Developing an effectively functional video player is an exciting task that can give you a deep insight into the world of React. In this tutorial, you will learn how to create a video player component with essential control functions like Play, Pause, and Stop. The focus is on keeping the logic clear and optimizing user interaction.
Key Takeaways
- You will learn to create an independent video player component.
- The implementation of Play, Pause, and Stop buttons is explained step by step.
- You will gain insights into working with hooks in React, particularly useEffect.
Step-by-Step Guide
Step 1: Creating the Video Player Component
First, you need to create a new file for your video player component. Name it Videoplayer.jsx. Initially, you can copy and modify the code from your existing app component to adopt the basic structure of the new component. Then, remove all unnecessary imports that are not needed.
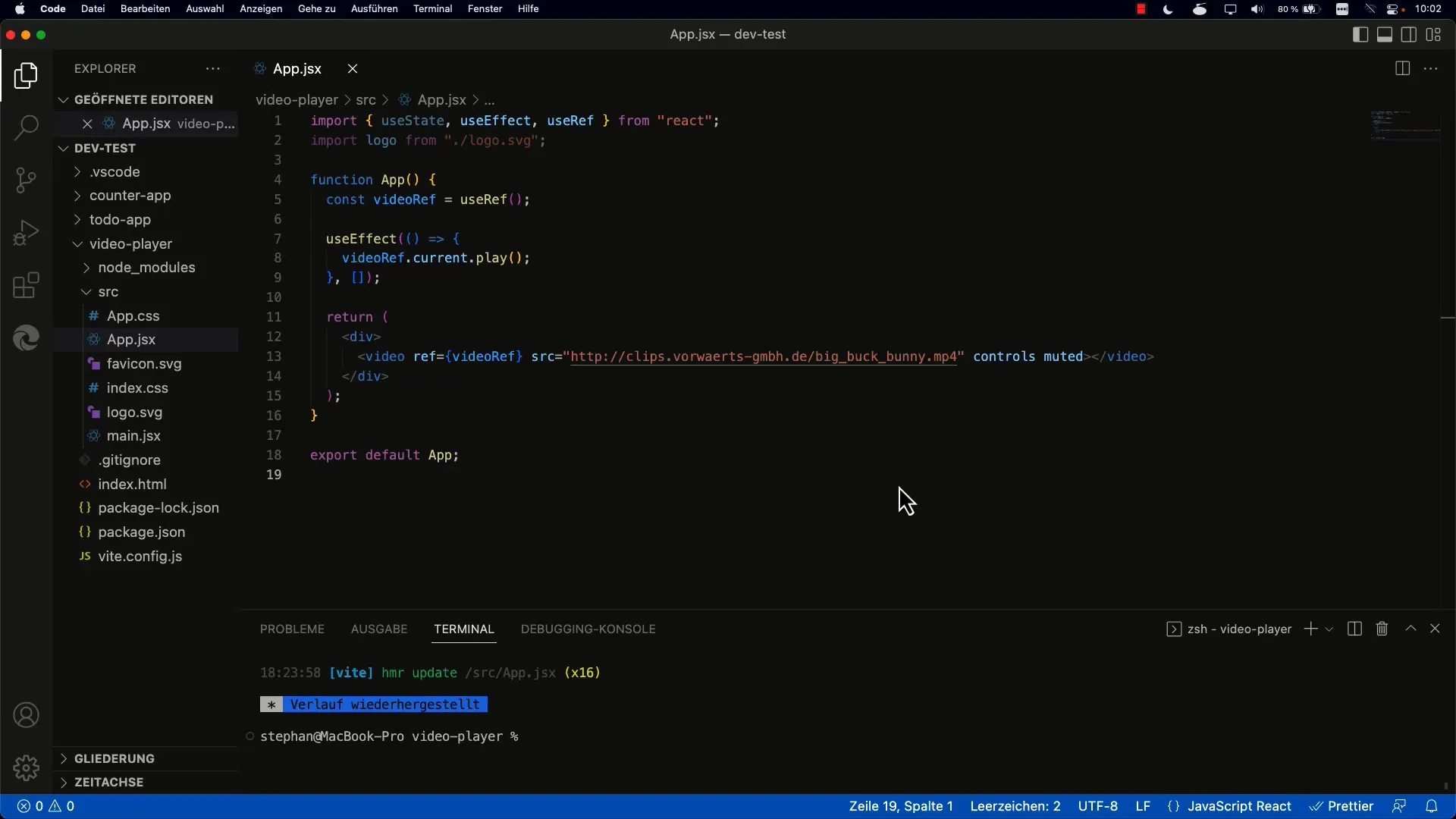
This is the first step to separate the player from your main application and enhance the maintainability of your code.
Step 2: Integrating the Videoplayer Component into the App
After creating the basic structure, you need to integrate the new Videoplayer component into your main application. Replace the existing component tag with Videoplayer in your app component.
Ensure that you also correctly import the component for everything to work. You will see that the component is now independent and can play the video.
Step 3: Adding Control Buttons
Now is the time to add the control area for the video player. Create a new div element below the video where you insert the buttons for "Play," "Pause," and "Stop."
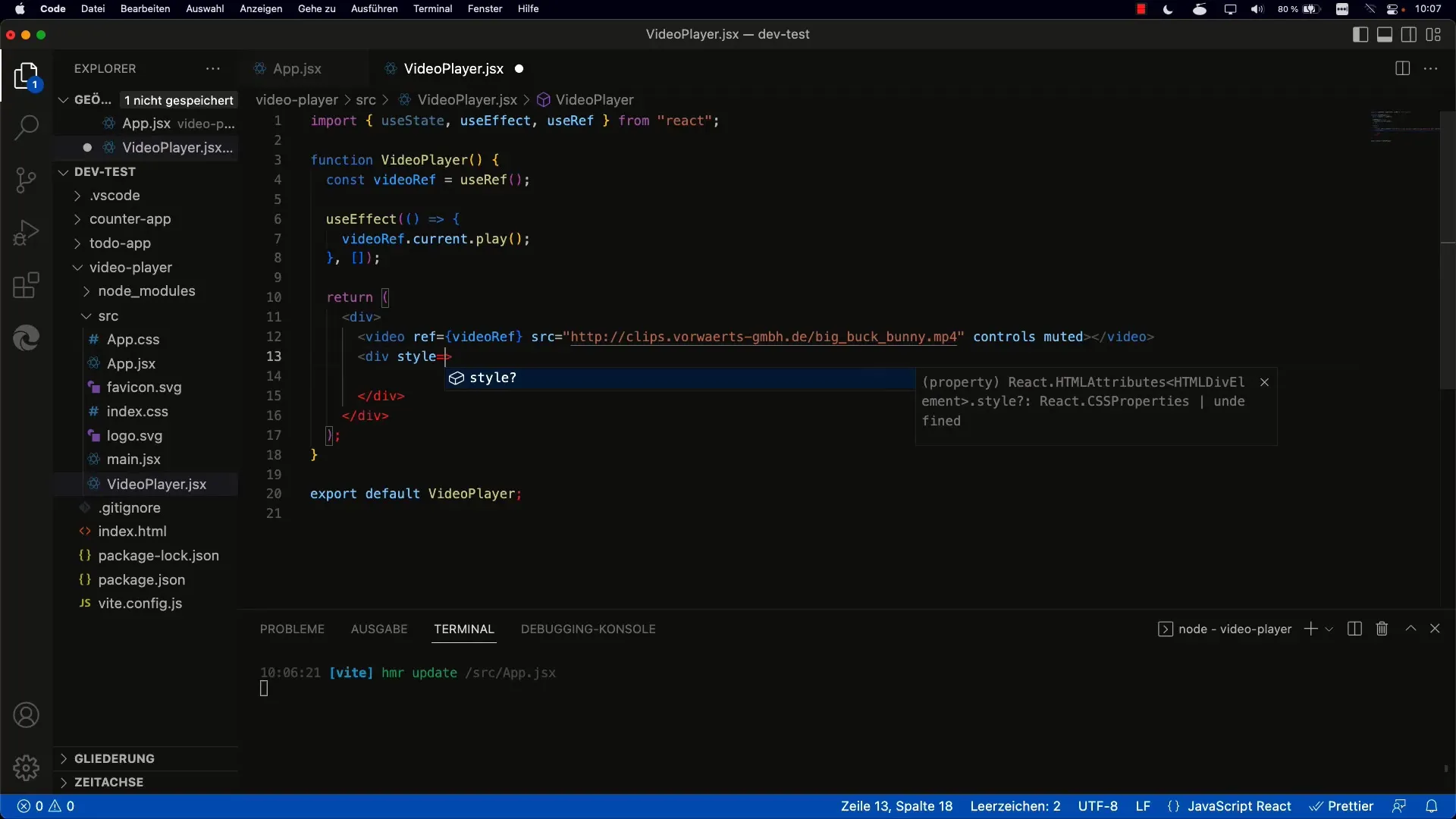
In this section, also set the CSS properties for the div to ensure that the buttons are neatly arranged.
Step 4: Centering the Buttons
To make the user interface more appealing, center the buttons below the video by applying Flexbox styling. Make sure to set the justify-content property to "Center."
A well-structured layout significantly enhances the user experience.
Step 5: Implementing Button Functions
Now comes the most exciting part: implementing the button functionalities! Use onClick handlers to implement the Play, Pause, and Stop logic. The basic functionality here is quite straightforward: For the Play button, call the respective Play function, for Pause the Pause function.
The Stop function requires a bit more consideration. It must first pause the video and reset the playback position to zero, so the video starts from the beginning on the next play.
Step 6: Testing the Functionality
At this point, you should test your code to ensure that all buttons function as desired. Refresh the page and check if Play, Pause, and Stop work correctly. The video should no longer autoplay since there is no autoplay logic anymore.
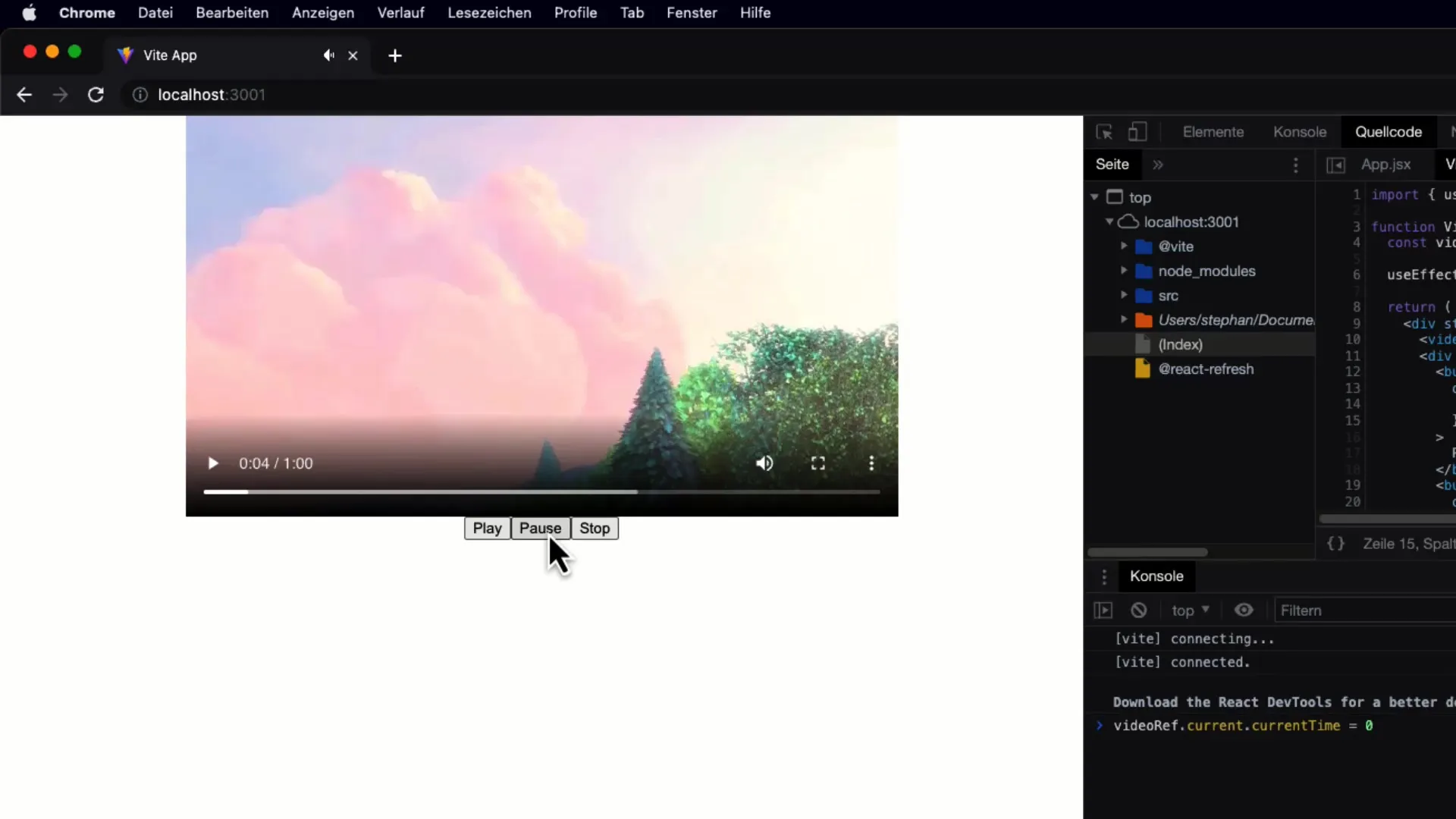
Step 7: Managing the Video Player's State
An important enhancement is managing the state of the video player. Implement a state to keep track of whether the video is currently playing, paused, or stopped. This allows you to merge the Play and Pause buttons into a single button that reacts accordingly based on the state.
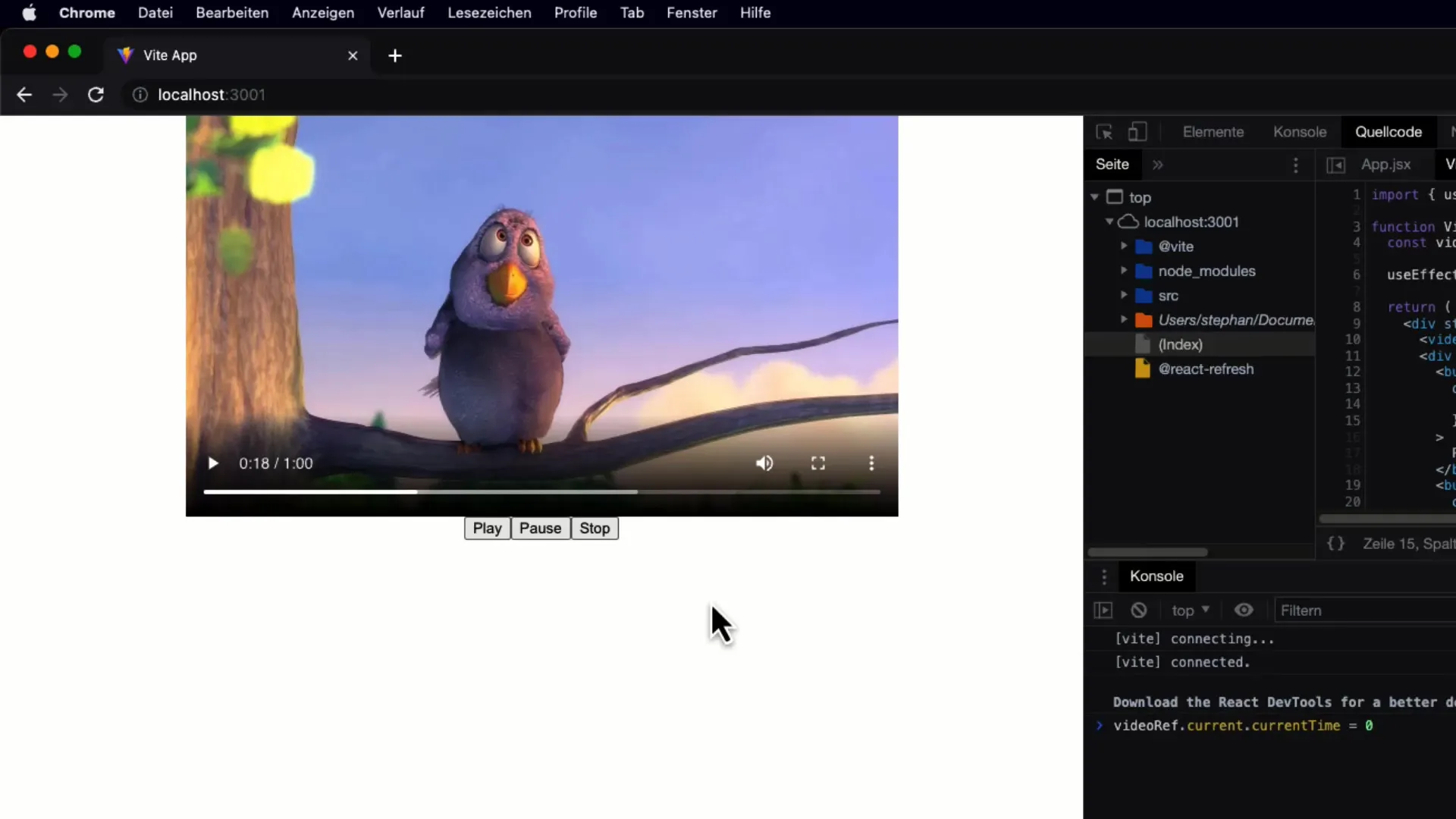
This further optimizes the user interface and enables you to handle the button representation more effectively.
Summary
You have now learned how to create a functional video player component in React. From creating the component to implementing the controls and managing the state, you have gone through all the crucial steps. Experiment with the code, extend the functionalities, and refine the design according to your preferences.
Frequently Asked Questions
How do I import the video player component into my app?In your App.jsx file, you need to import the component with import Videoplayer from './Videoplayer.jsx';
How does the stop button work exactly?The stop button pauses the video and sets the playback position to 0, allowing the video to be restarted from the beginning.
Can I further customize the buttons?Definitely! You can customize the styles and icons of the buttons as you like to personalize the appearance according to your preferences.