Creating a Video Player App is an exciting way to expand your skills in React and Vite. In this guide, I will walk you through the process of setting up a simple Video Player App that allows you to create a playlist of videos and play them. You will learn how to structure an app, use components, and create a dynamic experience through simple user interactions.
Key Takeaways
The tutorial demonstrates how to create a React app with Create Vite, set up the code for a video player, and implement a playlist with video files. Additionally, we will learn how to add basic functions for video playback.
Step-by-Step Guide
Step 1: Create Vite Project
Firstly, you need a new project created with Vite. Go to the parent directory where you want to save your project. If you have already created a ToDo app, you can simply switch one level up.
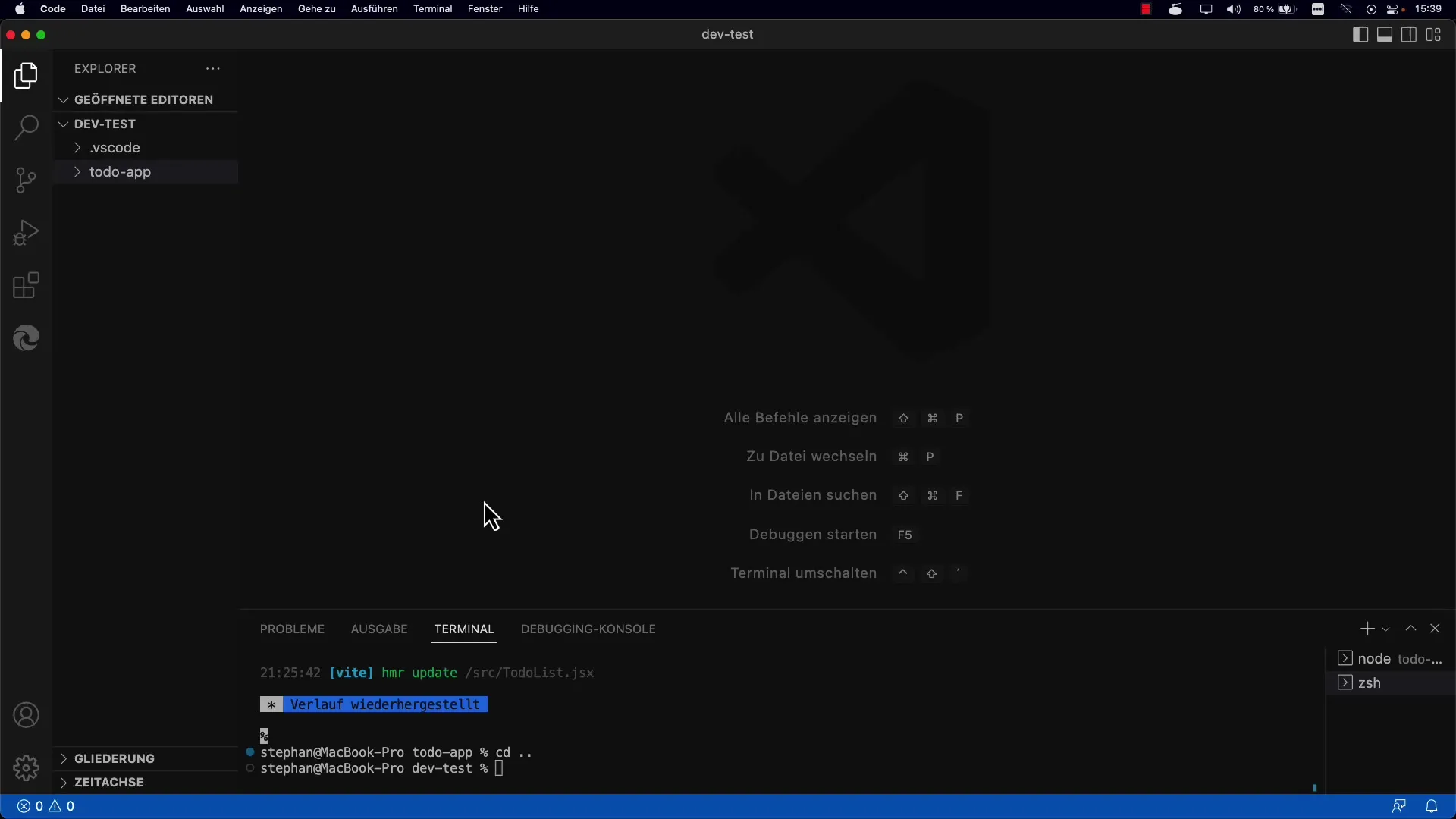
Now you can initialize a new Vite project with the command npm create vite. You will be prompted to enter the project name. Simply name it "Video Player" and choose React without TypeScript.
After creating the project, navigate to the subdirectory "videoplayer". Install the dependencies with the command npm install. Once the installation is complete, you can start the app with npm run dev.
Step 2: Prepare for the App
After starting the app, you should customize the source code. Open the file src/Main.jsx. You can keep the content as is and leave the Strict Mode active. This is important for adhering to best practices in React.
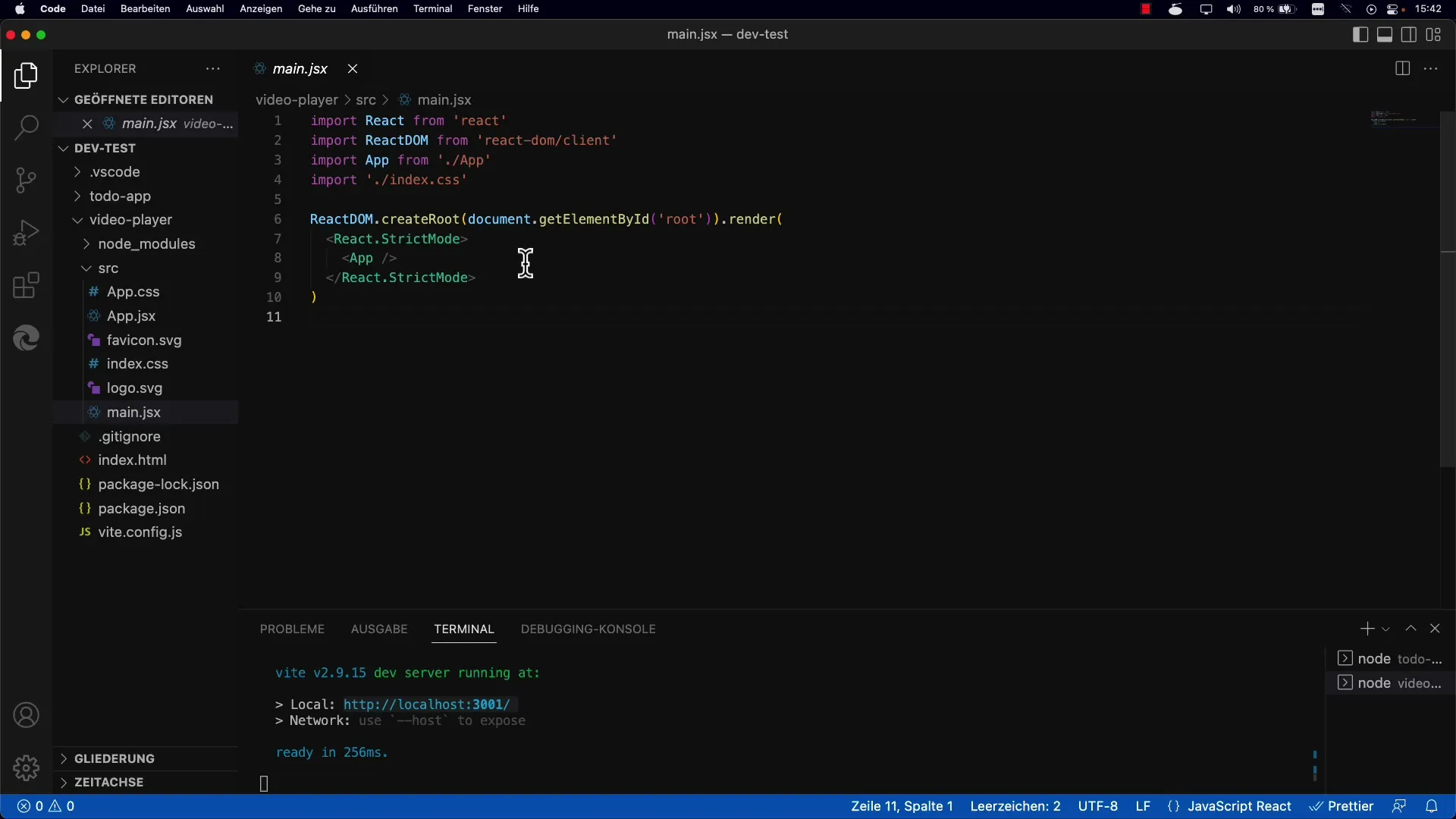
Step 3: Customize App Component
Now navigate to the file src/App.jsx. Here, you can delete the existing code, except for the outer div. The goal is to create the foundation for the video player app. Since we are starting from scratch, you no longer need the previous code.
Step 4: Define Playlist Structure
Consider how you want the user interface to look. We need an area for the playlist where video URLs can be added. The playback area will be below, and we will ensure that there are controls like Play and Pause.
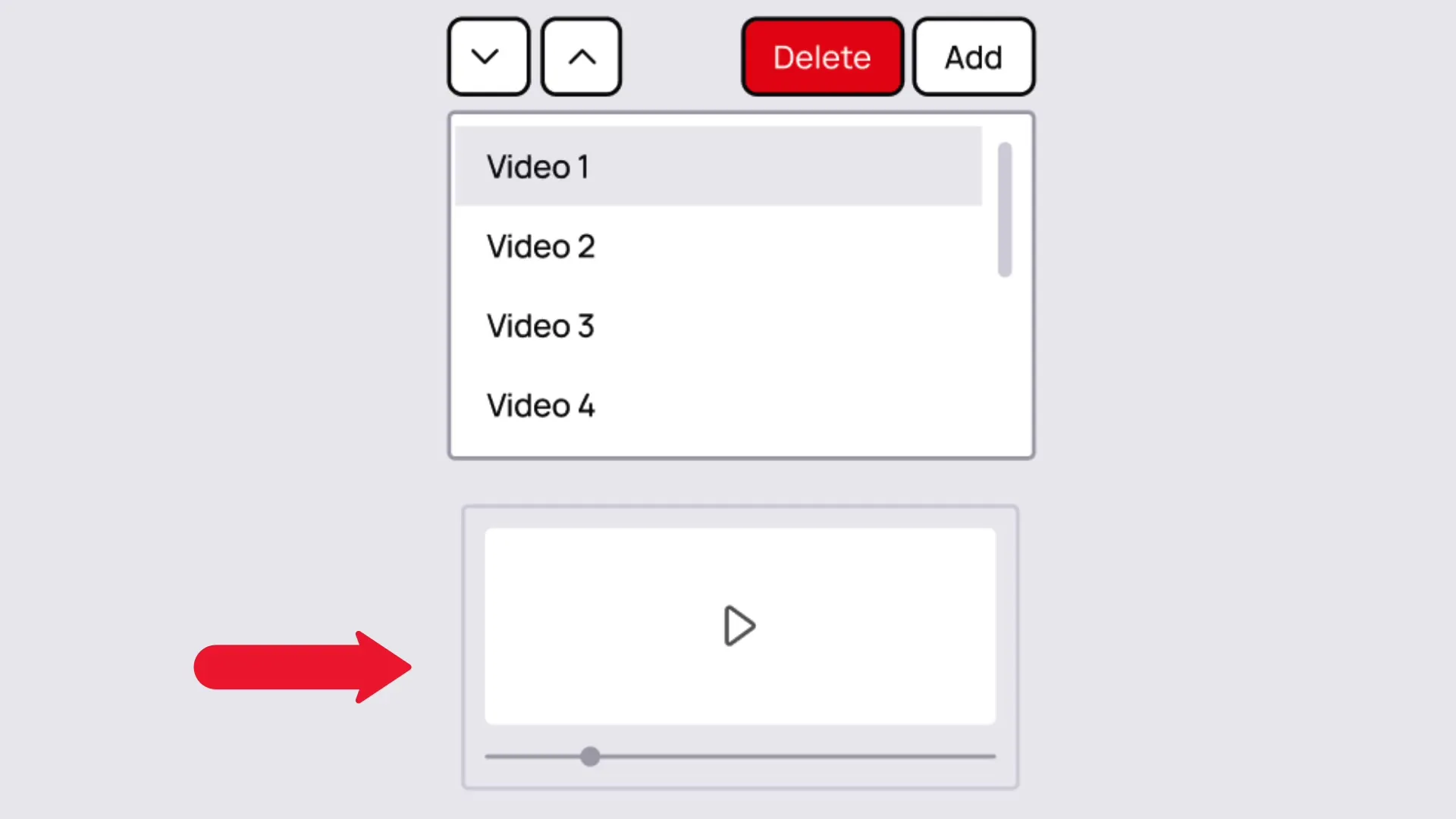
The concept is simple: The playlist consists of different URLs representing the videos. Users can add or remove videos, creating a personalized list of videos.
Step 5: Implement Basic Interactions
To ensure interactivity, you will use some new hooks. The goal is to dynamically adjust the playlist based on which videos the user adds or removes.
Here, you have the opportunity to further customize and enhance the video player app. You can add additional features such as volume control or automatic playback of the next video.
Summary
This guide has shown you how to create a basic Video Player App using React and Vite. You have learned how to initialize a Vite app, create the structure for your app, and enable basic interactions. With these foundations, you are well-equipped to further enhance and customize your app.
Frequently Asked Questions
How do I start a new Vite project?Use the command npm create vite and give your project a name.
Can I use TypeScript in my project?Yes, you can also choose TypeScript during project creation.
How do I add video URLs to my playlist?Use an input mechanism to add URLs, which then need to be managed in the state.
How do I test my app?Start the app with npm run dev and open the specified address in your browser.