The creation of User Interfaces (UIs) in React is simplified by JSX, a syntactic extension of JavaScript. JSX allows you to use HTML-like syntax in JavaScript, making the creation of components more intuitive. This guide shows you how to effectively use JSX to create components in React and provides valuable insights into the workings of JSX behind the scenes.
Key Insights
- JSX is a syntax extension for JavaScript that allows creating HTML-like structures.
- JSX is transpiled into regular JavaScript to ensure browser compatibility.
- With the createElement method, you can create React elements directly without JSX.
- There are some differences between traditional HTML and JSX that should be noted.
Step-by-Step Guide
Step 1: Introducing JSX
First, open your React application and examine the basic structure. You will notice that JSX is already used in your project. A simple example of JSX would be:
. You can try this by adjusting the main component of your app accordingly.
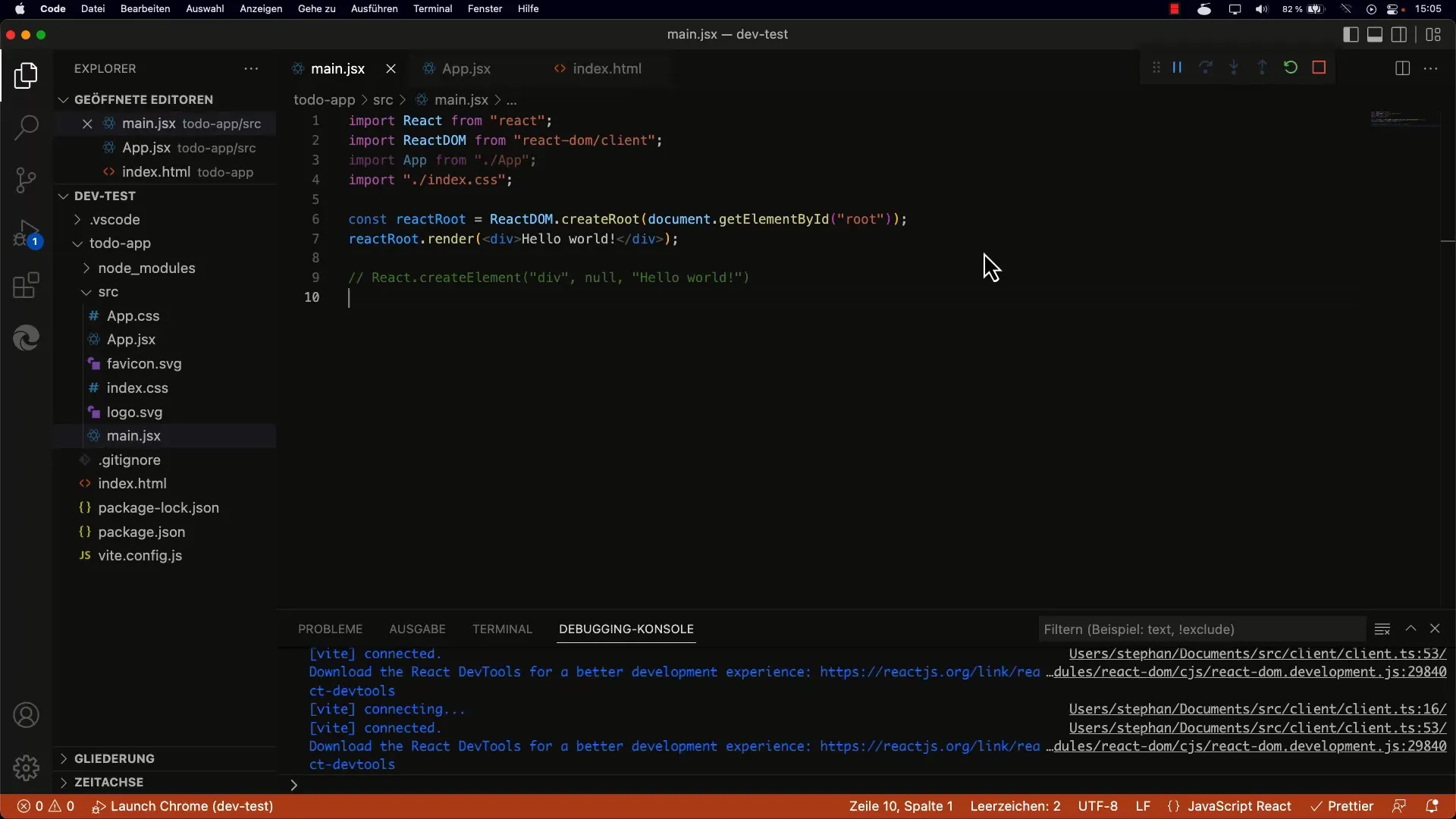
Step 2: Understanding JSX Syntax
When using JSX in your code, it looks like HTML. However, note that this code will not work directly in a normal JavaScript environment. Try running the JSX code in the browser, and you will get a syntax error. This is because JSX needs to be translated into valid JavaScript through a transpiler.
Step 3: Working with React.createElement
To understand how JSX works, take a look at React's createElement method. This method allows you to create a React element by passing the tag name, possibly props, and children. So, instead of the JSX code, you could use code like the following:
This code produces the same result as the JSX expression.
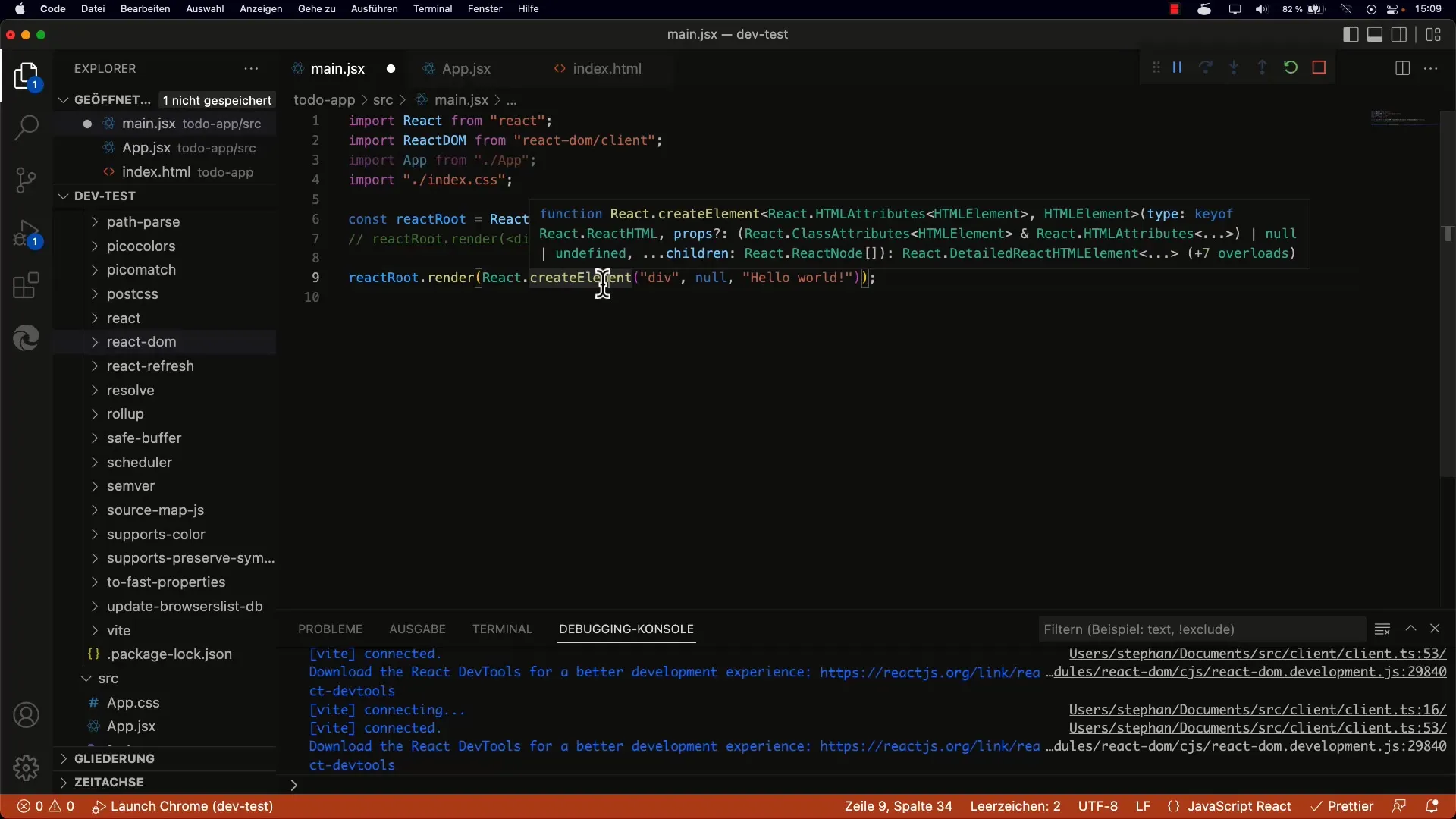
Step 4: Creating Nested Elements with createElement
To create a more complex element with nested structures, you can call createElement multiple times. For example, if you want to create a div element inside another div element, here is an example:
This creates an outer div containing an inner div with the text "Hello World."
Step 5: Using Components in JSX
If you want to create your own components, you can directly use JSX to include them. Define a simple function that returns your component and then use it within JSX:
// In your main render block:
This component will then be rendered correctly in your application. Be aware that some attributes in JSX have different spellings than in traditional HTML. For example, class in JSX is called className, as class is a reserved keyword in JavaScript. So you could write something like: Using JSX in React allows you to create and structure User Interfaces in a declarative way. While JSX provides an HTML-like syntax, it is internally translated into JavaScript, improving readability and maintainability. This guide covered the basics of JSX, the usage of the createElement method, and important differences between JSX and HTML. Understanding these concepts is crucial for developing efficient React applications. What is JSX?JSX is a syntax extension for JavaScript that allows using HTML-like syntax within JavaScript. How is JSX transpiled to JavaScript?JSX is translated into regular JavaScript by a transpiler like Babel to ensure browser compatibility. Are there differences between JSX and regular HTML?Yes, some attributes have different names, for example, class is changed to className to avoid conflicts with reserved keywords in JavaScript. Can I work in React without JSX?Yes, you can use React's createElement method to create elements without JSX, but this can quickly become more complex and harder to read. When should I use JSX?JSX is generally recommended as it increases readability and simplifies component structuring.Step 6: Differences Between HTML and JSX
Summary
Frequently Asked Questions