Using JavaScript in JSX is a key aspect when developing React applications. Here you will learn how to efficiently integrate data and JavaScript expressions into JSX to make your applications more dynamic and flexible. Let's dive right in by exploring the basics of JavaScript expressions in JSX.
Key Takeaways
- JavaScript expressions can be integrated into JSX by using curly braces.
- It is important to use the correct syntax to avoid errors.
- Style attributes expect JavaScript objects.
- Functions can be passed as event handlers.
Step-by-Step Guide
1. Introduction to JavaScript Expressions in JSX
To use JavaScript expressions in JSX, you need to understand how to properly include them. In JSX, expressions can be enclosed in curly braces {} which means you can use variables or calculations within your JSX structure.
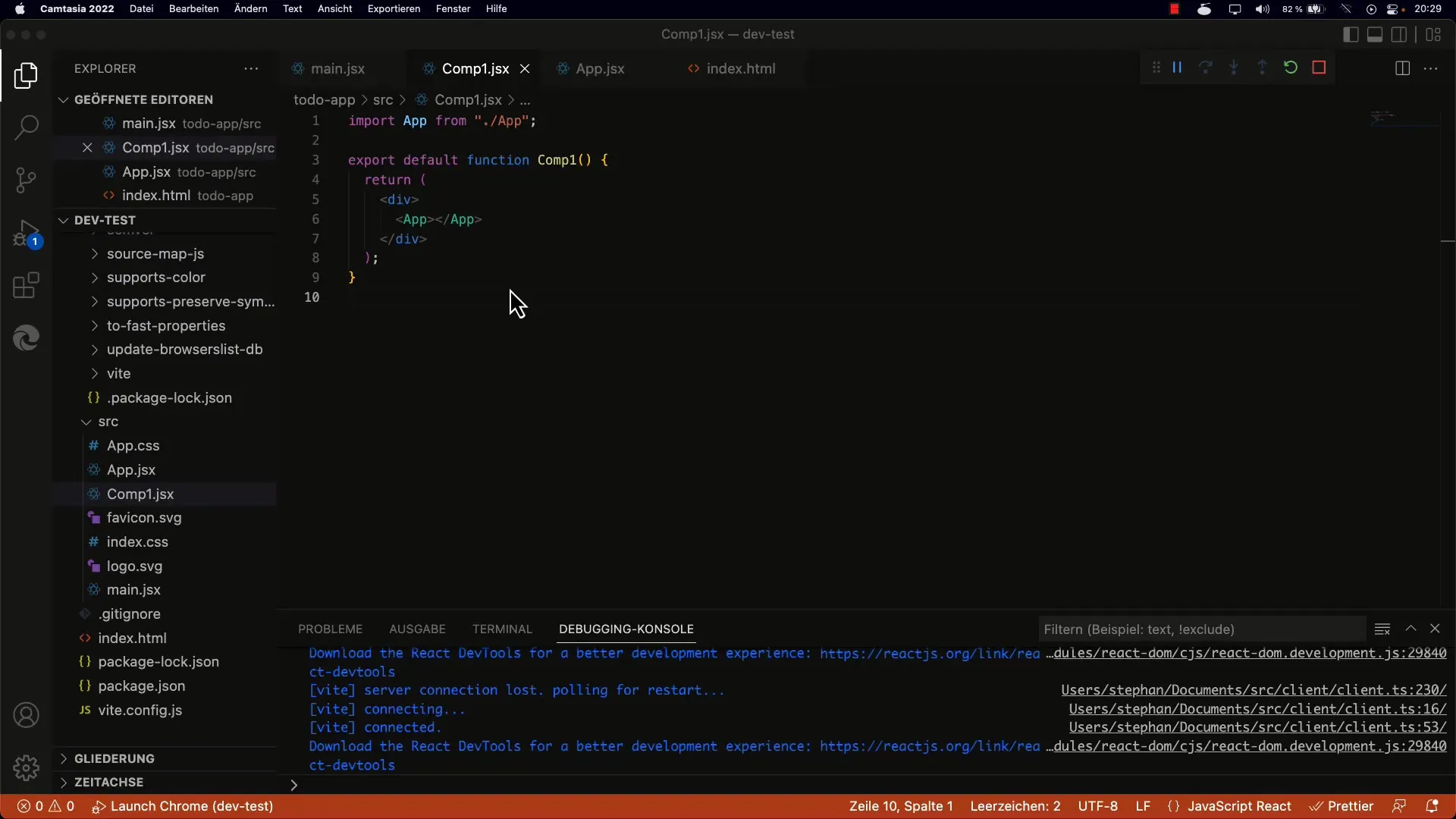
2. Define a Constant
Start by defining a constant within your render function. For example, you can create a constant a that contains the string "Hello World".
3. Insert into JSX
If you want to display the value of constant a in JSX, you should enclose it in curly braces. This way the value will be rendered correctly and not interpreted as text.
4. Check Graphic Structure
It is important to check the structure of your components. Add a div and make sure that the value of a is rendered as the inner text of the div. Check the resulting HTML structure to ensure everything is displayed correctly.
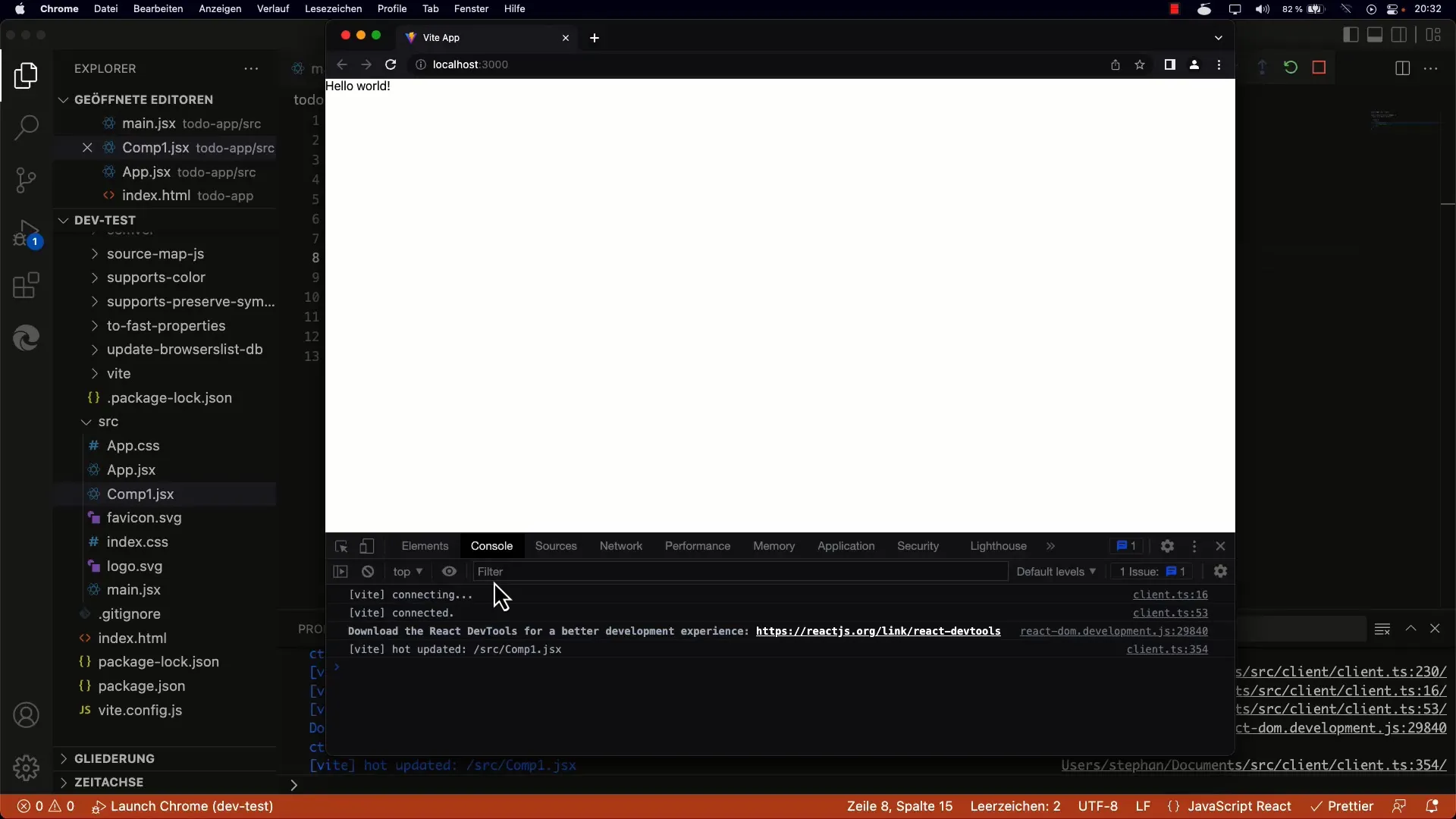
5. Using JavaScript Expressions
You can also use any valid JavaScript expression in JSX. For example, instead of a, you can use numerical expressions like 1 + 2 or constants like Math.PI. This gives you the flexibility to display various dynamic data.
6. Restrictions on Multi-Line Expressions
An important point is that you cannot use multi-line expressions or blocks directly in JSX. Each expression must be written in a single line as the JSX parser expects a simple, linear expression.
7. Passing Objects and Styles
When it comes to CSS styles, objects can be used as JavaScript expressions. You need to use curly braces for the entire style and then define the object within another set of curly braces.
8. Adjusting Typical Properties in Style
It is important to note that CSS properties in JavaScript should be written in CamelCase style. Instead of "font-size", you would use "fontSize". This peculiarity needs to be considered when passing JavaScript objects for applying styles in JSX.
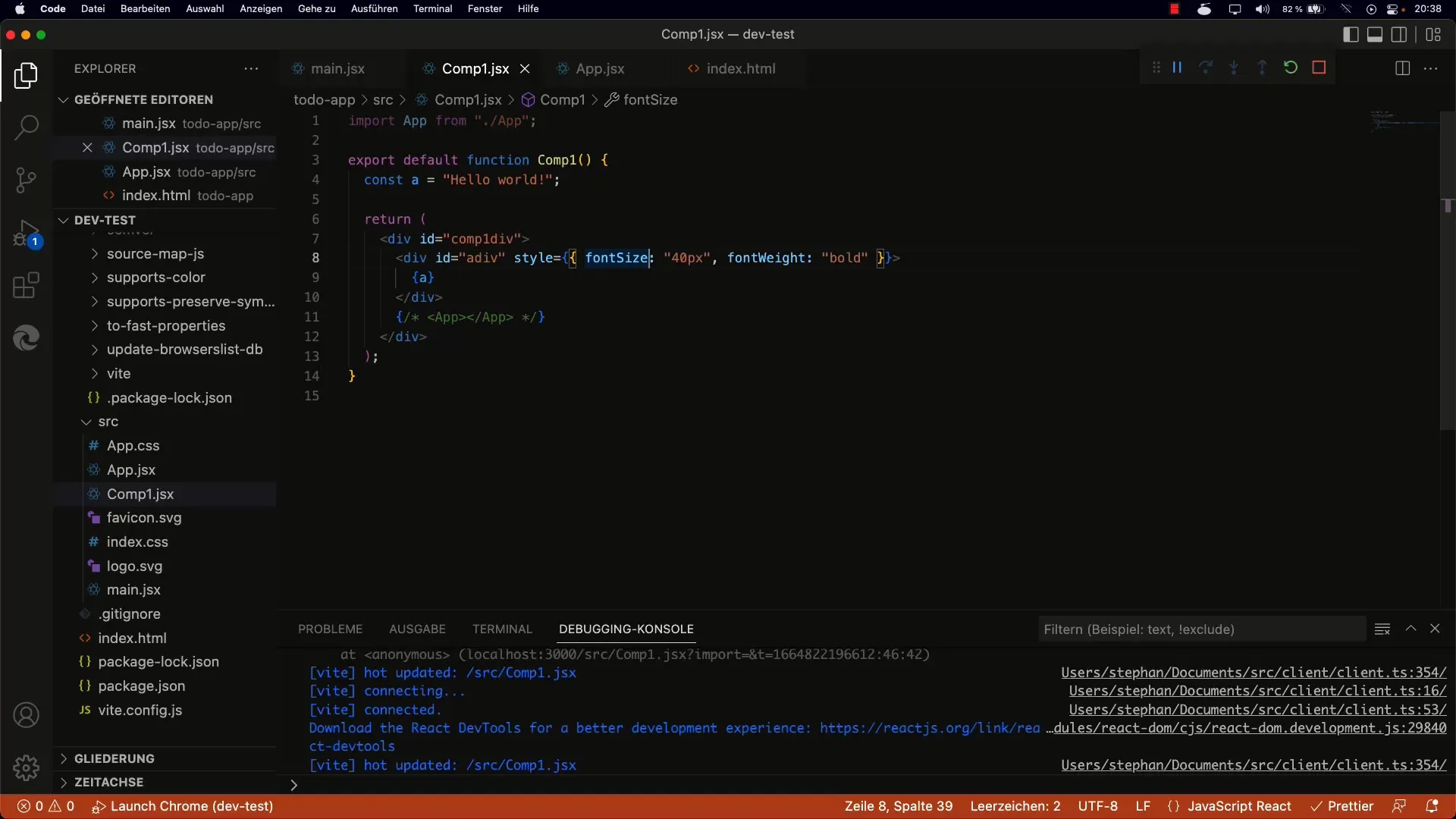
9. Examples of Dynamic Styles
To observe changes in elements, you can programmatically control properties like font size or font weight of a text. For instance, setting the font size of "Hello World" to 40px and making it bold.
10. Complex Structures with JSX
You can create deeper nesting in JSX by creating new JSX elements within the expression. For example, you could insert a paragraph (
) within the returned JSX and display the text "Hello World".
11. Defining Event Handlers
A common use case is adding event handlers, such as for a button. You can define a function in JSX and attach it to the button using the onClick attribute.
12. Function Call and Feedback
When you click the button, an alert is triggered showing you a confirmation. This is a simple way to demonstrate user interactions and showcase error-free event handling.
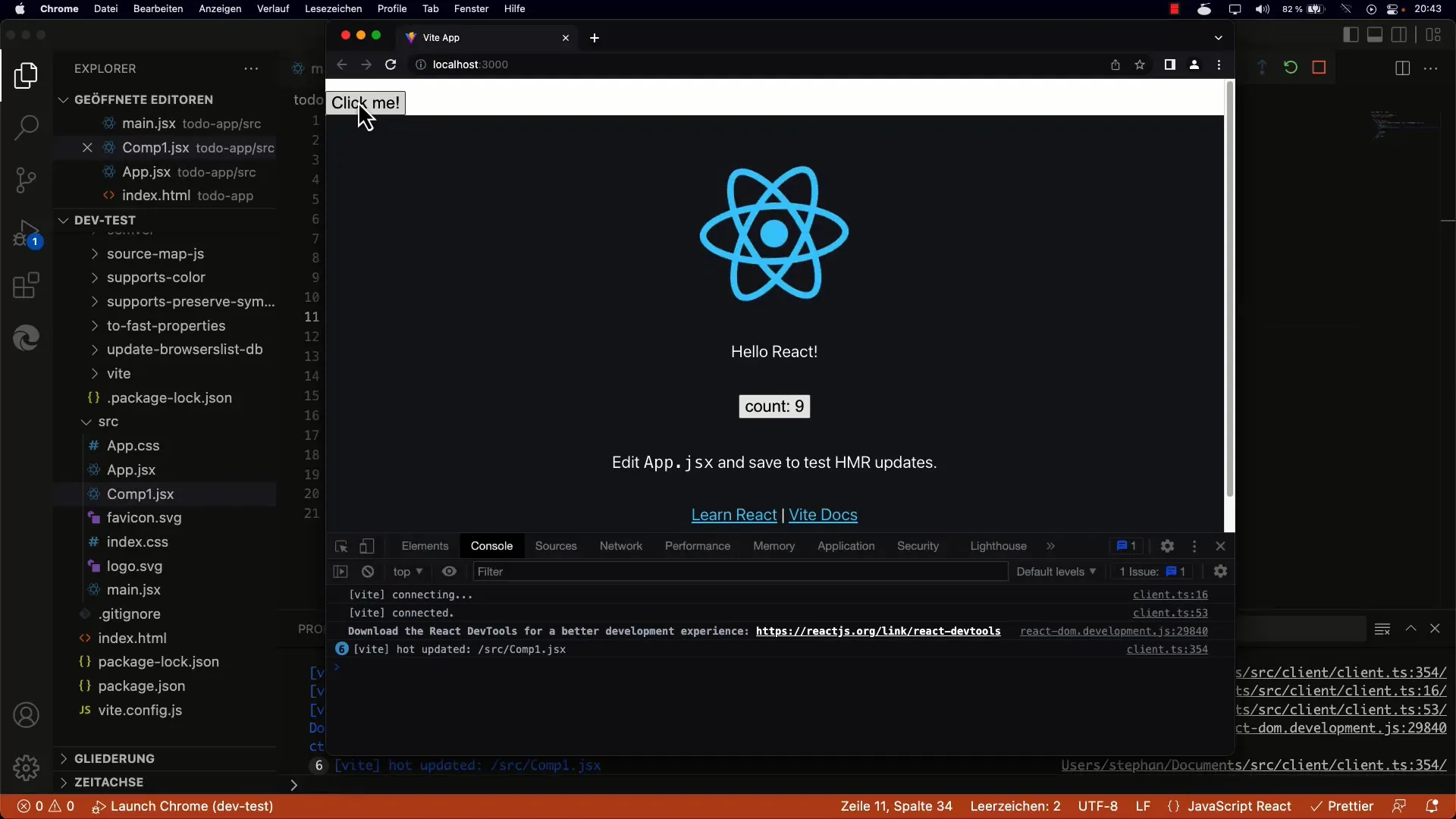
Summary
You have now gained an overview of using JavaScript expressions in JSX. You can use variables and expressions to generate dynamic content and apply styles. By applying the right techniques, you can make your applications more user-friendly and interactive.
Frequently Asked Questions
How can I use JavaScript within JSX?You can insert JavaScript into your JSX using curly braces {}.
Are multiline expressions possible in JSX?No, expressions in JSX should not span multiple lines.
How do I pass CSS styles in JSX?Styles are passed as JavaScript objects formatted in CamelCase.
What happens if I pass an object in JSX?A regular JavaScript object cannot be directly used as a child in a JSX element.
Can I define functions in JSX?Yes, functions can be passed as event handlers within JSX.