Integrating local storage into your React app allows you to store data between sessions and thus create a seamless user experience. In this tutorial, I will show you how to store and load a playlist of videos in your video player by using the browser's local storage. We will follow the method we have already used with the to-do app.
Key Insights
- Use of localStorage for persistent data storage.
- Implementation of useEffect to manage saving and loading data.
- Simple methods to add and remove videos.
Step-by-Step Guide
1. Setting Up the State
Start by setting up the basic state for your video list in the main component of your app. This can be done similarly to how you did it in the to-do app.
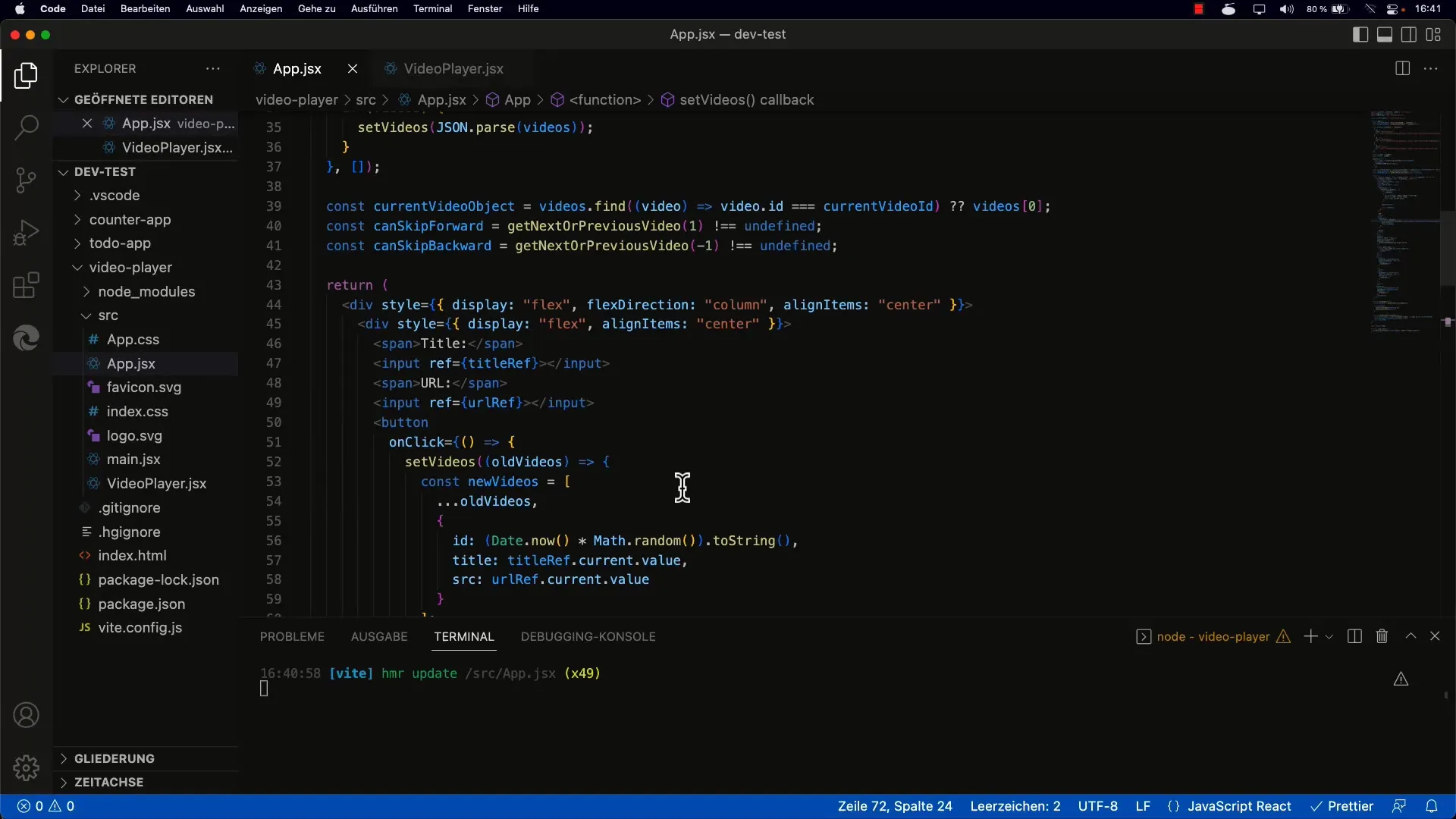
Here you define an array that will store the videos in your playlist.
2. Implementing useEffect
Use the useEffect hook to load the videos from local storage when the component first renders.
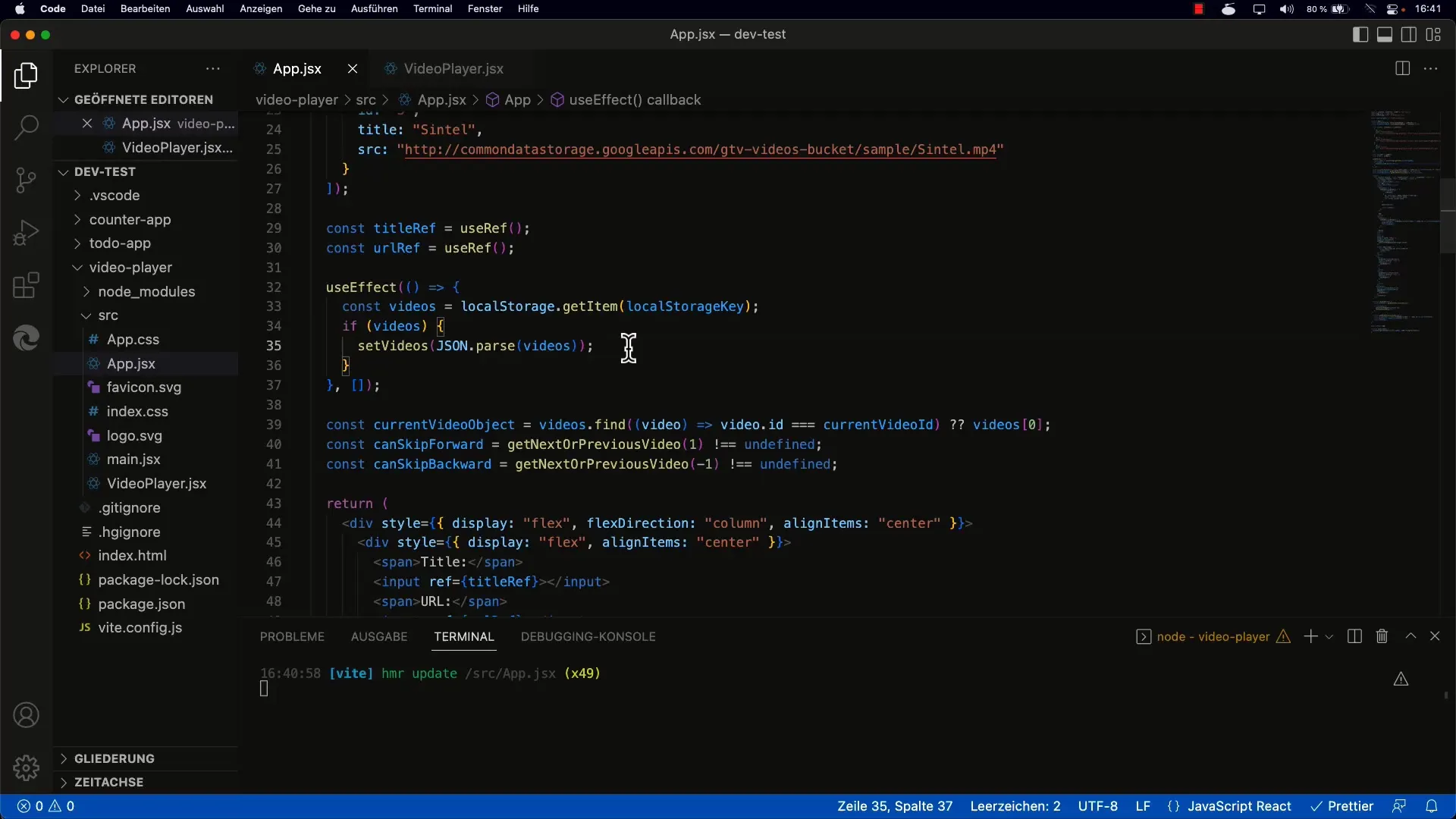
It is important to use the correct key here to store and retrieve the data in local storage.
3. Loading the Videos
With localStorage.getItem, you can retrieve the stored string and convert it into a JavaScript array using JSON.parse.
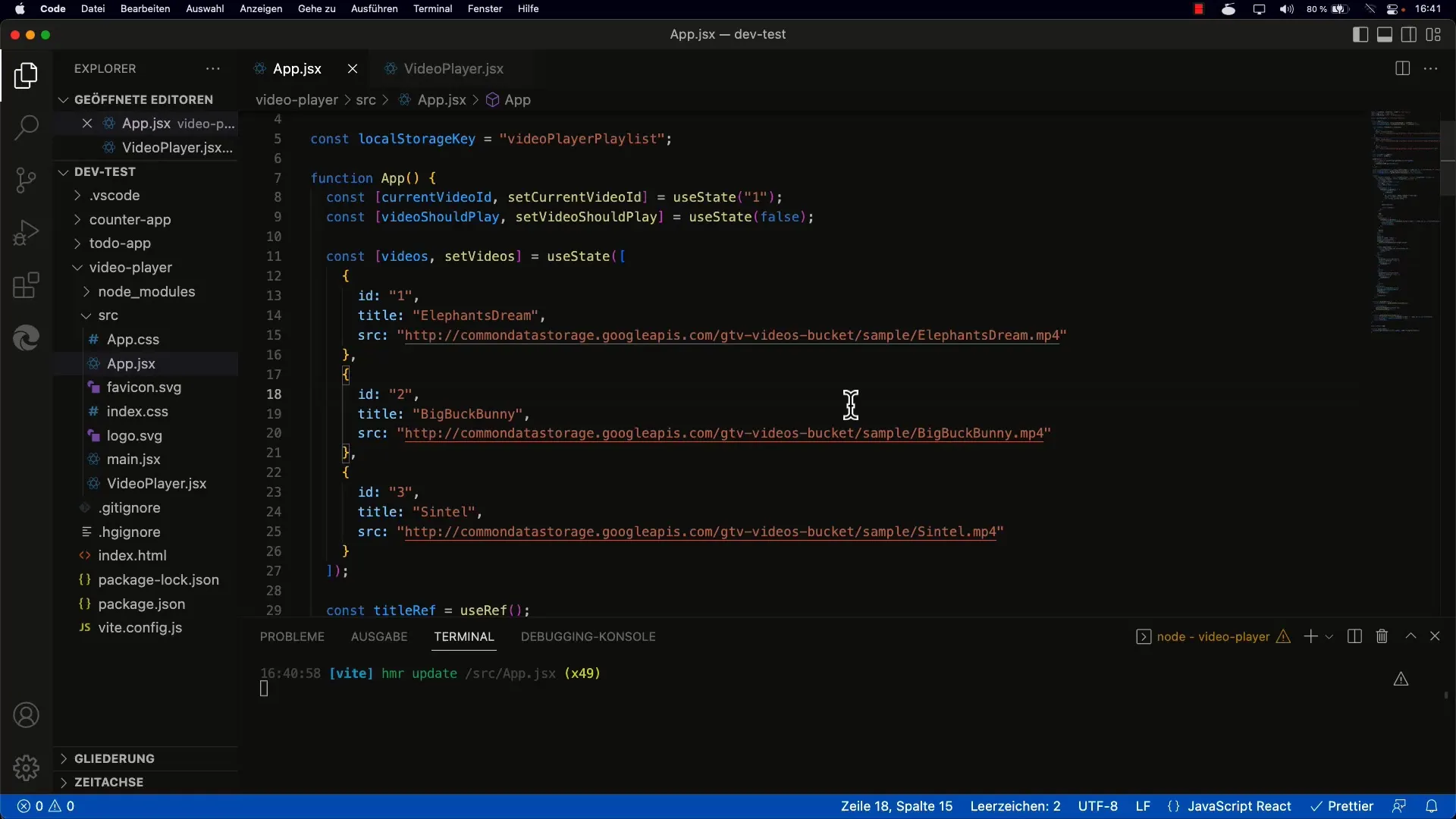
Make sure to check if the item actually exists before trying to parse it to avoid errors.
4. Creating a Function to Save the Videos
Create a function that writes the current video list to local storage whenever the array changes. To do this, use localStorage.setItem along with JSON.stringify.
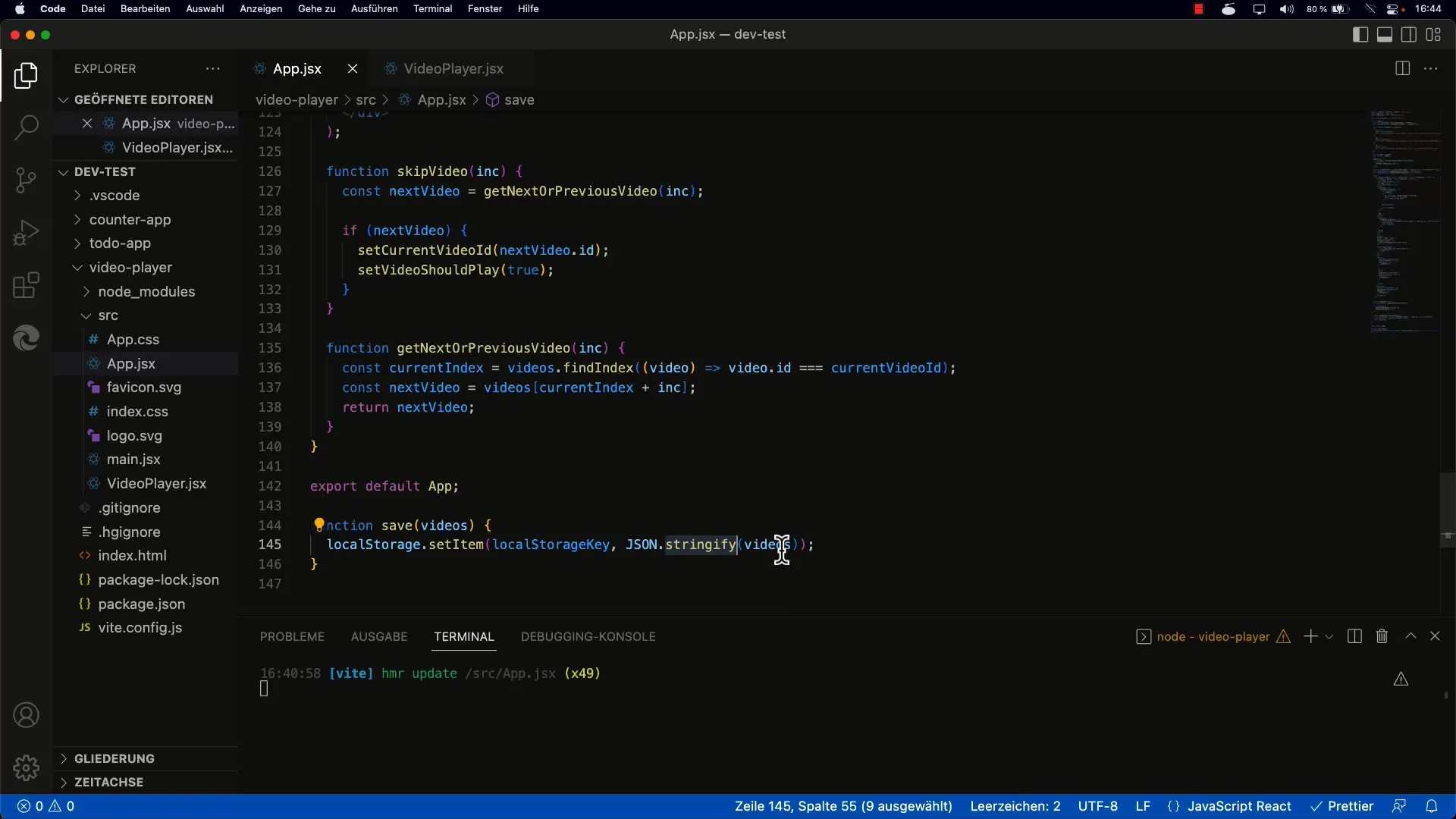
Through this function, you can ensure that your list is saved whenever a video is added or removed.
5. Adding a New Video
Add a button to add new videos to the list. When clicking this button, call the storage method mentioned earlier to save the updated list in local storage.
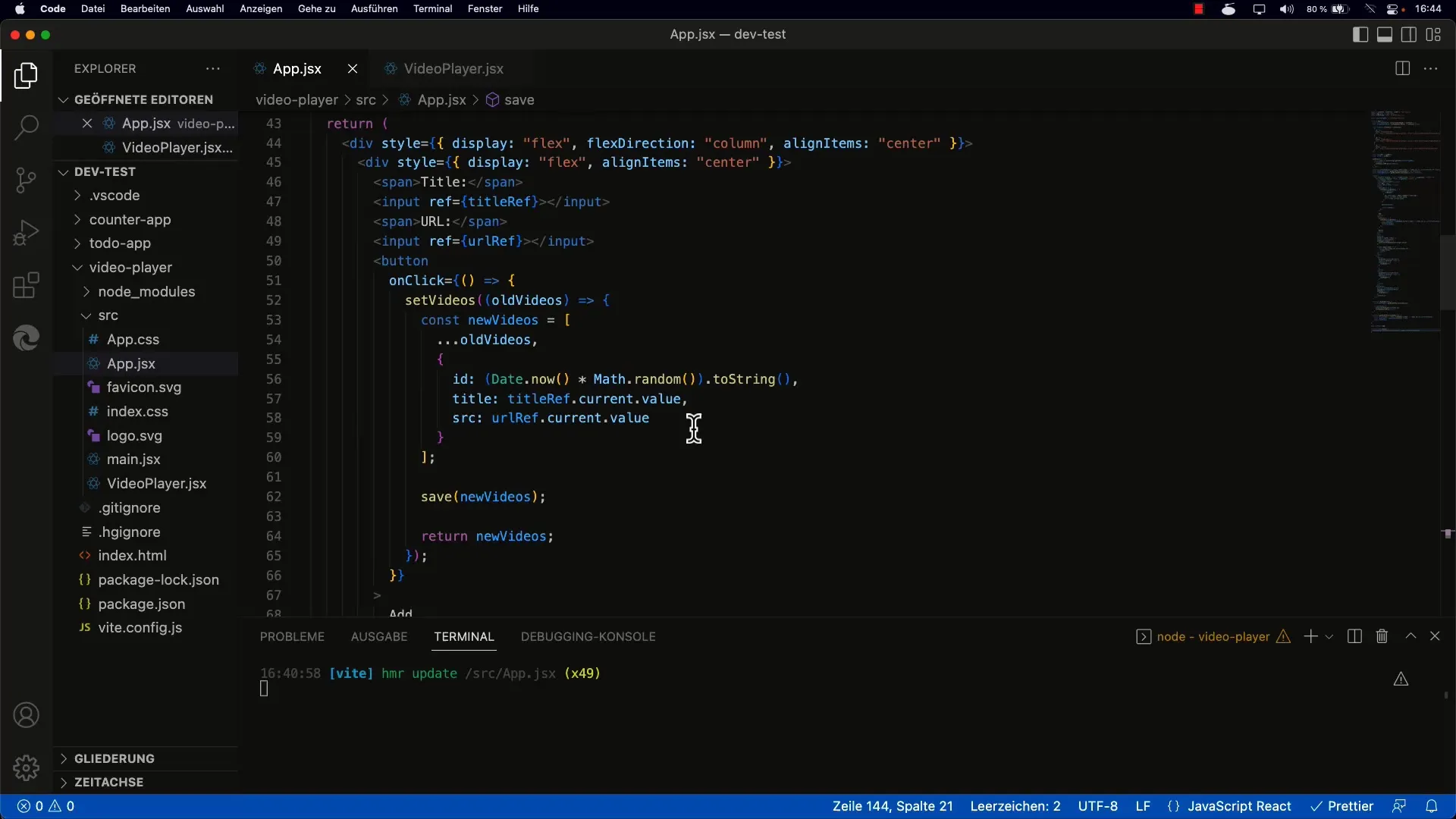
It is important that the function creates and stores the new array with the new entry.
6. Removing Videos
Implement a mechanism to delete videos. Here too, update the stored list and then call the storage method.
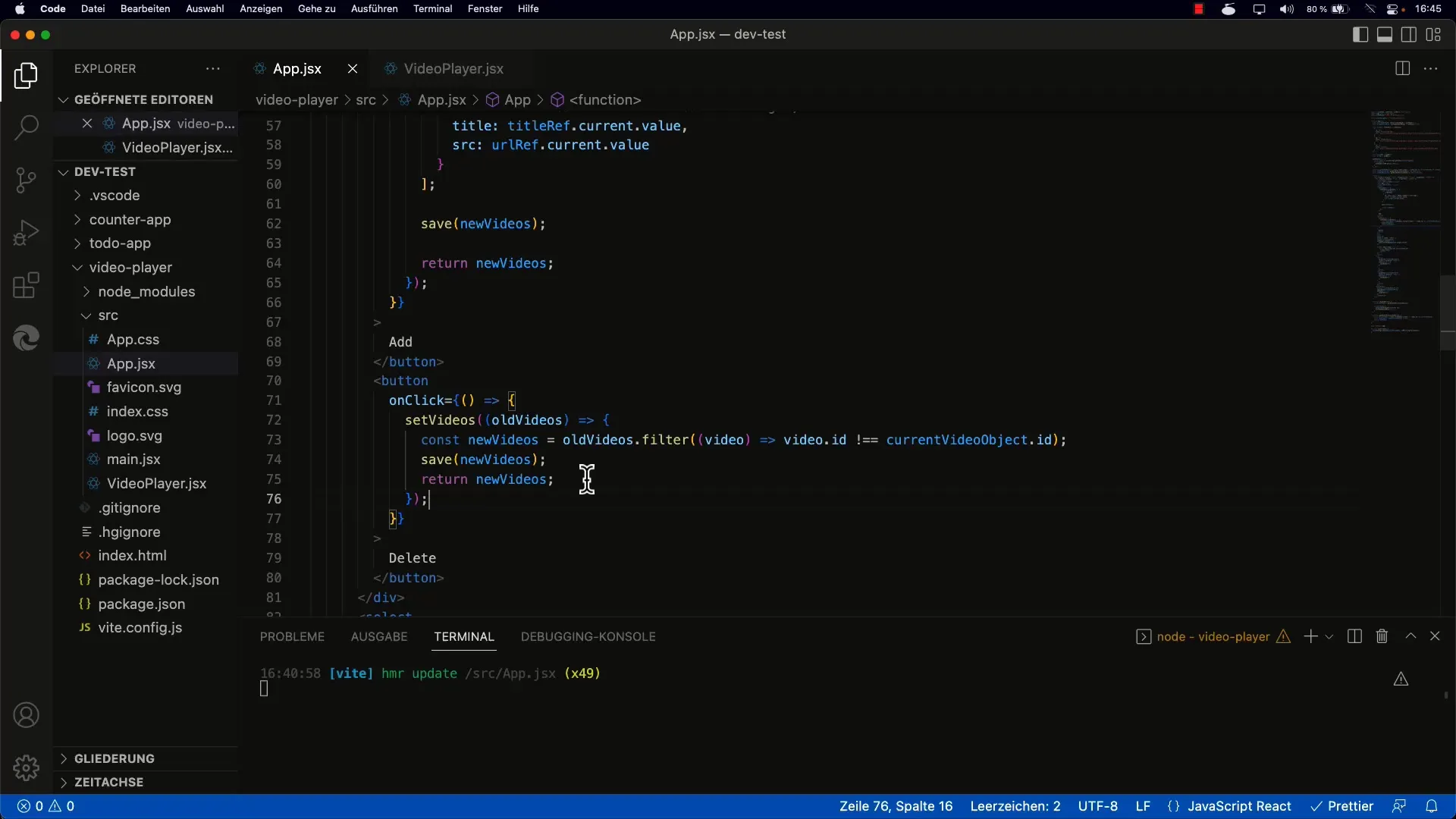
Develop a clear and user-friendly way for the user to remove videos from their playlist.
7. Test Your Implementation
Once the basic functions are implemented, test if the saving and loading of data work as expected. Add some items, reload the page, and check if the list is preserved.
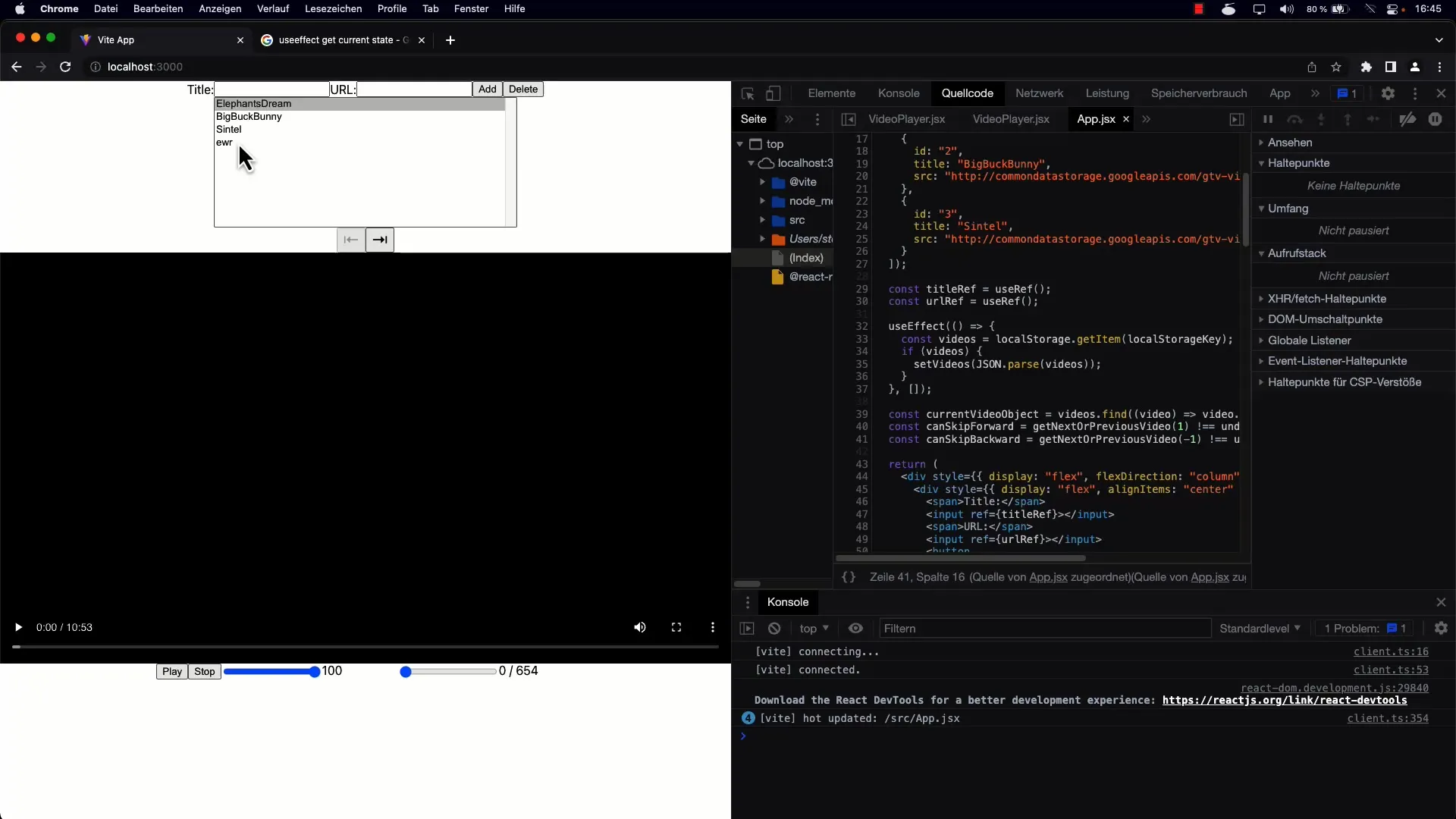
If everything is correctly configured, you should be able to see the videos even after reloading the app.
8. Examining the Local Storage
Check your browser's local storage to see how the data is stored.
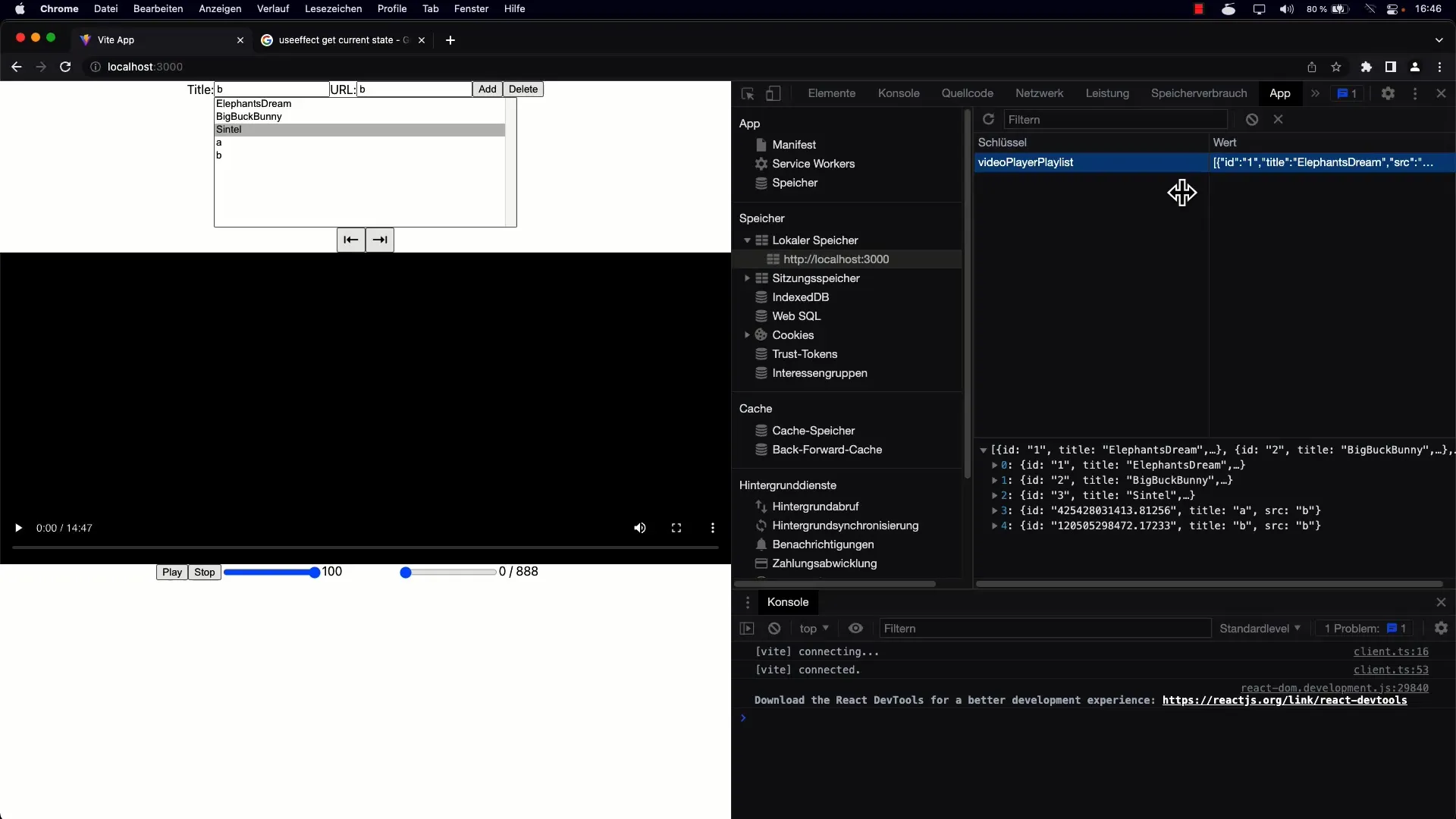
Here you can view the stored string, and you should recognize the structure you used for saving the videos.
9. App Extensions
You could consider integrating additional functions for editing entries, or supporting multiple playlists. Think about how you can improve the user experience.
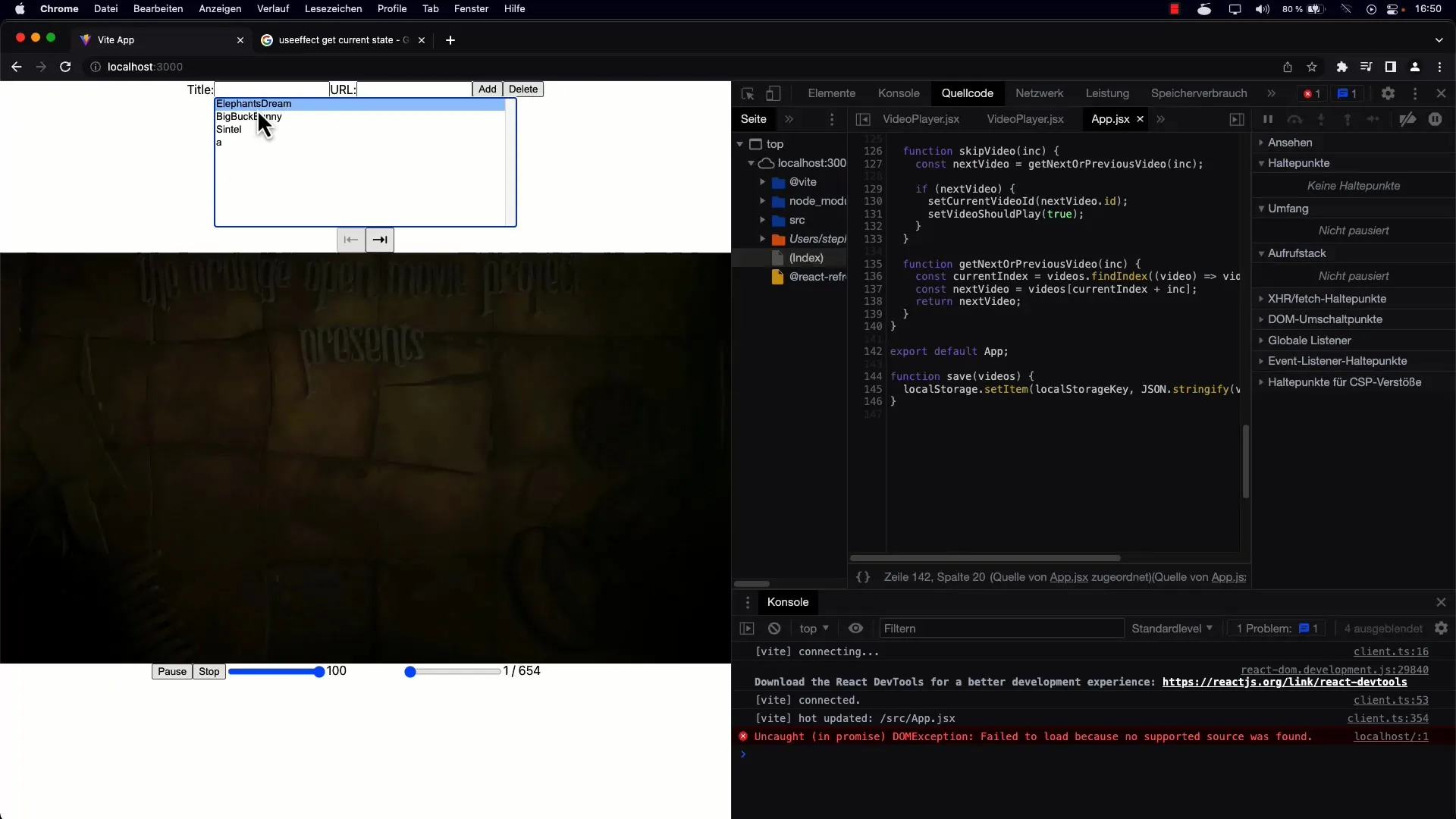
Additional features could include editing and supporting multiple lists.
Summary
Implementing persistent data storage for your video list enables a better user experience and ensures that user data remains even when the app is closed. While these techniques are simple, they provide a solid foundation for developing more complex applications.
Frequently Asked Questions
How do I save my list permanently?Use localStorage.setItem to save the list after it has been modified.
What happens if I reload the page?The list will be loaded from local storage, so all changes will be preserved.
Can I create multiple playlists with the app?Yes, you can expand the logic to manage and save multiple playlists.