Today you are taking the first step in creating your own to-do app with React. After the previous exercises, it is now time to apply the knowledge you have learned and create a practical project. In this tutorial, you will not only learn about the structure of the to-do app, but also about the components necessary to implement the functionality. So let's dive straight into it!
Key Insights
- You will learn how to create the basic structure of a to-do app.
- The app consists of two main components: ToDoInput for entering new to-dos and ToDoList for displaying the to-dos.
- React states are used to manage the list of to-dos.
Step-by-Step Guide
Step 1: Setting Up the Project
To get started, you will create a new array of components and remove all unnecessary parts from the app.jsx. So delete all previous implementations in these files.
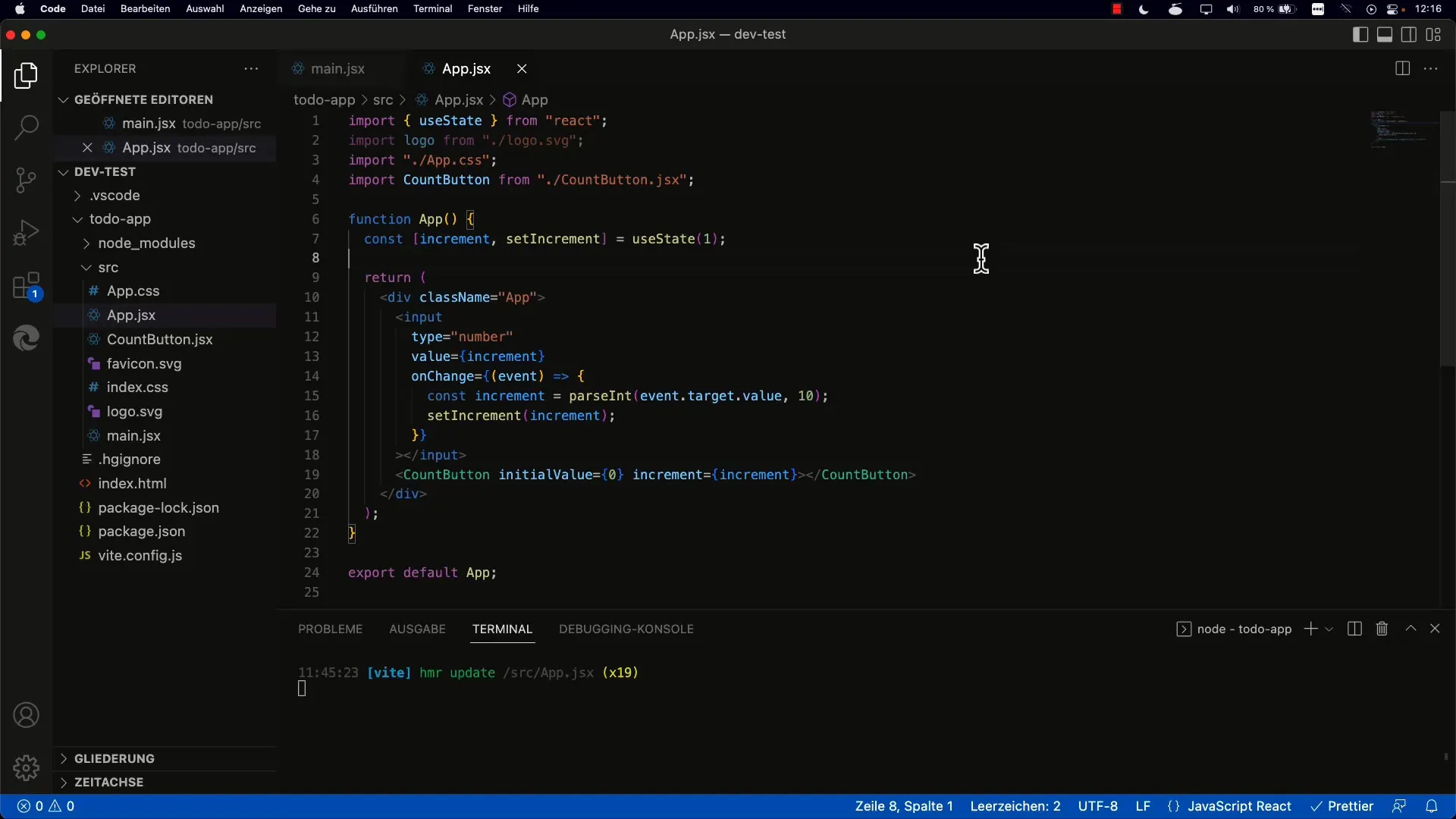
Step 2: Planning the Components
Plan the structure of the to-do app. Identify the two main components: ToDoInput, where new to-dos will be entered, and ToDoList, where the to-dos will be displayed.
Step 3: Creating Components
Create the ToDoInput component. You can start by building the basic structure of the input component. This should include an input field for the to-do text and a button to add it.
Step 4: Adding the ToDoList Component
Now it's time to create the second component: ToDoList. These components that need to be displayed should still remain empty as we will fill them with content later on.
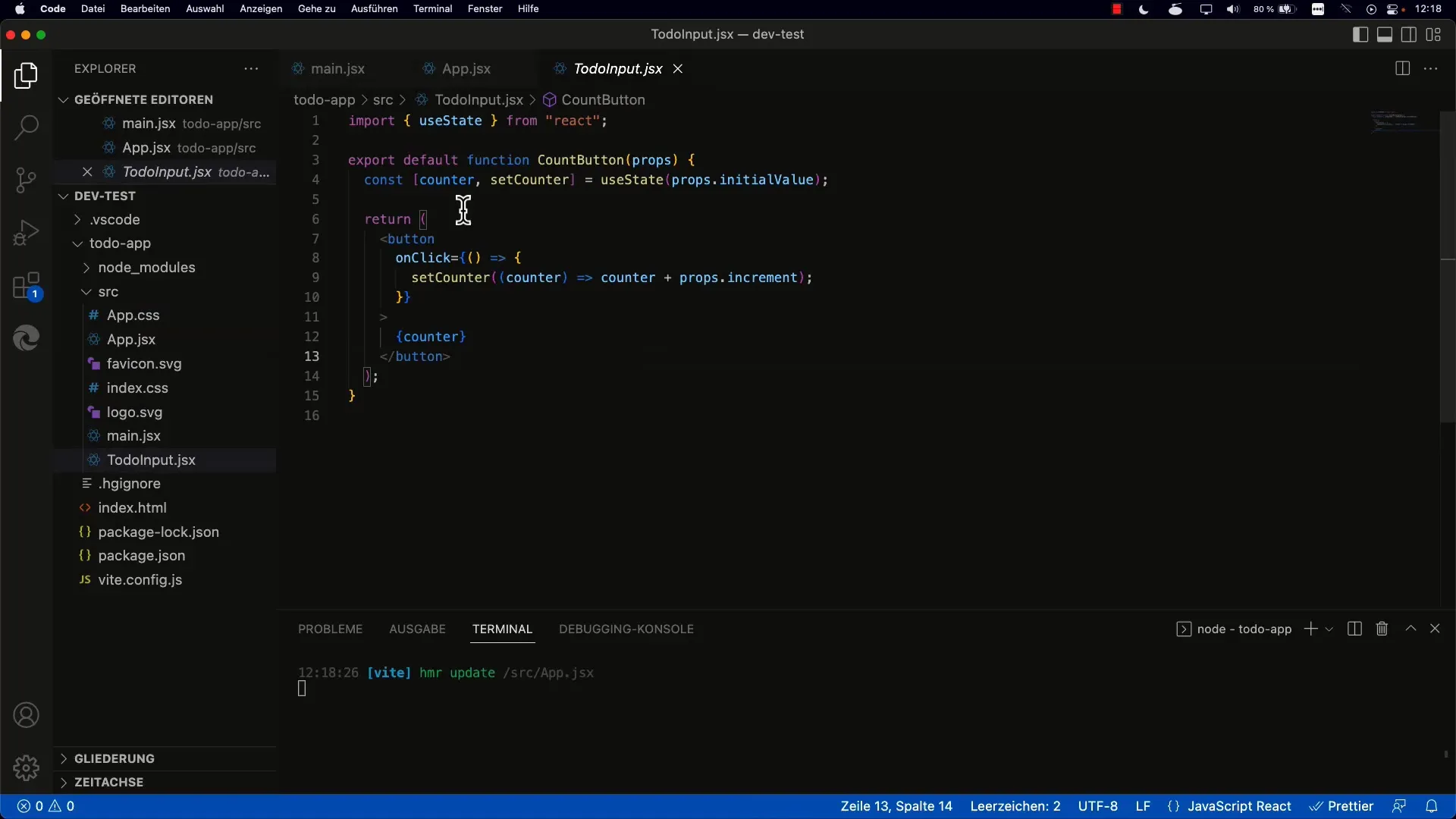
Step 5: Importing Components into app.jsx
In app.jsx, you will import the two new components. Make sure to use the correct names. Then add the two components to the render method.
Step 6: Styling Elements in ToDoInput
Style the ToDoInput by adding a element for the title "New To Do:" and an input field. Set the type of the input field to text to allow users to enter text.
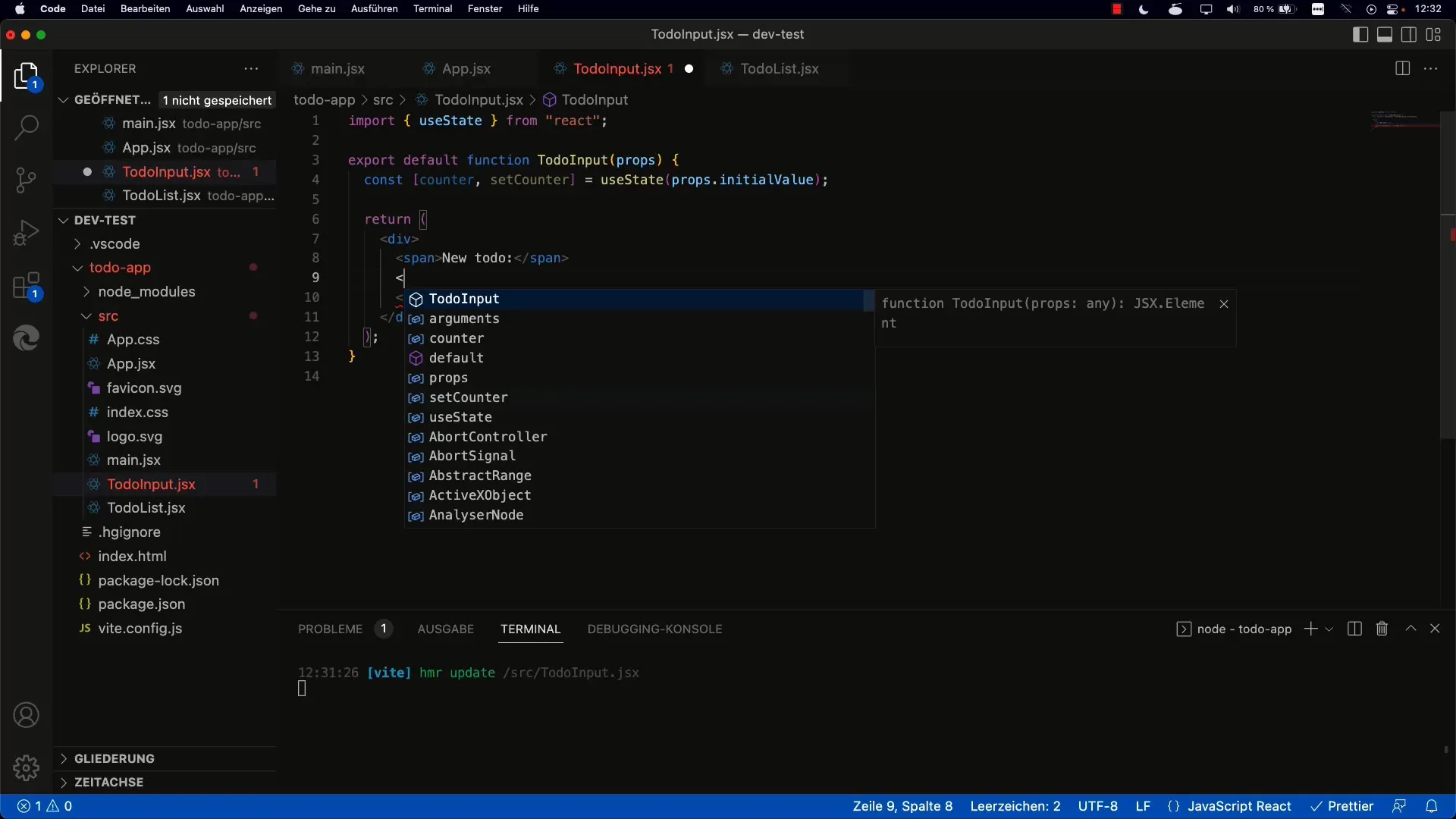
Step 7: Managing State
Create a state for the to-do text. Use useState to manage the input value and set the initial value to an empty string. Also, add an onChange event to update the state with each input.
Step 8: Preparing App Component for To-Do List
Think about how app.jsx can also store the list of to-dos. You will need to create a state for the to-do items to manage them later on.
Step 9: Initializing To-Dos
Initialize your to-do array in the App component with at least one to-do object containing the text and a status (e.g., false for incomplete).
Step 10: Populating ToDoList with Props
Pass the to-do array as props to the ToDoList component. Make sure to define the structure of the props correctly to ensure that ToDoList can access the array.
Step 11: Displaying To-dos
Use the map function to display the to-dos in the ToDoList. For each to-do element, create a
Step 12: Completion and Testing
Run your application to ensure that the basic structure functions correctly. Check if the input fields and the list are displayed correctly and if the data is being passed between components properly.
Summary
In this guide, you have created the basic structure of the to-do app in React. You have learned the importance of planning components and using state to manage the to-dos. Your app now has the ability to capture and display to-dos!
Frequently Asked Questions
How do I create new To-dos in the app?You still need to implement the feature for adding To-dos. Use the state to save the To-dos.
What should I do if the To-do list is not displayed?Check if you have correctly imported the ToDoList in app.jsx and filled it with the appropriate props.
Can I change the design of the To-do app?Yes, you can use CSS to customize the design of the app according to your preferences.