You have a fixed set of videos in your application and want to dynamically style this overview? Then you are in the right place! In this tutorial, I'll show you how to replace a static video list with a dynamic array. This allows you to flexibly customize the options of your playlist and adjust them quickly if needed.
Key Takeaways
- Using useState to create a dynamic array.
- Using map to render the videos from the array.
- Applying the key Prop in list components to avoid warnings.
- Handling IDs to identify videos within the playlist.
Step-by-Step Guide
Step 1: Define the Array
First, you need to create an array that contains the video data. To dynamically render the videos later, you will use the useState hook from React.
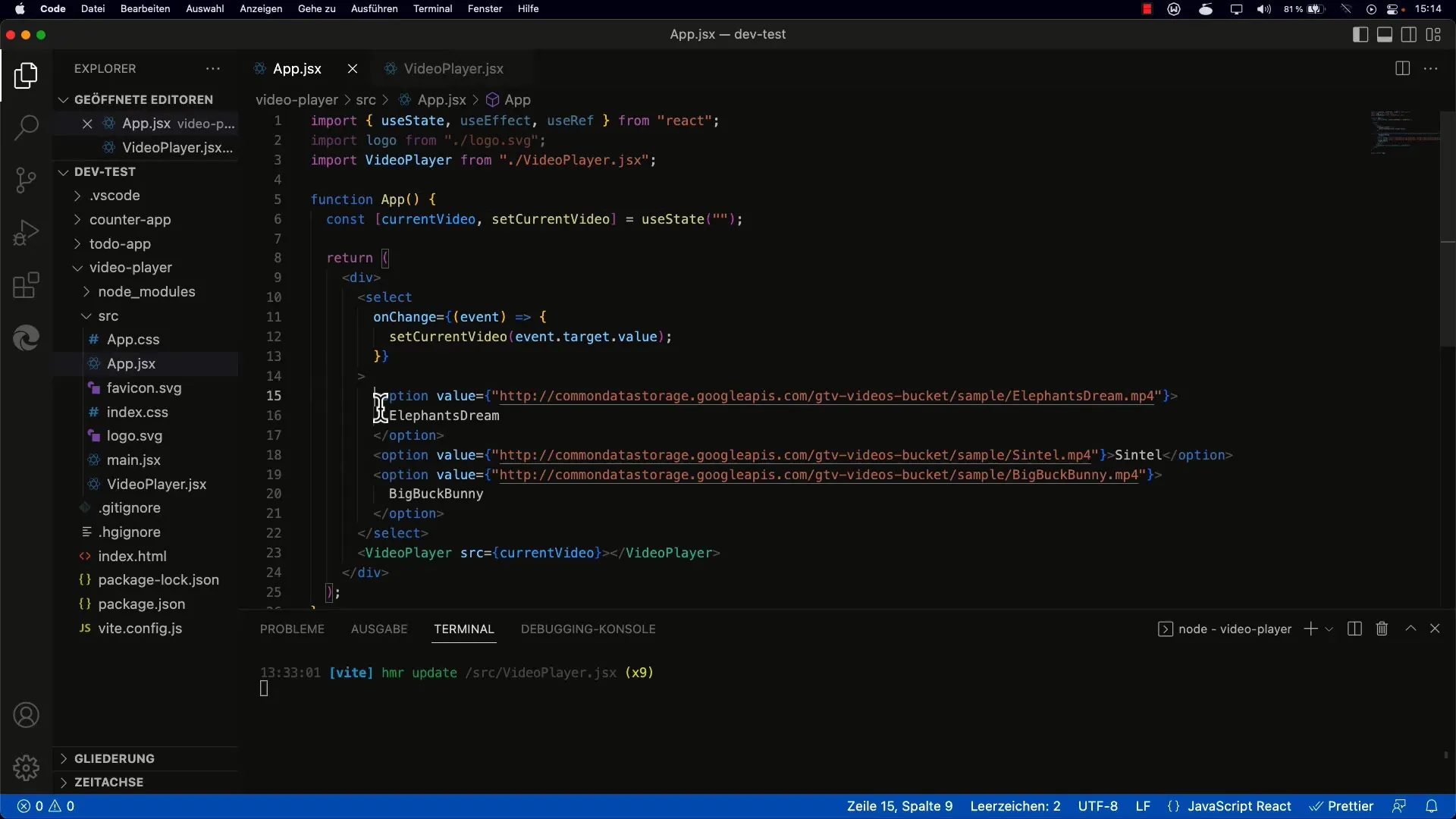
You start by using a useState hook. In this first step, you define your video entries in an array.
It is important that each object in the array contains an ID, a source for the video, and a title. This structure ensures that you can easily access the data later on.
Step 2: Render the Videos
Once you have created the array, it is time to display these entries on the user interface. For this, you can use the map function of JavaScript.
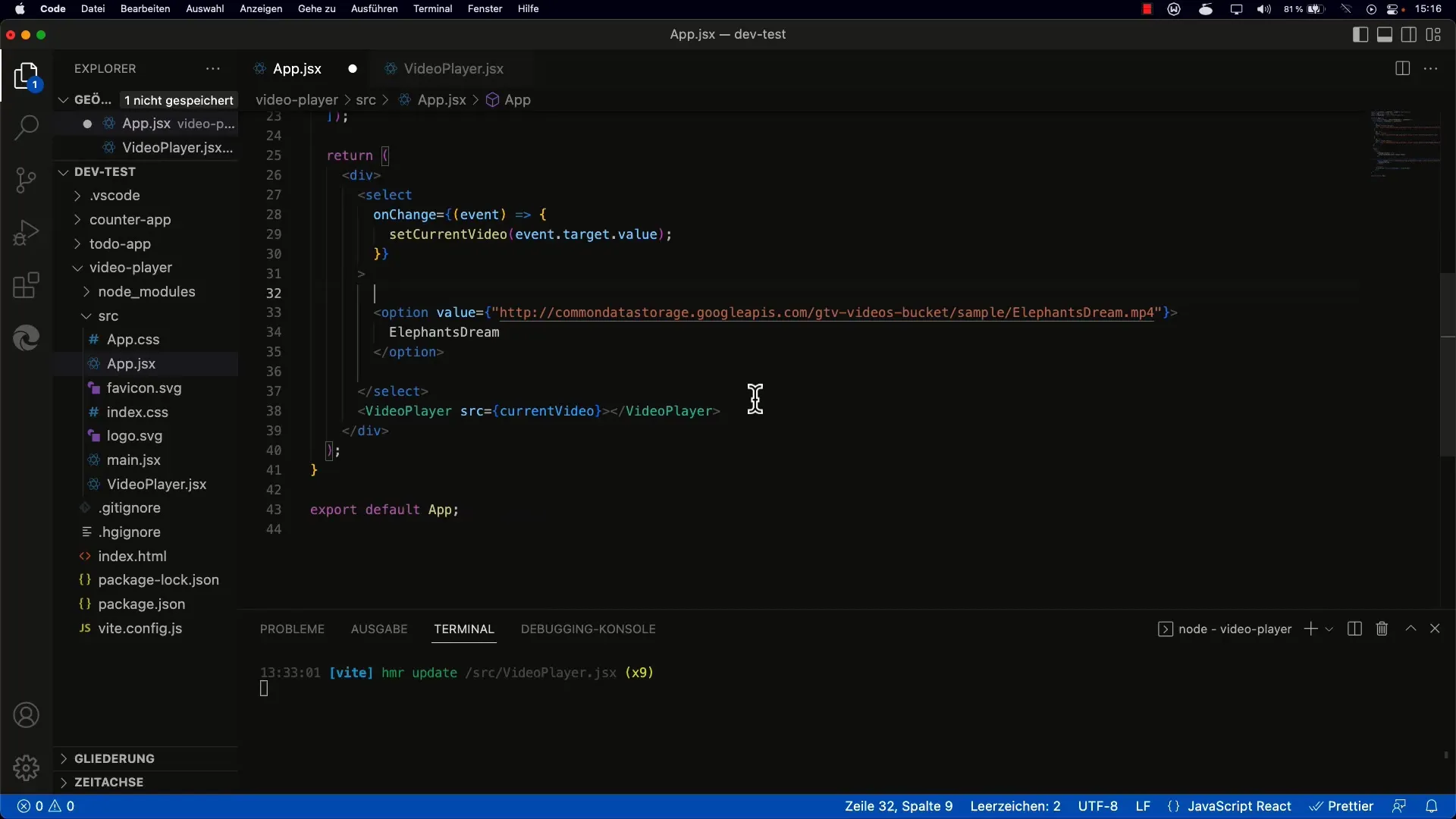
With the map method, you iterate over each video in the array and return an option element for each one. In this element, you set the value attribute to the ID of the video.
The title of the video should be displayed as visible text in the dropdown list. Make sure to use the properties already defined.
Step 3: Add the key Prop
To avoid warnings, you need to assign a unique key Prop to each element in your list. This helps React efficiently re-render the elements.
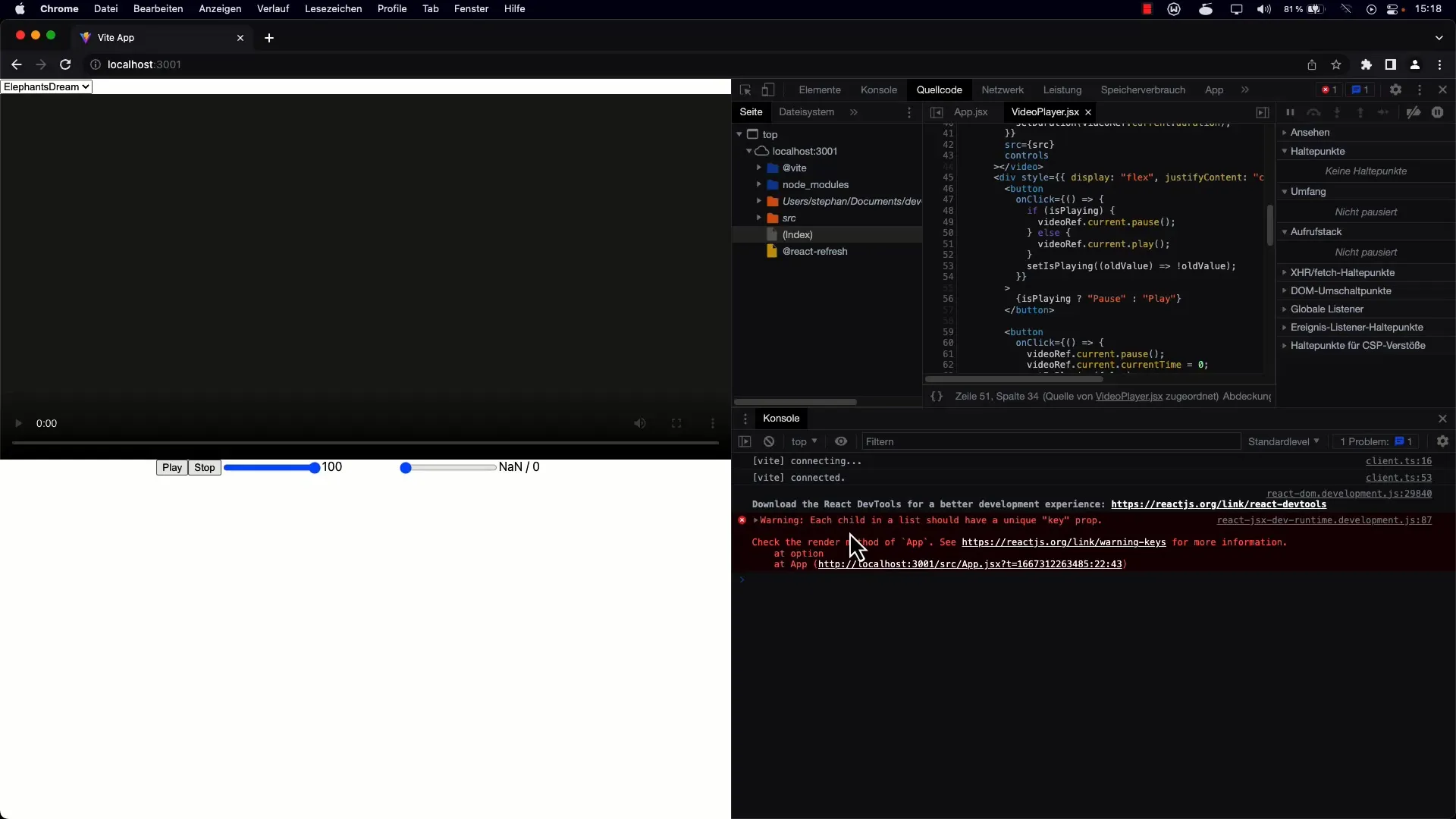
Add the key Prop in your option element and set it equal to the ID of the video. This is crucial to ensure that your application runs smoothly.
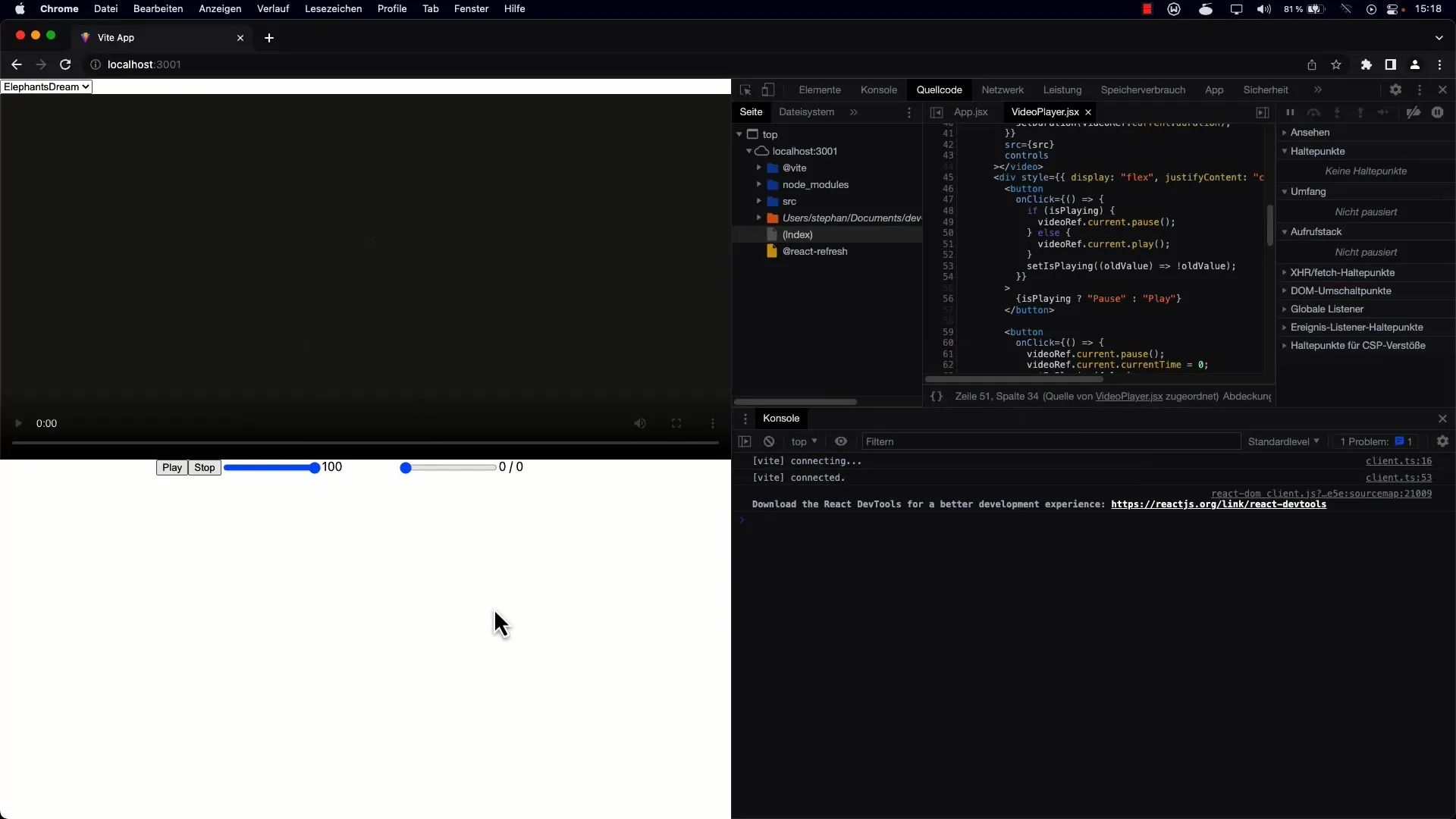
Step 4: Enable the First Video
When your list is displayed, you also want to ensure that the first video in the list plays when the page first loads. For this, you need to correctly set the value of the select element.
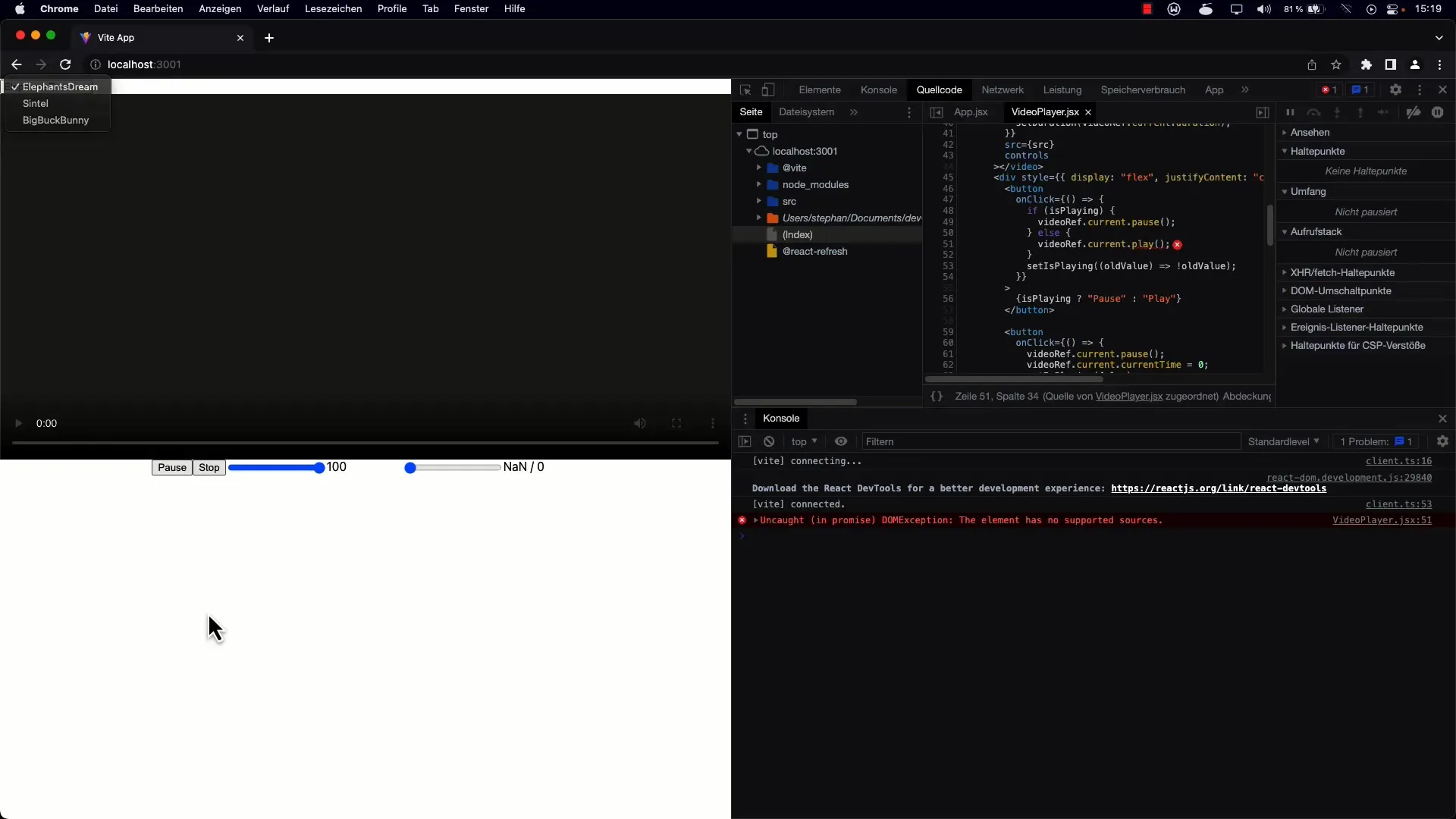
You pass the source of the first video to the video element. If no video is selected yet, you can set the first video from the array as the default.
Step 5: Test the Implementation
Reload the page to ensure that everything works as expected. The dropdown list should now be dynamically filled with the videos, and the first video should be automatically selected when the page loads.
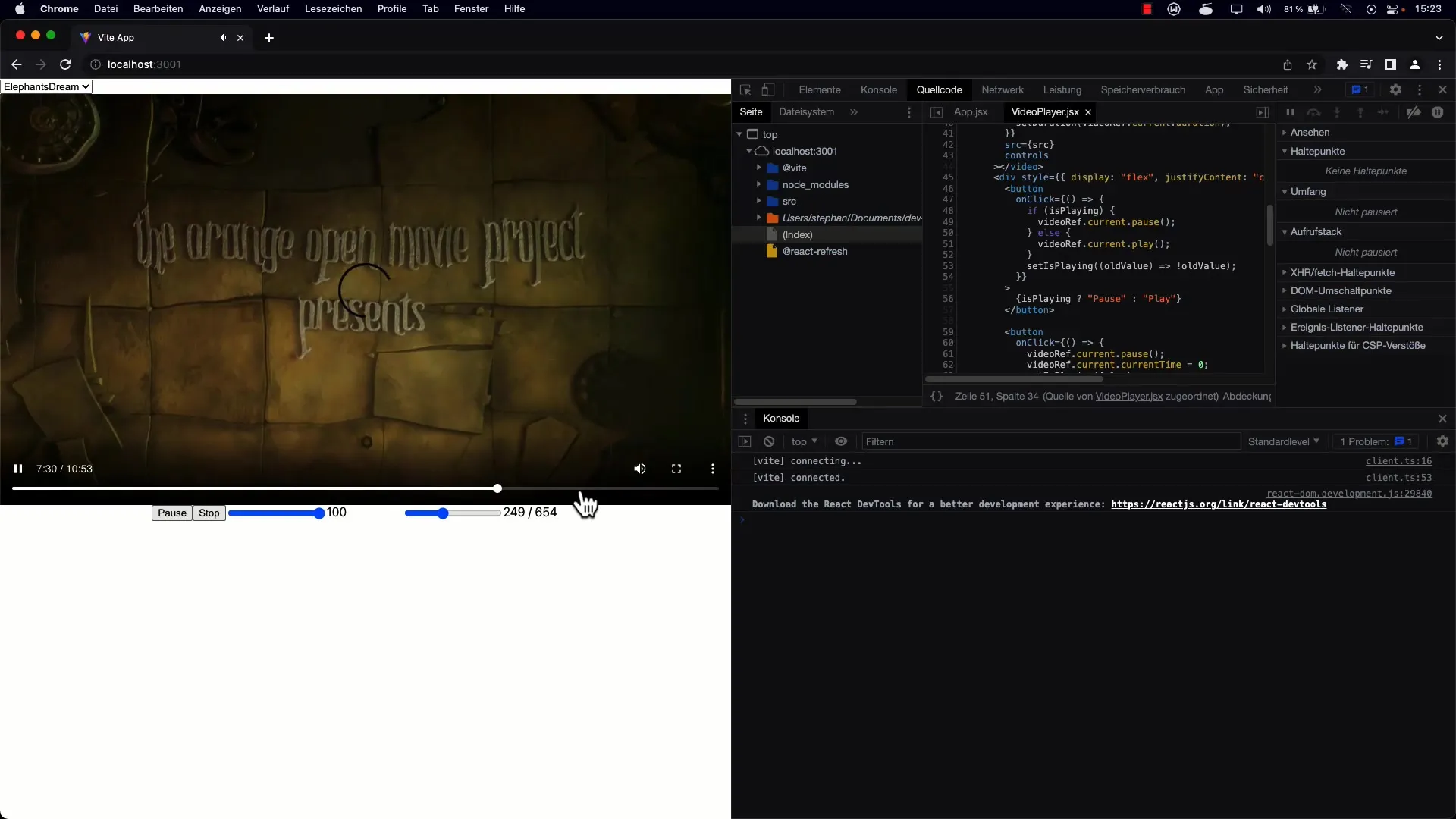
Also, make sure that the IDs and key Props are correctly set to avoid a warning. This ensures that you provide a smooth user experience.
Step 6: Adjustments for Dynamic Inputs
In future steps, you can also add a button and two input fields to add new videos. These should allow you to enter a new URL for the video and a title.
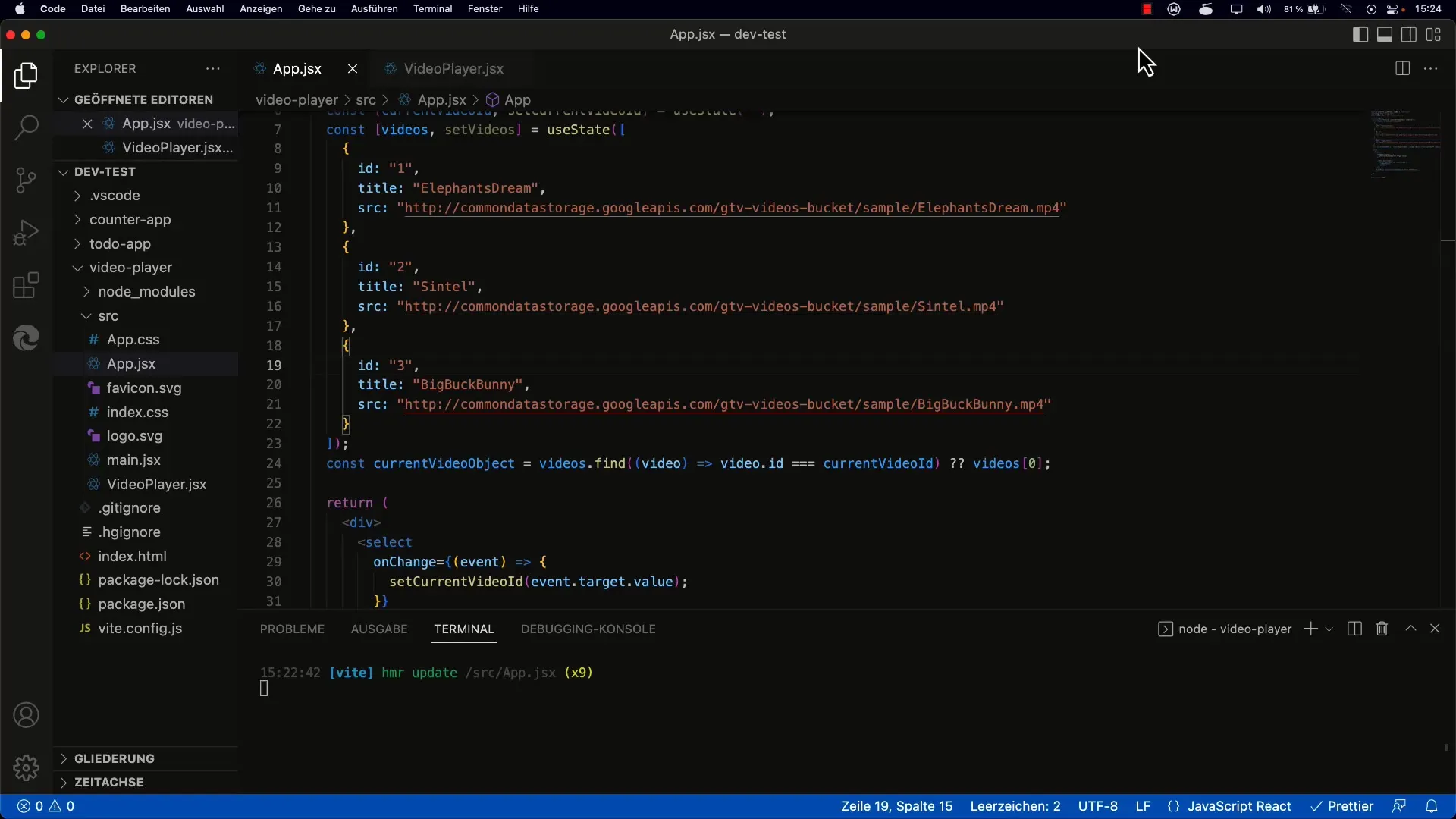
This functionality increases the flexibility of your application by allowing users to create and edit their own playlist.
Summary
You have learned how to replace a static list of video entries with a dynamic array in React. By using the useState hook and the map function, you can create a customizable and user-friendly playlist that can be easily expanded.
Frequently Asked Questions
What is the reason for using key in lists?React uses the key prop to track elementary expressions and enable more efficient updating of the UI.
How can I add more videos to my list?You can create a form with input fields for the URL and title of the videos and insert the new data into your array.
What happens if I don't set a key for an element?The absence of a key can lead to warnings in the console and affect the performance of your application, as React does not render optimally.