With React, you have a powerful tool at your disposal for creating dynamic user interfaces. A central part of this data manipulation is the useEffect hook. In this tutorial, you will learn how to efficiently use useEffect as a Mounted Handler. This means you will be able to execute specific logic when a component enters the DOM. This functionality is not only important for data movement to and from servers but also for handling side effects.
Key Takeaways
- useEffect allows you to handle side effects in functional components.
- When using useEffect, you can specify when your effect should be executed based on dependencies.
- You can efficiently incorporate asynchronous data operations, such as data loading, in your React application build.
Step-by-Step Guide
Let's start with the basics to understand how useEffect works and how to customize it for our specific needs.
Step 1: Introduction to useEffect
First, you define the component where you want to use the hook. Create a new function and import useEffect from React.
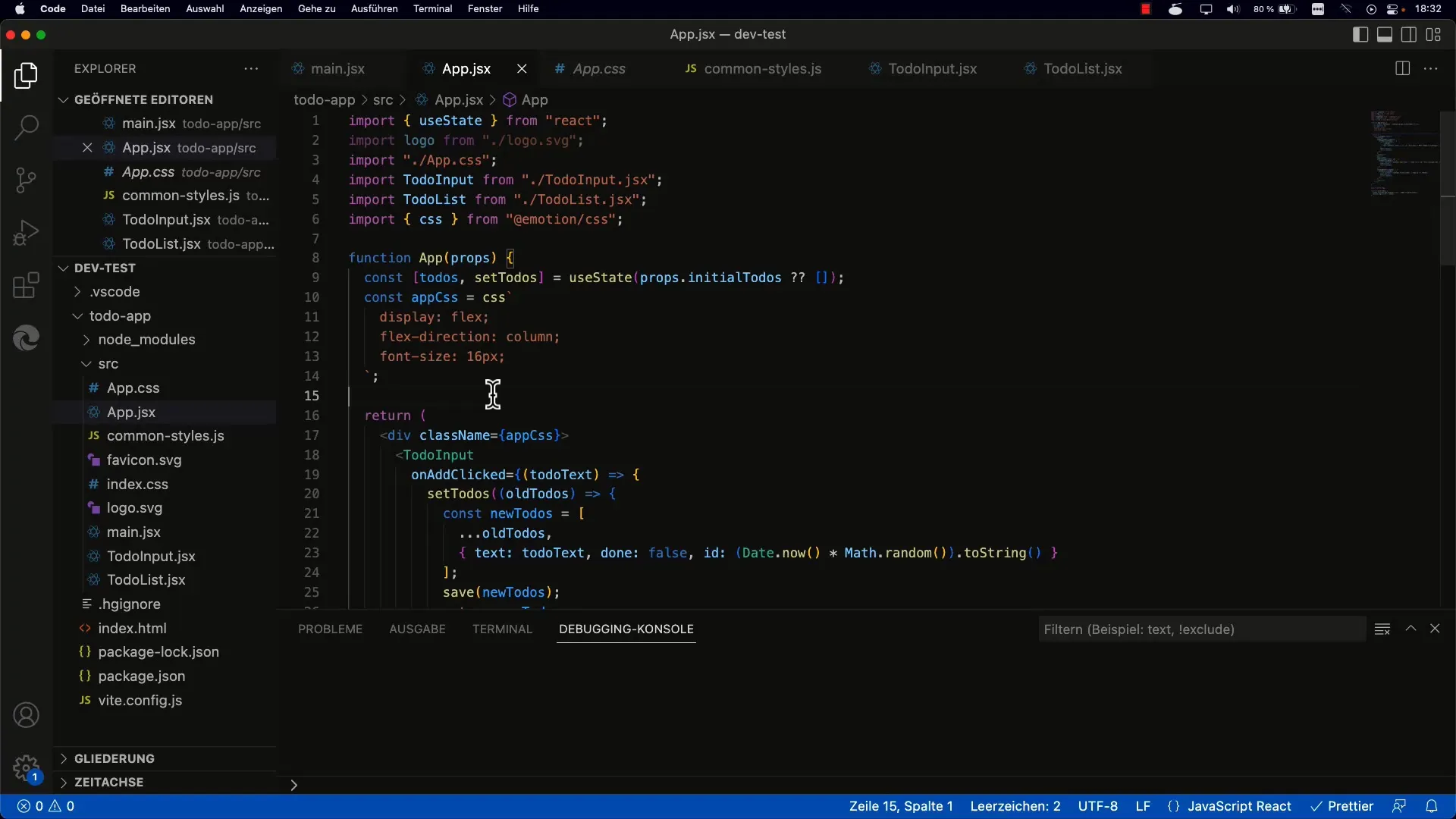
With useEffect, you have the ability to execute logical code snippets when the component is initially rendered or changes.
Step 2: Simple Usage of useEffect
First, you should insert a simple output in your component using useEffect. You can achieve this by adding a function to the hook that should be called during rendering.
This is the callback that will be invoked every time the component is rendered. When you render the component in the browser, you will see the output in the console.
Step 3: Understanding the Order of Calls
One of the first insights when working with useEffect is that it is called on every render. So if you do not want your effect to be executed multiple times, you should manage the appropriate dependencies.
If you only want your callback to be executed once when the component is mounted, you should pass an empty array as the second parameter at this point.
Step 4: Adding Asynchronous Functionality
Now we want to perform some asynchronous operations within our hooks, such as data loading. We can simulate this by using setTimeout to create a delay as if data were being loaded from a server.
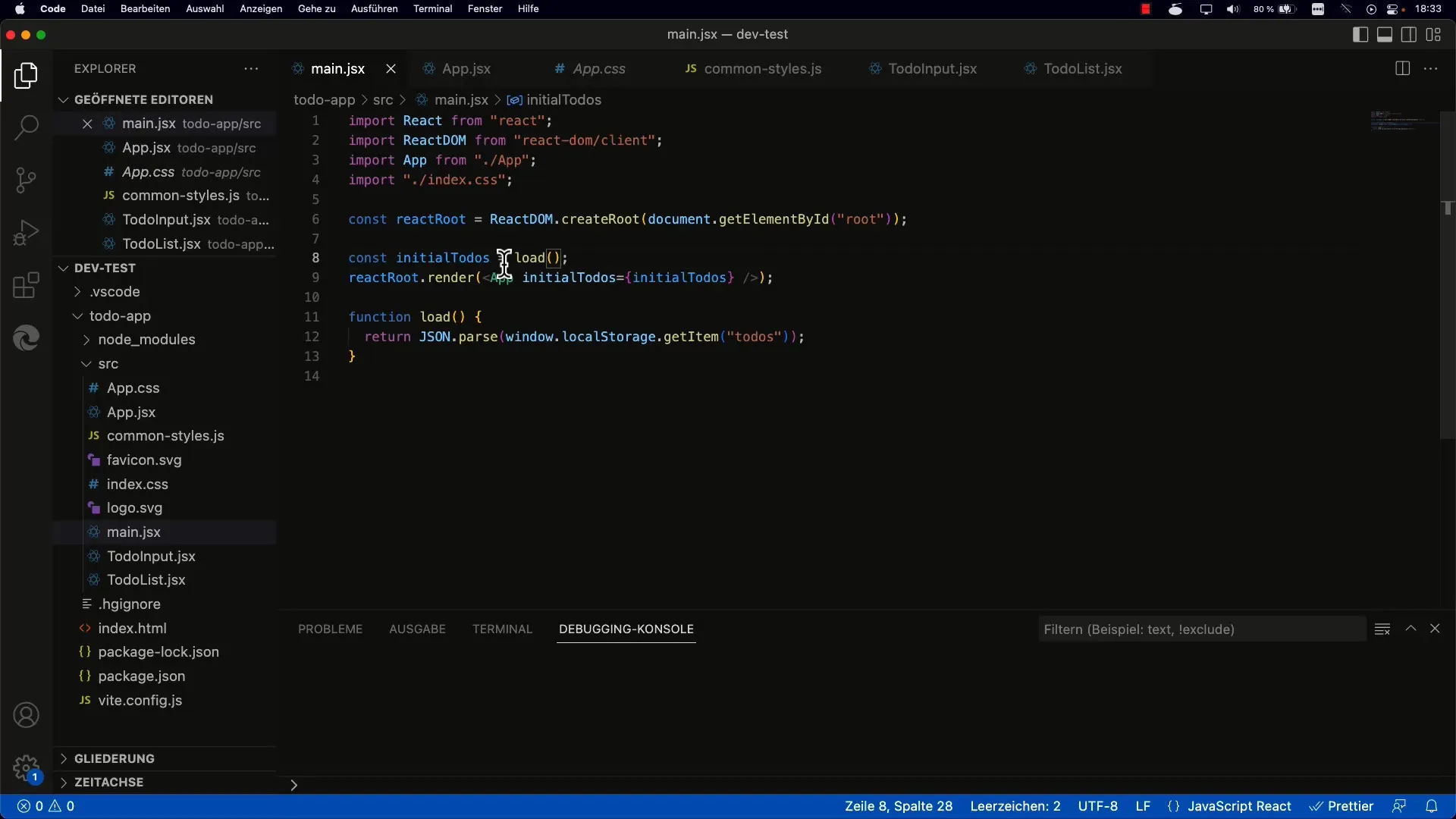
By inserting the loading logic into the useEffect callback, you only run the function once when the component is inserted into the DOM.
Step 5: Using Promises to Handle Asynchronous Logic
To enable the asynchronous nature of data processing, you can use promises. You will create a load function that loads the data and returns a promise with the data.
By chaining the resolved data from your promise in your useEffect callback, you achieve a clean design that takes all dependencies into account.
Step 6: Understanding the Importance of the Cleanup Function
When using useEffect, there is a case where you can return a cleanup function. This function will be called when the component is unmounted, or removed from the DOM.
This is important to avoid memory leaks and should be integrated into your workflow, especially with subscriptions or asynchronous processes.
Step 7: Utilizing Dependencies
Managing dependencies in useEffect is crucial. You can specify one or more variables as dependencies so that the effect is executed when any of them change.
Whether you address setTodo externally or observe specific values, this will affect your ability to effectively respond to changes in your app state.
Step 8: Testing the Implementation
Reload your app to see if the implementation is working. Check the console for errors and the output data.
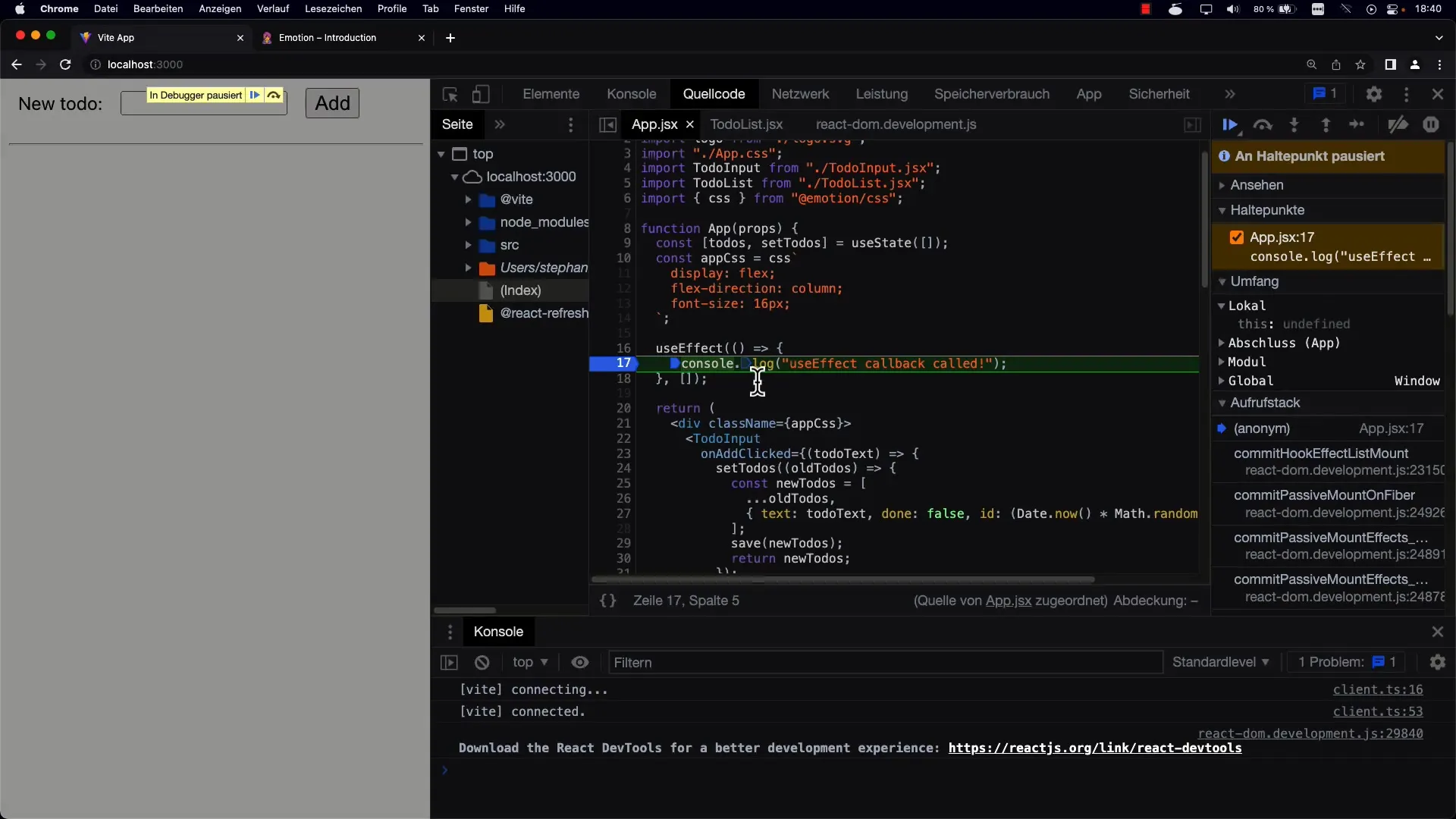
If everything is set up correctly, you should be able to see your to-do items as expected and observe the corresponding actions when the length of this list changes.
Step 9: Conclusion and Outlook
Now that you understand the basics of useEffect, you can expand this knowledge and apply it to more complex structures.
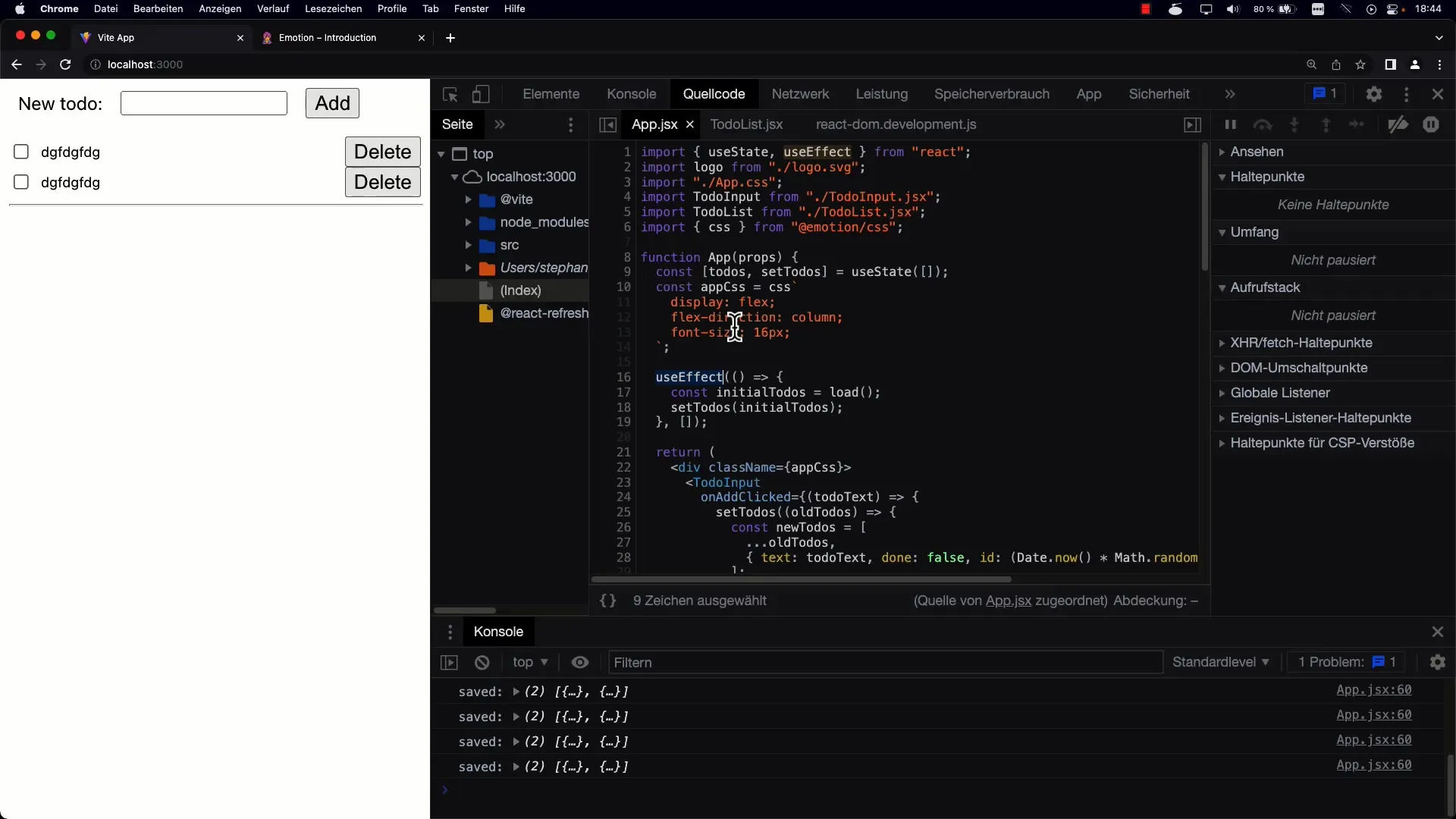
Use the principles of useEffect as a foundation and build upon it to develop more complex applications where the management of side effects becomes even more crucial.
Summary
In this tutorial, you learned all about using useEffect as a mounted handler in React. You understand the basic principles of dependencies, asynchronous operations, and the need for cleanup functions within your React components.
Frequently Asked Questions
What is useEffect?useEffect is a hook in React that allows you to handle side effects in functional components.
When is useEffect executed?useEffect is executed after the component renders. If you pass an empty array, it will only be called once during mounting.
How do I handle asynchronous data with useEffect?You can handle asynchronous logic by creating promises within the useEffect callback.
What is the cleanup function of useEffect?The cleanup function is called when the component is unmounted to perform cleanup tasks like stopping subscriptions.
What happens if I forget dependencies?If you forget dependencies and leave the array empty, your effect will only be executed once during mounting, not on subsequent updates.