Oftentimes, the rendering process in React applications is a crucial factor for performance. When components are rendered unnecessarily, this can lead to a noticeable slowdown in the application. To avoid this issue, React provides hooks like useCallback. In this tutorial, you will learn how to optimize the rendering performance of your components using useCallback, especially when using callback props.
Key Takeaways
- useCallback stores a function between render cycles.
- Correct usage of useCallback reduces unnecessary renderings.
- When using useCallback, attention should always be paid to the dependencies.
Step-by-Step Guide
1. Introduction to Callback Props
To understand how useCallback works, you should first clarify the meaning of callback props. Callback props are functions passed to child components. In our example, we have a simple button component that receives a function as a prop to perform an action.
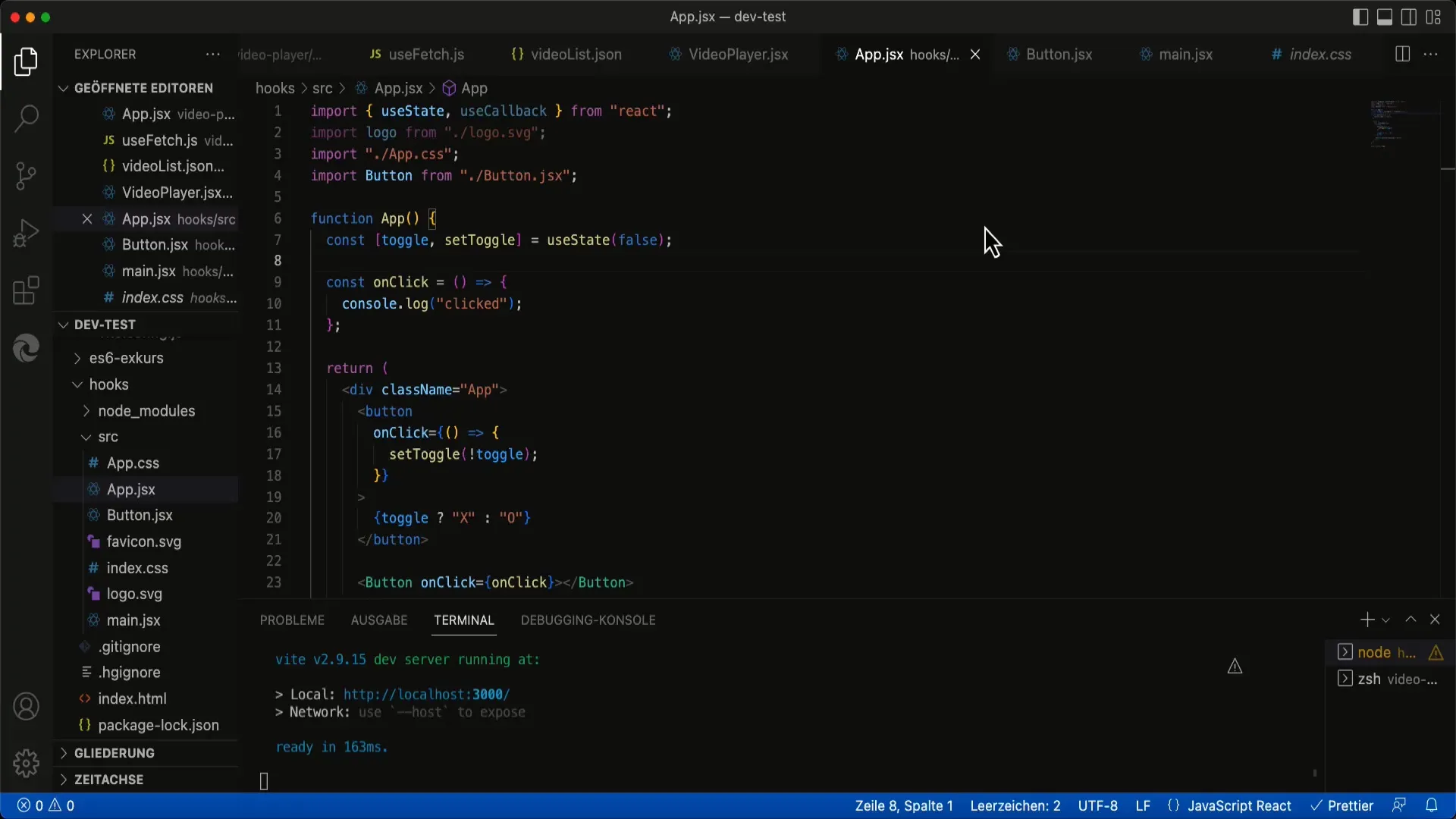
With this structure, imagine that when rendering the child component, the callback function is repeatedly regenerated. This means that every time the parent component is re-rendered, the callback function is changed, even if its logic remains the same.
2. Implementation without useCallback
Let's say you have already created your button component without using useCallback. In this case, your code may look like this: you declare the onClick function directly in the parent component. Now if the state of the parent component changes, the button gets rerendered, and the callback function is recreated.
3. Introducing useCallback
This is where useCallback comes into play. With useCallback, you can "cache" your callback function so that it is regenerated only when the specified dependencies change. To correctly use useCallback, you need to wrap your callback function in the hook.
This ensures that the original function is remembered as long as the dependencies remain unchanged. This means that during repeated rendering of the parent component, the old function is always retained as long as the dependencies do not change.
4. Specifying Dependencies
This is also the crucial point when using useCallback. You must make sure to define the correct dependencies in the empty array. If you have bound the function to variables, these variables should be in the dependencies array.
When changes in the state occur, React will understand that the function needs to be regenerated because one of the specified variables has changed.
5. Testing the Implementation
To verify that the implementation works, you can test the app in the browser. If you click the button without using useCallback, you will see that the button and other components are repeatedly rerendered.
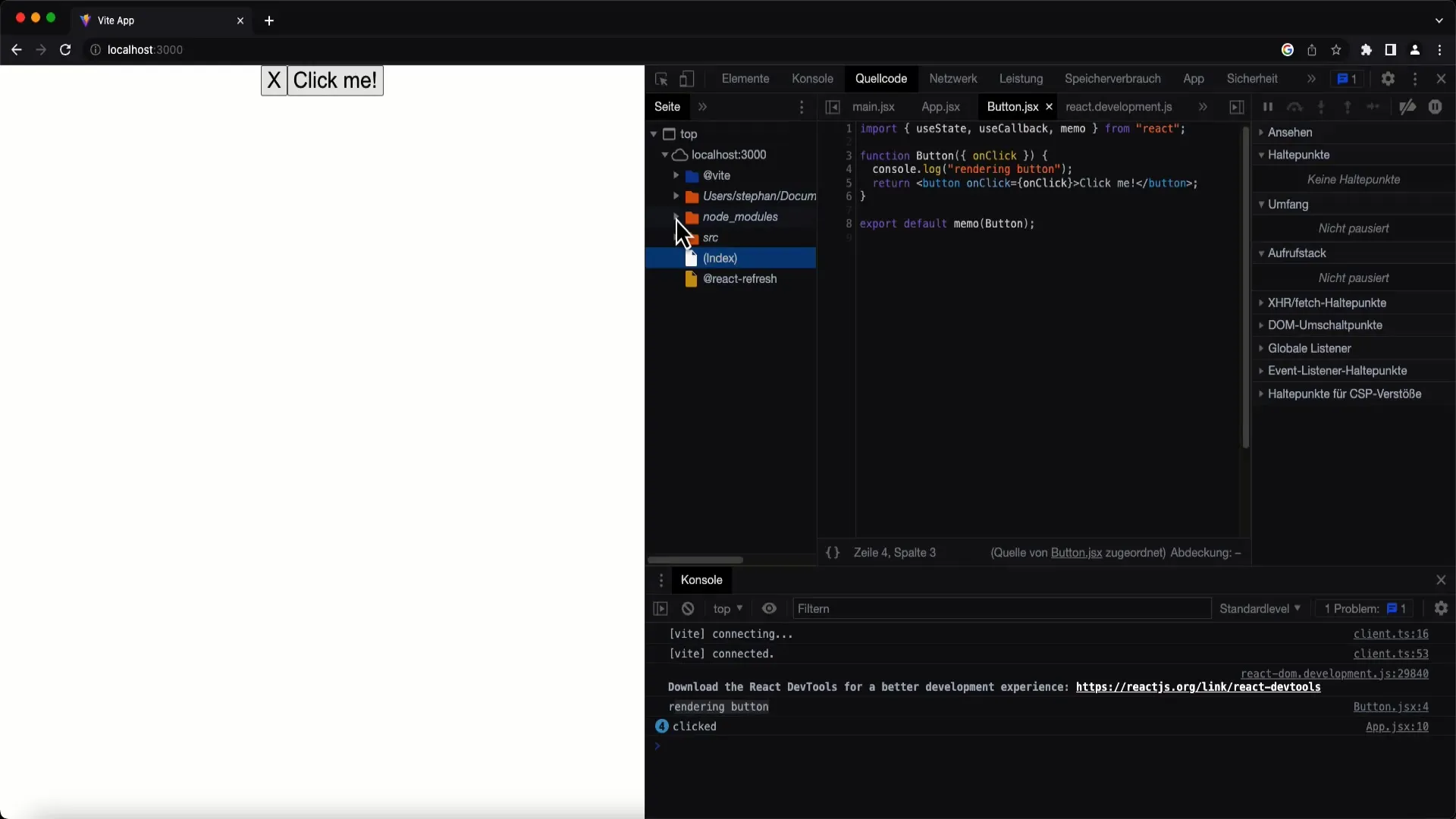
Now add useCallback and observe the performance. If everything is correctly implemented, the rendering of the button should stop when the prop no longer changes.
6. Weighing the Pros and Cons
It is important to weigh the use of useCallback. In many cases, its use requires additional effort, and it may be that the optimization is not necessary in simpler components. Therefore, consider whether it is worth using useCallback based on the complexity of your components.
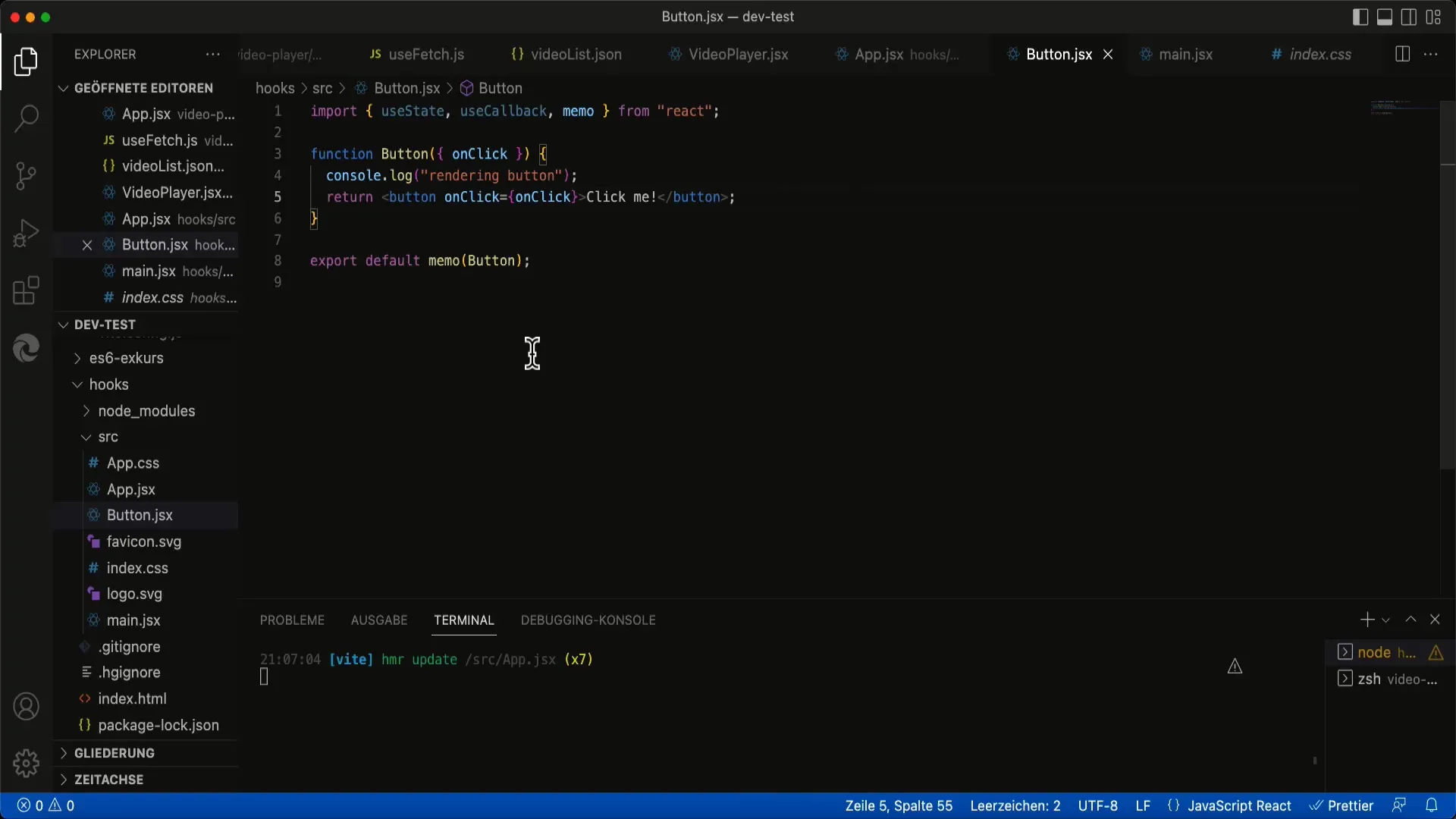
Keep in mind that useCallback really brings a benefit when also using memoization optimization. Otherwise, you may just be accessing the same callback function while your component is still being repeatedly rerendered.
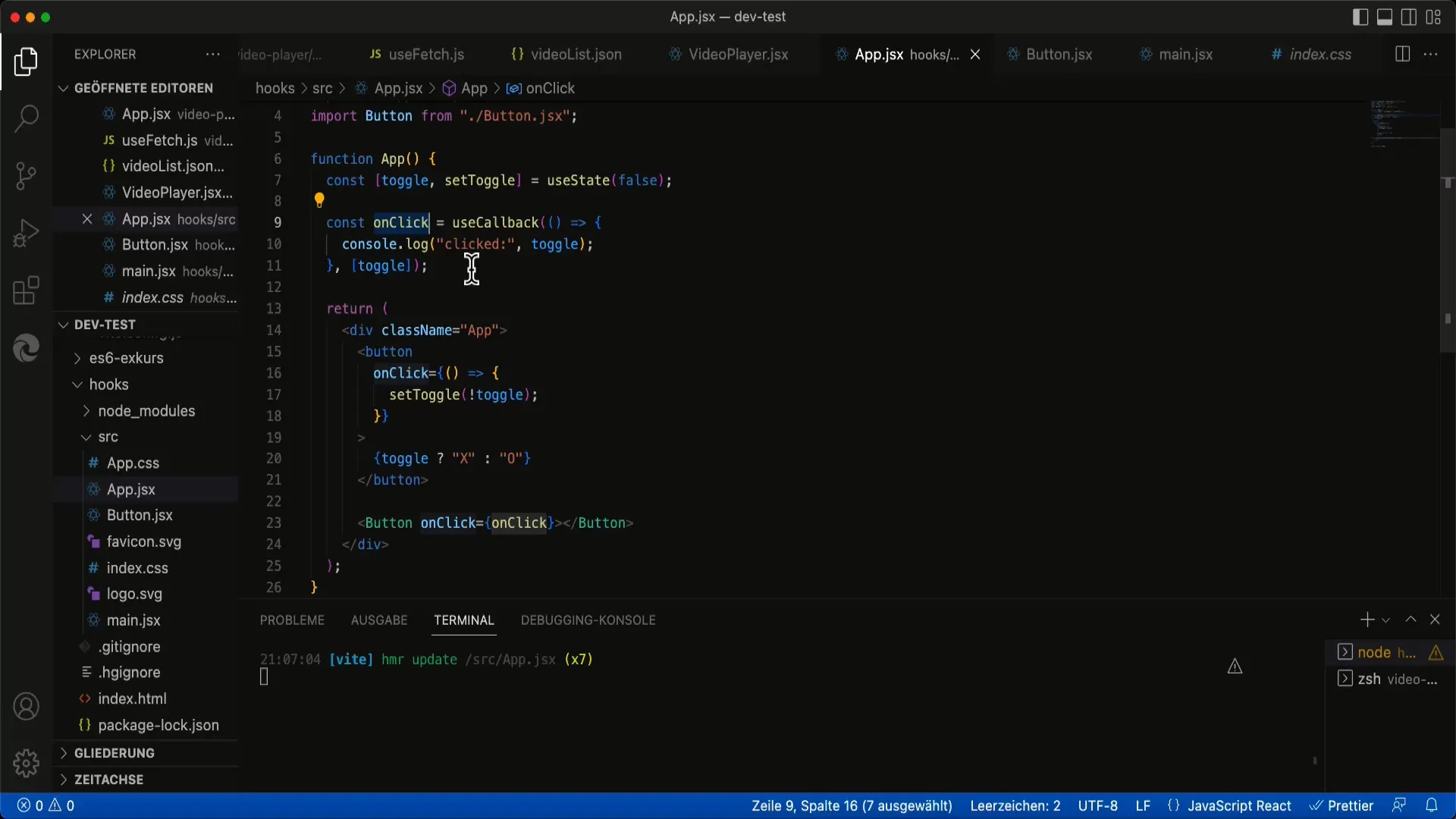
Summary
Implementing useCallback allows you to avoid unnecessary renderings and optimize the performance of your React application. Always make sure to define the correct dependencies and assess the use of the hook according to the complexity of your component.
Frequently Asked Questions
How does useCallback work?useCallback stores a function between render cycles and only recreates it when a specified dependency changes.
When should I use useCallback?Use useCallback when you have callback props that cause unwanted renders in child components.
Do I always need useCallback for every function?No, you should only use useCallback when it benefits the performance of your application, especially with more complex and frequently rendering components.
Which dependencies should I specify?Specify all variables used in your callback function that can change in the dependencies array.
Can I use useCallback alone?Not necessarily. A combination with other hooks like React.memo is often recommended to achieve the desired performance improvements.