Storing states is an essential concept when developing applications with React. Unlike class-based components, functional components use hooks to efficiently manage state. A widely used hook in React is useState, which allows you to store and update a component's state. In this guide, you will learn how to correctly apply and consider useState.
Key Takeaways
- The useState hook provides a way to manage states in functional components.
- You can set initial values and update the state through a specific setter function.
- It is important to adhere to the rules of using hooks to avoid unexpected errors.
Step-by-Step Guide
1. Import the useState Hook
First, you need to import the useState hook from the React library. This is usually done at the beginning of your component.
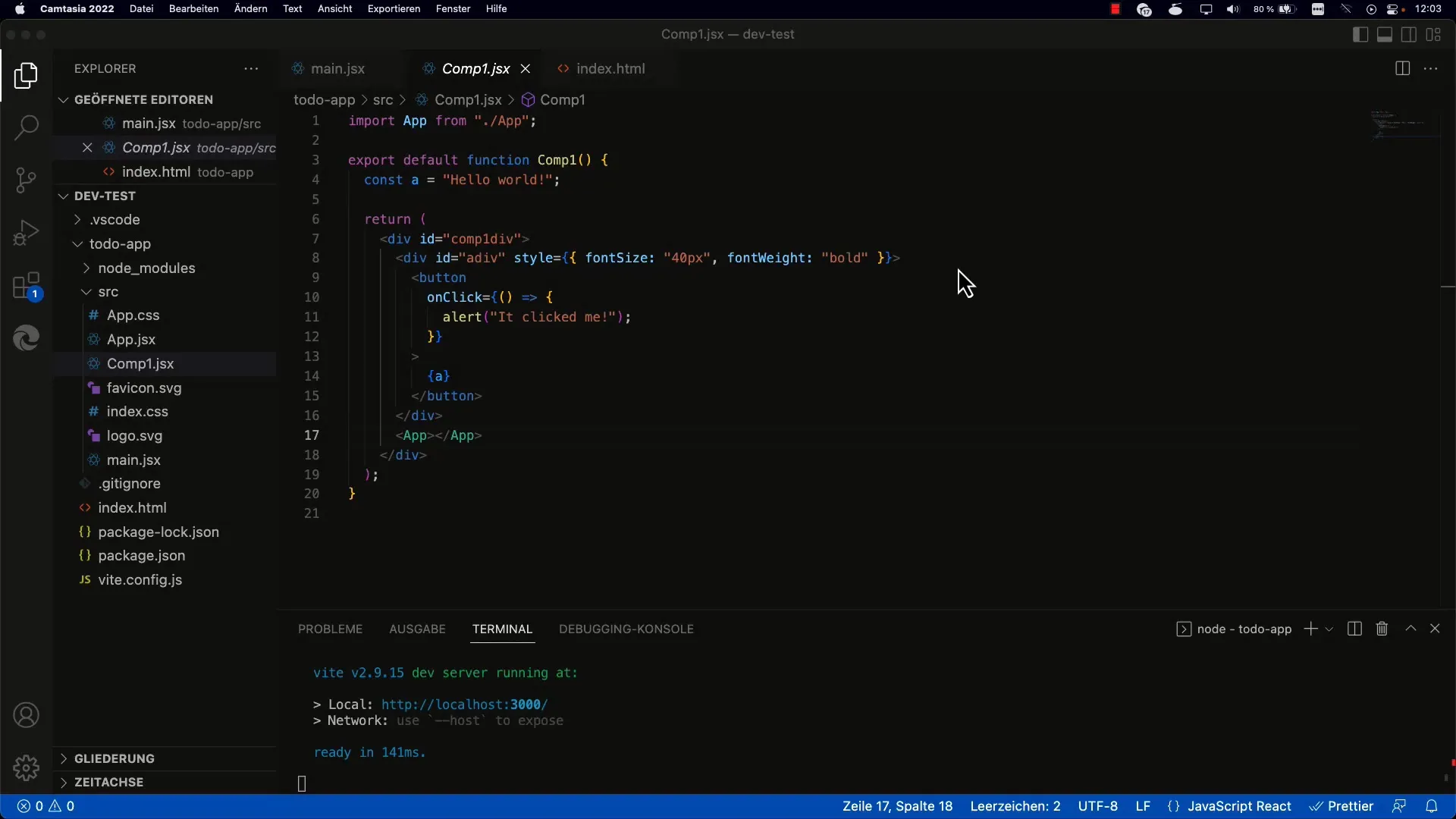
2. Initialize the State
Use useState to create a state variable. As an argument, you pass the initial state that the element should take. In this case, we start with 0 for a counter.
3. Destructure the Returned Array
Calling useState returns an array with two elements: the current state and the setter function. You should capture these two values with destructuring so you can continue working with them.
4. Implement a Button
For interacting with your state, we create a button that allows you to increase the counter. The button will display the counter value.
5. Add a Click Handler Function
Depending on the requirements, you need to define a function that will be executed when the button is clicked. This function should utilize the setter function to update the state.
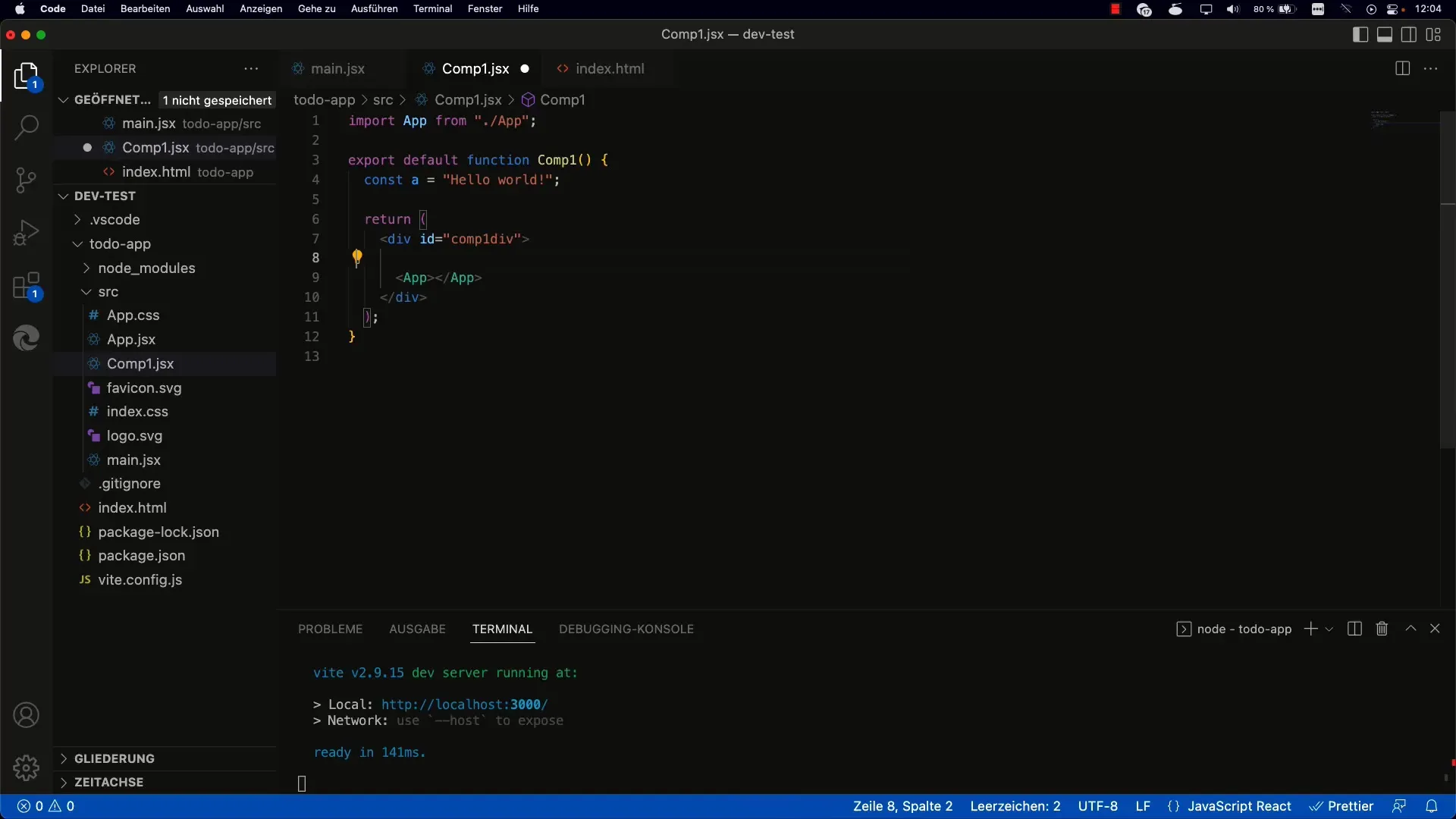
6. Set the New Value in State
Modify the state by calling setCounter with the new value in the click handler function. It is important to consider the old state in this process.
7. Test the Functionality
Reload the app to ensure that the counter is correctly incremented after clicking the button. The current value of the counter should be displayed in the button.
8. Use the setState Functionality
In some cases, it may be useful or necessary to use the setState functionality. This allows you to ensure that the correct version of the previous state is used, especially with asynchronous events.
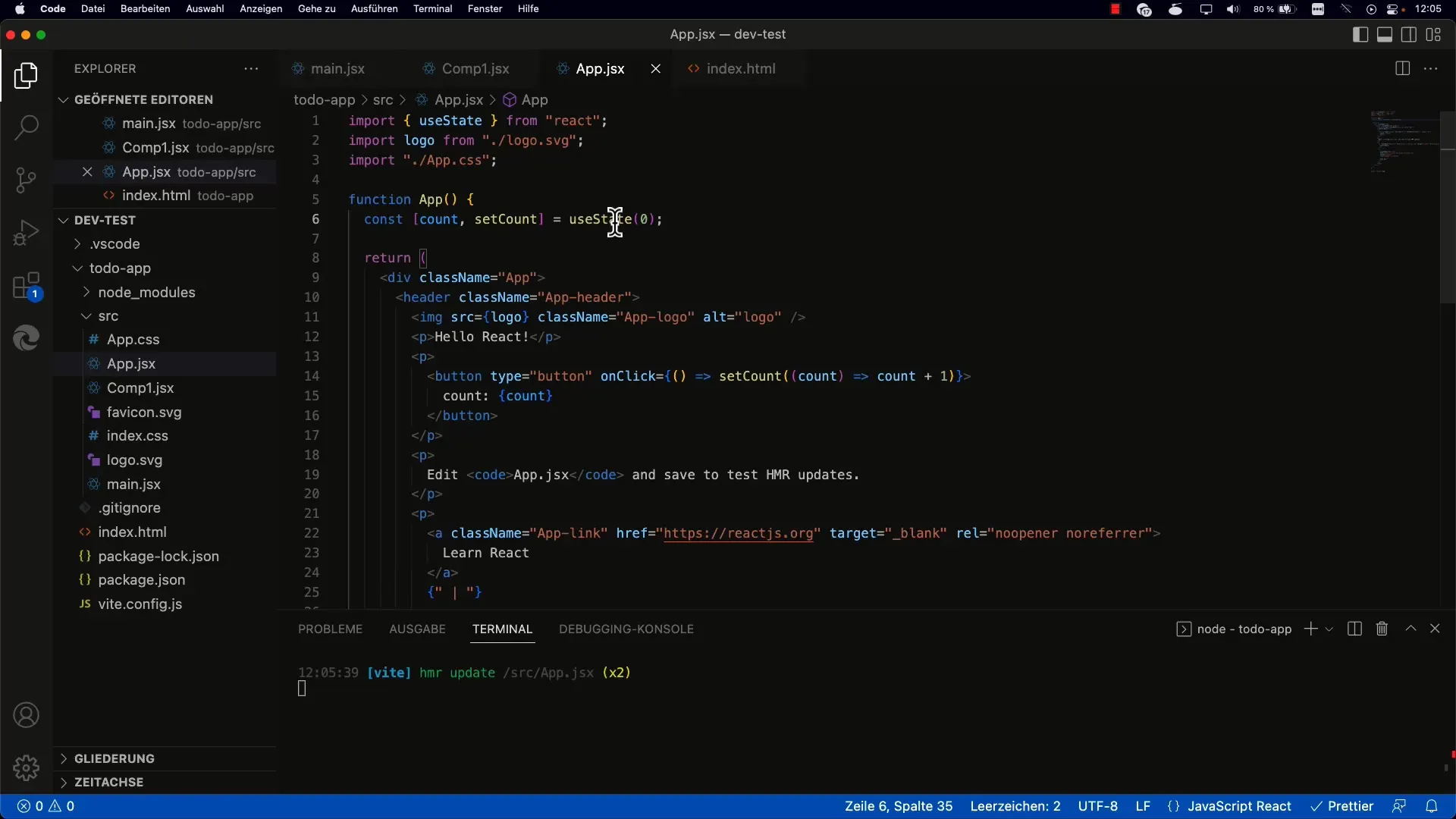
9. Managing Multiple States
If your component requires multiple state variables, you can call useState multiple times to define them. Make sure the order of calls remains the same.
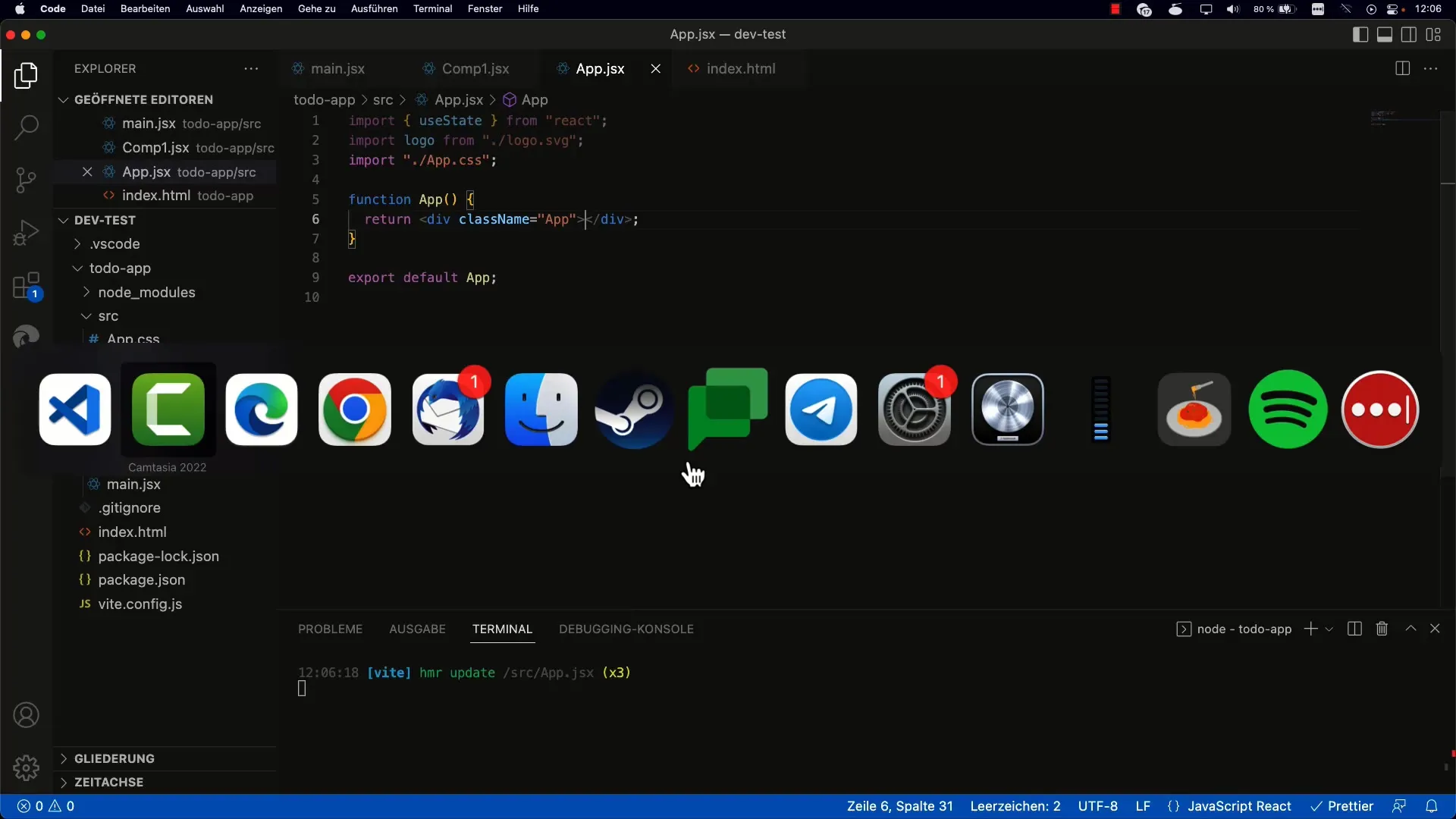
10. Avoid Errors in Using Hooks
Follow the rules of using hooks: All useState calls should be at the beginning of the component without being influenced by conditions on whether useState is called or not. This rule helps to avoid errors that can arise from changing the order of hook calls.
Summary
In this guide, you have learned how important the useState hook is for managing component state in React. The process begins with importing the hook, initializing the state, and proceeding to actionable implementation. By following the structured approach and specific interactions, you should be able to effectively manage state in functional components.
Frequently Asked Questions
How does the useState hook work?The useState hook stores the state of a component and returns a setter function to update this state.
Can I use multiple useState hooks in a component?Yes, you can use multiple useState hooks, but the order of calls must remain the same.
Why can't I use useState in loops or conditions?The order of hooks must not be changed, as React internally tracks them. Otherwise, unexpected behavior may occur.
Do I need to manually reset the state?Not necessarily. The state persists until the component is unmounted or you manually change it.
When should I use the setState functionality?If the new state depends on an old value, it's better to use the function via setState to ensure the latest state version is used.