It's time to optimize your video application by implementing a playlist feature that allows videos to play automatically one after another. This enhances the user experience and ensures that the user does not have to constantly intervene manually to start the next video. In this tutorial, you will learn step by step how to implement this functionality in React.
Key Insights
- You will learn how to set up an event for video playback.
- You implement the logic to determine the next video in the playlist.
- You add the necessary states and props to control the interactions.
Step-by-Step Guide
To implement the playlist functionality, follow these steps:
Step 1: Add Event Listener for Video End
First, you must ensure that your video player fires an event when a video is fully played. We use the onEnded event of the video element for this purpose.
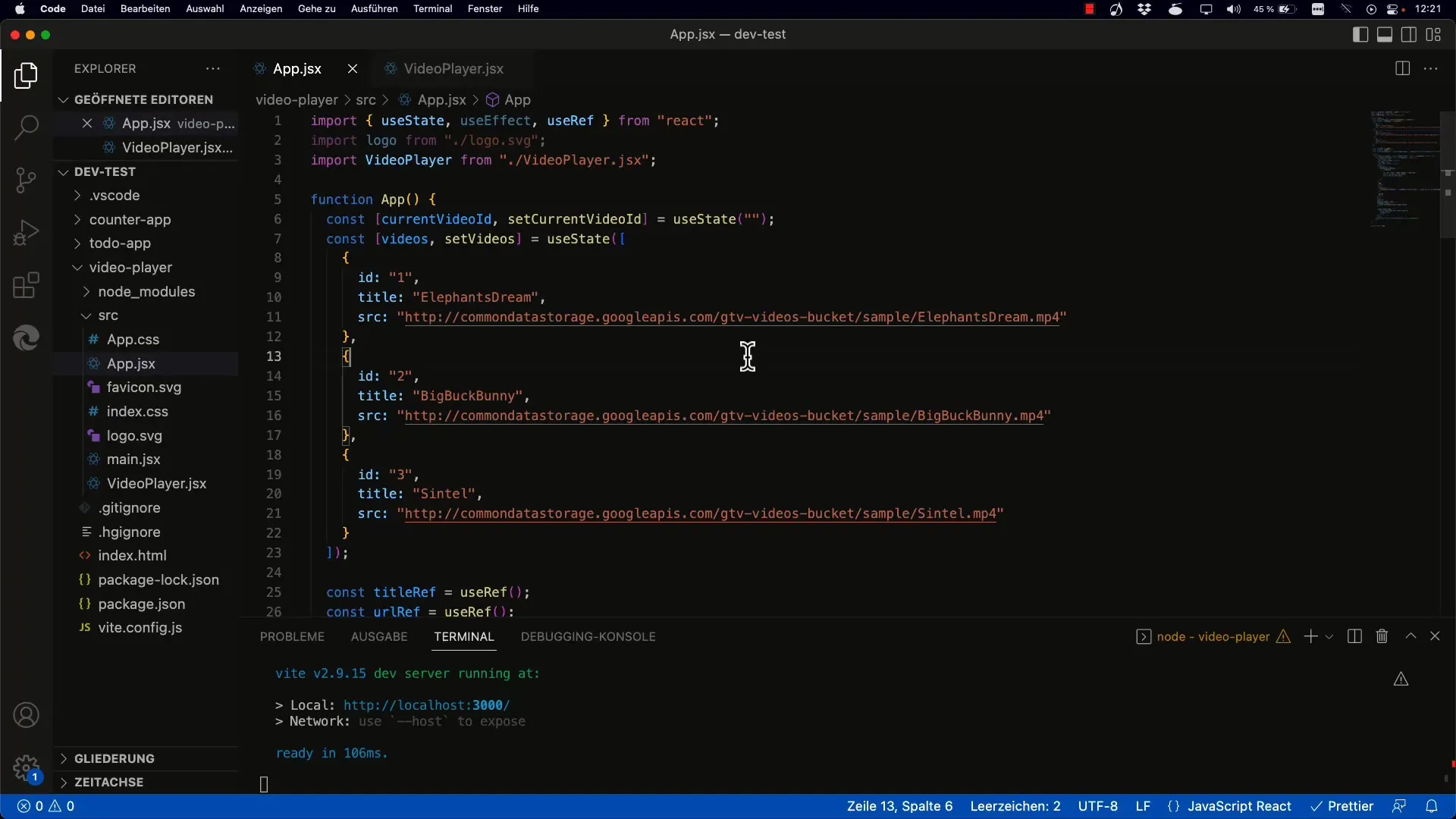
In your component file, add an event handler for the onEnded event. This handler will be forwarded to the parent component.
Step 2: Implement Logic to Determine the Next Video
Now that we know when a video ends, we need to find out which is the next video in the playlist. To do this, we check the index of the current video and increment it by one.
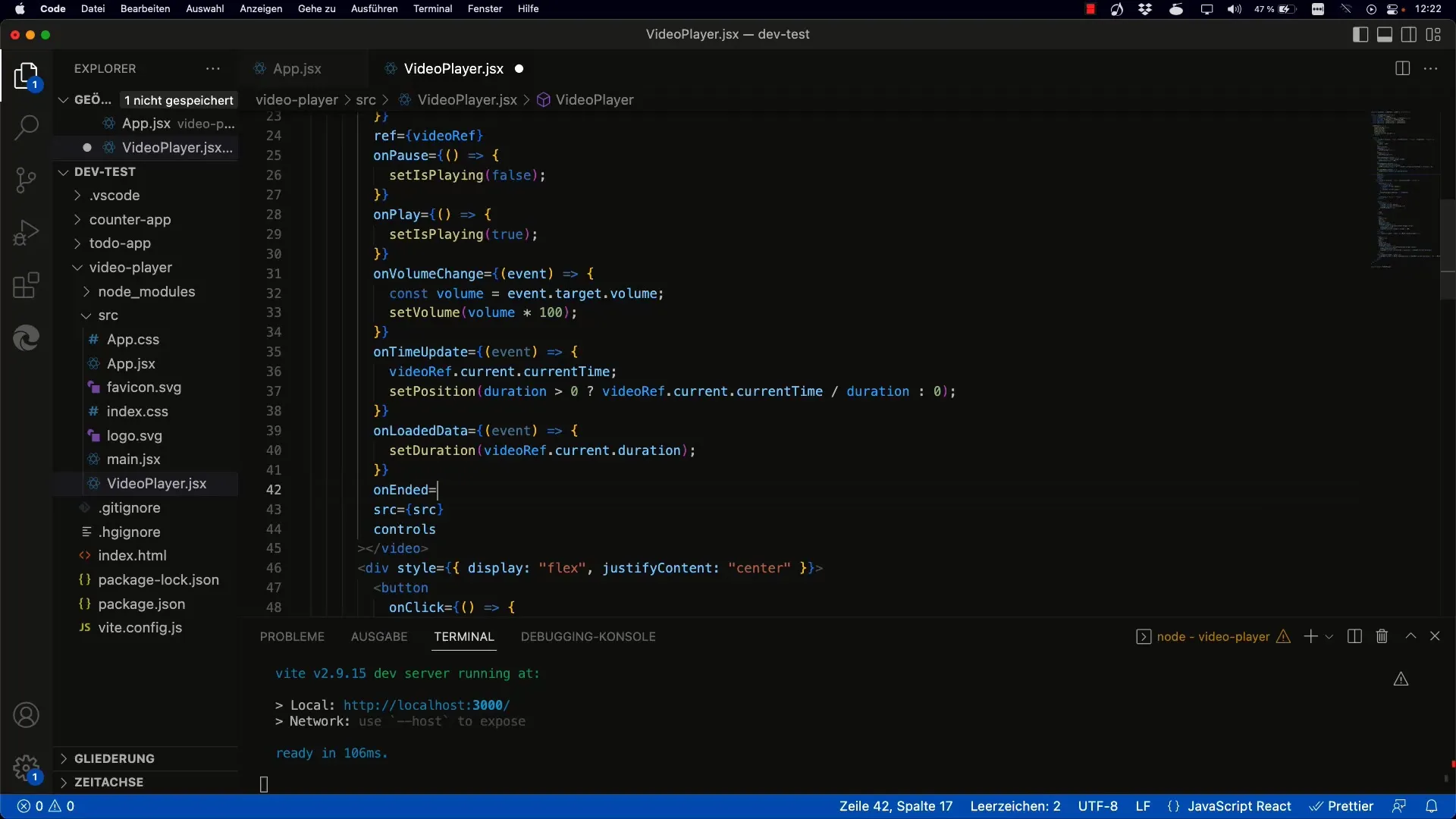
Once we have determined the next index, we must ensure it stays within the limits of the playlist.
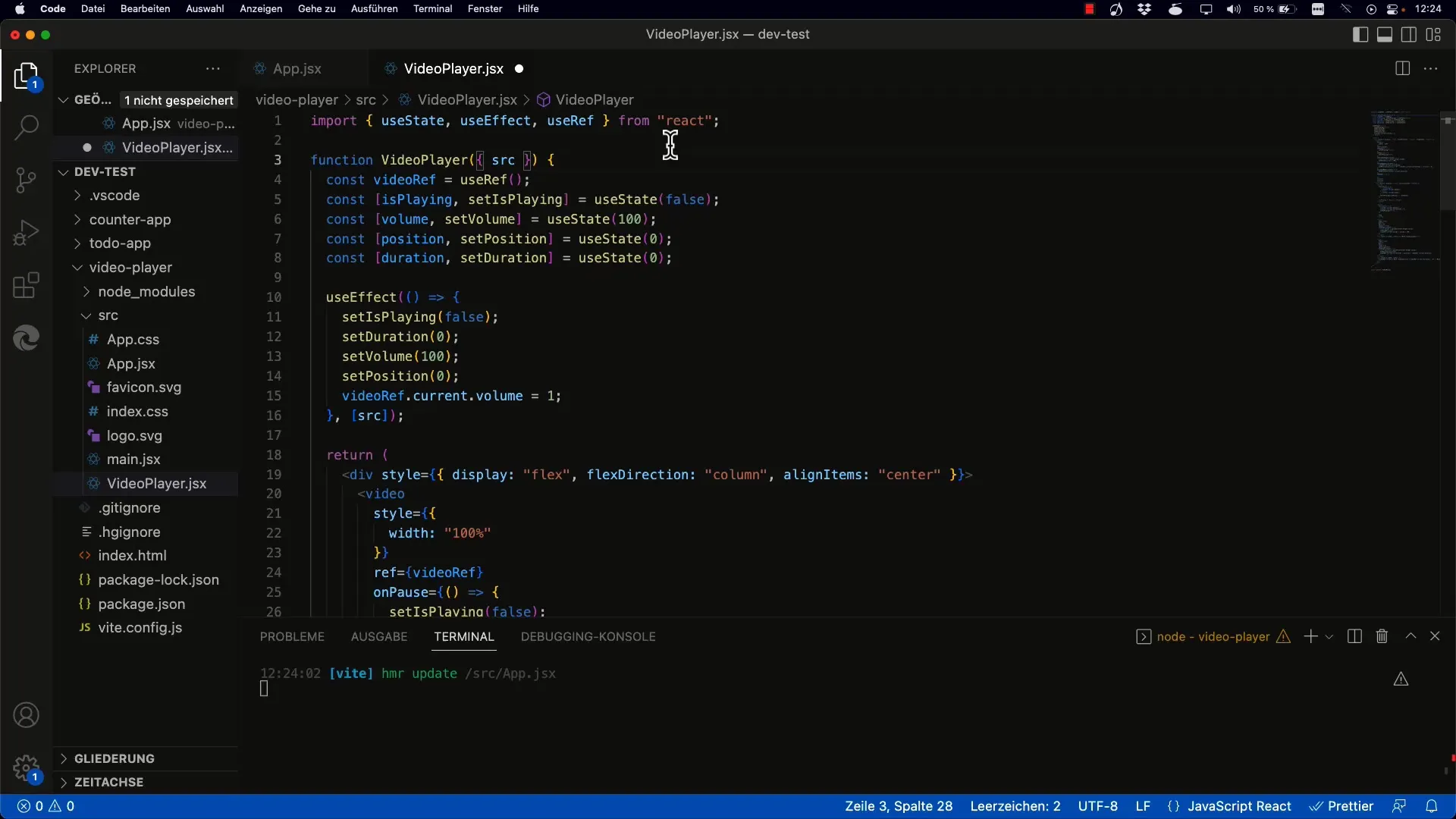
Step 3: Setting the Current Video
After determining the next video, we set the ID of the current video to the ID of the next video using the state management function setCurrentVideoID.
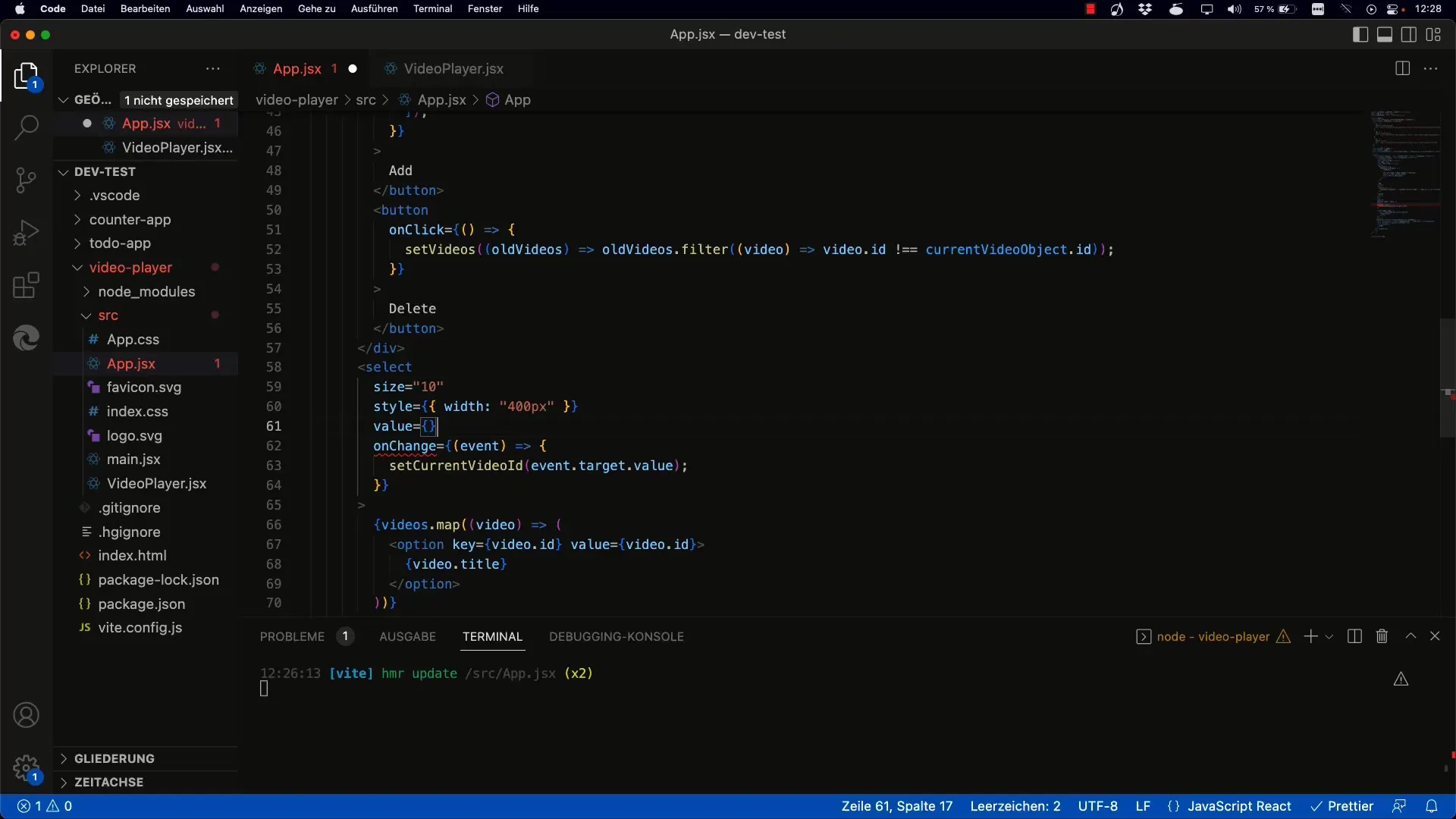
The value for the Select element (the dropdown showing the videos) is also updated accordingly.
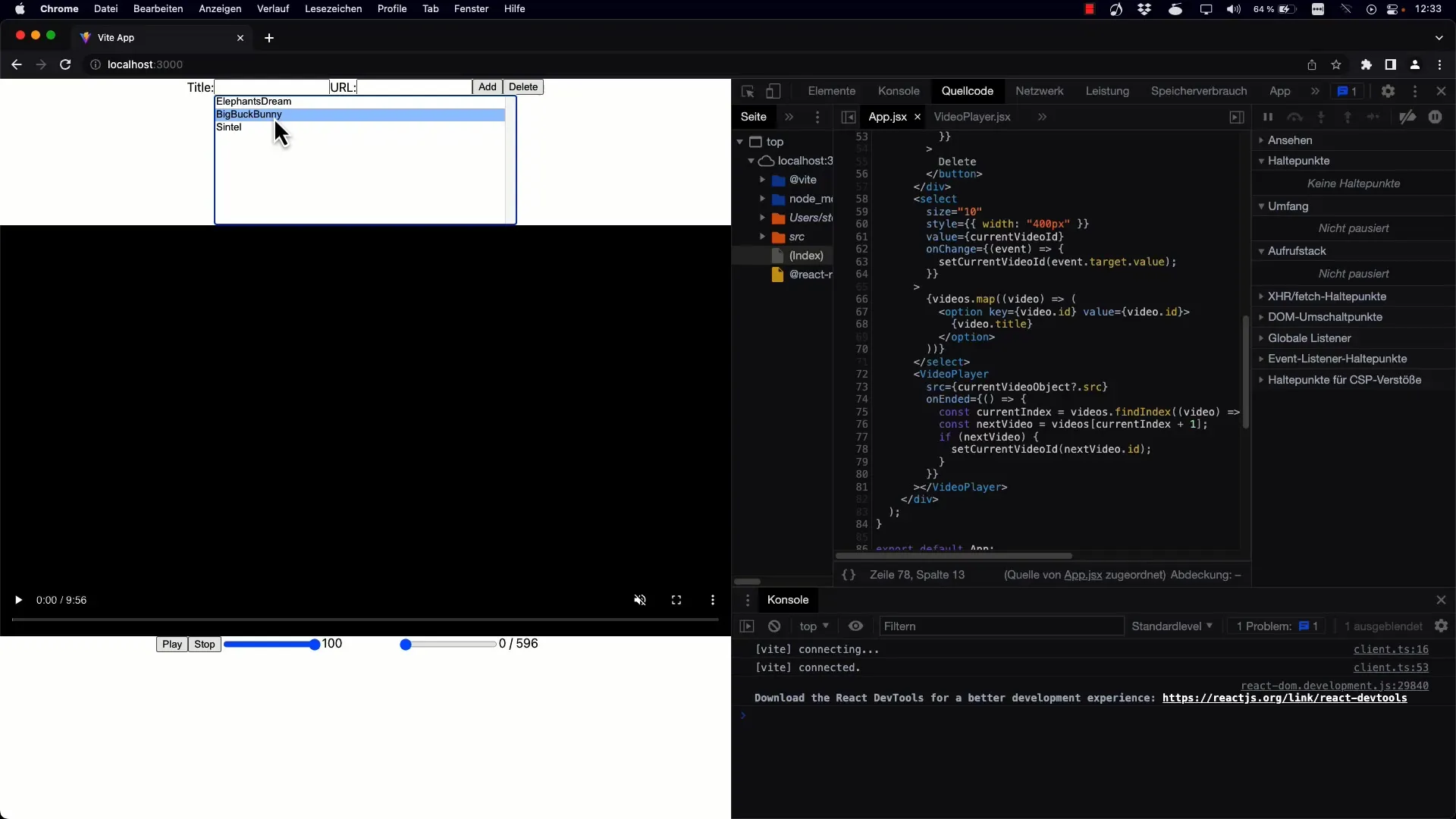
Step 4: Automatically Play the Next Video
To ensure that the next video plays automatically, we must implement the shouldPlay logic. If the next video is set, the shouldPlay state should also be set to true.
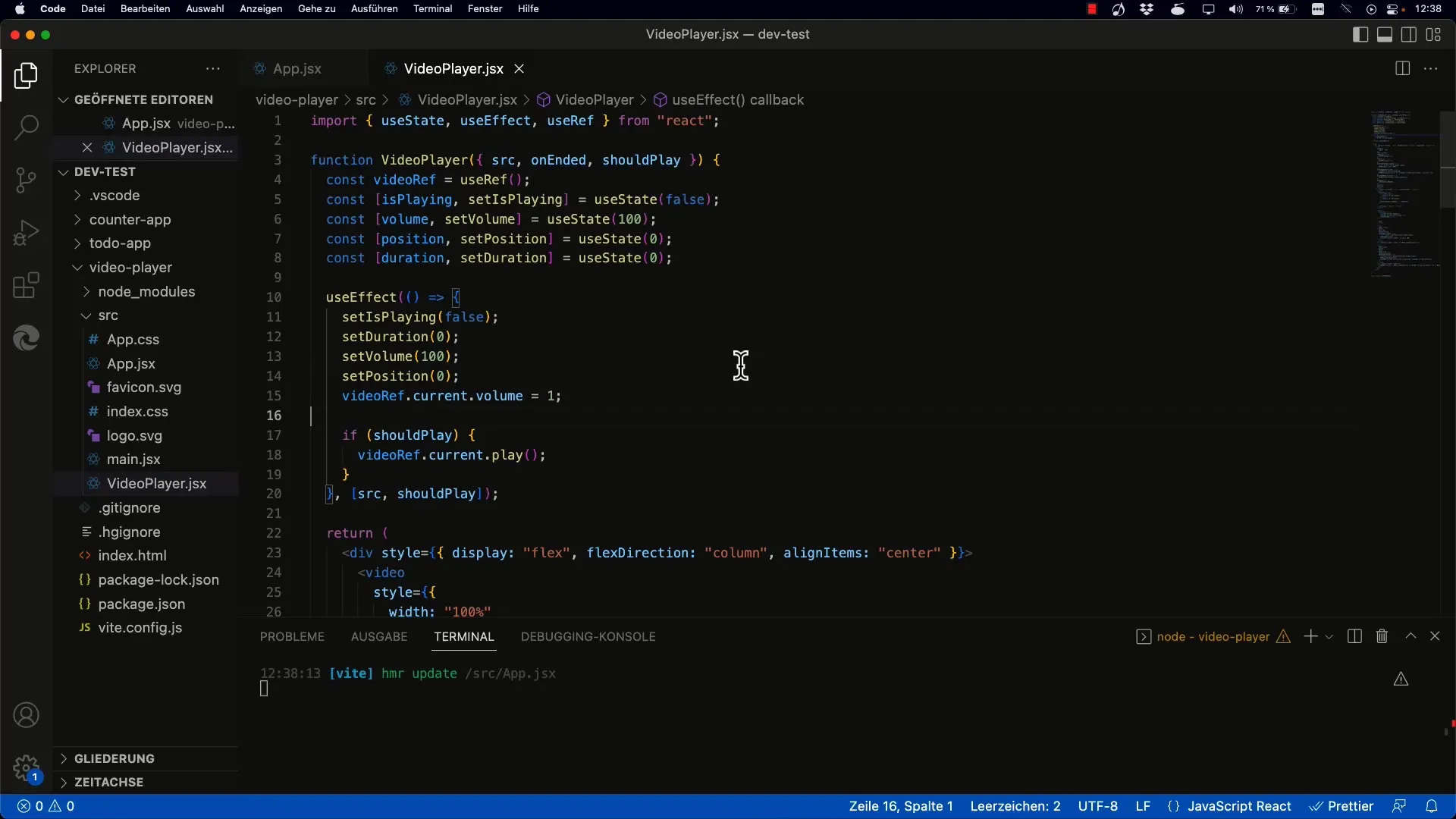
To do this, you add a new State object and check in useEffect if the shouldPlay value changes.
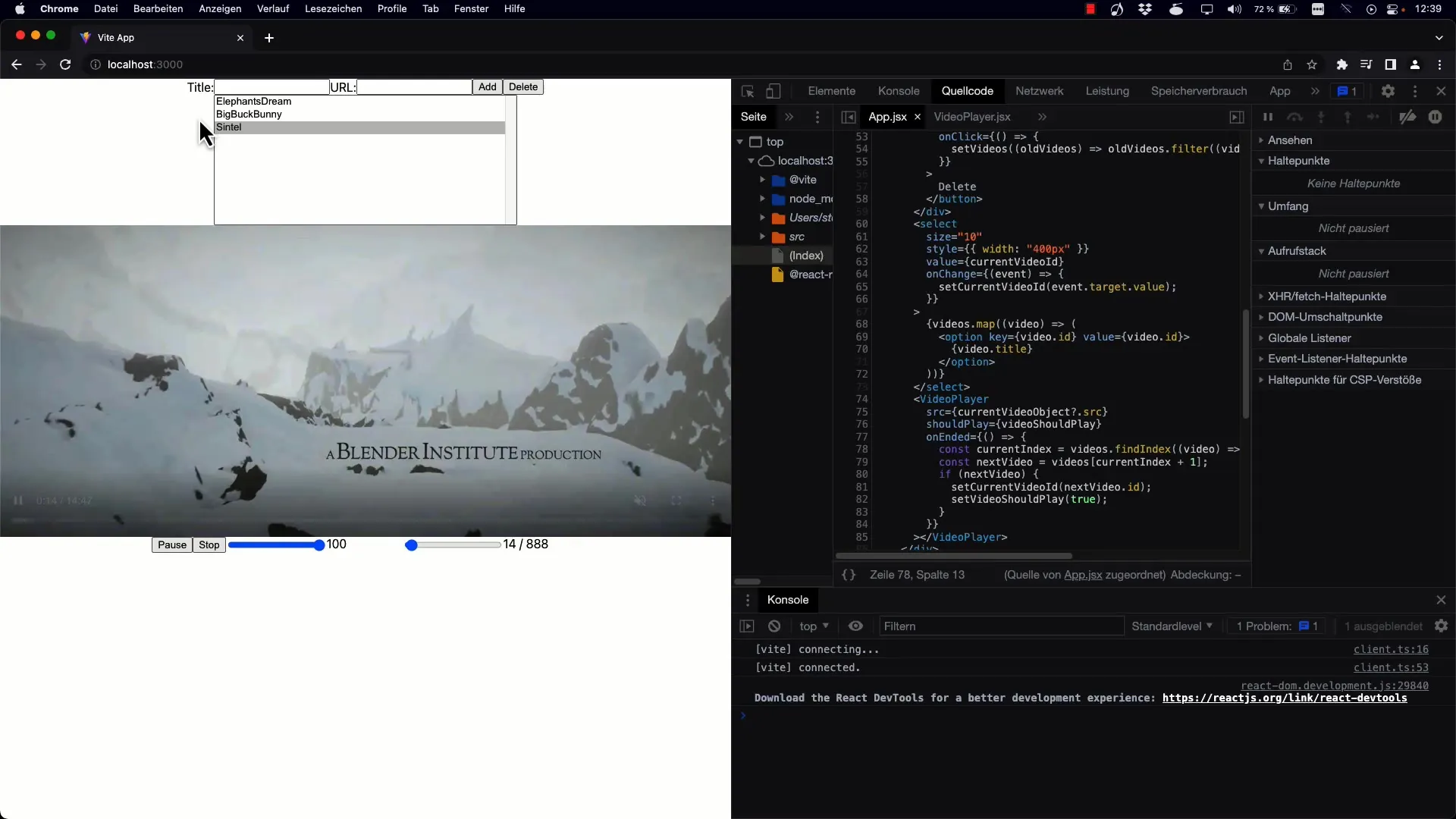
Step 5: Checking the Functionality
You can now test if the functionality works as expected. Start playing a video and fast forward it close to the end. You should see that the next video starts automatically.
Step 6: Pause and Play Functions
It is important to also implement the pause function so that the user can interrupt the playback. Make sure that the logic for shouldPlay is appropriately handled when switching between videos.
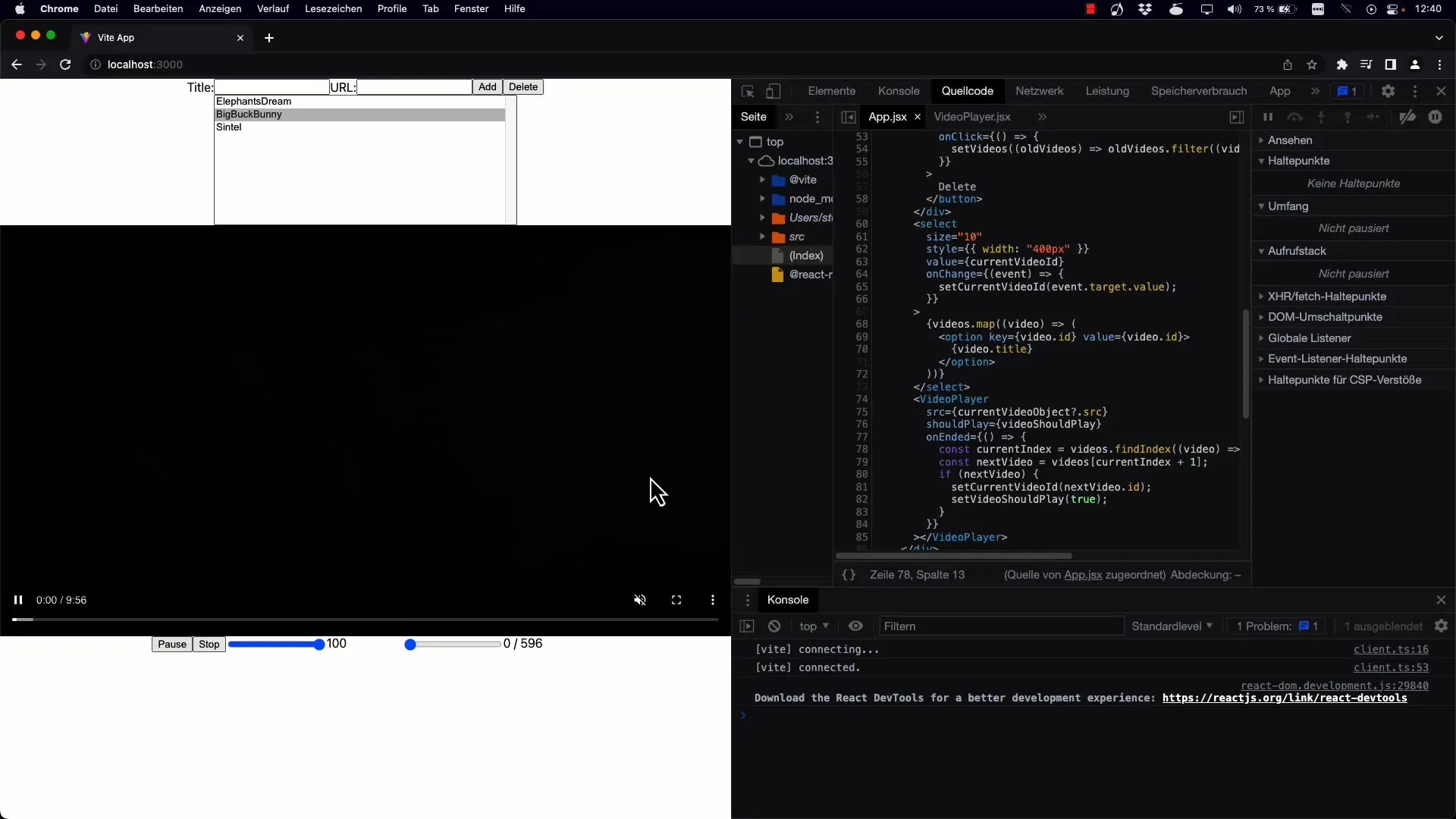
Summary
In this tutorial, you have learned how to implement a playlist feature for a video application in React, from handling events to determining the next video to implementing automatic playback – you have learned all the necessary steps.
Frequently Asked Questions
How can I add more videos to the playlist?You can dynamically expand the list by adding new video objects to the state as needed.
What happens when I reach the end of the playlist?When the last video finishes playing, the playback will stop and no further video will be automatically loaded.
Can I manually select the current video?Yes, you can manually select the current video from the list. The dropdown allows you to switch between different videos.
How can I pause the playback?By using an appropriate button that changes the isPlaying state, you can pause the playback.
Which React Hooks have been used in this tutorial?This tutorial mainly used the useState and useEffect hooks to manage the state and side effects.