Rendering components in React can often lead to unnecessary performance issues, especially when components are being updated repetitively even if their props have not changed. Here's how you can optimize the performance of your React applications using the memo function of React. With memo, you can ensure that components are only re-rendered when relevant data changes. This not only speeds up the user interface but also improves responsiveness to user input.
Key Takeaways
- The memo function prevents unnecessary re-rendering processes.
- Components are only re-rendered when their props change.
- Optimization is advisable when the component has complex calculations or subcomponents that need to render.
Step-by-Step Guide
Step 1: Basic Setup
To start optimizing components, you need a simple example. First, create two buttons in your React application. The first button toggles between "X" and "O," while the second button simply outputs a message in the console.
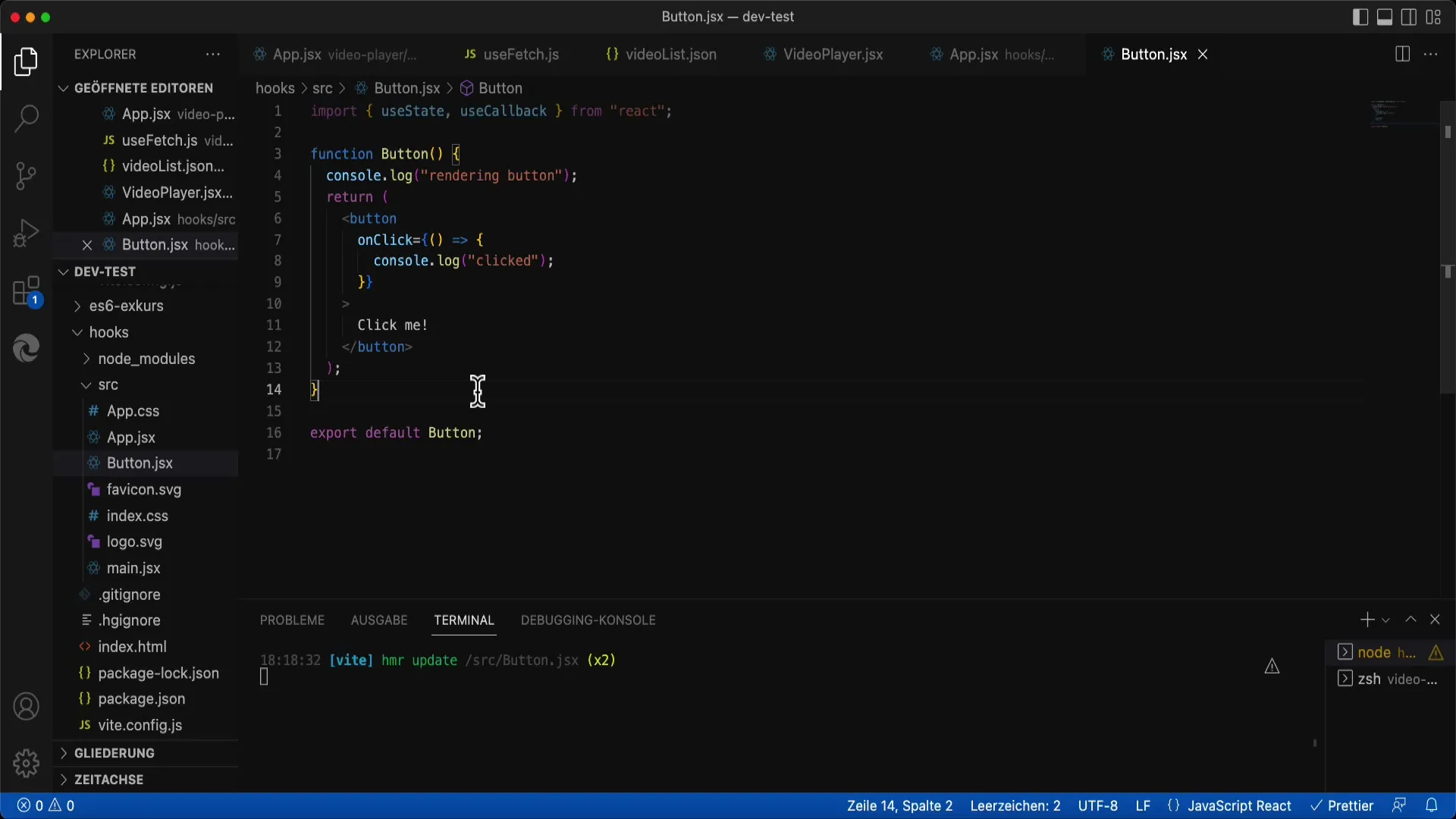
In the source code, define the toggle button using a useState to keep track of the current state and change it with each click.
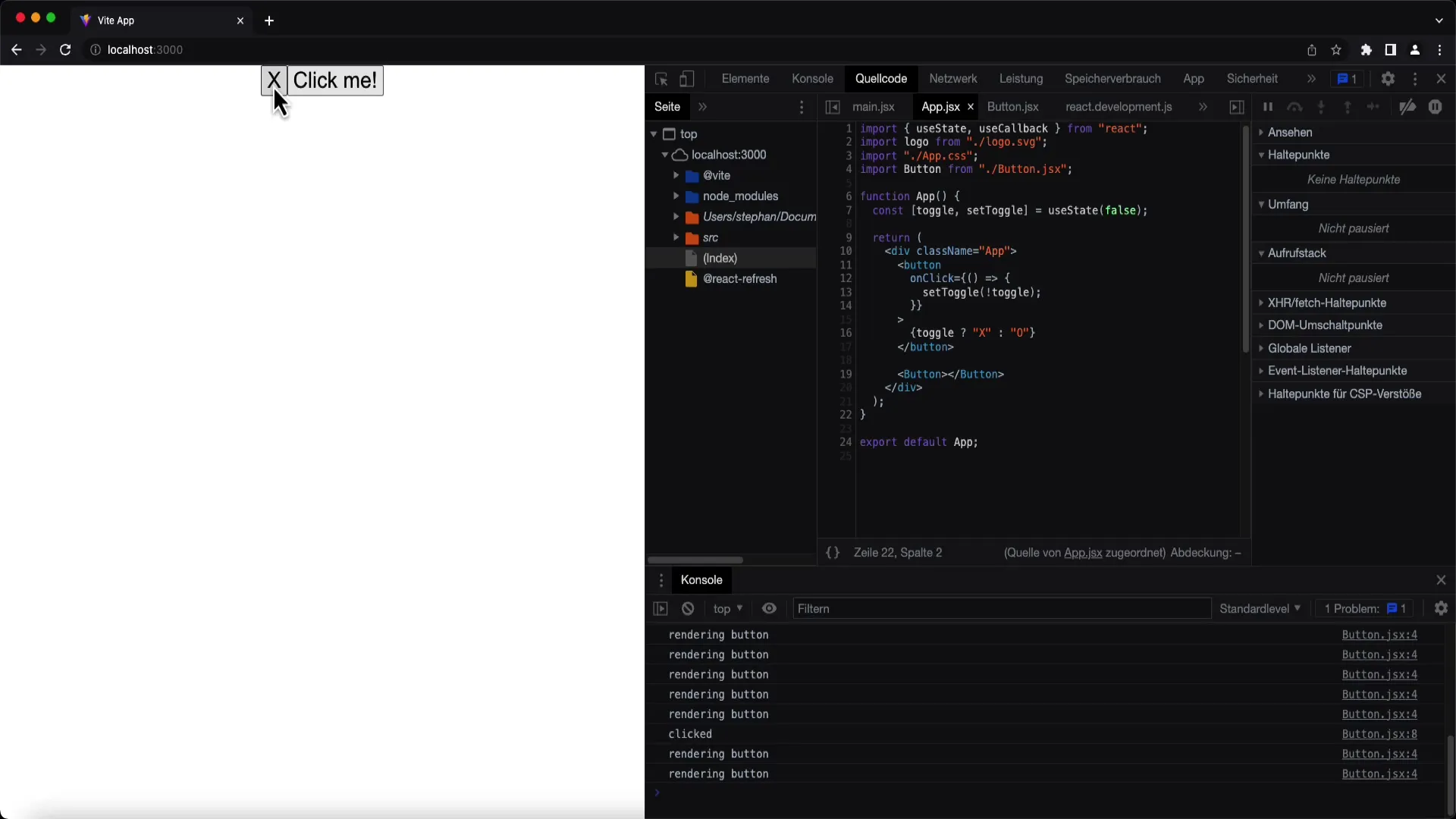
Step 2: Analyzing Component Structure
When you run your app in the browser and click on the toggle button, you'll notice that the second button in the console still gets re-rendered. This is because React re-renders all affected components on every state change, even if nothing has changed.
Although the second button has no props, its render function is still called, which is suboptimal. You want the button to only re-render when necessary.
Step 3: Introducing memo
Here's where memo comes in. You can optimize the button component by importing memo from React. This ensures that the component's render function is not called unless props change.
Step 4: Using memo
Wrap your button component with memo by placing the function call around the button component. Now, the component has the ability to only re-render when props change.
After that, check if the optimization works. When you reload the app and click the toggle button, the button should not be re-rendered as long as the props remain unchanged. You can also set breakpoints to verify if the render function is being called.
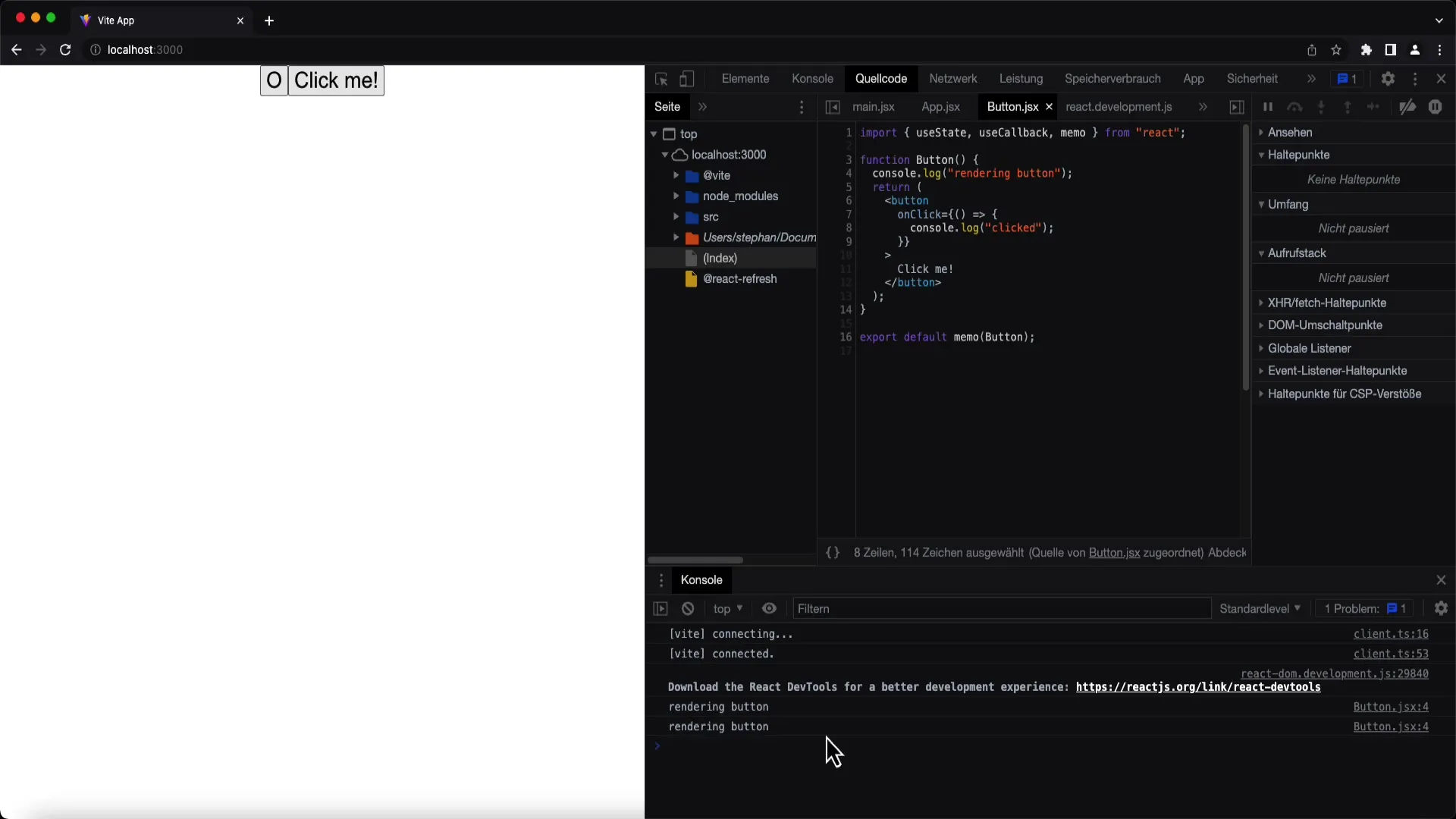
Step 5: Testing the Optimization
To test the efficiency, you can pass additional props to your button by displaying the toggle value in the button component. Add logic that changes the button's text based on the toggle status.
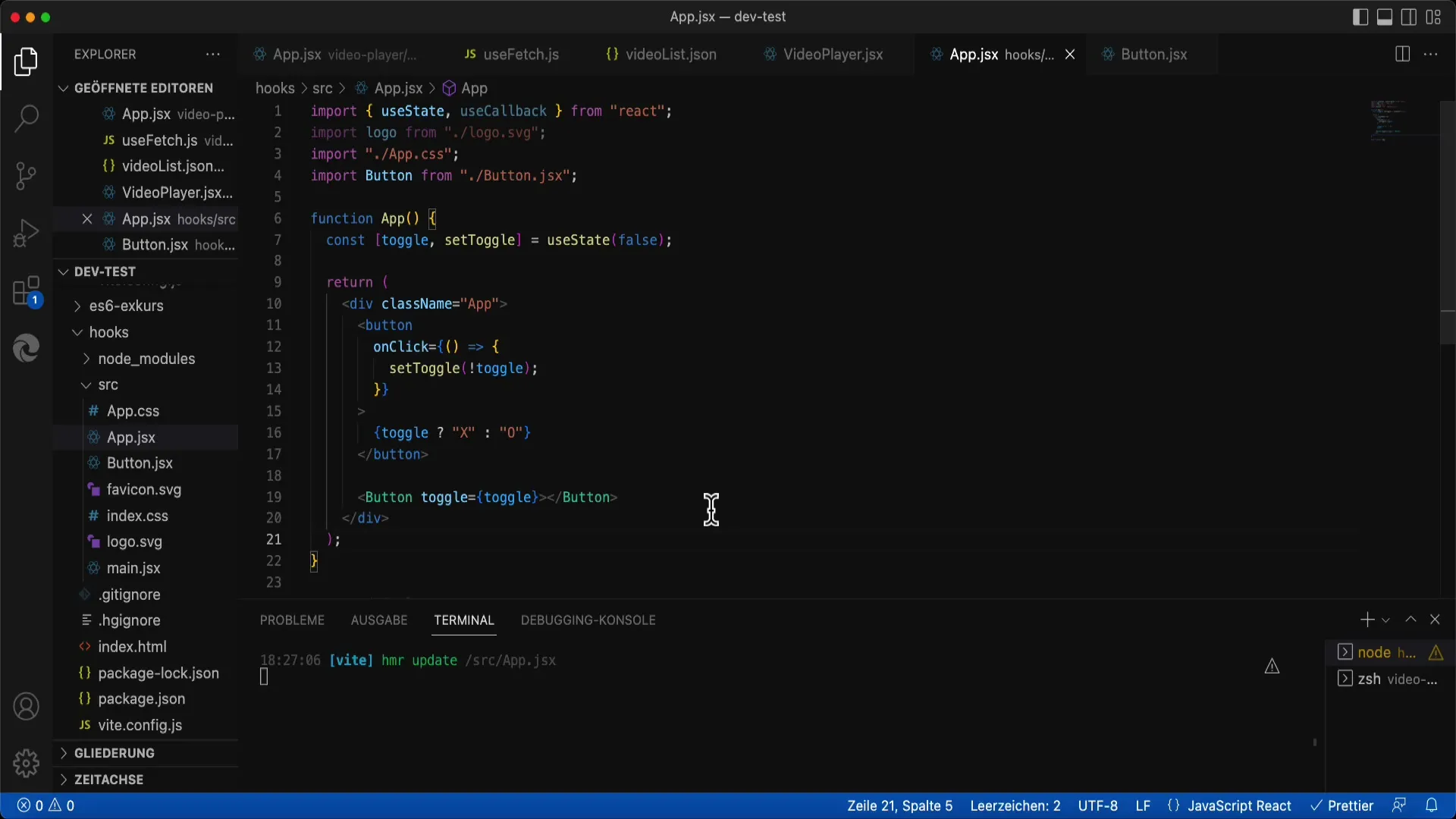
Now, when you retest the application and switch between the buttons, you'll see that the button is only rendered when the toggle prop changes. This demonstrates how the optimization works.
Step 6: Checking for Changes
When you click the toggle button again and the toggle switches from false to true, the button component will be correctly re-rendered as its props change due to the transfer of the toggle value.
Conclusion
You have now successfully implemented the memo function and optimized your button component to only re-render when necessary. This is a simple yet effective method for optimizing the performance of your React application.
Summary
In this guide, you have learned how to optimize the rendering performance of your React components using memo. You have learned when it is advisable to optimize components and how to reduce the frequency of render function calls.
Frequently Asked Questions
How does memo work in React?memo stores the result of a component and only re-renders it when its props change.
When should I use memo?memo is useful for components that perform a lot of rendering work or are present in larger applications with many state changes.
Can I use memo for every component?It is not always necessary. Use memo where it significantly improves performance, especially with complex components.