Modules are a central component in modern JavaScript development, especially since the introduction of ES6. With ES6, you can structure and maintain your code more effectively by using import and export. In this guide, you will learn how to effectively use ES6 modules to modularize your React applications.
Key Takeaways
- ES6 modules use the keywords import and export.
- To load a module in an HTML file, use the type="module" attribute in the
- You can use both default and named exports from a module.
- When using import, there are different syntaxes available that can be used as needed.
Step-by-Step Guide
1. Introduction to ES6 Modules
First, you need to understand what ES6 modules are. These provide a simple way to organize code into separate files. With ES6, it's necessary to use a
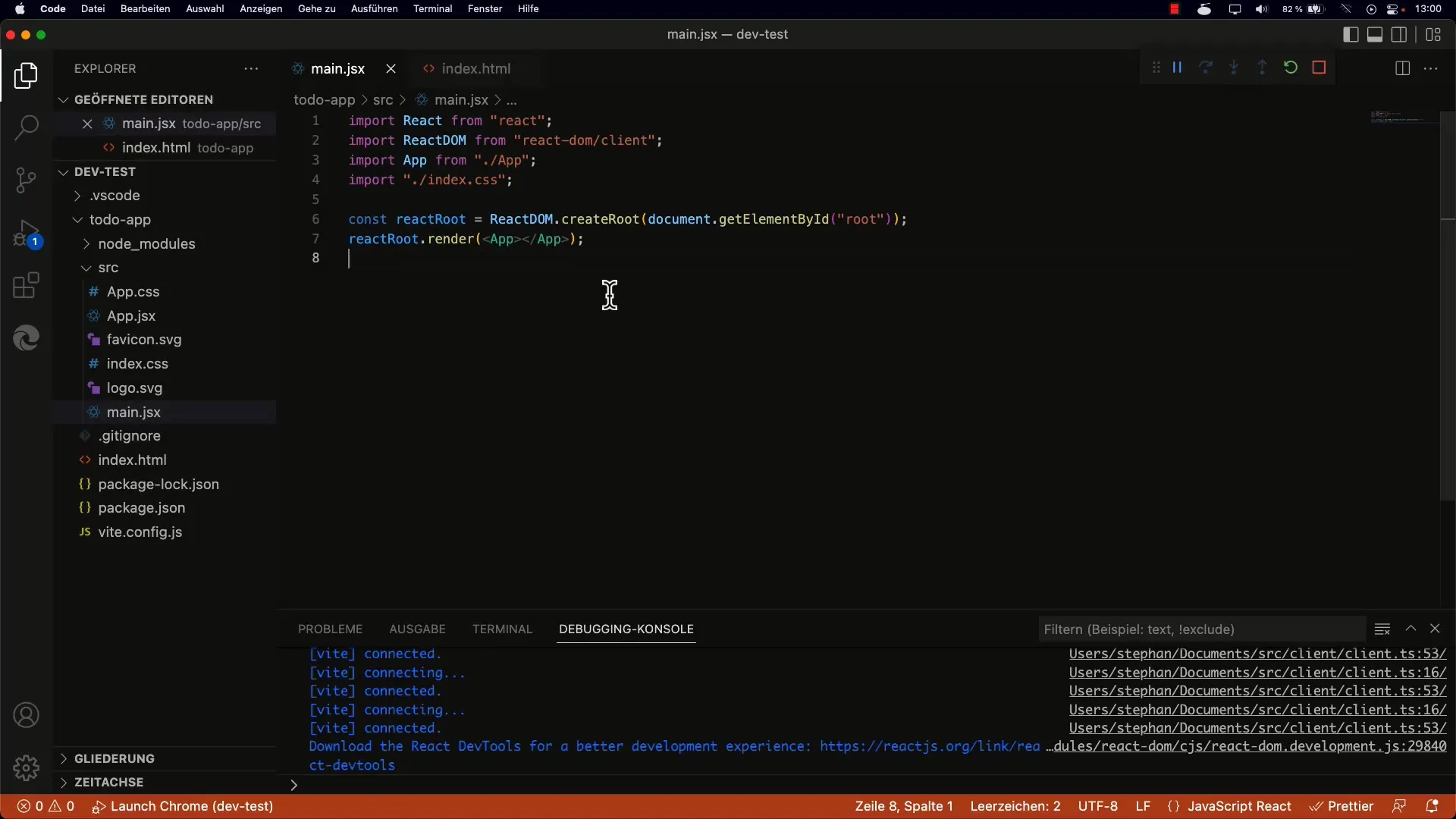
2. Creating a Module
Now, create a new module that you want to import into your main file main.jsx. Create a file named Mod1.js. In this file, you can define various functions that you want to use later.
3. Basics of Imports
Next, you will import your module in main.jsx. Importing is done using the syntax import { Function } from './Mod1.js';. You can also include the file without the .js extension as long as your development server is properly configured.
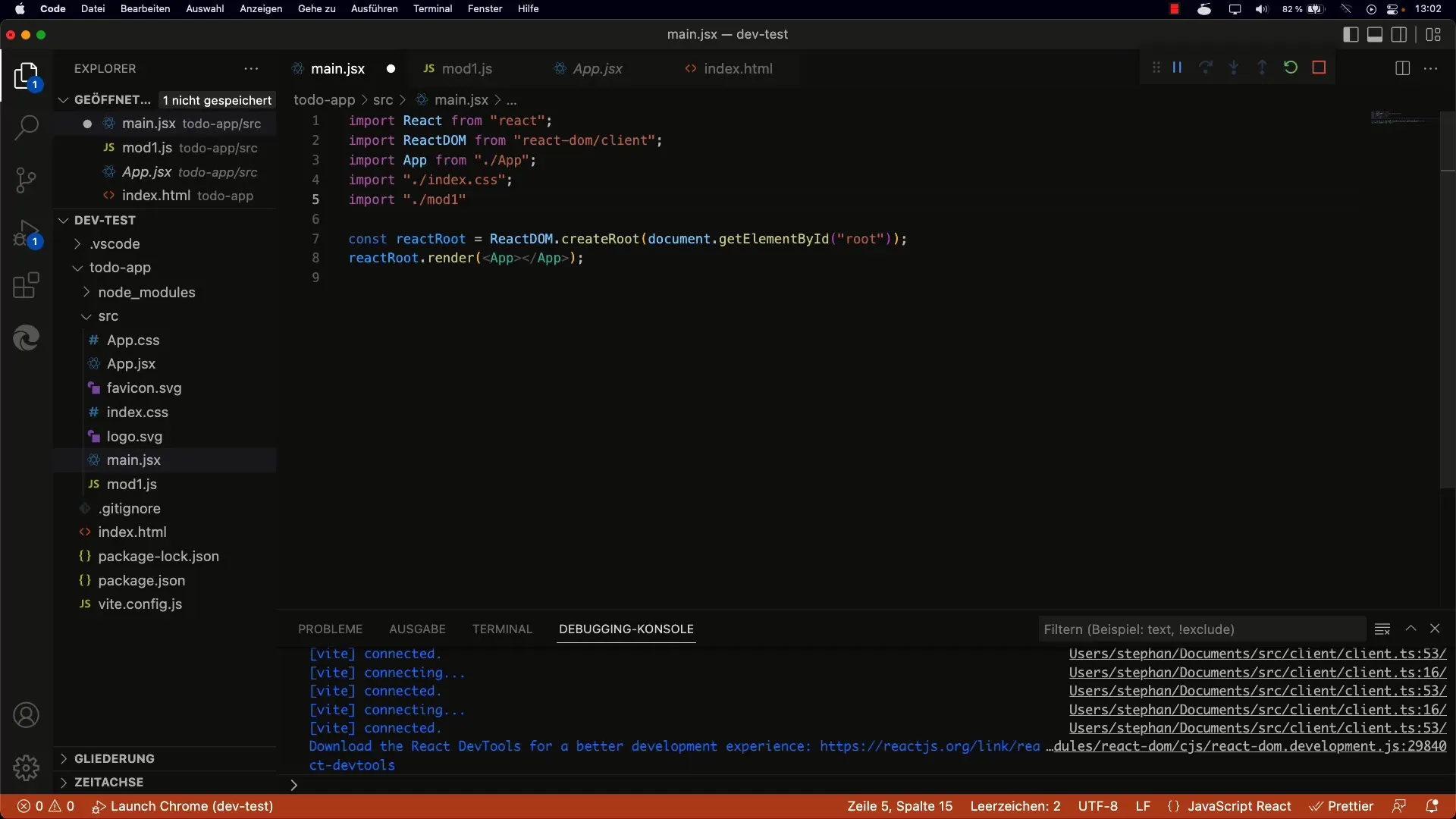
4. Exporting Functions in the Module
In order to be able to use the functions you have created, you need to export them. This is done by prefixing the function with the export keyword. For example:
Now you can import this function into other files.
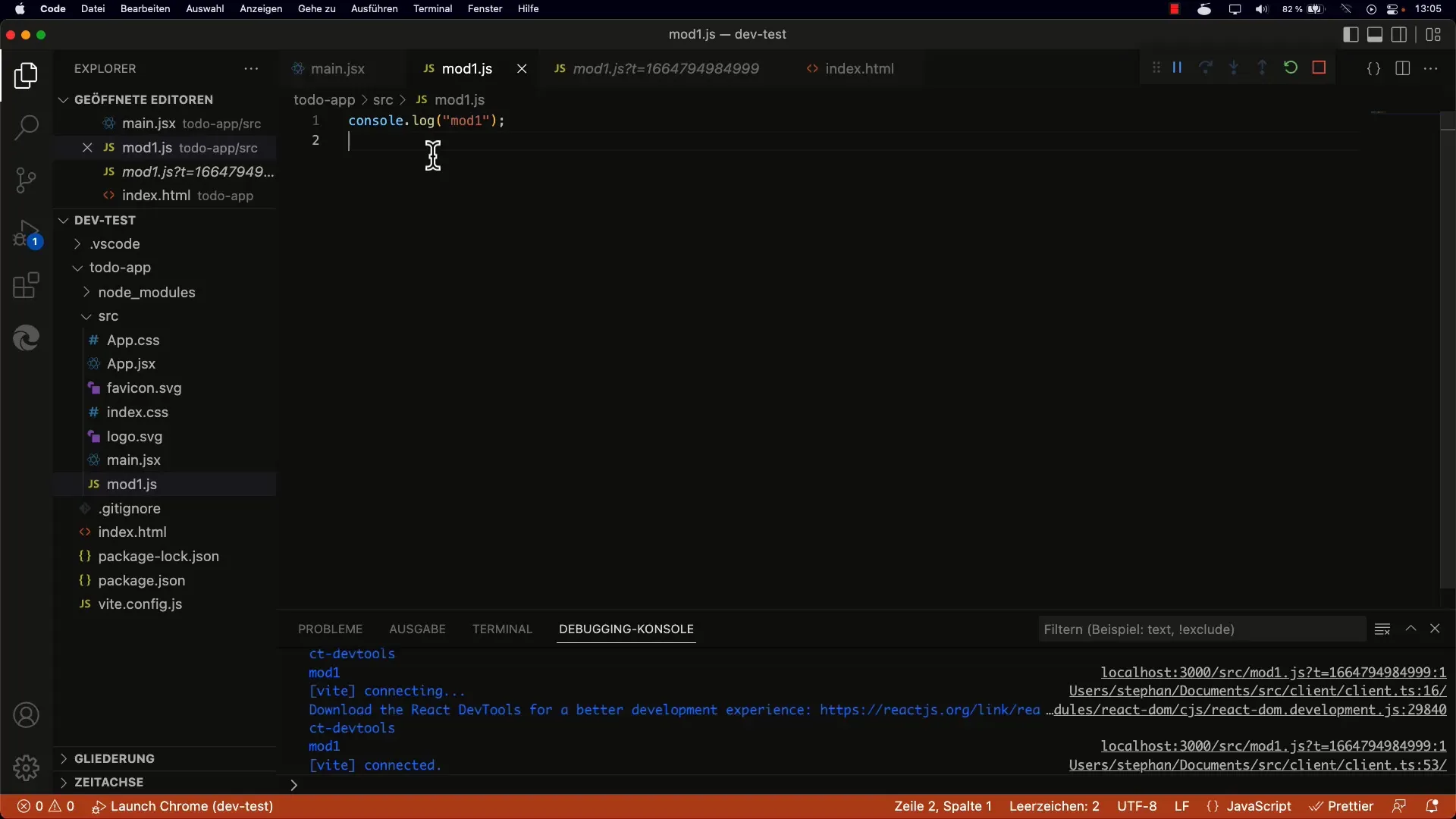
5. Importing the Functions
To be able to use the exported function in main.jsx, use the import syntax:
This way, you have access to the doIt function and can call it in your code as needed.
6. Using Default Exports
In addition to named exports, there is also the option to use a default export. This means that you can define a function as a default export, which is then imported without curly braces. Simply write:
In your import file, you can then call it as follows:
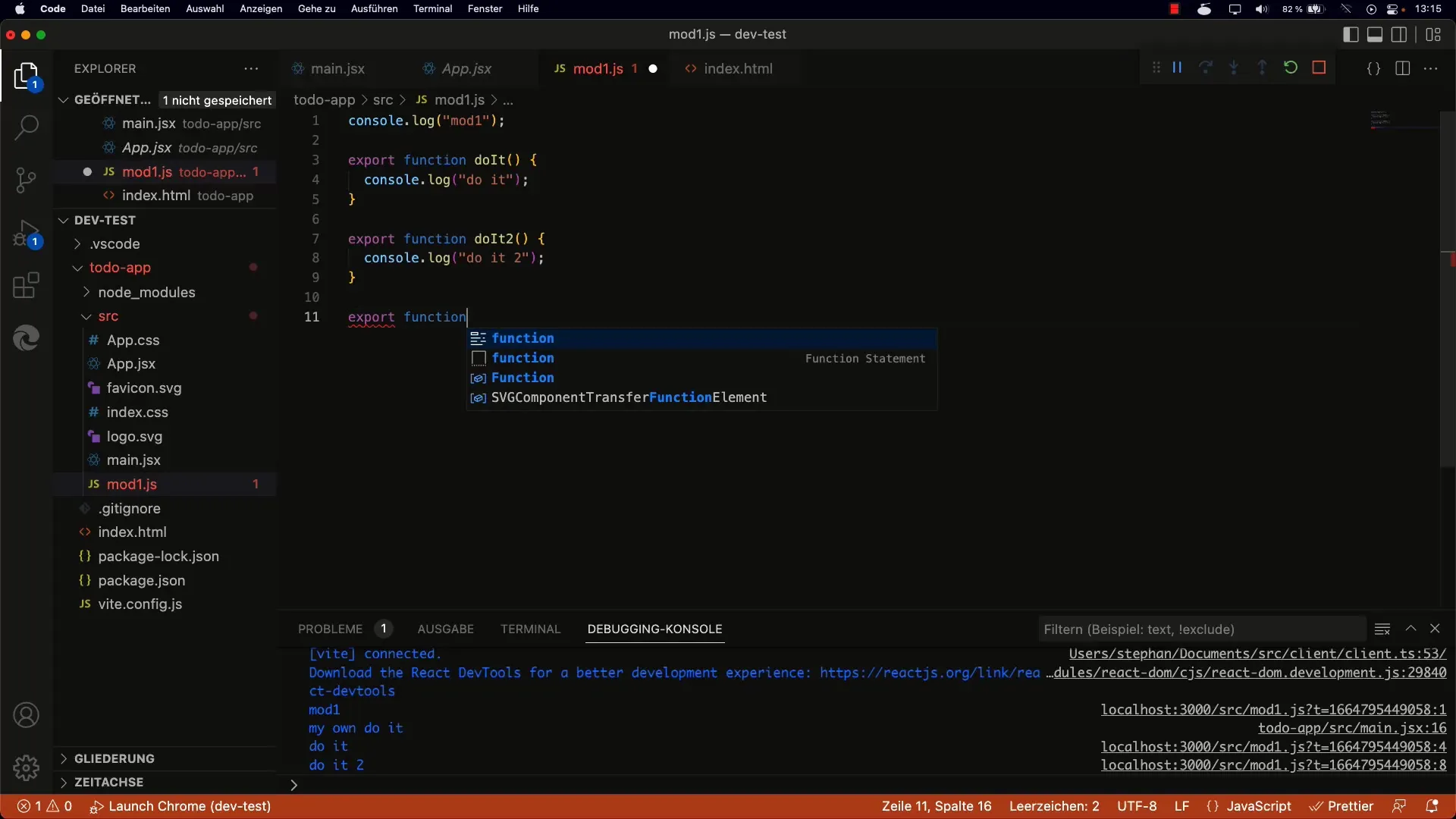
7. Complex Modules and Name Conflicts
When importing multiple functions from a module, there may be name conflicts. In such cases, it's useful to rename the imported functions. You can do this by using the syntax import { doIt as myDoIt } from './Mod1.js';.
8. Importing the Entire Module
Sometimes you may want to import all functions of a module at once. In this case, you can use the following syntax:
Now you have access to all exports of this module using the identifier Mod1.
9. Conclusion on ES6 Modules
In conclusion, it can be said that the module system in ES6 helps you improve the structure of your JavaScript applications. Many benefits, such as code reusability, better readability, and easier maintainability, are achieved through the modular approach.
Summary
In this guide, you have explored the basics of ES6 modules and learned how to effectively use them in your React applications. Solid knowledge of modules is essential to succeed in modern web development. Utilize the techniques described to make your projects modular and organized.
Frequently Asked Questions
What are the main advantages of ES6 modules?They improve code reusability, readability, and code structuring.
How do I import a function from a module?Use the syntax import { Function } from './Module.js';.
What is the difference between named exports and default exports?Named exports must be written within curly braces, while default exports are imported without them.
Can I dynamically import modules?Yes, ES6 also supports dynamic imports, which can be useful in certain scenarios.
Why should I prefer ES6 modules?They support better organization and modularization of your code in larger codebases.