Functions are the heart of JavaScript. While they were previously bound to the use of the function keyword, ES6 introduces a new and more elegant way of writing functions with the so-called Arrow functions. This new syntax not only shortens your code but also addresses some problems related to the this context. In this guide, you will learn everything you need to know about Arrow functions.
Key Takeaways
- Arrow functions offer a shorter syntax compared to traditional functions.
- They do not have their own this context, making them particularly practical for use in callback functions.
- Removing curly braces and the return statement is possible when the function only returns one expression.
Introduction to Arrow Functions
Arrow functions are typically used to make the code more readable and compact. Instead of writing function, you can use the new syntax to declare the function more concisely.
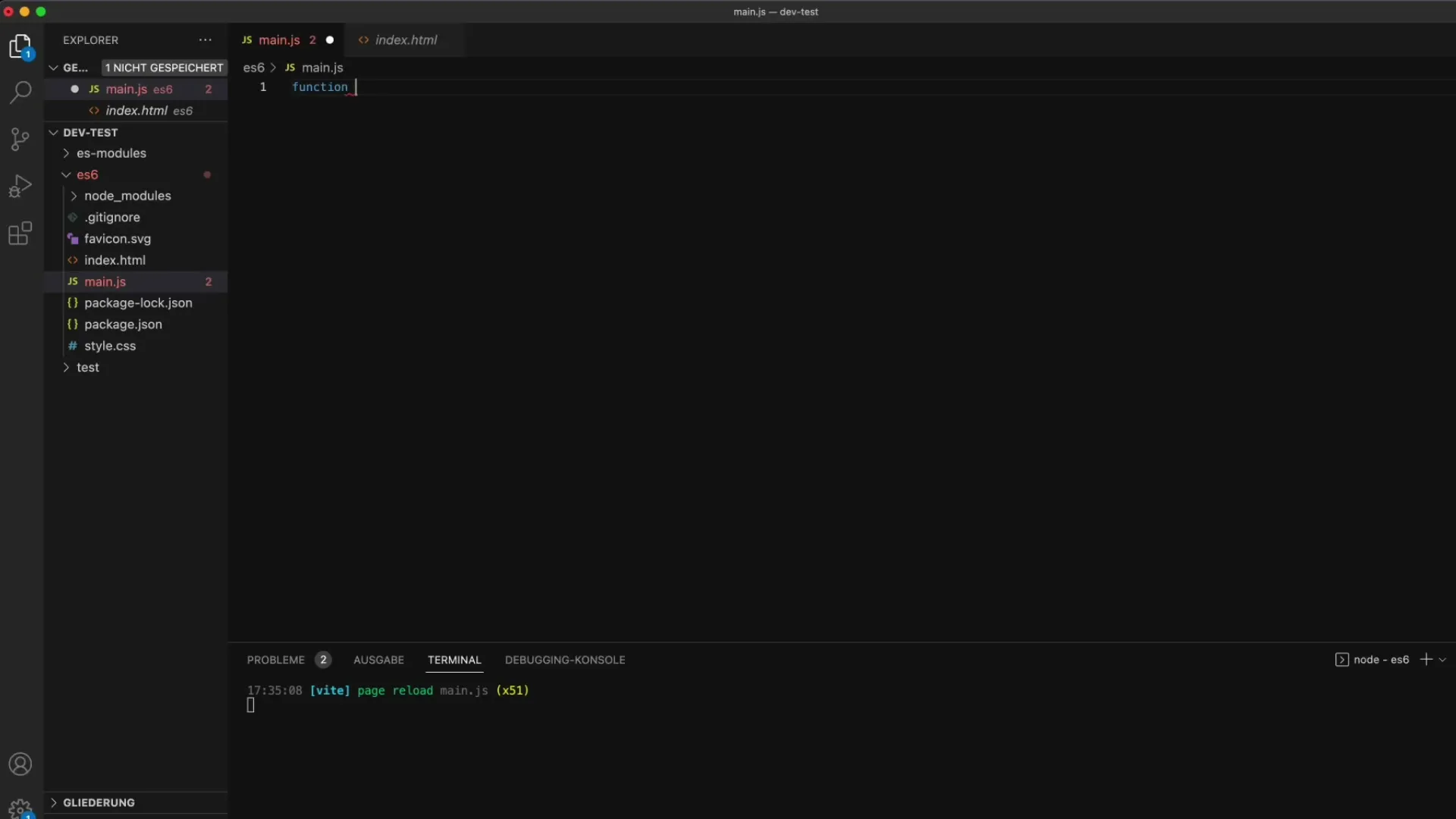
To understand what Arrow functions are, traditional functions are a good starting point. When you declare a function with the function keyword, it is usually a bit longer and less elegant. Here is an example of a standard function in JavaScript:
This is the starting point from which we move on to Arrow functions.
Step 1: Declaring an Arrow Function
Instead of the function keyword, you can simply set the parameters in parentheses and then use an arrow followed by the function body. Here is how the previous function looks as an Arrow function:
As you can see, the syntax is much more compact. After the first attempt, you can check if the function works correctly.
Step 2: Using Arrow Functions with Arrays
Another great advantage of Arrow functions is evident when using them with arrays. Let's take an example. Suppose you have an array of numbers and you want to find the position of a specific number. Here we use the findIndex method to find the index:
Arrow functions greatly simplify the readability of the code and reduce the need for an additional function keyword.
Step 3: Shortening Arrow Functions
If an Arrow function has only one expression, you can omit the curly braces and the return keyword. Here's what it looks like when we further shorten the findIndex function:
This is not only shorter but also more elegant. You can test the function to ensure that it still works correctly.
Step 4: Handling the this Keyword
A crucial advantage of Arrow functions is how they handle the this context. In traditional functions, this often becomes unpredictable, especially when the function is used as a callback. Arrow functions, on the other hand, bind this to the context in which they were defined.
Take a look at the following example:
obj.print(); // Outputs "Text"
However, when you use setTimeout with a traditional function, the context of this changes:
By using an Arrow function within setTimeout, you get the same result as with the traditional function without losing the context:
Step 5: Maintaining the this Binding
You can also bind this to a variable to ensure you can access it. However, this pattern is often less elegant than using Arrow functions.
Advantages of Arrow Functions
- They are shorter and more elegant compared to traditional function declarations.
- They do not have their own this context, which simplifies their use in callback functions.
- The syntax allows you to make the code much more readable.
Summary
Arrow functions provide a convenient way to declare JavaScript functions efficiently. The new syntax not only improves readability but also greatly simplifies the handling of the this context. You should use arrow functions especially when you need callback functions or shorter function expressions.
Frequently Asked Questions
What are Arrow Functions?Arrow functions are a shorter syntax for functions in JavaScript introduced with ES6.
When should I use Arrow Functions?Use arrow functions especially in callback scenarios or when you need anonymous functions.
What are the advantages of Arrow Functions regarding the this context?Arrow functions bind this to the surrounding context, which often prevents unpredictable behaviors in normal functions.