Deletion of entries from a list is one of the fundamental tasks in web development. When developing a to-do app or a playlist with videos, it is important not only to add entries but also to be able to remove them effectively. In this tutorial, I will show you how to implement a button in a React project that allows deleting a selected entry. We will use the filter method of arrays to achieve the desired functionality.
Key Insights
- You add a button that allows deleting entries from a list.
- The filter method is used to create a new array that does not contain the element to be deleted.
- Working with IDs instead of indices is advisable to avoid issues when deleting entries.
Step-by-Step Guide
First, you should create a new button responsible for deleting an entry.
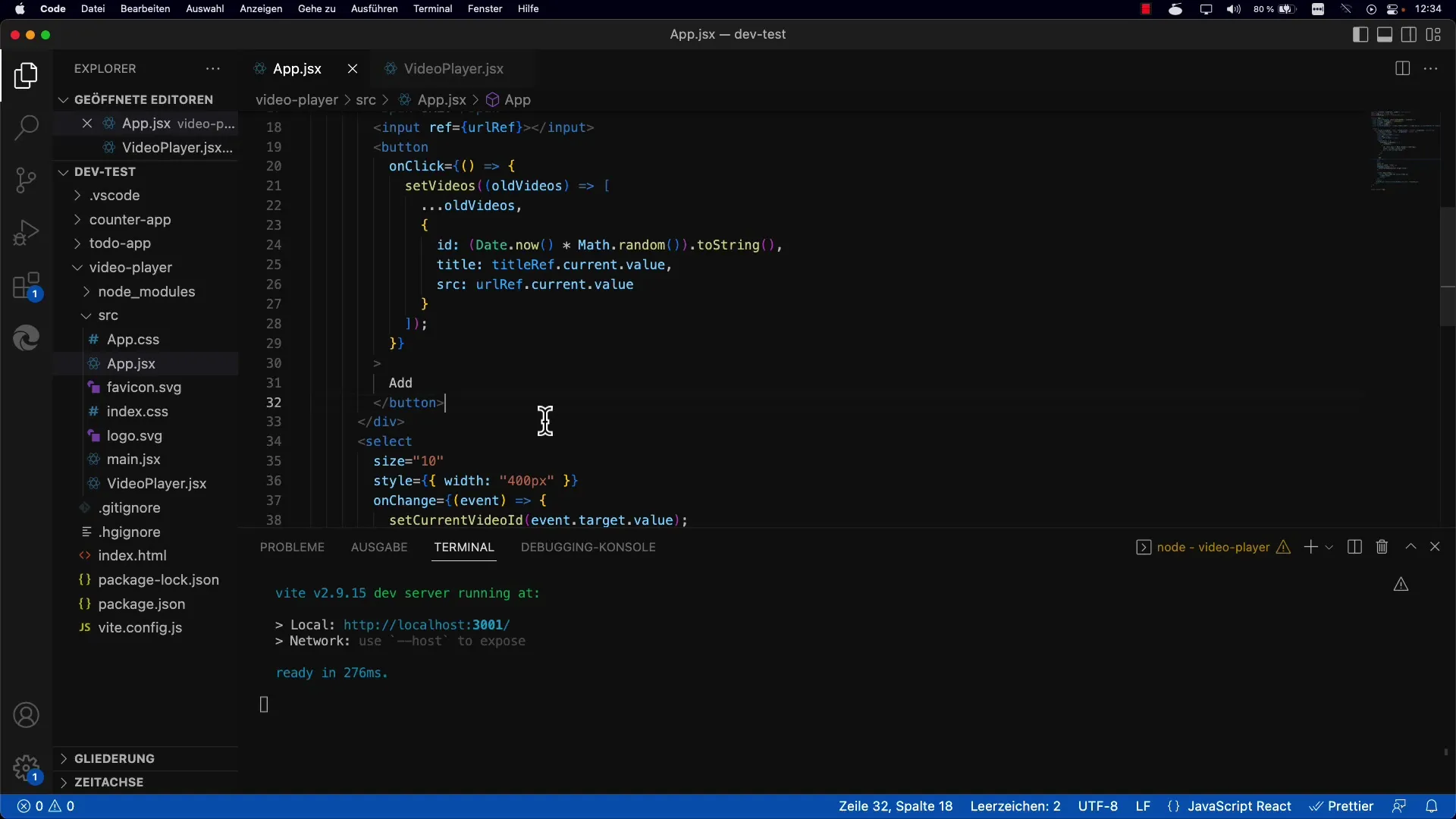
Here, you can create a delete button similar to the add button. The crucial difference is that the delete button removes a selected entry instead of adding a new one.
To implement the deletion function, you use the Set Videos method. This method sets the videos in your state management, allowing you to dynamically adjust the list.
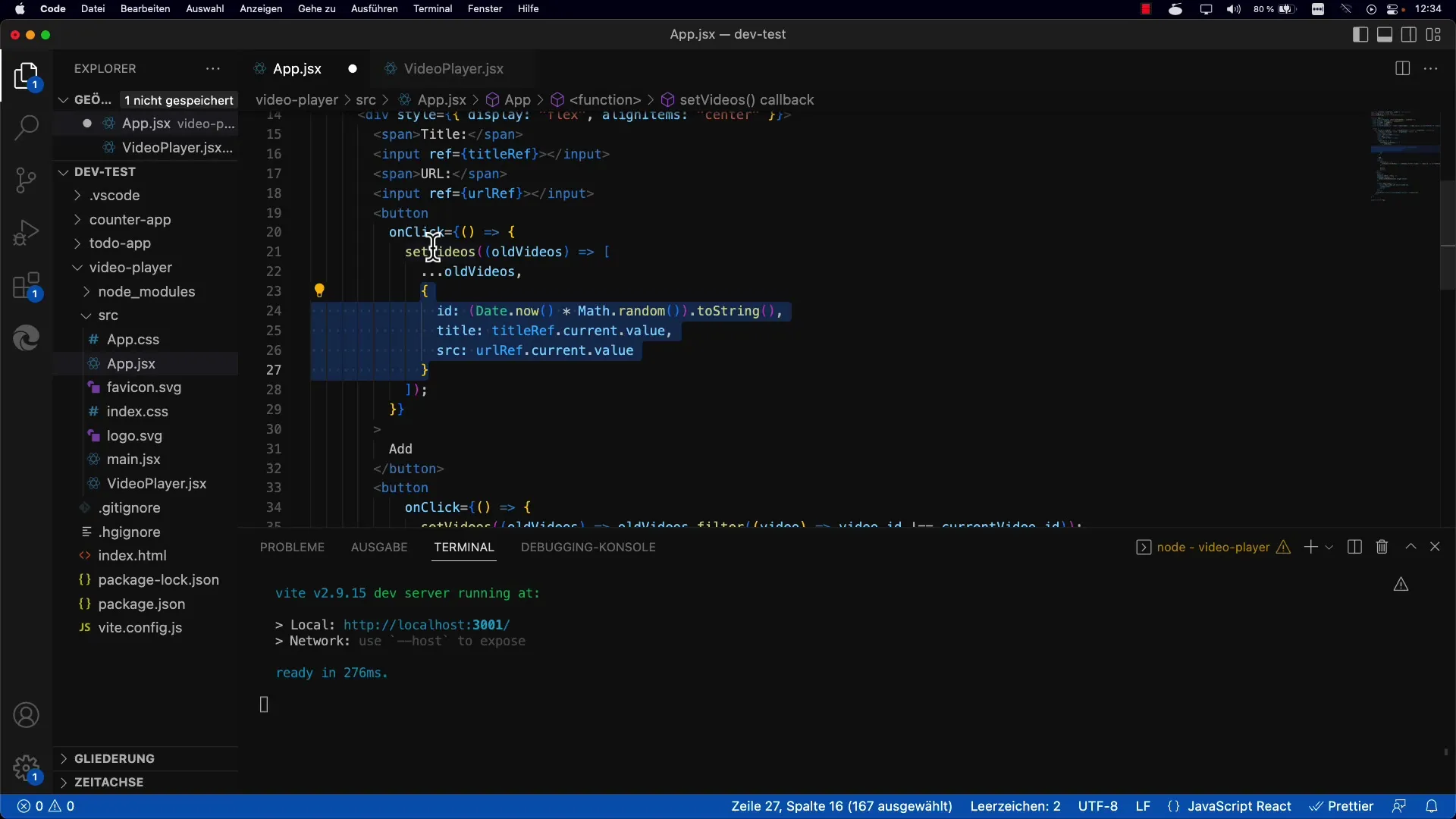
Now the actual deletion process comes into play. You use the filter method to keep all videos in a new array that do not match the ID of the currently selected video.
The condition defines that you want to keep only the IDs that are not equal to the ID of the selected video. This way, the filter method excludes the video to be deleted from the new array.
It would be advisable to save the code after implementation and test its functionality to ensure everything works correctly. You can now test the delete button by selecting different videos and trying to delete them.
A practical tip: It is possible to select the same video multiple times, so don't worry if you have many identical entries. Also, make sure to have a valid URL for the videos to test the deletion function.
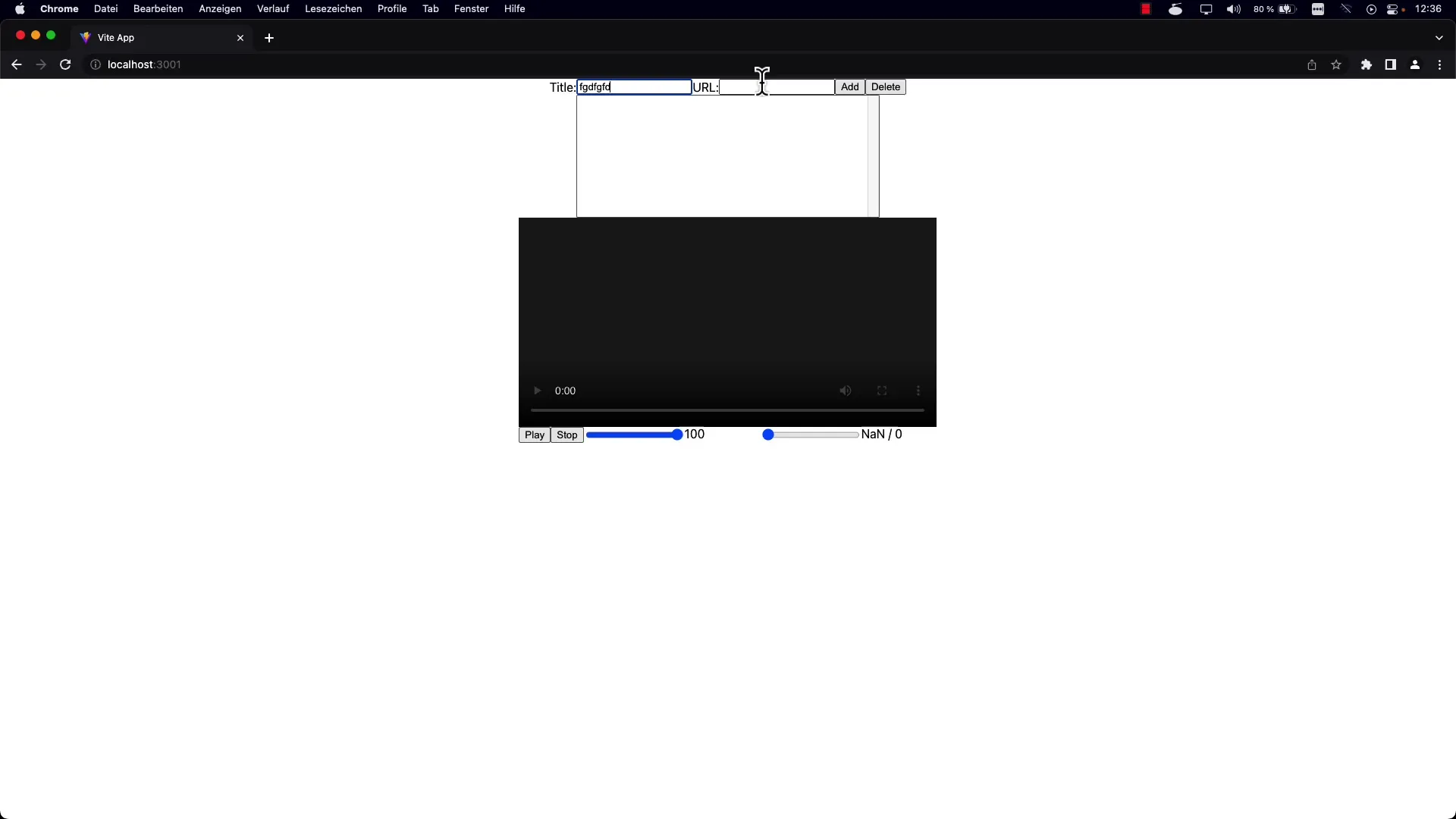
Display the deletion process and observe that pressing the button makes the video disappear. If you encounter an error, such as an undefined value, check your logic and ensure that the correct object is being used.
Experimenting with different videos and removing them with the delete button, you will see that the function works as intended.
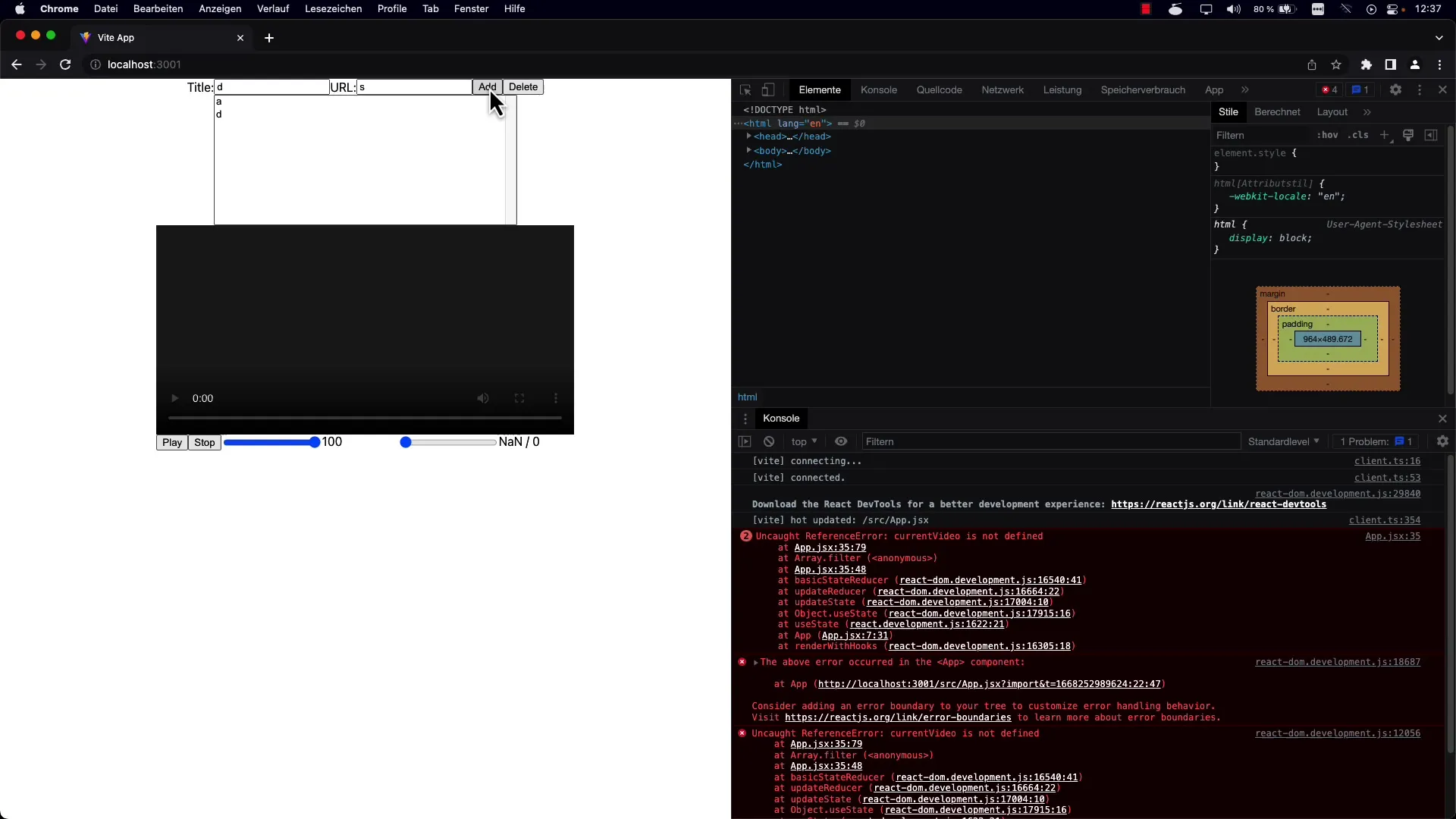
Working with IDs instead of indices simplifies the process significantly. If you were to work with indices, errors and chaos could arise when deleting entries, which would be very tedious to fix.
Another advantage of using IDs is that all other IDs remain intact, allowing for better management of your list. This helps you save a lot of time and effort and avoid issues when editing your list.
The new logic for the deletion function is now implemented. You can add videos and remove them through the delete button at any time. This flexibility is crucial for a functional application.
Now that the deletion of videos in your playlist is correctly implemented, the next step is to proceed. You can now implement the autoplay feature for your playlist so that the next video starts automatically after the current one finishes.
Summary
You have successfully learned how to delete entries from a list in a React project by applying the filter approach. By using IDs, you were able to create a robust and error-free user experience. The next challenge will be to implement the playlist logic to further enhance the user experience.
Frequently Asked Questions
How do I use the filter method in React?You can use the filter method to create a new array that contains only the elements that meet a certain condition. In this case, you are filtering out the ID of a selected video.
What are the advantages of using IDs instead of indexes?IDs help you to identify entries more uniquely, making deletion or sorting easier without unexpected shifts in the array.
How do I test the delete function?To test the delete function, select different videos and click on the delete button. Monitor if the selected videos are removed from the list.
How do I handle errors during implementation?Check your logic and variables, especially ensuring that you are accessing the right objects and not using undefined values.
What sensitivities exist in UI design when deleting entries?Make sure users are warned before deleting to avoid accidental deletion actions. A confirmation dialog can be helpful here.