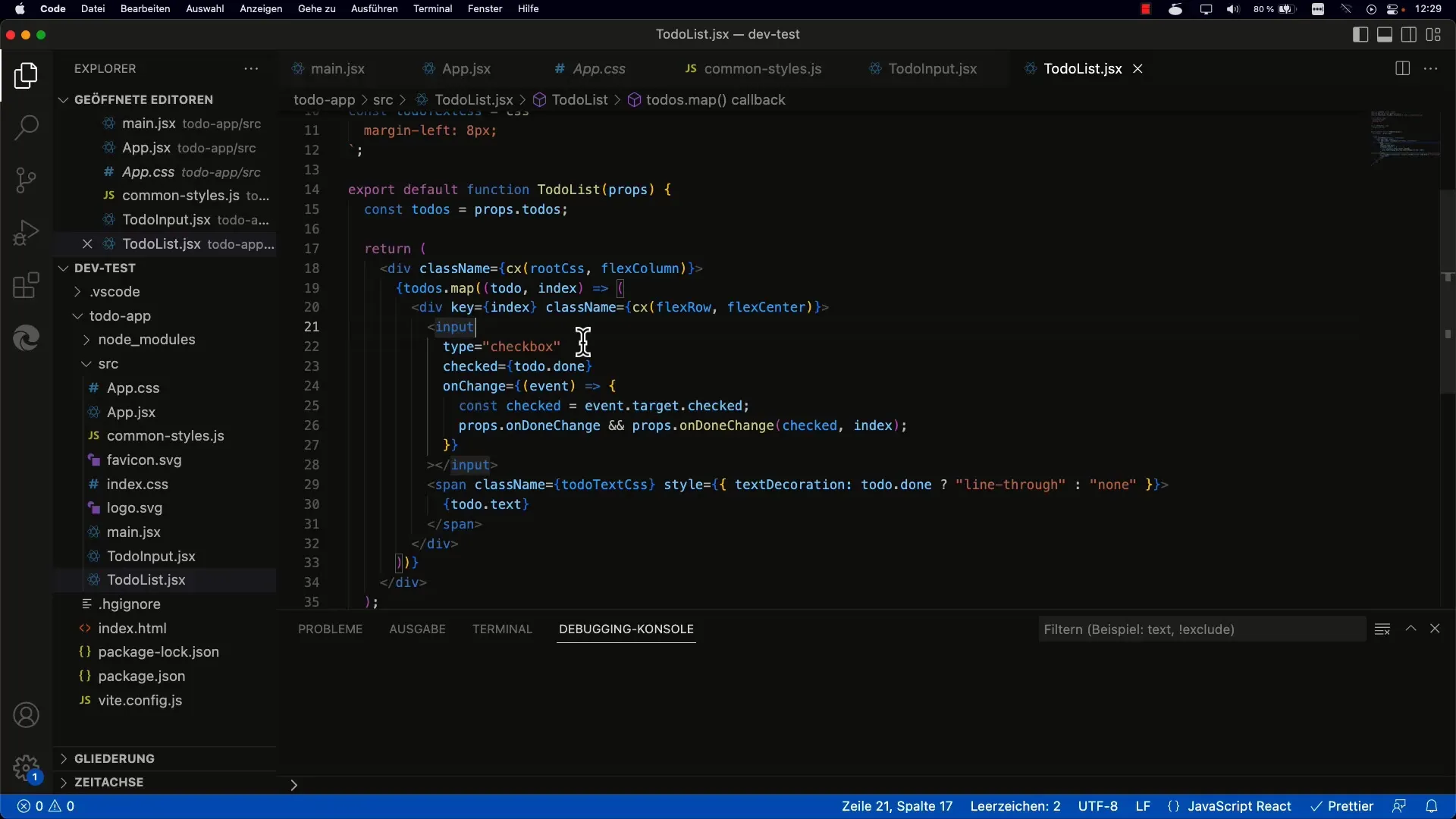
To achieve this, you can use two separate map functions within your component. This allows you to split the to-dos in the user interface.
Step 2: Filtering the To-dos
Filtering to-dos is done using the filter method. You specify that you only want to have to-dos that have the status "not done." This is done by checking if the done property is set to false in the filter call.
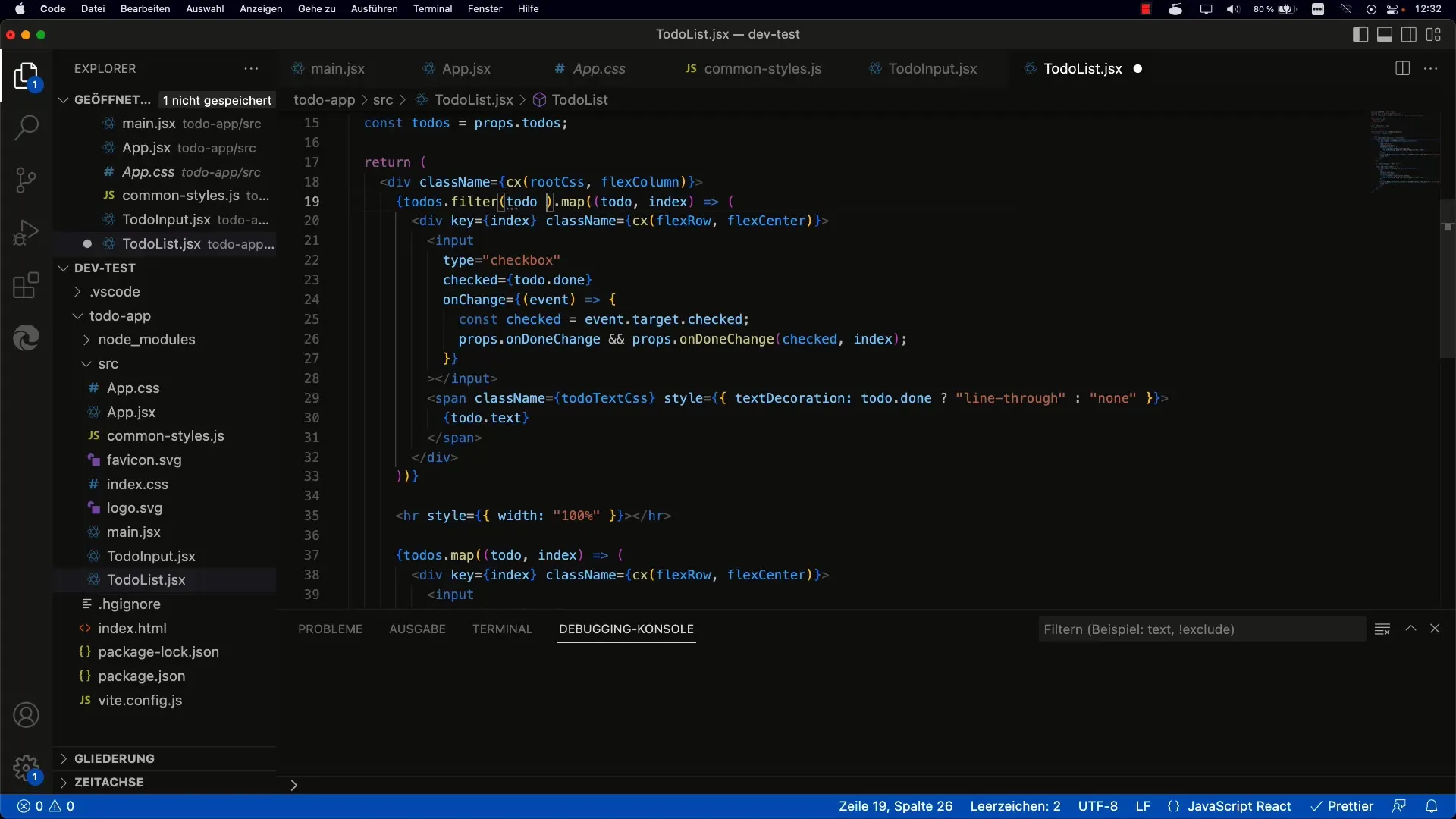
You must apply the same technique for the done to-dos as well. Here, you specify that you only want the to-dos where done is set to true.
Step 3: Identifying To-dos with Unique IDs
A common pitfall is using the array index as the key for the to-dos. This is not recommended as indexes can change when the array is filtered. Instead, make sure each to-do has a unique ID.
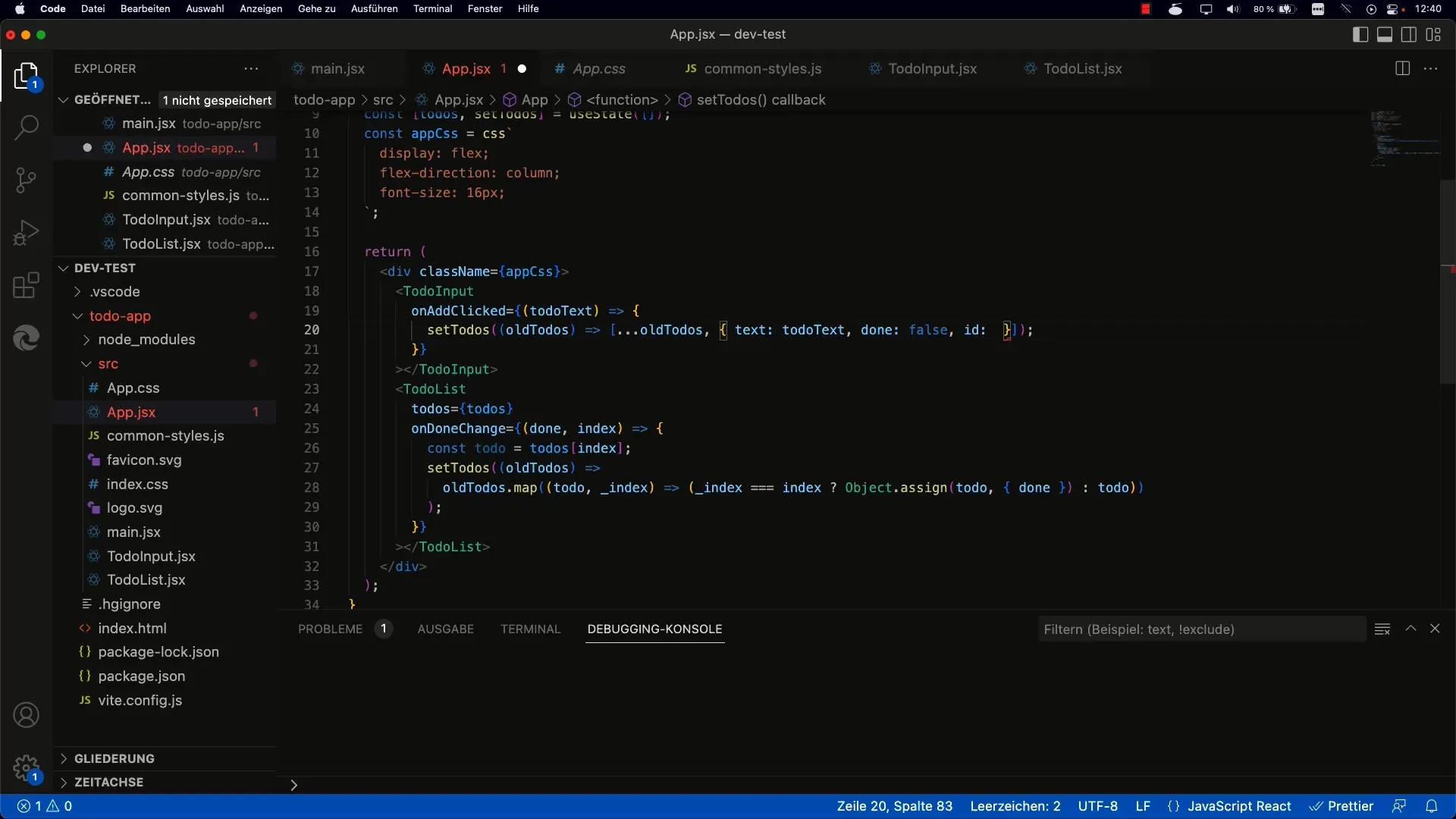
When creating a new to-do, use a method to generate a unique ID, such as Date.now() or a combination of a timestamp and a random number.
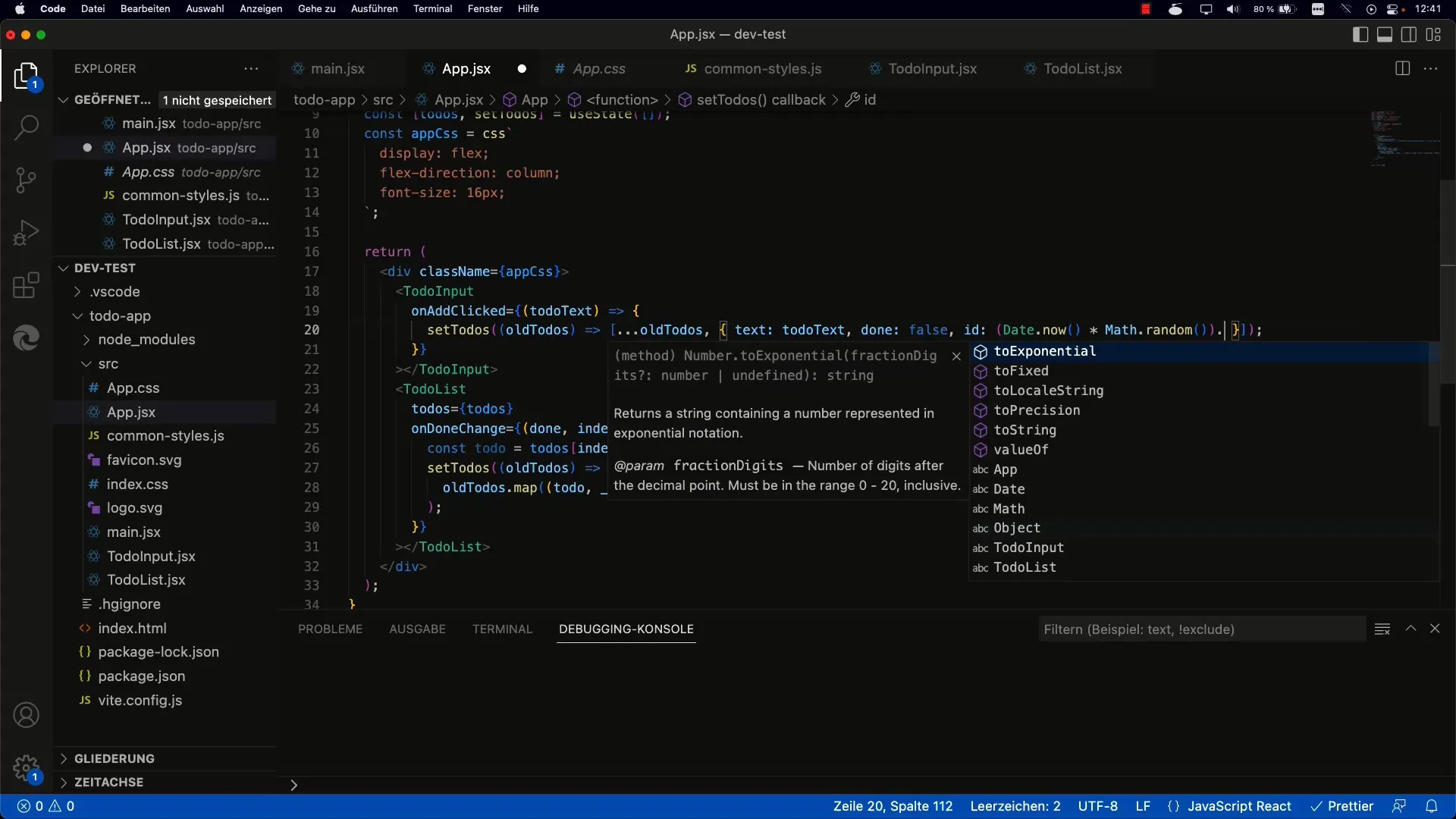
Step 4: Changing To-do Status
To change the status of a to-do, moving it from "not done" to "done," you need to update the onChange event handler. Make sure to use the ID instead of the index to identify the to-dos.
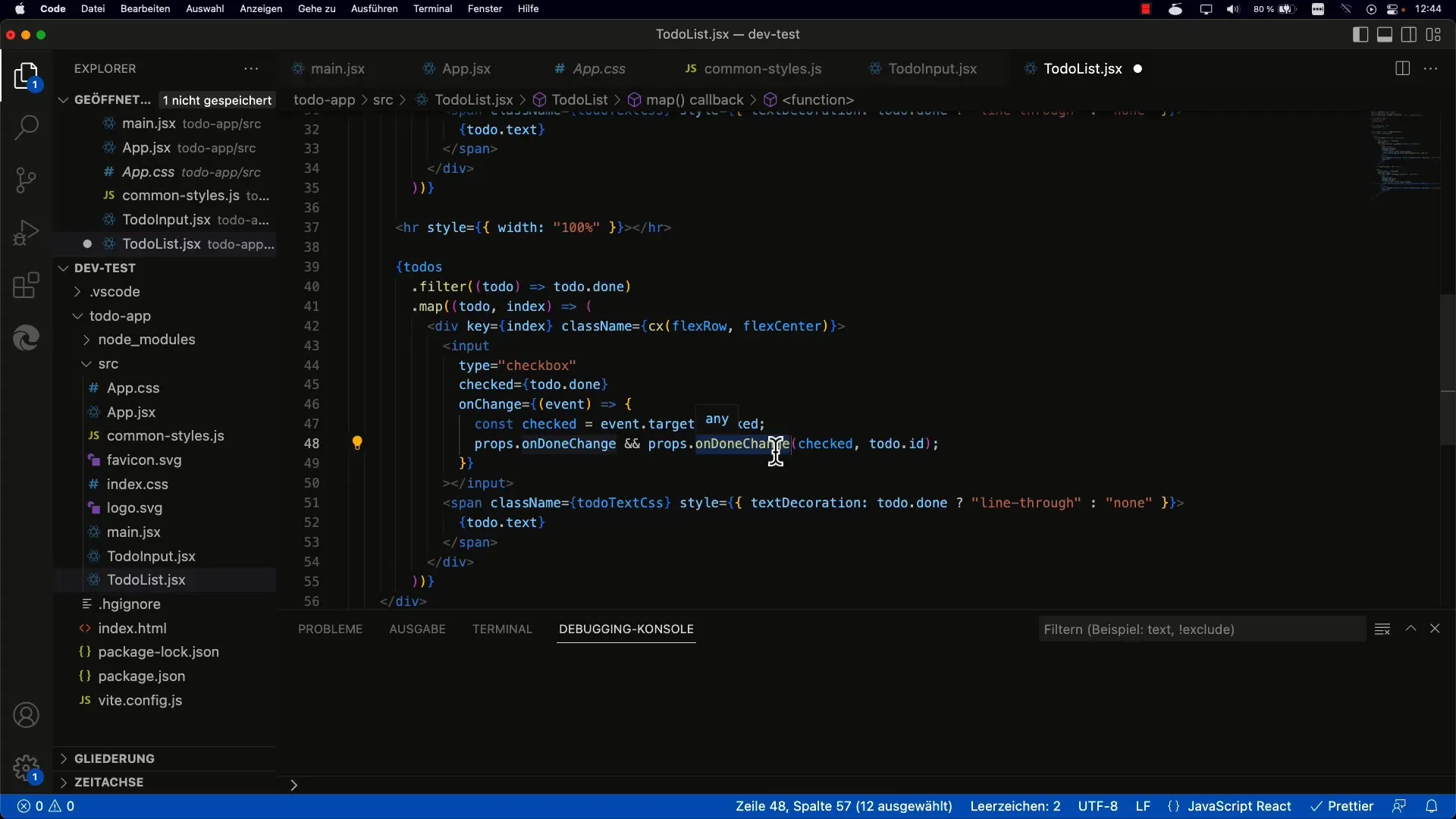
Test the application to ensure that changing the completion of to-dos works. You should be able to move to-dos from the top list to the bottom list and vice versa.
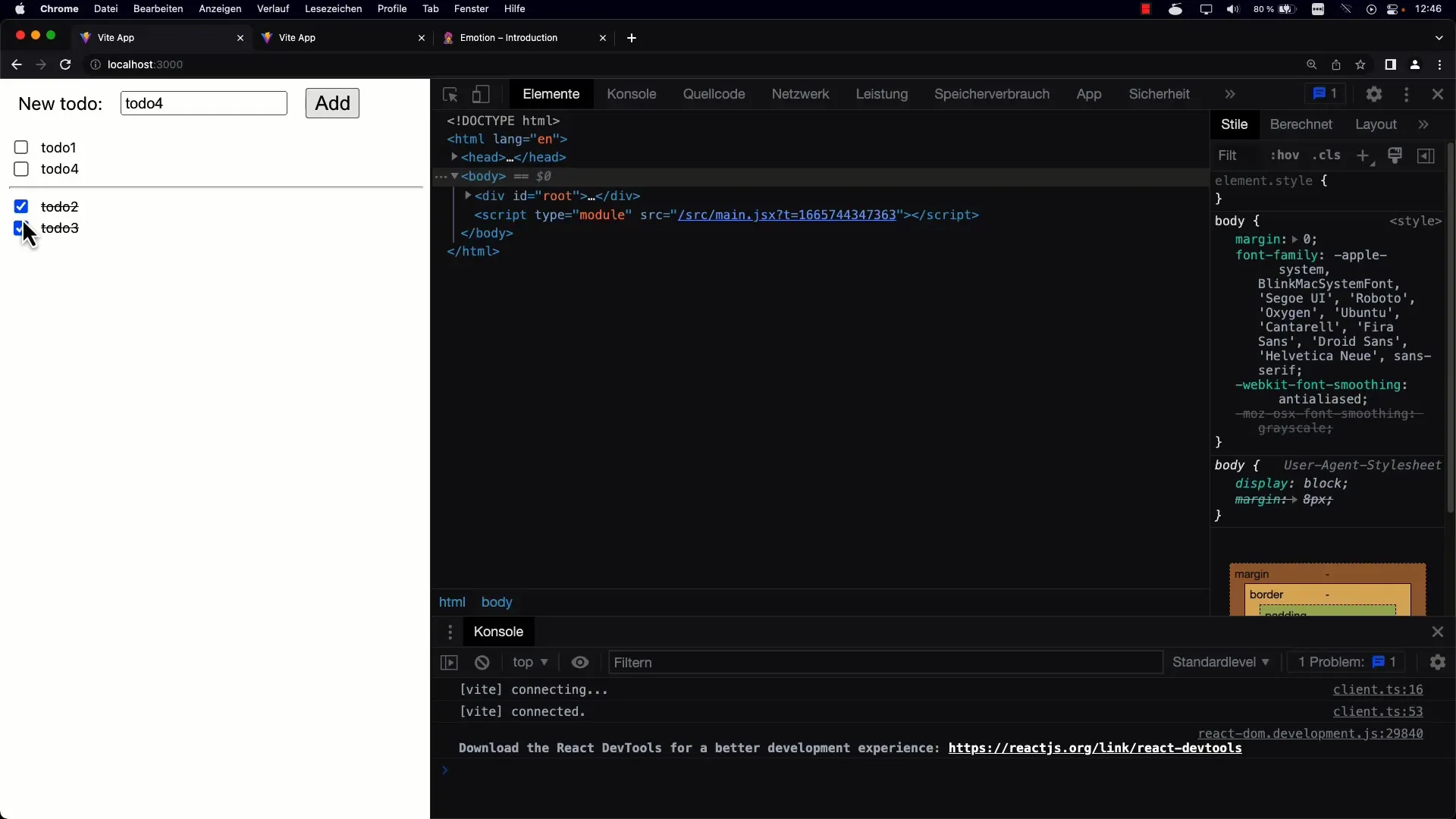
Step 5: Code Cleanup
This step is crucial. Make sure your code does not contain unnecessary references to indexes. By reverting to unique IDs, not only does your code become cleaner, but the behavior of your application also becomes more stable.
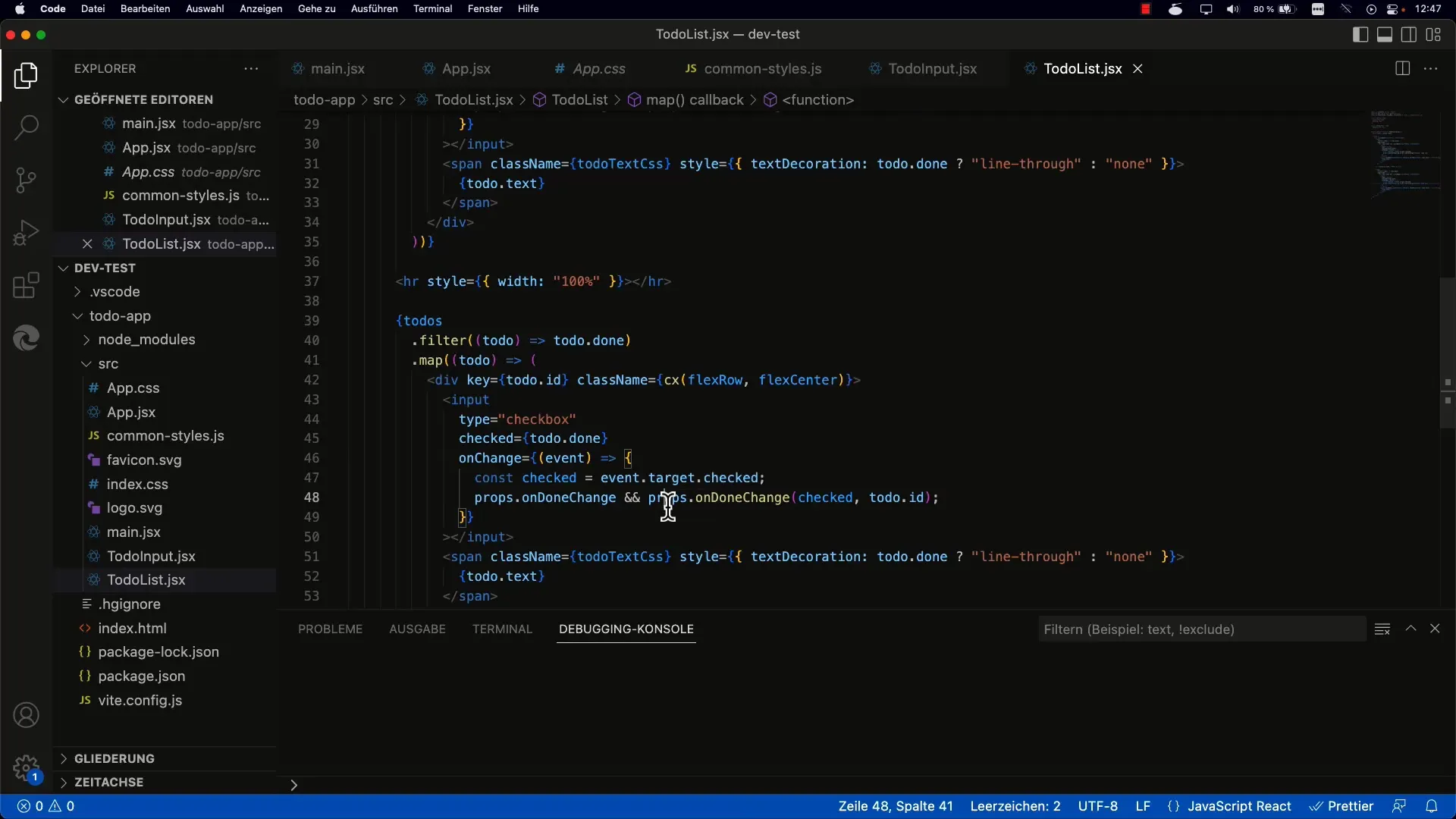
Summary
In this guide, you have learned how to create a to-do app in React that allows you to effectively group and manage tasks. Splitting into "not done" and "done" helps with task overview and provides an improved user experience. Avoid using indexes as keys to prevent issues with to-do identification.
Frequently Asked Questions
How can I filter to-dos?Use the filter method to sort to-dos based on their completion status.
Why should unique IDs be used?Unique IDs help avoid issues with identifying to-dos that can arise from changing indexes.
Can I expand the structure of the app?Yes, you can implement additional features such as deleting to-dos or using local storage to store data.
What is the next step to improve the app?The next step could be to persistently store to-dos so they are not lost when the page is reloaded.
Can I sort the to-dos?Yes, after using IDs instead of indexes, you can also add a sorting function to arrange to-dos.